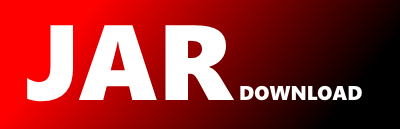
com.netflix.conductor.proto.TaskPb Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: model/task.proto
package com.netflix.conductor.proto;
public final class TaskPb {
private TaskPb() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface TaskOrBuilder extends
// @@protoc_insertion_point(interface_extends:conductor.proto.Task)
com.google.protobuf.MessageOrBuilder {
/**
* string task_type = 1;
* @return The taskType.
*/
java.lang.String getTaskType();
/**
* string task_type = 1;
* @return The bytes for taskType.
*/
com.google.protobuf.ByteString
getTaskTypeBytes();
/**
* .conductor.proto.Task.Status status = 2;
* @return The enum numeric value on the wire for status.
*/
int getStatusValue();
/**
* .conductor.proto.Task.Status status = 2;
* @return The status.
*/
com.netflix.conductor.proto.TaskPb.Task.Status getStatus();
/**
* map<string, .google.protobuf.Value> input_data = 3;
*/
int getInputDataCount();
/**
* map<string, .google.protobuf.Value> input_data = 3;
*/
boolean containsInputData(
java.lang.String key);
/**
* Use {@link #getInputDataMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getInputData();
/**
* map<string, .google.protobuf.Value> input_data = 3;
*/
java.util.Map
getInputDataMap();
/**
* map<string, .google.protobuf.Value> input_data = 3;
*/
/* nullable */
com.google.protobuf.Value getInputDataOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Value defaultValue);
/**
* map<string, .google.protobuf.Value> input_data = 3;
*/
com.google.protobuf.Value getInputDataOrThrow(
java.lang.String key);
/**
* string reference_task_name = 4;
* @return The referenceTaskName.
*/
java.lang.String getReferenceTaskName();
/**
* string reference_task_name = 4;
* @return The bytes for referenceTaskName.
*/
com.google.protobuf.ByteString
getReferenceTaskNameBytes();
/**
* int32 retry_count = 5;
* @return The retryCount.
*/
int getRetryCount();
/**
* int32 seq = 6;
* @return The seq.
*/
int getSeq();
/**
* string correlation_id = 7;
* @return The correlationId.
*/
java.lang.String getCorrelationId();
/**
* string correlation_id = 7;
* @return The bytes for correlationId.
*/
com.google.protobuf.ByteString
getCorrelationIdBytes();
/**
* int32 poll_count = 8;
* @return The pollCount.
*/
int getPollCount();
/**
* string task_def_name = 9;
* @return The taskDefName.
*/
java.lang.String getTaskDefName();
/**
* string task_def_name = 9;
* @return The bytes for taskDefName.
*/
com.google.protobuf.ByteString
getTaskDefNameBytes();
/**
* int64 scheduled_time = 10;
* @return The scheduledTime.
*/
long getScheduledTime();
/**
* int64 start_time = 11;
* @return The startTime.
*/
long getStartTime();
/**
* int64 end_time = 12;
* @return The endTime.
*/
long getEndTime();
/**
* int64 update_time = 13;
* @return The updateTime.
*/
long getUpdateTime();
/**
* int32 start_delay_in_seconds = 14;
* @return The startDelayInSeconds.
*/
int getStartDelayInSeconds();
/**
* string retried_task_id = 15;
* @return The retriedTaskId.
*/
java.lang.String getRetriedTaskId();
/**
* string retried_task_id = 15;
* @return The bytes for retriedTaskId.
*/
com.google.protobuf.ByteString
getRetriedTaskIdBytes();
/**
* bool retried = 16;
* @return The retried.
*/
boolean getRetried();
/**
* bool executed = 17;
* @return The executed.
*/
boolean getExecuted();
/**
* bool callback_from_worker = 18;
* @return The callbackFromWorker.
*/
boolean getCallbackFromWorker();
/**
* int64 response_timeout_seconds = 19;
* @return The responseTimeoutSeconds.
*/
long getResponseTimeoutSeconds();
/**
* string workflow_instance_id = 20;
* @return The workflowInstanceId.
*/
java.lang.String getWorkflowInstanceId();
/**
* string workflow_instance_id = 20;
* @return The bytes for workflowInstanceId.
*/
com.google.protobuf.ByteString
getWorkflowInstanceIdBytes();
/**
* string workflow_type = 21;
* @return The workflowType.
*/
java.lang.String getWorkflowType();
/**
* string workflow_type = 21;
* @return The bytes for workflowType.
*/
com.google.protobuf.ByteString
getWorkflowTypeBytes();
/**
* string task_id = 22;
* @return The taskId.
*/
java.lang.String getTaskId();
/**
* string task_id = 22;
* @return The bytes for taskId.
*/
com.google.protobuf.ByteString
getTaskIdBytes();
/**
* string reason_for_incompletion = 23;
* @return The reasonForIncompletion.
*/
java.lang.String getReasonForIncompletion();
/**
* string reason_for_incompletion = 23;
* @return The bytes for reasonForIncompletion.
*/
com.google.protobuf.ByteString
getReasonForIncompletionBytes();
/**
* int64 callback_after_seconds = 24;
* @return The callbackAfterSeconds.
*/
long getCallbackAfterSeconds();
/**
* string worker_id = 25;
* @return The workerId.
*/
java.lang.String getWorkerId();
/**
* string worker_id = 25;
* @return The bytes for workerId.
*/
com.google.protobuf.ByteString
getWorkerIdBytes();
/**
* map<string, .google.protobuf.Value> output_data = 26;
*/
int getOutputDataCount();
/**
* map<string, .google.protobuf.Value> output_data = 26;
*/
boolean containsOutputData(
java.lang.String key);
/**
* Use {@link #getOutputDataMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getOutputData();
/**
* map<string, .google.protobuf.Value> output_data = 26;
*/
java.util.Map
getOutputDataMap();
/**
* map<string, .google.protobuf.Value> output_data = 26;
*/
/* nullable */
com.google.protobuf.Value getOutputDataOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Value defaultValue);
/**
* map<string, .google.protobuf.Value> output_data = 26;
*/
com.google.protobuf.Value getOutputDataOrThrow(
java.lang.String key);
/**
* .conductor.proto.WorkflowTask workflow_task = 27;
* @return Whether the workflowTask field is set.
*/
boolean hasWorkflowTask();
/**
* .conductor.proto.WorkflowTask workflow_task = 27;
* @return The workflowTask.
*/
com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask getWorkflowTask();
/**
* .conductor.proto.WorkflowTask workflow_task = 27;
*/
com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTaskOrBuilder getWorkflowTaskOrBuilder();
/**
* string domain = 28;
* @return The domain.
*/
java.lang.String getDomain();
/**
* string domain = 28;
* @return The bytes for domain.
*/
com.google.protobuf.ByteString
getDomainBytes();
/**
* .google.protobuf.Any input_message = 29;
* @return Whether the inputMessage field is set.
*/
boolean hasInputMessage();
/**
* .google.protobuf.Any input_message = 29;
* @return The inputMessage.
*/
com.google.protobuf.Any getInputMessage();
/**
* .google.protobuf.Any input_message = 29;
*/
com.google.protobuf.AnyOrBuilder getInputMessageOrBuilder();
/**
* .google.protobuf.Any output_message = 30;
* @return Whether the outputMessage field is set.
*/
boolean hasOutputMessage();
/**
* .google.protobuf.Any output_message = 30;
* @return The outputMessage.
*/
com.google.protobuf.Any getOutputMessage();
/**
* .google.protobuf.Any output_message = 30;
*/
com.google.protobuf.AnyOrBuilder getOutputMessageOrBuilder();
/**
* int32 rate_limit_per_frequency = 32;
* @return The rateLimitPerFrequency.
*/
int getRateLimitPerFrequency();
/**
* int32 rate_limit_frequency_in_seconds = 33;
* @return The rateLimitFrequencyInSeconds.
*/
int getRateLimitFrequencyInSeconds();
/**
* string external_input_payload_storage_path = 34;
* @return The externalInputPayloadStoragePath.
*/
java.lang.String getExternalInputPayloadStoragePath();
/**
* string external_input_payload_storage_path = 34;
* @return The bytes for externalInputPayloadStoragePath.
*/
com.google.protobuf.ByteString
getExternalInputPayloadStoragePathBytes();
/**
* string external_output_payload_storage_path = 35;
* @return The externalOutputPayloadStoragePath.
*/
java.lang.String getExternalOutputPayloadStoragePath();
/**
* string external_output_payload_storage_path = 35;
* @return The bytes for externalOutputPayloadStoragePath.
*/
com.google.protobuf.ByteString
getExternalOutputPayloadStoragePathBytes();
/**
* int32 workflow_priority = 36;
* @return The workflowPriority.
*/
int getWorkflowPriority();
/**
* string execution_name_space = 37;
* @return The executionNameSpace.
*/
java.lang.String getExecutionNameSpace();
/**
* string execution_name_space = 37;
* @return The bytes for executionNameSpace.
*/
com.google.protobuf.ByteString
getExecutionNameSpaceBytes();
/**
* string isolation_group_id = 38;
* @return The isolationGroupId.
*/
java.lang.String getIsolationGroupId();
/**
* string isolation_group_id = 38;
* @return The bytes for isolationGroupId.
*/
com.google.protobuf.ByteString
getIsolationGroupIdBytes();
/**
* int32 iteration = 40;
* @return The iteration.
*/
int getIteration();
/**
* string sub_workflow_id = 41;
* @return The subWorkflowId.
*/
java.lang.String getSubWorkflowId();
/**
* string sub_workflow_id = 41;
* @return The bytes for subWorkflowId.
*/
com.google.protobuf.ByteString
getSubWorkflowIdBytes();
/**
* bool subworkflow_changed = 42;
* @return The subworkflowChanged.
*/
boolean getSubworkflowChanged();
}
/**
* Protobuf type {@code conductor.proto.Task}
*/
public static final class Task extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:conductor.proto.Task)
TaskOrBuilder {
private static final long serialVersionUID = 0L;
// Use Task.newBuilder() to construct.
private Task(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Task() {
taskType_ = "";
status_ = 0;
referenceTaskName_ = "";
correlationId_ = "";
taskDefName_ = "";
retriedTaskId_ = "";
workflowInstanceId_ = "";
workflowType_ = "";
taskId_ = "";
reasonForIncompletion_ = "";
workerId_ = "";
domain_ = "";
externalInputPayloadStoragePath_ = "";
externalOutputPayloadStoragePath_ = "";
executionNameSpace_ = "";
isolationGroupId_ = "";
subWorkflowId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Task();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.netflix.conductor.proto.TaskPb.internal_static_conductor_proto_Task_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 3:
return internalGetInputData();
case 26:
return internalGetOutputData();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.netflix.conductor.proto.TaskPb.internal_static_conductor_proto_Task_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.netflix.conductor.proto.TaskPb.Task.class, com.netflix.conductor.proto.TaskPb.Task.Builder.class);
}
/**
* Protobuf enum {@code conductor.proto.Task.Status}
*/
public enum Status
implements com.google.protobuf.ProtocolMessageEnum {
/**
* IN_PROGRESS = 0;
*/
IN_PROGRESS(0),
/**
* CANCELED = 1;
*/
CANCELED(1),
/**
* FAILED = 2;
*/
FAILED(2),
/**
* FAILED_WITH_TERMINAL_ERROR = 3;
*/
FAILED_WITH_TERMINAL_ERROR(3),
/**
* COMPLETED = 4;
*/
COMPLETED(4),
/**
* COMPLETED_WITH_ERRORS = 5;
*/
COMPLETED_WITH_ERRORS(5),
/**
* SCHEDULED = 6;
*/
SCHEDULED(6),
/**
* TIMED_OUT = 7;
*/
TIMED_OUT(7),
/**
* SKIPPED = 8;
*/
SKIPPED(8),
UNRECOGNIZED(-1),
;
/**
* IN_PROGRESS = 0;
*/
public static final int IN_PROGRESS_VALUE = 0;
/**
* CANCELED = 1;
*/
public static final int CANCELED_VALUE = 1;
/**
* FAILED = 2;
*/
public static final int FAILED_VALUE = 2;
/**
* FAILED_WITH_TERMINAL_ERROR = 3;
*/
public static final int FAILED_WITH_TERMINAL_ERROR_VALUE = 3;
/**
* COMPLETED = 4;
*/
public static final int COMPLETED_VALUE = 4;
/**
* COMPLETED_WITH_ERRORS = 5;
*/
public static final int COMPLETED_WITH_ERRORS_VALUE = 5;
/**
* SCHEDULED = 6;
*/
public static final int SCHEDULED_VALUE = 6;
/**
* TIMED_OUT = 7;
*/
public static final int TIMED_OUT_VALUE = 7;
/**
* SKIPPED = 8;
*/
public static final int SKIPPED_VALUE = 8;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Status valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Status forNumber(int value) {
switch (value) {
case 0: return IN_PROGRESS;
case 1: return CANCELED;
case 2: return FAILED;
case 3: return FAILED_WITH_TERMINAL_ERROR;
case 4: return COMPLETED;
case 5: return COMPLETED_WITH_ERRORS;
case 6: return SCHEDULED;
case 7: return TIMED_OUT;
case 8: return SKIPPED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Status> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Status findValueByNumber(int number) {
return Status.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.netflix.conductor.proto.TaskPb.Task.getDescriptor().getEnumTypes().get(0);
}
private static final Status[] VALUES = values();
public static Status valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Status(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:conductor.proto.Task.Status)
}
public static final int TASK_TYPE_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object taskType_ = "";
/**
* string task_type = 1;
* @return The taskType.
*/
@java.lang.Override
public java.lang.String getTaskType() {
java.lang.Object ref = taskType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
taskType_ = s;
return s;
}
}
/**
* string task_type = 1;
* @return The bytes for taskType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTaskTypeBytes() {
java.lang.Object ref = taskType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
taskType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 2;
private int status_ = 0;
/**
* .conductor.proto.Task.Status status = 2;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
* .conductor.proto.Task.Status status = 2;
* @return The status.
*/
@java.lang.Override public com.netflix.conductor.proto.TaskPb.Task.Status getStatus() {
com.netflix.conductor.proto.TaskPb.Task.Status result = com.netflix.conductor.proto.TaskPb.Task.Status.forNumber(status_);
return result == null ? com.netflix.conductor.proto.TaskPb.Task.Status.UNRECOGNIZED : result;
}
public static final int INPUT_DATA_FIELD_NUMBER = 3;
private static final class InputDataDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.google.protobuf.Value> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.netflix.conductor.proto.TaskPb.internal_static_conductor_proto_Task_InputDataEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.protobuf.Value.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, com.google.protobuf.Value> inputData_;
private com.google.protobuf.MapField
internalGetInputData() {
if (inputData_ == null) {
return com.google.protobuf.MapField.emptyMapField(
InputDataDefaultEntryHolder.defaultEntry);
}
return inputData_;
}
public int getInputDataCount() {
return internalGetInputData().getMap().size();
}
/**
* map<string, .google.protobuf.Value> input_data = 3;
*/
@java.lang.Override
public boolean containsInputData(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetInputData().getMap().containsKey(key);
}
/**
* Use {@link #getInputDataMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getInputData() {
return getInputDataMap();
}
/**
* map<string, .google.protobuf.Value> input_data = 3;
*/
@java.lang.Override
public java.util.Map getInputDataMap() {
return internalGetInputData().getMap();
}
/**
* map<string, .google.protobuf.Value> input_data = 3;
*/
@java.lang.Override
public /* nullable */
com.google.protobuf.Value getInputDataOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Value defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetInputData().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .google.protobuf.Value> input_data = 3;
*/
@java.lang.Override
public com.google.protobuf.Value getInputDataOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetInputData().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int REFERENCE_TASK_NAME_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object referenceTaskName_ = "";
/**
* string reference_task_name = 4;
* @return The referenceTaskName.
*/
@java.lang.Override
public java.lang.String getReferenceTaskName() {
java.lang.Object ref = referenceTaskName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
referenceTaskName_ = s;
return s;
}
}
/**
* string reference_task_name = 4;
* @return The bytes for referenceTaskName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getReferenceTaskNameBytes() {
java.lang.Object ref = referenceTaskName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
referenceTaskName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RETRY_COUNT_FIELD_NUMBER = 5;
private int retryCount_ = 0;
/**
* int32 retry_count = 5;
* @return The retryCount.
*/
@java.lang.Override
public int getRetryCount() {
return retryCount_;
}
public static final int SEQ_FIELD_NUMBER = 6;
private int seq_ = 0;
/**
* int32 seq = 6;
* @return The seq.
*/
@java.lang.Override
public int getSeq() {
return seq_;
}
public static final int CORRELATION_ID_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private volatile java.lang.Object correlationId_ = "";
/**
* string correlation_id = 7;
* @return The correlationId.
*/
@java.lang.Override
public java.lang.String getCorrelationId() {
java.lang.Object ref = correlationId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
correlationId_ = s;
return s;
}
}
/**
* string correlation_id = 7;
* @return The bytes for correlationId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCorrelationIdBytes() {
java.lang.Object ref = correlationId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
correlationId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int POLL_COUNT_FIELD_NUMBER = 8;
private int pollCount_ = 0;
/**
* int32 poll_count = 8;
* @return The pollCount.
*/
@java.lang.Override
public int getPollCount() {
return pollCount_;
}
public static final int TASK_DEF_NAME_FIELD_NUMBER = 9;
@SuppressWarnings("serial")
private volatile java.lang.Object taskDefName_ = "";
/**
* string task_def_name = 9;
* @return The taskDefName.
*/
@java.lang.Override
public java.lang.String getTaskDefName() {
java.lang.Object ref = taskDefName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
taskDefName_ = s;
return s;
}
}
/**
* string task_def_name = 9;
* @return The bytes for taskDefName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTaskDefNameBytes() {
java.lang.Object ref = taskDefName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
taskDefName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SCHEDULED_TIME_FIELD_NUMBER = 10;
private long scheduledTime_ = 0L;
/**
* int64 scheduled_time = 10;
* @return The scheduledTime.
*/
@java.lang.Override
public long getScheduledTime() {
return scheduledTime_;
}
public static final int START_TIME_FIELD_NUMBER = 11;
private long startTime_ = 0L;
/**
* int64 start_time = 11;
* @return The startTime.
*/
@java.lang.Override
public long getStartTime() {
return startTime_;
}
public static final int END_TIME_FIELD_NUMBER = 12;
private long endTime_ = 0L;
/**
* int64 end_time = 12;
* @return The endTime.
*/
@java.lang.Override
public long getEndTime() {
return endTime_;
}
public static final int UPDATE_TIME_FIELD_NUMBER = 13;
private long updateTime_ = 0L;
/**
* int64 update_time = 13;
* @return The updateTime.
*/
@java.lang.Override
public long getUpdateTime() {
return updateTime_;
}
public static final int START_DELAY_IN_SECONDS_FIELD_NUMBER = 14;
private int startDelayInSeconds_ = 0;
/**
* int32 start_delay_in_seconds = 14;
* @return The startDelayInSeconds.
*/
@java.lang.Override
public int getStartDelayInSeconds() {
return startDelayInSeconds_;
}
public static final int RETRIED_TASK_ID_FIELD_NUMBER = 15;
@SuppressWarnings("serial")
private volatile java.lang.Object retriedTaskId_ = "";
/**
* string retried_task_id = 15;
* @return The retriedTaskId.
*/
@java.lang.Override
public java.lang.String getRetriedTaskId() {
java.lang.Object ref = retriedTaskId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
retriedTaskId_ = s;
return s;
}
}
/**
* string retried_task_id = 15;
* @return The bytes for retriedTaskId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRetriedTaskIdBytes() {
java.lang.Object ref = retriedTaskId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
retriedTaskId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RETRIED_FIELD_NUMBER = 16;
private boolean retried_ = false;
/**
* bool retried = 16;
* @return The retried.
*/
@java.lang.Override
public boolean getRetried() {
return retried_;
}
public static final int EXECUTED_FIELD_NUMBER = 17;
private boolean executed_ = false;
/**
* bool executed = 17;
* @return The executed.
*/
@java.lang.Override
public boolean getExecuted() {
return executed_;
}
public static final int CALLBACK_FROM_WORKER_FIELD_NUMBER = 18;
private boolean callbackFromWorker_ = false;
/**
* bool callback_from_worker = 18;
* @return The callbackFromWorker.
*/
@java.lang.Override
public boolean getCallbackFromWorker() {
return callbackFromWorker_;
}
public static final int RESPONSE_TIMEOUT_SECONDS_FIELD_NUMBER = 19;
private long responseTimeoutSeconds_ = 0L;
/**
* int64 response_timeout_seconds = 19;
* @return The responseTimeoutSeconds.
*/
@java.lang.Override
public long getResponseTimeoutSeconds() {
return responseTimeoutSeconds_;
}
public static final int WORKFLOW_INSTANCE_ID_FIELD_NUMBER = 20;
@SuppressWarnings("serial")
private volatile java.lang.Object workflowInstanceId_ = "";
/**
* string workflow_instance_id = 20;
* @return The workflowInstanceId.
*/
@java.lang.Override
public java.lang.String getWorkflowInstanceId() {
java.lang.Object ref = workflowInstanceId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workflowInstanceId_ = s;
return s;
}
}
/**
* string workflow_instance_id = 20;
* @return The bytes for workflowInstanceId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkflowInstanceIdBytes() {
java.lang.Object ref = workflowInstanceId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workflowInstanceId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int WORKFLOW_TYPE_FIELD_NUMBER = 21;
@SuppressWarnings("serial")
private volatile java.lang.Object workflowType_ = "";
/**
* string workflow_type = 21;
* @return The workflowType.
*/
@java.lang.Override
public java.lang.String getWorkflowType() {
java.lang.Object ref = workflowType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workflowType_ = s;
return s;
}
}
/**
* string workflow_type = 21;
* @return The bytes for workflowType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkflowTypeBytes() {
java.lang.Object ref = workflowType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workflowType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TASK_ID_FIELD_NUMBER = 22;
@SuppressWarnings("serial")
private volatile java.lang.Object taskId_ = "";
/**
* string task_id = 22;
* @return The taskId.
*/
@java.lang.Override
public java.lang.String getTaskId() {
java.lang.Object ref = taskId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
taskId_ = s;
return s;
}
}
/**
* string task_id = 22;
* @return The bytes for taskId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTaskIdBytes() {
java.lang.Object ref = taskId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
taskId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REASON_FOR_INCOMPLETION_FIELD_NUMBER = 23;
@SuppressWarnings("serial")
private volatile java.lang.Object reasonForIncompletion_ = "";
/**
* string reason_for_incompletion = 23;
* @return The reasonForIncompletion.
*/
@java.lang.Override
public java.lang.String getReasonForIncompletion() {
java.lang.Object ref = reasonForIncompletion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
reasonForIncompletion_ = s;
return s;
}
}
/**
* string reason_for_incompletion = 23;
* @return The bytes for reasonForIncompletion.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getReasonForIncompletionBytes() {
java.lang.Object ref = reasonForIncompletion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reasonForIncompletion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CALLBACK_AFTER_SECONDS_FIELD_NUMBER = 24;
private long callbackAfterSeconds_ = 0L;
/**
* int64 callback_after_seconds = 24;
* @return The callbackAfterSeconds.
*/
@java.lang.Override
public long getCallbackAfterSeconds() {
return callbackAfterSeconds_;
}
public static final int WORKER_ID_FIELD_NUMBER = 25;
@SuppressWarnings("serial")
private volatile java.lang.Object workerId_ = "";
/**
* string worker_id = 25;
* @return The workerId.
*/
@java.lang.Override
public java.lang.String getWorkerId() {
java.lang.Object ref = workerId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workerId_ = s;
return s;
}
}
/**
* string worker_id = 25;
* @return The bytes for workerId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkerIdBytes() {
java.lang.Object ref = workerId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OUTPUT_DATA_FIELD_NUMBER = 26;
private static final class OutputDataDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.google.protobuf.Value> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.netflix.conductor.proto.TaskPb.internal_static_conductor_proto_Task_OutputDataEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.protobuf.Value.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, com.google.protobuf.Value> outputData_;
private com.google.protobuf.MapField
internalGetOutputData() {
if (outputData_ == null) {
return com.google.protobuf.MapField.emptyMapField(
OutputDataDefaultEntryHolder.defaultEntry);
}
return outputData_;
}
public int getOutputDataCount() {
return internalGetOutputData().getMap().size();
}
/**
* map<string, .google.protobuf.Value> output_data = 26;
*/
@java.lang.Override
public boolean containsOutputData(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetOutputData().getMap().containsKey(key);
}
/**
* Use {@link #getOutputDataMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getOutputData() {
return getOutputDataMap();
}
/**
* map<string, .google.protobuf.Value> output_data = 26;
*/
@java.lang.Override
public java.util.Map getOutputDataMap() {
return internalGetOutputData().getMap();
}
/**
* map<string, .google.protobuf.Value> output_data = 26;
*/
@java.lang.Override
public /* nullable */
com.google.protobuf.Value getOutputDataOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Value defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetOutputData().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .google.protobuf.Value> output_data = 26;
*/
@java.lang.Override
public com.google.protobuf.Value getOutputDataOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetOutputData().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int WORKFLOW_TASK_FIELD_NUMBER = 27;
private com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask workflowTask_;
/**
* .conductor.proto.WorkflowTask workflow_task = 27;
* @return Whether the workflowTask field is set.
*/
@java.lang.Override
public boolean hasWorkflowTask() {
return workflowTask_ != null;
}
/**
* .conductor.proto.WorkflowTask workflow_task = 27;
* @return The workflowTask.
*/
@java.lang.Override
public com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask getWorkflowTask() {
return workflowTask_ == null ? com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask.getDefaultInstance() : workflowTask_;
}
/**
* .conductor.proto.WorkflowTask workflow_task = 27;
*/
@java.lang.Override
public com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTaskOrBuilder getWorkflowTaskOrBuilder() {
return workflowTask_ == null ? com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask.getDefaultInstance() : workflowTask_;
}
public static final int DOMAIN_FIELD_NUMBER = 28;
@SuppressWarnings("serial")
private volatile java.lang.Object domain_ = "";
/**
* string domain = 28;
* @return The domain.
*/
@java.lang.Override
public java.lang.String getDomain() {
java.lang.Object ref = domain_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
domain_ = s;
return s;
}
}
/**
* string domain = 28;
* @return The bytes for domain.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDomainBytes() {
java.lang.Object ref = domain_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
domain_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INPUT_MESSAGE_FIELD_NUMBER = 29;
private com.google.protobuf.Any inputMessage_;
/**
* .google.protobuf.Any input_message = 29;
* @return Whether the inputMessage field is set.
*/
@java.lang.Override
public boolean hasInputMessage() {
return inputMessage_ != null;
}
/**
* .google.protobuf.Any input_message = 29;
* @return The inputMessage.
*/
@java.lang.Override
public com.google.protobuf.Any getInputMessage() {
return inputMessage_ == null ? com.google.protobuf.Any.getDefaultInstance() : inputMessage_;
}
/**
* .google.protobuf.Any input_message = 29;
*/
@java.lang.Override
public com.google.protobuf.AnyOrBuilder getInputMessageOrBuilder() {
return inputMessage_ == null ? com.google.protobuf.Any.getDefaultInstance() : inputMessage_;
}
public static final int OUTPUT_MESSAGE_FIELD_NUMBER = 30;
private com.google.protobuf.Any outputMessage_;
/**
* .google.protobuf.Any output_message = 30;
* @return Whether the outputMessage field is set.
*/
@java.lang.Override
public boolean hasOutputMessage() {
return outputMessage_ != null;
}
/**
* .google.protobuf.Any output_message = 30;
* @return The outputMessage.
*/
@java.lang.Override
public com.google.protobuf.Any getOutputMessage() {
return outputMessage_ == null ? com.google.protobuf.Any.getDefaultInstance() : outputMessage_;
}
/**
* .google.protobuf.Any output_message = 30;
*/
@java.lang.Override
public com.google.protobuf.AnyOrBuilder getOutputMessageOrBuilder() {
return outputMessage_ == null ? com.google.protobuf.Any.getDefaultInstance() : outputMessage_;
}
public static final int RATE_LIMIT_PER_FREQUENCY_FIELD_NUMBER = 32;
private int rateLimitPerFrequency_ = 0;
/**
* int32 rate_limit_per_frequency = 32;
* @return The rateLimitPerFrequency.
*/
@java.lang.Override
public int getRateLimitPerFrequency() {
return rateLimitPerFrequency_;
}
public static final int RATE_LIMIT_FREQUENCY_IN_SECONDS_FIELD_NUMBER = 33;
private int rateLimitFrequencyInSeconds_ = 0;
/**
* int32 rate_limit_frequency_in_seconds = 33;
* @return The rateLimitFrequencyInSeconds.
*/
@java.lang.Override
public int getRateLimitFrequencyInSeconds() {
return rateLimitFrequencyInSeconds_;
}
public static final int EXTERNAL_INPUT_PAYLOAD_STORAGE_PATH_FIELD_NUMBER = 34;
@SuppressWarnings("serial")
private volatile java.lang.Object externalInputPayloadStoragePath_ = "";
/**
* string external_input_payload_storage_path = 34;
* @return The externalInputPayloadStoragePath.
*/
@java.lang.Override
public java.lang.String getExternalInputPayloadStoragePath() {
java.lang.Object ref = externalInputPayloadStoragePath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
externalInputPayloadStoragePath_ = s;
return s;
}
}
/**
* string external_input_payload_storage_path = 34;
* @return The bytes for externalInputPayloadStoragePath.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getExternalInputPayloadStoragePathBytes() {
java.lang.Object ref = externalInputPayloadStoragePath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
externalInputPayloadStoragePath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EXTERNAL_OUTPUT_PAYLOAD_STORAGE_PATH_FIELD_NUMBER = 35;
@SuppressWarnings("serial")
private volatile java.lang.Object externalOutputPayloadStoragePath_ = "";
/**
* string external_output_payload_storage_path = 35;
* @return The externalOutputPayloadStoragePath.
*/
@java.lang.Override
public java.lang.String getExternalOutputPayloadStoragePath() {
java.lang.Object ref = externalOutputPayloadStoragePath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
externalOutputPayloadStoragePath_ = s;
return s;
}
}
/**
* string external_output_payload_storage_path = 35;
* @return The bytes for externalOutputPayloadStoragePath.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getExternalOutputPayloadStoragePathBytes() {
java.lang.Object ref = externalOutputPayloadStoragePath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
externalOutputPayloadStoragePath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int WORKFLOW_PRIORITY_FIELD_NUMBER = 36;
private int workflowPriority_ = 0;
/**
* int32 workflow_priority = 36;
* @return The workflowPriority.
*/
@java.lang.Override
public int getWorkflowPriority() {
return workflowPriority_;
}
public static final int EXECUTION_NAME_SPACE_FIELD_NUMBER = 37;
@SuppressWarnings("serial")
private volatile java.lang.Object executionNameSpace_ = "";
/**
* string execution_name_space = 37;
* @return The executionNameSpace.
*/
@java.lang.Override
public java.lang.String getExecutionNameSpace() {
java.lang.Object ref = executionNameSpace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
executionNameSpace_ = s;
return s;
}
}
/**
* string execution_name_space = 37;
* @return The bytes for executionNameSpace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getExecutionNameSpaceBytes() {
java.lang.Object ref = executionNameSpace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
executionNameSpace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ISOLATION_GROUP_ID_FIELD_NUMBER = 38;
@SuppressWarnings("serial")
private volatile java.lang.Object isolationGroupId_ = "";
/**
* string isolation_group_id = 38;
* @return The isolationGroupId.
*/
@java.lang.Override
public java.lang.String getIsolationGroupId() {
java.lang.Object ref = isolationGroupId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
isolationGroupId_ = s;
return s;
}
}
/**
* string isolation_group_id = 38;
* @return The bytes for isolationGroupId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIsolationGroupIdBytes() {
java.lang.Object ref = isolationGroupId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
isolationGroupId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ITERATION_FIELD_NUMBER = 40;
private int iteration_ = 0;
/**
* int32 iteration = 40;
* @return The iteration.
*/
@java.lang.Override
public int getIteration() {
return iteration_;
}
public static final int SUB_WORKFLOW_ID_FIELD_NUMBER = 41;
@SuppressWarnings("serial")
private volatile java.lang.Object subWorkflowId_ = "";
/**
* string sub_workflow_id = 41;
* @return The subWorkflowId.
*/
@java.lang.Override
public java.lang.String getSubWorkflowId() {
java.lang.Object ref = subWorkflowId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subWorkflowId_ = s;
return s;
}
}
/**
* string sub_workflow_id = 41;
* @return The bytes for subWorkflowId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSubWorkflowIdBytes() {
java.lang.Object ref = subWorkflowId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
subWorkflowId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUBWORKFLOW_CHANGED_FIELD_NUMBER = 42;
private boolean subworkflowChanged_ = false;
/**
* bool subworkflow_changed = 42;
* @return The subworkflowChanged.
*/
@java.lang.Override
public boolean getSubworkflowChanged() {
return subworkflowChanged_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(taskType_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, taskType_);
}
if (status_ != com.netflix.conductor.proto.TaskPb.Task.Status.IN_PROGRESS.getNumber()) {
output.writeEnum(2, status_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetInputData(),
InputDataDefaultEntryHolder.defaultEntry,
3);
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(referenceTaskName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, referenceTaskName_);
}
if (retryCount_ != 0) {
output.writeInt32(5, retryCount_);
}
if (seq_ != 0) {
output.writeInt32(6, seq_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(correlationId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, correlationId_);
}
if (pollCount_ != 0) {
output.writeInt32(8, pollCount_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(taskDefName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, taskDefName_);
}
if (scheduledTime_ != 0L) {
output.writeInt64(10, scheduledTime_);
}
if (startTime_ != 0L) {
output.writeInt64(11, startTime_);
}
if (endTime_ != 0L) {
output.writeInt64(12, endTime_);
}
if (updateTime_ != 0L) {
output.writeInt64(13, updateTime_);
}
if (startDelayInSeconds_ != 0) {
output.writeInt32(14, startDelayInSeconds_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(retriedTaskId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, retriedTaskId_);
}
if (retried_ != false) {
output.writeBool(16, retried_);
}
if (executed_ != false) {
output.writeBool(17, executed_);
}
if (callbackFromWorker_ != false) {
output.writeBool(18, callbackFromWorker_);
}
if (responseTimeoutSeconds_ != 0L) {
output.writeInt64(19, responseTimeoutSeconds_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workflowInstanceId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 20, workflowInstanceId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workflowType_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 21, workflowType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(taskId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 22, taskId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(reasonForIncompletion_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 23, reasonForIncompletion_);
}
if (callbackAfterSeconds_ != 0L) {
output.writeInt64(24, callbackAfterSeconds_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workerId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 25, workerId_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetOutputData(),
OutputDataDefaultEntryHolder.defaultEntry,
26);
if (workflowTask_ != null) {
output.writeMessage(27, getWorkflowTask());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(domain_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 28, domain_);
}
if (inputMessage_ != null) {
output.writeMessage(29, getInputMessage());
}
if (outputMessage_ != null) {
output.writeMessage(30, getOutputMessage());
}
if (rateLimitPerFrequency_ != 0) {
output.writeInt32(32, rateLimitPerFrequency_);
}
if (rateLimitFrequencyInSeconds_ != 0) {
output.writeInt32(33, rateLimitFrequencyInSeconds_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(externalInputPayloadStoragePath_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 34, externalInputPayloadStoragePath_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(externalOutputPayloadStoragePath_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 35, externalOutputPayloadStoragePath_);
}
if (workflowPriority_ != 0) {
output.writeInt32(36, workflowPriority_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(executionNameSpace_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 37, executionNameSpace_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(isolationGroupId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 38, isolationGroupId_);
}
if (iteration_ != 0) {
output.writeInt32(40, iteration_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(subWorkflowId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 41, subWorkflowId_);
}
if (subworkflowChanged_ != false) {
output.writeBool(42, subworkflowChanged_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(taskType_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, taskType_);
}
if (status_ != com.netflix.conductor.proto.TaskPb.Task.Status.IN_PROGRESS.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, status_);
}
for (java.util.Map.Entry entry
: internalGetInputData().getMap().entrySet()) {
com.google.protobuf.MapEntry
inputData__ = InputDataDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, inputData__);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(referenceTaskName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, referenceTaskName_);
}
if (retryCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, retryCount_);
}
if (seq_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(6, seq_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(correlationId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, correlationId_);
}
if (pollCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(8, pollCount_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(taskDefName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, taskDefName_);
}
if (scheduledTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(10, scheduledTime_);
}
if (startTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(11, startTime_);
}
if (endTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(12, endTime_);
}
if (updateTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(13, updateTime_);
}
if (startDelayInSeconds_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(14, startDelayInSeconds_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(retriedTaskId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, retriedTaskId_);
}
if (retried_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(16, retried_);
}
if (executed_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(17, executed_);
}
if (callbackFromWorker_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(18, callbackFromWorker_);
}
if (responseTimeoutSeconds_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(19, responseTimeoutSeconds_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workflowInstanceId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(20, workflowInstanceId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workflowType_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(21, workflowType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(taskId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(22, taskId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(reasonForIncompletion_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(23, reasonForIncompletion_);
}
if (callbackAfterSeconds_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(24, callbackAfterSeconds_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workerId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(25, workerId_);
}
for (java.util.Map.Entry entry
: internalGetOutputData().getMap().entrySet()) {
com.google.protobuf.MapEntry
outputData__ = OutputDataDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(26, outputData__);
}
if (workflowTask_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(27, getWorkflowTask());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(domain_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(28, domain_);
}
if (inputMessage_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(29, getInputMessage());
}
if (outputMessage_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(30, getOutputMessage());
}
if (rateLimitPerFrequency_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(32, rateLimitPerFrequency_);
}
if (rateLimitFrequencyInSeconds_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(33, rateLimitFrequencyInSeconds_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(externalInputPayloadStoragePath_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(34, externalInputPayloadStoragePath_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(externalOutputPayloadStoragePath_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(35, externalOutputPayloadStoragePath_);
}
if (workflowPriority_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(36, workflowPriority_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(executionNameSpace_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(37, executionNameSpace_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(isolationGroupId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(38, isolationGroupId_);
}
if (iteration_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(40, iteration_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(subWorkflowId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(41, subWorkflowId_);
}
if (subworkflowChanged_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(42, subworkflowChanged_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.netflix.conductor.proto.TaskPb.Task)) {
return super.equals(obj);
}
com.netflix.conductor.proto.TaskPb.Task other = (com.netflix.conductor.proto.TaskPb.Task) obj;
if (!getTaskType()
.equals(other.getTaskType())) return false;
if (status_ != other.status_) return false;
if (!internalGetInputData().equals(
other.internalGetInputData())) return false;
if (!getReferenceTaskName()
.equals(other.getReferenceTaskName())) return false;
if (getRetryCount()
!= other.getRetryCount()) return false;
if (getSeq()
!= other.getSeq()) return false;
if (!getCorrelationId()
.equals(other.getCorrelationId())) return false;
if (getPollCount()
!= other.getPollCount()) return false;
if (!getTaskDefName()
.equals(other.getTaskDefName())) return false;
if (getScheduledTime()
!= other.getScheduledTime()) return false;
if (getStartTime()
!= other.getStartTime()) return false;
if (getEndTime()
!= other.getEndTime()) return false;
if (getUpdateTime()
!= other.getUpdateTime()) return false;
if (getStartDelayInSeconds()
!= other.getStartDelayInSeconds()) return false;
if (!getRetriedTaskId()
.equals(other.getRetriedTaskId())) return false;
if (getRetried()
!= other.getRetried()) return false;
if (getExecuted()
!= other.getExecuted()) return false;
if (getCallbackFromWorker()
!= other.getCallbackFromWorker()) return false;
if (getResponseTimeoutSeconds()
!= other.getResponseTimeoutSeconds()) return false;
if (!getWorkflowInstanceId()
.equals(other.getWorkflowInstanceId())) return false;
if (!getWorkflowType()
.equals(other.getWorkflowType())) return false;
if (!getTaskId()
.equals(other.getTaskId())) return false;
if (!getReasonForIncompletion()
.equals(other.getReasonForIncompletion())) return false;
if (getCallbackAfterSeconds()
!= other.getCallbackAfterSeconds()) return false;
if (!getWorkerId()
.equals(other.getWorkerId())) return false;
if (!internalGetOutputData().equals(
other.internalGetOutputData())) return false;
if (hasWorkflowTask() != other.hasWorkflowTask()) return false;
if (hasWorkflowTask()) {
if (!getWorkflowTask()
.equals(other.getWorkflowTask())) return false;
}
if (!getDomain()
.equals(other.getDomain())) return false;
if (hasInputMessage() != other.hasInputMessage()) return false;
if (hasInputMessage()) {
if (!getInputMessage()
.equals(other.getInputMessage())) return false;
}
if (hasOutputMessage() != other.hasOutputMessage()) return false;
if (hasOutputMessage()) {
if (!getOutputMessage()
.equals(other.getOutputMessage())) return false;
}
if (getRateLimitPerFrequency()
!= other.getRateLimitPerFrequency()) return false;
if (getRateLimitFrequencyInSeconds()
!= other.getRateLimitFrequencyInSeconds()) return false;
if (!getExternalInputPayloadStoragePath()
.equals(other.getExternalInputPayloadStoragePath())) return false;
if (!getExternalOutputPayloadStoragePath()
.equals(other.getExternalOutputPayloadStoragePath())) return false;
if (getWorkflowPriority()
!= other.getWorkflowPriority()) return false;
if (!getExecutionNameSpace()
.equals(other.getExecutionNameSpace())) return false;
if (!getIsolationGroupId()
.equals(other.getIsolationGroupId())) return false;
if (getIteration()
!= other.getIteration()) return false;
if (!getSubWorkflowId()
.equals(other.getSubWorkflowId())) return false;
if (getSubworkflowChanged()
!= other.getSubworkflowChanged()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TASK_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getTaskType().hashCode();
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
if (!internalGetInputData().getMap().isEmpty()) {
hash = (37 * hash) + INPUT_DATA_FIELD_NUMBER;
hash = (53 * hash) + internalGetInputData().hashCode();
}
hash = (37 * hash) + REFERENCE_TASK_NAME_FIELD_NUMBER;
hash = (53 * hash) + getReferenceTaskName().hashCode();
hash = (37 * hash) + RETRY_COUNT_FIELD_NUMBER;
hash = (53 * hash) + getRetryCount();
hash = (37 * hash) + SEQ_FIELD_NUMBER;
hash = (53 * hash) + getSeq();
hash = (37 * hash) + CORRELATION_ID_FIELD_NUMBER;
hash = (53 * hash) + getCorrelationId().hashCode();
hash = (37 * hash) + POLL_COUNT_FIELD_NUMBER;
hash = (53 * hash) + getPollCount();
hash = (37 * hash) + TASK_DEF_NAME_FIELD_NUMBER;
hash = (53 * hash) + getTaskDefName().hashCode();
hash = (37 * hash) + SCHEDULED_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getScheduledTime());
hash = (37 * hash) + START_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getStartTime());
hash = (37 * hash) + END_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getEndTime());
hash = (37 * hash) + UPDATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getUpdateTime());
hash = (37 * hash) + START_DELAY_IN_SECONDS_FIELD_NUMBER;
hash = (53 * hash) + getStartDelayInSeconds();
hash = (37 * hash) + RETRIED_TASK_ID_FIELD_NUMBER;
hash = (53 * hash) + getRetriedTaskId().hashCode();
hash = (37 * hash) + RETRIED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getRetried());
hash = (37 * hash) + EXECUTED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getExecuted());
hash = (37 * hash) + CALLBACK_FROM_WORKER_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getCallbackFromWorker());
hash = (37 * hash) + RESPONSE_TIMEOUT_SECONDS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getResponseTimeoutSeconds());
hash = (37 * hash) + WORKFLOW_INSTANCE_ID_FIELD_NUMBER;
hash = (53 * hash) + getWorkflowInstanceId().hashCode();
hash = (37 * hash) + WORKFLOW_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getWorkflowType().hashCode();
hash = (37 * hash) + TASK_ID_FIELD_NUMBER;
hash = (53 * hash) + getTaskId().hashCode();
hash = (37 * hash) + REASON_FOR_INCOMPLETION_FIELD_NUMBER;
hash = (53 * hash) + getReasonForIncompletion().hashCode();
hash = (37 * hash) + CALLBACK_AFTER_SECONDS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCallbackAfterSeconds());
hash = (37 * hash) + WORKER_ID_FIELD_NUMBER;
hash = (53 * hash) + getWorkerId().hashCode();
if (!internalGetOutputData().getMap().isEmpty()) {
hash = (37 * hash) + OUTPUT_DATA_FIELD_NUMBER;
hash = (53 * hash) + internalGetOutputData().hashCode();
}
if (hasWorkflowTask()) {
hash = (37 * hash) + WORKFLOW_TASK_FIELD_NUMBER;
hash = (53 * hash) + getWorkflowTask().hashCode();
}
hash = (37 * hash) + DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getDomain().hashCode();
if (hasInputMessage()) {
hash = (37 * hash) + INPUT_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getInputMessage().hashCode();
}
if (hasOutputMessage()) {
hash = (37 * hash) + OUTPUT_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getOutputMessage().hashCode();
}
hash = (37 * hash) + RATE_LIMIT_PER_FREQUENCY_FIELD_NUMBER;
hash = (53 * hash) + getRateLimitPerFrequency();
hash = (37 * hash) + RATE_LIMIT_FREQUENCY_IN_SECONDS_FIELD_NUMBER;
hash = (53 * hash) + getRateLimitFrequencyInSeconds();
hash = (37 * hash) + EXTERNAL_INPUT_PAYLOAD_STORAGE_PATH_FIELD_NUMBER;
hash = (53 * hash) + getExternalInputPayloadStoragePath().hashCode();
hash = (37 * hash) + EXTERNAL_OUTPUT_PAYLOAD_STORAGE_PATH_FIELD_NUMBER;
hash = (53 * hash) + getExternalOutputPayloadStoragePath().hashCode();
hash = (37 * hash) + WORKFLOW_PRIORITY_FIELD_NUMBER;
hash = (53 * hash) + getWorkflowPriority();
hash = (37 * hash) + EXECUTION_NAME_SPACE_FIELD_NUMBER;
hash = (53 * hash) + getExecutionNameSpace().hashCode();
hash = (37 * hash) + ISOLATION_GROUP_ID_FIELD_NUMBER;
hash = (53 * hash) + getIsolationGroupId().hashCode();
hash = (37 * hash) + ITERATION_FIELD_NUMBER;
hash = (53 * hash) + getIteration();
hash = (37 * hash) + SUB_WORKFLOW_ID_FIELD_NUMBER;
hash = (53 * hash) + getSubWorkflowId().hashCode();
hash = (37 * hash) + SUBWORKFLOW_CHANGED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSubworkflowChanged());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.netflix.conductor.proto.TaskPb.Task parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.TaskPb.Task parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.TaskPb.Task parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.TaskPb.Task parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.TaskPb.Task parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.TaskPb.Task parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.TaskPb.Task parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.TaskPb.Task parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.netflix.conductor.proto.TaskPb.Task parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.TaskPb.Task parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.netflix.conductor.proto.TaskPb.Task parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.TaskPb.Task parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.netflix.conductor.proto.TaskPb.Task prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code conductor.proto.Task}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:conductor.proto.Task)
com.netflix.conductor.proto.TaskPb.TaskOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.netflix.conductor.proto.TaskPb.internal_static_conductor_proto_Task_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 3:
return internalGetInputData();
case 26:
return internalGetOutputData();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 3:
return internalGetMutableInputData();
case 26:
return internalGetMutableOutputData();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.netflix.conductor.proto.TaskPb.internal_static_conductor_proto_Task_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.netflix.conductor.proto.TaskPb.Task.class, com.netflix.conductor.proto.TaskPb.Task.Builder.class);
}
// Construct using com.netflix.conductor.proto.TaskPb.Task.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
bitField1_ = 0;
taskType_ = "";
status_ = 0;
internalGetMutableInputData().clear();
referenceTaskName_ = "";
retryCount_ = 0;
seq_ = 0;
correlationId_ = "";
pollCount_ = 0;
taskDefName_ = "";
scheduledTime_ = 0L;
startTime_ = 0L;
endTime_ = 0L;
updateTime_ = 0L;
startDelayInSeconds_ = 0;
retriedTaskId_ = "";
retried_ = false;
executed_ = false;
callbackFromWorker_ = false;
responseTimeoutSeconds_ = 0L;
workflowInstanceId_ = "";
workflowType_ = "";
taskId_ = "";
reasonForIncompletion_ = "";
callbackAfterSeconds_ = 0L;
workerId_ = "";
internalGetMutableOutputData().clear();
workflowTask_ = null;
if (workflowTaskBuilder_ != null) {
workflowTaskBuilder_.dispose();
workflowTaskBuilder_ = null;
}
domain_ = "";
inputMessage_ = null;
if (inputMessageBuilder_ != null) {
inputMessageBuilder_.dispose();
inputMessageBuilder_ = null;
}
outputMessage_ = null;
if (outputMessageBuilder_ != null) {
outputMessageBuilder_.dispose();
outputMessageBuilder_ = null;
}
rateLimitPerFrequency_ = 0;
rateLimitFrequencyInSeconds_ = 0;
externalInputPayloadStoragePath_ = "";
externalOutputPayloadStoragePath_ = "";
workflowPriority_ = 0;
executionNameSpace_ = "";
isolationGroupId_ = "";
iteration_ = 0;
subWorkflowId_ = "";
subworkflowChanged_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.netflix.conductor.proto.TaskPb.internal_static_conductor_proto_Task_descriptor;
}
@java.lang.Override
public com.netflix.conductor.proto.TaskPb.Task getDefaultInstanceForType() {
return com.netflix.conductor.proto.TaskPb.Task.getDefaultInstance();
}
@java.lang.Override
public com.netflix.conductor.proto.TaskPb.Task build() {
com.netflix.conductor.proto.TaskPb.Task result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.netflix.conductor.proto.TaskPb.Task buildPartial() {
com.netflix.conductor.proto.TaskPb.Task result = new com.netflix.conductor.proto.TaskPb.Task(this);
if (bitField0_ != 0) { buildPartial0(result); }
if (bitField1_ != 0) { buildPartial1(result); }
onBuilt();
return result;
}
private void buildPartial0(com.netflix.conductor.proto.TaskPb.Task result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.taskType_ = taskType_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.status_ = status_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.inputData_ = internalGetInputData();
result.inputData_.makeImmutable();
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.referenceTaskName_ = referenceTaskName_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.retryCount_ = retryCount_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.seq_ = seq_;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.correlationId_ = correlationId_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.pollCount_ = pollCount_;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.taskDefName_ = taskDefName_;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.scheduledTime_ = scheduledTime_;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.startTime_ = startTime_;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.endTime_ = endTime_;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.updateTime_ = updateTime_;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.startDelayInSeconds_ = startDelayInSeconds_;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.retriedTaskId_ = retriedTaskId_;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
result.retried_ = retried_;
}
if (((from_bitField0_ & 0x00010000) != 0)) {
result.executed_ = executed_;
}
if (((from_bitField0_ & 0x00020000) != 0)) {
result.callbackFromWorker_ = callbackFromWorker_;
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.responseTimeoutSeconds_ = responseTimeoutSeconds_;
}
if (((from_bitField0_ & 0x00080000) != 0)) {
result.workflowInstanceId_ = workflowInstanceId_;
}
if (((from_bitField0_ & 0x00100000) != 0)) {
result.workflowType_ = workflowType_;
}
if (((from_bitField0_ & 0x00200000) != 0)) {
result.taskId_ = taskId_;
}
if (((from_bitField0_ & 0x00400000) != 0)) {
result.reasonForIncompletion_ = reasonForIncompletion_;
}
if (((from_bitField0_ & 0x00800000) != 0)) {
result.callbackAfterSeconds_ = callbackAfterSeconds_;
}
if (((from_bitField0_ & 0x01000000) != 0)) {
result.workerId_ = workerId_;
}
if (((from_bitField0_ & 0x02000000) != 0)) {
result.outputData_ = internalGetOutputData();
result.outputData_.makeImmutable();
}
if (((from_bitField0_ & 0x04000000) != 0)) {
result.workflowTask_ = workflowTaskBuilder_ == null
? workflowTask_
: workflowTaskBuilder_.build();
}
if (((from_bitField0_ & 0x08000000) != 0)) {
result.domain_ = domain_;
}
if (((from_bitField0_ & 0x10000000) != 0)) {
result.inputMessage_ = inputMessageBuilder_ == null
? inputMessage_
: inputMessageBuilder_.build();
}
if (((from_bitField0_ & 0x20000000) != 0)) {
result.outputMessage_ = outputMessageBuilder_ == null
? outputMessage_
: outputMessageBuilder_.build();
}
if (((from_bitField0_ & 0x40000000) != 0)) {
result.rateLimitPerFrequency_ = rateLimitPerFrequency_;
}
if (((from_bitField0_ & 0x80000000) != 0)) {
result.rateLimitFrequencyInSeconds_ = rateLimitFrequencyInSeconds_;
}
}
private void buildPartial1(com.netflix.conductor.proto.TaskPb.Task result) {
int from_bitField1_ = bitField1_;
if (((from_bitField1_ & 0x00000001) != 0)) {
result.externalInputPayloadStoragePath_ = externalInputPayloadStoragePath_;
}
if (((from_bitField1_ & 0x00000002) != 0)) {
result.externalOutputPayloadStoragePath_ = externalOutputPayloadStoragePath_;
}
if (((from_bitField1_ & 0x00000004) != 0)) {
result.workflowPriority_ = workflowPriority_;
}
if (((from_bitField1_ & 0x00000008) != 0)) {
result.executionNameSpace_ = executionNameSpace_;
}
if (((from_bitField1_ & 0x00000010) != 0)) {
result.isolationGroupId_ = isolationGroupId_;
}
if (((from_bitField1_ & 0x00000020) != 0)) {
result.iteration_ = iteration_;
}
if (((from_bitField1_ & 0x00000040) != 0)) {
result.subWorkflowId_ = subWorkflowId_;
}
if (((from_bitField1_ & 0x00000080) != 0)) {
result.subworkflowChanged_ = subworkflowChanged_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.netflix.conductor.proto.TaskPb.Task) {
return mergeFrom((com.netflix.conductor.proto.TaskPb.Task)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.netflix.conductor.proto.TaskPb.Task other) {
if (other == com.netflix.conductor.proto.TaskPb.Task.getDefaultInstance()) return this;
if (!other.getTaskType().isEmpty()) {
taskType_ = other.taskType_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
internalGetMutableInputData().mergeFrom(
other.internalGetInputData());
bitField0_ |= 0x00000004;
if (!other.getReferenceTaskName().isEmpty()) {
referenceTaskName_ = other.referenceTaskName_;
bitField0_ |= 0x00000008;
onChanged();
}
if (other.getRetryCount() != 0) {
setRetryCount(other.getRetryCount());
}
if (other.getSeq() != 0) {
setSeq(other.getSeq());
}
if (!other.getCorrelationId().isEmpty()) {
correlationId_ = other.correlationId_;
bitField0_ |= 0x00000040;
onChanged();
}
if (other.getPollCount() != 0) {
setPollCount(other.getPollCount());
}
if (!other.getTaskDefName().isEmpty()) {
taskDefName_ = other.taskDefName_;
bitField0_ |= 0x00000100;
onChanged();
}
if (other.getScheduledTime() != 0L) {
setScheduledTime(other.getScheduledTime());
}
if (other.getStartTime() != 0L) {
setStartTime(other.getStartTime());
}
if (other.getEndTime() != 0L) {
setEndTime(other.getEndTime());
}
if (other.getUpdateTime() != 0L) {
setUpdateTime(other.getUpdateTime());
}
if (other.getStartDelayInSeconds() != 0) {
setStartDelayInSeconds(other.getStartDelayInSeconds());
}
if (!other.getRetriedTaskId().isEmpty()) {
retriedTaskId_ = other.retriedTaskId_;
bitField0_ |= 0x00004000;
onChanged();
}
if (other.getRetried() != false) {
setRetried(other.getRetried());
}
if (other.getExecuted() != false) {
setExecuted(other.getExecuted());
}
if (other.getCallbackFromWorker() != false) {
setCallbackFromWorker(other.getCallbackFromWorker());
}
if (other.getResponseTimeoutSeconds() != 0L) {
setResponseTimeoutSeconds(other.getResponseTimeoutSeconds());
}
if (!other.getWorkflowInstanceId().isEmpty()) {
workflowInstanceId_ = other.workflowInstanceId_;
bitField0_ |= 0x00080000;
onChanged();
}
if (!other.getWorkflowType().isEmpty()) {
workflowType_ = other.workflowType_;
bitField0_ |= 0x00100000;
onChanged();
}
if (!other.getTaskId().isEmpty()) {
taskId_ = other.taskId_;
bitField0_ |= 0x00200000;
onChanged();
}
if (!other.getReasonForIncompletion().isEmpty()) {
reasonForIncompletion_ = other.reasonForIncompletion_;
bitField0_ |= 0x00400000;
onChanged();
}
if (other.getCallbackAfterSeconds() != 0L) {
setCallbackAfterSeconds(other.getCallbackAfterSeconds());
}
if (!other.getWorkerId().isEmpty()) {
workerId_ = other.workerId_;
bitField0_ |= 0x01000000;
onChanged();
}
internalGetMutableOutputData().mergeFrom(
other.internalGetOutputData());
bitField0_ |= 0x02000000;
if (other.hasWorkflowTask()) {
mergeWorkflowTask(other.getWorkflowTask());
}
if (!other.getDomain().isEmpty()) {
domain_ = other.domain_;
bitField0_ |= 0x08000000;
onChanged();
}
if (other.hasInputMessage()) {
mergeInputMessage(other.getInputMessage());
}
if (other.hasOutputMessage()) {
mergeOutputMessage(other.getOutputMessage());
}
if (other.getRateLimitPerFrequency() != 0) {
setRateLimitPerFrequency(other.getRateLimitPerFrequency());
}
if (other.getRateLimitFrequencyInSeconds() != 0) {
setRateLimitFrequencyInSeconds(other.getRateLimitFrequencyInSeconds());
}
if (!other.getExternalInputPayloadStoragePath().isEmpty()) {
externalInputPayloadStoragePath_ = other.externalInputPayloadStoragePath_;
bitField1_ |= 0x00000001;
onChanged();
}
if (!other.getExternalOutputPayloadStoragePath().isEmpty()) {
externalOutputPayloadStoragePath_ = other.externalOutputPayloadStoragePath_;
bitField1_ |= 0x00000002;
onChanged();
}
if (other.getWorkflowPriority() != 0) {
setWorkflowPriority(other.getWorkflowPriority());
}
if (!other.getExecutionNameSpace().isEmpty()) {
executionNameSpace_ = other.executionNameSpace_;
bitField1_ |= 0x00000008;
onChanged();
}
if (!other.getIsolationGroupId().isEmpty()) {
isolationGroupId_ = other.isolationGroupId_;
bitField1_ |= 0x00000010;
onChanged();
}
if (other.getIteration() != 0) {
setIteration(other.getIteration());
}
if (!other.getSubWorkflowId().isEmpty()) {
subWorkflowId_ = other.subWorkflowId_;
bitField1_ |= 0x00000040;
onChanged();
}
if (other.getSubworkflowChanged() != false) {
setSubworkflowChanged(other.getSubworkflowChanged());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
taskType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
status_ = input.readEnum();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26: {
com.google.protobuf.MapEntry
inputData__ = input.readMessage(
InputDataDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
internalGetMutableInputData().getMutableMap().put(
inputData__.getKey(), inputData__.getValue());
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
referenceTaskName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 34
case 40: {
retryCount_ = input.readInt32();
bitField0_ |= 0x00000010;
break;
} // case 40
case 48: {
seq_ = input.readInt32();
bitField0_ |= 0x00000020;
break;
} // case 48
case 58: {
correlationId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 58
case 64: {
pollCount_ = input.readInt32();
bitField0_ |= 0x00000080;
break;
} // case 64
case 74: {
taskDefName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000100;
break;
} // case 74
case 80: {
scheduledTime_ = input.readInt64();
bitField0_ |= 0x00000200;
break;
} // case 80
case 88: {
startTime_ = input.readInt64();
bitField0_ |= 0x00000400;
break;
} // case 88
case 96: {
endTime_ = input.readInt64();
bitField0_ |= 0x00000800;
break;
} // case 96
case 104: {
updateTime_ = input.readInt64();
bitField0_ |= 0x00001000;
break;
} // case 104
case 112: {
startDelayInSeconds_ = input.readInt32();
bitField0_ |= 0x00002000;
break;
} // case 112
case 122: {
retriedTaskId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00004000;
break;
} // case 122
case 128: {
retried_ = input.readBool();
bitField0_ |= 0x00008000;
break;
} // case 128
case 136: {
executed_ = input.readBool();
bitField0_ |= 0x00010000;
break;
} // case 136
case 144: {
callbackFromWorker_ = input.readBool();
bitField0_ |= 0x00020000;
break;
} // case 144
case 152: {
responseTimeoutSeconds_ = input.readInt64();
bitField0_ |= 0x00040000;
break;
} // case 152
case 162: {
workflowInstanceId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00080000;
break;
} // case 162
case 170: {
workflowType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00100000;
break;
} // case 170
case 178: {
taskId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00200000;
break;
} // case 178
case 186: {
reasonForIncompletion_ = input.readStringRequireUtf8();
bitField0_ |= 0x00400000;
break;
} // case 186
case 192: {
callbackAfterSeconds_ = input.readInt64();
bitField0_ |= 0x00800000;
break;
} // case 192
case 202: {
workerId_ = input.readStringRequireUtf8();
bitField0_ |= 0x01000000;
break;
} // case 202
case 210: {
com.google.protobuf.MapEntry
outputData__ = input.readMessage(
OutputDataDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
internalGetMutableOutputData().getMutableMap().put(
outputData__.getKey(), outputData__.getValue());
bitField0_ |= 0x02000000;
break;
} // case 210
case 218: {
input.readMessage(
getWorkflowTaskFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x04000000;
break;
} // case 218
case 226: {
domain_ = input.readStringRequireUtf8();
bitField0_ |= 0x08000000;
break;
} // case 226
case 234: {
input.readMessage(
getInputMessageFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x10000000;
break;
} // case 234
case 242: {
input.readMessage(
getOutputMessageFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x20000000;
break;
} // case 242
case 256: {
rateLimitPerFrequency_ = input.readInt32();
bitField0_ |= 0x40000000;
break;
} // case 256
case 264: {
rateLimitFrequencyInSeconds_ = input.readInt32();
bitField0_ |= 0x80000000;
break;
} // case 264
case 274: {
externalInputPayloadStoragePath_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000001;
break;
} // case 274
case 282: {
externalOutputPayloadStoragePath_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000002;
break;
} // case 282
case 288: {
workflowPriority_ = input.readInt32();
bitField1_ |= 0x00000004;
break;
} // case 288
case 298: {
executionNameSpace_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000008;
break;
} // case 298
case 306: {
isolationGroupId_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000010;
break;
} // case 306
case 320: {
iteration_ = input.readInt32();
bitField1_ |= 0x00000020;
break;
} // case 320
case 330: {
subWorkflowId_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000040;
break;
} // case 330
case 336: {
subworkflowChanged_ = input.readBool();
bitField1_ |= 0x00000080;
break;
} // case 336
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int bitField1_;
private java.lang.Object taskType_ = "";
/**
* string task_type = 1;
* @return The taskType.
*/
public java.lang.String getTaskType() {
java.lang.Object ref = taskType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
taskType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string task_type = 1;
* @return The bytes for taskType.
*/
public com.google.protobuf.ByteString
getTaskTypeBytes() {
java.lang.Object ref = taskType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
taskType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string task_type = 1;
* @param value The taskType to set.
* @return This builder for chaining.
*/
public Builder setTaskType(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
taskType_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string task_type = 1;
* @return This builder for chaining.
*/
public Builder clearTaskType() {
taskType_ = getDefaultInstance().getTaskType();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string task_type = 1;
* @param value The bytes for taskType to set.
* @return This builder for chaining.
*/
public Builder setTaskTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
taskType_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private int status_ = 0;
/**
* .conductor.proto.Task.Status status = 2;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
* .conductor.proto.Task.Status status = 2;
* @param value The enum numeric value on the wire for status to set.
* @return This builder for chaining.
*/
public Builder setStatusValue(int value) {
status_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .conductor.proto.Task.Status status = 2;
* @return The status.
*/
@java.lang.Override
public com.netflix.conductor.proto.TaskPb.Task.Status getStatus() {
com.netflix.conductor.proto.TaskPb.Task.Status result = com.netflix.conductor.proto.TaskPb.Task.Status.forNumber(status_);
return result == null ? com.netflix.conductor.proto.TaskPb.Task.Status.UNRECOGNIZED : result;
}
/**
* .conductor.proto.Task.Status status = 2;
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(com.netflix.conductor.proto.TaskPb.Task.Status value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
status_ = value.getNumber();
onChanged();
return this;
}
/**
* .conductor.proto.Task.Status status = 2;
* @return This builder for chaining.
*/
public Builder clearStatus() {
bitField0_ = (bitField0_ & ~0x00000002);
status_ = 0;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, com.google.protobuf.Value> inputData_;
private com.google.protobuf.MapField
internalGetInputData() {
if (inputData_ == null) {
return com.google.protobuf.MapField.emptyMapField(
InputDataDefaultEntryHolder.defaultEntry);
}
return inputData_;
}
private com.google.protobuf.MapField
internalGetMutableInputData() {
if (inputData_ == null) {
inputData_ = com.google.protobuf.MapField.newMapField(
InputDataDefaultEntryHolder.defaultEntry);
}
if (!inputData_.isMutable()) {
inputData_ = inputData_.copy();
}
bitField0_ |= 0x00000004;
onChanged();
return inputData_;
}
public int getInputDataCount() {
return internalGetInputData().getMap().size();
}
/**
* map<string, .google.protobuf.Value> input_data = 3;
*/
@java.lang.Override
public boolean containsInputData(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetInputData().getMap().containsKey(key);
}
/**
* Use {@link #getInputDataMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getInputData() {
return getInputDataMap();
}
/**
* map<string, .google.protobuf.Value> input_data = 3;
*/
@java.lang.Override
public java.util.Map getInputDataMap() {
return internalGetInputData().getMap();
}
/**
* map<string, .google.protobuf.Value> input_data = 3;
*/
@java.lang.Override
public /* nullable */
com.google.protobuf.Value getInputDataOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Value defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetInputData().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .google.protobuf.Value> input_data = 3;
*/
@java.lang.Override
public com.google.protobuf.Value getInputDataOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetInputData().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearInputData() {
bitField0_ = (bitField0_ & ~0x00000004);
internalGetMutableInputData().getMutableMap()
.clear();
return this;
}
/**
* map<string, .google.protobuf.Value> input_data = 3;
*/
public Builder removeInputData(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableInputData().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableInputData() {
bitField0_ |= 0x00000004;
return internalGetMutableInputData().getMutableMap();
}
/**
* map<string, .google.protobuf.Value> input_data = 3;
*/
public Builder putInputData(
java.lang.String key,
com.google.protobuf.Value value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) { throw new NullPointerException("map value"); }
internalGetMutableInputData().getMutableMap()
.put(key, value);
bitField0_ |= 0x00000004;
return this;
}
/**
* map<string, .google.protobuf.Value> input_data = 3;
*/
public Builder putAllInputData(
java.util.Map values) {
internalGetMutableInputData().getMutableMap()
.putAll(values);
bitField0_ |= 0x00000004;
return this;
}
private java.lang.Object referenceTaskName_ = "";
/**
* string reference_task_name = 4;
* @return The referenceTaskName.
*/
public java.lang.String getReferenceTaskName() {
java.lang.Object ref = referenceTaskName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
referenceTaskName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string reference_task_name = 4;
* @return The bytes for referenceTaskName.
*/
public com.google.protobuf.ByteString
getReferenceTaskNameBytes() {
java.lang.Object ref = referenceTaskName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
referenceTaskName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string reference_task_name = 4;
* @param value The referenceTaskName to set.
* @return This builder for chaining.
*/
public Builder setReferenceTaskName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
referenceTaskName_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* string reference_task_name = 4;
* @return This builder for chaining.
*/
public Builder clearReferenceTaskName() {
referenceTaskName_ = getDefaultInstance().getReferenceTaskName();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
* string reference_task_name = 4;
* @param value The bytes for referenceTaskName to set.
* @return This builder for chaining.
*/
public Builder setReferenceTaskNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
referenceTaskName_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private int retryCount_ ;
/**
* int32 retry_count = 5;
* @return The retryCount.
*/
@java.lang.Override
public int getRetryCount() {
return retryCount_;
}
/**
* int32 retry_count = 5;
* @param value The retryCount to set.
* @return This builder for chaining.
*/
public Builder setRetryCount(int value) {
retryCount_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* int32 retry_count = 5;
* @return This builder for chaining.
*/
public Builder clearRetryCount() {
bitField0_ = (bitField0_ & ~0x00000010);
retryCount_ = 0;
onChanged();
return this;
}
private int seq_ ;
/**
* int32 seq = 6;
* @return The seq.
*/
@java.lang.Override
public int getSeq() {
return seq_;
}
/**
* int32 seq = 6;
* @param value The seq to set.
* @return This builder for chaining.
*/
public Builder setSeq(int value) {
seq_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* int32 seq = 6;
* @return This builder for chaining.
*/
public Builder clearSeq() {
bitField0_ = (bitField0_ & ~0x00000020);
seq_ = 0;
onChanged();
return this;
}
private java.lang.Object correlationId_ = "";
/**
* string correlation_id = 7;
* @return The correlationId.
*/
public java.lang.String getCorrelationId() {
java.lang.Object ref = correlationId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
correlationId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string correlation_id = 7;
* @return The bytes for correlationId.
*/
public com.google.protobuf.ByteString
getCorrelationIdBytes() {
java.lang.Object ref = correlationId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
correlationId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string correlation_id = 7;
* @param value The correlationId to set.
* @return This builder for chaining.
*/
public Builder setCorrelationId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
correlationId_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
* string correlation_id = 7;
* @return This builder for chaining.
*/
public Builder clearCorrelationId() {
correlationId_ = getDefaultInstance().getCorrelationId();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
* string correlation_id = 7;
* @param value The bytes for correlationId to set.
* @return This builder for chaining.
*/
public Builder setCorrelationIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
correlationId_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private int pollCount_ ;
/**
* int32 poll_count = 8;
* @return The pollCount.
*/
@java.lang.Override
public int getPollCount() {
return pollCount_;
}
/**
* int32 poll_count = 8;
* @param value The pollCount to set.
* @return This builder for chaining.
*/
public Builder setPollCount(int value) {
pollCount_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
* int32 poll_count = 8;
* @return This builder for chaining.
*/
public Builder clearPollCount() {
bitField0_ = (bitField0_ & ~0x00000080);
pollCount_ = 0;
onChanged();
return this;
}
private java.lang.Object taskDefName_ = "";
/**
* string task_def_name = 9;
* @return The taskDefName.
*/
public java.lang.String getTaskDefName() {
java.lang.Object ref = taskDefName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
taskDefName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string task_def_name = 9;
* @return The bytes for taskDefName.
*/
public com.google.protobuf.ByteString
getTaskDefNameBytes() {
java.lang.Object ref = taskDefName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
taskDefName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string task_def_name = 9;
* @param value The taskDefName to set.
* @return This builder for chaining.
*/
public Builder setTaskDefName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
taskDefName_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
* string task_def_name = 9;
* @return This builder for chaining.
*/
public Builder clearTaskDefName() {
taskDefName_ = getDefaultInstance().getTaskDefName();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
/**
* string task_def_name = 9;
* @param value The bytes for taskDefName to set.
* @return This builder for chaining.
*/
public Builder setTaskDefNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
taskDefName_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
private long scheduledTime_ ;
/**
* int64 scheduled_time = 10;
* @return The scheduledTime.
*/
@java.lang.Override
public long getScheduledTime() {
return scheduledTime_;
}
/**
* int64 scheduled_time = 10;
* @param value The scheduledTime to set.
* @return This builder for chaining.
*/
public Builder setScheduledTime(long value) {
scheduledTime_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
* int64 scheduled_time = 10;
* @return This builder for chaining.
*/
public Builder clearScheduledTime() {
bitField0_ = (bitField0_ & ~0x00000200);
scheduledTime_ = 0L;
onChanged();
return this;
}
private long startTime_ ;
/**
* int64 start_time = 11;
* @return The startTime.
*/
@java.lang.Override
public long getStartTime() {
return startTime_;
}
/**
* int64 start_time = 11;
* @param value The startTime to set.
* @return This builder for chaining.
*/
public Builder setStartTime(long value) {
startTime_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
* int64 start_time = 11;
* @return This builder for chaining.
*/
public Builder clearStartTime() {
bitField0_ = (bitField0_ & ~0x00000400);
startTime_ = 0L;
onChanged();
return this;
}
private long endTime_ ;
/**
* int64 end_time = 12;
* @return The endTime.
*/
@java.lang.Override
public long getEndTime() {
return endTime_;
}
/**
* int64 end_time = 12;
* @param value The endTime to set.
* @return This builder for chaining.
*/
public Builder setEndTime(long value) {
endTime_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
* int64 end_time = 12;
* @return This builder for chaining.
*/
public Builder clearEndTime() {
bitField0_ = (bitField0_ & ~0x00000800);
endTime_ = 0L;
onChanged();
return this;
}
private long updateTime_ ;
/**
* int64 update_time = 13;
* @return The updateTime.
*/
@java.lang.Override
public long getUpdateTime() {
return updateTime_;
}
/**
* int64 update_time = 13;
* @param value The updateTime to set.
* @return This builder for chaining.
*/
public Builder setUpdateTime(long value) {
updateTime_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
* int64 update_time = 13;
* @return This builder for chaining.
*/
public Builder clearUpdateTime() {
bitField0_ = (bitField0_ & ~0x00001000);
updateTime_ = 0L;
onChanged();
return this;
}
private int startDelayInSeconds_ ;
/**
* int32 start_delay_in_seconds = 14;
* @return The startDelayInSeconds.
*/
@java.lang.Override
public int getStartDelayInSeconds() {
return startDelayInSeconds_;
}
/**
* int32 start_delay_in_seconds = 14;
* @param value The startDelayInSeconds to set.
* @return This builder for chaining.
*/
public Builder setStartDelayInSeconds(int value) {
startDelayInSeconds_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
* int32 start_delay_in_seconds = 14;
* @return This builder for chaining.
*/
public Builder clearStartDelayInSeconds() {
bitField0_ = (bitField0_ & ~0x00002000);
startDelayInSeconds_ = 0;
onChanged();
return this;
}
private java.lang.Object retriedTaskId_ = "";
/**
* string retried_task_id = 15;
* @return The retriedTaskId.
*/
public java.lang.String getRetriedTaskId() {
java.lang.Object ref = retriedTaskId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
retriedTaskId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string retried_task_id = 15;
* @return The bytes for retriedTaskId.
*/
public com.google.protobuf.ByteString
getRetriedTaskIdBytes() {
java.lang.Object ref = retriedTaskId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
retriedTaskId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string retried_task_id = 15;
* @param value The retriedTaskId to set.
* @return This builder for chaining.
*/
public Builder setRetriedTaskId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
retriedTaskId_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
* string retried_task_id = 15;
* @return This builder for chaining.
*/
public Builder clearRetriedTaskId() {
retriedTaskId_ = getDefaultInstance().getRetriedTaskId();
bitField0_ = (bitField0_ & ~0x00004000);
onChanged();
return this;
}
/**
* string retried_task_id = 15;
* @param value The bytes for retriedTaskId to set.
* @return This builder for chaining.
*/
public Builder setRetriedTaskIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
retriedTaskId_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
private boolean retried_ ;
/**
* bool retried = 16;
* @return The retried.
*/
@java.lang.Override
public boolean getRetried() {
return retried_;
}
/**
* bool retried = 16;
* @param value The retried to set.
* @return This builder for chaining.
*/
public Builder setRetried(boolean value) {
retried_ = value;
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
* bool retried = 16;
* @return This builder for chaining.
*/
public Builder clearRetried() {
bitField0_ = (bitField0_ & ~0x00008000);
retried_ = false;
onChanged();
return this;
}
private boolean executed_ ;
/**
* bool executed = 17;
* @return The executed.
*/
@java.lang.Override
public boolean getExecuted() {
return executed_;
}
/**
* bool executed = 17;
* @param value The executed to set.
* @return This builder for chaining.
*/
public Builder setExecuted(boolean value) {
executed_ = value;
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
* bool executed = 17;
* @return This builder for chaining.
*/
public Builder clearExecuted() {
bitField0_ = (bitField0_ & ~0x00010000);
executed_ = false;
onChanged();
return this;
}
private boolean callbackFromWorker_ ;
/**
* bool callback_from_worker = 18;
* @return The callbackFromWorker.
*/
@java.lang.Override
public boolean getCallbackFromWorker() {
return callbackFromWorker_;
}
/**
* bool callback_from_worker = 18;
* @param value The callbackFromWorker to set.
* @return This builder for chaining.
*/
public Builder setCallbackFromWorker(boolean value) {
callbackFromWorker_ = value;
bitField0_ |= 0x00020000;
onChanged();
return this;
}
/**
* bool callback_from_worker = 18;
* @return This builder for chaining.
*/
public Builder clearCallbackFromWorker() {
bitField0_ = (bitField0_ & ~0x00020000);
callbackFromWorker_ = false;
onChanged();
return this;
}
private long responseTimeoutSeconds_ ;
/**
* int64 response_timeout_seconds = 19;
* @return The responseTimeoutSeconds.
*/
@java.lang.Override
public long getResponseTimeoutSeconds() {
return responseTimeoutSeconds_;
}
/**
* int64 response_timeout_seconds = 19;
* @param value The responseTimeoutSeconds to set.
* @return This builder for chaining.
*/
public Builder setResponseTimeoutSeconds(long value) {
responseTimeoutSeconds_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
* int64 response_timeout_seconds = 19;
* @return This builder for chaining.
*/
public Builder clearResponseTimeoutSeconds() {
bitField0_ = (bitField0_ & ~0x00040000);
responseTimeoutSeconds_ = 0L;
onChanged();
return this;
}
private java.lang.Object workflowInstanceId_ = "";
/**
* string workflow_instance_id = 20;
* @return The workflowInstanceId.
*/
public java.lang.String getWorkflowInstanceId() {
java.lang.Object ref = workflowInstanceId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workflowInstanceId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string workflow_instance_id = 20;
* @return The bytes for workflowInstanceId.
*/
public com.google.protobuf.ByteString
getWorkflowInstanceIdBytes() {
java.lang.Object ref = workflowInstanceId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workflowInstanceId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string workflow_instance_id = 20;
* @param value The workflowInstanceId to set.
* @return This builder for chaining.
*/
public Builder setWorkflowInstanceId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
workflowInstanceId_ = value;
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
* string workflow_instance_id = 20;
* @return This builder for chaining.
*/
public Builder clearWorkflowInstanceId() {
workflowInstanceId_ = getDefaultInstance().getWorkflowInstanceId();
bitField0_ = (bitField0_ & ~0x00080000);
onChanged();
return this;
}
/**
* string workflow_instance_id = 20;
* @param value The bytes for workflowInstanceId to set.
* @return This builder for chaining.
*/
public Builder setWorkflowInstanceIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
workflowInstanceId_ = value;
bitField0_ |= 0x00080000;
onChanged();
return this;
}
private java.lang.Object workflowType_ = "";
/**
* string workflow_type = 21;
* @return The workflowType.
*/
public java.lang.String getWorkflowType() {
java.lang.Object ref = workflowType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workflowType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string workflow_type = 21;
* @return The bytes for workflowType.
*/
public com.google.protobuf.ByteString
getWorkflowTypeBytes() {
java.lang.Object ref = workflowType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workflowType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string workflow_type = 21;
* @param value The workflowType to set.
* @return This builder for chaining.
*/
public Builder setWorkflowType(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
workflowType_ = value;
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
* string workflow_type = 21;
* @return This builder for chaining.
*/
public Builder clearWorkflowType() {
workflowType_ = getDefaultInstance().getWorkflowType();
bitField0_ = (bitField0_ & ~0x00100000);
onChanged();
return this;
}
/**
* string workflow_type = 21;
* @param value The bytes for workflowType to set.
* @return This builder for chaining.
*/
public Builder setWorkflowTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
workflowType_ = value;
bitField0_ |= 0x00100000;
onChanged();
return this;
}
private java.lang.Object taskId_ = "";
/**
* string task_id = 22;
* @return The taskId.
*/
public java.lang.String getTaskId() {
java.lang.Object ref = taskId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
taskId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string task_id = 22;
* @return The bytes for taskId.
*/
public com.google.protobuf.ByteString
getTaskIdBytes() {
java.lang.Object ref = taskId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
taskId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string task_id = 22;
* @param value The taskId to set.
* @return This builder for chaining.
*/
public Builder setTaskId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
taskId_ = value;
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
* string task_id = 22;
* @return This builder for chaining.
*/
public Builder clearTaskId() {
taskId_ = getDefaultInstance().getTaskId();
bitField0_ = (bitField0_ & ~0x00200000);
onChanged();
return this;
}
/**
* string task_id = 22;
* @param value The bytes for taskId to set.
* @return This builder for chaining.
*/
public Builder setTaskIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
taskId_ = value;
bitField0_ |= 0x00200000;
onChanged();
return this;
}
private java.lang.Object reasonForIncompletion_ = "";
/**
* string reason_for_incompletion = 23;
* @return The reasonForIncompletion.
*/
public java.lang.String getReasonForIncompletion() {
java.lang.Object ref = reasonForIncompletion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
reasonForIncompletion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string reason_for_incompletion = 23;
* @return The bytes for reasonForIncompletion.
*/
public com.google.protobuf.ByteString
getReasonForIncompletionBytes() {
java.lang.Object ref = reasonForIncompletion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reasonForIncompletion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string reason_for_incompletion = 23;
* @param value The reasonForIncompletion to set.
* @return This builder for chaining.
*/
public Builder setReasonForIncompletion(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
reasonForIncompletion_ = value;
bitField0_ |= 0x00400000;
onChanged();
return this;
}
/**
* string reason_for_incompletion = 23;
* @return This builder for chaining.
*/
public Builder clearReasonForIncompletion() {
reasonForIncompletion_ = getDefaultInstance().getReasonForIncompletion();
bitField0_ = (bitField0_ & ~0x00400000);
onChanged();
return this;
}
/**
* string reason_for_incompletion = 23;
* @param value The bytes for reasonForIncompletion to set.
* @return This builder for chaining.
*/
public Builder setReasonForIncompletionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
reasonForIncompletion_ = value;
bitField0_ |= 0x00400000;
onChanged();
return this;
}
private long callbackAfterSeconds_ ;
/**
* int64 callback_after_seconds = 24;
* @return The callbackAfterSeconds.
*/
@java.lang.Override
public long getCallbackAfterSeconds() {
return callbackAfterSeconds_;
}
/**
* int64 callback_after_seconds = 24;
* @param value The callbackAfterSeconds to set.
* @return This builder for chaining.
*/
public Builder setCallbackAfterSeconds(long value) {
callbackAfterSeconds_ = value;
bitField0_ |= 0x00800000;
onChanged();
return this;
}
/**
* int64 callback_after_seconds = 24;
* @return This builder for chaining.
*/
public Builder clearCallbackAfterSeconds() {
bitField0_ = (bitField0_ & ~0x00800000);
callbackAfterSeconds_ = 0L;
onChanged();
return this;
}
private java.lang.Object workerId_ = "";
/**
* string worker_id = 25;
* @return The workerId.
*/
public java.lang.String getWorkerId() {
java.lang.Object ref = workerId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workerId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string worker_id = 25;
* @return The bytes for workerId.
*/
public com.google.protobuf.ByteString
getWorkerIdBytes() {
java.lang.Object ref = workerId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string worker_id = 25;
* @param value The workerId to set.
* @return This builder for chaining.
*/
public Builder setWorkerId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
workerId_ = value;
bitField0_ |= 0x01000000;
onChanged();
return this;
}
/**
* string worker_id = 25;
* @return This builder for chaining.
*/
public Builder clearWorkerId() {
workerId_ = getDefaultInstance().getWorkerId();
bitField0_ = (bitField0_ & ~0x01000000);
onChanged();
return this;
}
/**
* string worker_id = 25;
* @param value The bytes for workerId to set.
* @return This builder for chaining.
*/
public Builder setWorkerIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
workerId_ = value;
bitField0_ |= 0x01000000;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, com.google.protobuf.Value> outputData_;
private com.google.protobuf.MapField
internalGetOutputData() {
if (outputData_ == null) {
return com.google.protobuf.MapField.emptyMapField(
OutputDataDefaultEntryHolder.defaultEntry);
}
return outputData_;
}
private com.google.protobuf.MapField
internalGetMutableOutputData() {
if (outputData_ == null) {
outputData_ = com.google.protobuf.MapField.newMapField(
OutputDataDefaultEntryHolder.defaultEntry);
}
if (!outputData_.isMutable()) {
outputData_ = outputData_.copy();
}
bitField0_ |= 0x02000000;
onChanged();
return outputData_;
}
public int getOutputDataCount() {
return internalGetOutputData().getMap().size();
}
/**
* map<string, .google.protobuf.Value> output_data = 26;
*/
@java.lang.Override
public boolean containsOutputData(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetOutputData().getMap().containsKey(key);
}
/**
* Use {@link #getOutputDataMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getOutputData() {
return getOutputDataMap();
}
/**
* map<string, .google.protobuf.Value> output_data = 26;
*/
@java.lang.Override
public java.util.Map getOutputDataMap() {
return internalGetOutputData().getMap();
}
/**
* map<string, .google.protobuf.Value> output_data = 26;
*/
@java.lang.Override
public /* nullable */
com.google.protobuf.Value getOutputDataOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Value defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetOutputData().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .google.protobuf.Value> output_data = 26;
*/
@java.lang.Override
public com.google.protobuf.Value getOutputDataOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetOutputData().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearOutputData() {
bitField0_ = (bitField0_ & ~0x02000000);
internalGetMutableOutputData().getMutableMap()
.clear();
return this;
}
/**
* map<string, .google.protobuf.Value> output_data = 26;
*/
public Builder removeOutputData(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableOutputData().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableOutputData() {
bitField0_ |= 0x02000000;
return internalGetMutableOutputData().getMutableMap();
}
/**
* map<string, .google.protobuf.Value> output_data = 26;
*/
public Builder putOutputData(
java.lang.String key,
com.google.protobuf.Value value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) { throw new NullPointerException("map value"); }
internalGetMutableOutputData().getMutableMap()
.put(key, value);
bitField0_ |= 0x02000000;
return this;
}
/**
* map<string, .google.protobuf.Value> output_data = 26;
*/
public Builder putAllOutputData(
java.util.Map values) {
internalGetMutableOutputData().getMutableMap()
.putAll(values);
bitField0_ |= 0x02000000;
return this;
}
private com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask workflowTask_;
private com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask, com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask.Builder, com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTaskOrBuilder> workflowTaskBuilder_;
/**
* .conductor.proto.WorkflowTask workflow_task = 27;
* @return Whether the workflowTask field is set.
*/
public boolean hasWorkflowTask() {
return ((bitField0_ & 0x04000000) != 0);
}
/**
* .conductor.proto.WorkflowTask workflow_task = 27;
* @return The workflowTask.
*/
public com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask getWorkflowTask() {
if (workflowTaskBuilder_ == null) {
return workflowTask_ == null ? com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask.getDefaultInstance() : workflowTask_;
} else {
return workflowTaskBuilder_.getMessage();
}
}
/**
* .conductor.proto.WorkflowTask workflow_task = 27;
*/
public Builder setWorkflowTask(com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask value) {
if (workflowTaskBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
workflowTask_ = value;
} else {
workflowTaskBuilder_.setMessage(value);
}
bitField0_ |= 0x04000000;
onChanged();
return this;
}
/**
* .conductor.proto.WorkflowTask workflow_task = 27;
*/
public Builder setWorkflowTask(
com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask.Builder builderForValue) {
if (workflowTaskBuilder_ == null) {
workflowTask_ = builderForValue.build();
} else {
workflowTaskBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x04000000;
onChanged();
return this;
}
/**
* .conductor.proto.WorkflowTask workflow_task = 27;
*/
public Builder mergeWorkflowTask(com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask value) {
if (workflowTaskBuilder_ == null) {
if (((bitField0_ & 0x04000000) != 0) &&
workflowTask_ != null &&
workflowTask_ != com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask.getDefaultInstance()) {
getWorkflowTaskBuilder().mergeFrom(value);
} else {
workflowTask_ = value;
}
} else {
workflowTaskBuilder_.mergeFrom(value);
}
bitField0_ |= 0x04000000;
onChanged();
return this;
}
/**
* .conductor.proto.WorkflowTask workflow_task = 27;
*/
public Builder clearWorkflowTask() {
bitField0_ = (bitField0_ & ~0x04000000);
workflowTask_ = null;
if (workflowTaskBuilder_ != null) {
workflowTaskBuilder_.dispose();
workflowTaskBuilder_ = null;
}
onChanged();
return this;
}
/**
* .conductor.proto.WorkflowTask workflow_task = 27;
*/
public com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask.Builder getWorkflowTaskBuilder() {
bitField0_ |= 0x04000000;
onChanged();
return getWorkflowTaskFieldBuilder().getBuilder();
}
/**
* .conductor.proto.WorkflowTask workflow_task = 27;
*/
public com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTaskOrBuilder getWorkflowTaskOrBuilder() {
if (workflowTaskBuilder_ != null) {
return workflowTaskBuilder_.getMessageOrBuilder();
} else {
return workflowTask_ == null ?
com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask.getDefaultInstance() : workflowTask_;
}
}
/**
* .conductor.proto.WorkflowTask workflow_task = 27;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask, com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask.Builder, com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTaskOrBuilder>
getWorkflowTaskFieldBuilder() {
if (workflowTaskBuilder_ == null) {
workflowTaskBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask, com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTask.Builder, com.netflix.conductor.proto.WorkflowTaskPb.WorkflowTaskOrBuilder>(
getWorkflowTask(),
getParentForChildren(),
isClean());
workflowTask_ = null;
}
return workflowTaskBuilder_;
}
private java.lang.Object domain_ = "";
/**
* string domain = 28;
* @return The domain.
*/
public java.lang.String getDomain() {
java.lang.Object ref = domain_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
domain_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string domain = 28;
* @return The bytes for domain.
*/
public com.google.protobuf.ByteString
getDomainBytes() {
java.lang.Object ref = domain_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
domain_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string domain = 28;
* @param value The domain to set.
* @return This builder for chaining.
*/
public Builder setDomain(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
domain_ = value;
bitField0_ |= 0x08000000;
onChanged();
return this;
}
/**
* string domain = 28;
* @return This builder for chaining.
*/
public Builder clearDomain() {
domain_ = getDefaultInstance().getDomain();
bitField0_ = (bitField0_ & ~0x08000000);
onChanged();
return this;
}
/**
* string domain = 28;
* @param value The bytes for domain to set.
* @return This builder for chaining.
*/
public Builder setDomainBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
domain_ = value;
bitField0_ |= 0x08000000;
onChanged();
return this;
}
private com.google.protobuf.Any inputMessage_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> inputMessageBuilder_;
/**
* .google.protobuf.Any input_message = 29;
* @return Whether the inputMessage field is set.
*/
public boolean hasInputMessage() {
return ((bitField0_ & 0x10000000) != 0);
}
/**
* .google.protobuf.Any input_message = 29;
* @return The inputMessage.
*/
public com.google.protobuf.Any getInputMessage() {
if (inputMessageBuilder_ == null) {
return inputMessage_ == null ? com.google.protobuf.Any.getDefaultInstance() : inputMessage_;
} else {
return inputMessageBuilder_.getMessage();
}
}
/**
* .google.protobuf.Any input_message = 29;
*/
public Builder setInputMessage(com.google.protobuf.Any value) {
if (inputMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
inputMessage_ = value;
} else {
inputMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x10000000;
onChanged();
return this;
}
/**
* .google.protobuf.Any input_message = 29;
*/
public Builder setInputMessage(
com.google.protobuf.Any.Builder builderForValue) {
if (inputMessageBuilder_ == null) {
inputMessage_ = builderForValue.build();
} else {
inputMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x10000000;
onChanged();
return this;
}
/**
* .google.protobuf.Any input_message = 29;
*/
public Builder mergeInputMessage(com.google.protobuf.Any value) {
if (inputMessageBuilder_ == null) {
if (((bitField0_ & 0x10000000) != 0) &&
inputMessage_ != null &&
inputMessage_ != com.google.protobuf.Any.getDefaultInstance()) {
getInputMessageBuilder().mergeFrom(value);
} else {
inputMessage_ = value;
}
} else {
inputMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x10000000;
onChanged();
return this;
}
/**
* .google.protobuf.Any input_message = 29;
*/
public Builder clearInputMessage() {
bitField0_ = (bitField0_ & ~0x10000000);
inputMessage_ = null;
if (inputMessageBuilder_ != null) {
inputMessageBuilder_.dispose();
inputMessageBuilder_ = null;
}
onChanged();
return this;
}
/**
* .google.protobuf.Any input_message = 29;
*/
public com.google.protobuf.Any.Builder getInputMessageBuilder() {
bitField0_ |= 0x10000000;
onChanged();
return getInputMessageFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Any input_message = 29;
*/
public com.google.protobuf.AnyOrBuilder getInputMessageOrBuilder() {
if (inputMessageBuilder_ != null) {
return inputMessageBuilder_.getMessageOrBuilder();
} else {
return inputMessage_ == null ?
com.google.protobuf.Any.getDefaultInstance() : inputMessage_;
}
}
/**
* .google.protobuf.Any input_message = 29;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getInputMessageFieldBuilder() {
if (inputMessageBuilder_ == null) {
inputMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
getInputMessage(),
getParentForChildren(),
isClean());
inputMessage_ = null;
}
return inputMessageBuilder_;
}
private com.google.protobuf.Any outputMessage_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> outputMessageBuilder_;
/**
* .google.protobuf.Any output_message = 30;
* @return Whether the outputMessage field is set.
*/
public boolean hasOutputMessage() {
return ((bitField0_ & 0x20000000) != 0);
}
/**
* .google.protobuf.Any output_message = 30;
* @return The outputMessage.
*/
public com.google.protobuf.Any getOutputMessage() {
if (outputMessageBuilder_ == null) {
return outputMessage_ == null ? com.google.protobuf.Any.getDefaultInstance() : outputMessage_;
} else {
return outputMessageBuilder_.getMessage();
}
}
/**
* .google.protobuf.Any output_message = 30;
*/
public Builder setOutputMessage(com.google.protobuf.Any value) {
if (outputMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
outputMessage_ = value;
} else {
outputMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x20000000;
onChanged();
return this;
}
/**
* .google.protobuf.Any output_message = 30;
*/
public Builder setOutputMessage(
com.google.protobuf.Any.Builder builderForValue) {
if (outputMessageBuilder_ == null) {
outputMessage_ = builderForValue.build();
} else {
outputMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x20000000;
onChanged();
return this;
}
/**
* .google.protobuf.Any output_message = 30;
*/
public Builder mergeOutputMessage(com.google.protobuf.Any value) {
if (outputMessageBuilder_ == null) {
if (((bitField0_ & 0x20000000) != 0) &&
outputMessage_ != null &&
outputMessage_ != com.google.protobuf.Any.getDefaultInstance()) {
getOutputMessageBuilder().mergeFrom(value);
} else {
outputMessage_ = value;
}
} else {
outputMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x20000000;
onChanged();
return this;
}
/**
* .google.protobuf.Any output_message = 30;
*/
public Builder clearOutputMessage() {
bitField0_ = (bitField0_ & ~0x20000000);
outputMessage_ = null;
if (outputMessageBuilder_ != null) {
outputMessageBuilder_.dispose();
outputMessageBuilder_ = null;
}
onChanged();
return this;
}
/**
* .google.protobuf.Any output_message = 30;
*/
public com.google.protobuf.Any.Builder getOutputMessageBuilder() {
bitField0_ |= 0x20000000;
onChanged();
return getOutputMessageFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Any output_message = 30;
*/
public com.google.protobuf.AnyOrBuilder getOutputMessageOrBuilder() {
if (outputMessageBuilder_ != null) {
return outputMessageBuilder_.getMessageOrBuilder();
} else {
return outputMessage_ == null ?
com.google.protobuf.Any.getDefaultInstance() : outputMessage_;
}
}
/**
* .google.protobuf.Any output_message = 30;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getOutputMessageFieldBuilder() {
if (outputMessageBuilder_ == null) {
outputMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
getOutputMessage(),
getParentForChildren(),
isClean());
outputMessage_ = null;
}
return outputMessageBuilder_;
}
private int rateLimitPerFrequency_ ;
/**
* int32 rate_limit_per_frequency = 32;
* @return The rateLimitPerFrequency.
*/
@java.lang.Override
public int getRateLimitPerFrequency() {
return rateLimitPerFrequency_;
}
/**
* int32 rate_limit_per_frequency = 32;
* @param value The rateLimitPerFrequency to set.
* @return This builder for chaining.
*/
public Builder setRateLimitPerFrequency(int value) {
rateLimitPerFrequency_ = value;
bitField0_ |= 0x40000000;
onChanged();
return this;
}
/**
* int32 rate_limit_per_frequency = 32;
* @return This builder for chaining.
*/
public Builder clearRateLimitPerFrequency() {
bitField0_ = (bitField0_ & ~0x40000000);
rateLimitPerFrequency_ = 0;
onChanged();
return this;
}
private int rateLimitFrequencyInSeconds_ ;
/**
* int32 rate_limit_frequency_in_seconds = 33;
* @return The rateLimitFrequencyInSeconds.
*/
@java.lang.Override
public int getRateLimitFrequencyInSeconds() {
return rateLimitFrequencyInSeconds_;
}
/**
* int32 rate_limit_frequency_in_seconds = 33;
* @param value The rateLimitFrequencyInSeconds to set.
* @return This builder for chaining.
*/
public Builder setRateLimitFrequencyInSeconds(int value) {
rateLimitFrequencyInSeconds_ = value;
bitField0_ |= 0x80000000;
onChanged();
return this;
}
/**
* int32 rate_limit_frequency_in_seconds = 33;
* @return This builder for chaining.
*/
public Builder clearRateLimitFrequencyInSeconds() {
bitField0_ = (bitField0_ & ~0x80000000);
rateLimitFrequencyInSeconds_ = 0;
onChanged();
return this;
}
private java.lang.Object externalInputPayloadStoragePath_ = "";
/**
* string external_input_payload_storage_path = 34;
* @return The externalInputPayloadStoragePath.
*/
public java.lang.String getExternalInputPayloadStoragePath() {
java.lang.Object ref = externalInputPayloadStoragePath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
externalInputPayloadStoragePath_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string external_input_payload_storage_path = 34;
* @return The bytes for externalInputPayloadStoragePath.
*/
public com.google.protobuf.ByteString
getExternalInputPayloadStoragePathBytes() {
java.lang.Object ref = externalInputPayloadStoragePath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
externalInputPayloadStoragePath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string external_input_payload_storage_path = 34;
* @param value The externalInputPayloadStoragePath to set.
* @return This builder for chaining.
*/
public Builder setExternalInputPayloadStoragePath(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
externalInputPayloadStoragePath_ = value;
bitField1_ |= 0x00000001;
onChanged();
return this;
}
/**
* string external_input_payload_storage_path = 34;
* @return This builder for chaining.
*/
public Builder clearExternalInputPayloadStoragePath() {
externalInputPayloadStoragePath_ = getDefaultInstance().getExternalInputPayloadStoragePath();
bitField1_ = (bitField1_ & ~0x00000001);
onChanged();
return this;
}
/**
* string external_input_payload_storage_path = 34;
* @param value The bytes for externalInputPayloadStoragePath to set.
* @return This builder for chaining.
*/
public Builder setExternalInputPayloadStoragePathBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
externalInputPayloadStoragePath_ = value;
bitField1_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object externalOutputPayloadStoragePath_ = "";
/**
* string external_output_payload_storage_path = 35;
* @return The externalOutputPayloadStoragePath.
*/
public java.lang.String getExternalOutputPayloadStoragePath() {
java.lang.Object ref = externalOutputPayloadStoragePath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
externalOutputPayloadStoragePath_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string external_output_payload_storage_path = 35;
* @return The bytes for externalOutputPayloadStoragePath.
*/
public com.google.protobuf.ByteString
getExternalOutputPayloadStoragePathBytes() {
java.lang.Object ref = externalOutputPayloadStoragePath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
externalOutputPayloadStoragePath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string external_output_payload_storage_path = 35;
* @param value The externalOutputPayloadStoragePath to set.
* @return This builder for chaining.
*/
public Builder setExternalOutputPayloadStoragePath(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
externalOutputPayloadStoragePath_ = value;
bitField1_ |= 0x00000002;
onChanged();
return this;
}
/**
* string external_output_payload_storage_path = 35;
* @return This builder for chaining.
*/
public Builder clearExternalOutputPayloadStoragePath() {
externalOutputPayloadStoragePath_ = getDefaultInstance().getExternalOutputPayloadStoragePath();
bitField1_ = (bitField1_ & ~0x00000002);
onChanged();
return this;
}
/**
* string external_output_payload_storage_path = 35;
* @param value The bytes for externalOutputPayloadStoragePath to set.
* @return This builder for chaining.
*/
public Builder setExternalOutputPayloadStoragePathBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
externalOutputPayloadStoragePath_ = value;
bitField1_ |= 0x00000002;
onChanged();
return this;
}
private int workflowPriority_ ;
/**
* int32 workflow_priority = 36;
* @return The workflowPriority.
*/
@java.lang.Override
public int getWorkflowPriority() {
return workflowPriority_;
}
/**
* int32 workflow_priority = 36;
* @param value The workflowPriority to set.
* @return This builder for chaining.
*/
public Builder setWorkflowPriority(int value) {
workflowPriority_ = value;
bitField1_ |= 0x00000004;
onChanged();
return this;
}
/**
* int32 workflow_priority = 36;
* @return This builder for chaining.
*/
public Builder clearWorkflowPriority() {
bitField1_ = (bitField1_ & ~0x00000004);
workflowPriority_ = 0;
onChanged();
return this;
}
private java.lang.Object executionNameSpace_ = "";
/**
* string execution_name_space = 37;
* @return The executionNameSpace.
*/
public java.lang.String getExecutionNameSpace() {
java.lang.Object ref = executionNameSpace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
executionNameSpace_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string execution_name_space = 37;
* @return The bytes for executionNameSpace.
*/
public com.google.protobuf.ByteString
getExecutionNameSpaceBytes() {
java.lang.Object ref = executionNameSpace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
executionNameSpace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string execution_name_space = 37;
* @param value The executionNameSpace to set.
* @return This builder for chaining.
*/
public Builder setExecutionNameSpace(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
executionNameSpace_ = value;
bitField1_ |= 0x00000008;
onChanged();
return this;
}
/**
* string execution_name_space = 37;
* @return This builder for chaining.
*/
public Builder clearExecutionNameSpace() {
executionNameSpace_ = getDefaultInstance().getExecutionNameSpace();
bitField1_ = (bitField1_ & ~0x00000008);
onChanged();
return this;
}
/**
* string execution_name_space = 37;
* @param value The bytes for executionNameSpace to set.
* @return This builder for chaining.
*/
public Builder setExecutionNameSpaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
executionNameSpace_ = value;
bitField1_ |= 0x00000008;
onChanged();
return this;
}
private java.lang.Object isolationGroupId_ = "";
/**
* string isolation_group_id = 38;
* @return The isolationGroupId.
*/
public java.lang.String getIsolationGroupId() {
java.lang.Object ref = isolationGroupId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
isolationGroupId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string isolation_group_id = 38;
* @return The bytes for isolationGroupId.
*/
public com.google.protobuf.ByteString
getIsolationGroupIdBytes() {
java.lang.Object ref = isolationGroupId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
isolationGroupId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string isolation_group_id = 38;
* @param value The isolationGroupId to set.
* @return This builder for chaining.
*/
public Builder setIsolationGroupId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
isolationGroupId_ = value;
bitField1_ |= 0x00000010;
onChanged();
return this;
}
/**
* string isolation_group_id = 38;
* @return This builder for chaining.
*/
public Builder clearIsolationGroupId() {
isolationGroupId_ = getDefaultInstance().getIsolationGroupId();
bitField1_ = (bitField1_ & ~0x00000010);
onChanged();
return this;
}
/**
* string isolation_group_id = 38;
* @param value The bytes for isolationGroupId to set.
* @return This builder for chaining.
*/
public Builder setIsolationGroupIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
isolationGroupId_ = value;
bitField1_ |= 0x00000010;
onChanged();
return this;
}
private int iteration_ ;
/**
* int32 iteration = 40;
* @return The iteration.
*/
@java.lang.Override
public int getIteration() {
return iteration_;
}
/**
* int32 iteration = 40;
* @param value The iteration to set.
* @return This builder for chaining.
*/
public Builder setIteration(int value) {
iteration_ = value;
bitField1_ |= 0x00000020;
onChanged();
return this;
}
/**
* int32 iteration = 40;
* @return This builder for chaining.
*/
public Builder clearIteration() {
bitField1_ = (bitField1_ & ~0x00000020);
iteration_ = 0;
onChanged();
return this;
}
private java.lang.Object subWorkflowId_ = "";
/**
* string sub_workflow_id = 41;
* @return The subWorkflowId.
*/
public java.lang.String getSubWorkflowId() {
java.lang.Object ref = subWorkflowId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subWorkflowId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sub_workflow_id = 41;
* @return The bytes for subWorkflowId.
*/
public com.google.protobuf.ByteString
getSubWorkflowIdBytes() {
java.lang.Object ref = subWorkflowId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
subWorkflowId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sub_workflow_id = 41;
* @param value The subWorkflowId to set.
* @return This builder for chaining.
*/
public Builder setSubWorkflowId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
subWorkflowId_ = value;
bitField1_ |= 0x00000040;
onChanged();
return this;
}
/**
* string sub_workflow_id = 41;
* @return This builder for chaining.
*/
public Builder clearSubWorkflowId() {
subWorkflowId_ = getDefaultInstance().getSubWorkflowId();
bitField1_ = (bitField1_ & ~0x00000040);
onChanged();
return this;
}
/**
* string sub_workflow_id = 41;
* @param value The bytes for subWorkflowId to set.
* @return This builder for chaining.
*/
public Builder setSubWorkflowIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
subWorkflowId_ = value;
bitField1_ |= 0x00000040;
onChanged();
return this;
}
private boolean subworkflowChanged_ ;
/**
* bool subworkflow_changed = 42;
* @return The subworkflowChanged.
*/
@java.lang.Override
public boolean getSubworkflowChanged() {
return subworkflowChanged_;
}
/**
* bool subworkflow_changed = 42;
* @param value The subworkflowChanged to set.
* @return This builder for chaining.
*/
public Builder setSubworkflowChanged(boolean value) {
subworkflowChanged_ = value;
bitField1_ |= 0x00000080;
onChanged();
return this;
}
/**
* bool subworkflow_changed = 42;
* @return This builder for chaining.
*/
public Builder clearSubworkflowChanged() {
bitField1_ = (bitField1_ & ~0x00000080);
subworkflowChanged_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:conductor.proto.Task)
}
// @@protoc_insertion_point(class_scope:conductor.proto.Task)
private static final com.netflix.conductor.proto.TaskPb.Task DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.netflix.conductor.proto.TaskPb.Task();
}
public static com.netflix.conductor.proto.TaskPb.Task getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Task parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.netflix.conductor.proto.TaskPb.Task getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_conductor_proto_Task_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_conductor_proto_Task_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_conductor_proto_Task_InputDataEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_conductor_proto_Task_InputDataEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_conductor_proto_Task_OutputDataEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_conductor_proto_Task_OutputDataEntry_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\020model/task.proto\022\017conductor.proto\032\030mod" +
"el/workflowtask.proto\032\034google/protobuf/s" +
"truct.proto\032\031google/protobuf/any.proto\"\355" +
"\013\n\004Task\022\021\n\ttask_type\030\001 \001(\t\022,\n\006status\030\002 \001" +
"(\0162\034.conductor.proto.Task.Status\0228\n\ninpu" +
"t_data\030\003 \003(\0132$.conductor.proto.Task.Inpu" +
"tDataEntry\022\033\n\023reference_task_name\030\004 \001(\t\022" +
"\023\n\013retry_count\030\005 \001(\005\022\013\n\003seq\030\006 \001(\005\022\026\n\016cor" +
"relation_id\030\007 \001(\t\022\022\n\npoll_count\030\010 \001(\005\022\025\n" +
"\rtask_def_name\030\t \001(\t\022\026\n\016scheduled_time\030\n" +
" \001(\003\022\022\n\nstart_time\030\013 \001(\003\022\020\n\010end_time\030\014 \001" +
"(\003\022\023\n\013update_time\030\r \001(\003\022\036\n\026start_delay_i" +
"n_seconds\030\016 \001(\005\022\027\n\017retried_task_id\030\017 \001(\t" +
"\022\017\n\007retried\030\020 \001(\010\022\020\n\010executed\030\021 \001(\010\022\034\n\024c" +
"allback_from_worker\030\022 \001(\010\022 \n\030response_ti" +
"meout_seconds\030\023 \001(\003\022\034\n\024workflow_instance" +
"_id\030\024 \001(\t\022\025\n\rworkflow_type\030\025 \001(\t\022\017\n\007task" +
"_id\030\026 \001(\t\022\037\n\027reason_for_incompletion\030\027 \001" +
"(\t\022\036\n\026callback_after_seconds\030\030 \001(\003\022\021\n\two" +
"rker_id\030\031 \001(\t\022:\n\013output_data\030\032 \003(\0132%.con" +
"ductor.proto.Task.OutputDataEntry\0224\n\rwor" +
"kflow_task\030\033 \001(\0132\035.conductor.proto.Workf" +
"lowTask\022\016\n\006domain\030\034 \001(\t\022+\n\rinput_message" +
"\030\035 \001(\0132\024.google.protobuf.Any\022,\n\016output_m" +
"essage\030\036 \001(\0132\024.google.protobuf.Any\022 \n\030ra" +
"te_limit_per_frequency\030 \001(\005\022\'\n\037rate_lim" +
"it_frequency_in_seconds\030! \001(\005\022+\n#externa" +
"l_input_payload_storage_path\030\" \001(\t\022,\n$ex" +
"ternal_output_payload_storage_path\030# \001(\t" +
"\022\031\n\021workflow_priority\030$ \001(\005\022\034\n\024execution" +
"_name_space\030% \001(\t\022\032\n\022isolation_group_id\030" +
"& \001(\t\022\021\n\titeration\030( \001(\005\022\027\n\017sub_workflow" +
"_id\030) \001(\t\022\033\n\023subworkflow_changed\030* \001(\010\032H" +
"\n\016InputDataEntry\022\013\n\003key\030\001 \001(\t\022%\n\005value\030\002" +
" \001(\0132\026.google.protobuf.Value:\0028\001\032I\n\017Outp" +
"utDataEntry\022\013\n\003key\030\001 \001(\t\022%\n\005value\030\002 \001(\0132" +
"\026.google.protobuf.Value:\0028\001\"\250\001\n\006Status\022\017" +
"\n\013IN_PROGRESS\020\000\022\014\n\010CANCELED\020\001\022\n\n\006FAILED\020" +
"\002\022\036\n\032FAILED_WITH_TERMINAL_ERROR\020\003\022\r\n\tCOM" +
"PLETED\020\004\022\031\n\025COMPLETED_WITH_ERRORS\020\005\022\r\n\tS" +
"CHEDULED\020\006\022\r\n\tTIMED_OUT\020\007\022\013\n\007SKIPPED\020\010Ba" +
"\n\033com.netflix.conductor.protoB\006TaskPbZ:g" +
"ithub.com/netflix/conductor/client/gogrp" +
"c/conductor/modelb\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.netflix.conductor.proto.WorkflowTaskPb.getDescriptor(),
com.google.protobuf.StructProto.getDescriptor(),
com.google.protobuf.AnyProto.getDescriptor(),
});
internal_static_conductor_proto_Task_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_conductor_proto_Task_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_conductor_proto_Task_descriptor,
new java.lang.String[] { "TaskType", "Status", "InputData", "ReferenceTaskName", "RetryCount", "Seq", "CorrelationId", "PollCount", "TaskDefName", "ScheduledTime", "StartTime", "EndTime", "UpdateTime", "StartDelayInSeconds", "RetriedTaskId", "Retried", "Executed", "CallbackFromWorker", "ResponseTimeoutSeconds", "WorkflowInstanceId", "WorkflowType", "TaskId", "ReasonForIncompletion", "CallbackAfterSeconds", "WorkerId", "OutputData", "WorkflowTask", "Domain", "InputMessage", "OutputMessage", "RateLimitPerFrequency", "RateLimitFrequencyInSeconds", "ExternalInputPayloadStoragePath", "ExternalOutputPayloadStoragePath", "WorkflowPriority", "ExecutionNameSpace", "IsolationGroupId", "Iteration", "SubWorkflowId", "SubworkflowChanged", });
internal_static_conductor_proto_Task_InputDataEntry_descriptor =
internal_static_conductor_proto_Task_descriptor.getNestedTypes().get(0);
internal_static_conductor_proto_Task_InputDataEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_conductor_proto_Task_InputDataEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_conductor_proto_Task_OutputDataEntry_descriptor =
internal_static_conductor_proto_Task_descriptor.getNestedTypes().get(1);
internal_static_conductor_proto_Task_OutputDataEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_conductor_proto_Task_OutputDataEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
com.netflix.conductor.proto.WorkflowTaskPb.getDescriptor();
com.google.protobuf.StructProto.getDescriptor();
com.google.protobuf.AnyProto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy