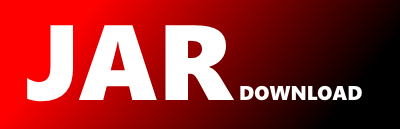
ch.hortis.sonar.mvn.SonarRemoteServices Maven / Gradle / Ivy
/*
* Sonar, entreprise quality control tool.
* Copyright (C) 2007-2008 Hortis-GRC SA
* mailto:be_agile HAT hortis DOT ch
*
* Sonar is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* Sonar is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Sonar; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02
*/
package ch.hortis.sonar.mvn;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class SonarRemoteServices {
private String remoteHostURL;
private static final int CONNECT_TIMEOUT_MILLISECONDS = 10000;
private static final int READ_TIMEOUT_MILLISECONDS = 15000;
public SonarRemoteServices(String remoteHostURL) {
this.remoteHostURL = remoteHostURL;
if ( this.remoteHostURL.endsWith("/") ) {
this.remoteHostURL = this.remoteHostURL.substring(0,this.remoteHostURL.length() -1);
}
}
public boolean ping() {
ByteArrayOutputStream target = new ByteArrayOutputStream();
try {
callRemoteFile("/remote_services/ping", target);
} catch (IOException e) {
return false;
}
return new String(target.toByteArray()).equals("ping");
}
public String getVersion() throws IOException {
ByteArrayOutputStream target = new ByteArrayOutputStream();
callRemoteFile("/remote_services/version", target);
String version = new String(target.toByteArray());
if ( version.trim().length() == 0 ) {
throw new IOException("Empty version returned from server");
}
return new String(target.toByteArray());
}
private InputStream getRemoteFile(URL url) throws IOException {
HttpURLConnection conn = (HttpURLConnection)url.openConnection();
conn.setConnectTimeout(CONNECT_TIMEOUT_MILLISECONDS);
conn.setReadTimeout(READ_TIMEOUT_MILLISECONDS);
conn.connect();
return conn.getInputStream();
}
private void callRemoteFile(String filePathOnServer, OutputStream target) throws IOException {
String fullFileURL = remoteHostURL + filePathOnServer;
try {
InputStream in = getRemoteFile( new URL(fullFileURL) );
byte[] buffer = new byte[64];
int readen;
while ( ( readen = in.read( buffer ) ) != -1 ) {
target.write( buffer, 0, readen );
}
in.close();
target.close();
} catch (IOException ex) {
IOException ioEx = new IOException("Error occured during file " + fullFileURL + " download");
ioEx.initCause(ex);
throw ioEx;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy