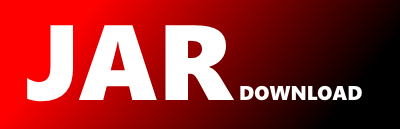
com.o19s.es.ltr.rest.RestAddFeatureToSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch-learning-to-rank Show documentation
Show all versions of elasticsearch-learning-to-rank Show documentation
Learing to Rank Query w/ RankLib Models
/*
* Copyright [2017] Wikimedia Foundation
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.o19s.es.ltr.rest;
import com.o19s.es.ltr.action.AddFeaturesToSetAction;
import com.o19s.es.ltr.action.AddFeaturesToSetAction.AddFeaturesToSetRequestBuilder;
import com.o19s.es.ltr.feature.FeatureValidation;
import com.o19s.es.ltr.feature.store.StoredFeature;
import org.elasticsearch.client.node.NodeClient;
import org.elasticsearch.common.ParseField;
import org.elasticsearch.common.settings.Settings;
import org.elasticsearch.common.xcontent.ObjectParser;
import org.elasticsearch.common.xcontent.XContentParser;
import org.elasticsearch.rest.RestController;
import org.elasticsearch.rest.RestRequest;
import org.elasticsearch.rest.action.RestStatusToXContentListener;
import java.io.IOException;
import java.util.List;
public class RestAddFeatureToSet extends FeatureStoreBaseRestHandler {
public RestAddFeatureToSet(Settings settings, RestController controller) {
super(settings);
controller.registerHandler(RestRequest.Method.POST, "/_ltr/_featureset/{name}/_addfeatures/{query}", this);
controller.registerHandler(RestRequest.Method.POST, "/_ltr/{store}/_featureset/{name}/_addfeatures/{query}", this);
controller.registerHandler(RestRequest.Method.POST, "/_ltr/_featureset/{name}/_addfeatures", this);
controller.registerHandler(RestRequest.Method.POST, "/_ltr/{store}/_featureset/{name}/_addfeatures", this);
}
@Override
public String getName() {
return "Add a feature to the set of features";
}
@Override
protected RestChannelConsumer prepareRequest(RestRequest request, NodeClient client) throws IOException {
String store = indexName(request);
String setName = request.param("name");
String routing = request.param("routing");
String featureQuery = null;
List features = null;
boolean merge = request.paramAsBoolean("merge", false);
if (request.hasParam("query")) {
featureQuery = request.param("query");
}
FeatureValidation validation = null;
if (request.hasContentOrSourceParam()) {
FeaturesParserState featuresParser = new FeaturesParserState();
request.applyContentParser(featuresParser::parse);
features = featuresParser.features;
validation = featuresParser.validation;
}
if (featureQuery == null && (features == null || features.isEmpty())) {
throw new IllegalArgumentException("features must be provided as a query for the feature store " +
"or in the body, none provided");
}
if (featureQuery != null && (features != null && !features.isEmpty())) {
throw new IllegalArgumentException("features must be provided as a query for the feature store " +
"or directly in the body not both");
}
AddFeaturesToSetRequestBuilder builder = AddFeaturesToSetAction.INSTANCE.newRequestBuilder(client);
builder.request().setStore(store);
builder.request().setFeatureSet(setName);
builder.request().setFeatureNameQuery(featureQuery);
builder.request().setRouting(routing);
builder.request().setFeatures(features);
builder.request().setMerge(merge);
builder.request().setValidation(validation);
return (channel) -> builder.execute(new RestStatusToXContentListener<>(channel, (r) -> r.getResponse().getLocation(routing)));
}
static class FeaturesParserState {
public static final ObjectParser PARSER = new ObjectParser<>("features");
private List features;
private FeatureValidation validation;
static {
PARSER.declareObjectArray(
FeaturesParserState::setFeatures,
(parser, context) -> StoredFeature.parse(parser),
new ParseField("features"));
PARSER.declareObject(
FeaturesParserState::setValidation,
FeatureValidation.PARSER::apply,
new ParseField("validation"));
}
public void parse(XContentParser parser) throws IOException {
PARSER.parse(parser, this, null);
}
List getFeatures() {
return features;
}
public void setFeatures(List features) {
this.features = features;
}
public FeatureValidation getValidation() {
return validation;
}
public void setValidation(FeatureValidation validation) {
this.validation = validation;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy