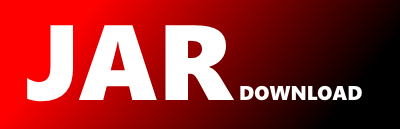
org.sonar.plugins.sources.SourcesJavaMavenCollector Maven / Gradle / Ivy
/*
* Sonar, entreprise quality control tool.
* Copyright (C) 2007-2008 Hortis-GRC SA
* mailto:be_agile HAT hortis DOT ch
*
* Sonar is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* Sonar is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Sonar; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02
*/
package org.sonar.plugins.sources;
import org.sonar.plugins.java.AbstractJavaMavenCollector;
import org.sonar.plugins.java.JavaMeasuresRecorder;
import org.apache.maven.project.MavenProject;
import org.apache.commons.io.FileUtils;
import org.apache.commons.lang.StringUtils;
import java.io.File;
import java.io.IOException;
import java.util.Iterator;
public class SourcesJavaMavenCollector extends AbstractJavaMavenCollector {
public SourcesJavaMavenCollector(JavaMeasuresRecorder measuresRecorder) {
super(measuresRecorder);
}
protected boolean shouldCollectIfNoTests() {
return true;
}
protected boolean shouldCollectIfNoSources() {
return false;
}
public boolean shouldStopOnFailure() {
return true;
}
public void collect(MavenProject pom) {
try {
parseDirectory(new File(pom.getBuild().getSourceDirectory()), false);
parseDirectory(new File(pom.getBuild().getTestSourceDirectory()), true);
} catch (IOException e) {
e.printStackTrace();
}
}
private void parseDirectory(File directory, boolean unitTest) throws IOException {
if (directory.exists()) {
Iterator fileIterator = FileUtils.iterateFiles(directory, new String[]{"java"}, true);
while (fileIterator.hasNext()) {
File file = (File) fileIterator.next();
String filename = StringUtils.substringBeforeLast(file.getName(), ".");
String source = FileUtils.readFileToString(file);
getMeasuresRecorder().createClassSource(extractPackageName(file, directory), filename, source, unitTest);
}
}
}
protected String extractPackageName(File file, File directory) {
String packageName = StringUtils.removeStart(file.getPath(), directory.getPath());
packageName = StringUtils.replaceChars(packageName, '\\', '/');
packageName = StringUtils.substringBeforeLast(packageName, "/");
packageName = StringUtils.replaceChars(packageName, '/', '.');
if (packageName.endsWith(".")) {
packageName = StringUtils.chop(packageName);
}
if (packageName.startsWith(".")) {
packageName = StringUtils.substringAfter(packageName, ".");
}
return packageName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy