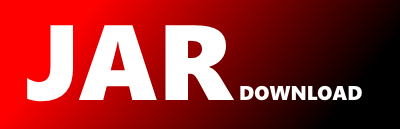
com.netflix.conductor.grpc.ProtoMapper Maven / Gradle / Ivy
/*
* Copyright 2023 Conductor authors
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*/
package com.netflix.conductor.grpc;
import com.google.protobuf.Any;
import com.google.protobuf.ListValue;
import com.google.protobuf.NullValue;
import com.google.protobuf.Struct;
import com.google.protobuf.Value;
import com.netflix.conductor.common.metadata.workflow.WorkflowTask;
import com.netflix.conductor.proto.WorkflowTaskPb;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
/**
* ProtoMapper implements conversion code between the internal models
* used by Conductor (POJOs) and their corresponding equivalents in
* the exposed Protocol Buffers interface.
*
* The vast majority of the mapping logic is implemented in the autogenerated
* {@link AbstractProtoMapper} class. This class only implements the custom
* logic for objects that need to be special cased in the API.
*/
public final class ProtoMapper extends AbstractProtoMapper {
public static final ProtoMapper INSTANCE = new ProtoMapper();
private static final int NO_RETRY_VALUE = -1;
private ProtoMapper() {}
/**
* Convert an {@link Object} instance into its equivalent {@link Value}
* ProtoBuf object.
*
* The {@link Value} ProtoBuf message is a variant type that can define any
* value representable as a native JSON type. Consequently, this method expects
* the given {@link Object} instance to be a Java object instance of JSON-native
* value, namely: null, {@link Boolean}, {@link Double}, {@link String},
* {@link Map}, {@link List}.
*
* Any other values will cause an exception to be thrown.
* See {@link ProtoMapper#fromProto(Value)} for the reverse mapping.
*
* @param val a Java object that can be represented natively in JSON
* @return an instance of a {@link Value} ProtoBuf message
*/
@Override
public Value toProto(Object val) {
Value.Builder builder = Value.newBuilder();
if (val == null) {
builder.setNullValue(NullValue.NULL_VALUE);
} else if (val instanceof Boolean) {
builder.setBoolValue((Boolean) val);
} else if (val instanceof Double) {
builder.setNumberValue((Double) val);
} else if (val instanceof String) {
builder.setStringValue((String) val);
} else if (val instanceof Map) {
Map map = (Map) val;
Struct.Builder struct = Struct.newBuilder();
for (Map.Entry pair : map.entrySet()) {
struct.putFields(pair.getKey(), toProto(pair.getValue()));
}
builder.setStructValue(struct.build());
} else if (val instanceof List) {
ListValue.Builder list = ListValue.newBuilder();
for (Object obj : (List