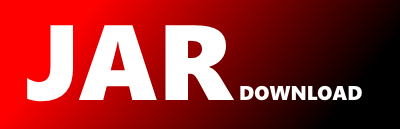
org.sonar.plugins.pmd.PmdMavenCollector Maven / Gradle / Ivy
/*
* Sonar, entreprise quality control tool.
* Copyright (C) 2007-2008 Hortis-GRC SA
* mailto:be_agile HAT hortis DOT ch
*
* Sonar is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* Sonar is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Sonar; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02
*/
package org.sonar.plugins.pmd;
import ch.hortis.sonar.model.Rule;
import ch.hortis.sonar.model.RuleFailureLevel;
import ch.hortis.sonar.model.RuleFailureParam;
import org.apache.maven.project.MavenProject;
import org.sonar.plugins.api.PluginContext;
import org.sonar.plugins.java.AbstractJavaMavenCollector;
import org.sonar.plugins.java.JavaMeasuresRecorder;
import org.sonar.plugins.rules.RulesPluginContext;
import org.sonar.plugins.utils.CollectorUtils;
import org.sonar.plugins.utils.XmlParserException;
import org.sonar.plugins.utils.XmlReportParser;
import org.w3c.dom.Element;
import org.w3c.dom.NodeList;
import java.io.File;
import java.text.ParseException;
import java.util.List;
public class PmdMavenCollector extends AbstractJavaMavenCollector {
private RulesPluginContext rulesPluginContext;
public PmdMavenCollector(JavaMeasuresRecorder measuresRecorder, PluginContext pluginContext) {
super(measuresRecorder);
this.rulesPluginContext = pluginContext.getRulesContext(PmdPlugin.KEY);
}
protected PmdMavenCollector(JavaMeasuresRecorder measuresRecorder, RulesPluginContext rulesPluginContext) {
super(measuresRecorder);
this.rulesPluginContext = rulesPluginContext;
}
public boolean shouldStopOnFailure() {
return true;
}
protected boolean shouldCollectIfNoTests() {
return true;
}
protected boolean shouldCollectIfNoSources() {
return false;
}
public void collect(MavenProject pom) {
List sourceDirs = pom.getCompileSourceRoots();
File reportFile = CollectorUtils.findFileFromBuildDirectory(pom, "pmd.xml");
collect(reportFile, sourceDirs);
}
protected void collect(File reportFile, List sourceDirs) {
XmlReportParser parser = new XmlReportParser();
parser.parse(reportFile);
try {
NodeList files = parser.executeXPathNodeList("/pmd/file");
if (files != null) {
for (int i = 0; i < files.getLength(); i++) {
Element element = (Element) files.item(i);
String pmdName = element.getAttribute("name");
List violations = parser.getChildElements(element, "violation");
for (Element violation : violations) {
String ruleKey = violation.getAttribute("rule");
Rule rule = rulesPluginContext.getRule(ruleKey);
if (rule == null) {
continue;
}
String[] s = CollectorUtils.getJavaPackageAndClass(pmdName, sourceDirs);
if (s == null) {
continue;
}
String message = violation.getFirstChild().getNodeValue();
RuleFailureLevel level = RuleFailureLevel.INFO;
int priority = Integer.parseInt(violation.getAttribute("priority"));
if (priority <= 2) {
level = RuleFailureLevel.ERROR;
} else if (priority == 3 || priority == 4) {
level = RuleFailureLevel.WARNING;
}
RuleFailureParam lineParam = null, columnParam = null;
String line = violation.getAttribute("beginline");
if (line != null && !"".equals(line)) {
lineParam = new RuleFailureParam("line", CollectorUtils.parseNumber(line), null);
}
String column = violation.getAttribute("begincolumn");
if (column != null && !"".equals(column)) {
columnParam = new RuleFailureParam("column", CollectorUtils.parseNumber(column), null);
}
getMeasuresRecorder().createClassRuleFailure(rule, s[0], s[1], false, message, level, lineParam, columnParam);
}
}
}
} catch (ParseException e) {
throw new XmlParserException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy