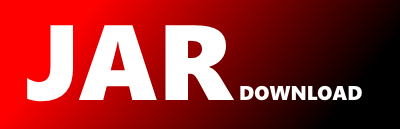
org.codehaus.mojo.weblogic.util.Bootstrapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of weblogic-maven-plugin Show documentation
Show all versions of weblogic-maven-plugin Show documentation
This plugin will support various tasks within the Weblogic 8.1,
9.x, 10.x and 12.x environment. This version starts to support weblogic 10 and
11. The mojo supports such tasks as deploy,
undeploy,clientgen,servicegen, and appc are supported as well as
many others. The plugin uses exposed API's that are subject to
change but have been tested in 8.1 SP 4-6 and 9.0 - 9.2 MP3, 10.x.
There are two versions of the plugin to support the two
environments based on differences in the JDK. The 9.x version is
currently being refactored to support the standard JSR supported
deployment interface. The code used in the plugin has been
contributed to the Cargo project however to date has not be
integrated into the codebase. Please feel free to suggest
improvements or help support this plugin effort.
The newest version!
package org.codehaus.mojo.weblogic.util;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.lang.reflect.Method;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.jar.Attributes;
import java.util.jar.JarOutputStream;
import java.util.jar.Manifest;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* This class does boot strapping by loading all of the
* resources under the passed in directories
*
* @author josborn
* @since Jan 21, 2010
*/
public class Bootstrapper
{
private static final Pattern WINDOWS_SPECIAL_CHAR =
Pattern.compile( "[\\ \\&\\(\\)\\[\\]\\{\\}\\^\\=\\;\\!\\'\\+\\,\\`\\~]" );
/**
* For debugging
*/
private static boolean DELETE_JAR = true;
/**
* Exec the external java process. we do some tricks here. Create a jar with a classpath and a main-class. invoke the
* java using 'java -jar mytempjar.jar aruments...' Since we access the jar, the classpath is extended with
* the appropriate stuff.
*
* @param classPath the classpath to put on the command line (via jar)
* @param args the arguments to pass to the execution target class
* @throws IOException the io exception
* @throws InterruptedException if the thread is interrupted
* @throws java.net.URISyntaxException the uri exception
*/
public static void exec( String classPath, String[] args )
throws IOException, InterruptedException, URISyntaxException
{
File classPathJar = null;
try
{
final Runtime rt = Runtime.getRuntime();
final String osName = System.getProperty( "os.name" );
List cmd = new ArrayList();
if ( osName.equals( "Windows NT" ) )
{
cmd.add( "cmd.exe" );
cmd.add( "/C" );
}
cmd.add( System.getProperty( "java.home" ) + "/bin/java" );
final String myClassPath =
Bootstrapper.class.getProtectionDomain().getCodeSource().getLocation().toURI().toString();
final StringBuilder sb = new StringBuilder( myClassPath ).append( ' ' ).append( classPath );
classPathJar = buildClasspathJar( sb.toString(), Bootstrapper.class.getName() );
cmd.add( "-jar" );
cmd.add( classPathJar.getPath() );
//now add the arguments
for ( String arg : args )
{
//handle special characters in command line args for quoting for windows
if ( "Windows NT".equals( osName ) )
{
final Matcher matcher = WINDOWS_SPECIAL_CHAR.matcher( arg );
if ( matcher.find() )
{
cmd.add( "\"" + arg + "\"" );
}
else
{
cmd.add( arg );
}
}
else
{
//does linux have special characters and quoting requirements?
cmd.add( arg );
}
}
System.out.println( cmd );
Process proc = rt.exec( cmd.toArray( new String[cmd.size()] ) );
// any error message?
final StreamGobbler errorGobbler = new StreamGobbler( proc.getErrorStream(), "[ERROR]" );
// any output?
final StreamGobbler outputGobbler = new StreamGobbler( proc.getInputStream(), "[INFO]" );
// kick them off
errorGobbler.start();
outputGobbler.start();
// any error???
int exitVal = proc.waitFor();
System.out.println( "result: " + exitVal );
if ( exitVal != 0 )
{
throw new RuntimeException( String.format( "Bootstrapper returned %d exit code!", exitVal ) );
}
}
finally
{
if ( classPathJar != null && DELETE_JAR )
{
classPathJar.delete();
}
}
}
/**
* This method builds a jar containing a manifest that will serve as our classpath hydration. This is
* because there is a 32K limit on the number of characters we can provide as command line arguments.
*
* @param classPath the classpath string
* @param mainClass the main class to boot from
* @return the file we created
* @throws IOException if the file cannot be created
*/
public static File buildClasspathJar( String classPath, String mainClass )
throws IOException
{
File file = null;
JarOutputStream jarOut = null;
try
{
Manifest manifest = new Manifest();
Attributes attributes = manifest.getMainAttributes();
attributes.putValue( "Create-Date", new Date().toString() );
attributes.put( Attributes.Name.CLASS_PATH, classPath );
//we require the version for the manifest to actually be written
attributes.put( Attributes.Name.MANIFEST_VERSION, "1.0" );
attributes.put( Attributes.Name.IMPLEMENTATION_TITLE, "JWSC Classpath Jar" );
attributes.put( Attributes.Name.MAIN_CLASS, mainClass );
file = File.createTempFile( "weblogicMojoBooter", ".jar" );
jarOut = new JarOutputStream( new FileOutputStream( file ), manifest );
jarOut.flush();
}
finally
{
if ( jarOut != null )
{
try
{
jarOut.close();
}
catch ( IOException e )
{
//eat
}
}
}
return file;
}
/**
* Arguments are the path separated list of folders to load
* the urls to the classloader. Each directory gets on the classpath
* along with all of the files for the classpath
*
* @param args arg0 is the list of classes
* @throws Exception
*/
public static void main( String[] args )
throws Exception
{
final String pathSeparator = System.getProperty( "path.separator" );
if ( pathSeparator == null )
{
throw new IllegalArgumentException( "System Property 'path.separator' was not supplied and is required." );
}
if ( args.length == 0 )
{
throw new IllegalArgumentException( "Must specify at least the class name to load from the classloader." );
}
final String executableClassName = args[0];
final Class mainClass = Thread.currentThread().getContextClassLoader().loadClass( executableClassName );
final Method main = mainClass.getMethod( "main", args.getClass() );
if ( main == null )
{
throw new IllegalArgumentException( "Must specific main method in class " + executableClassName );
}
// prune the first argument off
if ( args.length > 1 )
{
final String[] nextArgs = new String[args.length - 1];
System.arraycopy( args, 1, nextArgs, 0, nextArgs.length );
main.invoke( null, new Object[]{ nextArgs } );
}
else
{
main.invoke( null, new Object[0] );
}
}
/**
* Retrieve the file. If the descriptor is not a valid URI, assume it is a
* file descriptor
*
* @param descriptor from a uri or just a plain file
* @return the file
*/
private static File getFile( String descriptor )
{
File file;
try
{
file = new File( new URI( descriptor ) );
}
catch ( Exception e )
{
//oops, the descriptor was not a uri, assume it is just a file then
//or there was some other issue with the URI conversion so just treat as file
file = new File( descriptor );
}
return file;
}
static class StreamGobbler
extends Thread
{
private InputStream is;
String type;
StreamGobbler( InputStream is, String type )
{
this.is = is;
this.type = type;
this.setDaemon( true );
this.setName( type );
}
public void run()
{
try
{
InputStreamReader isr = new InputStreamReader( is );
BufferedReader br = new BufferedReader( isr );
String line;
while ( ( line = br.readLine() ) != null )
{
System.out.println( type + ">" + line );
}
}
catch ( IOException ioe )
{
ioe.printStackTrace();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy