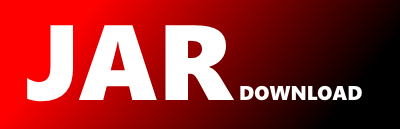
org.codehaus.plexus.redback.role.model.ModelRole Maven / Gradle / Ivy
The newest version!
/*
* $Id$
*/
package org.codehaus.plexus.redback.role.model;
//---------------------------------/
//- Imported classes and packages -/
//---------------------------------/
import java.util.Date;
/**
*
* model roles specify a role and its corresponding
* permissions,
* child roles and parent roles to link to
*
*
* @version $Revision$ $Date$
*/
public class ModelRole implements java.io.Serializable {
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
* Field id
*/
private String id;
/**
* Field name
*/
private String name;
/**
* Field description
*/
private String description;
/**
* Field assignable
*/
private boolean assignable = false;
/**
* Field permanent
*/
private boolean permanent = false;
/**
* Field permissions
*/
private java.util.List permissions;
/**
* Field parentRoles
*/
private java.util.List parentRoles;
/**
* Field childRoles
*/
private java.util.List childRoles;
//-----------/
//- Methods -/
//-----------/
/**
* Method addChildRole
*
* @param string
*/
public void addChildRole(String string)
{
if ( !(string instanceof String) )
{
throw new ClassCastException( "ModelRole.addChildRoles(string) parameter must be instanceof " + String.class.getName() );
}
getChildRoles().add( string );
} //-- void addChildRole(String)
/**
* Method addParentRole
*
* @param string
*/
public void addParentRole(String string)
{
if ( !(string instanceof String) )
{
throw new ClassCastException( "ModelRole.addParentRoles(string) parameter must be instanceof " + String.class.getName() );
}
getParentRoles().add( string );
} //-- void addParentRole(String)
/**
* Method addPermission
*
* @param modelPermission
*/
public void addPermission(ModelPermission modelPermission)
{
if ( !(modelPermission instanceof ModelPermission) )
{
throw new ClassCastException( "ModelRole.addPermissions(modelPermission) parameter must be instanceof " + ModelPermission.class.getName() );
}
getPermissions().add( modelPermission );
} //-- void addPermission(ModelPermission)
/**
* Method equals
*
* @param other
*/
public boolean equals(Object other)
{
if ( this == other)
{
return true;
}
if ( !(other instanceof ModelRole) )
{
return false;
}
ModelRole that = (ModelRole) other;
boolean result = true;
result = result && ( getId() == null ? that.getId() == null : getId().equals( that.getId() ) );
return result;
} //-- boolean equals(Object)
/**
* Method getChildRoles
*/
public java.util.List getChildRoles()
{
if ( this.childRoles == null )
{
this.childRoles = new java.util.ArrayList();
}
return this.childRoles;
} //-- java.util.List getChildRoles()
/**
* Get description of this role
*/
public String getDescription()
{
return this.description;
} //-- String getDescription()
/**
* Get null
*/
public String getId()
{
return this.id;
} //-- String getId()
/**
* Get
* either a normal name, or 'name - ${resource}'
*
*/
public String getName()
{
return this.name;
} //-- String getName()
/**
* Method getParentRoles
*/
public java.util.List getParentRoles()
{
if ( this.parentRoles == null )
{
this.parentRoles = new java.util.ArrayList();
}
return this.parentRoles;
} //-- java.util.List getParentRoles()
/**
* Method getPermissions
*/
public java.util.List getPermissions()
{
if ( this.permissions == null )
{
this.permissions = new java.util.ArrayList();
}
return this.permissions;
} //-- java.util.List getPermissions()
/**
* Method hashCode
*/
public int hashCode()
{
int result = 17;
long tmp;
result = 37 * result + ( id != null ? id.hashCode() : 0 );
return result;
} //-- int hashCode()
/**
* Get
* boolean specifying if a given role can assigned
*
*/
public boolean isAssignable()
{
return this.assignable;
} //-- boolean isAssignable()
/**
* Get
* boolean specifying if a given role can be
* removed once
* created
*
*/
public boolean isPermanent()
{
return this.permanent;
} //-- boolean isPermanent()
/**
* Method removeChildRole
*
* @param string
*/
public void removeChildRole(String string)
{
if ( !(string instanceof String) )
{
throw new ClassCastException( "ModelRole.removeChildRoles(string) parameter must be instanceof " + String.class.getName() );
}
getChildRoles().remove( string );
} //-- void removeChildRole(String)
/**
* Method removeParentRole
*
* @param string
*/
public void removeParentRole(String string)
{
if ( !(string instanceof String) )
{
throw new ClassCastException( "ModelRole.removeParentRoles(string) parameter must be instanceof " + String.class.getName() );
}
getParentRoles().remove( string );
} //-- void removeParentRole(String)
/**
* Method removePermission
*
* @param modelPermission
*/
public void removePermission(ModelPermission modelPermission)
{
if ( !(modelPermission instanceof ModelPermission) )
{
throw new ClassCastException( "ModelRole.removePermissions(modelPermission) parameter must be instanceof " + ModelPermission.class.getName() );
}
getPermissions().remove( modelPermission );
} //-- void removePermission(ModelPermission)
/**
* Set
* boolean specifying if a given role can assigned
*
*
* @param assignable
*/
public void setAssignable(boolean assignable)
{
this.assignable = assignable;
} //-- void setAssignable(boolean)
/**
* Set
* the id of other role profiles to assign as
* children to this
* role, can accept 'foo - ${resource}'
*
*
* @param childRoles
*/
public void setChildRoles(java.util.List childRoles)
{
this.childRoles = childRoles;
} //-- void setChildRoles(java.util.List)
/**
* Set description of this role
*
* @param description
*/
public void setDescription(String description)
{
this.description = description;
} //-- void setDescription(String)
/**
* Set null
*
* @param id
*/
public void setId(String id)
{
this.id = id;
} //-- void setId(String)
/**
* Set
* either a normal name, or 'name - ${resource}'
*
*
* @param name
*/
public void setName(String name)
{
this.name = name;
} //-- void setName(String)
/**
* Set
* the id of other role profiles to have create a
* child
* relationship to this role, can accept 'foo -
* ${resource}'
*
*
* @param parentRoles
*/
public void setParentRoles(java.util.List parentRoles)
{
this.parentRoles = parentRoles;
} //-- void setParentRoles(java.util.List)
/**
* Set
* boolean specifying if a given role can be
* removed once
* created
*
*
* @param permanent
*/
public void setPermanent(boolean permanent)
{
this.permanent = permanent;
} //-- void setPermanent(boolean)
/**
* Set null
*
* @param permissions
*/
public void setPermissions(java.util.List permissions)
{
this.permissions = permissions;
} //-- void setPermissions(java.util.List)
/**
* Method toString
*/
public java.lang.String toString()
{
StringBuffer buf = new StringBuffer();
buf.append( "id = '" );
buf.append( getId() + "'" );
return buf.toString();
} //-- java.lang.String toString()
private String modelEncoding = "UTF-8";
public void setModelEncoding( String modelEncoding )
{
this.modelEncoding = modelEncoding;
}
public String getModelEncoding()
{
return modelEncoding;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy