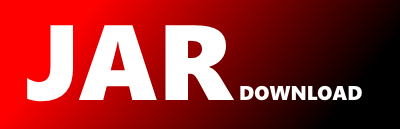
org.sonar.plugins.dotnet.api.microsoft.VisualStudioWebProject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sonar-dotnet-plugin Show documentation
Show all versions of sonar-dotnet-plugin Show documentation
Plugin that defines the core APIs for the .NET Ecosystem.
/*
* Sonar .NET Plugin :: Core
* Copyright (C) 2010 Jose Chillan, Alexandre Victoor and SonarSource
* [email protected]
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02
*/
package org.sonar.plugins.dotnet.api.microsoft;
import org.apache.commons.lang.StringUtils;
import java.io.File;
import java.util.HashSet;
import java.util.Set;
/**
* Represent a web/asp folder/project Visual Studio handle in a weird way asp projects since there is no csproj files. The description of
* the project is embedded in the sln solution file.
*
* @author Fabrice BELLINGARD
* @author Alexandre Victoor
*/
public class VisualStudioWebProject extends VisualStudioProject {
public VisualStudioWebProject() {
setType(ArtifactType.WEB);
}
/**
* @return the generated web dlls
*
*/
@Override
public Set getGeneratedAssemblies(String buildConfigurations, String buildPlatform) {
Set result = new HashSet();
// we need to exclude all the dll files
// that correspond to references
Set exclusions = new HashSet();
Set references = getReferences();
for (File file : references) {
exclusions.add(file.getName());
}
File precompilationDirectory = getWebPrecompilationDirectory(buildConfigurations, buildPlatform);
if (precompilationDirectory != null && precompilationDirectory.isDirectory()) {
File[] files = precompilationDirectory.listFiles();
for (File file : files) {
String name = file.getName();
if (StringUtils.endsWith(name, "dll") && !exclusions.contains(name)) {
result.add(file);
}
}
}
return result;
}
/**
* @param buildConfigurations
* Visual Studio build configurations used to generate the project
* @return the directory where asp.net pages are precompiled. null for a non web project
*/
public File getWebPrecompilationDirectory(String buildConfigurations, String buildPlatform) {
return new File(getArtifactDirectory(buildConfigurations, buildPlatform), "bin");
}
/**
* @return the generated web dll names otherwise.
*/
public Set getWebAssemblyNames() {
Set assemblies = getGeneratedAssemblies(null, null);
Set assemblyNames = new HashSet();
for (File assembly : assemblies) {
assemblyNames.add(StringUtils.substringBeforeLast(assembly.getName(), ".dll"));
}
return assemblyNames;
}
/**
* @return the dll files that correspond to VS references
*/
public Set getReferences() {
File binDirectory = new File(getDirectory(), "Bin");
Set result = new HashSet();
if (binDirectory.exists()) {
File[] files = binDirectory.listFiles();
for (File file : files) {
String name = file.getName();
if (StringUtils.endsWith(name, "dll")) {
result.add(file);
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy