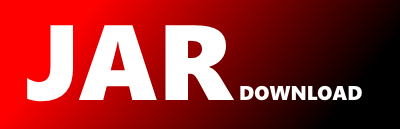
org.codelibs.fess.es.config.bsbhv.BsFailureUrlBhv Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fess Show documentation
Show all versions of fess Show documentation
Fess is Full tExt Search System.
/*
* Copyright 2012-2020 CodeLibs Project and the Others.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.codelibs.fess.es.config.bsbhv;
import java.util.List;
import java.util.Map;
import org.codelibs.fess.es.config.allcommon.EsAbstractBehavior;
import org.codelibs.fess.es.config.allcommon.EsAbstractEntity.RequestOptionCall;
import org.codelibs.fess.es.config.bsentity.dbmeta.FailureUrlDbm;
import org.codelibs.fess.es.config.cbean.FailureUrlCB;
import org.codelibs.fess.es.config.exentity.FailureUrl;
import org.dbflute.Entity;
import org.dbflute.bhv.readable.CBCall;
import org.dbflute.bhv.readable.EntityRowHandler;
import org.dbflute.cbean.ConditionBean;
import org.dbflute.cbean.result.ListResultBean;
import org.dbflute.cbean.result.PagingResultBean;
import org.dbflute.exception.IllegalBehaviorStateException;
import org.dbflute.optional.OptionalEntity;
import org.dbflute.util.DfTypeUtil;
import org.elasticsearch.action.bulk.BulkRequestBuilder;
import org.elasticsearch.action.delete.DeleteRequestBuilder;
import org.elasticsearch.action.index.IndexRequestBuilder;
/**
* @author ESFlute (using FreeGen)
*/
public abstract class BsFailureUrlBhv extends EsAbstractBehavior {
// ===================================================================================
// Control Override
// ================
@Override
public String asTableDbName() {
return asEsIndexType();
}
@Override
protected String asEsIndex() {
return ".fess_config.failure_url";
}
@Override
public String asEsIndexType() {
return "failure_url";
}
@Override
public String asEsSearchType() {
return "failure_url";
}
@Override
public FailureUrlDbm asDBMeta() {
return FailureUrlDbm.getInstance();
}
@Override
protected RESULT createEntity(Map source, Class extends RESULT> entityType) {
try {
final RESULT result = entityType.newInstance();
result.setConfigId(DfTypeUtil.toString(source.get("configId")));
result.setErrorCount(DfTypeUtil.toInteger(source.get("errorCount")));
result.setErrorLog(DfTypeUtil.toString(source.get("errorLog")));
result.setErrorName(DfTypeUtil.toString(source.get("errorName")));
result.setLastAccessTime(DfTypeUtil.toLong(source.get("lastAccessTime")));
result.setThreadName(DfTypeUtil.toString(source.get("threadName")));
result.setUrl(DfTypeUtil.toString(source.get("url")));
return updateEntity(source, result);
} catch (InstantiationException | IllegalAccessException e) {
final String msg = "Cannot create a new instance: " + entityType.getName();
throw new IllegalBehaviorStateException(msg, e);
}
}
protected RESULT updateEntity(Map source, RESULT result) {
return result;
}
// ===================================================================================
// Select
// ======
public int selectCount(CBCall cbLambda) {
return facadeSelectCount(createCB(cbLambda));
}
public OptionalEntity selectEntity(CBCall cbLambda) {
return facadeSelectEntity(createCB(cbLambda));
}
protected OptionalEntity facadeSelectEntity(FailureUrlCB cb) {
return doSelectOptionalEntity(cb, typeOfSelectedEntity());
}
protected OptionalEntity doSelectOptionalEntity(FailureUrlCB cb, Class extends ENTITY> tp) {
return createOptionalEntity(doSelectEntity(cb, tp), cb);
}
@Override
public FailureUrlCB newConditionBean() {
return new FailureUrlCB();
}
@Override
protected Entity doReadEntity(ConditionBean cb) {
return facadeSelectEntity(downcast(cb)).orElse(null);
}
public FailureUrl selectEntityWithDeletedCheck(CBCall cbLambda) {
return facadeSelectEntityWithDeletedCheck(createCB(cbLambda));
}
public OptionalEntity selectByPK(String id) {
return facadeSelectByPK(id);
}
protected OptionalEntity facadeSelectByPK(String id) {
return doSelectOptionalByPK(id, typeOfSelectedEntity());
}
protected ENTITY doSelectByPK(String id, Class extends ENTITY> tp) {
return doSelectEntity(xprepareCBAsPK(id), tp);
}
protected FailureUrlCB xprepareCBAsPK(String id) {
assertObjectNotNull("id", id);
return newConditionBean().acceptPK(id);
}
protected OptionalEntity doSelectOptionalByPK(String id, Class extends ENTITY> tp) {
return createOptionalEntity(doSelectByPK(id, tp), id);
}
@Override
protected Class extends FailureUrl> typeOfSelectedEntity() {
return FailureUrl.class;
}
@Override
protected Class typeOfHandlingEntity() {
return FailureUrl.class;
}
@Override
protected Class typeOfHandlingConditionBean() {
return FailureUrlCB.class;
}
public ListResultBean selectList(CBCall cbLambda) {
return facadeSelectList(createCB(cbLambda));
}
public PagingResultBean selectPage(CBCall cbLambda) {
// #pending same?
return (PagingResultBean) facadeSelectList(createCB(cbLambda));
}
public void selectCursor(CBCall cbLambda, EntityRowHandler entityLambda) {
facadeSelectCursor(createCB(cbLambda), entityLambda);
}
public void selectBulk(CBCall cbLambda, EntityRowHandler> entityLambda) {
delegateSelectBulk(createCB(cbLambda), entityLambda, typeOfSelectedEntity());
}
// ===================================================================================
// Update
// ======
public void insert(FailureUrl entity) {
doInsert(entity, null);
}
public void insert(FailureUrl entity, RequestOptionCall opLambda) {
entity.asDocMeta().indexOption(opLambda);
doInsert(entity, null);
}
public void update(FailureUrl entity) {
doUpdate(entity, null);
}
public void update(FailureUrl entity, RequestOptionCall opLambda) {
entity.asDocMeta().indexOption(opLambda);
doUpdate(entity, null);
}
public void insertOrUpdate(FailureUrl entity) {
doInsertOrUpdate(entity, null, null);
}
public void insertOrUpdate(FailureUrl entity, RequestOptionCall opLambda) {
entity.asDocMeta().indexOption(opLambda);
doInsertOrUpdate(entity, null, null);
}
public void delete(FailureUrl entity) {
doDelete(entity, null);
}
public void delete(FailureUrl entity, RequestOptionCall opLambda) {
entity.asDocMeta().deleteOption(opLambda);
doDelete(entity, null);
}
public int queryDelete(CBCall cbLambda) {
return doQueryDelete(createCB(cbLambda), null);
}
public int[] batchInsert(List list) {
return batchInsert(list, null, null);
}
public int[] batchInsert(List list, RequestOptionCall call) {
return batchInsert(list, call, null);
}
public int[] batchInsert(List list, RequestOptionCall call,
RequestOptionCall entityCall) {
return doBatchInsert(new BulkList<>(list, call, entityCall), null);
}
public int[] batchUpdate(List list) {
return batchUpdate(list, null, null);
}
public int[] batchUpdate(List list, RequestOptionCall call) {
return batchUpdate(list, call, null);
}
public int[] batchUpdate(List list, RequestOptionCall call,
RequestOptionCall entityCall) {
return doBatchUpdate(new BulkList<>(list, call, entityCall), null);
}
public int[] batchDelete(List list) {
return batchDelete(list, null, null);
}
public int[] batchDelete(List list, RequestOptionCall call) {
return batchDelete(list, call, null);
}
public int[] batchDelete(List list, RequestOptionCall call,
RequestOptionCall entityCall) {
return doBatchDelete(new BulkList<>(list, call, entityCall), null);
}
// #pending create, modify, remove
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy