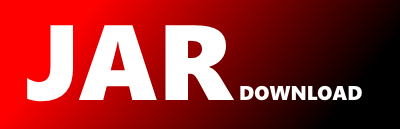
jcifs.SmbResourceLocator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jcifs Show documentation
Show all versions of jcifs Show documentation
JCIFS is an Open Source client library that implements the CIFS/SMB networking protocol in 100% Java
/*
* © 2017 AgNO3 Gmbh & Co. KG
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package jcifs;
import java.net.URL;
/**
* Location information for a SMB resource
*
* @author mbechler
*
*/
public interface SmbResourceLocator {
/**
* Returns the last component of the target URL. This will
* effectively be the name of the file or directory represented by this
* SmbFile
or in the case of URLs that only specify a server
* or workgroup, the server or workgroup will be returned. The name of
* the root URL smb://
is also smb://
. If this
* SmbFile refers to a workgroup, server, share, or directory,
* the name will include a trailing slash '/' so that composing new
* SmbFiles will maintain the trailing slash requirement.
*
* @return The last component of the URL associated with this SMB
* resource or smb://
if the resource is smb://
* itself.
*/
String getName ();
/**
*
* @return dfs referral data
*/
DfsReferralData getDfsReferral ();
/**
* Everything but the last component of the URL representing this SMB
* resource is effectively it's parent. The root URL smb://
* does not have a parent. In this case smb://
is returned.
*
* @return The parent directory of this SMB resource or
* smb://
if the resource refers to the root of the URL
* hierarchy which incidentally is also smb://
.
*/
String getParent ();
/**
* Returns the full uncanonicalized URL of this SMB resource. An
* SmbFile
constructed with the result of this method will
* result in an SmbFile
that is equal to the original.
*
* @return The uncanonicalized full URL of this SMB resource.
*/
String getPath ();
/**
* Returns the full URL of this SMB resource with '.' and '..' components
* factored out. An SmbFile
constructed with the result of
* this method will result in an SmbFile
that is equal to
* the original.
*
* @return The canonicalized URL of this SMB resource.
*/
String getCanonicalURL ();
/**
* @return The canonicalized UNC path of this SMB resource (relative to it's share)
*/
String getUNCPath ();
/**
* @return The canonicalized URL path (relative to the server/domain)
*/
String getURLPath ();
/**
* Retrieves the share associated with this SMB resource. In
* the case of smb://
, smb://workgroup/
,
* and smb://server/
URLs which do not specify a share,
* null
will be returned.
*
* @return The share component or null
if there is no share
*/
String getShare ();
/**
* Retrieve the hostname of the server for this SMB resource. If the resources has been resolved by DFS this will
* return the target name.
*
* @return The server name
*/
String getServerWithDfs ();
/**
* Retrieve the hostname of the server for this SMB resource. If this
* SmbFile
references a workgroup, the name of the workgroup
* is returned. If this SmbFile
refers to the root of this
* SMB network hierarchy, null
is returned.
*
* @return The server or workgroup name or null
if this
* SmbFile
refers to the root smb://
resource.
*/
String getServer ();
/**
* If the path of this SmbFile
falls within a DFS volume,
* this method will return the referral path to which it maps. Otherwise
* null
is returned.
*
* @return URL to the DFS volume
*/
String getDfsPath ();
/**
* @return the transport port, if specified
*/
int getPort ();
/**
* @return the original URL
*/
URL getURL ();
/**
* @return resolved server address
* @throws CIFSException
*/
Address getAddress () throws CIFSException;
/**
* @return whether this is a IPC connection
*/
boolean isIPC ();
/**
* Returns type of of object this SmbFile represents.
*
* @return TYPE_FILESYSTEM, TYPE_WORKGROUP, TYPE_SERVER,
* TYPE_NAMED_PIPE, or TYPE_SHARE in which case it may be either TYPE_SHARE,
* TYPE_PRINTER or TYPE_COMM.
* @throws CIFSException
*/
int getType () throws CIFSException;
/**
* @return whether this is a workgroup reference
* @throws CIFSException
*/
boolean isWorkgroup () throws CIFSException;
/**
*
* @return whether this is a root resource
*/
boolean isRoot ();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy