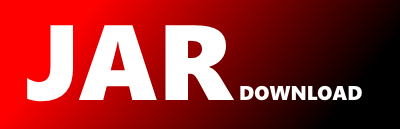
at.spardat.xma.boot.antext.AppDescriptor Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2003, 2007 s IT Solutions AT Spardat GmbH .
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* s IT Solutions AT Spardat GmbH - initial API and implementation
*******************************************************************************/
/*
*
* Created on : 23.06.2003
* Created by : s3595
*
* Maintainer Date Defect Modification
* ------------ ------ -------- ------------
* s1462 07-02-05 doesn't delete MD5 files; only change app descriptor, if any entry change.
*/
package at.spardat.xma.boot.antext;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.PrintStream;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import org.apache.tools.ant.BuildException;
import org.apache.tools.ant.Task;
import at.spardat.xma.boot.Statics;
import at.spardat.xma.boot.comp.AppLoader;
import at.spardat.xma.boot.comp.data.Version;
import at.spardat.xma.boot.comp.data.XMAApp;
import at.spardat.xma.boot.comp.data.XMAAppParser;
import at.spardat.xma.boot.comp.data.XMAPluginSpec;
import at.spardat.xma.boot.comp.data.XMAResource;
import at.spardat.xma.boot.logger.LogLevel;
import at.spardat.xma.boot.logger.Logger;
/**
* AppDescriptor
*
*
* this class is a custom ant task, that handles the application descriptor during the build
* of a project.
* The project?s build has to include this task by using the custom taskdef element: taskdef.
* The class finds the required webapplication and its descriptor by using the input parameters: dir and file
.
* The parameter file should point to the application descriptor that should be checked.
* The webapplication is searched under dir.
*
* @author s1462 Ferry Malzer (Maf)
* @version $Id: AppDescriptor.java 3096 2009-02-02 16:45:19Z gub $
*
*/
public class AppDescriptor extends Task {
/* file extension for md5 hash files */
public static String STR_HASHF_EXT = ".MD5";
/* the length of each hash value string */
public static int HASHLENGTH = 32;
/* working directory */
private File dir;
/* verbose output */
private boolean verbose;
/* relative path and filename from strdir to the appxml or plugins.xml */
private String strFile;
/* the xma file we are working on */
private File xmafile;
/* parsed application descriptor */
private XMAApp xmaapp;
/* fast development mode (omits component jar files) */
private boolean fdm=false;
/* constructor */
public AppDescriptor() {
strFile = ""; //$NON-NLS-1$
verbose = false;
xmaapp = null;
}
/**
* main excecution method
*/
public void execute() throws BuildException {
if(verbose == true) debugIn();
prepareInput();
checkVersions();
saveDescriptor();
if(verbose == true) log("Exit AppDescriptor::execute");
}
/**
* debug method used in verbose mode
*/
private void debugIn() {
log("AppDescriptor::execute");
log( "root dir: " + this.dir.toString() );
log( "verbose : " + this.verbose );
log( "file dir: " + strFile );
}
/**
* save the application descriptor file to disk
*/
public void saveDescriptor() {
if(verbose == true) log("saving descriptor");
File newfile = new File( dir, "temp.xml");
if( newfile.exists() ) {
if(verbose == true) log("cleaning old existing temp.xml file");
newfile.delete();
}
try {
newfile.createNewFile();
} catch (IOException e1) {
throw new BuildException( "error creating temp xml file: " + e1.getMessage());
}
PrintStream os = null;
try {
os = new PrintStream( new FileOutputStream( newfile ));
} catch (FileNotFoundException e) {
throw new BuildException( "error creating temp xml file: " + e.getMessage());
}
xmaapp.writeXML(os);
os.flush();
os.close();
xmafile.delete();
newfile.renameTo( xmafile );
if(verbose == true) log("descriptor saved");
}
/**
* check and replace version id?s for all resources
*/
public void checkVersions() {
HashMap resources;
if(fdm) { resources = xmaapp.getAllResources(fdm); }
else { resources = xmaapp.getAllResources(); } // keep compatible with older bootrt if fdm is not used
for( Iterator iter = resources.keySet().iterator(); iter.hasNext();) {
String key = (String)iter.next();
XMAResource res = (XMAResource)resources.get(key);
String href = res.getHref_();
File fHash = new File( dir, href + STR_HASHF_EXT );
BufferedReader br = null;
String strHash;
try {
br = new BufferedReader(new InputStreamReader(new FileInputStream(fHash)));
strHash = br.readLine();
} catch (FileNotFoundException e) {
throw new BuildException( "md5 file not found: " + fHash.toString() );
} catch (IOException e1) {
throw new BuildException( "IO Error reading md5 file: " + fHash.toString() );
} finally {
try {if (br != null) br.close();} catch (IOException ex) {}
}
if( strHash != null && strHash.length()==HASHLENGTH) {
if( strHash.equals( res.getVersion_().getVersion() ) ) {
if(verbose == true) log( "version is equal for resource: " + href + " version id: " + strHash );
} else {
res.setVersion_( new Version( strHash ) );
if(verbose == true) log( "replacing version for resource: " + href + " new version id: " + strHash );
}
} else {
throw new BuildException("no valid hashvalue found for resource: " + href + " in file " + fHash.toString());
}
} // checkVersions
}
/**
* check input variables, directories, load files
* and parse application descriptor
*/
public void prepareInput() {
if( !strFile.equals( Statics.APP_DISCRIPTOR) && !strFile.equals(Statics.PLUGIN_DISCRIPTOR)) {
throw new BuildException( "invalid filename: \"" + strFile + "\" for an application descriptor");
}
xmafile = new File( dir, strFile);
if( !xmafile.exists() ) {
throw new BuildException( "application descriptor file not found: " + xmafile.toString());
}
InputStream is = null;
XMAAppParser parser;
try {
is = new FileInputStream(xmafile);
Logger logger = Logger.getLogger("build");
logger.setLevel(LogLevel.SEVERE);
parser = new XMAAppParser(logger, true );
xmaapp = parser.parse( is );
AppLoader.checkResourceIntegrity( xmaapp );
ArrayList missing = AppLoader.checkPluginSpecImpl( xmaapp );
for (int i=0; i< missing.size(); i++) {
XMAPluginSpec spec = (XMAPluginSpec)missing.get(i);
if(verbose == true) log( "ATTENTION: plugin deliverable must implement unresolved external: " + spec.getRequires_() );
}
} catch (Exception e) {
e.printStackTrace();
try { if(is != null) is.close(); } catch (IOException e2) { }
throw new BuildException( "parse error on application descriptor: " + e.getMessage());
}
if(verbose == true) log( "application descriptor parsed successfully: " + xmafile.toString());
} // prepareInput
/* set dir property */
public void setDir( File in) {
this.dir = in;
}
/* set verbose property */
public void setVerbose( boolean in ) {
this.verbose = in;
}
/* set file property */
public void setFile( String in ) {
this.strFile = in;
}
/** set fast development mode (omits component jar files)*/
public void setFdm(boolean fdm) {
this.fdm = fdm;
}
/* (non-Javadoc)
* @see org.apache.tools.ant.Task#init()
*/
public void init() throws BuildException {
super.init();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy