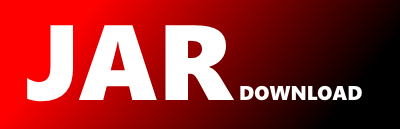
org.codelibs.fess.app.web.api.ApiResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fess Show documentation
Show all versions of fess Show documentation
Fess is Full tExt Search System.
/*
* Copyright 2012-2019 CodeLibs Project and the Others.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.codelibs.fess.app.web.api;
import java.util.HashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.stream.Collectors;
import org.codelibs.fess.entity.SearchRenderData;
import org.codelibs.fess.mylasta.action.FessMessages;
import org.codelibs.fess.util.ComponentUtil;
import org.codelibs.fess.util.FacetResponse;
import org.lastaflute.web.util.LaRequestUtil;
import org.lastaflute.web.validation.VaMessenger;
public class ApiResult {
protected ApiResponse response = null;
public ApiResult(final ApiResponse response) {
this.response = response;
}
public enum Status {
OK(0), BAD_REQUEST(1), SYSTEM_ERROR(2), UNAUTHORIZED(3);
private final int id;
private Status(final int id) {
this.id = id;
}
public int getId() {
return id;
}
}
public static class ApiResponse {
protected String version = ComponentUtil.getSystemHelper().getProductVersion();
protected int status;
public ApiResponse status(final Status status) {
this.status = status.getId();
return this;
}
public ApiResult result() {
return new ApiResult(this);
}
}
public static class ApiUpdateResponse extends ApiResponse {
protected String id;
protected boolean created;
public ApiUpdateResponse id(final String id) {
this.id = id;
return this;
}
public ApiUpdateResponse created(final boolean created) {
this.created = created;
return this;
}
@Override
public ApiResult result() {
return new ApiResult(this);
}
}
public static class ApiDeleteResponse extends ApiResponse {
protected long count = 1;
public ApiDeleteResponse count(final long count) {
this.count = count;
return this;
}
@Override
public ApiResult result() {
return new ApiResult(this);
}
}
public static class ApiConfigResponse extends ApiResponse {
protected Object setting;
public ApiConfigResponse setting(final Object setting) {
this.setting = setting;
return this;
}
@Override
public ApiResult result() {
return new ApiResult(this);
}
}
public static class ApiConfigsResponse extends ApiResponse {
protected List settings;
protected long total = 0;
public ApiConfigsResponse settings(final List settings) {
this.settings = settings;
this.total = settings.size();
return this;
}
public ApiConfigsResponse total(final long total) {
this.total = total;
return this;
}
@Override
public ApiResult result() {
return new ApiResult(this);
}
}
public static class ApiDocResponse extends ApiResponse {
protected Object doc;
public ApiDocResponse doc(final Object doc) {
this.doc = doc;
return this;
}
@Override
public ApiResult result() {
return new ApiResult(this);
}
}
public static class ApiDocsResponse extends ApiResponse {
protected String queryId;
protected List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy