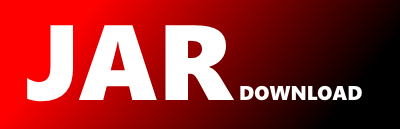
org.apache.maven.dotnet.HelpMojo Maven / Gradle / Ivy
Go to download
A plugin that provides general build and test facilities for .Net projects and solutions
The newest version!
package org.apache.maven.dotnet;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
/**
* Display help information on maven-dotnet-plugin.
Call mvn dotnet:help -Ddetail=true -Dgoal=<goal-name>
to display parameter details.
*
* @version generated on Tue Feb 07 10:32:41 CET 2012
* @author org.apache.maven.tools.plugin.generator.PluginHelpGenerator (version 2.6)
* @goal help
* @requiresProject false
*/
public class HelpMojo
extends AbstractMojo
{
/**
* If true
, display all settable properties for each goal.
*
* @parameter expression="${detail}" default-value="false"
*/
private boolean detail;
/**
* The name of the goal for which to show help. If unspecified, all goals will be displayed.
*
* @parameter expression="${goal}"
*/
private java.lang.String goal;
/**
* The maximum length of a display line, should be positive.
*
* @parameter expression="${lineLength}" default-value="80"
*/
private int lineLength;
/**
* The number of spaces per indentation level, should be positive.
*
* @parameter expression="${indentSize}" default-value="2"
*/
private int indentSize;
/** {@inheritDoc} */
public void execute()
throws MojoExecutionException
{
if ( lineLength <= 0 )
{
getLog().warn( "The parameter 'lineLength' should be positive, using '80' as default." );
lineLength = 80;
}
if ( indentSize <= 0 )
{
getLog().warn( "The parameter 'indentSize' should be positive, using '2' as default." );
indentSize = 2;
}
StringBuffer sb = new StringBuffer();
append( sb, "org.codehaus.sonar-plugins.dotnet:maven-dotnet-plugin:1.2", 0 );
append( sb, "", 0 );
append( sb, ".Net build plugin project", 0 );
append( sb, "A plugin that provides general build and test facilities for .Net projects and solutions", 1 );
append( sb, "", 0 );
if ( goal == null || goal.length() <= 0 )
{
append( sb, "This plugin has 13 goals:", 0 );
append( sb, "", 0 );
}
if ( goal == null || goal.length() <= 0 || "clean".equals( goal ) )
{
append( sb, "dotnet:clean", 0 );
append( sb, "Cleans the build objects generated for a .Net project or solution", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "assemblyDirectories", 2 );
append( sb, "Locations of the assemblies that should be analysed in case default location should not be used. Actually AOP tools such as PostSharp mess up the cil and may provoke false positives. Project names should be used as keys. Values should be the path to the wanted DLLs. If the assembly of the \'Cache\' project is located in the \'compile\' directory instead of usual \'bin/Debug\', you can add in the configuration section of the plugin something like compile ", 3 );
append( sb, "", 0 );
append( sb, "buildConfigurations (Default: Debug)", 2 );
append( sb, "The build configurations to use for the project or solution, separated by colons or semi-colons as in \'Debug,Release\'. Default value is \'Debug\'. If value is \'ALL\', all the build configurations defined in the sln file will be used.", 3 );
append( sb, "", 0 );
append( sb, "dotnet_2_0_sdk_directory (Default: C:/WINDOWS/Microsoft.NET/Framework/v2.0.50727)", 2 );
append( sb, "Installation directory of the .Net SDK 2.0", 3 );
append( sb, "", 0 );
append( sb, "dotnet_3_5_sdk_directory (Default: C:/WINDOWS/Microsoft.NET/Framework/v3.5)", 2 );
append( sb, "Installation directory of the .Net SDK 3.5", 3 );
append( sb, "", 0 );
append( sb, "dotnet_4_0_sdk_directory (Default: C:/WINDOWS/Microsoft.NET/Framework/v4.0.30319)", 2 );
append( sb, "Installation directory of the .Net SDK 4.0", 3 );
append( sb, "", 0 );
append( sb, "generatePdb (Default: true)", 2 );
append( sb, "Defines if the build should generate debug symbols (typically .pdb files)", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "Location of the output files", 3 );
append( sb, "", 0 );
append( sb, "parallelBuild (Default: false)", 2 );
append( sb, "Flag that indicates if parallel builds are activated. When equals to true, \'/m\' MsBuild option is added to the command line and MsBuild will try to use all available cpu cores.", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "The maven project.", 3 );
append( sb, "", 0 );
append( sb, "projectName", 2 );
append( sb, "The name of the project to use if it doesn\'t have the same name as the artifact with a \'.csproj\' extension.", 3 );
append( sb, "", 0 );
append( sb, "skippedProjects", 2 );
append( sb, "List of the excluded projects, using \',\' as delimiter. C# files of these projects will be ignored. No violation on files of these projects should be reported.", 3 );
append( sb, "", 0 );
append( sb, "solutionName", 2 );
append( sb, "The name of the solution to use in case there are multiple solutions in the folder AND none has the same name as the artifact with a \'.sln\' extension.", 3 );
append( sb, "", 0 );
append( sb, "testProjectPattern (Default: *.Tests)", 2 );
append( sb, "A pattern that will help to figure out if a project is a test project from its name. The \'*\' and \'?\' common jokers are accepted. Several patterns may be specified using \';\' as a delimiter.", 3 );
append( sb, "", 0 );
append( sb, "toolVersion (Default: 3.5)", 2 );
append( sb, "Version of the MSBuild tool to use, which is the installed version of the SDK. Possible values are 2.0, 3.5 and 4.0.", 3 );
append( sb, "", 0 );
append( sb, "traceCommands", 2 );
append( sb, "Defines if the launched commands should be appended into a \'command.txt\' file. For debug purpose.", 3 );
append( sb, "", 0 );
append( sb, "useEmbbededRuntime (Default: true)", 2 );
append( sb, "Defines if the plugin can use the maven-dotnet-runtime artifact to export the relevant .Net quality applications instead of using their global path. The defined path is always taken in priority.", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "compile".equals( goal ) )
{
append( sb, "dotnet:compile", 0 );
append( sb, "Builds a .Net project of solution using MSBuild", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "assemblyDirectories", 2 );
append( sb, "Locations of the assemblies that should be analysed in case default location should not be used. Actually AOP tools such as PostSharp mess up the cil and may provoke false positives. Project names should be used as keys. Values should be the path to the wanted DLLs. If the assembly of the \'Cache\' project is located in the \'compile\' directory instead of usual \'bin/Debug\', you can add in the configuration section of the plugin something like compile ", 3 );
append( sb, "", 0 );
append( sb, "buildConfigurations (Default: Debug)", 2 );
append( sb, "The build configurations to use for the project or solution, separated by colons or semi-colons as in \'Debug,Release\'. Default value is \'Debug\'. If value is \'ALL\', all the build configurations defined in the sln file will be used.", 3 );
append( sb, "", 0 );
append( sb, "disablePostBuildEvent (Default: false)", 2 );
append( sb, "A flag to disable the post build events during a compilation.", 3 );
append( sb, "", 0 );
append( sb, "disablePreBuildEvent (Default: false)", 2 );
append( sb, "A flag to disable the pre-build events during a compilation.", 3 );
append( sb, "", 0 );
append( sb, "dotnet_2_0_sdk_directory (Default: C:/WINDOWS/Microsoft.NET/Framework/v2.0.50727)", 2 );
append( sb, "Installation directory of the .Net SDK 2.0", 3 );
append( sb, "", 0 );
append( sb, "dotnet_3_5_sdk_directory (Default: C:/WINDOWS/Microsoft.NET/Framework/v3.5)", 2 );
append( sb, "Installation directory of the .Net SDK 3.5", 3 );
append( sb, "", 0 );
append( sb, "dotnet_4_0_sdk_directory (Default: C:/WINDOWS/Microsoft.NET/Framework/v4.0.30319)", 2 );
append( sb, "Installation directory of the .Net SDK 4.0", 3 );
append( sb, "", 0 );
append( sb, "generatePdb (Default: true)", 2 );
append( sb, "Defines if the build should generate debug symbols (typically .pdb files)", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "Location of the output files", 3 );
append( sb, "", 0 );
append( sb, "parallelBuild (Default: false)", 2 );
append( sb, "Flag that indicates if parallel builds are activated. When equals to true, \'/m\' MsBuild option is added to the command line and MsBuild will try to use all available cpu cores.", 3 );
append( sb, "", 0 );
append( sb, "platforms (Default: Any CPU)", 2 );
append( sb, "The target platforms for the compilation. Comma may be used to specify several platforms. This parameter is not mandatory.", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "The maven project.", 3 );
append( sb, "", 0 );
append( sb, "projectName", 2 );
append( sb, "The name of the project to use if it doesn\'t have the same name as the artifact with a \'.csproj\' extension.", 3 );
append( sb, "", 0 );
append( sb, "rebuild (Default: false)", 2 );
append( sb, "A flag to use to force the rebuild of the project or solution.", 3 );
append( sb, "", 0 );
append( sb, "skippedProjects", 2 );
append( sb, "List of the excluded projects, using \',\' as delimiter. C# files of these projects will be ignored. No violation on files of these projects should be reported.", 3 );
append( sb, "", 0 );
append( sb, "solutionName", 2 );
append( sb, "The name of the solution to use in case there are multiple solutions in the folder AND none has the same name as the artifact with a \'.sln\' extension.", 3 );
append( sb, "", 0 );
append( sb, "testProjectPattern (Default: *.Tests)", 2 );
append( sb, "A pattern that will help to figure out if a project is a test project from its name. The \'*\' and \'?\' common jokers are accepted. Several patterns may be specified using \';\' as a delimiter.", 3 );
append( sb, "", 0 );
append( sb, "toolVersion (Default: 3.5)", 2 );
append( sb, "Version of the MSBuild tool to use, which is the installed version of the SDK. Possible values are 2.0, 3.5 and 4.0.", 3 );
append( sb, "", 0 );
append( sb, "traceCommands", 2 );
append( sb, "Defines if the launched commands should be appended into a \'command.txt\' file. For debug purpose.", 3 );
append( sb, "", 0 );
append( sb, "useEmbbededRuntime (Default: true)", 2 );
append( sb, "Defines if the plugin can use the maven-dotnet-runtime artifact to export the relevant .Net quality applications instead of using their global path. The defined path is always taken in priority.", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "coverage".equals( goal ) )
{
append( sb, "dotnet:coverage", 0 );
append( sb, "Generates a coverage report on a .Net project or solution using the unit tests and PartCover/NCover", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "assemblyDirectories", 2 );
append( sb, "Locations of the assemblies that should be analysed in case default location should not be used. Actually AOP tools such as PostSharp mess up the cil and may provoke false positives. Project names should be used as keys. Values should be the path to the wanted DLLs. If the assembly of the \'Cache\' project is located in the \'compile\' directory instead of usual \'bin/Debug\', you can add in the configuration section of the plugin something like compile ", 3 );
append( sb, "", 0 );
append( sb, "buildConfigurations (Default: Debug)", 2 );
append( sb, "The build configurations to use for the project or solution, separated by colons or semi-colons as in \'Debug,Release\'. Default value is \'Debug\'. If value is \'ALL\', all the build configurations defined in the sln file will be used.", 3 );
append( sb, "", 0 );
append( sb, "coverageExcludes", 2 );
append( sb, "Namespaces and assemblies excluded from the code coverage. The format is the PartCover format: \'[assembly]namespace\'", 3 );
append( sb, "", 0 );
append( sb, "coverageReportFileName (Default: coverage-test-report.xml)", 2 );
append( sb, "The output file for test.", 3 );
append( sb, "", 0 );
append( sb, "coverageReportName (Default: coverage-report.xml)", 2 );
append( sb, "Name of the coverage report XML file.", 3 );
append( sb, "", 0 );
append( sb, "filter", 2 );
append( sb, "Optional test filter for gallio. This can be used to execute only a specific test category (i.e. CategotyName:unit to consider only tests from the \'unit\' category)", 3 );
append( sb, "", 0 );
append( sb, "gallioDirectory", 2 );
append( sb, "Location of the Gallio installation", 3 );
append( sb, "", 0 );
append( sb, "gallioExecutable (Default: Gallio.Echo.exe)", 2 );
append( sb, "Name of the Gallio executable file", 3 );
append( sb, "", 0 );
append( sb, "gallioRunner (Default: IsolatedProcess)", 2 );
append( sb, "Name of the Gallio runner to use", 3 );
append( sb, "", 0 );
append( sb, "generatePdb (Default: true)", 2 );
append( sb, "Defines if the build should generate debug symbols (typically .pdb files)", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "Location of the output files", 3 );
append( sb, "", 0 );
append( sb, "partCoverDirectory", 2 );
append( sb, "Location of the PartCover installation.", 3 );
append( sb, "", 0 );
append( sb, "partCoverExecutable (Default: PartCover.exe)", 2 );
append( sb, "Name of the PartCover executable.", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "The maven project.", 3 );
append( sb, "", 0 );
append( sb, "projectName", 2 );
append( sb, "The name of the project to use if it doesn\'t have the same name as the artifact with a \'.csproj\' extension.", 3 );
append( sb, "", 0 );
append( sb, "reportFileName (Default: gallio-report.xml)", 2 );
append( sb, "The output file for Gallio.", 3 );
append( sb, "", 0 );
append( sb, "skip", 2 );
append( sb, "Set this to \'true\' to bypass unit tests entirely. Its use is NOT RECOMMENDED, especially if you enable it using the \'maven.test.skip\' property, because maven.test.skip disables both running the tests and compiling the tests. Consider using the skipTests parameter instead.", 3 );
append( sb, "", 0 );
append( sb, "skippedProjects", 2 );
append( sb, "List of the excluded projects, using \',\' as delimiter. C# files of these projects will be ignored.", 3 );
append( sb, "", 0 );
append( sb, "skipTests", 2 );
append( sb, "Set this to \'true\' to skip running tests, but still compile them. Its use is NOT RECOMMENDED, but quite convenient on occasion.", 3 );
append( sb, "", 0 );
append( sb, "solutionName", 2 );
append( sb, "The name of the solution to use in case there are multiple solutions in the folder AND none has the same name as the artifact with a \'.sln\' extension.", 3 );
append( sb, "", 0 );
append( sb, "testFailureIgnore (Default: false)", 2 );
append( sb, "Set this to true to ignore a failure during testing. Its use is NOT RECOMMENDED, but quite convenient on occasion.", 3 );
append( sb, "", 0 );
append( sb, "testProjectPattern (Default: *.Tests)", 2 );
append( sb, "A pattern that will help to figure out if a project is a test project from its name. The \'*\' and \'?\' common jokers are accepted. Several patterns may be specified using \';\' as a delimiter.", 3 );
append( sb, "", 0 );
append( sb, "traceCommands", 2 );
append( sb, "Defines if the launched commands should be appended into a \'command.txt\' file. For debug purpose.", 3 );
append( sb, "", 0 );
append( sb, "useEmbbededRuntime (Default: true)", 2 );
append( sb, "Defines if the plugin can use the maven-dotnet-runtime artifact to export the relevant .Net quality applications instead of using their global path. The defined path is always taken in priority.", 3 );
append( sb, "", 0 );
append( sb, "useNCover", 2 );
append( sb, "Set this true to use NCover3 instead of PartCover", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "cpd".equals( goal ) )
{
append( sb, "dotnet:cpd", 0 );
append( sb, "Maven plugin for dotnet cpd analysis. Contains copy/paste code from class CpdReport (it was impossible to inherit from AbstractDotNetMojo and CpdReport at the same time)", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "aggregate (Default: false)", 2 );
append( sb, "Whether to build an aggregated report at the root, or build individual reports.", 3 );
append( sb, "", 0 );
append( sb, "assemblyDirectories", 2 );
append( sb, "Locations of the assemblies that should be analysed in case default location should not be used. Actually AOP tools such as PostSharp mess up the cil and may provoke false positives. Project names should be used as keys. Values should be the path to the wanted DLLs. If the assembly of the \'Cache\' project is located in the \'compile\' directory instead of usual \'bin/Debug\', you can add in the configuration section of the plugin something like compile ", 3 );
append( sb, "", 0 );
append( sb, "buildConfigurations (Default: Debug)", 2 );
append( sb, "The build configurations to use for the project or solution, separated by colons or semi-colons as in \'Debug,Release\'. Default value is \'Debug\'. If value is \'ALL\', all the build configurations defined in the sln file will be used.", 3 );
append( sb, "", 0 );
append( sb, "format (Default: xml)", 2 );
append( sb, "Set the output format type, in addition to the HTML report. Must be one of: \'none\', \'csv\', \'xml\', \'txt\' or the full class name of the PMD renderer to use. See the net.sourceforge.pmd.renderers package javadoc for available renderers. XML is required if the pmd:check goal is being used.", 3 );
append( sb, "", 0 );
append( sb, "generatePdb (Default: true)", 2 );
append( sb, "Defines if the build should generate debug symbols (typically .pdb files)", 3 );
append( sb, "", 0 );
append( sb, "includeTests (Default: false)", 2 );
append( sb, "Run PMD on the tests.", 3 );
append( sb, "", 0 );
append( sb, "minimumTokens (Default: 50)", 2 );
append( sb, "The minimum number of tokens that need to be duplicated before it causes a violation.", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "Location of the output files", 3 );
append( sb, "", 0 );
append( sb, "outputReportingDirectory", 2 );
append( sb, "The output directory for the final HTML report. Note that this parameter is only evaluated if the goal is run directly from the command line or during the default lifecycle. If the goal is run indirectly as part of a site generation, the output directory configured in the Maven Site Plugin is used instead.", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "The maven project.", 3 );
append( sb, "", 0 );
append( sb, "projectName", 2 );
append( sb, "The name of the project to use if it doesn\'t have the same name as the artifact with a \'.csproj\' extension.", 3 );
append( sb, "", 0 );
append( sb, "skip (Default: false)", 2 );
append( sb, "Skip the CPD report generation. Most useful on the command line via \'-Dcpd.skip=true\'.", 3 );
append( sb, "", 0 );
append( sb, "skippedProjects", 2 );
append( sb, "List of the excluded projects, using \',\' as delimiter. C# files of these projects will be ignored.", 3 );
append( sb, "", 0 );
append( sb, "solutionName", 2 );
append( sb, "The name of the solution to use in case there are multiple solutions in the folder AND none has the same name as the artifact with a \'.sln\' extension.", 3 );
append( sb, "", 0 );
append( sb, "sourceEncoding (Default: ${project.build.sourceEncoding})", 2 );
append( sb, "The file encoding to use when reading the Java sources.", 3 );
append( sb, "", 0 );
append( sb, "testProjectPattern (Default: *.Tests)", 2 );
append( sb, "A pattern that will help to figure out if a project is a test project from its name. The \'*\' and \'?\' common jokers are accepted. Several patterns may be specified using \';\' as a delimiter.", 3 );
append( sb, "", 0 );
append( sb, "traceCommands", 2 );
append( sb, "Defines if the launched commands should be appended into a \'command.txt\' file. For debug purpose.", 3 );
append( sb, "", 0 );
append( sb, "useEmbbededRuntime (Default: true)", 2 );
append( sb, "Defines if the plugin can use the maven-dotnet-runtime artifact to export the relevant .Net quality applications instead of using their global path. The defined path is always taken in priority.", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "fxcop".equals( goal ) )
{
append( sb, "dotnet:fxcop", 0 );
append( sb, "Generates a quality report for a .Net project or solution using FxCop", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "assemblyDirectories", 2 );
append( sb, "Locations of the assemblies that should be analysed in case default location should not be used. Actually AOP tools such as PostSharp mess up the cil and may provoke false positives. Project names should be used as keys. Values should be the path to the wanted DLLs. If the assembly of the \'Cache\' project is located in the \'compile\' directory instead of usual \'bin/Debug\', you can add in the configuration section of the plugin something like compile ", 3 );
append( sb, "", 0 );
append( sb, "buildConfigurations (Default: Debug)", 2 );
append( sb, "The build configurations to use for the project or solution, separated by colons or semi-colons as in \'Debug,Release\'. Default value is \'Debug\'. If value is \'ALL\', all the build configurations defined in the sln file will be used.", 3 );
append( sb, "", 0 );
append( sb, "fxCopAdditionalDirectoryPaths", 2 );
append( sb, "Specifies additional directories to search for assembly dependencies. Useful in particular when assembly bindings have been used. \';\' is used as a delimiter between paths.", 3 );
append( sb, "", 0 );
append( sb, "fxCopConfigPath", 2 );
append( sb, "Name of the FxCop configuration that contains the rules to use", 3 );
append( sb, "", 0 );
append( sb, "fxCopDirectoryPath", 2 );
append( sb, "Location of the FxCop installation", 3 );
append( sb, "", 0 );
append( sb, "fxCopExecutable (Default: FxCopCmd.exe)", 2 );
append( sb, "Name of the FxCop command line executable.", 3 );
append( sb, "", 0 );
append( sb, "fxCopReportName (Default: fxcop-report.xml)", 2 );
append( sb, "Name of the FxCop report file", 3 );
append( sb, "", 0 );
append( sb, "generatePdb (Default: true)", 2 );
append( sb, "Defines if the build should generate debug symbols (typically .pdb files)", 3 );
append( sb, "", 0 );
append( sb, "ignoreGeneratedCode (Default: true)", 2 );
append( sb, "Enable/disable the ignore generated code option", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "Location of the output files", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "The maven project.", 3 );
append( sb, "", 0 );
append( sb, "projectName", 2 );
append( sb, "The name of the project to use if it doesn\'t have the same name as the artifact with a \'.csproj\' extension.", 3 );
append( sb, "", 0 );
append( sb, "silverlight_3_MscorlibLocation", 2 );
append( sb, "Location of the dotnet mscorlib.dll file to use when analyzing silverlight3 projects", 3 );
append( sb, "", 0 );
append( sb, "silverlight_4_MscorlibLocation", 2 );
append( sb, "Location of the dotnet mscorlib.dll file to use when analyzing silverlight4 projects", 3 );
append( sb, "", 0 );
append( sb, "silverlightFxCopReportName (Default: silverlight-fxcop-report.xml)", 2 );
append( sb, "Name of the FxCop report file for the silverlight projects", 3 );
append( sb, "", 0 );
append( sb, "silverlightVersion (Default: 4)", 2 );
append( sb, "Version of Silverlight used in the analysed solution Possible values are 3 and 4", 3 );
append( sb, "", 0 );
append( sb, "skippedProjects", 2 );
append( sb, "List of the excluded projects, using \',\' as delimiter. C# files of these projects will be ignored. No violation on files of these projects should be reported.", 3 );
append( sb, "", 0 );
append( sb, "solutionName", 2 );
append( sb, "The name of the solution to use in case there are multiple solutions in the folder AND none has the same name as the artifact with a \'.sln\' extension.", 3 );
append( sb, "", 0 );
append( sb, "testProjectPattern (Default: *.Tests)", 2 );
append( sb, "A pattern that will help to figure out if a project is a test project from its name. The \'*\' and \'?\' common jokers are accepted. Several patterns may be specified using \';\' as a delimiter.", 3 );
append( sb, "", 0 );
append( sb, "traceCommands", 2 );
append( sb, "Defines if the launched commands should be appended into a \'command.txt\' file. For debug purpose.", 3 );
append( sb, "", 0 );
append( sb, "useEmbbededRuntime (Default: true)", 2 );
append( sb, "Defines if the plugin can use the maven-dotnet-runtime artifact to export the relevant .Net quality applications instead of using their global path. The defined path is always taken in priority.", 3 );
append( sb, "", 0 );
append( sb, "verbose", 2 );
append( sb, "Enable/disable the verbose mode", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "gendarme".equals( goal ) )
{
append( sb, "dotnet:gendarme", 0 );
append( sb, "Generates a quality report for a .Net project or solution using mono gendarme", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "assemblyDirectories", 2 );
append( sb, "Locations of the assemblies that should be analysed in case default location should not be used. Actually AOP tools such as PostSharp mess up the cil and may provoke false positives. Project names should be used as keys. Values should be the path to the wanted DLLs. If the assembly of the \'Cache\' project is located in the \'compile\' directory instead of usual \'bin/Debug\', you can add in the configuration section of the plugin something like compile ", 3 );
append( sb, "", 0 );
append( sb, "buildConfigurations (Default: Debug)", 2 );
append( sb, "The build configurations to use for the project or solution, separated by colons or semi-colons as in \'Debug,Release\'. Default value is \'Debug\'. If value is \'ALL\', all the build configurations defined in the sln file will be used.", 3 );
append( sb, "", 0 );
append( sb, "confidence (Default: normal+)", 2 );
append( sb, "Filter defects for the specified confidence levels. confidence [all | [[low | normal | high | total][+|-]],...", 3 );
append( sb, "", 0 );
append( sb, "gendarmeConfigFile", 2 );
append( sb, "Path to the gendarme config file that specifies rule settings", 3 );
append( sb, "", 0 );
append( sb, "gendarmeDirectoryPath", 2 );
append( sb, "Location of the mono gendarme installation", 3 );
append( sb, "", 0 );
append( sb, "gendarmeExecutable (Default: gendarme.exe)", 2 );
append( sb, "Name of the mono gendarme command line executable.", 3 );
append( sb, "", 0 );
append( sb, "gendarmeIgnoreFile", 2 );
append( sb, "Path to the gendarme ignore config file", 3 );
append( sb, "", 0 );
append( sb, "gendarmeReportName (Default: gendarme-report-{0}.xml)", 2 );
append( sb, "Pattern name of the gendarme report files. One file per project of the solution. The pattern syntax is the good old java.text.MessageFormat one. {0} is the project/assembly name.", 3 );
append( sb, "", 0 );
append( sb, "generatePdb (Default: true)", 2 );
append( sb, "Defines if the build should generate debug symbols (typically .pdb files)", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "Location of the output files", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "The maven project.", 3 );
append( sb, "", 0 );
append( sb, "projectName", 2 );
append( sb, "The name of the project to use if it doesn\'t have the same name as the artifact with a \'.csproj\' extension.", 3 );
append( sb, "", 0 );
append( sb, "severity (Default: all)", 2 );
append( sb, "Filter defects for the specified severity levels. severity [all | [[audit | low | medium | high | critical][+|-]]],...", 3 );
append( sb, "", 0 );
append( sb, "silverlight_3_MscorlibLocation", 2 );
append( sb, "Location of the dotnet mscorlib.dll file to use when analyzing silverlight3 projects", 3 );
append( sb, "", 0 );
append( sb, "silverlight_4_MscorlibLocation", 2 );
append( sb, "Location of the dotnet mscorlib.dll file to use when analyzing silverlight4 projects", 3 );
append( sb, "", 0 );
append( sb, "silverlightVersion (Default: 4)", 2 );
append( sb, "Version of Silverlight used in the analysed solution Possible values are 3 and 4", 3 );
append( sb, "", 0 );
append( sb, "skippedProjects", 2 );
append( sb, "List of the excluded projects, using \',\' as delimiter. C# files of these projects will be ignored. No violation on files of these projects should be reported.", 3 );
append( sb, "", 0 );
append( sb, "solutionName", 2 );
append( sb, "The name of the solution to use in case there are multiple solutions in the folder AND none has the same name as the artifact with a \'.sln\' extension.", 3 );
append( sb, "", 0 );
append( sb, "testProjectPattern (Default: *.Tests)", 2 );
append( sb, "A pattern that will help to figure out if a project is a test project from its name. The \'*\' and \'?\' common jokers are accepted. Several patterns may be specified using \';\' as a delimiter.", 3 );
append( sb, "", 0 );
append( sb, "traceCommands", 2 );
append( sb, "Defines if the launched commands should be appended into a \'command.txt\' file. For debug purpose.", 3 );
append( sb, "", 0 );
append( sb, "useEmbbededRuntime (Default: true)", 2 );
append( sb, "Defines if the plugin can use the maven-dotnet-runtime artifact to export the relevant .Net quality applications instead of using their global path. The defined path is always taken in priority.", 3 );
append( sb, "", 0 );
append( sb, "verbose", 2 );
append( sb, "Enable/disable the verbose mode", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "help".equals( goal ) )
{
append( sb, "dotnet:help", 0 );
append( sb, "Display help information on maven-dotnet-plugin.\nCall\n\u00a0\u00a0mvn\u00a0dotnet:help\u00a0-Ddetail=true\u00a0-Dgoal=\nto display parameter details.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "detail (Default: false)", 2 );
append( sb, "If true, display all settable properties for each goal.", 3 );
append( sb, "", 0 );
append( sb, "goal", 2 );
append( sb, "The name of the goal for which to show help. If unspecified, all goals will be displayed.", 3 );
append( sb, "", 0 );
append( sb, "indentSize (Default: 2)", 2 );
append( sb, "The number of spaces per indentation level, should be positive.", 3 );
append( sb, "", 0 );
append( sb, "lineLength (Default: 80)", 2 );
append( sb, "The maximum length of a display line, should be positive.", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "metrics".equals( goal ) )
{
append( sb, "dotnet:metrics", 0 );
append( sb, "Generates the metrics for a C# project or solution using SourceMonitor", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "assemblyDirectories", 2 );
append( sb, "Locations of the assemblies that should be analysed in case default location should not be used. Actually AOP tools such as PostSharp mess up the cil and may provoke false positives. Project names should be used as keys. Values should be the path to the wanted DLLs. If the assembly of the \'Cache\' project is located in the \'compile\' directory instead of usual \'bin/Debug\', you can add in the configuration section of the plugin something like compile ", 3 );
append( sb, "", 0 );
append( sb, "buildConfigurations (Default: Debug)", 2 );
append( sb, "The build configurations to use for the project or solution, separated by colons or semi-colons as in \'Debug,Release\'. Default value is \'Debug\'. If value is \'ALL\', all the build configurations defined in the sln file will be used.", 3 );
append( sb, "", 0 );
append( sb, "excludedExtensions", 2 );
append( sb, "List of the excluded extensions for the metrics. For example \'*.Designer.cs\' which is the default value", 3 );
append( sb, "", 0 );
append( sb, "excludedSubTree", 2 );
append( sb, "List of the sub-trees of the project to excluded from the analysis", 3 );
append( sb, "", 0 );
append( sb, "generatePdb (Default: true)", 2 );
append( sb, "Defines if the build should generate debug symbols (typically .pdb files)", 3 );
append( sb, "", 0 );
append( sb, "metricsReportFileName (Default: metrics-report.xml)", 2 );
append( sb, "Name of the generated metrics report.", 3 );
append( sb, "", 0 );
append( sb, "metricsSrcDirectory (Default: ${project.basedir})", 2 );
append( sb, "Root directory of the solution. SourceMonitor will analyse C# source files located in this directory and sub directories.", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "Location of the output files", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "The maven project.", 3 );
append( sb, "", 0 );
append( sb, "projectName", 2 );
append( sb, "The name of the project to use if it doesn\'t have the same name as the artifact with a \'.csproj\' extension.", 3 );
append( sb, "", 0 );
append( sb, "skippedProjects", 2 );
append( sb, "List of the excluded projects, using \',\' as delimiter. C# files of these projects will be ignored. No violation on files of these projects should be reported.", 3 );
append( sb, "", 0 );
append( sb, "solutionName", 2 );
append( sb, "The name of the solution to use in case there are multiple solutions in the folder AND none has the same name as the artifact with a \'.sln\' extension.", 3 );
append( sb, "", 0 );
append( sb, "sourceMonitorDirectory", 2 );
append( sb, "Installation directory of the SourceMonitor application", 3 );
append( sb, "", 0 );
append( sb, "sourceMonitorExecutable (Default: SourceMonitor.exe)", 2 );
append( sb, "Simple name of the Source Monitor executable", 3 );
append( sb, "", 0 );
append( sb, "testProjectPattern (Default: *.Tests)", 2 );
append( sb, "A pattern that will help to figure out if a project is a test project from its name. The \'*\' and \'?\' common jokers are accepted. Several patterns may be specified using \';\' as a delimiter.", 3 );
append( sb, "", 0 );
append( sb, "traceCommands", 2 );
append( sb, "Defines if the launched commands should be appended into a \'command.txt\' file. For debug purpose.", 3 );
append( sb, "", 0 );
append( sb, "useEmbbededRuntime (Default: true)", 2 );
append( sb, "Defines if the plugin can use the maven-dotnet-runtime artifact to export the relevant .Net quality applications instead of using their global path. The defined path is always taken in priority.", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "msbuild".equals( goal ) )
{
append( sb, "dotnet:msbuild", 0 );
append( sb, "Generic MsBuild mojo. Any msbuild script file with its parameters may be set up from maven, the execution may be attached to any maven phase.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "assemblyDirectories", 2 );
append( sb, "Locations of the assemblies that should be analysed in case default location should not be used. Actually AOP tools such as PostSharp mess up the cil and may provoke false positives. Project names should be used as keys. Values should be the path to the wanted DLLs. If the assembly of the \'Cache\' project is located in the \'compile\' directory instead of usual \'bin/Debug\', you can add in the configuration section of the plugin something like compile ", 3 );
append( sb, "", 0 );
append( sb, "buildConfigurations (Default: Debug)", 2 );
append( sb, "The build configurations to use for the project or solution, separated by colons or semi-colons as in \'Debug,Release\'. Default value is \'Debug\'. If value is \'ALL\', all the build configurations defined in the sln file will be used.", 3 );
append( sb, "", 0 );
append( sb, "dotnet_2_0_sdk_directory (Default: C:/WINDOWS/Microsoft.NET/Framework/v2.0.50727)", 2 );
append( sb, "Installation directory of the .Net SDK 2.0", 3 );
append( sb, "", 0 );
append( sb, "dotnet_3_5_sdk_directory (Default: C:/WINDOWS/Microsoft.NET/Framework/v3.5)", 2 );
append( sb, "Installation directory of the .Net SDK 3.5", 3 );
append( sb, "", 0 );
append( sb, "dotnet_4_0_sdk_directory (Default: C:/WINDOWS/Microsoft.NET/Framework/v4.0.30319)", 2 );
append( sb, "Installation directory of the .Net SDK 4.0", 3 );
append( sb, "", 0 );
append( sb, "generatePdb (Default: true)", 2 );
append( sb, "Defines if the build should generate debug symbols (typically .pdb files)", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "Location of the output files", 3 );
append( sb, "", 0 );
append( sb, "parallelBuild (Default: false)", 2 );
append( sb, "Flag that indicates if parallel builds are activated. When equals to true, \'/m\' MsBuild option is added to the command line and MsBuild will try to use all available cpu cores.", 3 );
append( sb, "", 0 );
append( sb, "parameters", 2 );
append( sb, "Command line parameters for MsBuild", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "The maven project.", 3 );
append( sb, "", 0 );
append( sb, "projectName", 2 );
append( sb, "The name of the project to use if it doesn\'t have the same name as the artifact with a \'.csproj\' extension.", 3 );
append( sb, "", 0 );
append( sb, "script", 2 );
append( sb, "MsBuild script file to execute.", 3 );
append( sb, "", 0 );
append( sb, "skippedProjects", 2 );
append( sb, "List of the excluded projects, using \',\' as delimiter. C# files of these projects will be ignored. No violation on files of these projects should be reported.", 3 );
append( sb, "", 0 );
append( sb, "solutionName", 2 );
append( sb, "The name of the solution to use in case there are multiple solutions in the folder AND none has the same name as the artifact with a \'.sln\' extension.", 3 );
append( sb, "", 0 );
append( sb, "target", 2 );
append( sb, "MsBuild target (optional). If no target specified, the default target of the msbuild script will be executed.", 3 );
append( sb, "", 0 );
append( sb, "testProjectPattern (Default: *.Tests)", 2 );
append( sb, "A pattern that will help to figure out if a project is a test project from its name. The \'*\' and \'?\' common jokers are accepted. Several patterns may be specified using \';\' as a delimiter.", 3 );
append( sb, "", 0 );
append( sb, "toolVersion (Default: 3.5)", 2 );
append( sb, "Version of the MSBuild tool to use, which is the installed version of the SDK. Possible values are 2.0, 3.5 and 4.0.", 3 );
append( sb, "", 0 );
append( sb, "traceCommands", 2 );
append( sb, "Defines if the launched commands should be appended into a \'command.txt\' file. For debug purpose.", 3 );
append( sb, "", 0 );
append( sb, "useEmbbededRuntime (Default: true)", 2 );
append( sb, "Defines if the plugin can use the maven-dotnet-runtime artifact to export the relevant .Net quality applications instead of using their global path. The defined path is always taken in priority.", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "package".equals( goal ) )
{
append( sb, "dotnet:package", 0 );
append( sb, "Package in distributable format SLN (zip)", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "assemblyDirectories", 2 );
append( sb, "Locations of the assemblies that should be analysed in case default location should not be used. Actually AOP tools such as PostSharp mess up the cil and may provoke false positives. Project names should be used as keys. Values should be the path to the wanted DLLs. If the assembly of the \'Cache\' project is located in the \'compile\' directory instead of usual \'bin/Debug\', you can add in the configuration section of the plugin something like compile ", 3 );
append( sb, "", 0 );
append( sb, "buildConfigurations (Default: Debug)", 2 );
append( sb, "The build configurations to use for the project or solution, separated by colons or semi-colons as in \'Debug,Release\'. Default value is \'Debug\'. If value is \'ALL\', all the build configurations defined in the sln file will be used.", 3 );
append( sb, "", 0 );
append( sb, "generatePdb (Default: true)", 2 );
append( sb, "Defines if the build should generate debug symbols (typically .pdb files)", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "Location of the output files", 3 );
append( sb, "", 0 );
append( sb, "packFiles", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "The maven project.", 3 );
append( sb, "", 0 );
append( sb, "projectName", 2 );
append( sb, "The name of the project to use if it doesn\'t have the same name as the artifact with a \'.csproj\' extension.", 3 );
append( sb, "", 0 );
append( sb, "skippedProjects", 2 );
append( sb, "List of the excluded projects, using \',\' as delimiter. C# files of these projects will be ignored. No violation on files of these projects should be reported.", 3 );
append( sb, "", 0 );
append( sb, "solutionName", 2 );
append( sb, "The name of the solution to use in case there are multiple solutions in the folder AND none has the same name as the artifact with a \'.sln\' extension.", 3 );
append( sb, "", 0 );
append( sb, "testProjectPattern (Default: *.Tests)", 2 );
append( sb, "A pattern that will help to figure out if a project is a test project from its name. The \'*\' and \'?\' common jokers are accepted. Several patterns may be specified using \';\' as a delimiter.", 3 );
append( sb, "", 0 );
append( sb, "traceCommands", 2 );
append( sb, "Defines if the launched commands should be appended into a \'command.txt\' file. For debug purpose.", 3 );
append( sb, "", 0 );
append( sb, "useEmbbededRuntime (Default: true)", 2 );
append( sb, "Defines if the plugin can use the maven-dotnet-runtime artifact to export the relevant .Net quality applications instead of using their global path. The defined path is always taken in priority.", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "stylecop".equals( goal ) )
{
append( sb, "dotnet:stylecop", 0 );
append( sb, "Generates a quality report for a .Net project or solution using StyleCop", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "assemblyDirectories", 2 );
append( sb, "Locations of the assemblies that should be analysed in case default location should not be used. Actually AOP tools such as PostSharp mess up the cil and may provoke false positives. Project names should be used as keys. Values should be the path to the wanted DLLs. If the assembly of the \'Cache\' project is located in the \'compile\' directory instead of usual \'bin/Debug\', you can add in the configuration section of the plugin something like compile ", 3 );
append( sb, "", 0 );
append( sb, "buildConfigurations (Default: Debug)", 2 );
append( sb, "The build configurations to use for the project or solution, separated by colons or semi-colons as in \'Debug,Release\'. Default value is \'Debug\'. If value is \'ALL\', all the build configurations defined in the sln file will be used.", 3 );
append( sb, "", 0 );
append( sb, "dotnet_2_0_sdk_directory (Default: C:/WINDOWS/Microsoft.NET/Framework/v2.0.50727)", 2 );
append( sb, "Installation directory of the .Net SDK 2.0", 3 );
append( sb, "", 0 );
append( sb, "dotnet_3_5_sdk_directory (Default: C:/WINDOWS/Microsoft.NET/Framework/v3.5)", 2 );
append( sb, "Installation directory of the .Net SDK 3.5", 3 );
append( sb, "", 0 );
append( sb, "dotnet_4_0_sdk_directory (Default: C:/WINDOWS/Microsoft.NET/Framework/v4.0.30319)", 2 );
append( sb, "Installation directory of the .Net SDK 4.0", 3 );
append( sb, "", 0 );
append( sb, "generatePdb (Default: true)", 2 );
append( sb, "Defines if the build should generate debug symbols (typically .pdb files)", 3 );
append( sb, "", 0 );
append( sb, "ignores", 2 );
append( sb, "Patterns for ignored files", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "Location of the output files", 3 );
append( sb, "", 0 );
append( sb, "parallelBuild (Default: false)", 2 );
append( sb, "Flag that indicates if parallel builds are activated. When equals to true, \'/m\' MsBuild option is added to the command line and MsBuild will try to use all available cpu cores.", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "The maven project.", 3 );
append( sb, "", 0 );
append( sb, "projectName", 2 );
append( sb, "The name of the project to use if it doesn\'t have the same name as the artifact with a \'.csproj\' extension.", 3 );
append( sb, "", 0 );
append( sb, "projectRoot (Default: ${project.basedir})", 2 );
append( sb, "Root directory of the solution. Stylecop will analyse C# source files located in this directory and sub directories.", 3 );
append( sb, "", 0 );
append( sb, "skippedProjects", 2 );
append( sb, "List of the excluded projects, using \',\' as delimiter. C# files of these projects will be ignored. No violation on files of these projects should be reported.", 3 );
append( sb, "", 0 );
append( sb, "solutionName", 2 );
append( sb, "The name of the solution to use in case there are multiple solutions in the folder AND none has the same name as the artifact with a \'.sln\' extension.", 3 );
append( sb, "", 0 );
append( sb, "styleCopConfigPath", 2 );
append( sb, "Name of the file that contains the StyleCop rules to use", 3 );
append( sb, "", 0 );
append( sb, "styleCopReportName (Default: stylecop-report.xml)", 2 );
append( sb, "Name of the generated StyleCop report", 3 );
append( sb, "", 0 );
append( sb, "styleCopRootPath", 2 );
append( sb, "Name of the folder that will contain the stylecop installation", 3 );
append( sb, "", 0 );
append( sb, "testProjectPattern (Default: *.Tests)", 2 );
append( sb, "A pattern that will help to figure out if a project is a test project from its name. The \'*\' and \'?\' common jokers are accepted. Several patterns may be specified using \';\' as a delimiter.", 3 );
append( sb, "", 0 );
append( sb, "toolVersion (Default: 3.5)", 2 );
append( sb, "Version of the MSBuild tool to use, which is the installed version of the SDK. Possible values are 2.0, 3.5 and 4.0.", 3 );
append( sb, "", 0 );
append( sb, "traceCommands", 2 );
append( sb, "Defines if the launched commands should be appended into a \'command.txt\' file. For debug purpose.", 3 );
append( sb, "", 0 );
append( sb, "useEmbbededRuntime (Default: true)", 2 );
append( sb, "Defines if the plugin can use the maven-dotnet-runtime artifact to export the relevant .Net quality applications instead of using their global path. The defined path is always taken in priority.", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "test".equals( goal ) )
{
append( sb, "dotnet:test", 0 );
append( sb, "Launches the unit tests for a .Net solution or a test project using Gallio", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "assemblyDirectories", 2 );
append( sb, "Locations of the assemblies that should be analysed in case default location should not be used. Actually AOP tools such as PostSharp mess up the cil and may provoke false positives. Project names should be used as keys. Values should be the path to the wanted DLLs. If the assembly of the \'Cache\' project is located in the \'compile\' directory instead of usual \'bin/Debug\', you can add in the configuration section of the plugin something like compile ", 3 );
append( sb, "", 0 );
append( sb, "buildConfigurations (Default: Debug)", 2 );
append( sb, "The build configurations to use for the project or solution, separated by colons or semi-colons as in \'Debug,Release\'. Default value is \'Debug\'. If value is \'ALL\', all the build configurations defined in the sln file will be used.", 3 );
append( sb, "", 0 );
append( sb, "filter", 2 );
append( sb, "Optional test filter for gallio. This can be used to execute only a specific test category (i.e. CategotyName:unit to consider only tests from the \'unit\' category)", 3 );
append( sb, "", 0 );
append( sb, "gallioDirectory", 2 );
append( sb, "Location of the Gallio installation", 3 );
append( sb, "", 0 );
append( sb, "gallioExecutable (Default: Gallio.Echo.exe)", 2 );
append( sb, "Name of the Gallio executable file", 3 );
append( sb, "", 0 );
append( sb, "gallioRunner (Default: IsolatedProcess)", 2 );
append( sb, "Name of the Gallio runner to use", 3 );
append( sb, "", 0 );
append( sb, "generatePdb (Default: true)", 2 );
append( sb, "Defines if the build should generate debug symbols (typically .pdb files)", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "Location of the output files", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "The maven project.", 3 );
append( sb, "", 0 );
append( sb, "projectName", 2 );
append( sb, "The name of the project to use if it doesn\'t have the same name as the artifact with a \'.csproj\' extension.", 3 );
append( sb, "", 0 );
append( sb, "reportFileName (Default: gallio-report.xml)", 2 );
append( sb, "The output file for Gallio.", 3 );
append( sb, "", 0 );
append( sb, "skip", 2 );
append( sb, "Set this to \'true\' to bypass unit tests entirely. Its use is NOT RECOMMENDED, especially if you enable it using the \'maven.test.skip\' property, because maven.test.skip disables both running the tests and compiling the tests. Consider using the skipTests parameter instead.", 3 );
append( sb, "", 0 );
append( sb, "skippedProjects", 2 );
append( sb, "List of the excluded projects, using \',\' as delimiter. C# files of these projects will be ignored. No violation on files of these projects should be reported.", 3 );
append( sb, "", 0 );
append( sb, "skipTests", 2 );
append( sb, "Set this to \'true\' to skip running tests, but still compile them. Its use is NOT RECOMMENDED, but quite convenient on occasion.", 3 );
append( sb, "", 0 );
append( sb, "solutionName", 2 );
append( sb, "The name of the solution to use in case there are multiple solutions in the folder AND none has the same name as the artifact with a \'.sln\' extension.", 3 );
append( sb, "", 0 );
append( sb, "testFailureIgnore (Default: false)", 2 );
append( sb, "Set this to true to ignore a failure during testing. Its use is NOT RECOMMENDED, but quite convenient on occasion.", 3 );
append( sb, "", 0 );
append( sb, "testProjectPattern (Default: *.Tests)", 2 );
append( sb, "A pattern that will help to figure out if a project is a test project from its name. The \'*\' and \'?\' common jokers are accepted. Several patterns may be specified using \';\' as a delimiter.", 3 );
append( sb, "", 0 );
append( sb, "traceCommands", 2 );
append( sb, "Defines if the launched commands should be appended into a \'command.txt\' file. For debug purpose.", 3 );
append( sb, "", 0 );
append( sb, "useEmbbededRuntime (Default: true)", 2 );
append( sb, "Defines if the plugin can use the maven-dotnet-runtime artifact to export the relevant .Net quality applications instead of using their global path. The defined path is always taken in priority.", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "unpack".equals( goal ) )
{
append( sb, "dotnet:unpack", 0 );
append( sb, "Download dependencies and unpack format SLN (zip)", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "assemblyDirectories", 2 );
append( sb, "Locations of the assemblies that should be analysed in case default location should not be used. Actually AOP tools such as PostSharp mess up the cil and may provoke false positives. Project names should be used as keys. Values should be the path to the wanted DLLs. If the assembly of the \'Cache\' project is located in the \'compile\' directory instead of usual \'bin/Debug\', you can add in the configuration section of the plugin something like compile ", 3 );
append( sb, "", 0 );
append( sb, "buildConfigurations (Default: Debug)", 2 );
append( sb, "The build configurations to use for the project or solution, separated by colons or semi-colons as in \'Debug,Release\'. Default value is \'Debug\'. If value is \'ALL\', all the build configurations defined in the sln file will be used.", 3 );
append( sb, "", 0 );
append( sb, "csprojvars", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "generatePdb (Default: true)", 2 );
append( sb, "Defines if the build should generate debug symbols (typically .pdb files)", 3 );
append( sb, "", 0 );
append( sb, "localRepository (Default: ${localRepository})", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "offset", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "Location of the output files", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "The maven project.", 3 );
append( sb, "", 0 );
append( sb, "projectName", 2 );
append( sb, "The name of the project to use if it doesn\'t have the same name as the artifact with a \'.csproj\' extension.", 3 );
append( sb, "", 0 );
append( sb, "skippedProjects", 2 );
append( sb, "List of the excluded projects, using \',\' as delimiter. C# files of these projects will be ignored. No violation on files of these projects should be reported.", 3 );
append( sb, "", 0 );
append( sb, "solutionName", 2 );
append( sb, "The name of the solution to use in case there are multiple solutions in the folder AND none has the same name as the artifact with a \'.sln\' extension.", 3 );
append( sb, "", 0 );
append( sb, "testProjectPattern (Default: *.Tests)", 2 );
append( sb, "A pattern that will help to figure out if a project is a test project from its name. The \'*\' and \'?\' common jokers are accepted. Several patterns may be specified using \';\' as a delimiter.", 3 );
append( sb, "", 0 );
append( sb, "traceCommands", 2 );
append( sb, "Defines if the launched commands should be appended into a \'command.txt\' file. For debug purpose.", 3 );
append( sb, "", 0 );
append( sb, "unPackRoot", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "useEmbbededRuntime (Default: true)", 2 );
append( sb, "Defines if the plugin can use the maven-dotnet-runtime artifact to export the relevant .Net quality applications instead of using their global path. The defined path is always taken in priority.", 3 );
append( sb, "", 0 );
}
}
if ( getLog().isInfoEnabled() )
{
getLog().info( sb.toString() );
}
}
/**
* Repeat a String n
times to form a new string.
*
* @param str String to repeat
* @param repeat number of times to repeat str
* @return String with repeated String
* @throws NegativeArraySizeException if repeat < 0
* @throws NullPointerException if str is null
*/
private static String repeat( String str, int repeat )
{
StringBuffer buffer = new StringBuffer( repeat * str.length() );
for ( int i = 0; i < repeat; i++ )
{
buffer.append( str );
}
return buffer.toString();
}
/**
* Append a description to the buffer by respecting the indentSize and lineLength parameters.
* Note: The last character is always a new line.
*
* @param sb The buffer to append the description, not null
.
* @param description The description, not null
.
* @param indent The base indentation level of each line, must not be negative.
*/
private void append( StringBuffer sb, String description, int indent )
{
for ( Iterator it = toLines( description, indent, indentSize, lineLength ).iterator(); it.hasNext(); )
{
sb.append( it.next().toString() ).append( '\n' );
}
}
/**
* Splits the specified text into lines of convenient display length.
*
* @param text The text to split into lines, must not be null
.
* @param indent The base indentation level of each line, must not be negative.
* @param indentSize The size of each indentation, must not be negative.
* @param lineLength The length of the line, must not be negative.
* @return The sequence of display lines, never null
.
* @throws NegativeArraySizeException if indent < 0
*/
private static List toLines( String text, int indent, int indentSize, int lineLength )
{
List lines = new ArrayList();
String ind = repeat( "\t", indent );
String[] plainLines = text.split( "(\r\n)|(\r)|(\n)" );
for ( int i = 0; i < plainLines.length; i++ )
{
toLines( lines, ind + plainLines[i], indentSize, lineLength );
}
return lines;
}
/**
* Adds the specified line to the output sequence, performing line wrapping if necessary.
*
* @param lines The sequence of display lines, must not be null
.
* @param line The line to add, must not be null
.
* @param indentSize The size of each indentation, must not be negative.
* @param lineLength The length of the line, must not be negative.
*/
private static void toLines( List lines, String line, int indentSize, int lineLength )
{
int lineIndent = getIndentLevel( line );
StringBuffer buf = new StringBuffer( 256 );
String[] tokens = line.split( " +" );
for ( int i = 0; i < tokens.length; i++ )
{
String token = tokens[i];
if ( i > 0 )
{
if ( buf.length() + token.length() >= lineLength )
{
lines.add( buf.toString() );
buf.setLength( 0 );
buf.append( repeat( " ", lineIndent * indentSize ) );
}
else
{
buf.append( ' ' );
}
}
for ( int j = 0; j < token.length(); j++ )
{
char c = token.charAt( j );
if ( c == '\t' )
{
buf.append( repeat( " ", indentSize - buf.length() % indentSize ) );
}
else if ( c == '\u00A0' )
{
buf.append( ' ' );
}
else
{
buf.append( c );
}
}
}
lines.add( buf.toString() );
}
/**
* Gets the indentation level of the specified line.
*
* @param line The line whose indentation level should be retrieved, must not be null
.
* @return The indentation level of the line.
*/
private static int getIndentLevel( String line )
{
int level = 0;
for ( int i = 0; i < line.length() && line.charAt( i ) == '\t'; i++ )
{
level++;
}
for ( int i = level + 1; i <= level + 4 && i < line.length(); i++ )
{
if ( line.charAt( i ) == '\t' )
{
level++;
break;
}
}
return level;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy