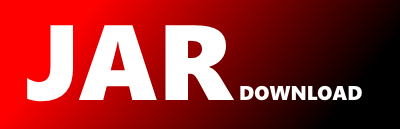
org.codelibs.elasticsearch.index.query.TermsQueryBuilder Maven / Gradle / Ivy
/*
* Licensed to Elasticsearch under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.codelibs.elasticsearch.index.query;
import org.apache.lucene.search.Query;
import org.apache.lucene.util.BytesRef;
import org.apache.lucene.util.BytesRefBuilder;
import org.codelibs.elasticsearch.common.ParseField;
import org.codelibs.elasticsearch.common.ParsingException;
import org.codelibs.elasticsearch.common.Strings;
import org.codelibs.elasticsearch.common.bytes.BytesReference;
import org.codelibs.elasticsearch.common.io.stream.BytesStreamOutput;
import org.codelibs.elasticsearch.common.io.stream.StreamInput;
import org.codelibs.elasticsearch.common.io.stream.StreamOutput;
import org.codelibs.elasticsearch.common.xcontent.XContentBuilder;
import org.codelibs.elasticsearch.common.xcontent.XContentParser;
import java.io.IOException;
import java.util.AbstractList;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
/**
* A filter for a field based on several terms matching on any of them.
*/
public class TermsQueryBuilder extends AbstractQueryBuilder {
public static final String NAME = "terms";
public static final ParseField QUERY_NAME_FIELD = new ParseField(NAME, "in");
private final String fieldName;
private final List> values;
/**
* A filter for a field based on several terms matching on any of them.
*
* @param fieldName The field name
* @param values The terms
*/
public TermsQueryBuilder(String fieldName, String... values) {
this(fieldName, values != null ? Arrays.asList(values) : null);
}
/**
* A filter for a field based on several terms matching on any of them.
*
* @param fieldName The field name
* @param values The terms
*/
public TermsQueryBuilder(String fieldName, int... values) {
this(fieldName, values != null ? Arrays.stream(values).mapToObj(s -> s).collect(Collectors.toList()) : (Iterable>) null);
}
/**
* A filter for a field based on several terms matching on any of them.
*
* @param fieldName The field name
* @param values The terms
*/
public TermsQueryBuilder(String fieldName, long... values) {
this(fieldName, values != null ? Arrays.stream(values).mapToObj(s -> s).collect(Collectors.toList()) : (Iterable>) null);
}
/**
* A filter for a field based on several terms matching on any of them.
*
* @param fieldName The field name
* @param values The terms
*/
public TermsQueryBuilder(String fieldName, float... values) {
this(fieldName, values != null ? IntStream.range(0, values.length)
.mapToObj(i -> values[i]).collect(Collectors.toList()) : (Iterable>) null);
}
/**
* A filter for a field based on several terms matching on any of them.
*
* @param fieldName The field name
* @param values The terms
*/
public TermsQueryBuilder(String fieldName, double... values) {
this(fieldName, values != null ? Arrays.stream(values).mapToObj(s -> s).collect(Collectors.toList()) : (Iterable>) null);
}
/**
* A filter for a field based on several terms matching on any of them.
*
* @param fieldName The field name
* @param values The terms
*/
public TermsQueryBuilder(String fieldName, Object... values) {
this(fieldName, values != null ? Arrays.asList(values) : (Iterable>) null);
}
/**
* A filter for a field based on several terms matching on any of them.
*
* @param fieldName The field name
* @param values The terms
*/
public TermsQueryBuilder(String fieldName, Iterable> values) {
if (Strings.isEmpty(fieldName)) {
throw new IllegalArgumentException("field name cannot be null.");
}
if (values == null) {
throw new IllegalArgumentException("No value specified for terms query");
}
this.fieldName = fieldName;
this.values = convert(values);
}
/**
* Read from a stream.
*/
public TermsQueryBuilder(StreamInput in) throws IOException {
super(in);
fieldName = in.readString();
values = (List>) in.readGenericValue();
}
@Override
protected void doWriteTo(StreamOutput out) throws IOException {
out.writeString(fieldName);
out.writeGenericValue(values);
}
public String fieldName() {
return this.fieldName;
}
public List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy