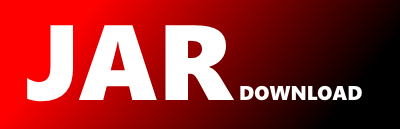
org.commonjava.maven.ext.manip.resolver.MavenLocationExpander Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pom-manipulation-io Show documentation
Show all versions of pom-manipulation-io Show documentation
Input/Output Library (POM File, Maven Repository and REST)
/**
* Copyright (C) 2012 Red Hat, Inc. ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.commonjava.maven.ext.manip.resolver;
import org.apache.maven.artifact.repository.ArtifactRepository;
import org.apache.maven.artifact.repository.ArtifactRepositoryPolicy;
import org.apache.maven.artifact.repository.MavenArtifactRepository;
import org.apache.maven.artifact.repository.layout.ArtifactRepositoryLayout;
import org.apache.maven.artifact.repository.layout.DefaultRepositoryLayout;
import org.apache.maven.repository.MirrorSelector;
import org.apache.maven.settings.Mirror;
import org.apache.maven.settings.Profile;
import org.apache.maven.settings.Repository;
import org.apache.maven.settings.RepositoryPolicy;
import org.apache.maven.settings.Settings;
import org.commonjava.maven.atlas.ident.util.JoinString;
import org.commonjava.maven.galley.TransferException;
import org.commonjava.maven.galley.model.ConcreteResource;
import org.commonjava.maven.galley.model.Location;
import org.commonjava.maven.galley.model.Resource;
import org.commonjava.maven.galley.model.SimpleLocation;
import org.commonjava.maven.galley.model.VirtualResource;
import org.commonjava.maven.galley.spi.transport.LocationExpander;
import org.commonjava.maven.galley.transport.htcli.model.SimpleHttpLocation;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.net.MalformedURLException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* Galley {@link LocationExpander} implementation that expands a shorthand URI
* given in the betterdep goals into the actual list of locations to check for
* artifacts.
*
* @author jdcasey
*/
public class MavenLocationExpander
implements LocationExpander
{
public static final Location EXPANSION_TARGET = new SimpleLocation( "maven:repositories" );
static
{
EXPANSION_TARGET.setAttribute(Location.CONNECTION_TIMEOUT_SECONDS, 60);
}
private final List locations;
private final Logger logger = LoggerFactory.getLogger( getClass() );
public MavenLocationExpander( final List customLocations,
final List artifactRepositories,
final ArtifactRepository localRepository, final MirrorSelector mirrorSelector,
final Settings settings, final List activeProfiles )
throws MalformedURLException
{
final Set locs = new LinkedHashSet<>();
if ( localRepository != null )
{
locs.add( new SimpleLocation( localRepository.getId(), new File( localRepository.getBasedir() ).toURI()
.toString() ) );
}
if ( customLocations != null )
{
locs.addAll( customLocations );
}
addSettingsProfileRepositoriesTo( locs, settings, activeProfiles, mirrorSelector );
addRequestRepositoriesTo( locs, artifactRepositories, settings, mirrorSelector );
logger.debug( "Configured to use Maven locations:\n {}", new JoinString( "\n ", locs ) );
this.locations = new ArrayList<>( locs );
}
private void addRequestRepositoriesTo( final Set locs,
final List artifactRepositories,
final Settings settings, final MirrorSelector mirrorSelector )
throws MalformedURLException
{
if ( artifactRepositories != null )
{
for ( final ArtifactRepository repo : artifactRepositories )
{
// TODO: Authentication via memory password manager.
String id = repo.getId();
String url = repo.getUrl();
if ( url.startsWith( "file:" ) )
{
locs.add( new SimpleLocation( id, url ) );
}
else
{
final List mirrors = settings.getMirrors();
if ( mirrors != null )
{
final Mirror mirror = mirrorSelector == null ? null : mirrorSelector.getMirror( repo, mirrors );
if ( mirror != null )
{
id = mirror.getId();
url = mirror.getUrl();
}
}
final ArtifactRepositoryPolicy releases = repo.getReleases();
final ArtifactRepositoryPolicy snapshots = repo.getSnapshots();
SimpleHttpLocation addition = new SimpleHttpLocation( id, url,
snapshots != null && snapshots.isEnabled(),
releases == null || releases.isEnabled(), true, false,
null );
addition.setAttribute(Location.CONNECTION_TIMEOUT_SECONDS, 60);
locs.add (addition);
}
}
}
}
private void addSettingsProfileRepositoriesTo( final Set locs, final Settings settings,
final List activeProfiles,
final MirrorSelector mirrorSelector )
throws MalformedURLException
{
if ( settings != null )
{
final Map profiles = settings.getProfilesAsMap();
if ( profiles != null && activeProfiles != null && !activeProfiles.isEmpty() )
{
final LinkedHashSet active = new LinkedHashSet<>( activeProfiles );
final List settingsActiveProfiles = settings.getActiveProfiles();
if ( settingsActiveProfiles != null && !settingsActiveProfiles.isEmpty() )
{
active.addAll( settingsActiveProfiles );
}
for ( final String profileId : active )
{
final Profile profile = profiles.get( profileId );
if ( profile != null )
{
final List repositories = profile.getRepositories();
if ( repositories != null )
{
final List mirrors = settings.getMirrors();
final ArtifactRepositoryLayout layout = new DefaultRepositoryLayout();
for ( final Repository repo : repositories )
{
String id = repo.getId();
String url = repo.getUrl();
if ( mirrors != null )
{
final ArtifactRepositoryPolicy snapshots = convertPolicy( repo.getSnapshots() );
final ArtifactRepositoryPolicy releases = convertPolicy( repo.getReleases() );
final MavenArtifactRepository arepo =
new MavenArtifactRepository( id, url, layout, snapshots, releases );
final Mirror mirror =
mirrorSelector == null ? null : mirrorSelector.getMirror( arepo, mirrors );
if ( mirror != null )
{
id = mirror.getId();
url = mirror.getUrl();
}
SimpleHttpLocation addition = new SimpleHttpLocation( id, url, snapshots.isEnabled(), releases.isEnabled(), true, false, null );
addition.setAttribute(Location.CONNECTION_TIMEOUT_SECONDS, 60);
locs.add (addition);
}
}
}
}
}
}
}
}
private ArtifactRepositoryPolicy convertPolicy( final RepositoryPolicy policy )
{
if ( policy == null )
{
return new ArtifactRepositoryPolicy();
}
return new ArtifactRepositoryPolicy( policy.isEnabled(), policy.getUpdatePolicy(), policy.getChecksumPolicy() );
}
@Override
public List expand( final Location... locations )
throws TransferException
{
final List result = new ArrayList<>();
for ( final Location loc : locations )
{
expandSingle( loc, result );
}
logger.debug( "Expanded to:\n {}", new JoinString( "\n ", result ) );
return result;
}
@Override
public List expand( final Collection locations )
throws TransferException
{
final List result = new ArrayList<>();
for ( final Location loc : locations )
{
expandSingle( loc, result );
}
logger.debug( "Expanded to:\n {}", new JoinString( "\n ", result ) );
return result;
}
@Override
public VirtualResource expand( final Resource resource )
throws TransferException
{
final List result = new ArrayList<>();
if ( resource instanceof ConcreteResource )
{
expandSingle( (ConcreteResource) resource, result );
}
else
{
for ( final ConcreteResource cr : ( (VirtualResource) resource ).toConcreteResources() )
{
expandSingle( cr, result );
}
}
logger.debug( "Expanded to:\n {}", new JoinString( "\n ", result ) );
return new VirtualResource( result );
}
private void expandSingle( final ConcreteResource cr, final List result )
{
final Location loc = cr.getLocation();
final List expanded = new ArrayList<>();
expandSingle( loc, expanded );
final String path = cr.getPath();
for ( final Location location : expanded )
{
result.add( new ConcreteResource( location, path ) );
}
}
private void expandSingle( final Location loc, final List result )
{
logger.debug( "Expanding: {}", loc );
if ( EXPANSION_TARGET.equals( loc ) )
{
logger.debug( "Expanding..." );
result.addAll( this.locations );
}
else
{
logger.debug( "No expansion available." );
result.add( loc );
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy