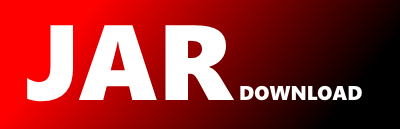
org.sonar.ide.idea.editor.ShowViolationsTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of idea-sonar-plugin Show documentation
Show all versions of idea-sonar-plugin Show documentation
An IntelliJ IDEA Plugin for Sonar.
The newest version!
/*
* Copyright (C) 2010 Evgeny Mandrikov
*
* Sonar-IDE is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* Sonar-IDE is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Sonar-IDE; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02
*/
package org.sonar.ide.idea.editor;
import com.intellij.openapi.editor.Document;
import com.intellij.openapi.editor.markup.HighlighterLayer;
import com.intellij.openapi.editor.markup.MarkupModel;
import com.intellij.openapi.editor.markup.RangeHighlighter;
import com.intellij.openapi.progress.ProgressIndicator;
import com.intellij.openapi.progress.Task;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.util.Key;
import com.intellij.util.ui.UIUtil;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import org.sonar.ide.idea.utils.SonarUtils;
import org.sonar.ide.shared.ViolationUtils;
import org.sonar.ide.shared.ViolationsLoader;
import org.sonar.wsclient.Sonar;
import org.sonar.wsclient.services.Violation;
import java.util.Collection;
import java.util.List;
import java.util.Map;
/**
* Task for loading violations.
*
* @author Evgeny Mandrikov
*/
public class ShowViolationsTask extends Task.Backgroundable {
private static final Key SONAR_DATA_KEY = Key.create("SONAR_DATA_KEY");
private Document document;
private String resourceKey;
public ShowViolationsTask(@Nullable Project project, Document document, String resourceKey) {
super(project, "Loading violations from Sonar for " + resourceKey);
this.document = document;
this.resourceKey = resourceKey;
}
@Override
public void run(@NotNull ProgressIndicator progressIndicator) {
try {
Project project = getProject();
final Sonar sonar = SonarUtils.getSonar(project);
// Violations
String text = document.getText();
final Collection violations = ViolationsLoader.getViolations(sonar, resourceKey, text);
final Map> violationsByLine = ViolationUtils.splitByLines(violations);
final MarkupModel markupModel = document.getMarkupModel(project);
UIUtil.invokeLaterIfNeeded(new Runnable() {
@Override
public void run() {
removeSonarHighlighters(document.getMarkupModel(getProject()));
for (Map.Entry> entry : violationsByLine.entrySet()) {
addHighlighter(markupModel, entry.getKey() - 1, entry.getValue());
}
}
});
} catch (Exception e) {
// Ignore, because notification about exception is very annoying.
e.printStackTrace();
}
}
/*
protected static void addCoverageHighlighter(MarkupModel markupModel, int line, String value) {
RangeHighlighter highlighter = addSonarHighlighter(markupModel, line, HighlighterLayer.ERROR + 1);
final int intValue = Integer.parseInt(value);
highlighter.setLineMarkerRenderer(new LineMarkerRenderer() {
@Override
public void paint(Editor editor, Graphics graphics, Rectangle rectangle) {
graphics.setColor(intValue == 0 ? Color.RED : Color.GREEN);
graphics.fillRect(rectangle.x, rectangle.y, 10, 10);
}
});
}
*/
protected static RangeHighlighter addSonarHighlighter(MarkupModel markupModel, int line, int layer) {
RangeHighlighter highlighter = markupModel.addLineHighlighter(line, layer, null);
// RangeHighlighter highlighter = markupModel.addRangeHighlighter(startOffset, endOffset);
highlighter.putUserData(SONAR_DATA_KEY, true);
highlighter.setGreedyToRight(true);
highlighter.setGreedyToLeft(true);
return highlighter;
}
/**
* Adds highlighter for specified violations.
*
* @param markupModel markup model
* @param line number of line
* @param violations violations
*/
protected static void addHighlighter(MarkupModel markupModel, int line, List violations) {
RangeHighlighter highlighter = addSonarHighlighter(markupModel, line, HighlighterLayer.ERROR + 1);
ViolationGutterIconRenderer renderer = new ViolationGutterIconRenderer(violations);
highlighter.setGutterIconRenderer(renderer);
highlighter.setErrorStripeMarkColor(renderer.getErrorStripeMarkColor());
highlighter.setErrorStripeTooltip(renderer.getTooltipText());
}
/**
* Removes all Sonar highlighters. Should be called before adding new markers to avoid duplicate markers.
*
* @param markupModel markup model
*/
protected static void removeSonarHighlighters(MarkupModel markupModel) {
for (RangeHighlighter rangeHighlighter : markupModel.getAllHighlighters()) {
Boolean marker = rangeHighlighter.getUserData(ShowViolationsTask.SONAR_DATA_KEY);
if (marker != null) {
markupModel.removeHighlighter(rangeHighlighter);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy