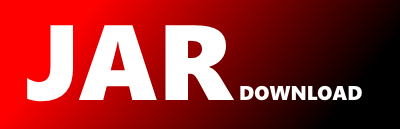
org.codehaus.plexus.redback.role.util.RoleModelUtils Maven / Gradle / Ivy
The newest version!
package org.codehaus.plexus.redback.role.util;
/*
* Copyright 2005 The Codehaus.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
import org.codehaus.plexus.redback.role.model.ModelApplication;
import org.codehaus.plexus.redback.role.model.ModelOperation;
import org.codehaus.plexus.redback.role.model.ModelResource;
import org.codehaus.plexus.redback.role.model.ModelRole;
import org.codehaus.plexus.redback.role.model.ModelTemplate;
import org.codehaus.plexus.redback.role.model.RedbackRoleModel;
import org.codehaus.plexus.util.dag.CycleDetectedException;
import org.codehaus.plexus.util.dag.DAG;
import org.codehaus.plexus.util.dag.TopologicalSorter;
/**
* RoleModelUtils:
*
* @author: Jesse McConnell
* @version: $Id: RoleModelUtils.java 769 2009-03-10 17:07:53Z brett $
*/
public class RoleModelUtils
{
@SuppressWarnings("unchecked")
public static List getRoles( RedbackRoleModel model )
{
List roleList = new ArrayList();
for ( ModelApplication application : (List) model.getApplications() )
{
roleList.addAll( application.getRoles() );
}
return roleList;
}
@SuppressWarnings("unchecked")
public static List getTemplates( RedbackRoleModel model )
{
List templateList = new ArrayList();
for ( ModelApplication application : (List) model.getApplications() )
{
templateList.addAll( application.getTemplates() );
}
return templateList;
}
@SuppressWarnings("unchecked")
public static List getOperationIdList( RedbackRoleModel model )
{
List operationsIdList = new ArrayList();
for ( ModelApplication application : (List) model.getApplications() )
{
for ( ModelOperation operation : (List) application.getOperations() )
{
operationsIdList.add( operation.getId() );
}
}
return operationsIdList;
}
@SuppressWarnings("unchecked")
public static List getResourceIdList( RedbackRoleModel model )
{
List resourceIdList = new ArrayList();
for ( ModelApplication application : (List) model.getApplications() )
{
for ( ModelResource resource : (List) application.getResources() )
{
resourceIdList.add( resource.getId() );
}
}
return resourceIdList;
}
@SuppressWarnings("unchecked")
public static List getRoleIdList( RedbackRoleModel model )
{
List roleIdList = new ArrayList();
for ( ModelApplication application : (List) model.getApplications() )
{
for ( ModelRole role : (List) application.getRoles() )
{
roleIdList.add( role.getId() );
}
}
return roleIdList;
}
@SuppressWarnings("unchecked")
public static List getTemplateIdList( RedbackRoleModel model )
{
List templateIdList = new ArrayList();
for ( ModelApplication application : (List) model.getApplications() )
{
for ( ModelTemplate template : (List) application.getTemplates() )
{
templateIdList.add( template.getId() );
}
}
return templateIdList;
}
/**
* WARNING: can return null
*
* @param model
* @param roleId
* @return
*/
@SuppressWarnings("unchecked")
public static ModelRole getModelRole( RedbackRoleModel model, String roleId )
{
ModelRole mrole = null;
for ( ModelApplication application : (List) model.getApplications() )
{
for ( ModelRole role : (List) application.getRoles() )
{
if ( roleId.equals( role.getId() ) )
{
mrole = role;
}
}
}
return mrole;
}
/**
* WARNING: can return null
*
* @param model
* @param templateId
* @return
*/
@SuppressWarnings("unchecked")
public static ModelTemplate getModelTemplate( RedbackRoleModel model, String templateId )
{
ModelTemplate mtemplate = null;
for ( ModelApplication application : (List) model.getApplications() )
{
for ( ModelTemplate template : (List) application.getTemplates() )
{
if ( templateId.equals( template.getId() ) )
{
mtemplate = template;
}
}
}
return mtemplate;
}
/**
* WARNING: can return null
*
* @param model
* @param operationId
* @return
*/
@SuppressWarnings("unchecked")
public static ModelOperation getModelOperation( RedbackRoleModel model, String operationId )
{
ModelOperation moperation = null;
for ( ModelApplication application : (List) model.getApplications() )
{
for ( ModelOperation operation : (List) application.getOperations() )
{
if ( operationId.equals( operation.getId() ) )
{
moperation = operation;
}
}
}
return moperation;
}
@SuppressWarnings( "unchecked" )
public static ModelResource getModelResource( RedbackRoleModel model, String resourceId )
{
ModelResource mresource = null;
for ( ModelApplication application : (List) model.getApplications() )
{
for ( ModelResource resource : (List) application.getResources() )
{
if ( resourceId.equals( resource.getId() ) )
{
mresource = resource;
}
}
}
return mresource;
}
@SuppressWarnings( "unchecked" )
public static DAG generateRoleGraph( RedbackRoleModel model )
throws CycleDetectedException
{
DAG roleGraph = new DAG();
for ( ModelApplication application : (List) model.getApplications() )
{
for ( ModelRole role : (List) application.getRoles() )
{
roleGraph.addVertex( role.getId() );
if ( role.getChildRoles() != null )
{
for ( String childRole : (List) role.getChildRoles() )
{
roleGraph.addVertex( childRole );
roleGraph.addEdge( role.getId(), childRole );
}
}
if ( role.getParentRoles() != null )
{
for ( String parentRole : (List) role.getParentRoles() )
{
roleGraph.addVertex( parentRole );
roleGraph.addEdge( parentRole, role.getId() );
}
}
}
}
return roleGraph;
}
@SuppressWarnings( "unchecked" )
public static DAG generateTemplateGraph( RedbackRoleModel model )
throws CycleDetectedException
{
DAG templateGraph = generateRoleGraph( model );
for ( ModelApplication application : (List) model.getApplications() )
{
for ( ModelTemplate template : (List) application.getTemplates() )
{
templateGraph.addVertex( template.getId() );
if ( template.getChildRoles() != null )
{
for ( String childRole : (List) template.getChildRoles() )
{
templateGraph.addVertex( childRole );
templateGraph.addEdge( template.getId(), childRole );
}
}
if ( template.getParentRoles() != null )
{
for ( String parentRole : (List) template.getParentRoles() )
{
templateGraph.addVertex( parentRole );
templateGraph.addEdge( parentRole, template.getId() );
}
}
if ( template.getChildTemplates() != null )
{
for ( String childTemplate : (List) template.getChildTemplates() )
{
templateGraph.addVertex( childTemplate );
templateGraph.addEdge( template.getId(), childTemplate );
}
}
if ( template.getParentTemplates() != null )
{
for ( String parentTemplate : (List) template.getParentTemplates() )
{
templateGraph.addVertex( parentTemplate );
templateGraph.addEdge( parentTemplate, template.getId() );
}
}
}
}
return templateGraph;
}
@SuppressWarnings( "unchecked" )
public static List reverseTopologicalSortedRoleList( RedbackRoleModel model )
throws CycleDetectedException
{
LinkedList sortedGraph =
(LinkedList) TopologicalSorter.sort( RoleModelUtils.generateRoleGraph( model ) );
List resortedGraph = new LinkedList();
while ( !sortedGraph.isEmpty() )
{
resortedGraph.add( sortedGraph.removeLast() );
}
return resortedGraph;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy