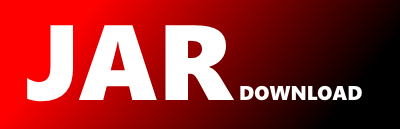
at.spardat.xma.help.HelpView Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2003, 2007 s IT Solutions AT Spardat GmbH .
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* s IT Solutions AT Spardat GmbH - initial API and implementation
*******************************************************************************/
/*
* Created on 24.06.2003
*
*
*
*/
package at.spardat.xma.help;
import java.util.ResourceBundle;
import org.eclipse.swt.SWT;
import org.eclipse.swt.SWTException;
import org.eclipse.swt.browser.Browser;
import org.eclipse.swt.browser.CloseWindowListener;
import org.eclipse.swt.browser.ProgressEvent;
import org.eclipse.swt.browser.ProgressListener;
import org.eclipse.swt.browser.StatusTextEvent;
import org.eclipse.swt.browser.StatusTextListener;
import org.eclipse.swt.browser.WindowEvent;
import org.eclipse.swt.events.DisposeEvent;
import org.eclipse.swt.events.DisposeListener;
import org.eclipse.swt.graphics.Image;
import org.eclipse.swt.graphics.ImageData;
import org.eclipse.swt.layout.GridData;
import org.eclipse.swt.layout.GridLayout;
import org.eclipse.swt.widgets.Composite;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Event;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Listener;
import org.eclipse.swt.widgets.ProgressBar;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.ToolBar;
import org.eclipse.swt.widgets.ToolItem;
/**
* Instances of this class display the Help Files of a XMA - Component
* using the SWT browser widget.
*
* @author S2267
*/
public class HelpView {
//The Display for the Component
private Display display;
//the SWT Shell
private Shell shell;
//The composite in which the browser widget is enbedded.
private Composite browserComposite;
//The SWT broswer control
private Browser swtBrowser;
// progress bar to display downloading progress information
private ProgressBar webProgress;
//a label for displaying status messages as they are received from the control
private Label webStatus;
//true if the doVerb command was successful.
private boolean activated = false;
static ResourceBundle resources = ResourceBundle.getBundle("at.spardat.xma.help.helpview");
// optional custom title
private String title;
// enable Toolbar or not
private boolean showButtons = true;
// default width
private int width = 800;
// default height
private int height = 500;
// shell style
private int style = SWT.SHELL_TRIM;
private ToolItem webCommandBackward;
private ToolItem webCommandForward;
private ToolItem webCommandStop;
//private ToolItem webCommandRefresh;
//private ToolItem webCommandSearch;
private ToolItem webCommandPrint;
static final String[] imageLocations = {
"icons/backward_nav.gif",
"icons/forward_nav.gif",
"icons/home_nav.gif",
"icons/stop_nav.gif",
"icons/refresh_nav.gif",
"icons/search_nav.gif",
"icons/webbrowser.gif",
"icons/print.gif" };
static Image images[];
static final int
biBack = 0,
biForward = 1,
biHome = 2,
biStop = 3,
biRefresh = 4,
biSearch = 5,
biWebbrowser = 6,
biPrint = 7;
/**
* Constructor of HelpView. Initializes the resources.
*/
public HelpView() {
initResources();
}
/**
* Creates a HelpView object with some customization. Initializes the resources.
* @param title showed in titlebar of the browser window. If null the default title "XMA Help" is shown".
* @param showButtons if true the tool bar is visible
* @param width of the browser window. SWT.DEFAULT means the default width.
* @param height of the browser window. SWT.DEFAULT means the default height.
*/
public HelpView(String title, boolean showButtons, int width, int height) {
this.title = title;
this.showButtons = showButtons;
if(width!=SWT.DEFAULT) this.width=width;
if(height!=SWT.DEFAULT) this.height=height;
initResources();
}
/**
* Creates a HelpView object with some customization. Initializes the resources.
* @param title showed in titlebar of the browser window. If null the default title "XMA Help" is shown".
* @param showButtons if true the tool bar is visible
* @param width of the browser window. SWT.DEFAULT means the default width.
* @param height of the browser window. SWT.DEFAULT means the default height.
* @param style SWT-Style of the shell containing the browser.
*/
public HelpView(String title, boolean showButtons, int width, int height,int style) {
this.title = title;
this.showButtons = showButtons;
if(width!=SWT.DEFAULT) this.width=width;
if(height!=SWT.DEFAULT) this.height=height;
this.style=style;
initResources();
}
/**
* Creates the SWT Shell and adds the DisposeListener.
*/
private void createShell (Display display_){
display = display_;
shell = new Shell (display,style);
if(title!=null) {
shell.setText(title);
} else {
shell.setText (resources.getString("Window.title"));
}
shell.setImage(images[biWebbrowser]);
GridLayout layout = new GridLayout();
layout.marginHeight=0;
layout.marginWidth=0;
layout.horizontalSpacing=3;
layout.verticalSpacing=3;
layout.numColumns = 3;
shell.setLayout(layout);
shell.addDisposeListener (new DisposeListener () {
public void widgetDisposed (DisposeEvent e) {
swtBrowser.dispose();
freeResources();
display.setData("help",null);
}
});
}
/**
* Creates the Web browser Component.
*/
private void createBrowserComp() {
browserComposite = new Composite(shell, SWT.NONE);
browserComposite.setLayout(new GridLayout());
GridData gridData = new GridData(GridData.FILL_HORIZONTAL | GridData.FILL_VERTICAL);
gridData.horizontalSpan = 3;
browserComposite.setLayoutData(gridData);
}
/**
* Creates the Web browser status area.
*/
private void createStatusArea() {
// Add a progress bar to display downloading progress information
webProgress = new ProgressBar(shell, SWT.BORDER);
GridData gridData = new GridData();
gridData.horizontalAlignment = GridData.BEGINNING;
gridData.verticalAlignment = GridData.FILL;
webProgress.setLayoutData(gridData);
// Add a label for displaying status messages as they are received from the control
webStatus = new Label(shell, SWT.SINGLE | SWT.READ_ONLY | SWT.BORDER);
gridData = new GridData(GridData.FILL_HORIZONTAL | GridData.VERTICAL_ALIGN_FILL);
gridData.horizontalSpan = 2;
webStatus.setLayoutData(gridData);
}
/**
* Activates and deactivates the buttons
* @param finished true if the webpage was fuly loaded, otherwise false.
* Determine by ProgressListener
* @since version_number
* @author S3460
*/
private void activateButtons(boolean finished){
if(showButtons){
webCommandBackward.setEnabled(swtBrowser.isBackEnabled());
webCommandForward.setEnabled(swtBrowser.isForwardEnabled());
webCommandStop.setEnabled(!finished);
webCommandPrint.setEnabled(finished);
}
}
/**
* Creates Web browser control.
*/
private void createSWTBrowser() {
try {
swtBrowser = new Browser(browserComposite,SWT.NONE);
GridData gridData = new GridData(GridData.FILL_HORIZONTAL | GridData.FILL_VERTICAL);
gridData.horizontalSpan = 3;
swtBrowser.setLayoutData(gridData);
activated = true;
} catch (SWTException ex) {
// Creation may have failed because control is not installed on machine
Label label = new Label(shell, SWT.BORDER);
label.setText(resources.getString("error.CouldNotCreateBrowserControl"));
return;
}
swtBrowser.addProgressListener(new ProgressListener() {
public void changed(ProgressEvent event) {
if (event.total == 0){
webProgress.setSelection(0);
activateButtons(true);
}else{
int ratio = event.current * 100 / event.total;
webProgress.setSelection(ratio);
activateButtons(false);
}
}
public void completed(ProgressEvent event) {
webProgress.setSelection(0);
activateButtons(true);
}
});
swtBrowser.addStatusTextListener(new StatusTextListener() {
public void changed(StatusTextEvent event) {
webStatus.setText(event.text);
}
});
swtBrowser.addCloseWindowListener(new CloseWindowListener(){
public void close(WindowEvent event) {
shell.close();
}});
}
/**
* Creates the Web browser toolbar.
*/
private void createToolbar() {
// Add a toolbar
ToolBar bar = new ToolBar(shell, SWT.NONE);
GridData gridData = new GridData(GridData.FILL_HORIZONTAL);
gridData.horizontalSpan = 3;
bar.setLayoutData(gridData);
// Add a button to navigate backwards through previously visited web sites
webCommandBackward = new ToolItem(bar, SWT.NONE);
webCommandBackward.setToolTipText(resources.getString("browser.Back.tooltip"));
//webCommandBackward.setText(resources.getString("browser.Back.text"));
webCommandBackward.setImage(images[biBack]);
webCommandBackward.setEnabled(false);
webCommandBackward.addListener(SWT.Selection, new Listener() {
public void handleEvent(Event e) {
// if (webBrowser == null) return;
// webBrowser.GoBack();
if (swtBrowser != null)
swtBrowser.back();
}
});
// Add a button to navigate forward through previously visited web sites
webCommandForward = new ToolItem(bar, SWT.NONE);
webCommandForward.setToolTipText(resources.getString("browser.Forward.tooltip"));
//webCommandForward.setText(resources.getString("browser.Forward.text"));
webCommandForward.setImage(images[biForward]);
webCommandForward.setEnabled(false);
webCommandForward.addListener(SWT.Selection, new Listener() {
public void handleEvent(Event e) {
// if (webBrowser == null) return;
// webBrowser.GoForward();
if (swtBrowser != null)
swtBrowser.forward();
}
});
// Add a separator
new ToolItem(bar, SWT.SEPARATOR);
// Add a button to abort web page loading
webCommandStop = new ToolItem(bar, SWT.NONE);
webCommandStop.setToolTipText(resources.getString("browser.Stop.tooltip"));
//webCommandStop.setText(resources.getString("browser.Stop.text"));
webCommandStop.setImage(images[biStop]);
webCommandStop.setEnabled(false);
webCommandStop.addListener(SWT.Selection, new Listener() {
public void handleEvent(Event e) {
if (swtBrowser != null)
swtBrowser.stop();
}
});
// Add a button to refresh the current web page
/*
webCommandRefresh = new ToolItem(bar, SWT.NONE);
webCommandRefresh.setToolTipText(resources.getString("browser.Refresh.tooltip"));
// webCommandRefresh.setText(resources.getString("browser.Refresh.text"));
webCommandRefresh.setImage(images[biRefresh]);
webCommandRefresh.setEnabled(false);
webCommandRefresh.addListener(SWT.Selection, new Listener() {
public void handleEvent(Event e) {
if (webBrowser == null) return;
webBrowser.Refresh();
}
});
*/
// Add a separator
new ToolItem(bar, SWT.SEPARATOR);
// Add a button to print the page
webCommandPrint = new ToolItem(bar, SWT.NONE);
webCommandPrint.setToolTipText(resources.getString("browser.Print.tooltip"));
//webCommandPrint.setText(resources.getString("browser.Print.text"));
webCommandPrint.setImage(images[biPrint]);
webCommandPrint.setEnabled(false);
webCommandPrint.addListener(SWT.Selection, new Listener() {
public void handleEvent(Event e) {
if (swtBrowser != null)
swtBrowser.execute("print()");
//swtBrowser.execute("window.print()");
//swtBrowser.execute("javascript:print()");
}
});
}
/**
* Frees the resources
*/
private void freeResources() {
if (images != null) {
for (int i = 0; i < images.length; ++i) {
final Image image = images[i];
if (image != null) image.dispose();
}
images = null;
}
}
/**
* Loads the resources.
*/
private void initResources() {
final Class clazz = HelpView.class;
if (images == null) {
images = new Image[imageLocations.length];
for (int i = 0; i < imageLocations.length; ++i) {
ImageData source = new ImageData(clazz.getResourceAsStream(
imageLocations[i]));
ImageData mask = source.getTransparencyMask();
images[i] = new Image(null, source, mask);
}
}
if (images == null ) {
freeResources();
throw new IllegalStateException();
}
}
/**
* Opens the browser to show the help page.
* @param display the SWT-Display to use.
* @return the shell containing the browser window.
*/
public Shell open (Display display) {
createShell (display);
if(showButtons) {
createToolbar();
}
createBrowserComp();
createStatusArea();
createSWTBrowser();
shell.setSize(width, height);
shell.open ();
return shell;
}
/**
* Navigates the browser to the given url.
* @param url the url to show in the browser.
*/
public void Navigate(String url) {
shell.setActive();
swtBrowser.setUrl(url);
}
/**
* Determine if the browser is activated.
* @return true if the brower is activated.
*/
public boolean isActivated() {
return activated;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy