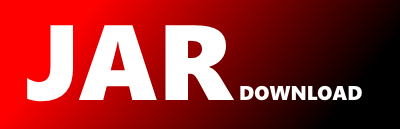
org.sonar.plugins.scmstats.measures.ChangeLogHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sonar-scm-stats-plugin Show documentation
Show all versions of sonar-scm-stats-plugin Show documentation
Feed Sonar with Statistics about SCM system
/*
* Sonar SCM Stats Plugin
* Copyright (C) 2012 Patroklos PAPAPETROU
* [email protected]
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02
*/
package org.sonar.plugins.scmstats.measures;
import com.google.common.annotations.VisibleForTesting;
import java.util.*;
import org.apache.commons.lang.ObjectUtils;
import org.joda.time.DateTime;
import org.sonar.api.batch.SensorContext;
import org.sonar.plugins.scmstats.ScmStatsConstants;
import org.sonar.plugins.scmstats.model.ChangeLogInfo;
import org.sonar.plugins.scmstats.model.CommitsList;
import org.sonar.plugins.scmstats.utils.MapUtils;
public class ChangeLogHandler {
private Map commitsPerUser = new HashMap();
private Map commitsPerClockHour = new HashMap();
private Map commitsPerWeekDay = new HashMap();
private Map commitsPerMonth = new HashMap();
private final List changeLogs = new ArrayList();
public final void addChangeLog(String authorName, Date commitDate, Map fileStatus) {
changeLogs.add(new ChangeLogInfo(authorName, commitDate, fileStatus));
}
public void generateMeasures() {
for (ChangeLogInfo changeLogInfo : changeLogs) {
commitsPerUser = updateAuthorActivity(commitsPerUser, changeLogInfo);
DateTime dt = new DateTime(changeLogInfo.getCommitDate());
commitsPerClockHour = MapUtils.updateMap(commitsPerClockHour, String.format("%2d", dt.getHourOfDay()).replace(' ', '0'));
commitsPerWeekDay = MapUtils.updateMap(commitsPerWeekDay, dt.dayOfWeek().getAsString());
commitsPerMonth = MapUtils.updateMap(commitsPerMonth, String.format("%2d", dt.getMonthOfYear()).replace(' ', '0'));
}
}
public void saveMeasures(SensorContext context,String period) {
PeriodMeasuresCreatorFactory factory = new PeriodMeasuresCreatorFactory();
AbstractPeriodMeasuresCreator measuresCreator =
factory.getPeriodMeasureCreator(context,period);
measuresCreator.getCommitsPerMonthMeasure(commitsPerMonth).save();
measuresCreator.getCommitsPerWeekDayMeasure(commitsPerWeekDay).save();
measuresCreator.getCommitsPerClockHourMeasure(commitsPerClockHour).save();
measuresCreator.getCommitsPerUserMeasure(commitsPerUser).save();
}
@VisibleForTesting
protected Map updateAuthorActivity(
final Map map,
final ChangeLogInfo changeLogInfo) {
final String author = changeLogInfo.getAuthor();
final Map authorActivity = new HashMap();
authorActivity.putAll(map);
final Map activity = changeLogInfo.getActivity();
final List stats;
if (authorActivity.get(author) == null){
stats = getInitialActivity(activity);
} else {
stats = authorActivity.get(author).getCommits();
}
if (authorActivity.containsKey(author)) {
final Integer commits = stats.get(0) + 1;
stats.set(0, commits);
updateActivity( stats, activity, 1, ScmStatsConstants.ACTIVITY_ADD);
updateActivity( stats, activity, 2, ScmStatsConstants.ACTIVITY_MODIFY);
updateActivity( stats, activity, 3, ScmStatsConstants.ACTIVITY_DELETE);
}
authorActivity.put(author, new CommitsList(stats));
return authorActivity;
}
private void updateActivity(final List stats,
final Map activityType,
final int pos,
final String action) {
final Integer actions = stats.get(pos) +
org.apache.commons.collections.MapUtils.getInteger(activityType, action, 0);
stats.set(pos, actions);
}
private List getInitialActivity(final Map activity){
return Arrays.asList(1,
org.apache.commons.collections.MapUtils.getInteger(activity, ScmStatsConstants.ACTIVITY_ADD, 0),
org.apache.commons.collections.MapUtils.getInteger(activity, ScmStatsConstants.ACTIVITY_MODIFY, 0),
org.apache.commons.collections.MapUtils.getInteger(activity, ScmStatsConstants.ACTIVITY_DELETE, 0));
}
public Map getCommitsPerClockHour() {
return commitsPerClockHour;
}
public Map getCommitsPerMonth() {
return commitsPerMonth;
}
public Map getCommitsPerUser() {
return commitsPerUser;
}
public Map getCommitsPerWeekDay() {
return commitsPerWeekDay;
}
public List getChangeLogs(){
return changeLogs;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy