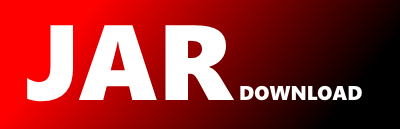
org.sonar.maven.DependenciesInjectionMojo Maven / Gradle / Ivy
/*
* Sonar, open source software quality management tool.
* Copyright (C) 2009 SonarSource SA
* mailto:contact AT sonarsource DOT com
*
* Sonar is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* Sonar is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Sonar; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02
*/
package org.sonar.maven;
import org.apache.maven.model.Repository;
import org.apache.maven.model.RepositoryPolicy;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.project.MavenProject;
import org.sonar.commons.ServerHttpClient;
import org.sonar.plugins.api.maven.model.MavenPlugin;
import org.sonar.plugins.api.maven.model.MavenPom;
import java.io.IOException;
import java.util.List;
/**
* @goal dependencies
*/
public class DependenciesInjectionMojo extends AbstractMojo {
/**
* The maven project running this plugin
* @parameter expression="${project}"
* @required
* @readonly
*/
private MavenProject mavenProject;
/**
* Sonar host URL
* @parameter expression="${sonar.host.url}" default-value="http://localhost:9000" alias="sonar.host.url"
*/
private String sonarHostURL;
/**
* Sonar server version
* @parameter expression="${sonar.server.version}" alias="sonar.server.version"
*/
private String sonarServerVersion;
private ServerHttpClient serverHttpClient;
public DependenciesInjectionMojo() {
}
public void setServiceApi( ServerHttpClient serverHttpClient) {
this.serverHttpClient = serverHttpClient;
}
public void setMavenProject(MavenProject mavenProject) {
this.mavenProject = mavenProject;
}
public void execute() throws MojoExecutionException {
if ( serverHttpClient == null ) {
serverHttpClient = new ServerHttpClient( sonarHostURL );
}
try {
preparePomForSonar();
} catch (IOException e) {
throw new RuntimeException("Can't access to sonar server", e);
}
}
private void preparePomForSonar() throws MojoExecutionException, IOException {
// adding the repository
List repositories = mavenProject.getRepositories();
for (Repository repository : repositories) {
if ("sonar".equals(repository.getId())) {
throw new MojoExecutionException("Pom already contains a repository with id 'sonar', please remove it");
}
}
Repository sonarRepository = new Repository();
sonarRepository.setId("sonar");
sonarRepository.setName("Sonar server maven repository");
RepositoryPolicy releasePolicy = new RepositoryPolicy();
releasePolicy.setChecksumPolicy("ignore");
releasePolicy.setEnabled(true);
releasePolicy.setUpdatePolicy("never");
sonarRepository.setReleases(releasePolicy);
RepositoryPolicy snapshotPolicy = new RepositoryPolicy();
snapshotPolicy.setEnabled(false);
sonarRepository.setSnapshots(snapshotPolicy);
sonarRepository.setUrl(serverHttpClient.getMavenRepositoryUrl());
repositories.add(sonarRepository);
MavenPom pom = new MavenPom(mavenProject);
MavenPlugin plugin = pom.findOrCreateBuildPlugin("org.codehaus.sonar", "sonar-core-maven-plugin", sonarServerVersion);
String serverId = serverHttpClient.getId();
plugin.addDependency("org.codehaus.sonar.runtime.jdbc-driver", "parent", serverId, "pom");
plugin.addDependency("org.codehaus.sonar.runtime.plugins", "parent", serverId, "pom");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy