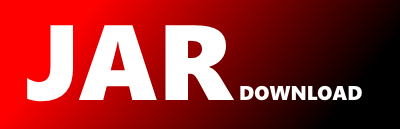
org.sonar.css.parser.TreeFactory Maven / Gradle / Ivy
/*
* SonarQube CSS / SCSS / Less Analyzer
* Copyright (C) 2013-2016 Tamas Kende and David RACODON
* mailto: [email protected] and [email protected]
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, write to the Free Software Foundation,
* Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package org.sonar.css.parser;
import com.google.common.collect.Lists;
import com.sonar.sslr.api.typed.Optional;
import org.sonar.css.tree.impl.SeparatedList;
import org.sonar.css.tree.impl.TreeImpl;
import org.sonar.css.tree.impl.css.*;
import org.sonar.css.tree.impl.embedded.CssInStyleTagTreeImpl;
import org.sonar.css.tree.impl.embedded.FileWithEmbeddedCssTreeImpl;
import org.sonar.css.tree.impl.less.*;
import org.sonar.css.tree.impl.scss.*;
import org.sonar.plugins.css.api.tree.Tree;
import org.sonar.plugins.css.api.tree.css.*;
import org.sonar.plugins.css.api.tree.embedded.CssInStyleTagTree;
import org.sonar.plugins.css.api.tree.embedded.FileWithEmbeddedCssTree;
import org.sonar.plugins.css.api.tree.less.*;
import org.sonar.plugins.css.api.tree.scss.*;
import java.util.List;
public class TreeFactory {
public StyleSheetTree stylesheet(Optional byteOrderMark, Optional> statements, SyntaxToken eof) {
return new StyleSheetTreeImpl(byteOrderMark.orNull(), statements.orNull(), eof);
}
public AtRuleTree atRule(AtKeywordTree atKeyword, Optional prelude, Optional block, Optional semicolon) {
return new AtRuleTreeImpl(atKeyword, prelude.orNull(), block.orNull(), semicolon.orNull());
}
public RulesetTree ruleset(Optional spacing, Optional selectors, StatementBlockTree block) {
return new RulesetTreeImpl(selectors.orNull(), block);
}
public StatementBlockTree atRuleBlock(SyntaxToken openCurlyBrace, Optional> content, SyntaxToken closeCurlyBrace) {
return new StatementBlockTreeImpl(openCurlyBrace, content.orNull(), closeCurlyBrace);
}
public StatementBlockTree rulesetBlock(SyntaxToken openCurlyBrace, Optional> content, SyntaxToken closeCurlyBrace) {
return new StatementBlockTreeImpl(openCurlyBrace, content.orNull(), closeCurlyBrace);
}
public ParenthesisBlockTree parenthesisBlock(SyntaxToken openParenthesis, Optional> content, SyntaxToken closeParenthesis) {
return new ParenthesisBlockTreeImpl(openParenthesis, content.orNull(), closeParenthesis);
}
public BracketBlockTree bracketBlock(SyntaxToken openBracket, Optional> content, SyntaxToken closeBracket) {
return new BracketBlockTreeImpl(openBracket, content.orNull(), closeBracket);
}
public UriTree uri(SyntaxToken urlFunction, SyntaxToken openParenthesis, Optional uriContent, SyntaxToken closeParenthesis) {
return new UriTreeImpl(urlFunction, openParenthesis, uriContent.orNull(), closeParenthesis);
}
public UriContentTree uriContent(SyntaxToken ident) {
return new UriContentTreeImpl(ident);
}
public UriContentTree uriContent(StringTree string) {
return new UriContentTreeImpl(string);
}
public PropertyDeclarationTree propertyDeclaration(PropertyTree property, SyntaxToken colon, ValueTree value, Optional semicolon) {
return new PropertyDeclarationTreeImpl(property, colon, value, semicolon.orNull());
}
public VariableDeclarationTree variableDeclaration(VariableTree variable, SyntaxToken colon, ValueTree value, Optional semicolon) {
return new VariableDeclarationTreeImpl(variable, colon, value, semicolon.orNull());
}
public EmptyStatementTree emptyStatement(SyntaxToken semicolon) {
return new EmptyStatementTreeImpl(semicolon);
}
public PropertyTree property(IdentifierTree property) {
return new PropertyTreeImpl(property, null);
}
public ValueTree value(List valueElements) {
return new ValueTreeImpl(valueElements);
}
public ValueTree declarationValue(List valueElements) {
return new ValueTreeImpl(valueElements);
}
public ValueTree simpleValueWithoutCommaDeclarationList(List valueElements) {
return new ValueTreeImpl(valueElements);
}
public ValueTree simpleValue(List valueElements) {
return new ValueTreeImpl(valueElements);
}
public FunctionTree function(IdentifierTree functionName, ParametersTree parameters) {
return new FunctionTreeImpl(functionName, parameters);
}
public ParametersTree parameters(SyntaxToken openParenthesis, Optional> parameters, SyntaxToken closeParenthesis) {
return new ParametersTreeImpl(openParenthesis, parameters.orNull(), closeParenthesis);
}
public SeparatedList parameterList(ValueTree parameter, Optional>> subsequentParameters) {
List parameters = Lists.newArrayList(parameter);
List separators = Lists.newArrayList();
if (subsequentParameters.isPresent()) {
for (Tuple t : subsequentParameters.get()) {
separators.add(t.first());
parameters.add(t.second());
}
}
return new SeparatedList<>(parameters, separators);
}
public SelectorsTree selectors(SeparatedList selectors) {
return new SelectorsTreeImpl(selectors);
}
public SeparatedList selectorList(SelectorTree selector, Optional>> subsequentSelectors, Optional trailingComma) {
List selectors = Lists.newArrayList(selector);
List separators = Lists.newArrayList();
if (subsequentSelectors.isPresent()) {
for (Tuple t : subsequentSelectors.get()) {
separators.add(t.first());
selectors.add(t.second());
}
}
if (trailingComma.isPresent()) {
separators.add(trailingComma.get());
}
return new SeparatedList<>(selectors, separators);
}
public SelectorTree selector(SeparatedList compoundSelectors) {
return new SelectorTreeImpl(compoundSelectors);
}
public SeparatedList selectorCombinationList(CompoundSelectorTree selector, Optional>> subsequentSelectors) {
List compoundSelectors = Lists.newArrayList(selector);
List selectorCombinators = Lists.newArrayList();
if (subsequentSelectors.isPresent()) {
for (Tuple t : subsequentSelectors.get()) {
selectorCombinators.add(t.first());
compoundSelectors.add(t.second());
}
}
return new SeparatedList<>(compoundSelectors, selectorCombinators);
}
public SelectorCombinatorTree selectorCombinator(SyntaxToken combinator) {
return new SelectorCombinatorTreeImpl(combinator);
}
public CompoundSelectorTree compoundSelector(List selectors) {
return new CompoundSelectorTreeImpl(selectors);
}
public KeyframesSelectorTree keyframesSelector(Tree selector) {
return new KeyframesSelectorTreeImpl(selector);
}
public TypeSelectorTree typeSelector(Optional namespace, IdentifierTree identifier) {
return new TypeSelectorTreeImpl(namespace.orNull(), identifier);
}
public ClassSelectorTree classSelector(SyntaxToken dot, IdentifierTree className) {
return new ClassSelectorTreeImpl(dot, className);
}
public IdSelectorTree idSelector(SyntaxToken hash, IdentifierTree identifier) {
return new IdSelectorTreeImpl(hash, identifier);
}
public PseudoSelectorTree pseudoSelector(Optional spacing, PseudoComponentTree pseudo) {
return new PseudoSelectorTreeImpl(pseudo);
}
public PseudoSelectorTree pseudoSelector(PseudoComponentTree pseudo) {
return new PseudoSelectorTreeImpl(pseudo);
}
public PseudoFunctionTree pseudoFunction(SyntaxToken prefix, IdentifierTree pseudoFunctionName, ParametersTree parameters) {
return new PseudoFunctionTreeImpl(prefix, pseudoFunctionName, parameters);
}
public PseudoIdentifierTree pseudoIdentifier(SyntaxToken prefix, IdentifierTree identifier) {
return new PseudoIdentifierTreeImpl(prefix, identifier);
}
public AttributeSelectorTree attributeSelector(SyntaxToken openBracket, IdentifierTree attribute,
Optional matcherExpression, SyntaxToken closeBracket) {
return new AttributeSelectorTreeImpl(openBracket, null, attribute, matcherExpression.orNull(), closeBracket);
}
public AttributeSelectorTree attributeSelector(SyntaxToken openBracket, TreeImpl spacing, NamespaceTree namespace, IdentifierTree attribute,
Optional matcherExpression, SyntaxToken closeBracket) {
return new AttributeSelectorTreeImpl(openBracket, namespace, attribute, matcherExpression.orNull(), closeBracket);
}
public AttributeMatcherTree attributeMatcher(SyntaxToken matcher) {
return new AttributeMatcherTreeImpl(matcher);
}
public AttributeMatcherExpressionTree attributeMatcherExpression(AttributeMatcherTree attributeMatcher, Tree toMatch, Optional caseInsensitiveFlag) {
return new AttributeMatcherExpressionTreeImpl(attributeMatcher, toMatch, caseInsensitiveFlag.orNull());
}
public NamespaceTree namespace(Optional namespace, SyntaxToken pipe) {
return new NamespaceTreeImpl(namespace.orNull(), pipe);
}
public ImportantFlagTree important(SyntaxToken exclamationMark, SyntaxToken importantKeyword) {
return new ImportantFlagTreeImpl(exclamationMark, importantKeyword);
}
public IdentifierTree identifier(SyntaxToken identifier) {
return new IdentifierTreeImpl(identifier);
}
public IdentifierTree identifierNoWs(SyntaxToken identifier) {
return identifier(identifier);
}
public AtKeywordTree atKeyword(SyntaxToken atSymbol, IdentifierTree keyword) {
return new AtKeywordTreeImpl(atSymbol, keyword);
}
public HashTree hash(SyntaxToken hashSymbol, SyntaxToken value) {
return new HashTreeImpl(hashSymbol, value);
}
public PercentageTree percentage(NumberTree value, SyntaxToken percentageSymbol) {
return new PercentageTreeImpl(value, percentageSymbol);
}
public DimensionTree dimension(NumberTree value, UnitTree unit) {
return new DimensionTreeImpl(value, unit);
}
public StringTree string(SyntaxToken string) {
return new StringTreeImpl(string);
}
public StringTree stringNoWs(SyntaxToken string) {
return new StringTreeImpl(string);
}
public NumberTree number(SyntaxToken number) {
return new NumberTreeImpl(number);
}
public ValueCommaSeparatedListTree valueCommaSeparatedList(ValueTree value, List> subsequentValues, Optional trailingComma) {
List values = Lists.newArrayList(value);
List separators = Lists.newArrayList();
for (Tuple t : subsequentValues) {
separators.add(t.first());
values.add(t.second());
}
if (trailingComma.isPresent()) {
separators.add(trailingComma.get());
}
return new ValueCommaSeparatedListTreeImpl(new SeparatedList<>(values, separators));
}
public ValueCommaSeparatedListTree valueCommaSeparatedListOneSingleValue(ValueTree value, DelimiterTree trailingComma) {
List values = Lists.newArrayList(value);
List separators = Lists.newArrayList(trailingComma);
return new ValueCommaSeparatedListTreeImpl(new SeparatedList<>(values, separators));
}
public DelimiterTree delimiter(SyntaxToken delimiter) {
return new DelimiterTreeImpl(delimiter);
}
public DelimiterTree commaDelimiter(SyntaxToken comma) {
return new DelimiterTreeImpl(comma);
}
public CaseInsensitiveFlagTree caseInsensitiveFlag(SyntaxToken flag) {
return new CaseInsensitiveFlagTreeImpl(flag);
}
public UnicodeRangeTree unicodeRange(SyntaxToken unicodeRange) {
return new UnicodeRangeTreeImpl(unicodeRange);
}
public VariableTree variable(SyntaxToken variablePrefix, IdentifierTree variable) {
return new VariableTreeImpl(variablePrefix, variable);
}
public UnitTree unit(SyntaxToken unit) {
return new UnitTreeImpl(unit);
}
// ---------------------------------
// Embedded CSS
// ---------------------------------
public StyleSheetTree stylesheet(Optional> statements) {
return new StyleSheetTreeImpl(null, statements.orNull(), null);
}
public CssInStyleTagTree cssInStyleTag(SyntaxToken openingTag, StyleSheetTree styleSheet, SyntaxToken closingTag) {
return new CssInStyleTagTreeImpl(openingTag, styleSheet, closingTag);
}
public FileWithEmbeddedCssTree fileWithEmbeddedCss(Optional> trees, SyntaxToken eof) {
return new FileWithEmbeddedCssTreeImpl(trees.orNull(), eof);
}
// ---------------------------------
// Common to SCSS and Less
// ---------------------------------
public StatementBlockTree rulesetBlock(StatementBlockTree block) {
return new StatementBlockTreeImpl(block);
}
public StatementBlockTree atRuleBlock(StatementBlockTree block) {
return new StatementBlockTreeImpl(block);
}
public StatementBlockTree statementBlock(SyntaxToken openCurlyBrace, Optional> content, SyntaxToken closeCurlyBrace) {
return new StatementBlockTreeImpl(openCurlyBrace, content.orNull(), closeCurlyBrace);
}
// ---------------------------------
// SCSS
// ---------------------------------
public SelectorTree scssSelector(Optional parentCombinator, SeparatedList selectors, Optional blockCombinator) {
return new SelectorTreeImpl(parentCombinator.orNull(), selectors, blockCombinator.orNull());
}
public ScssParentSelectorTree scssParentSelector(SyntaxToken parentSelector) {
return new ScssParentSelectorTreeImpl(parentSelector);
}
public ScssPlaceholderSelectorTree scssPlaceholderSelector(SyntaxToken prefix, IdentifierTree name) {
return new ScssPlaceholderSelectorTreeImpl(prefix, name);
}
public SelectorCombinatorTree scssParentSelectorCombinator(SyntaxToken combinator) {
return new SelectorCombinatorTreeImpl(combinator);
}
public ScssNestedPropertiesDeclarationTree scssNestedPropertiesDeclaration(PropertyTree namespace, SyntaxToken colon, StatementBlockTree block) {
return new ScssNestedPropertiesDeclarationTreeImpl(namespace, colon, block);
}
public ScssVariableDeclarationTree scssVariableDeclaration(ScssVariableTree variable, SyntaxToken colon, ValueTree value, Optional semicolon) {
return new ScssVariableDeclarationTreeImpl(variable, colon, value, semicolon.orNull());
}
public ScssVariableDeclarationTree scssVariableDeclarationAsParameter(ScssVariableTree variable, SyntaxToken colon, ValueTree value) {
return new ScssVariableDeclarationTreeImpl(variable, colon, value, null);
}
public ScssVariableTree scssVariable(SyntaxToken prefix, IdentifierTree name) {
return new ScssVariableTreeImpl(prefix, name);
}
public ScssVariableArgumentTree scssVariableArgument(SyntaxToken prefix, IdentifierTree name, SyntaxToken ellipsis) {
return new ScssVariableArgumentTreeImpl(prefix, name, ellipsis);
}
public ScssDefaultFlagTree scssDefaultFlag(SyntaxToken flag) {
return new ScssDefaultFlagTreeImpl(flag);
}
public ScssGlobalFlagTree scssGlobalFlag(SyntaxToken flag) {
return new ScssGlobalFlagTreeImpl(flag);
}
public ScssOptionalFlagTree scssOptionalFlag(SyntaxToken flag) {
return new ScssOptionalFlagTreeImpl(flag);
}
public ScssFunctionTree scssFunction(ScssDirectiveTree directive, IdentifierTree name, Optional parameters, StatementBlockTree block) {
return new ScssFunctionTreeImpl(directive, name, parameters.orNull(), block);
}
public ScssMixinTree scssMixin(ScssDirectiveTree directive, IdentifierTree name, Optional parameters, StatementBlockTree block) {
return new ScssMixinTreeImpl(directive, name, parameters.orNull(), block);
}
public ScssIncludeTree scssInclude(ScssDirectiveTree directive, IdentifierTree name, Optional parameters, Optional block, Optional semicolon) {
return new ScssIncludeTreeImpl(directive, name, parameters.orNull(), block.orNull(), semicolon.orNull());
}
public ScssParametersTree scssParameters(SyntaxToken openParenthesis, Optional> parameters, SyntaxToken closeParenthesis) {
return new ScssParametersTreeImpl(openParenthesis, parameters.orNull(), closeParenthesis);
}
public ScssParametersTree scssCallParameters(SyntaxToken openParenthesis, Optional> parameters, SyntaxToken closeParenthesis) {
return new ScssParametersTreeImpl(openParenthesis, parameters.orNull(), closeParenthesis);
}
public SeparatedList scssCallParameterList(ScssParameterTree parameter, Optional>> subsequentParameters) {
return scssParameterList(parameter, subsequentParameters);
}
public SeparatedList scssParameterList(ScssParameterTree parameter, Optional>> subsequentParameters) {
List parameters = Lists.newArrayList(parameter);
List separators = Lists.newArrayList();
if (subsequentParameters.isPresent()) {
for (Tuple t : subsequentParameters.get()) {
separators.add(t.first());
parameters.add(t.second());
}
}
return new SeparatedList<>(parameters, separators);
}
public ScssParameterTree scssParameter(Tree parameter) {
return new ScssParameterTreeImpl(parameter);
}
public ScssParameterTree scssCallParameter(Tree parameter) {
return new ScssParameterTreeImpl(parameter);
}
public ScssExtendTree scssExtend(ScssDirectiveTree directive, CompoundSelectorTree compoundSelector, Optional optionalFlag, Optional semicolon) {
return new ScssExtendTreeImpl(directive, compoundSelector, optionalFlag.orNull(), semicolon.orNull());
}
public ScssContentTree scssContent(ScssDirectiveTree directive, Optional semicolon) {
return new ScssContentTreeImpl(directive, semicolon.orNull());
}
public ScssDebugTree scssDebug(ScssDirectiveTree directive, ValueTree value, Optional semicolon) {
return new ScssDebugTreeImpl(directive, value, semicolon.orNull());
}
public ScssWarnTree scssWarn(ScssDirectiveTree directive, ValueTree value, Optional semicolon) {
return new ScssWarnTreeImpl(directive, value, semicolon.orNull());
}
public ScssErrorTree scssError(ScssDirectiveTree directive, ValueTree value, Optional semicolon) {
return new ScssErrorTreeImpl(directive, value, semicolon.orNull());
}
public ScssReturnTree scssReturn(ScssDirectiveTree directive, ValueTree value, Optional semicolon) {
return new ScssReturnTreeImpl(directive, value, semicolon.orNull());
}
public ScssIfElseIfElseTree scssIfElseIfElse(ScssIfTree ife, Optional> elseIf, Optional elsee) {
return new ScssIfElseIfElseTreeImpl(ife, elseIf.orNull(), elsee.orNull());
}
public ScssIfTree scssIf(ScssDirectiveTree directive, ScssConditionTree condition, StatementBlockTree block) {
return new ScssIfTreeImpl(directive, condition, block);
}
public ScssElseIfTree scssElseIf(ScssDirectiveTree directive, ScssConditionTree condition, StatementBlockTree block) {
return new ScssElseIfTreeImpl(directive, condition, block);
}
public ScssWhileTree scssWhile(ScssDirectiveTree directive, ScssConditionTree condition, StatementBlockTree block) {
return new ScssWhileTreeImpl(directive, condition, block);
}
public ScssElseTree scssElse(ScssDirectiveTree directive, StatementBlockTree block) {
return new ScssElseTreeImpl(directive, block);
}
public ScssForTree scssFor(ScssDirectiveTree directive, ScssConditionTree condition, StatementBlockTree block) {
return new ScssForTreeImpl(directive, condition, block);
}
public ScssEachTree scssEach(ScssDirectiveTree directive, ScssConditionTree condition, StatementBlockTree block) {
return new ScssEachTreeImpl(directive, condition, block);
}
public ScssAtRootTree scssAtRoot(ScssDirectiveTree directive, Optional parameters, Tree content) {
return new ScssAtRootTreeImpl(directive, parameters.orNull(), content);
}
public ScssAtRootParametersTree scssAtRootParameters(SyntaxToken openParenthesis, SyntaxToken parameter, SyntaxToken colon, List values, SyntaxToken closeParenthesis) {
return new ScssAtRootParametersTreeImpl(openParenthesis, parameter, colon, values, closeParenthesis);
}
public IdentifierTree scssInterpolatedIdentifier(SyntaxToken identifier) {
return identifier(identifier);
}
public IdentifierTree scssInterpolatedIdentifierNoWs(SyntaxToken identifier) {
return identifier(identifier);
}
public ScssOperatorTree scssOperator(SyntaxToken operator) {
return new ScssOperatorTreeImpl(operator);
}
public ScssMultilineStringTree scssMultilineString(SyntaxToken string) {
return new ScssMultilineStringTreeImpl(string);
}
public ScssConditionTree scssCondition(ValueTree condition) {
return new ScssConditionTreeImpl(condition);
}
public ScssDirectiveTree scssExtendDirective(SyntaxToken at, SyntaxToken name) {
return new ScssDirectiveTreeImpl(at, name);
}
public ScssDirectiveTree scssContentDirective(SyntaxToken at, SyntaxToken name) {
return new ScssDirectiveTreeImpl(at, name);
}
public ScssDirectiveTree scssDebugDirective(SyntaxToken at, SyntaxToken name) {
return new ScssDirectiveTreeImpl(at, name);
}
public ScssDirectiveTree scssWarnDirective(SyntaxToken at, SyntaxToken name) {
return new ScssDirectiveTreeImpl(at, name);
}
public ScssDirectiveTree scssErrorDirective(SyntaxToken at, SyntaxToken name) {
return new ScssDirectiveTreeImpl(at, name);
}
public ScssDirectiveTree scssIfDirective(SyntaxToken at, SyntaxToken name) {
return new ScssDirectiveTreeImpl(at, name);
}
public ScssDirectiveTree scssElseIfDirective(SyntaxToken at, SyntaxToken name) {
return new ScssDirectiveTreeImpl(at, name);
}
public ScssDirectiveTree scssElseDirective(SyntaxToken at, SyntaxToken name) {
return new ScssDirectiveTreeImpl(at, name);
}
public ScssDirectiveTree scssForDirective(SyntaxToken at, SyntaxToken name) {
return new ScssDirectiveTreeImpl(at, name);
}
public ScssDirectiveTree scssWhileDirective(SyntaxToken at, SyntaxToken name) {
return new ScssDirectiveTreeImpl(at, name);
}
public ScssDirectiveTree scssEachDirective(SyntaxToken at, SyntaxToken name) {
return new ScssDirectiveTreeImpl(at, name);
}
public ScssDirectiveTree scssMixinDirective(SyntaxToken at, SyntaxToken name) {
return new ScssDirectiveTreeImpl(at, name);
}
public ScssDirectiveTree scssIncludeDirective(SyntaxToken at, SyntaxToken name) {
return new ScssDirectiveTreeImpl(at, name);
}
public ScssDirectiveTree scssFunctionDirective(SyntaxToken at, SyntaxToken name) {
return new ScssDirectiveTreeImpl(at, name);
}
public ScssDirectiveTree scssReturnDirective(SyntaxToken at, SyntaxToken name) {
return new ScssDirectiveTreeImpl(at, name);
}
public ScssDirectiveTree scssAtRootDirective(SyntaxToken at, SyntaxToken name) {
return new ScssDirectiveTreeImpl(at, name);
}
public ScssMapTree scssMap(SyntaxToken openParenthesis, SeparatedList entries, SyntaxToken closeParenthesis) {
return new ScssMapTreeImpl(openParenthesis, entries, closeParenthesis);
}
public SeparatedList scssMapEntryList(ScssMapEntryTree mapEntry, Optional>> subsequentMapEntries, Optional trailingComma) {
List mapEntries = Lists.newArrayList(mapEntry);
List commas = Lists.newArrayList();
if (subsequentMapEntries.isPresent()) {
for (Tuple t : subsequentMapEntries.get()) {
commas.add(t.first());
mapEntries.add(t.second());
}
}
if (trailingComma.isPresent()) {
commas.add(trailingComma.get());
}
return new SeparatedList<>(mapEntries, commas);
}
public ScssMapEntryTree scssMapEntry(ValueTree key, SyntaxToken colon, ValueTree value) {
return new ScssMapEntryTreeImpl(key, colon, value);
}
public ValueTree simpleValueSassScriptExpression(List valueElements) {
return new ValueTreeImpl(valueElements);
}
public ValueTree simpleValueSassScriptExpressionWithoutCommaSeparatedList(List valueElements) {
return new ValueTreeImpl(valueElements);
}
// ---------------------------------
// Less
// ---------------------------------
public PropertyTree property(IdentifierTree property, Optional merge) {
return new PropertyTreeImpl(property, merge.orNull());
}
public SelectorTree lessSelector(Optional parentCombinator, SeparatedList selectors, Optional extend,
Optional parameters, Optional guard) {
return new SelectorTreeImpl(parentCombinator.orNull(), selectors, extend.orNull(), parameters.orNull(), guard.orNull());
}
public LessVariableDeclarationTree lessVariableDeclaration(LessVariableTree variable, SyntaxToken colon, ValueTree value, Optional semicolon) {
return new LessVariableDeclarationTreeImpl(variable, colon, value, semicolon.orNull());
}
public LessVariableTree lessVariable(SyntaxToken variablePrefix, IdentifierTree variable) {
return new LessVariableTreeImpl(variablePrefix, variable);
}
public IdentifierTree lessInterpolatedIdentifier(SyntaxToken identifier) {
return identifier(identifier);
}
public IdentifierTree lessInterpolatedIdentifierNoWs(SyntaxToken identifier) {
return identifier(identifier);
}
public IdentifierTree lessIdentifierNoWsNorWhen(SyntaxToken identifier) {
return identifier(identifier);
}
public LessExtendTree lessExtend(SyntaxToken extendKeyword, SyntaxToken openParenthesis, List parameterElements, SyntaxToken closeParenthesis) {
return new LessExtendTreeImpl(extendKeyword, openParenthesis, parameterElements, closeParenthesis);
}
public LessParentSelectorTree lessParentSelector(SyntaxToken parentSelector) {
return new LessParentSelectorTreeImpl(parentSelector);
}
public SelectorCombinatorTree lessParentSelectorCombinator(SyntaxToken combinator) {
return new SelectorCombinatorTreeImpl(combinator);
}
public LessMixinCallTree lessMixinCall(Optional parentCombinator, Optional spacing, SelectorTree selector, Optional important,
Optional semicolon) {
return new LessMixinCallTreeImpl(parentCombinator.orNull(), selector, important.orNull(), semicolon.orNull());
}
public LessMixinGuardTree lessMixinGuard(SyntaxToken when, Optional not, SeparatedList conditions) {
return new LessMixinGuardTreeImpl(when, not.orNull(), conditions);
}
public SeparatedList lessMixinGuardConditionList(ParenthesisBlockTree condition, Optional>> subsequentConditions) {
List conditions = Lists.newArrayList(condition);
List separators = Lists.newArrayList();
if (subsequentConditions.isPresent()) {
for (Tuple t : subsequentConditions.get()) {
separators.add(t.first());
conditions.add(t.second());
}
}
return new SeparatedList<>(conditions, separators);
}
public LessMixinParametersTree lessMixinParameters(SyntaxToken openParenthesis, Optional> parameters, SyntaxToken closeParenthesis) {
return new LessMixinParametersTreeImpl(openParenthesis, parameters.orNull(), closeParenthesis);
}
public SeparatedList lessMixinParameterList(LessMixinParameterTree parameter, Optional>> subsequentParameters, Optional trailingSeparator) {
List parameters = Lists.newArrayList(parameter);
List separators = Lists.newArrayList();
if (subsequentParameters.isPresent()) {
for (Tuple t : subsequentParameters.get()) {
separators.add(t.first());
parameters.add(t.second());
}
}
if (trailingSeparator.isPresent()) {
separators.add(trailingSeparator.get());
}
return new SeparatedList<>(parameters, separators);
}
public LessMixinParameterTree lessMixinParameter(LessVariableTree variable, Optional colon, Optional value) {
return new LessMixinParameterTreeImpl(variable, colon.orNull(), value.orNull());
}
public LessMixinParameterTree lessMixinParameter(ValueTree value) {
return new LessMixinParameterTreeImpl(null, null, value);
}
public LessEscapingTree lessEscaping(SyntaxToken escapingSymbol, StringTree string) {
return new LessEscapingTreeImpl(escapingSymbol, string);
}
public LessOperatorTree lessOperator(SyntaxToken operator) {
return new LessOperatorTreeImpl(operator);
}
// ---------------------------------
// Tuple
// ---------------------------------
public static class Tuple {
private final T first;
private final U second;
public Tuple(T first, U second) {
super();
this.first = first;
this.second = second;
}
public T first() {
return first;
}
public U second() {
return second;
}
}
private static Tuple newTuple(T first, U second) {
return new Tuple<>(first, second);
}
public Tuple newTuple1(T first, U second) {
return newTuple(first, second);
}
public Tuple newTuple2(T first, U second) {
return newTuple(first, second);
}
public Tuple newTuple3(T first, U second) {
return newTuple(first, second);
}
public Tuple newTuple4(T first, U second) {
return newTuple(first, second);
}
public Tuple newTuple5(T first, U second) {
return newTuple(first, second);
}
public Tuple newTuple6(T first, U second) {
return newTuple(first, second);
}
public Tuple newTuple7(T first, U second) {
return newTuple(first, second);
}
public Tuple newTuple8(T first, U second) {
return newTuple(first, second);
}
public Tuple newTuple9(T first, U second) {
return newTuple(first, second);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy