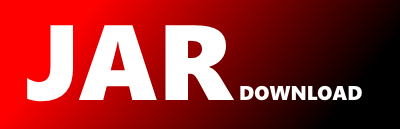
org.sonar.plugins.pmd.PmdRulesRepository Maven / Gradle / Ivy
/*
* Sonar, entreprise quality control tool.
* Copyright (C) 2007-2008 Hortis-GRC SA
* mailto:be_agile HAT hortis DOT ch
*
* Sonar is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* Sonar is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Sonar; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02
*/
package org.sonar.plugins.pmd;
import ch.hortis.sonar.model.ActiveRule;
import ch.hortis.sonar.model.RuleFailureLevel;
import ch.hortis.sonar.model.RulesProfile;
import com.thoughtworks.xstream.XStream;
import org.apache.commons.io.IOUtils;
import org.sonar.commons.rules.ActiveRuleParam;
import org.sonar.commons.rules.RuleParam;
import org.sonar.plugins.api.*;
import org.sonar.plugins.pmd.xml.Property;
import org.sonar.plugins.pmd.xml.Rule;
import org.sonar.plugins.pmd.xml.Ruleset;
import org.sonar.plugins.rules.StandardRulesXmlParser;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class PmdRulesRepository implements RulesRepository, ConfigurationExportable, ConfigurationImportable {
public Language getLanguage() {
return Languages.JAVA;
}
public List getInitialReferential() {
InputStream input = getClass().getResourceAsStream("/org/sonar/plugins/pmd/rules.xml");
try {
return new StandardRulesXmlParser().parse(input);
} finally {
IOUtils.closeQuietly(input);
}
}
public List parseReferential(String fileContent) {
return new StandardRulesXmlParser().parse(fileContent);
}
public List getProvidedProfiles() {
return Arrays.asList(
loadProvidedProfile(RulesProfile.SONAR_WAY_NAME, "/org/sonar/plugins/pmd/profile-sonar-way.xml"),
loadProvidedProfile(RulesProfile.SUN_CONVENTIONS_NAME, "/org/sonar/plugins/pmd/profile-sun-conventions.xml"));
}
private RulesProfile loadProvidedProfile(String name, String filename) {
try {
InputStream sonarWayProfile = getClass().getResourceAsStream(filename);
RulesProfile profile = new RulesProfile(name);
// TODO set as mandatory into the RulesProfile constructor
profile.setLanguage(Languages.JAVA.getKey());
profile.setActiveRules(importConfiguration(IOUtils.toString(sonarWayProfile)));
return profile;
} catch (IOException e) {
throw new RuntimeException("Configuration file not found for the profile : " + name, e);
}
}
public String exportConfiguration(RulesProfile activeProfile) {
Ruleset tree = buildModuleTree(activeProfile.getActiveRulesByPlugin(PmdPlugin.KEY));
String xmlModules = buildXmlFromModuleTree(tree);
return addHeaderToXml(xmlModules);
}
public List importConfiguration(String configuration) {
List activeRules = new ArrayList();
Ruleset moduleTree = buildModuleTreeFromXml(configuration);
buildActiveRulesFromModuleTree(moduleTree, activeRules);
return activeRules;
}
protected Ruleset buildModuleTree(List activeRules) {
Ruleset ruleset = new Ruleset("Sonar PMD rules");
for (ActiveRule activeRule : activeRules) {
if (activeRule.getRule().getPluginName().equals(PmdPlugin.KEY)) {
String configKey = activeRule.getRule().getConfigKey();
Rule rule = new Rule(configKey, retrieveRuleFailureLevel(activeRule.getLevel().name()));
List properties = null;
if (activeRule.getActiveRuleParams() != null && !activeRule.getActiveRuleParams().isEmpty()) {
properties = new ArrayList();
for (ActiveRuleParam activeRuleParam : activeRule.getActiveRuleParams()) {
properties.add(new Property(activeRuleParam.getRuleParam().getKey(), activeRuleParam.getValue()));
}
}
rule.setProperties(properties);
ruleset.addRule(rule);
}
}
return ruleset;
}
protected String buildXmlFromModuleTree(Ruleset tree) {
XStream xstream = new XStream();
xstream.processAnnotations(Ruleset.class);
xstream.processAnnotations(Rule.class);
xstream.processAnnotations(Property.class);
return xstream.toXML(tree);
}
protected String addHeaderToXml(String xmlModules) {
String header = "\n";
// String header = "\n" +
// "" +
// "\n";
return header + xmlModules;
}
protected Ruleset buildModuleTreeFromXml(String configuration) {
XStream xstream = new XStream();
xstream.processAnnotations(Ruleset.class);
xstream.processAnnotations(ch.hortis.sonar.model.Rule.class);
xstream.processAnnotations(Property.class);
Ruleset ruleset = (Ruleset) xstream.fromXML(configuration);
return ruleset;
}
protected void buildActiveRulesFromModuleTree(Ruleset ruleset, List activeRules) {
if (ruleset.getRules() != null && !ruleset.getRules().isEmpty()) {
for (Rule rule : ruleset.getRules()) {
String ref = rule.getRef();
for (ch.hortis.sonar.model.Rule dbRule : getInitialReferential()) {
if (dbRule.getConfigKey().equals(ref)) {
RuleFailureLevel ruleFailureLevel = getRuleFailureLevel(rule);
if (ruleFailureLevel != null) {
ActiveRule activeRule = new ActiveRule(null, dbRule, ruleFailureLevel);
activeRule.setActiveRuleParams(getActiveRuleParams(rule, dbRule, activeRule));
activeRules.add(activeRule);
break;
}
}
}
}
}
}
private String retrieveRuleFailureLevel(String levelName) {
String level = "5";
if (RuleFailureLevel.ERROR.toString().equals(levelName)) {
level = "1";
} else if (RuleFailureLevel.WARNING.toString().equals(levelName)) {
level = "3";
}
return level;
}
private RuleFailureLevel getRuleFailureLevel(Rule rule) {
RuleFailureLevel level = RuleFailureLevel.INFO;
int priority = Integer.parseInt(rule.getPriority());
if (priority <= 2) {
level = RuleFailureLevel.ERROR;
} else if (priority == 3 || priority == 4) {
level = RuleFailureLevel.WARNING;
}
return level;
}
private List getActiveRuleParams(Rule rule, ch.hortis.sonar.model.Rule dbRule, ActiveRule activeRule) {
List activeRuleParams = new ArrayList();
if (rule.getProperties() != null) {
for (Property property : rule.getProperties()) {
if (dbRule.getParams() != null) {
for (RuleParam ruleParam : dbRule.getParams()) {
if (ruleParam.getKey().equals(property.getName())) {
activeRuleParams.add(new ActiveRuleParam(activeRule, ruleParam, property.getValue()));
}
}
}
}
}
return activeRuleParams;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy