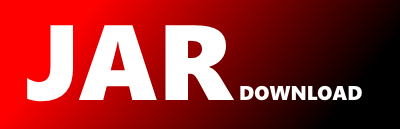
org.sonar.json.parser.JSONGrammar Maven / Gradle / Ivy
/*
* SonarQube JSON Analyzer
* Copyright (C) 2015-2017 David RACODON
* [email protected]
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, write to the Free Software Foundation,
* Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package org.sonar.json.parser;
import com.sonar.sslr.api.typed.GrammarBuilder;
import org.sonar.json.tree.impl.InternalSyntaxToken;
import org.sonar.json.tree.impl.SeparatedList;
import org.sonar.plugins.json.api.tree.*;
public class JSONGrammar {
private final GrammarBuilder b;
private final TreeFactory f;
public JSONGrammar(GrammarBuilder b, TreeFactory f) {
this.b = b;
this.f = f;
}
public JsonTree JSON() {
return b.nonterminal(JSONLexicalGrammar.JSON).is(
f.json(
b.optional(b.token(JSONLexicalGrammar.BOM)),
b.optional(VALUE()),
b.token(JSONLexicalGrammar.EOF)));
}
public ObjectTree OBJECT() {
return b.nonterminal(JSONLexicalGrammar.OBJECT).is(
f.object(
b.token(JSONLexicalGrammar.LEFT_BRACE),
b.optional(PAIR_LIST()),
b.token(JSONLexicalGrammar.RIGHT_BRACE)));
}
public ArrayTree ARRAY() {
return b.nonterminal(JSONLexicalGrammar.ARRAY).is(
f.array(
b.token(JSONLexicalGrammar.LEFT_BRACKET),
b.optional(VALUE_LIST()),
b.token(JSONLexicalGrammar.RIGHT_BRACKET)));
}
public SeparatedList VALUE_LIST() {
return b.>nonterminal().is(
f.valueList(
VALUE(),
b.zeroOrMore(f.newTuple1(b.token(JSONLexicalGrammar.COMMA), VALUE()))));
}
public SeparatedList PAIR_LIST() {
return b.>nonterminal().is(
f.pairList(
PAIR(),
b.zeroOrMore(f.newTuple2(b.token(JSONLexicalGrammar.COMMA), PAIR()))));
}
public PairTree PAIR() {
return b.nonterminal(JSONLexicalGrammar.PAIR).is(
f.pair(
KEY(),
b.token(JSONLexicalGrammar.COLON),
VALUE()));
}
public KeyTree KEY() {
return b.nonterminal(JSONLexicalGrammar.KEY).is(
f.key(b.token(JSONLexicalGrammar.STRING)));
}
public ValueTree VALUE() {
return b.nonterminal(JSONLexicalGrammar.VALUE).is(
f.value(
b.firstOf(
OBJECT(),
ARRAY(),
TRUE(),
FALSE(),
NULL(),
NUMBER(),
STRING())));
}
public StringTree STRING() {
return b.nonterminal().is(
f.string(b.token(JSONLexicalGrammar.STRING)));
}
public LiteralTree NUMBER() {
return b.nonterminal().is(
f.number(b.token(JSONLexicalGrammar.NUMBER)));
}
public LiteralTree FALSE() {
return b.nonterminal().is(
f.falsee(b.token(JSONLexicalGrammar.FALSE)));
}
public LiteralTree TRUE() {
return b.nonterminal().is(
f.truee(b.token(JSONLexicalGrammar.TRUE)));
}
public LiteralTree NULL() {
return b.nonterminal().is(
f.nul(b.token(JSONLexicalGrammar.NULL)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy