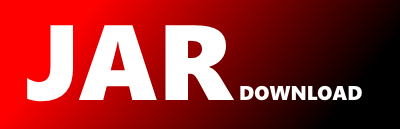
org.cp.elements.util.MapBuilder Maven / Gradle / Ivy
/*
* Copyright 2011-Present Author or Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.cp.elements.util;
import java.util.HashMap;
import java.util.Map;
import java.util.SortedMap;
import java.util.TreeMap;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
import org.cp.elements.lang.Builder;
import org.cp.elements.lang.ObjectUtils;
import org.cp.elements.lang.annotation.NotNull;
/**
* The {@link MapBuilder} class is an implementation of the Builder Software Design Pattern and is used to build
* a {@link Map} implementation with a mapping of keys and values.
*
* @author John Blum
* @param {@link Class type} of the {@link Map} key.
* @param {@link Class type} of the {@link Map} value.
* @see java.util.HashMap
* @see java.util.Map
* @see java.util.SortedMap
* @see java.util.concurrent.ConcurrentMap
* @see org.cp.elements.lang.Builder
* @since 1.0.0
*/
@SuppressWarnings("all")
public class MapBuilder implements Builder
© 2015 - 2025 Weber Informatics LLC | Privacy Policy