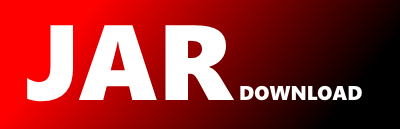
org.sonar.plugins.pmd.PmdMavenPluginHandler Maven / Gradle / Ivy
/*
* Sonar, entreprise quality control tool.
* Copyright (C) 2007-2008 Hortis-GRC SA
* mailto:be_agile HAT hortis DOT ch
*
* Sonar is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* Sonar is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Sonar; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02
*/
package org.sonar.plugins.pmd;
import org.apache.commons.io.FileUtils;
import org.apache.maven.model.Plugin;
import org.apache.maven.project.MavenProject;
import org.codehaus.plexus.util.xml.Xpp3Dom;
import org.sonar.plugins.api.Languages;
import org.sonar.plugins.api.PluginContext;
import org.sonar.plugins.maven.AbstractMavenPluginHandler;
import org.sonar.plugins.maven.MavenUtils;
import org.sonar.plugins.rules.RulesPluginContext;
import java.io.File;
import java.io.IOException;
public class PmdMavenPluginHandler extends AbstractMavenPluginHandler {
private RulesPluginContext rulesPluginContext;
private PmdRulesRepository pmdRulesRepository;
public PmdMavenPluginHandler(PluginContext pluginContext, PmdRulesRepository pmdRulesRepository) {
this.rulesPluginContext = pluginContext.getRulesContext(PmdPlugin.KEY);
this.pmdRulesRepository = pmdRulesRepository;
}
protected PmdMavenPluginHandler(RulesPluginContext rulesPluginContext, PmdRulesRepository pmdRulesRepository) {
this.rulesPluginContext = rulesPluginContext;
this.pmdRulesRepository = pmdRulesRepository;
}
public boolean shouldStopOnFailure() {
return true;
}
public boolean shouldExecuteOn(MavenProject pom) {
return Languages.JAVA.equals(MavenUtils.getLanguage(pom));
}
public String getGroupId() {
return MavenUtils.APACHE_MOJO_GROUP_ID;
}
public String getArtifactId() {
return "maven-pmd-plugin";
}
public String getVersion() {
return "2.3";
}
public boolean isFixedVersion() {
return true;
}
public String[] getGoals() {
return new String[]{"pmd"};
}
public void configurePlugin(MavenProject pom, Plugin plugin) {
unsetConfigParameter(plugin, "outputDirectory");
unsetConfigParameter(plugin, "targetDirectory");
setConfigParameter(plugin, "format", "xml");
setConfigParameter(plugin, "linkXRef", "false");
String javaVersion = MavenUtils.getJavaVersion(pom);
if (javaVersion != null) {
if (javaVersion.equals("1.1") || javaVersion.equals("1.2")) {
setConfigParameter(plugin, "targetJdk", "1.3");
} else {
setConfigParameter(plugin, "targetJdk", javaVersion);
}
}
unsetConfigParameter(plugin, "rulesets");
Xpp3Dom conf = (Xpp3Dom) plugin.getConfiguration();
Xpp3Dom rulesets = new Xpp3Dom("rulesets");
Xpp3Dom ruleset = new Xpp3Dom("ruleset");
ruleset.setValue(pom.getBuild().getDirectory() + "/sonar/pmd.xml");
rulesets.addChild(ruleset);
conf.addChild(rulesets);
MavenUtils.copyPluginDependencies(pom, plugin);
File xmlFile = null;
try {
xmlFile = saveConfigXml(pom);
setConfigParameter(plugin, "configLocation", xmlFile.getCanonicalPath());
} catch (IOException e) {
throw new RuntimeException("fail to save the pmd XML configuration", e);
}
addRuleExtensionsDependency(plugin);
}
private File saveConfigXml(MavenProject pom) throws IOException {
File pmd = new File(getWorkingDirectory(pom), "pmd.xml");
String xml = pmdRulesRepository.exportConfiguration(rulesPluginContext.getActiveProfile());
FileUtils.writeStringToFile(pmd, xml);
return pmd;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy