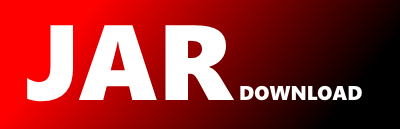
org.codehaus.plexus.redback.rbac.jdo.JdoUserAssignment Maven / Gradle / Ivy
/*
* $Id$
*/
package org.codehaus.plexus.redback.rbac.jdo;
//---------------------------------/
//- Imported classes and packages -/
//---------------------------------/
import java.util.Date;
/**
* binding of a principal to a role
*
* @version $Revision$ $Date$
*/
public class JdoUserAssignment extends org.codehaus.plexus.redback.rbac.AbstractUserAssignment
implements org.codehaus.plexus.redback.rbac.UserAssignment, java.io.Serializable
{
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
* Field principal
*/
private String principal;
/**
* Field timestamp
*/
private java.util.Date timestamp;
/**
* Field permanent
*/
private boolean permanent = false;
/**
* Field roleNames
*/
private java.util.List roleNames = new java.util.ArrayList();
//-----------/
//- Methods -/
//-----------/
/**
* Method equals
*
* @param other
*/
public boolean equals(Object other)
{
if ( this == other)
{
return true;
}
if ( !(other instanceof JdoUserAssignment) )
{
return false;
}
JdoUserAssignment that = (JdoUserAssignment) other;
boolean result = true;
result = result && ( getPrincipal() == null ? that.getPrincipal() == null : getPrincipal().equals( that.getPrincipal() ) );
return result;
} //-- boolean equals(Object)
/**
* Get null
*/
public String getPrincipal()
{
return this.principal;
} //-- String getPrincipal()
/**
* Method getRoleNames
*/
public java.util.List getRoleNames()
{
return this.roleNames;
} //-- java.util.List getRoleNames()
/**
* Get null
*/
public java.util.Date getTimestamp()
{
return this.timestamp;
} //-- java.util.Date getTimestamp()
/**
* Method hashCode
*/
public int hashCode()
{
int result = 17;
long tmp;
result = 37 * result + ( principal != null ? principal.hashCode() : 0 );
return result;
} //-- int hashCode()
/**
* Get
* true if this object is permanent.
*
*/
public boolean isPermanent()
{
return this.permanent;
} //-- boolean isPermanent()
/**
* Set
* true if this object is permanent.
*
*
* @param permanent
*/
public void setPermanent(boolean permanent)
{
this.permanent = permanent;
} //-- void setPermanent(boolean)
/**
* Set null
*
* @param principal
*/
public void setPrincipal(String principal)
{
this.principal = principal;
} //-- void setPrincipal(String)
/**
* Set null
*
* @param roleNames
*/
public void setRoleNames(java.util.List roleNames)
{
this.roleNames = roleNames;
} //-- void setRoleNames(java.util.List)
/**
* Set null
*
* @param timestamp
*/
public void setTimestamp(java.util.Date timestamp)
{
this.timestamp = timestamp;
} //-- void setTimestamp(java.util.Date)
/**
* Method toString
*/
public java.lang.String toString()
{
StringBuffer buf = new StringBuffer();
buf.append( "principal = '" );
buf.append( getPrincipal() + "'" );
return buf.toString();
} //-- java.lang.String toString()
private String modelEncoding = "UTF-8";
public void setModelEncoding( String modelEncoding )
{
this.modelEncoding = modelEncoding;
}
public String getModelEncoding()
{
return modelEncoding;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy