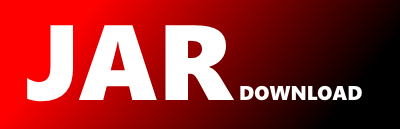
org.codehaus.plexus.redback.role.model.ModelApplication Maven / Gradle / Ivy
The newest version!
/*
* $Id$
*/
package org.codehaus.plexus.redback.role.model;
//---------------------------------/
//- Imported classes and packages -/
//---------------------------------/
import java.util.Date;
/**
*
* container for applications
*
*
* @version $Revision$ $Date$
*/
public class ModelApplication implements java.io.Serializable {
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
* Field version
*/
private String version;
/**
* Field id
*/
private String id;
/**
* Field description
*/
private String description;
/**
* Field longDescription
*/
private String longDescription;
/**
* Field resources
*/
private java.util.List resources;
/**
* Field operations
*/
private java.util.List operations;
/**
* Field roles
*/
private java.util.List roles;
/**
* Field templates
*/
private java.util.List templates;
//-----------/
//- Methods -/
//-----------/
/**
* Method addOperation
*
* @param modelOperation
*/
public void addOperation(ModelOperation modelOperation)
{
if ( !(modelOperation instanceof ModelOperation) )
{
throw new ClassCastException( "ModelApplication.addOperations(modelOperation) parameter must be instanceof " + ModelOperation.class.getName() );
}
getOperations().add( modelOperation );
} //-- void addOperation(ModelOperation)
/**
* Method addResource
*
* @param modelResource
*/
public void addResource(ModelResource modelResource)
{
if ( !(modelResource instanceof ModelResource) )
{
throw new ClassCastException( "ModelApplication.addResources(modelResource) parameter must be instanceof " + ModelResource.class.getName() );
}
getResources().add( modelResource );
} //-- void addResource(ModelResource)
/**
* Method addRole
*
* @param modelRole
*/
public void addRole(ModelRole modelRole)
{
if ( !(modelRole instanceof ModelRole) )
{
throw new ClassCastException( "ModelApplication.addRoles(modelRole) parameter must be instanceof " + ModelRole.class.getName() );
}
getRoles().add( modelRole );
} //-- void addRole(ModelRole)
/**
* Method addTemplate
*
* @param modelTemplate
*/
public void addTemplate(ModelTemplate modelTemplate)
{
if ( !(modelTemplate instanceof ModelTemplate) )
{
throw new ClassCastException( "ModelApplication.addTemplates(modelTemplate) parameter must be instanceof " + ModelTemplate.class.getName() );
}
getTemplates().add( modelTemplate );
} //-- void addTemplate(ModelTemplate)
/**
* Get null
*/
public String getDescription()
{
return this.description;
} //-- String getDescription()
/**
* Get null
*/
public String getId()
{
return this.id;
} //-- String getId()
/**
* Get null
*/
public String getLongDescription()
{
return this.longDescription;
} //-- String getLongDescription()
/**
* Method getOperations
*/
public java.util.List getOperations()
{
if ( this.operations == null )
{
this.operations = new java.util.ArrayList();
}
return this.operations;
} //-- java.util.List getOperations()
/**
* Method getResources
*/
public java.util.List getResources()
{
if ( this.resources == null )
{
this.resources = new java.util.ArrayList();
}
return this.resources;
} //-- java.util.List getResources()
/**
* Method getRoles
*/
public java.util.List getRoles()
{
if ( this.roles == null )
{
this.roles = new java.util.ArrayList();
}
return this.roles;
} //-- java.util.List getRoles()
/**
* Method getTemplates
*/
public java.util.List getTemplates()
{
if ( this.templates == null )
{
this.templates = new java.util.ArrayList();
}
return this.templates;
} //-- java.util.List getTemplates()
/**
* Get null
*/
public String getVersion()
{
return this.version;
} //-- String getVersion()
/**
* Method removeOperation
*
* @param modelOperation
*/
public void removeOperation(ModelOperation modelOperation)
{
if ( !(modelOperation instanceof ModelOperation) )
{
throw new ClassCastException( "ModelApplication.removeOperations(modelOperation) parameter must be instanceof " + ModelOperation.class.getName() );
}
getOperations().remove( modelOperation );
} //-- void removeOperation(ModelOperation)
/**
* Method removeResource
*
* @param modelResource
*/
public void removeResource(ModelResource modelResource)
{
if ( !(modelResource instanceof ModelResource) )
{
throw new ClassCastException( "ModelApplication.removeResources(modelResource) parameter must be instanceof " + ModelResource.class.getName() );
}
getResources().remove( modelResource );
} //-- void removeResource(ModelResource)
/**
* Method removeRole
*
* @param modelRole
*/
public void removeRole(ModelRole modelRole)
{
if ( !(modelRole instanceof ModelRole) )
{
throw new ClassCastException( "ModelApplication.removeRoles(modelRole) parameter must be instanceof " + ModelRole.class.getName() );
}
getRoles().remove( modelRole );
} //-- void removeRole(ModelRole)
/**
* Method removeTemplate
*
* @param modelTemplate
*/
public void removeTemplate(ModelTemplate modelTemplate)
{
if ( !(modelTemplate instanceof ModelTemplate) )
{
throw new ClassCastException( "ModelApplication.removeTemplates(modelTemplate) parameter must be instanceof " + ModelTemplate.class.getName() );
}
getTemplates().remove( modelTemplate );
} //-- void removeTemplate(ModelTemplate)
/**
* Set null
*
* @param description
*/
public void setDescription(String description)
{
this.description = description;
} //-- void setDescription(String)
/**
* Set null
*
* @param id
*/
public void setId(String id)
{
this.id = id;
} //-- void setId(String)
/**
* Set null
*
* @param longDescription
*/
public void setLongDescription(String longDescription)
{
this.longDescription = longDescription;
} //-- void setLongDescription(String)
/**
* Set null
*
* @param operations
*/
public void setOperations(java.util.List operations)
{
this.operations = operations;
} //-- void setOperations(java.util.List)
/**
* Set null
*
* @param resources
*/
public void setResources(java.util.List resources)
{
this.resources = resources;
} //-- void setResources(java.util.List)
/**
* Set null
*
* @param roles
*/
public void setRoles(java.util.List roles)
{
this.roles = roles;
} //-- void setRoles(java.util.List)
/**
* Set null
*
* @param templates
*/
public void setTemplates(java.util.List templates)
{
this.templates = templates;
} //-- void setTemplates(java.util.List)
/**
* Set null
*
* @param version
*/
public void setVersion(String version)
{
this.version = version;
} //-- void setVersion(String)
private String modelEncoding = "UTF-8";
public void setModelEncoding( String modelEncoding )
{
this.modelEncoding = modelEncoding;
}
public String getModelEncoding()
{
return modelEncoding;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy