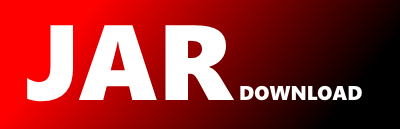
org.codelibs.fess.helper.RelatedContentHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fess Show documentation
Show all versions of fess Show documentation
Fess is Full tExt Search System.
/*
* Copyright 2012-2019 CodeLibs Project and the Others.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.codelibs.fess.helper;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.regex.Pattern;
import javax.annotation.PostConstruct;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.codelibs.core.lang.StringUtil;
import org.codelibs.core.misc.Pair;
import org.codelibs.fess.es.config.exbhv.RelatedContentBhv;
import org.codelibs.fess.es.config.exentity.RelatedContent;
import org.codelibs.fess.util.ComponentUtil;
public class RelatedContentHelper {
private static final Logger logger = LogManager.getLogger(RelatedContentHelper.class);
protected volatile Map, List>>> relatedContentMap = Collections.emptyMap();
protected String regexPrefix = "regex:";
protected String queryPlaceHolder = "__QUERY__";
@PostConstruct
public void init() {
if (logger.isDebugEnabled()) {
logger.debug("Initialize {}", this.getClass().getSimpleName());
}
reload();
}
public int update() {
return reload();
}
public List getAvailableRelatedContentList() {
return ComponentUtil.getComponent(RelatedContentBhv.class).selectList(cb -> {
cb.query().matchAll();
cb.query().addOrderBy_SortOrder_Asc();
cb.query().addOrderBy_Term_Asc();
cb.fetchFirst(ComponentUtil.getFessConfig().getPageRelatedcontentMaxFetchSizeAsInteger());
});
}
protected int reload() {
final Map, List>>> relatedContentMap = new HashMap<>();
getAvailableRelatedContentList().stream().forEach(entity -> {
final String key = getHostKey(entity);
Pair
© 2015 - 2025 Weber Informatics LLC | Privacy Policy