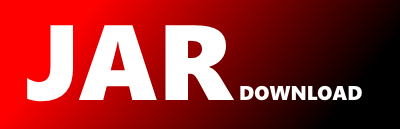
org.computate.vertx.api.ApiRequest Maven / Gradle / Ivy
/*
* Copyright (c) 2018-2022 Computate Limited Liability Company in Utah, USA.
*
* This program and the accompanying materials are made available under the
* terms of the GNU GENERAL PUBLIC LICENSE Version 3 which is available at
*
* https://www.gnu.org/licenses/gpl-3.0.en.html
*
* You may not propagate or modify a covered work except as expressly provided
* under this License. Any attempt otherwise to propagate or modify it is void,
* and will automatically terminate your rights under this License (including
* any patent licenses granted under the third paragraph of section 11).
*/
package org.computate.vertx.api;
import java.time.ZoneId;
import java.time.ZonedDateTime;
import java.time.temporal.ChronoUnit;
import java.util.List;
import java.util.UUID;
import org.computate.search.wrap.Wrap;
import org.computate.vertx.config.ComputateConfigKeys;
import org.computate.vertx.request.ComputateSiteRequest;
/**
* Keyword: classSimpleNameApiRequest
*/
public class ApiRequest extends ApiRequestGen
© 2015 - 2025 Weber Informatics LLC | Privacy Policy