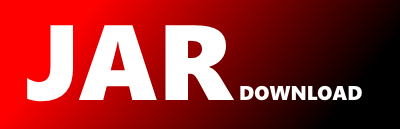
org.sonar.batch.protocol.output.BatchReport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sonar-batch-protocol Show documentation
Show all versions of sonar-batch-protocol Show documentation
Classes used for communication between batch and server
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: batch_report.proto
package org.sonar.batch.protocol.output;
public final class BatchReport {
private BatchReport() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public interface MetadataOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional int64 analysis_date = 1;
/**
* optional int64 analysis_date = 1;
*/
boolean hasAnalysisDate();
/**
* optional int64 analysis_date = 1;
*/
long getAnalysisDate();
// optional string project_key = 2;
/**
* optional string project_key = 2;
*/
boolean hasProjectKey();
/**
* optional string project_key = 2;
*/
java.lang.String getProjectKey();
/**
* optional string project_key = 2;
*/
com.google.protobuf.ByteString
getProjectKeyBytes();
// optional int32 root_component_ref = 3;
/**
* optional int32 root_component_ref = 3;
*/
boolean hasRootComponentRef();
/**
* optional int32 root_component_ref = 3;
*/
int getRootComponentRef();
// optional int64 snapshot_id = 4;
/**
* optional int64 snapshot_id = 4;
*
*
* temporary fields used during development of computation stack
*
*/
boolean hasSnapshotId();
/**
* optional int64 snapshot_id = 4;
*
*
* temporary fields used during development of computation stack
*
*/
long getSnapshotId();
// optional int32 deleted_components_count = 5;
/**
* optional int32 deleted_components_count = 5;
*/
boolean hasDeletedComponentsCount();
/**
* optional int32 deleted_components_count = 5;
*/
int getDeletedComponentsCount();
}
/**
* Protobuf type {@code Metadata}
*/
public static final class Metadata extends
com.google.protobuf.GeneratedMessage
implements MetadataOrBuilder {
// Use Metadata.newBuilder() to construct.
private Metadata(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Metadata(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Metadata defaultInstance;
public static Metadata getDefaultInstance() {
return defaultInstance;
}
public Metadata getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Metadata(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
analysisDate_ = input.readInt64();
break;
}
case 18: {
bitField0_ |= 0x00000002;
projectKey_ = input.readBytes();
break;
}
case 24: {
bitField0_ |= 0x00000004;
rootComponentRef_ = input.readInt32();
break;
}
case 32: {
bitField0_ |= 0x00000008;
snapshotId_ = input.readInt64();
break;
}
case 40: {
bitField0_ |= 0x00000010;
deletedComponentsCount_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Metadata_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Metadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.batch.protocol.output.BatchReport.Metadata.class, org.sonar.batch.protocol.output.BatchReport.Metadata.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Metadata parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Metadata(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional int64 analysis_date = 1;
public static final int ANALYSIS_DATE_FIELD_NUMBER = 1;
private long analysisDate_;
/**
* optional int64 analysis_date = 1;
*/
public boolean hasAnalysisDate() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 analysis_date = 1;
*/
public long getAnalysisDate() {
return analysisDate_;
}
// optional string project_key = 2;
public static final int PROJECT_KEY_FIELD_NUMBER = 2;
private java.lang.Object projectKey_;
/**
* optional string project_key = 2;
*/
public boolean hasProjectKey() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string project_key = 2;
*/
public java.lang.String getProjectKey() {
java.lang.Object ref = projectKey_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
projectKey_ = s;
}
return s;
}
}
/**
* optional string project_key = 2;
*/
public com.google.protobuf.ByteString
getProjectKeyBytes() {
java.lang.Object ref = projectKey_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
projectKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional int32 root_component_ref = 3;
public static final int ROOT_COMPONENT_REF_FIELD_NUMBER = 3;
private int rootComponentRef_;
/**
* optional int32 root_component_ref = 3;
*/
public boolean hasRootComponentRef() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int32 root_component_ref = 3;
*/
public int getRootComponentRef() {
return rootComponentRef_;
}
// optional int64 snapshot_id = 4;
public static final int SNAPSHOT_ID_FIELD_NUMBER = 4;
private long snapshotId_;
/**
* optional int64 snapshot_id = 4;
*
*
* temporary fields used during development of computation stack
*
*/
public boolean hasSnapshotId() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int64 snapshot_id = 4;
*
*
* temporary fields used during development of computation stack
*
*/
public long getSnapshotId() {
return snapshotId_;
}
// optional int32 deleted_components_count = 5;
public static final int DELETED_COMPONENTS_COUNT_FIELD_NUMBER = 5;
private int deletedComponentsCount_;
/**
* optional int32 deleted_components_count = 5;
*/
public boolean hasDeletedComponentsCount() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int32 deleted_components_count = 5;
*/
public int getDeletedComponentsCount() {
return deletedComponentsCount_;
}
private void initFields() {
analysisDate_ = 0L;
projectKey_ = "";
rootComponentRef_ = 0;
snapshotId_ = 0L;
deletedComponentsCount_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(1, analysisDate_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getProjectKeyBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt32(3, rootComponentRef_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt64(4, snapshotId_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeInt32(5, deletedComponentsCount_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, analysisDate_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getProjectKeyBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, rootComponentRef_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, snapshotId_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, deletedComponentsCount_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.sonar.batch.protocol.output.BatchReport.Metadata parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.batch.protocol.output.BatchReport.Metadata parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.batch.protocol.output.BatchReport.Metadata parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.batch.protocol.output.BatchReport.Metadata parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.batch.protocol.output.BatchReport.Metadata parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.batch.protocol.output.BatchReport.Metadata parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.batch.protocol.output.BatchReport.Metadata parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.batch.protocol.output.BatchReport.Metadata parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.batch.protocol.output.BatchReport.Metadata parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.batch.protocol.output.BatchReport.Metadata parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.sonar.batch.protocol.output.BatchReport.Metadata prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Metadata}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.sonar.batch.protocol.output.BatchReport.MetadataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Metadata_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Metadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.batch.protocol.output.BatchReport.Metadata.class, org.sonar.batch.protocol.output.BatchReport.Metadata.Builder.class);
}
// Construct using org.sonar.batch.protocol.output.BatchReport.Metadata.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
analysisDate_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
projectKey_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
rootComponentRef_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
snapshotId_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
deletedComponentsCount_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Metadata_descriptor;
}
public org.sonar.batch.protocol.output.BatchReport.Metadata getDefaultInstanceForType() {
return org.sonar.batch.protocol.output.BatchReport.Metadata.getDefaultInstance();
}
public org.sonar.batch.protocol.output.BatchReport.Metadata build() {
org.sonar.batch.protocol.output.BatchReport.Metadata result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.batch.protocol.output.BatchReport.Metadata buildPartial() {
org.sonar.batch.protocol.output.BatchReport.Metadata result = new org.sonar.batch.protocol.output.BatchReport.Metadata(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.analysisDate_ = analysisDate_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.projectKey_ = projectKey_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.rootComponentRef_ = rootComponentRef_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.snapshotId_ = snapshotId_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.deletedComponentsCount_ = deletedComponentsCount_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.batch.protocol.output.BatchReport.Metadata) {
return mergeFrom((org.sonar.batch.protocol.output.BatchReport.Metadata)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.batch.protocol.output.BatchReport.Metadata other) {
if (other == org.sonar.batch.protocol.output.BatchReport.Metadata.getDefaultInstance()) return this;
if (other.hasAnalysisDate()) {
setAnalysisDate(other.getAnalysisDate());
}
if (other.hasProjectKey()) {
bitField0_ |= 0x00000002;
projectKey_ = other.projectKey_;
onChanged();
}
if (other.hasRootComponentRef()) {
setRootComponentRef(other.getRootComponentRef());
}
if (other.hasSnapshotId()) {
setSnapshotId(other.getSnapshotId());
}
if (other.hasDeletedComponentsCount()) {
setDeletedComponentsCount(other.getDeletedComponentsCount());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.batch.protocol.output.BatchReport.Metadata parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.batch.protocol.output.BatchReport.Metadata) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional int64 analysis_date = 1;
private long analysisDate_ ;
/**
* optional int64 analysis_date = 1;
*/
public boolean hasAnalysisDate() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 analysis_date = 1;
*/
public long getAnalysisDate() {
return analysisDate_;
}
/**
* optional int64 analysis_date = 1;
*/
public Builder setAnalysisDate(long value) {
bitField0_ |= 0x00000001;
analysisDate_ = value;
onChanged();
return this;
}
/**
* optional int64 analysis_date = 1;
*/
public Builder clearAnalysisDate() {
bitField0_ = (bitField0_ & ~0x00000001);
analysisDate_ = 0L;
onChanged();
return this;
}
// optional string project_key = 2;
private java.lang.Object projectKey_ = "";
/**
* optional string project_key = 2;
*/
public boolean hasProjectKey() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string project_key = 2;
*/
public java.lang.String getProjectKey() {
java.lang.Object ref = projectKey_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
projectKey_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string project_key = 2;
*/
public com.google.protobuf.ByteString
getProjectKeyBytes() {
java.lang.Object ref = projectKey_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
projectKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string project_key = 2;
*/
public Builder setProjectKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
projectKey_ = value;
onChanged();
return this;
}
/**
* optional string project_key = 2;
*/
public Builder clearProjectKey() {
bitField0_ = (bitField0_ & ~0x00000002);
projectKey_ = getDefaultInstance().getProjectKey();
onChanged();
return this;
}
/**
* optional string project_key = 2;
*/
public Builder setProjectKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
projectKey_ = value;
onChanged();
return this;
}
// optional int32 root_component_ref = 3;
private int rootComponentRef_ ;
/**
* optional int32 root_component_ref = 3;
*/
public boolean hasRootComponentRef() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int32 root_component_ref = 3;
*/
public int getRootComponentRef() {
return rootComponentRef_;
}
/**
* optional int32 root_component_ref = 3;
*/
public Builder setRootComponentRef(int value) {
bitField0_ |= 0x00000004;
rootComponentRef_ = value;
onChanged();
return this;
}
/**
* optional int32 root_component_ref = 3;
*/
public Builder clearRootComponentRef() {
bitField0_ = (bitField0_ & ~0x00000004);
rootComponentRef_ = 0;
onChanged();
return this;
}
// optional int64 snapshot_id = 4;
private long snapshotId_ ;
/**
* optional int64 snapshot_id = 4;
*
*
* temporary fields used during development of computation stack
*
*/
public boolean hasSnapshotId() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int64 snapshot_id = 4;
*
*
* temporary fields used during development of computation stack
*
*/
public long getSnapshotId() {
return snapshotId_;
}
/**
* optional int64 snapshot_id = 4;
*
*
* temporary fields used during development of computation stack
*
*/
public Builder setSnapshotId(long value) {
bitField0_ |= 0x00000008;
snapshotId_ = value;
onChanged();
return this;
}
/**
* optional int64 snapshot_id = 4;
*
*
* temporary fields used during development of computation stack
*
*/
public Builder clearSnapshotId() {
bitField0_ = (bitField0_ & ~0x00000008);
snapshotId_ = 0L;
onChanged();
return this;
}
// optional int32 deleted_components_count = 5;
private int deletedComponentsCount_ ;
/**
* optional int32 deleted_components_count = 5;
*/
public boolean hasDeletedComponentsCount() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int32 deleted_components_count = 5;
*/
public int getDeletedComponentsCount() {
return deletedComponentsCount_;
}
/**
* optional int32 deleted_components_count = 5;
*/
public Builder setDeletedComponentsCount(int value) {
bitField0_ |= 0x00000010;
deletedComponentsCount_ = value;
onChanged();
return this;
}
/**
* optional int32 deleted_components_count = 5;
*/
public Builder clearDeletedComponentsCount() {
bitField0_ = (bitField0_ & ~0x00000010);
deletedComponentsCount_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:Metadata)
}
static {
defaultInstance = new Metadata(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:Metadata)
}
public interface ComponentOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional int32 ref = 1;
/**
* optional int32 ref = 1;
*/
boolean hasRef();
/**
* optional int32 ref = 1;
*/
int getRef();
// optional string path = 2;
/**
* optional string path = 2;
*/
boolean hasPath();
/**
* optional string path = 2;
*/
java.lang.String getPath();
/**
* optional string path = 2;
*/
com.google.protobuf.ByteString
getPathBytes();
// optional string name = 3;
/**
* optional string name = 3;
*/
boolean hasName();
/**
* optional string name = 3;
*/
java.lang.String getName();
/**
* optional string name = 3;
*/
com.google.protobuf.ByteString
getNameBytes();
// optional .ComponentType type = 4;
/**
* optional .ComponentType type = 4;
*/
boolean hasType();
/**
* optional .ComponentType type = 4;
*/
org.sonar.batch.protocol.Constants.ComponentType getType();
// optional bool is_test = 5;
/**
* optional bool is_test = 5;
*/
boolean hasIsTest();
/**
* optional bool is_test = 5;
*/
boolean getIsTest();
// optional string language = 6;
/**
* optional string language = 6;
*/
boolean hasLanguage();
/**
* optional string language = 6;
*/
java.lang.String getLanguage();
/**
* optional string language = 6;
*/
com.google.protobuf.ByteString
getLanguageBytes();
// repeated int32 child_refs = 7 [packed = true];
/**
* repeated int32 child_refs = 7 [packed = true];
*/
java.util.List getChildRefsList();
/**
* repeated int32 child_refs = 7 [packed = true];
*/
int getChildRefsCount();
/**
* repeated int32 child_refs = 7 [packed = true];
*/
int getChildRefs(int index);
// optional int32 snapshot_id = 8;
/**
* optional int32 snapshot_id = 8;
*
*
* temporary fields during development of computation stack
*
*/
boolean hasSnapshotId();
/**
* optional int32 snapshot_id = 8;
*
*
* temporary fields during development of computation stack
*
*/
int getSnapshotId();
// optional string uuid = 9;
/**
* optional string uuid = 9;
*/
boolean hasUuid();
/**
* optional string uuid = 9;
*/
java.lang.String getUuid();
/**
* optional string uuid = 9;
*/
com.google.protobuf.ByteString
getUuidBytes();
}
/**
* Protobuf type {@code Component}
*/
public static final class Component extends
com.google.protobuf.GeneratedMessage
implements ComponentOrBuilder {
// Use Component.newBuilder() to construct.
private Component(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Component(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Component defaultInstance;
public static Component getDefaultInstance() {
return defaultInstance;
}
public Component getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Component(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
ref_ = input.readInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
path_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
name_ = input.readBytes();
break;
}
case 32: {
int rawValue = input.readEnum();
org.sonar.batch.protocol.Constants.ComponentType value = org.sonar.batch.protocol.Constants.ComponentType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(4, rawValue);
} else {
bitField0_ |= 0x00000008;
type_ = value;
}
break;
}
case 40: {
bitField0_ |= 0x00000010;
isTest_ = input.readBool();
break;
}
case 50: {
bitField0_ |= 0x00000020;
language_ = input.readBytes();
break;
}
case 56: {
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
childRefs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
childRefs_.add(input.readInt32());
break;
}
case 58: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040) && input.getBytesUntilLimit() > 0) {
childRefs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
while (input.getBytesUntilLimit() > 0) {
childRefs_.add(input.readInt32());
}
input.popLimit(limit);
break;
}
case 64: {
bitField0_ |= 0x00000040;
snapshotId_ = input.readInt32();
break;
}
case 74: {
bitField0_ |= 0x00000080;
uuid_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
childRefs_ = java.util.Collections.unmodifiableList(childRefs_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Component_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Component_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.batch.protocol.output.BatchReport.Component.class, org.sonar.batch.protocol.output.BatchReport.Component.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Component parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Component(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional int32 ref = 1;
public static final int REF_FIELD_NUMBER = 1;
private int ref_;
/**
* optional int32 ref = 1;
*/
public boolean hasRef() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 ref = 1;
*/
public int getRef() {
return ref_;
}
// optional string path = 2;
public static final int PATH_FIELD_NUMBER = 2;
private java.lang.Object path_;
/**
* optional string path = 2;
*/
public boolean hasPath() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string path = 2;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
path_ = s;
}
return s;
}
}
/**
* optional string path = 2;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string name = 3;
public static final int NAME_FIELD_NUMBER = 3;
private java.lang.Object name_;
/**
* optional string name = 3;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string name = 3;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* optional string name = 3;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional .ComponentType type = 4;
public static final int TYPE_FIELD_NUMBER = 4;
private org.sonar.batch.protocol.Constants.ComponentType type_;
/**
* optional .ComponentType type = 4;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .ComponentType type = 4;
*/
public org.sonar.batch.protocol.Constants.ComponentType getType() {
return type_;
}
// optional bool is_test = 5;
public static final int IS_TEST_FIELD_NUMBER = 5;
private boolean isTest_;
/**
* optional bool is_test = 5;
*/
public boolean hasIsTest() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool is_test = 5;
*/
public boolean getIsTest() {
return isTest_;
}
// optional string language = 6;
public static final int LANGUAGE_FIELD_NUMBER = 6;
private java.lang.Object language_;
/**
* optional string language = 6;
*/
public boolean hasLanguage() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string language = 6;
*/
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
language_ = s;
}
return s;
}
}
/**
* optional string language = 6;
*/
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// repeated int32 child_refs = 7 [packed = true];
public static final int CHILD_REFS_FIELD_NUMBER = 7;
private java.util.List childRefs_;
/**
* repeated int32 child_refs = 7 [packed = true];
*/
public java.util.List
getChildRefsList() {
return childRefs_;
}
/**
* repeated int32 child_refs = 7 [packed = true];
*/
public int getChildRefsCount() {
return childRefs_.size();
}
/**
* repeated int32 child_refs = 7 [packed = true];
*/
public int getChildRefs(int index) {
return childRefs_.get(index);
}
private int childRefsMemoizedSerializedSize = -1;
// optional int32 snapshot_id = 8;
public static final int SNAPSHOT_ID_FIELD_NUMBER = 8;
private int snapshotId_;
/**
* optional int32 snapshot_id = 8;
*
*
* temporary fields during development of computation stack
*
*/
public boolean hasSnapshotId() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional int32 snapshot_id = 8;
*
*
* temporary fields during development of computation stack
*
*/
public int getSnapshotId() {
return snapshotId_;
}
// optional string uuid = 9;
public static final int UUID_FIELD_NUMBER = 9;
private java.lang.Object uuid_;
/**
* optional string uuid = 9;
*/
public boolean hasUuid() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional string uuid = 9;
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
uuid_ = s;
}
return s;
}
}
/**
* optional string uuid = 9;
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
ref_ = 0;
path_ = "";
name_ = "";
type_ = org.sonar.batch.protocol.Constants.ComponentType.PROJECT;
isTest_ = false;
language_ = "";
childRefs_ = java.util.Collections.emptyList();
snapshotId_ = 0;
uuid_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, ref_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getPathBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getNameBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeEnum(4, type_.getNumber());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBool(5, isTest_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBytes(6, getLanguageBytes());
}
if (getChildRefsList().size() > 0) {
output.writeRawVarint32(58);
output.writeRawVarint32(childRefsMemoizedSerializedSize);
}
for (int i = 0; i < childRefs_.size(); i++) {
output.writeInt32NoTag(childRefs_.get(i));
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeInt32(8, snapshotId_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeBytes(9, getUuidBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, ref_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getPathBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getNameBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, type_.getNumber());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, isTest_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, getLanguageBytes());
}
{
int dataSize = 0;
for (int i = 0; i < childRefs_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(childRefs_.get(i));
}
size += dataSize;
if (!getChildRefsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
childRefsMemoizedSerializedSize = dataSize;
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(8, snapshotId_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(9, getUuidBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.sonar.batch.protocol.output.BatchReport.Component parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.batch.protocol.output.BatchReport.Component parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.batch.protocol.output.BatchReport.Component parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.batch.protocol.output.BatchReport.Component parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.batch.protocol.output.BatchReport.Component parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.batch.protocol.output.BatchReport.Component parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.batch.protocol.output.BatchReport.Component parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.batch.protocol.output.BatchReport.Component parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.batch.protocol.output.BatchReport.Component parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.batch.protocol.output.BatchReport.Component parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.sonar.batch.protocol.output.BatchReport.Component prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Component}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.sonar.batch.protocol.output.BatchReport.ComponentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Component_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Component_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.batch.protocol.output.BatchReport.Component.class, org.sonar.batch.protocol.output.BatchReport.Component.Builder.class);
}
// Construct using org.sonar.batch.protocol.output.BatchReport.Component.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
ref_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
path_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
type_ = org.sonar.batch.protocol.Constants.ComponentType.PROJECT;
bitField0_ = (bitField0_ & ~0x00000008);
isTest_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
language_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
childRefs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
snapshotId_ = 0;
bitField0_ = (bitField0_ & ~0x00000080);
uuid_ = "";
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Component_descriptor;
}
public org.sonar.batch.protocol.output.BatchReport.Component getDefaultInstanceForType() {
return org.sonar.batch.protocol.output.BatchReport.Component.getDefaultInstance();
}
public org.sonar.batch.protocol.output.BatchReport.Component build() {
org.sonar.batch.protocol.output.BatchReport.Component result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.batch.protocol.output.BatchReport.Component buildPartial() {
org.sonar.batch.protocol.output.BatchReport.Component result = new org.sonar.batch.protocol.output.BatchReport.Component(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.ref_ = ref_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.path_ = path_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.isTest_ = isTest_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.language_ = language_;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
childRefs_ = java.util.Collections.unmodifiableList(childRefs_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.childRefs_ = childRefs_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000040;
}
result.snapshotId_ = snapshotId_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000080;
}
result.uuid_ = uuid_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.batch.protocol.output.BatchReport.Component) {
return mergeFrom((org.sonar.batch.protocol.output.BatchReport.Component)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.batch.protocol.output.BatchReport.Component other) {
if (other == org.sonar.batch.protocol.output.BatchReport.Component.getDefaultInstance()) return this;
if (other.hasRef()) {
setRef(other.getRef());
}
if (other.hasPath()) {
bitField0_ |= 0x00000002;
path_ = other.path_;
onChanged();
}
if (other.hasName()) {
bitField0_ |= 0x00000004;
name_ = other.name_;
onChanged();
}
if (other.hasType()) {
setType(other.getType());
}
if (other.hasIsTest()) {
setIsTest(other.getIsTest());
}
if (other.hasLanguage()) {
bitField0_ |= 0x00000020;
language_ = other.language_;
onChanged();
}
if (!other.childRefs_.isEmpty()) {
if (childRefs_.isEmpty()) {
childRefs_ = other.childRefs_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureChildRefsIsMutable();
childRefs_.addAll(other.childRefs_);
}
onChanged();
}
if (other.hasSnapshotId()) {
setSnapshotId(other.getSnapshotId());
}
if (other.hasUuid()) {
bitField0_ |= 0x00000100;
uuid_ = other.uuid_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.batch.protocol.output.BatchReport.Component parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.batch.protocol.output.BatchReport.Component) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional int32 ref = 1;
private int ref_ ;
/**
* optional int32 ref = 1;
*/
public boolean hasRef() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 ref = 1;
*/
public int getRef() {
return ref_;
}
/**
* optional int32 ref = 1;
*/
public Builder setRef(int value) {
bitField0_ |= 0x00000001;
ref_ = value;
onChanged();
return this;
}
/**
* optional int32 ref = 1;
*/
public Builder clearRef() {
bitField0_ = (bitField0_ & ~0x00000001);
ref_ = 0;
onChanged();
return this;
}
// optional string path = 2;
private java.lang.Object path_ = "";
/**
* optional string path = 2;
*/
public boolean hasPath() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string path = 2;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
path_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string path = 2;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string path = 2;
*/
public Builder setPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
path_ = value;
onChanged();
return this;
}
/**
* optional string path = 2;
*/
public Builder clearPath() {
bitField0_ = (bitField0_ & ~0x00000002);
path_ = getDefaultInstance().getPath();
onChanged();
return this;
}
/**
* optional string path = 2;
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
path_ = value;
onChanged();
return this;
}
// optional string name = 3;
private java.lang.Object name_ = "";
/**
* optional string name = 3;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string name = 3;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 3;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 3;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 3;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000004);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 3;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
name_ = value;
onChanged();
return this;
}
// optional .ComponentType type = 4;
private org.sonar.batch.protocol.Constants.ComponentType type_ = org.sonar.batch.protocol.Constants.ComponentType.PROJECT;
/**
* optional .ComponentType type = 4;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .ComponentType type = 4;
*/
public org.sonar.batch.protocol.Constants.ComponentType getType() {
return type_;
}
/**
* optional .ComponentType type = 4;
*/
public Builder setType(org.sonar.batch.protocol.Constants.ComponentType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
type_ = value;
onChanged();
return this;
}
/**
* optional .ComponentType type = 4;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000008);
type_ = org.sonar.batch.protocol.Constants.ComponentType.PROJECT;
onChanged();
return this;
}
// optional bool is_test = 5;
private boolean isTest_ ;
/**
* optional bool is_test = 5;
*/
public boolean hasIsTest() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool is_test = 5;
*/
public boolean getIsTest() {
return isTest_;
}
/**
* optional bool is_test = 5;
*/
public Builder setIsTest(boolean value) {
bitField0_ |= 0x00000010;
isTest_ = value;
onChanged();
return this;
}
/**
* optional bool is_test = 5;
*/
public Builder clearIsTest() {
bitField0_ = (bitField0_ & ~0x00000010);
isTest_ = false;
onChanged();
return this;
}
// optional string language = 6;
private java.lang.Object language_ = "";
/**
* optional string language = 6;
*/
public boolean hasLanguage() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string language = 6;
*/
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
language_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string language = 6;
*/
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string language = 6;
*/
public Builder setLanguage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
language_ = value;
onChanged();
return this;
}
/**
* optional string language = 6;
*/
public Builder clearLanguage() {
bitField0_ = (bitField0_ & ~0x00000020);
language_ = getDefaultInstance().getLanguage();
onChanged();
return this;
}
/**
* optional string language = 6;
*/
public Builder setLanguageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
language_ = value;
onChanged();
return this;
}
// repeated int32 child_refs = 7 [packed = true];
private java.util.List childRefs_ = java.util.Collections.emptyList();
private void ensureChildRefsIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
childRefs_ = new java.util.ArrayList(childRefs_);
bitField0_ |= 0x00000040;
}
}
/**
* repeated int32 child_refs = 7 [packed = true];
*/
public java.util.List
getChildRefsList() {
return java.util.Collections.unmodifiableList(childRefs_);
}
/**
* repeated int32 child_refs = 7 [packed = true];
*/
public int getChildRefsCount() {
return childRefs_.size();
}
/**
* repeated int32 child_refs = 7 [packed = true];
*/
public int getChildRefs(int index) {
return childRefs_.get(index);
}
/**
* repeated int32 child_refs = 7 [packed = true];
*/
public Builder setChildRefs(
int index, int value) {
ensureChildRefsIsMutable();
childRefs_.set(index, value);
onChanged();
return this;
}
/**
* repeated int32 child_refs = 7 [packed = true];
*/
public Builder addChildRefs(int value) {
ensureChildRefsIsMutable();
childRefs_.add(value);
onChanged();
return this;
}
/**
* repeated int32 child_refs = 7 [packed = true];
*/
public Builder addAllChildRefs(
java.lang.Iterable extends java.lang.Integer> values) {
ensureChildRefsIsMutable();
super.addAll(values, childRefs_);
onChanged();
return this;
}
/**
* repeated int32 child_refs = 7 [packed = true];
*/
public Builder clearChildRefs() {
childRefs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
// optional int32 snapshot_id = 8;
private int snapshotId_ ;
/**
* optional int32 snapshot_id = 8;
*
*
* temporary fields during development of computation stack
*
*/
public boolean hasSnapshotId() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional int32 snapshot_id = 8;
*
*
* temporary fields during development of computation stack
*
*/
public int getSnapshotId() {
return snapshotId_;
}
/**
* optional int32 snapshot_id = 8;
*
*
* temporary fields during development of computation stack
*
*/
public Builder setSnapshotId(int value) {
bitField0_ |= 0x00000080;
snapshotId_ = value;
onChanged();
return this;
}
/**
* optional int32 snapshot_id = 8;
*
*
* temporary fields during development of computation stack
*
*/
public Builder clearSnapshotId() {
bitField0_ = (bitField0_ & ~0x00000080);
snapshotId_ = 0;
onChanged();
return this;
}
// optional string uuid = 9;
private java.lang.Object uuid_ = "";
/**
* optional string uuid = 9;
*/
public boolean hasUuid() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional string uuid = 9;
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
uuid_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string uuid = 9;
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string uuid = 9;
*/
public Builder setUuid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
uuid_ = value;
onChanged();
return this;
}
/**
* optional string uuid = 9;
*/
public Builder clearUuid() {
bitField0_ = (bitField0_ & ~0x00000100);
uuid_ = getDefaultInstance().getUuid();
onChanged();
return this;
}
/**
* optional string uuid = 9;
*/
public Builder setUuidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
uuid_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:Component)
}
static {
defaultInstance = new Component(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:Component)
}
public interface IssueOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string rule_repository = 1;
/**
* optional string rule_repository = 1;
*/
boolean hasRuleRepository();
/**
* optional string rule_repository = 1;
*/
java.lang.String getRuleRepository();
/**
* optional string rule_repository = 1;
*/
com.google.protobuf.ByteString
getRuleRepositoryBytes();
// optional string rule_key = 2;
/**
* optional string rule_key = 2;
*/
boolean hasRuleKey();
/**
* optional string rule_key = 2;
*/
java.lang.String getRuleKey();
/**
* optional string rule_key = 2;
*/
com.google.protobuf.ByteString
getRuleKeyBytes();
// optional int32 line = 3;
/**
* optional int32 line = 3;
*/
boolean hasLine();
/**
* optional int32 line = 3;
*/
int getLine();
// optional string msg = 4;
/**
* optional string msg = 4;
*/
boolean hasMsg();
/**
* optional string msg = 4;
*/
java.lang.String getMsg();
/**
* optional string msg = 4;
*/
com.google.protobuf.ByteString
getMsgBytes();
// optional .Severity severity = 5;
/**
* optional .Severity severity = 5;
*/
boolean hasSeverity();
/**
* optional .Severity severity = 5;
*/
org.sonar.batch.protocol.Constants.Severity getSeverity();
// repeated string tags = 6;
/**
* repeated string tags = 6;
*/
java.util.List
getTagsList();
/**
* repeated string tags = 6;
*/
int getTagsCount();
/**
* repeated string tags = 6;
*/
java.lang.String getTags(int index);
/**
* repeated string tags = 6;
*/
com.google.protobuf.ByteString
getTagsBytes(int index);
// optional double effort_to_fix = 7;
/**
* optional double effort_to_fix = 7;
*
*
* temporary fields during development of computation stack
*
*/
boolean hasEffortToFix();
/**
* optional double effort_to_fix = 7;
*
*
* temporary fields during development of computation stack
*
*/
double getEffortToFix();
// optional bool is_new = 8;
/**
* optional bool is_new = 8;
*/
boolean hasIsNew();
/**
* optional bool is_new = 8;
*/
boolean getIsNew();
// optional string uuid = 9;
/**
* optional string uuid = 9;
*/
boolean hasUuid();
/**
* optional string uuid = 9;
*/
java.lang.String getUuid();
/**
* optional string uuid = 9;
*/
com.google.protobuf.ByteString
getUuidBytes();
// optional int64 debt_in_minutes = 10;
/**
* optional int64 debt_in_minutes = 10;
*/
boolean hasDebtInMinutes();
/**
* optional int64 debt_in_minutes = 10;
*/
long getDebtInMinutes();
// optional string resolution = 11;
/**
* optional string resolution = 11;
*/
boolean hasResolution();
/**
* optional string resolution = 11;
*/
java.lang.String getResolution();
/**
* optional string resolution = 11;
*/
com.google.protobuf.ByteString
getResolutionBytes();
// optional string status = 12;
/**
* optional string status = 12;
*/
boolean hasStatus();
/**
* optional string status = 12;
*/
java.lang.String getStatus();
/**
* optional string status = 12;
*/
com.google.protobuf.ByteString
getStatusBytes();
// optional string checksum = 13;
/**
* optional string checksum = 13;
*/
boolean hasChecksum();
/**
* optional string checksum = 13;
*/
java.lang.String getChecksum();
/**
* optional string checksum = 13;
*/
com.google.protobuf.ByteString
getChecksumBytes();
// optional bool manual_severity = 14;
/**
* optional bool manual_severity = 14;
*/
boolean hasManualSeverity();
/**
* optional bool manual_severity = 14;
*/
boolean getManualSeverity();
// optional string reporter = 15;
/**
* optional string reporter = 15;
*/
boolean hasReporter();
/**
* optional string reporter = 15;
*/
java.lang.String getReporter();
/**
* optional string reporter = 15;
*/
com.google.protobuf.ByteString
getReporterBytes();
// optional string assignee = 16;
/**
* optional string assignee = 16;
*/
boolean hasAssignee();
/**
* optional string assignee = 16;
*/
java.lang.String getAssignee();
/**
* optional string assignee = 16;
*/
com.google.protobuf.ByteString
getAssigneeBytes();
// optional string action_plan_key = 17;
/**
* optional string action_plan_key = 17;
*/
boolean hasActionPlanKey();
/**
* optional string action_plan_key = 17;
*/
java.lang.String getActionPlanKey();
/**
* optional string action_plan_key = 17;
*/
com.google.protobuf.ByteString
getActionPlanKeyBytes();
// optional string attributes = 18;
/**
* optional string attributes = 18;
*/
boolean hasAttributes();
/**
* optional string attributes = 18;
*/
java.lang.String getAttributes();
/**
* optional string attributes = 18;
*/
com.google.protobuf.ByteString
getAttributesBytes();
// optional string author_login = 19;
/**
* optional string author_login = 19;
*/
boolean hasAuthorLogin();
/**
* optional string author_login = 19;
*/
java.lang.String getAuthorLogin();
/**
* optional string author_login = 19;
*/
com.google.protobuf.ByteString
getAuthorLoginBytes();
// optional int64 creation_date = 20;
/**
* optional int64 creation_date = 20;
*/
boolean hasCreationDate();
/**
* optional int64 creation_date = 20;
*/
long getCreationDate();
// optional int64 close_date = 21;
/**
* optional int64 close_date = 21;
*/
boolean hasCloseDate();
/**
* optional int64 close_date = 21;
*/
long getCloseDate();
// optional int64 update_date = 22;
/**
* optional int64 update_date = 22;
*/
boolean hasUpdateDate();
/**
* optional int64 update_date = 22;
*/
long getUpdateDate();
// optional int64 selected_at = 23;
/**
* optional int64 selected_at = 23;
*/
boolean hasSelectedAt();
/**
* optional int64 selected_at = 23;
*/
long getSelectedAt();
// optional string diff_fields = 24;
/**
* optional string diff_fields = 24;
*/
boolean hasDiffFields();
/**
* optional string diff_fields = 24;
*/
java.lang.String getDiffFields();
/**
* optional string diff_fields = 24;
*/
com.google.protobuf.ByteString
getDiffFieldsBytes();
// optional bool is_changed = 25;
/**
* optional bool is_changed = 25;
*/
boolean hasIsChanged();
/**
* optional bool is_changed = 25;
*/
boolean getIsChanged();
// optional bool must_send_notification = 26;
/**
* optional bool must_send_notification = 26;
*/
boolean hasMustSendNotification();
/**
* optional bool must_send_notification = 26;
*/
boolean getMustSendNotification();
}
/**
* Protobuf type {@code Issue}
*/
public static final class Issue extends
com.google.protobuf.GeneratedMessage
implements IssueOrBuilder {
// Use Issue.newBuilder() to construct.
private Issue(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Issue(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Issue defaultInstance;
public static Issue getDefaultInstance() {
return defaultInstance;
}
public Issue getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Issue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
ruleRepository_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
ruleKey_ = input.readBytes();
break;
}
case 24: {
bitField0_ |= 0x00000004;
line_ = input.readInt32();
break;
}
case 34: {
bitField0_ |= 0x00000008;
msg_ = input.readBytes();
break;
}
case 40: {
int rawValue = input.readEnum();
org.sonar.batch.protocol.Constants.Severity value = org.sonar.batch.protocol.Constants.Severity.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(5, rawValue);
} else {
bitField0_ |= 0x00000010;
severity_ = value;
}
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
tags_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000020;
}
tags_.add(input.readBytes());
break;
}
case 57: {
bitField0_ |= 0x00000020;
effortToFix_ = input.readDouble();
break;
}
case 64: {
bitField0_ |= 0x00000040;
isNew_ = input.readBool();
break;
}
case 74: {
bitField0_ |= 0x00000080;
uuid_ = input.readBytes();
break;
}
case 80: {
bitField0_ |= 0x00000100;
debtInMinutes_ = input.readInt64();
break;
}
case 90: {
bitField0_ |= 0x00000200;
resolution_ = input.readBytes();
break;
}
case 98: {
bitField0_ |= 0x00000400;
status_ = input.readBytes();
break;
}
case 106: {
bitField0_ |= 0x00000800;
checksum_ = input.readBytes();
break;
}
case 112: {
bitField0_ |= 0x00001000;
manualSeverity_ = input.readBool();
break;
}
case 122: {
bitField0_ |= 0x00002000;
reporter_ = input.readBytes();
break;
}
case 130: {
bitField0_ |= 0x00004000;
assignee_ = input.readBytes();
break;
}
case 138: {
bitField0_ |= 0x00008000;
actionPlanKey_ = input.readBytes();
break;
}
case 146: {
bitField0_ |= 0x00010000;
attributes_ = input.readBytes();
break;
}
case 154: {
bitField0_ |= 0x00020000;
authorLogin_ = input.readBytes();
break;
}
case 160: {
bitField0_ |= 0x00040000;
creationDate_ = input.readInt64();
break;
}
case 168: {
bitField0_ |= 0x00080000;
closeDate_ = input.readInt64();
break;
}
case 176: {
bitField0_ |= 0x00100000;
updateDate_ = input.readInt64();
break;
}
case 184: {
bitField0_ |= 0x00200000;
selectedAt_ = input.readInt64();
break;
}
case 194: {
bitField0_ |= 0x00400000;
diffFields_ = input.readBytes();
break;
}
case 200: {
bitField0_ |= 0x00800000;
isChanged_ = input.readBool();
break;
}
case 208: {
bitField0_ |= 0x01000000;
mustSendNotification_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
tags_ = new com.google.protobuf.UnmodifiableLazyStringList(tags_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Issue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Issue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.batch.protocol.output.BatchReport.Issue.class, org.sonar.batch.protocol.output.BatchReport.Issue.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Issue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Issue(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional string rule_repository = 1;
public static final int RULE_REPOSITORY_FIELD_NUMBER = 1;
private java.lang.Object ruleRepository_;
/**
* optional string rule_repository = 1;
*/
public boolean hasRuleRepository() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string rule_repository = 1;
*/
public java.lang.String getRuleRepository() {
java.lang.Object ref = ruleRepository_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
ruleRepository_ = s;
}
return s;
}
}
/**
* optional string rule_repository = 1;
*/
public com.google.protobuf.ByteString
getRuleRepositoryBytes() {
java.lang.Object ref = ruleRepository_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ruleRepository_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string rule_key = 2;
public static final int RULE_KEY_FIELD_NUMBER = 2;
private java.lang.Object ruleKey_;
/**
* optional string rule_key = 2;
*/
public boolean hasRuleKey() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string rule_key = 2;
*/
public java.lang.String getRuleKey() {
java.lang.Object ref = ruleKey_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
ruleKey_ = s;
}
return s;
}
}
/**
* optional string rule_key = 2;
*/
public com.google.protobuf.ByteString
getRuleKeyBytes() {
java.lang.Object ref = ruleKey_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ruleKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional int32 line = 3;
public static final int LINE_FIELD_NUMBER = 3;
private int line_;
/**
* optional int32 line = 3;
*/
public boolean hasLine() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int32 line = 3;
*/
public int getLine() {
return line_;
}
// optional string msg = 4;
public static final int MSG_FIELD_NUMBER = 4;
private java.lang.Object msg_;
/**
* optional string msg = 4;
*/
public boolean hasMsg() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string msg = 4;
*/
public java.lang.String getMsg() {
java.lang.Object ref = msg_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
msg_ = s;
}
return s;
}
}
/**
* optional string msg = 4;
*/
public com.google.protobuf.ByteString
getMsgBytes() {
java.lang.Object ref = msg_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
msg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional .Severity severity = 5;
public static final int SEVERITY_FIELD_NUMBER = 5;
private org.sonar.batch.protocol.Constants.Severity severity_;
/**
* optional .Severity severity = 5;
*/
public boolean hasSeverity() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .Severity severity = 5;
*/
public org.sonar.batch.protocol.Constants.Severity getSeverity() {
return severity_;
}
// repeated string tags = 6;
public static final int TAGS_FIELD_NUMBER = 6;
private com.google.protobuf.LazyStringList tags_;
/**
* repeated string tags = 6;
*/
public java.util.List
getTagsList() {
return tags_;
}
/**
* repeated string tags = 6;
*/
public int getTagsCount() {
return tags_.size();
}
/**
* repeated string tags = 6;
*/
public java.lang.String getTags(int index) {
return tags_.get(index);
}
/**
* repeated string tags = 6;
*/
public com.google.protobuf.ByteString
getTagsBytes(int index) {
return tags_.getByteString(index);
}
// optional double effort_to_fix = 7;
public static final int EFFORT_TO_FIX_FIELD_NUMBER = 7;
private double effortToFix_;
/**
* optional double effort_to_fix = 7;
*
*
* temporary fields during development of computation stack
*
*/
public boolean hasEffortToFix() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional double effort_to_fix = 7;
*
*
* temporary fields during development of computation stack
*
*/
public double getEffortToFix() {
return effortToFix_;
}
// optional bool is_new = 8;
public static final int IS_NEW_FIELD_NUMBER = 8;
private boolean isNew_;
/**
* optional bool is_new = 8;
*/
public boolean hasIsNew() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bool is_new = 8;
*/
public boolean getIsNew() {
return isNew_;
}
// optional string uuid = 9;
public static final int UUID_FIELD_NUMBER = 9;
private java.lang.Object uuid_;
/**
* optional string uuid = 9;
*/
public boolean hasUuid() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional string uuid = 9;
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
uuid_ = s;
}
return s;
}
}
/**
* optional string uuid = 9;
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional int64 debt_in_minutes = 10;
public static final int DEBT_IN_MINUTES_FIELD_NUMBER = 10;
private long debtInMinutes_;
/**
* optional int64 debt_in_minutes = 10;
*/
public boolean hasDebtInMinutes() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional int64 debt_in_minutes = 10;
*/
public long getDebtInMinutes() {
return debtInMinutes_;
}
// optional string resolution = 11;
public static final int RESOLUTION_FIELD_NUMBER = 11;
private java.lang.Object resolution_;
/**
* optional string resolution = 11;
*/
public boolean hasResolution() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional string resolution = 11;
*/
public java.lang.String getResolution() {
java.lang.Object ref = resolution_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
resolution_ = s;
}
return s;
}
}
/**
* optional string resolution = 11;
*/
public com.google.protobuf.ByteString
getResolutionBytes() {
java.lang.Object ref = resolution_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resolution_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string status = 12;
public static final int STATUS_FIELD_NUMBER = 12;
private java.lang.Object status_;
/**
* optional string status = 12;
*/
public boolean hasStatus() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional string status = 12;
*/
public java.lang.String getStatus() {
java.lang.Object ref = status_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
status_ = s;
}
return s;
}
}
/**
* optional string status = 12;
*/
public com.google.protobuf.ByteString
getStatusBytes() {
java.lang.Object ref = status_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
status_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string checksum = 13;
public static final int CHECKSUM_FIELD_NUMBER = 13;
private java.lang.Object checksum_;
/**
* optional string checksum = 13;
*/
public boolean hasChecksum() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string checksum = 13;
*/
public java.lang.String getChecksum() {
java.lang.Object ref = checksum_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
checksum_ = s;
}
return s;
}
}
/**
* optional string checksum = 13;
*/
public com.google.protobuf.ByteString
getChecksumBytes() {
java.lang.Object ref = checksum_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
checksum_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional bool manual_severity = 14;
public static final int MANUAL_SEVERITY_FIELD_NUMBER = 14;
private boolean manualSeverity_;
/**
* optional bool manual_severity = 14;
*/
public boolean hasManualSeverity() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional bool manual_severity = 14;
*/
public boolean getManualSeverity() {
return manualSeverity_;
}
// optional string reporter = 15;
public static final int REPORTER_FIELD_NUMBER = 15;
private java.lang.Object reporter_;
/**
* optional string reporter = 15;
*/
public boolean hasReporter() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional string reporter = 15;
*/
public java.lang.String getReporter() {
java.lang.Object ref = reporter_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
reporter_ = s;
}
return s;
}
}
/**
* optional string reporter = 15;
*/
public com.google.protobuf.ByteString
getReporterBytes() {
java.lang.Object ref = reporter_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reporter_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string assignee = 16;
public static final int ASSIGNEE_FIELD_NUMBER = 16;
private java.lang.Object assignee_;
/**
* optional string assignee = 16;
*/
public boolean hasAssignee() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional string assignee = 16;
*/
public java.lang.String getAssignee() {
java.lang.Object ref = assignee_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
assignee_ = s;
}
return s;
}
}
/**
* optional string assignee = 16;
*/
public com.google.protobuf.ByteString
getAssigneeBytes() {
java.lang.Object ref = assignee_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
assignee_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string action_plan_key = 17;
public static final int ACTION_PLAN_KEY_FIELD_NUMBER = 17;
private java.lang.Object actionPlanKey_;
/**
* optional string action_plan_key = 17;
*/
public boolean hasActionPlanKey() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional string action_plan_key = 17;
*/
public java.lang.String getActionPlanKey() {
java.lang.Object ref = actionPlanKey_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
actionPlanKey_ = s;
}
return s;
}
}
/**
* optional string action_plan_key = 17;
*/
public com.google.protobuf.ByteString
getActionPlanKeyBytes() {
java.lang.Object ref = actionPlanKey_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
actionPlanKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string attributes = 18;
public static final int ATTRIBUTES_FIELD_NUMBER = 18;
private java.lang.Object attributes_;
/**
* optional string attributes = 18;
*/
public boolean hasAttributes() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional string attributes = 18;
*/
public java.lang.String getAttributes() {
java.lang.Object ref = attributes_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
attributes_ = s;
}
return s;
}
}
/**
* optional string attributes = 18;
*/
public com.google.protobuf.ByteString
getAttributesBytes() {
java.lang.Object ref = attributes_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
attributes_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string author_login = 19;
public static final int AUTHOR_LOGIN_FIELD_NUMBER = 19;
private java.lang.Object authorLogin_;
/**
* optional string author_login = 19;
*/
public boolean hasAuthorLogin() {
return ((bitField0_ & 0x00020000) == 0x00020000);
}
/**
* optional string author_login = 19;
*/
public java.lang.String getAuthorLogin() {
java.lang.Object ref = authorLogin_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
authorLogin_ = s;
}
return s;
}
}
/**
* optional string author_login = 19;
*/
public com.google.protobuf.ByteString
getAuthorLoginBytes() {
java.lang.Object ref = authorLogin_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
authorLogin_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional int64 creation_date = 20;
public static final int CREATION_DATE_FIELD_NUMBER = 20;
private long creationDate_;
/**
* optional int64 creation_date = 20;
*/
public boolean hasCreationDate() {
return ((bitField0_ & 0x00040000) == 0x00040000);
}
/**
* optional int64 creation_date = 20;
*/
public long getCreationDate() {
return creationDate_;
}
// optional int64 close_date = 21;
public static final int CLOSE_DATE_FIELD_NUMBER = 21;
private long closeDate_;
/**
* optional int64 close_date = 21;
*/
public boolean hasCloseDate() {
return ((bitField0_ & 0x00080000) == 0x00080000);
}
/**
* optional int64 close_date = 21;
*/
public long getCloseDate() {
return closeDate_;
}
// optional int64 update_date = 22;
public static final int UPDATE_DATE_FIELD_NUMBER = 22;
private long updateDate_;
/**
* optional int64 update_date = 22;
*/
public boolean hasUpdateDate() {
return ((bitField0_ & 0x00100000) == 0x00100000);
}
/**
* optional int64 update_date = 22;
*/
public long getUpdateDate() {
return updateDate_;
}
// optional int64 selected_at = 23;
public static final int SELECTED_AT_FIELD_NUMBER = 23;
private long selectedAt_;
/**
* optional int64 selected_at = 23;
*/
public boolean hasSelectedAt() {
return ((bitField0_ & 0x00200000) == 0x00200000);
}
/**
* optional int64 selected_at = 23;
*/
public long getSelectedAt() {
return selectedAt_;
}
// optional string diff_fields = 24;
public static final int DIFF_FIELDS_FIELD_NUMBER = 24;
private java.lang.Object diffFields_;
/**
* optional string diff_fields = 24;
*/
public boolean hasDiffFields() {
return ((bitField0_ & 0x00400000) == 0x00400000);
}
/**
* optional string diff_fields = 24;
*/
public java.lang.String getDiffFields() {
java.lang.Object ref = diffFields_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
diffFields_ = s;
}
return s;
}
}
/**
* optional string diff_fields = 24;
*/
public com.google.protobuf.ByteString
getDiffFieldsBytes() {
java.lang.Object ref = diffFields_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
diffFields_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional bool is_changed = 25;
public static final int IS_CHANGED_FIELD_NUMBER = 25;
private boolean isChanged_;
/**
* optional bool is_changed = 25;
*/
public boolean hasIsChanged() {
return ((bitField0_ & 0x00800000) == 0x00800000);
}
/**
* optional bool is_changed = 25;
*/
public boolean getIsChanged() {
return isChanged_;
}
// optional bool must_send_notification = 26;
public static final int MUST_SEND_NOTIFICATION_FIELD_NUMBER = 26;
private boolean mustSendNotification_;
/**
* optional bool must_send_notification = 26;
*/
public boolean hasMustSendNotification() {
return ((bitField0_ & 0x01000000) == 0x01000000);
}
/**
* optional bool must_send_notification = 26;
*/
public boolean getMustSendNotification() {
return mustSendNotification_;
}
private void initFields() {
ruleRepository_ = "";
ruleKey_ = "";
line_ = 0;
msg_ = "";
severity_ = org.sonar.batch.protocol.Constants.Severity.INFO;
tags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
effortToFix_ = 0D;
isNew_ = false;
uuid_ = "";
debtInMinutes_ = 0L;
resolution_ = "";
status_ = "";
checksum_ = "";
manualSeverity_ = false;
reporter_ = "";
assignee_ = "";
actionPlanKey_ = "";
attributes_ = "";
authorLogin_ = "";
creationDate_ = 0L;
closeDate_ = 0L;
updateDate_ = 0L;
selectedAt_ = 0L;
diffFields_ = "";
isChanged_ = false;
mustSendNotification_ = false;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getRuleRepositoryBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getRuleKeyBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt32(3, line_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, getMsgBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeEnum(5, severity_.getNumber());
}
for (int i = 0; i < tags_.size(); i++) {
output.writeBytes(6, tags_.getByteString(i));
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeDouble(7, effortToFix_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBool(8, isNew_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeBytes(9, getUuidBytes());
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeInt64(10, debtInMinutes_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeBytes(11, getResolutionBytes());
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
output.writeBytes(12, getStatusBytes());
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
output.writeBytes(13, getChecksumBytes());
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
output.writeBool(14, manualSeverity_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
output.writeBytes(15, getReporterBytes());
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
output.writeBytes(16, getAssigneeBytes());
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
output.writeBytes(17, getActionPlanKeyBytes());
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
output.writeBytes(18, getAttributesBytes());
}
if (((bitField0_ & 0x00020000) == 0x00020000)) {
output.writeBytes(19, getAuthorLoginBytes());
}
if (((bitField0_ & 0x00040000) == 0x00040000)) {
output.writeInt64(20, creationDate_);
}
if (((bitField0_ & 0x00080000) == 0x00080000)) {
output.writeInt64(21, closeDate_);
}
if (((bitField0_ & 0x00100000) == 0x00100000)) {
output.writeInt64(22, updateDate_);
}
if (((bitField0_ & 0x00200000) == 0x00200000)) {
output.writeInt64(23, selectedAt_);
}
if (((bitField0_ & 0x00400000) == 0x00400000)) {
output.writeBytes(24, getDiffFieldsBytes());
}
if (((bitField0_ & 0x00800000) == 0x00800000)) {
output.writeBool(25, isChanged_);
}
if (((bitField0_ & 0x01000000) == 0x01000000)) {
output.writeBool(26, mustSendNotification_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getRuleRepositoryBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getRuleKeyBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, line_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getMsgBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, severity_.getNumber());
}
{
int dataSize = 0;
for (int i = 0; i < tags_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(tags_.getByteString(i));
}
size += dataSize;
size += 1 * getTagsList().size();
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(7, effortToFix_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(8, isNew_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(9, getUuidBytes());
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(10, debtInMinutes_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(11, getResolutionBytes());
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(12, getStatusBytes());
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(13, getChecksumBytes());
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(14, manualSeverity_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(15, getReporterBytes());
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(16, getAssigneeBytes());
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(17, getActionPlanKeyBytes());
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(18, getAttributesBytes());
}
if (((bitField0_ & 0x00020000) == 0x00020000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(19, getAuthorLoginBytes());
}
if (((bitField0_ & 0x00040000) == 0x00040000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(20, creationDate_);
}
if (((bitField0_ & 0x00080000) == 0x00080000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(21, closeDate_);
}
if (((bitField0_ & 0x00100000) == 0x00100000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(22, updateDate_);
}
if (((bitField0_ & 0x00200000) == 0x00200000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(23, selectedAt_);
}
if (((bitField0_ & 0x00400000) == 0x00400000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(24, getDiffFieldsBytes());
}
if (((bitField0_ & 0x00800000) == 0x00800000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(25, isChanged_);
}
if (((bitField0_ & 0x01000000) == 0x01000000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(26, mustSendNotification_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.sonar.batch.protocol.output.BatchReport.Issue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.batch.protocol.output.BatchReport.Issue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.batch.protocol.output.BatchReport.Issue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.batch.protocol.output.BatchReport.Issue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.batch.protocol.output.BatchReport.Issue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.batch.protocol.output.BatchReport.Issue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.batch.protocol.output.BatchReport.Issue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.batch.protocol.output.BatchReport.Issue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.batch.protocol.output.BatchReport.Issue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.batch.protocol.output.BatchReport.Issue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.sonar.batch.protocol.output.BatchReport.Issue prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Issue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.sonar.batch.protocol.output.BatchReport.IssueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Issue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Issue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.batch.protocol.output.BatchReport.Issue.class, org.sonar.batch.protocol.output.BatchReport.Issue.Builder.class);
}
// Construct using org.sonar.batch.protocol.output.BatchReport.Issue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
ruleRepository_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
ruleKey_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
line_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
msg_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
severity_ = org.sonar.batch.protocol.Constants.Severity.INFO;
bitField0_ = (bitField0_ & ~0x00000010);
tags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
effortToFix_ = 0D;
bitField0_ = (bitField0_ & ~0x00000040);
isNew_ = false;
bitField0_ = (bitField0_ & ~0x00000080);
uuid_ = "";
bitField0_ = (bitField0_ & ~0x00000100);
debtInMinutes_ = 0L;
bitField0_ = (bitField0_ & ~0x00000200);
resolution_ = "";
bitField0_ = (bitField0_ & ~0x00000400);
status_ = "";
bitField0_ = (bitField0_ & ~0x00000800);
checksum_ = "";
bitField0_ = (bitField0_ & ~0x00001000);
manualSeverity_ = false;
bitField0_ = (bitField0_ & ~0x00002000);
reporter_ = "";
bitField0_ = (bitField0_ & ~0x00004000);
assignee_ = "";
bitField0_ = (bitField0_ & ~0x00008000);
actionPlanKey_ = "";
bitField0_ = (bitField0_ & ~0x00010000);
attributes_ = "";
bitField0_ = (bitField0_ & ~0x00020000);
authorLogin_ = "";
bitField0_ = (bitField0_ & ~0x00040000);
creationDate_ = 0L;
bitField0_ = (bitField0_ & ~0x00080000);
closeDate_ = 0L;
bitField0_ = (bitField0_ & ~0x00100000);
updateDate_ = 0L;
bitField0_ = (bitField0_ & ~0x00200000);
selectedAt_ = 0L;
bitField0_ = (bitField0_ & ~0x00400000);
diffFields_ = "";
bitField0_ = (bitField0_ & ~0x00800000);
isChanged_ = false;
bitField0_ = (bitField0_ & ~0x01000000);
mustSendNotification_ = false;
bitField0_ = (bitField0_ & ~0x02000000);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Issue_descriptor;
}
public org.sonar.batch.protocol.output.BatchReport.Issue getDefaultInstanceForType() {
return org.sonar.batch.protocol.output.BatchReport.Issue.getDefaultInstance();
}
public org.sonar.batch.protocol.output.BatchReport.Issue build() {
org.sonar.batch.protocol.output.BatchReport.Issue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.batch.protocol.output.BatchReport.Issue buildPartial() {
org.sonar.batch.protocol.output.BatchReport.Issue result = new org.sonar.batch.protocol.output.BatchReport.Issue(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.ruleRepository_ = ruleRepository_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.ruleKey_ = ruleKey_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.line_ = line_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.msg_ = msg_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.severity_ = severity_;
if (((bitField0_ & 0x00000020) == 0x00000020)) {
tags_ = new com.google.protobuf.UnmodifiableLazyStringList(
tags_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.tags_ = tags_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000020;
}
result.effortToFix_ = effortToFix_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000040;
}
result.isNew_ = isNew_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000080;
}
result.uuid_ = uuid_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000100;
}
result.debtInMinutes_ = debtInMinutes_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000200;
}
result.resolution_ = resolution_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000400;
}
result.status_ = status_;
if (((from_bitField0_ & 0x00001000) == 0x00001000)) {
to_bitField0_ |= 0x00000800;
}
result.checksum_ = checksum_;
if (((from_bitField0_ & 0x00002000) == 0x00002000)) {
to_bitField0_ |= 0x00001000;
}
result.manualSeverity_ = manualSeverity_;
if (((from_bitField0_ & 0x00004000) == 0x00004000)) {
to_bitField0_ |= 0x00002000;
}
result.reporter_ = reporter_;
if (((from_bitField0_ & 0x00008000) == 0x00008000)) {
to_bitField0_ |= 0x00004000;
}
result.assignee_ = assignee_;
if (((from_bitField0_ & 0x00010000) == 0x00010000)) {
to_bitField0_ |= 0x00008000;
}
result.actionPlanKey_ = actionPlanKey_;
if (((from_bitField0_ & 0x00020000) == 0x00020000)) {
to_bitField0_ |= 0x00010000;
}
result.attributes_ = attributes_;
if (((from_bitField0_ & 0x00040000) == 0x00040000)) {
to_bitField0_ |= 0x00020000;
}
result.authorLogin_ = authorLogin_;
if (((from_bitField0_ & 0x00080000) == 0x00080000)) {
to_bitField0_ |= 0x00040000;
}
result.creationDate_ = creationDate_;
if (((from_bitField0_ & 0x00100000) == 0x00100000)) {
to_bitField0_ |= 0x00080000;
}
result.closeDate_ = closeDate_;
if (((from_bitField0_ & 0x00200000) == 0x00200000)) {
to_bitField0_ |= 0x00100000;
}
result.updateDate_ = updateDate_;
if (((from_bitField0_ & 0x00400000) == 0x00400000)) {
to_bitField0_ |= 0x00200000;
}
result.selectedAt_ = selectedAt_;
if (((from_bitField0_ & 0x00800000) == 0x00800000)) {
to_bitField0_ |= 0x00400000;
}
result.diffFields_ = diffFields_;
if (((from_bitField0_ & 0x01000000) == 0x01000000)) {
to_bitField0_ |= 0x00800000;
}
result.isChanged_ = isChanged_;
if (((from_bitField0_ & 0x02000000) == 0x02000000)) {
to_bitField0_ |= 0x01000000;
}
result.mustSendNotification_ = mustSendNotification_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.batch.protocol.output.BatchReport.Issue) {
return mergeFrom((org.sonar.batch.protocol.output.BatchReport.Issue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.batch.protocol.output.BatchReport.Issue other) {
if (other == org.sonar.batch.protocol.output.BatchReport.Issue.getDefaultInstance()) return this;
if (other.hasRuleRepository()) {
bitField0_ |= 0x00000001;
ruleRepository_ = other.ruleRepository_;
onChanged();
}
if (other.hasRuleKey()) {
bitField0_ |= 0x00000002;
ruleKey_ = other.ruleKey_;
onChanged();
}
if (other.hasLine()) {
setLine(other.getLine());
}
if (other.hasMsg()) {
bitField0_ |= 0x00000008;
msg_ = other.msg_;
onChanged();
}
if (other.hasSeverity()) {
setSeverity(other.getSeverity());
}
if (!other.tags_.isEmpty()) {
if (tags_.isEmpty()) {
tags_ = other.tags_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureTagsIsMutable();
tags_.addAll(other.tags_);
}
onChanged();
}
if (other.hasEffortToFix()) {
setEffortToFix(other.getEffortToFix());
}
if (other.hasIsNew()) {
setIsNew(other.getIsNew());
}
if (other.hasUuid()) {
bitField0_ |= 0x00000100;
uuid_ = other.uuid_;
onChanged();
}
if (other.hasDebtInMinutes()) {
setDebtInMinutes(other.getDebtInMinutes());
}
if (other.hasResolution()) {
bitField0_ |= 0x00000400;
resolution_ = other.resolution_;
onChanged();
}
if (other.hasStatus()) {
bitField0_ |= 0x00000800;
status_ = other.status_;
onChanged();
}
if (other.hasChecksum()) {
bitField0_ |= 0x00001000;
checksum_ = other.checksum_;
onChanged();
}
if (other.hasManualSeverity()) {
setManualSeverity(other.getManualSeverity());
}
if (other.hasReporter()) {
bitField0_ |= 0x00004000;
reporter_ = other.reporter_;
onChanged();
}
if (other.hasAssignee()) {
bitField0_ |= 0x00008000;
assignee_ = other.assignee_;
onChanged();
}
if (other.hasActionPlanKey()) {
bitField0_ |= 0x00010000;
actionPlanKey_ = other.actionPlanKey_;
onChanged();
}
if (other.hasAttributes()) {
bitField0_ |= 0x00020000;
attributes_ = other.attributes_;
onChanged();
}
if (other.hasAuthorLogin()) {
bitField0_ |= 0x00040000;
authorLogin_ = other.authorLogin_;
onChanged();
}
if (other.hasCreationDate()) {
setCreationDate(other.getCreationDate());
}
if (other.hasCloseDate()) {
setCloseDate(other.getCloseDate());
}
if (other.hasUpdateDate()) {
setUpdateDate(other.getUpdateDate());
}
if (other.hasSelectedAt()) {
setSelectedAt(other.getSelectedAt());
}
if (other.hasDiffFields()) {
bitField0_ |= 0x00800000;
diffFields_ = other.diffFields_;
onChanged();
}
if (other.hasIsChanged()) {
setIsChanged(other.getIsChanged());
}
if (other.hasMustSendNotification()) {
setMustSendNotification(other.getMustSendNotification());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.batch.protocol.output.BatchReport.Issue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.batch.protocol.output.BatchReport.Issue) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string rule_repository = 1;
private java.lang.Object ruleRepository_ = "";
/**
* optional string rule_repository = 1;
*/
public boolean hasRuleRepository() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string rule_repository = 1;
*/
public java.lang.String getRuleRepository() {
java.lang.Object ref = ruleRepository_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
ruleRepository_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string rule_repository = 1;
*/
public com.google.protobuf.ByteString
getRuleRepositoryBytes() {
java.lang.Object ref = ruleRepository_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ruleRepository_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string rule_repository = 1;
*/
public Builder setRuleRepository(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
ruleRepository_ = value;
onChanged();
return this;
}
/**
* optional string rule_repository = 1;
*/
public Builder clearRuleRepository() {
bitField0_ = (bitField0_ & ~0x00000001);
ruleRepository_ = getDefaultInstance().getRuleRepository();
onChanged();
return this;
}
/**
* optional string rule_repository = 1;
*/
public Builder setRuleRepositoryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
ruleRepository_ = value;
onChanged();
return this;
}
// optional string rule_key = 2;
private java.lang.Object ruleKey_ = "";
/**
* optional string rule_key = 2;
*/
public boolean hasRuleKey() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string rule_key = 2;
*/
public java.lang.String getRuleKey() {
java.lang.Object ref = ruleKey_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
ruleKey_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string rule_key = 2;
*/
public com.google.protobuf.ByteString
getRuleKeyBytes() {
java.lang.Object ref = ruleKey_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ruleKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string rule_key = 2;
*/
public Builder setRuleKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
ruleKey_ = value;
onChanged();
return this;
}
/**
* optional string rule_key = 2;
*/
public Builder clearRuleKey() {
bitField0_ = (bitField0_ & ~0x00000002);
ruleKey_ = getDefaultInstance().getRuleKey();
onChanged();
return this;
}
/**
* optional string rule_key = 2;
*/
public Builder setRuleKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
ruleKey_ = value;
onChanged();
return this;
}
// optional int32 line = 3;
private int line_ ;
/**
* optional int32 line = 3;
*/
public boolean hasLine() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int32 line = 3;
*/
public int getLine() {
return line_;
}
/**
* optional int32 line = 3;
*/
public Builder setLine(int value) {
bitField0_ |= 0x00000004;
line_ = value;
onChanged();
return this;
}
/**
* optional int32 line = 3;
*/
public Builder clearLine() {
bitField0_ = (bitField0_ & ~0x00000004);
line_ = 0;
onChanged();
return this;
}
// optional string msg = 4;
private java.lang.Object msg_ = "";
/**
* optional string msg = 4;
*/
public boolean hasMsg() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string msg = 4;
*/
public java.lang.String getMsg() {
java.lang.Object ref = msg_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
msg_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string msg = 4;
*/
public com.google.protobuf.ByteString
getMsgBytes() {
java.lang.Object ref = msg_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
msg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string msg = 4;
*/
public Builder setMsg(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
msg_ = value;
onChanged();
return this;
}
/**
* optional string msg = 4;
*/
public Builder clearMsg() {
bitField0_ = (bitField0_ & ~0x00000008);
msg_ = getDefaultInstance().getMsg();
onChanged();
return this;
}
/**
* optional string msg = 4;
*/
public Builder setMsgBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
msg_ = value;
onChanged();
return this;
}
// optional .Severity severity = 5;
private org.sonar.batch.protocol.Constants.Severity severity_ = org.sonar.batch.protocol.Constants.Severity.INFO;
/**
* optional .Severity severity = 5;
*/
public boolean hasSeverity() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .Severity severity = 5;
*/
public org.sonar.batch.protocol.Constants.Severity getSeverity() {
return severity_;
}
/**
* optional .Severity severity = 5;
*/
public Builder setSeverity(org.sonar.batch.protocol.Constants.Severity value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
severity_ = value;
onChanged();
return this;
}
/**
* optional .Severity severity = 5;
*/
public Builder clearSeverity() {
bitField0_ = (bitField0_ & ~0x00000010);
severity_ = org.sonar.batch.protocol.Constants.Severity.INFO;
onChanged();
return this;
}
// repeated string tags = 6;
private com.google.protobuf.LazyStringList tags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureTagsIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
tags_ = new com.google.protobuf.LazyStringArrayList(tags_);
bitField0_ |= 0x00000020;
}
}
/**
* repeated string tags = 6;
*/
public java.util.List
getTagsList() {
return java.util.Collections.unmodifiableList(tags_);
}
/**
* repeated string tags = 6;
*/
public int getTagsCount() {
return tags_.size();
}
/**
* repeated string tags = 6;
*/
public java.lang.String getTags(int index) {
return tags_.get(index);
}
/**
* repeated string tags = 6;
*/
public com.google.protobuf.ByteString
getTagsBytes(int index) {
return tags_.getByteString(index);
}
/**
* repeated string tags = 6;
*/
public Builder setTags(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTagsIsMutable();
tags_.set(index, value);
onChanged();
return this;
}
/**
* repeated string tags = 6;
*/
public Builder addTags(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTagsIsMutable();
tags_.add(value);
onChanged();
return this;
}
/**
* repeated string tags = 6;
*/
public Builder addAllTags(
java.lang.Iterable values) {
ensureTagsIsMutable();
super.addAll(values, tags_);
onChanged();
return this;
}
/**
* repeated string tags = 6;
*/
public Builder clearTags() {
tags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
* repeated string tags = 6;
*/
public Builder addTagsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureTagsIsMutable();
tags_.add(value);
onChanged();
return this;
}
// optional double effort_to_fix = 7;
private double effortToFix_ ;
/**
* optional double effort_to_fix = 7;
*
*
* temporary fields during development of computation stack
*
*/
public boolean hasEffortToFix() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional double effort_to_fix = 7;
*
*
* temporary fields during development of computation stack
*
*/
public double getEffortToFix() {
return effortToFix_;
}
/**
* optional double effort_to_fix = 7;
*
*
* temporary fields during development of computation stack
*
*/
public Builder setEffortToFix(double value) {
bitField0_ |= 0x00000040;
effortToFix_ = value;
onChanged();
return this;
}
/**
* optional double effort_to_fix = 7;
*
*
* temporary fields during development of computation stack
*
*/
public Builder clearEffortToFix() {
bitField0_ = (bitField0_ & ~0x00000040);
effortToFix_ = 0D;
onChanged();
return this;
}
// optional bool is_new = 8;
private boolean isNew_ ;
/**
* optional bool is_new = 8;
*/
public boolean hasIsNew() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bool is_new = 8;
*/
public boolean getIsNew() {
return isNew_;
}
/**
* optional bool is_new = 8;
*/
public Builder setIsNew(boolean value) {
bitField0_ |= 0x00000080;
isNew_ = value;
onChanged();
return this;
}
/**
* optional bool is_new = 8;
*/
public Builder clearIsNew() {
bitField0_ = (bitField0_ & ~0x00000080);
isNew_ = false;
onChanged();
return this;
}
// optional string uuid = 9;
private java.lang.Object uuid_ = "";
/**
* optional string uuid = 9;
*/
public boolean hasUuid() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional string uuid = 9;
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
uuid_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string uuid = 9;
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string uuid = 9;
*/
public Builder setUuid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
uuid_ = value;
onChanged();
return this;
}
/**
* optional string uuid = 9;
*/
public Builder clearUuid() {
bitField0_ = (bitField0_ & ~0x00000100);
uuid_ = getDefaultInstance().getUuid();
onChanged();
return this;
}
/**
* optional string uuid = 9;
*/
public Builder setUuidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
uuid_ = value;
onChanged();
return this;
}
// optional int64 debt_in_minutes = 10;
private long debtInMinutes_ ;
/**
* optional int64 debt_in_minutes = 10;
*/
public boolean hasDebtInMinutes() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional int64 debt_in_minutes = 10;
*/
public long getDebtInMinutes() {
return debtInMinutes_;
}
/**
* optional int64 debt_in_minutes = 10;
*/
public Builder setDebtInMinutes(long value) {
bitField0_ |= 0x00000200;
debtInMinutes_ = value;
onChanged();
return this;
}
/**
* optional int64 debt_in_minutes = 10;
*/
public Builder clearDebtInMinutes() {
bitField0_ = (bitField0_ & ~0x00000200);
debtInMinutes_ = 0L;
onChanged();
return this;
}
// optional string resolution = 11;
private java.lang.Object resolution_ = "";
/**
* optional string resolution = 11;
*/
public boolean hasResolution() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional string resolution = 11;
*/
public java.lang.String getResolution() {
java.lang.Object ref = resolution_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
resolution_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string resolution = 11;
*/
public com.google.protobuf.ByteString
getResolutionBytes() {
java.lang.Object ref = resolution_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resolution_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string resolution = 11;
*/
public Builder setResolution(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
resolution_ = value;
onChanged();
return this;
}
/**
* optional string resolution = 11;
*/
public Builder clearResolution() {
bitField0_ = (bitField0_ & ~0x00000400);
resolution_ = getDefaultInstance().getResolution();
onChanged();
return this;
}
/**
* optional string resolution = 11;
*/
public Builder setResolutionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
resolution_ = value;
onChanged();
return this;
}
// optional string status = 12;
private java.lang.Object status_ = "";
/**
* optional string status = 12;
*/
public boolean hasStatus() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string status = 12;
*/
public java.lang.String getStatus() {
java.lang.Object ref = status_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
status_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string status = 12;
*/
public com.google.protobuf.ByteString
getStatusBytes() {
java.lang.Object ref = status_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
status_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string status = 12;
*/
public Builder setStatus(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
status_ = value;
onChanged();
return this;
}
/**
* optional string status = 12;
*/
public Builder clearStatus() {
bitField0_ = (bitField0_ & ~0x00000800);
status_ = getDefaultInstance().getStatus();
onChanged();
return this;
}
/**
* optional string status = 12;
*/
public Builder setStatusBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
status_ = value;
onChanged();
return this;
}
// optional string checksum = 13;
private java.lang.Object checksum_ = "";
/**
* optional string checksum = 13;
*/
public boolean hasChecksum() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional string checksum = 13;
*/
public java.lang.String getChecksum() {
java.lang.Object ref = checksum_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
checksum_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string checksum = 13;
*/
public com.google.protobuf.ByteString
getChecksumBytes() {
java.lang.Object ref = checksum_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
checksum_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string checksum = 13;
*/
public Builder setChecksum(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
checksum_ = value;
onChanged();
return this;
}
/**
* optional string checksum = 13;
*/
public Builder clearChecksum() {
bitField0_ = (bitField0_ & ~0x00001000);
checksum_ = getDefaultInstance().getChecksum();
onChanged();
return this;
}
/**
* optional string checksum = 13;
*/
public Builder setChecksumBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
checksum_ = value;
onChanged();
return this;
}
// optional bool manual_severity = 14;
private boolean manualSeverity_ ;
/**
* optional bool manual_severity = 14;
*/
public boolean hasManualSeverity() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional bool manual_severity = 14;
*/
public boolean getManualSeverity() {
return manualSeverity_;
}
/**
* optional bool manual_severity = 14;
*/
public Builder setManualSeverity(boolean value) {
bitField0_ |= 0x00002000;
manualSeverity_ = value;
onChanged();
return this;
}
/**
* optional bool manual_severity = 14;
*/
public Builder clearManualSeverity() {
bitField0_ = (bitField0_ & ~0x00002000);
manualSeverity_ = false;
onChanged();
return this;
}
// optional string reporter = 15;
private java.lang.Object reporter_ = "";
/**
* optional string reporter = 15;
*/
public boolean hasReporter() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional string reporter = 15;
*/
public java.lang.String getReporter() {
java.lang.Object ref = reporter_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
reporter_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string reporter = 15;
*/
public com.google.protobuf.ByteString
getReporterBytes() {
java.lang.Object ref = reporter_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reporter_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string reporter = 15;
*/
public Builder setReporter(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00004000;
reporter_ = value;
onChanged();
return this;
}
/**
* optional string reporter = 15;
*/
public Builder clearReporter() {
bitField0_ = (bitField0_ & ~0x00004000);
reporter_ = getDefaultInstance().getReporter();
onChanged();
return this;
}
/**
* optional string reporter = 15;
*/
public Builder setReporterBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00004000;
reporter_ = value;
onChanged();
return this;
}
// optional string assignee = 16;
private java.lang.Object assignee_ = "";
/**
* optional string assignee = 16;
*/
public boolean hasAssignee() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional string assignee = 16;
*/
public java.lang.String getAssignee() {
java.lang.Object ref = assignee_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
assignee_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string assignee = 16;
*/
public com.google.protobuf.ByteString
getAssigneeBytes() {
java.lang.Object ref = assignee_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
assignee_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string assignee = 16;
*/
public Builder setAssignee(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00008000;
assignee_ = value;
onChanged();
return this;
}
/**
* optional string assignee = 16;
*/
public Builder clearAssignee() {
bitField0_ = (bitField0_ & ~0x00008000);
assignee_ = getDefaultInstance().getAssignee();
onChanged();
return this;
}
/**
* optional string assignee = 16;
*/
public Builder setAssigneeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00008000;
assignee_ = value;
onChanged();
return this;
}
// optional string action_plan_key = 17;
private java.lang.Object actionPlanKey_ = "";
/**
* optional string action_plan_key = 17;
*/
public boolean hasActionPlanKey() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional string action_plan_key = 17;
*/
public java.lang.String getActionPlanKey() {
java.lang.Object ref = actionPlanKey_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
actionPlanKey_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string action_plan_key = 17;
*/
public com.google.protobuf.ByteString
getActionPlanKeyBytes() {
java.lang.Object ref = actionPlanKey_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
actionPlanKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string action_plan_key = 17;
*/
public Builder setActionPlanKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00010000;
actionPlanKey_ = value;
onChanged();
return this;
}
/**
* optional string action_plan_key = 17;
*/
public Builder clearActionPlanKey() {
bitField0_ = (bitField0_ & ~0x00010000);
actionPlanKey_ = getDefaultInstance().getActionPlanKey();
onChanged();
return this;
}
/**
* optional string action_plan_key = 17;
*/
public Builder setActionPlanKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00010000;
actionPlanKey_ = value;
onChanged();
return this;
}
// optional string attributes = 18;
private java.lang.Object attributes_ = "";
/**
* optional string attributes = 18;
*/
public boolean hasAttributes() {
return ((bitField0_ & 0x00020000) == 0x00020000);
}
/**
* optional string attributes = 18;
*/
public java.lang.String getAttributes() {
java.lang.Object ref = attributes_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
attributes_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string attributes = 18;
*/
public com.google.protobuf.ByteString
getAttributesBytes() {
java.lang.Object ref = attributes_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
attributes_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string attributes = 18;
*/
public Builder setAttributes(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00020000;
attributes_ = value;
onChanged();
return this;
}
/**
* optional string attributes = 18;
*/
public Builder clearAttributes() {
bitField0_ = (bitField0_ & ~0x00020000);
attributes_ = getDefaultInstance().getAttributes();
onChanged();
return this;
}
/**
* optional string attributes = 18;
*/
public Builder setAttributesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00020000;
attributes_ = value;
onChanged();
return this;
}
// optional string author_login = 19;
private java.lang.Object authorLogin_ = "";
/**
* optional string author_login = 19;
*/
public boolean hasAuthorLogin() {
return ((bitField0_ & 0x00040000) == 0x00040000);
}
/**
* optional string author_login = 19;
*/
public java.lang.String getAuthorLogin() {
java.lang.Object ref = authorLogin_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
authorLogin_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string author_login = 19;
*/
public com.google.protobuf.ByteString
getAuthorLoginBytes() {
java.lang.Object ref = authorLogin_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
authorLogin_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string author_login = 19;
*/
public Builder setAuthorLogin(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00040000;
authorLogin_ = value;
onChanged();
return this;
}
/**
* optional string author_login = 19;
*/
public Builder clearAuthorLogin() {
bitField0_ = (bitField0_ & ~0x00040000);
authorLogin_ = getDefaultInstance().getAuthorLogin();
onChanged();
return this;
}
/**
* optional string author_login = 19;
*/
public Builder setAuthorLoginBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00040000;
authorLogin_ = value;
onChanged();
return this;
}
// optional int64 creation_date = 20;
private long creationDate_ ;
/**
* optional int64 creation_date = 20;
*/
public boolean hasCreationDate() {
return ((bitField0_ & 0x00080000) == 0x00080000);
}
/**
* optional int64 creation_date = 20;
*/
public long getCreationDate() {
return creationDate_;
}
/**
* optional int64 creation_date = 20;
*/
public Builder setCreationDate(long value) {
bitField0_ |= 0x00080000;
creationDate_ = value;
onChanged();
return this;
}
/**
* optional int64 creation_date = 20;
*/
public Builder clearCreationDate() {
bitField0_ = (bitField0_ & ~0x00080000);
creationDate_ = 0L;
onChanged();
return this;
}
// optional int64 close_date = 21;
private long closeDate_ ;
/**
* optional int64 close_date = 21;
*/
public boolean hasCloseDate() {
return ((bitField0_ & 0x00100000) == 0x00100000);
}
/**
* optional int64 close_date = 21;
*/
public long getCloseDate() {
return closeDate_;
}
/**
* optional int64 close_date = 21;
*/
public Builder setCloseDate(long value) {
bitField0_ |= 0x00100000;
closeDate_ = value;
onChanged();
return this;
}
/**
* optional int64 close_date = 21;
*/
public Builder clearCloseDate() {
bitField0_ = (bitField0_ & ~0x00100000);
closeDate_ = 0L;
onChanged();
return this;
}
// optional int64 update_date = 22;
private long updateDate_ ;
/**
* optional int64 update_date = 22;
*/
public boolean hasUpdateDate() {
return ((bitField0_ & 0x00200000) == 0x00200000);
}
/**
* optional int64 update_date = 22;
*/
public long getUpdateDate() {
return updateDate_;
}
/**
* optional int64 update_date = 22;
*/
public Builder setUpdateDate(long value) {
bitField0_ |= 0x00200000;
updateDate_ = value;
onChanged();
return this;
}
/**
* optional int64 update_date = 22;
*/
public Builder clearUpdateDate() {
bitField0_ = (bitField0_ & ~0x00200000);
updateDate_ = 0L;
onChanged();
return this;
}
// optional int64 selected_at = 23;
private long selectedAt_ ;
/**
* optional int64 selected_at = 23;
*/
public boolean hasSelectedAt() {
return ((bitField0_ & 0x00400000) == 0x00400000);
}
/**
* optional int64 selected_at = 23;
*/
public long getSelectedAt() {
return selectedAt_;
}
/**
* optional int64 selected_at = 23;
*/
public Builder setSelectedAt(long value) {
bitField0_ |= 0x00400000;
selectedAt_ = value;
onChanged();
return this;
}
/**
* optional int64 selected_at = 23;
*/
public Builder clearSelectedAt() {
bitField0_ = (bitField0_ & ~0x00400000);
selectedAt_ = 0L;
onChanged();
return this;
}
// optional string diff_fields = 24;
private java.lang.Object diffFields_ = "";
/**
* optional string diff_fields = 24;
*/
public boolean hasDiffFields() {
return ((bitField0_ & 0x00800000) == 0x00800000);
}
/**
* optional string diff_fields = 24;
*/
public java.lang.String getDiffFields() {
java.lang.Object ref = diffFields_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
diffFields_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string diff_fields = 24;
*/
public com.google.protobuf.ByteString
getDiffFieldsBytes() {
java.lang.Object ref = diffFields_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
diffFields_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string diff_fields = 24;
*/
public Builder setDiffFields(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00800000;
diffFields_ = value;
onChanged();
return this;
}
/**
* optional string diff_fields = 24;
*/
public Builder clearDiffFields() {
bitField0_ = (bitField0_ & ~0x00800000);
diffFields_ = getDefaultInstance().getDiffFields();
onChanged();
return this;
}
/**
* optional string diff_fields = 24;
*/
public Builder setDiffFieldsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00800000;
diffFields_ = value;
onChanged();
return this;
}
// optional bool is_changed = 25;
private boolean isChanged_ ;
/**
* optional bool is_changed = 25;
*/
public boolean hasIsChanged() {
return ((bitField0_ & 0x01000000) == 0x01000000);
}
/**
* optional bool is_changed = 25;
*/
public boolean getIsChanged() {
return isChanged_;
}
/**
* optional bool is_changed = 25;
*/
public Builder setIsChanged(boolean value) {
bitField0_ |= 0x01000000;
isChanged_ = value;
onChanged();
return this;
}
/**
* optional bool is_changed = 25;
*/
public Builder clearIsChanged() {
bitField0_ = (bitField0_ & ~0x01000000);
isChanged_ = false;
onChanged();
return this;
}
// optional bool must_send_notification = 26;
private boolean mustSendNotification_ ;
/**
* optional bool must_send_notification = 26;
*/
public boolean hasMustSendNotification() {
return ((bitField0_ & 0x02000000) == 0x02000000);
}
/**
* optional bool must_send_notification = 26;
*/
public boolean getMustSendNotification() {
return mustSendNotification_;
}
/**
* optional bool must_send_notification = 26;
*/
public Builder setMustSendNotification(boolean value) {
bitField0_ |= 0x02000000;
mustSendNotification_ = value;
onChanged();
return this;
}
/**
* optional bool must_send_notification = 26;
*/
public Builder clearMustSendNotification() {
bitField0_ = (bitField0_ & ~0x02000000);
mustSendNotification_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:Issue)
}
static {
defaultInstance = new Issue(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:Issue)
}
public interface IssuesOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional int32 component_ref = 1;
/**
* optional int32 component_ref = 1;
*/
boolean hasComponentRef();
/**
* optional int32 component_ref = 1;
*/
int getComponentRef();
// repeated .Issue list = 2;
/**
* repeated .Issue list = 2;
*/
java.util.List
getListList();
/**
* repeated .Issue list = 2;
*/
org.sonar.batch.protocol.output.BatchReport.Issue getList(int index);
/**
* repeated .Issue list = 2;
*/
int getListCount();
/**
* repeated .Issue list = 2;
*/
java.util.List extends org.sonar.batch.protocol.output.BatchReport.IssueOrBuilder>
getListOrBuilderList();
/**
* repeated .Issue list = 2;
*/
org.sonar.batch.protocol.output.BatchReport.IssueOrBuilder getListOrBuilder(
int index);
// optional string component_uuid = 3;
/**
* optional string component_uuid = 3;
*
*
* Temporary field for issues on deleted components
*
*/
boolean hasComponentUuid();
/**
* optional string component_uuid = 3;
*
*
* Temporary field for issues on deleted components
*
*/
java.lang.String getComponentUuid();
/**
* optional string component_uuid = 3;
*
*
* Temporary field for issues on deleted components
*
*/
com.google.protobuf.ByteString
getComponentUuidBytes();
}
/**
* Protobuf type {@code Issues}
*/
public static final class Issues extends
com.google.protobuf.GeneratedMessage
implements IssuesOrBuilder {
// Use Issues.newBuilder() to construct.
private Issues(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Issues(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Issues defaultInstance;
public static Issues getDefaultInstance() {
return defaultInstance;
}
public Issues getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Issues(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
componentRef_ = input.readInt32();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
list_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
list_.add(input.readMessage(org.sonar.batch.protocol.output.BatchReport.Issue.PARSER, extensionRegistry));
break;
}
case 26: {
bitField0_ |= 0x00000002;
componentUuid_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
list_ = java.util.Collections.unmodifiableList(list_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Issues_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Issues_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.batch.protocol.output.BatchReport.Issues.class, org.sonar.batch.protocol.output.BatchReport.Issues.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Issues parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Issues(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional int32 component_ref = 1;
public static final int COMPONENT_REF_FIELD_NUMBER = 1;
private int componentRef_;
/**
* optional int32 component_ref = 1;
*/
public boolean hasComponentRef() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 component_ref = 1;
*/
public int getComponentRef() {
return componentRef_;
}
// repeated .Issue list = 2;
public static final int LIST_FIELD_NUMBER = 2;
private java.util.List list_;
/**
* repeated .Issue list = 2;
*/
public java.util.List getListList() {
return list_;
}
/**
* repeated .Issue list = 2;
*/
public java.util.List extends org.sonar.batch.protocol.output.BatchReport.IssueOrBuilder>
getListOrBuilderList() {
return list_;
}
/**
* repeated .Issue list = 2;
*/
public int getListCount() {
return list_.size();
}
/**
* repeated .Issue list = 2;
*/
public org.sonar.batch.protocol.output.BatchReport.Issue getList(int index) {
return list_.get(index);
}
/**
* repeated .Issue list = 2;
*/
public org.sonar.batch.protocol.output.BatchReport.IssueOrBuilder getListOrBuilder(
int index) {
return list_.get(index);
}
// optional string component_uuid = 3;
public static final int COMPONENT_UUID_FIELD_NUMBER = 3;
private java.lang.Object componentUuid_;
/**
* optional string component_uuid = 3;
*
*
* Temporary field for issues on deleted components
*
*/
public boolean hasComponentUuid() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string component_uuid = 3;
*
*
* Temporary field for issues on deleted components
*
*/
public java.lang.String getComponentUuid() {
java.lang.Object ref = componentUuid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
componentUuid_ = s;
}
return s;
}
}
/**
* optional string component_uuid = 3;
*
*
* Temporary field for issues on deleted components
*
*/
public com.google.protobuf.ByteString
getComponentUuidBytes() {
java.lang.Object ref = componentUuid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
componentUuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
componentRef_ = 0;
list_ = java.util.Collections.emptyList();
componentUuid_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, componentRef_);
}
for (int i = 0; i < list_.size(); i++) {
output.writeMessage(2, list_.get(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(3, getComponentUuidBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, componentRef_);
}
for (int i = 0; i < list_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, list_.get(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getComponentUuidBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.sonar.batch.protocol.output.BatchReport.Issues parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.batch.protocol.output.BatchReport.Issues parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.batch.protocol.output.BatchReport.Issues parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.batch.protocol.output.BatchReport.Issues parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.batch.protocol.output.BatchReport.Issues parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.batch.protocol.output.BatchReport.Issues parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.batch.protocol.output.BatchReport.Issues parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.batch.protocol.output.BatchReport.Issues parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.batch.protocol.output.BatchReport.Issues parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.batch.protocol.output.BatchReport.Issues parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.sonar.batch.protocol.output.BatchReport.Issues prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Issues}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.sonar.batch.protocol.output.BatchReport.IssuesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Issues_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Issues_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.batch.protocol.output.BatchReport.Issues.class, org.sonar.batch.protocol.output.BatchReport.Issues.Builder.class);
}
// Construct using org.sonar.batch.protocol.output.BatchReport.Issues.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getListFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
componentRef_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
if (listBuilder_ == null) {
list_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
listBuilder_.clear();
}
componentUuid_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.batch.protocol.output.BatchReport.internal_static_Issues_descriptor;
}
public org.sonar.batch.protocol.output.BatchReport.Issues getDefaultInstanceForType() {
return org.sonar.batch.protocol.output.BatchReport.Issues.getDefaultInstance();
}
public org.sonar.batch.protocol.output.BatchReport.Issues build() {
org.sonar.batch.protocol.output.BatchReport.Issues result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.batch.protocol.output.BatchReport.Issues buildPartial() {
org.sonar.batch.protocol.output.BatchReport.Issues result = new org.sonar.batch.protocol.output.BatchReport.Issues(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.componentRef_ = componentRef_;
if (listBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
list_ = java.util.Collections.unmodifiableList(list_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.list_ = list_;
} else {
result.list_ = listBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000002;
}
result.componentUuid_ = componentUuid_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.batch.protocol.output.BatchReport.Issues) {
return mergeFrom((org.sonar.batch.protocol.output.BatchReport.Issues)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.batch.protocol.output.BatchReport.Issues other) {
if (other == org.sonar.batch.protocol.output.BatchReport.Issues.getDefaultInstance()) return this;
if (other.hasComponentRef()) {
setComponentRef(other.getComponentRef());
}
if (listBuilder_ == null) {
if (!other.list_.isEmpty()) {
if (list_.isEmpty()) {
list_ = other.list_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureListIsMutable();
list_.addAll(other.list_);
}
onChanged();
}
} else {
if (!other.list_.isEmpty()) {
if (listBuilder_.isEmpty()) {
listBuilder_.dispose();
listBuilder_ = null;
list_ = other.list_;
bitField0_ = (bitField0_ & ~0x00000002);
listBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getListFieldBuilder() : null;
} else {
listBuilder_.addAllMessages(other.list_);
}
}
}
if (other.hasComponentUuid()) {
bitField0_ |= 0x00000004;
componentUuid_ = other.componentUuid_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.batch.protocol.output.BatchReport.Issues parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.batch.protocol.output.BatchReport.Issues) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional int32 component_ref = 1;
private int componentRef_ ;
/**
* optional int32 component_ref = 1;
*/
public boolean hasComponentRef() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 component_ref = 1;
*/
public int getComponentRef() {
return componentRef_;
}
/**
* optional int32 component_ref = 1;
*/
public Builder setComponentRef(int value) {
bitField0_ |= 0x00000001;
componentRef_ = value;
onChanged();
return this;
}
/**
* optional int32 component_ref = 1;
*/
public Builder clearComponentRef() {
bitField0_ = (bitField0_ & ~0x00000001);
componentRef_ = 0;
onChanged();
return this;
}
// repeated .Issue list = 2;
private java.util.List list_ =
java.util.Collections.emptyList();
private void ensureListIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
list_ = new java.util.ArrayList(list_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonar.batch.protocol.output.BatchReport.Issue, org.sonar.batch.protocol.output.BatchReport.Issue.Builder, org.sonar.batch.protocol.output.BatchReport.IssueOrBuilder> listBuilder_;
/**
* repeated .Issue list = 2;
*/
public java.util.List getListList() {
if (listBuilder_ == null) {
return java.util.Collections.unmodifiableList(list_);
} else {
return listBuilder_.getMessageList();
}
}
/**
* repeated .Issue list = 2;
*/
public int getListCount() {
if (listBuilder_ == null) {
return list_.size();
} else {
return listBuilder_.getCount();
}
}
/**
* repeated .Issue list = 2;
*/
public org.sonar.batch.protocol.output.BatchReport.Issue getList(int index) {
if (listBuilder_ == null) {
return list_.get(index);
} else {
return listBuilder_.getMessage(index);
}
}
/**
* repeated .Issue list = 2;
*/
public Builder setList(
int index, org.sonar.batch.protocol.output.BatchReport.Issue value) {
if (listBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureListIsMutable();
list_.set(index, value);
onChanged();
} else {
listBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .Issue list = 2;
*/
public Builder setList(
int index, org.sonar.batch.protocol.output.BatchReport.Issue.Builder builderForValue) {
if (listBuilder_ == null) {
ensureListIsMutable();
list_.set(index, builderForValue.build());
onChanged();
} else {
listBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .Issue list = 2;
*/
public Builder addList(org.sonar.batch.protocol.output.BatchReport.Issue value) {
if (listBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureListIsMutable();
list_.add(value);
onChanged();
} else {
listBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .Issue list = 2;
*/
public Builder addList(
int index, org.sonar.batch.protocol.output.BatchReport.Issue value) {
if (listBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureListIsMutable();
list_.add(index, value);
onChanged();
} else {
listBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .Issue list = 2;
*/
public Builder addList(
org.sonar.batch.protocol.output.BatchReport.Issue.Builder builderForValue) {
if (listBuilder_ == null) {
ensureListIsMutable();
list_.add(builderForValue.build());
onChanged();
} else {
listBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .Issue list = 2;
*/
public Builder addList(
int index, org.sonar.batch.protocol.output.BatchReport.Issue.Builder builderForValue) {
if (listBuilder_ == null) {
ensureListIsMutable();
list_.add(index, builderForValue.build());
onChanged();
} else {
listBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .Issue list = 2;
*/
public Builder addAllList(
java.lang.Iterable extends org.sonar.batch.protocol.output.BatchReport.Issue> values) {
if (listBuilder_ == null) {
ensureListIsMutable();
super.addAll(values, list_);
onChanged();
} else {
listBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .Issue list = 2;
*/
public Builder clearList() {
if (listBuilder_ == null) {
list_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
listBuilder_.clear();
}
return this;
}
/**
* repeated .Issue list = 2;
*/
public Builder removeList(int index) {
if (listBuilder_ == null) {
ensureListIsMutable();
list_.remove(index);
onChanged();
} else {
listBuilder_.remove(index);
}
return this;
}
/**
* repeated .Issue list = 2;
*/
public org.sonar.batch.protocol.output.BatchReport.Issue.Builder getListBuilder(
int index) {
return getListFieldBuilder().getBuilder(index);
}
/**
* repeated .Issue list = 2;
*/
public org.sonar.batch.protocol.output.BatchReport.IssueOrBuilder getListOrBuilder(
int index) {
if (listBuilder_ == null) {
return list_.get(index); } else {
return listBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .Issue list = 2;
*/
public java.util.List extends org.sonar.batch.protocol.output.BatchReport.IssueOrBuilder>
getListOrBuilderList() {
if (listBuilder_ != null) {
return listBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(list_);
}
}
/**
* repeated .Issue list = 2;
*/
public org.sonar.batch.protocol.output.BatchReport.Issue.Builder addListBuilder() {
return getListFieldBuilder().addBuilder(
org.sonar.batch.protocol.output.BatchReport.Issue.getDefaultInstance());
}
/**
* repeated .Issue list = 2;
*/
public org.sonar.batch.protocol.output.BatchReport.Issue.Builder addListBuilder(
int index) {
return getListFieldBuilder().addBuilder(
index, org.sonar.batch.protocol.output.BatchReport.Issue.getDefaultInstance());
}
/**
* repeated .Issue list = 2;
*/
public java.util.List
getListBuilderList() {
return getListFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonar.batch.protocol.output.BatchReport.Issue, org.sonar.batch.protocol.output.BatchReport.Issue.Builder, org.sonar.batch.protocol.output.BatchReport.IssueOrBuilder>
getListFieldBuilder() {
if (listBuilder_ == null) {
listBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.sonar.batch.protocol.output.BatchReport.Issue, org.sonar.batch.protocol.output.BatchReport.Issue.Builder, org.sonar.batch.protocol.output.BatchReport.IssueOrBuilder>(
list_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
list_ = null;
}
return listBuilder_;
}
// optional string component_uuid = 3;
private java.lang.Object componentUuid_ = "";
/**
* optional string component_uuid = 3;
*
*
* Temporary field for issues on deleted components
*
*/
public boolean hasComponentUuid() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string component_uuid = 3;
*
*
* Temporary field for issues on deleted components
*
*/
public java.lang.String getComponentUuid() {
java.lang.Object ref = componentUuid_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
componentUuid_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string component_uuid = 3;
*
*
* Temporary field for issues on deleted components
*
*/
public com.google.protobuf.ByteString
getComponentUuidBytes() {
java.lang.Object ref = componentUuid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
componentUuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string component_uuid = 3;
*
*
* Temporary field for issues on deleted components
*
*/
public Builder setComponentUuid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
componentUuid_ = value;
onChanged();
return this;
}
/**
* optional string component_uuid = 3;
*
*
* Temporary field for issues on deleted components
*
*/
public Builder clearComponentUuid() {
bitField0_ = (bitField0_ & ~0x00000004);
componentUuid_ = getDefaultInstance().getComponentUuid();
onChanged();
return this;
}
/**
* optional string component_uuid = 3;
*
*
* Temporary field for issues on deleted components
*
*/
public Builder setComponentUuidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
componentUuid_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:Issues)
}
static {
defaultInstance = new Issues(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:Issues)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Metadata_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Metadata_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Component_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Component_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Issue_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Issue_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Issues_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Issues_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\022batch_report.proto\032\017constants.proto\"\211\001" +
"\n\010Metadata\022\025\n\ranalysis_date\030\001 \001(\003\022\023\n\013pro" +
"ject_key\030\002 \001(\t\022\032\n\022root_component_ref\030\003 \001" +
"(\005\022\023\n\013snapshot_id\030\004 \001(\003\022 \n\030deleted_compo" +
"nents_count\030\005 \001(\005\"\260\001\n\tComponent\022\013\n\003ref\030\001" +
" \001(\005\022\014\n\004path\030\002 \001(\t\022\014\n\004name\030\003 \001(\t\022\034\n\004type" +
"\030\004 \001(\0162\016.ComponentType\022\017\n\007is_test\030\005 \001(\010\022" +
"\020\n\010language\030\006 \001(\t\022\026\n\nchild_refs\030\007 \003(\005B\002\020" +
"\001\022\023\n\013snapshot_id\030\010 \001(\005\022\014\n\004uuid\030\t \001(\t\"\232\004\n" +
"\005Issue\022\027\n\017rule_repository\030\001 \001(\t\022\020\n\010rule_",
"key\030\002 \001(\t\022\014\n\004line\030\003 \001(\005\022\013\n\003msg\030\004 \001(\t\022\033\n\010" +
"severity\030\005 \001(\0162\t.Severity\022\014\n\004tags\030\006 \003(\t\022" +
"\025\n\reffort_to_fix\030\007 \001(\001\022\016\n\006is_new\030\010 \001(\010\022\014" +
"\n\004uuid\030\t \001(\t\022\027\n\017debt_in_minutes\030\n \001(\003\022\022\n" +
"\nresolution\030\013 \001(\t\022\016\n\006status\030\014 \001(\t\022\020\n\010che" +
"cksum\030\r \001(\t\022\027\n\017manual_severity\030\016 \001(\010\022\020\n\010" +
"reporter\030\017 \001(\t\022\020\n\010assignee\030\020 \001(\t\022\027\n\017acti" +
"on_plan_key\030\021 \001(\t\022\022\n\nattributes\030\022 \001(\t\022\024\n" +
"\014author_login\030\023 \001(\t\022\025\n\rcreation_date\030\024 \001" +
"(\003\022\022\n\nclose_date\030\025 \001(\003\022\023\n\013update_date\030\026 ",
"\001(\003\022\023\n\013selected_at\030\027 \001(\003\022\023\n\013diff_fields\030" +
"\030 \001(\t\022\022\n\nis_changed\030\031 \001(\010\022\036\n\026must_send_n" +
"otification\030\032 \001(\010\"M\n\006Issues\022\025\n\rcomponent" +
"_ref\030\001 \001(\005\022\024\n\004list\030\002 \003(\0132\006.Issue\022\026\n\016comp" +
"onent_uuid\030\003 \001(\tB#\n\037org.sonar.batch.prot" +
"ocol.outputH\001"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_Metadata_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_Metadata_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Metadata_descriptor,
new java.lang.String[] { "AnalysisDate", "ProjectKey", "RootComponentRef", "SnapshotId", "DeletedComponentsCount", });
internal_static_Component_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_Component_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Component_descriptor,
new java.lang.String[] { "Ref", "Path", "Name", "Type", "IsTest", "Language", "ChildRefs", "SnapshotId", "Uuid", });
internal_static_Issue_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_Issue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Issue_descriptor,
new java.lang.String[] { "RuleRepository", "RuleKey", "Line", "Msg", "Severity", "Tags", "EffortToFix", "IsNew", "Uuid", "DebtInMinutes", "Resolution", "Status", "Checksum", "ManualSeverity", "Reporter", "Assignee", "ActionPlanKey", "Attributes", "AuthorLogin", "CreationDate", "CloseDate", "UpdateDate", "SelectedAt", "DiffFields", "IsChanged", "MustSendNotification", });
internal_static_Issues_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_Issues_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Issues_descriptor,
new java.lang.String[] { "ComponentRef", "List", "ComponentUuid", });
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
org.sonar.batch.protocol.Constants.getDescriptor(),
}, assigner);
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy