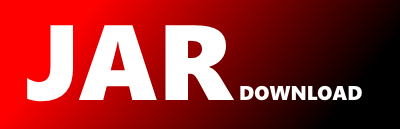
org.cometd.bayeux.Promise Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2008 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.cometd.bayeux;
import java.util.Objects;
import java.util.concurrent.CompletableFuture;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
/**
* The future result of an operation, either a value if the operation
* succeeded, or a failure if the operation failed.
*
* @param the type of the result value
*/
public interface Promise {
/**
* Shared instance whose methods are implemented empty,
* use {@link #noop()} to ease type inference.
*/
Promise> NOOP = new Promise<>() {};
/**
* Callback to invoke when the operation succeeds.
*
* @param result the result
* @see #fail(Throwable)
*/
default void succeed(C result) {
}
/**
* Callback to invoke when the operation fails.
*
* @param failure the operation failure
*/
default void fail(Throwable failure) {
}
/**
* Returns a {@code BiConsumer} that, when invoked,
* completes this Promise.
* Typical usage is with {@code CompletableFuture}:
* {@code
* public void process(ServerMessage message, Promise promise) {
* CompletableFuture.supplyAsync(() -> asyncOperation(message))
* .whenComplete(promise.complete());
* }
* }
*
* @return a BiConsumer that completes this Promise
* @see Completable
*/
default BiConsumer complete() {
return (r, x) -> {
if (x == null) {
succeed(r);
} else {
fail(x);
}
};
}
/**
* @param the type of the empty result
* @return a Promise whose methods are implemented empty.
*/
@SuppressWarnings("unchecked")
static Promise noop() {
return (Promise)NOOP;
}
/**
* @param succeed the Consumer to call in case of successful completion
* @param fail the Consumer to call in case of failed completion
* @param the type of the result value
* @return a Promise from the given consumers
*/
static Promise from(Consumer succeed, Consumer fail) {
return new Promise<>() {
@Override
public void succeed(T result) {
succeed.accept(result);
}
@Override
public void fail(Throwable failure) {
fail.accept(failure);
}
};
}
/**
* Returns a Promise that, when completed,
* invokes the given {@link BiConsumer} function.
*
* @param fn the function to invoke when the Promise is completed
* @param the type of the result value
* @return a Promise that invokes the BiConsumer function
*/
static Promise complete(BiConsumer fn) {
return new Promise<>() {
@Override
public void succeed(T result) {
fn.accept(result, null);
}
@Override
public void fail(Throwable failure) {
fn.accept(null, failure);
}
};
}
/**
* A CompletableFuture that is also a Promise.
*/
class Completable extends CompletableFuture implements Promise {
@Override
public void succeed(S result) {
complete(result);
}
@Override
public void fail(Throwable failure) {
completeExceptionally(failure);
}
}
/**
* A wrapper for {@link Promise} instances.
*
* @param the type of the result value
*/
class Wrapper implements Promise {
private final Promise wrapped;
public Wrapper(Promise wrapped) {
this.wrapped = Objects.requireNonNull(wrapped);
}
public Promise getWrapped() {
return wrapped;
}
@Override
public void succeed(W result) {
getWrapped().succeed(result);
}
@Override
public void fail(Throwable failure) {
getWrapped().fail(failure);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy