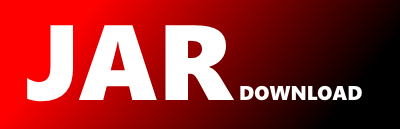
com.netflix.conductor.proto.EventHandlerPb Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: model/eventhandler.proto
package com.netflix.conductor.proto;
public final class EventHandlerPb {
private EventHandlerPb() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface EventHandlerOrBuilder extends
// @@protoc_insertion_point(interface_extends:conductor.proto.EventHandler)
com.google.protobuf.MessageOrBuilder {
/**
* string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* string event = 2;
* @return The event.
*/
java.lang.String getEvent();
/**
* string event = 2;
* @return The bytes for event.
*/
com.google.protobuf.ByteString
getEventBytes();
/**
* string condition = 3;
* @return The condition.
*/
java.lang.String getCondition();
/**
* string condition = 3;
* @return The bytes for condition.
*/
com.google.protobuf.ByteString
getConditionBytes();
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
java.util.List
getActionsList();
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action getActions(int index);
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
int getActionsCount();
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
java.util.List extends com.netflix.conductor.proto.EventHandlerPb.EventHandler.ActionOrBuilder>
getActionsOrBuilderList();
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
com.netflix.conductor.proto.EventHandlerPb.EventHandler.ActionOrBuilder getActionsOrBuilder(
int index);
/**
* bool active = 5;
* @return The active.
*/
boolean getActive();
/**
* string evaluator_type = 6;
* @return The evaluatorType.
*/
java.lang.String getEvaluatorType();
/**
* string evaluator_type = 6;
* @return The bytes for evaluatorType.
*/
com.google.protobuf.ByteString
getEvaluatorTypeBytes();
}
/**
* Protobuf type {@code conductor.proto.EventHandler}
*/
public static final class EventHandler extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:conductor.proto.EventHandler)
EventHandlerOrBuilder {
private static final long serialVersionUID = 0L;
// Use EventHandler.newBuilder() to construct.
private EventHandler(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EventHandler() {
name_ = "";
event_ = "";
condition_ = "";
actions_ = java.util.Collections.emptyList();
evaluatorType_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new EventHandler();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.class, com.netflix.conductor.proto.EventHandlerPb.EventHandler.Builder.class);
}
public interface UpdateWorkflowVariablesOrBuilder extends
// @@protoc_insertion_point(interface_extends:conductor.proto.EventHandler.UpdateWorkflowVariables)
com.google.protobuf.MessageOrBuilder {
/**
* string workflow_id = 1;
* @return The workflowId.
*/
java.lang.String getWorkflowId();
/**
* string workflow_id = 1;
* @return The bytes for workflowId.
*/
com.google.protobuf.ByteString
getWorkflowIdBytes();
/**
* map<string, .google.protobuf.Value> variables = 2;
*/
int getVariablesCount();
/**
* map<string, .google.protobuf.Value> variables = 2;
*/
boolean containsVariables(
java.lang.String key);
/**
* Use {@link #getVariablesMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getVariables();
/**
* map<string, .google.protobuf.Value> variables = 2;
*/
java.util.Map
getVariablesMap();
/**
* map<string, .google.protobuf.Value> variables = 2;
*/
/* nullable */
com.google.protobuf.Value getVariablesOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Value defaultValue);
/**
* map<string, .google.protobuf.Value> variables = 2;
*/
com.google.protobuf.Value getVariablesOrThrow(
java.lang.String key);
/**
* bool append_array = 3;
* @return The appendArray.
*/
boolean getAppendArray();
}
/**
* Protobuf type {@code conductor.proto.EventHandler.UpdateWorkflowVariables}
*/
public static final class UpdateWorkflowVariables extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:conductor.proto.EventHandler.UpdateWorkflowVariables)
UpdateWorkflowVariablesOrBuilder {
private static final long serialVersionUID = 0L;
// Use UpdateWorkflowVariables.newBuilder() to construct.
private UpdateWorkflowVariables(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UpdateWorkflowVariables() {
workflowId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new UpdateWorkflowVariables();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_UpdateWorkflowVariables_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetVariables();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_UpdateWorkflowVariables_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables.class, com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables.Builder.class);
}
public static final int WORKFLOW_ID_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object workflowId_ = "";
/**
* string workflow_id = 1;
* @return The workflowId.
*/
@java.lang.Override
public java.lang.String getWorkflowId() {
java.lang.Object ref = workflowId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workflowId_ = s;
return s;
}
}
/**
* string workflow_id = 1;
* @return The bytes for workflowId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkflowIdBytes() {
java.lang.Object ref = workflowId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workflowId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VARIABLES_FIELD_NUMBER = 2;
private static final class VariablesDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.google.protobuf.Value> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_UpdateWorkflowVariables_VariablesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.protobuf.Value.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, com.google.protobuf.Value> variables_;
private com.google.protobuf.MapField
internalGetVariables() {
if (variables_ == null) {
return com.google.protobuf.MapField.emptyMapField(
VariablesDefaultEntryHolder.defaultEntry);
}
return variables_;
}
public int getVariablesCount() {
return internalGetVariables().getMap().size();
}
/**
* map<string, .google.protobuf.Value> variables = 2;
*/
@java.lang.Override
public boolean containsVariables(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetVariables().getMap().containsKey(key);
}
/**
* Use {@link #getVariablesMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getVariables() {
return getVariablesMap();
}
/**
* map<string, .google.protobuf.Value> variables = 2;
*/
@java.lang.Override
public java.util.Map getVariablesMap() {
return internalGetVariables().getMap();
}
/**
* map<string, .google.protobuf.Value> variables = 2;
*/
@java.lang.Override
public /* nullable */
com.google.protobuf.Value getVariablesOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Value defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetVariables().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .google.protobuf.Value> variables = 2;
*/
@java.lang.Override
public com.google.protobuf.Value getVariablesOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetVariables().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int APPEND_ARRAY_FIELD_NUMBER = 3;
private boolean appendArray_ = false;
/**
* bool append_array = 3;
* @return The appendArray.
*/
@java.lang.Override
public boolean getAppendArray() {
return appendArray_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workflowId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, workflowId_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetVariables(),
VariablesDefaultEntryHolder.defaultEntry,
2);
if (appendArray_ != false) {
output.writeBool(3, appendArray_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workflowId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, workflowId_);
}
for (java.util.Map.Entry entry
: internalGetVariables().getMap().entrySet()) {
com.google.protobuf.MapEntry
variables__ = VariablesDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, variables__);
}
if (appendArray_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, appendArray_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables)) {
return super.equals(obj);
}
com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables other = (com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables) obj;
if (!getWorkflowId()
.equals(other.getWorkflowId())) return false;
if (!internalGetVariables().equals(
other.internalGetVariables())) return false;
if (getAppendArray()
!= other.getAppendArray()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + WORKFLOW_ID_FIELD_NUMBER;
hash = (53 * hash) + getWorkflowId().hashCode();
if (!internalGetVariables().getMap().isEmpty()) {
hash = (37 * hash) + VARIABLES_FIELD_NUMBER;
hash = (53 * hash) + internalGetVariables().hashCode();
}
hash = (37 * hash) + APPEND_ARRAY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getAppendArray());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code conductor.proto.EventHandler.UpdateWorkflowVariables}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:conductor.proto.EventHandler.UpdateWorkflowVariables)
com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariablesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_UpdateWorkflowVariables_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetVariables();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 2:
return internalGetMutableVariables();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_UpdateWorkflowVariables_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables.class, com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables.Builder.class);
}
// Construct using com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
workflowId_ = "";
internalGetMutableVariables().clear();
appendArray_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_UpdateWorkflowVariables_descriptor;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables getDefaultInstanceForType() {
return com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables.getDefaultInstance();
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables build() {
com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables buildPartial() {
com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables result = new com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.workflowId_ = workflowId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.variables_ = internalGetVariables();
result.variables_.makeImmutable();
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.appendArray_ = appendArray_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables) {
return mergeFrom((com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables other) {
if (other == com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables.getDefaultInstance()) return this;
if (!other.getWorkflowId().isEmpty()) {
workflowId_ = other.workflowId_;
bitField0_ |= 0x00000001;
onChanged();
}
internalGetMutableVariables().mergeFrom(
other.internalGetVariables());
bitField0_ |= 0x00000002;
if (other.getAppendArray() != false) {
setAppendArray(other.getAppendArray());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
workflowId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
com.google.protobuf.MapEntry
variables__ = input.readMessage(
VariablesDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
internalGetMutableVariables().getMutableMap().put(
variables__.getKey(), variables__.getValue());
bitField0_ |= 0x00000002;
break;
} // case 18
case 24: {
appendArray_ = input.readBool();
bitField0_ |= 0x00000004;
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object workflowId_ = "";
/**
* string workflow_id = 1;
* @return The workflowId.
*/
public java.lang.String getWorkflowId() {
java.lang.Object ref = workflowId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workflowId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string workflow_id = 1;
* @return The bytes for workflowId.
*/
public com.google.protobuf.ByteString
getWorkflowIdBytes() {
java.lang.Object ref = workflowId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workflowId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string workflow_id = 1;
* @param value The workflowId to set.
* @return This builder for chaining.
*/
public Builder setWorkflowId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
workflowId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string workflow_id = 1;
* @return This builder for chaining.
*/
public Builder clearWorkflowId() {
workflowId_ = getDefaultInstance().getWorkflowId();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string workflow_id = 1;
* @param value The bytes for workflowId to set.
* @return This builder for chaining.
*/
public Builder setWorkflowIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
workflowId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, com.google.protobuf.Value> variables_;
private com.google.protobuf.MapField
internalGetVariables() {
if (variables_ == null) {
return com.google.protobuf.MapField.emptyMapField(
VariablesDefaultEntryHolder.defaultEntry);
}
return variables_;
}
private com.google.protobuf.MapField
internalGetMutableVariables() {
if (variables_ == null) {
variables_ = com.google.protobuf.MapField.newMapField(
VariablesDefaultEntryHolder.defaultEntry);
}
if (!variables_.isMutable()) {
variables_ = variables_.copy();
}
bitField0_ |= 0x00000002;
onChanged();
return variables_;
}
public int getVariablesCount() {
return internalGetVariables().getMap().size();
}
/**
* map<string, .google.protobuf.Value> variables = 2;
*/
@java.lang.Override
public boolean containsVariables(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetVariables().getMap().containsKey(key);
}
/**
* Use {@link #getVariablesMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getVariables() {
return getVariablesMap();
}
/**
* map<string, .google.protobuf.Value> variables = 2;
*/
@java.lang.Override
public java.util.Map getVariablesMap() {
return internalGetVariables().getMap();
}
/**
* map<string, .google.protobuf.Value> variables = 2;
*/
@java.lang.Override
public /* nullable */
com.google.protobuf.Value getVariablesOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Value defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetVariables().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .google.protobuf.Value> variables = 2;
*/
@java.lang.Override
public com.google.protobuf.Value getVariablesOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetVariables().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearVariables() {
bitField0_ = (bitField0_ & ~0x00000002);
internalGetMutableVariables().getMutableMap()
.clear();
return this;
}
/**
* map<string, .google.protobuf.Value> variables = 2;
*/
public Builder removeVariables(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableVariables().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableVariables() {
bitField0_ |= 0x00000002;
return internalGetMutableVariables().getMutableMap();
}
/**
* map<string, .google.protobuf.Value> variables = 2;
*/
public Builder putVariables(
java.lang.String key,
com.google.protobuf.Value value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) { throw new NullPointerException("map value"); }
internalGetMutableVariables().getMutableMap()
.put(key, value);
bitField0_ |= 0x00000002;
return this;
}
/**
* map<string, .google.protobuf.Value> variables = 2;
*/
public Builder putAllVariables(
java.util.Map values) {
internalGetMutableVariables().getMutableMap()
.putAll(values);
bitField0_ |= 0x00000002;
return this;
}
private boolean appendArray_ ;
/**
* bool append_array = 3;
* @return The appendArray.
*/
@java.lang.Override
public boolean getAppendArray() {
return appendArray_;
}
/**
* bool append_array = 3;
* @param value The appendArray to set.
* @return This builder for chaining.
*/
public Builder setAppendArray(boolean value) {
appendArray_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* bool append_array = 3;
* @return This builder for chaining.
*/
public Builder clearAppendArray() {
bitField0_ = (bitField0_ & ~0x00000004);
appendArray_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:conductor.proto.EventHandler.UpdateWorkflowVariables)
}
// @@protoc_insertion_point(class_scope:conductor.proto.EventHandler.UpdateWorkflowVariables)
private static final com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables();
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public UpdateWorkflowVariables parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TerminateWorkflowOrBuilder extends
// @@protoc_insertion_point(interface_extends:conductor.proto.EventHandler.TerminateWorkflow)
com.google.protobuf.MessageOrBuilder {
/**
* string workflow_id = 1;
* @return The workflowId.
*/
java.lang.String getWorkflowId();
/**
* string workflow_id = 1;
* @return The bytes for workflowId.
*/
com.google.protobuf.ByteString
getWorkflowIdBytes();
/**
* string termination_reason = 2;
* @return The terminationReason.
*/
java.lang.String getTerminationReason();
/**
* string termination_reason = 2;
* @return The bytes for terminationReason.
*/
com.google.protobuf.ByteString
getTerminationReasonBytes();
}
/**
* Protobuf type {@code conductor.proto.EventHandler.TerminateWorkflow}
*/
public static final class TerminateWorkflow extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:conductor.proto.EventHandler.TerminateWorkflow)
TerminateWorkflowOrBuilder {
private static final long serialVersionUID = 0L;
// Use TerminateWorkflow.newBuilder() to construct.
private TerminateWorkflow(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TerminateWorkflow() {
workflowId_ = "";
terminationReason_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TerminateWorkflow();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_TerminateWorkflow_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_TerminateWorkflow_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow.class, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow.Builder.class);
}
public static final int WORKFLOW_ID_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object workflowId_ = "";
/**
* string workflow_id = 1;
* @return The workflowId.
*/
@java.lang.Override
public java.lang.String getWorkflowId() {
java.lang.Object ref = workflowId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workflowId_ = s;
return s;
}
}
/**
* string workflow_id = 1;
* @return The bytes for workflowId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkflowIdBytes() {
java.lang.Object ref = workflowId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workflowId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TERMINATION_REASON_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object terminationReason_ = "";
/**
* string termination_reason = 2;
* @return The terminationReason.
*/
@java.lang.Override
public java.lang.String getTerminationReason() {
java.lang.Object ref = terminationReason_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
terminationReason_ = s;
return s;
}
}
/**
* string termination_reason = 2;
* @return The bytes for terminationReason.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTerminationReasonBytes() {
java.lang.Object ref = terminationReason_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
terminationReason_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workflowId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, workflowId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(terminationReason_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, terminationReason_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workflowId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, workflowId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(terminationReason_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, terminationReason_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow)) {
return super.equals(obj);
}
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow other = (com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow) obj;
if (!getWorkflowId()
.equals(other.getWorkflowId())) return false;
if (!getTerminationReason()
.equals(other.getTerminationReason())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + WORKFLOW_ID_FIELD_NUMBER;
hash = (53 * hash) + getWorkflowId().hashCode();
hash = (37 * hash) + TERMINATION_REASON_FIELD_NUMBER;
hash = (53 * hash) + getTerminationReason().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code conductor.proto.EventHandler.TerminateWorkflow}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:conductor.proto.EventHandler.TerminateWorkflow)
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflowOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_TerminateWorkflow_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_TerminateWorkflow_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow.class, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow.Builder.class);
}
// Construct using com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
workflowId_ = "";
terminationReason_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_TerminateWorkflow_descriptor;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow getDefaultInstanceForType() {
return com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow.getDefaultInstance();
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow build() {
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow buildPartial() {
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow result = new com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.workflowId_ = workflowId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.terminationReason_ = terminationReason_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow) {
return mergeFrom((com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow other) {
if (other == com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow.getDefaultInstance()) return this;
if (!other.getWorkflowId().isEmpty()) {
workflowId_ = other.workflowId_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getTerminationReason().isEmpty()) {
terminationReason_ = other.terminationReason_;
bitField0_ |= 0x00000002;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
workflowId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
terminationReason_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object workflowId_ = "";
/**
* string workflow_id = 1;
* @return The workflowId.
*/
public java.lang.String getWorkflowId() {
java.lang.Object ref = workflowId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workflowId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string workflow_id = 1;
* @return The bytes for workflowId.
*/
public com.google.protobuf.ByteString
getWorkflowIdBytes() {
java.lang.Object ref = workflowId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workflowId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string workflow_id = 1;
* @param value The workflowId to set.
* @return This builder for chaining.
*/
public Builder setWorkflowId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
workflowId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string workflow_id = 1;
* @return This builder for chaining.
*/
public Builder clearWorkflowId() {
workflowId_ = getDefaultInstance().getWorkflowId();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string workflow_id = 1;
* @param value The bytes for workflowId to set.
* @return This builder for chaining.
*/
public Builder setWorkflowIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
workflowId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object terminationReason_ = "";
/**
* string termination_reason = 2;
* @return The terminationReason.
*/
public java.lang.String getTerminationReason() {
java.lang.Object ref = terminationReason_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
terminationReason_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string termination_reason = 2;
* @return The bytes for terminationReason.
*/
public com.google.protobuf.ByteString
getTerminationReasonBytes() {
java.lang.Object ref = terminationReason_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
terminationReason_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string termination_reason = 2;
* @param value The terminationReason to set.
* @return This builder for chaining.
*/
public Builder setTerminationReason(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
terminationReason_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string termination_reason = 2;
* @return This builder for chaining.
*/
public Builder clearTerminationReason() {
terminationReason_ = getDefaultInstance().getTerminationReason();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string termination_reason = 2;
* @param value The bytes for terminationReason to set.
* @return This builder for chaining.
*/
public Builder setTerminationReasonBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
terminationReason_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:conductor.proto.EventHandler.TerminateWorkflow)
}
// @@protoc_insertion_point(class_scope:conductor.proto.EventHandler.TerminateWorkflow)
private static final com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow();
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TerminateWorkflow parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StartWorkflowOrBuilder extends
// @@protoc_insertion_point(interface_extends:conductor.proto.EventHandler.StartWorkflow)
com.google.protobuf.MessageOrBuilder {
/**
* string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* int32 version = 2;
* @return The version.
*/
int getVersion();
/**
* string correlation_id = 3;
* @return The correlationId.
*/
java.lang.String getCorrelationId();
/**
* string correlation_id = 3;
* @return The bytes for correlationId.
*/
com.google.protobuf.ByteString
getCorrelationIdBytes();
/**
* map<string, .google.protobuf.Value> input = 4;
*/
int getInputCount();
/**
* map<string, .google.protobuf.Value> input = 4;
*/
boolean containsInput(
java.lang.String key);
/**
* Use {@link #getInputMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getInput();
/**
* map<string, .google.protobuf.Value> input = 4;
*/
java.util.Map
getInputMap();
/**
* map<string, .google.protobuf.Value> input = 4;
*/
/* nullable */
com.google.protobuf.Value getInputOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Value defaultValue);
/**
* map<string, .google.protobuf.Value> input = 4;
*/
com.google.protobuf.Value getInputOrThrow(
java.lang.String key);
/**
* .google.protobuf.Any input_message = 5;
* @return Whether the inputMessage field is set.
*/
boolean hasInputMessage();
/**
* .google.protobuf.Any input_message = 5;
* @return The inputMessage.
*/
com.google.protobuf.Any getInputMessage();
/**
* .google.protobuf.Any input_message = 5;
*/
com.google.protobuf.AnyOrBuilder getInputMessageOrBuilder();
/**
* map<string, string> task_to_domain = 6;
*/
int getTaskToDomainCount();
/**
* map<string, string> task_to_domain = 6;
*/
boolean containsTaskToDomain(
java.lang.String key);
/**
* Use {@link #getTaskToDomainMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getTaskToDomain();
/**
* map<string, string> task_to_domain = 6;
*/
java.util.Map
getTaskToDomainMap();
/**
* map<string, string> task_to_domain = 6;
*/
/* nullable */
java.lang.String getTaskToDomainOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
* map<string, string> task_to_domain = 6;
*/
java.lang.String getTaskToDomainOrThrow(
java.lang.String key);
}
/**
* Protobuf type {@code conductor.proto.EventHandler.StartWorkflow}
*/
public static final class StartWorkflow extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:conductor.proto.EventHandler.StartWorkflow)
StartWorkflowOrBuilder {
private static final long serialVersionUID = 0L;
// Use StartWorkflow.newBuilder() to construct.
private StartWorkflow(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StartWorkflow() {
name_ = "";
correlationId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new StartWorkflow();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_StartWorkflow_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 4:
return internalGetInput();
case 6:
return internalGetTaskToDomain();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_StartWorkflow_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow.class, com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
* string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VERSION_FIELD_NUMBER = 2;
private int version_ = 0;
/**
* int32 version = 2;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
public static final int CORRELATION_ID_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object correlationId_ = "";
/**
* string correlation_id = 3;
* @return The correlationId.
*/
@java.lang.Override
public java.lang.String getCorrelationId() {
java.lang.Object ref = correlationId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
correlationId_ = s;
return s;
}
}
/**
* string correlation_id = 3;
* @return The bytes for correlationId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCorrelationIdBytes() {
java.lang.Object ref = correlationId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
correlationId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INPUT_FIELD_NUMBER = 4;
private static final class InputDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.google.protobuf.Value> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_StartWorkflow_InputEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.protobuf.Value.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, com.google.protobuf.Value> input_;
private com.google.protobuf.MapField
internalGetInput() {
if (input_ == null) {
return com.google.protobuf.MapField.emptyMapField(
InputDefaultEntryHolder.defaultEntry);
}
return input_;
}
public int getInputCount() {
return internalGetInput().getMap().size();
}
/**
* map<string, .google.protobuf.Value> input = 4;
*/
@java.lang.Override
public boolean containsInput(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetInput().getMap().containsKey(key);
}
/**
* Use {@link #getInputMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getInput() {
return getInputMap();
}
/**
* map<string, .google.protobuf.Value> input = 4;
*/
@java.lang.Override
public java.util.Map getInputMap() {
return internalGetInput().getMap();
}
/**
* map<string, .google.protobuf.Value> input = 4;
*/
@java.lang.Override
public /* nullable */
com.google.protobuf.Value getInputOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Value defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetInput().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .google.protobuf.Value> input = 4;
*/
@java.lang.Override
public com.google.protobuf.Value getInputOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetInput().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int INPUT_MESSAGE_FIELD_NUMBER = 5;
private com.google.protobuf.Any inputMessage_;
/**
* .google.protobuf.Any input_message = 5;
* @return Whether the inputMessage field is set.
*/
@java.lang.Override
public boolean hasInputMessage() {
return inputMessage_ != null;
}
/**
* .google.protobuf.Any input_message = 5;
* @return The inputMessage.
*/
@java.lang.Override
public com.google.protobuf.Any getInputMessage() {
return inputMessage_ == null ? com.google.protobuf.Any.getDefaultInstance() : inputMessage_;
}
/**
* .google.protobuf.Any input_message = 5;
*/
@java.lang.Override
public com.google.protobuf.AnyOrBuilder getInputMessageOrBuilder() {
return inputMessage_ == null ? com.google.protobuf.Any.getDefaultInstance() : inputMessage_;
}
public static final int TASK_TO_DOMAIN_FIELD_NUMBER = 6;
private static final class TaskToDomainDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_StartWorkflow_TaskToDomainEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> taskToDomain_;
private com.google.protobuf.MapField
internalGetTaskToDomain() {
if (taskToDomain_ == null) {
return com.google.protobuf.MapField.emptyMapField(
TaskToDomainDefaultEntryHolder.defaultEntry);
}
return taskToDomain_;
}
public int getTaskToDomainCount() {
return internalGetTaskToDomain().getMap().size();
}
/**
* map<string, string> task_to_domain = 6;
*/
@java.lang.Override
public boolean containsTaskToDomain(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetTaskToDomain().getMap().containsKey(key);
}
/**
* Use {@link #getTaskToDomainMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getTaskToDomain() {
return getTaskToDomainMap();
}
/**
* map<string, string> task_to_domain = 6;
*/
@java.lang.Override
public java.util.Map getTaskToDomainMap() {
return internalGetTaskToDomain().getMap();
}
/**
* map<string, string> task_to_domain = 6;
*/
@java.lang.Override
public /* nullable */
java.lang.String getTaskToDomainOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetTaskToDomain().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> task_to_domain = 6;
*/
@java.lang.Override
public java.lang.String getTaskToDomainOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetTaskToDomain().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (version_ != 0) {
output.writeInt32(2, version_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(correlationId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, correlationId_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetInput(),
InputDefaultEntryHolder.defaultEntry,
4);
if (inputMessage_ != null) {
output.writeMessage(5, getInputMessage());
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetTaskToDomain(),
TaskToDomainDefaultEntryHolder.defaultEntry,
6);
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (version_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, version_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(correlationId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, correlationId_);
}
for (java.util.Map.Entry entry
: internalGetInput().getMap().entrySet()) {
com.google.protobuf.MapEntry
input__ = InputDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, input__);
}
if (inputMessage_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getInputMessage());
}
for (java.util.Map.Entry entry
: internalGetTaskToDomain().getMap().entrySet()) {
com.google.protobuf.MapEntry
taskToDomain__ = TaskToDomainDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, taskToDomain__);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow)) {
return super.equals(obj);
}
com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow other = (com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow) obj;
if (!getName()
.equals(other.getName())) return false;
if (getVersion()
!= other.getVersion()) return false;
if (!getCorrelationId()
.equals(other.getCorrelationId())) return false;
if (!internalGetInput().equals(
other.internalGetInput())) return false;
if (hasInputMessage() != other.hasInputMessage()) return false;
if (hasInputMessage()) {
if (!getInputMessage()
.equals(other.getInputMessage())) return false;
}
if (!internalGetTaskToDomain().equals(
other.internalGetTaskToDomain())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion();
hash = (37 * hash) + CORRELATION_ID_FIELD_NUMBER;
hash = (53 * hash) + getCorrelationId().hashCode();
if (!internalGetInput().getMap().isEmpty()) {
hash = (37 * hash) + INPUT_FIELD_NUMBER;
hash = (53 * hash) + internalGetInput().hashCode();
}
if (hasInputMessage()) {
hash = (37 * hash) + INPUT_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getInputMessage().hashCode();
}
if (!internalGetTaskToDomain().getMap().isEmpty()) {
hash = (37 * hash) + TASK_TO_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + internalGetTaskToDomain().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code conductor.proto.EventHandler.StartWorkflow}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:conductor.proto.EventHandler.StartWorkflow)
com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflowOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_StartWorkflow_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 4:
return internalGetInput();
case 6:
return internalGetTaskToDomain();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 4:
return internalGetMutableInput();
case 6:
return internalGetMutableTaskToDomain();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_StartWorkflow_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow.class, com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow.Builder.class);
}
// Construct using com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
name_ = "";
version_ = 0;
correlationId_ = "";
internalGetMutableInput().clear();
inputMessage_ = null;
if (inputMessageBuilder_ != null) {
inputMessageBuilder_.dispose();
inputMessageBuilder_ = null;
}
internalGetMutableTaskToDomain().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_StartWorkflow_descriptor;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow getDefaultInstanceForType() {
return com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow.getDefaultInstance();
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow build() {
com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow buildPartial() {
com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow result = new com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.name_ = name_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.version_ = version_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.correlationId_ = correlationId_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.input_ = internalGetInput();
result.input_.makeImmutable();
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.inputMessage_ = inputMessageBuilder_ == null
? inputMessage_
: inputMessageBuilder_.build();
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.taskToDomain_ = internalGetTaskToDomain();
result.taskToDomain_.makeImmutable();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow) {
return mergeFrom((com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow other) {
if (other == com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.getVersion() != 0) {
setVersion(other.getVersion());
}
if (!other.getCorrelationId().isEmpty()) {
correlationId_ = other.correlationId_;
bitField0_ |= 0x00000004;
onChanged();
}
internalGetMutableInput().mergeFrom(
other.internalGetInput());
bitField0_ |= 0x00000008;
if (other.hasInputMessage()) {
mergeInputMessage(other.getInputMessage());
}
internalGetMutableTaskToDomain().mergeFrom(
other.internalGetTaskToDomain());
bitField0_ |= 0x00000020;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
version_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26: {
correlationId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
com.google.protobuf.MapEntry
input__ = input.readMessage(
InputDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
internalGetMutableInput().getMutableMap().put(
input__.getKey(), input__.getValue());
bitField0_ |= 0x00000008;
break;
} // case 34
case 42: {
input.readMessage(
getInputMessageFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 42
case 50: {
com.google.protobuf.MapEntry
taskToDomain__ = input.readMessage(
TaskToDomainDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
internalGetMutableTaskToDomain().getMutableMap().put(
taskToDomain__.getKey(), taskToDomain__.getValue());
bitField0_ |= 0x00000020;
break;
} // case 50
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
* string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private int version_ ;
/**
* int32 version = 2;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
/**
* int32 version = 2;
* @param value The version to set.
* @return This builder for chaining.
*/
public Builder setVersion(int value) {
version_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 version = 2;
* @return This builder for chaining.
*/
public Builder clearVersion() {
bitField0_ = (bitField0_ & ~0x00000002);
version_ = 0;
onChanged();
return this;
}
private java.lang.Object correlationId_ = "";
/**
* string correlation_id = 3;
* @return The correlationId.
*/
public java.lang.String getCorrelationId() {
java.lang.Object ref = correlationId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
correlationId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string correlation_id = 3;
* @return The bytes for correlationId.
*/
public com.google.protobuf.ByteString
getCorrelationIdBytes() {
java.lang.Object ref = correlationId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
correlationId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string correlation_id = 3;
* @param value The correlationId to set.
* @return This builder for chaining.
*/
public Builder setCorrelationId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
correlationId_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* string correlation_id = 3;
* @return This builder for chaining.
*/
public Builder clearCorrelationId() {
correlationId_ = getDefaultInstance().getCorrelationId();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
* string correlation_id = 3;
* @param value The bytes for correlationId to set.
* @return This builder for chaining.
*/
public Builder setCorrelationIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
correlationId_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, com.google.protobuf.Value> input_;
private com.google.protobuf.MapField
internalGetInput() {
if (input_ == null) {
return com.google.protobuf.MapField.emptyMapField(
InputDefaultEntryHolder.defaultEntry);
}
return input_;
}
private com.google.protobuf.MapField
internalGetMutableInput() {
if (input_ == null) {
input_ = com.google.protobuf.MapField.newMapField(
InputDefaultEntryHolder.defaultEntry);
}
if (!input_.isMutable()) {
input_ = input_.copy();
}
bitField0_ |= 0x00000008;
onChanged();
return input_;
}
public int getInputCount() {
return internalGetInput().getMap().size();
}
/**
* map<string, .google.protobuf.Value> input = 4;
*/
@java.lang.Override
public boolean containsInput(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetInput().getMap().containsKey(key);
}
/**
* Use {@link #getInputMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getInput() {
return getInputMap();
}
/**
* map<string, .google.protobuf.Value> input = 4;
*/
@java.lang.Override
public java.util.Map getInputMap() {
return internalGetInput().getMap();
}
/**
* map<string, .google.protobuf.Value> input = 4;
*/
@java.lang.Override
public /* nullable */
com.google.protobuf.Value getInputOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Value defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetInput().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .google.protobuf.Value> input = 4;
*/
@java.lang.Override
public com.google.protobuf.Value getInputOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetInput().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearInput() {
bitField0_ = (bitField0_ & ~0x00000008);
internalGetMutableInput().getMutableMap()
.clear();
return this;
}
/**
* map<string, .google.protobuf.Value> input = 4;
*/
public Builder removeInput(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableInput().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableInput() {
bitField0_ |= 0x00000008;
return internalGetMutableInput().getMutableMap();
}
/**
* map<string, .google.protobuf.Value> input = 4;
*/
public Builder putInput(
java.lang.String key,
com.google.protobuf.Value value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) { throw new NullPointerException("map value"); }
internalGetMutableInput().getMutableMap()
.put(key, value);
bitField0_ |= 0x00000008;
return this;
}
/**
* map<string, .google.protobuf.Value> input = 4;
*/
public Builder putAllInput(
java.util.Map values) {
internalGetMutableInput().getMutableMap()
.putAll(values);
bitField0_ |= 0x00000008;
return this;
}
private com.google.protobuf.Any inputMessage_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> inputMessageBuilder_;
/**
* .google.protobuf.Any input_message = 5;
* @return Whether the inputMessage field is set.
*/
public boolean hasInputMessage() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* .google.protobuf.Any input_message = 5;
* @return The inputMessage.
*/
public com.google.protobuf.Any getInputMessage() {
if (inputMessageBuilder_ == null) {
return inputMessage_ == null ? com.google.protobuf.Any.getDefaultInstance() : inputMessage_;
} else {
return inputMessageBuilder_.getMessage();
}
}
/**
* .google.protobuf.Any input_message = 5;
*/
public Builder setInputMessage(com.google.protobuf.Any value) {
if (inputMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
inputMessage_ = value;
} else {
inputMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* .google.protobuf.Any input_message = 5;
*/
public Builder setInputMessage(
com.google.protobuf.Any.Builder builderForValue) {
if (inputMessageBuilder_ == null) {
inputMessage_ = builderForValue.build();
} else {
inputMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* .google.protobuf.Any input_message = 5;
*/
public Builder mergeInputMessage(com.google.protobuf.Any value) {
if (inputMessageBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0) &&
inputMessage_ != null &&
inputMessage_ != com.google.protobuf.Any.getDefaultInstance()) {
getInputMessageBuilder().mergeFrom(value);
} else {
inputMessage_ = value;
}
} else {
inputMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* .google.protobuf.Any input_message = 5;
*/
public Builder clearInputMessage() {
bitField0_ = (bitField0_ & ~0x00000010);
inputMessage_ = null;
if (inputMessageBuilder_ != null) {
inputMessageBuilder_.dispose();
inputMessageBuilder_ = null;
}
onChanged();
return this;
}
/**
* .google.protobuf.Any input_message = 5;
*/
public com.google.protobuf.Any.Builder getInputMessageBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getInputMessageFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Any input_message = 5;
*/
public com.google.protobuf.AnyOrBuilder getInputMessageOrBuilder() {
if (inputMessageBuilder_ != null) {
return inputMessageBuilder_.getMessageOrBuilder();
} else {
return inputMessage_ == null ?
com.google.protobuf.Any.getDefaultInstance() : inputMessage_;
}
}
/**
* .google.protobuf.Any input_message = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getInputMessageFieldBuilder() {
if (inputMessageBuilder_ == null) {
inputMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
getInputMessage(),
getParentForChildren(),
isClean());
inputMessage_ = null;
}
return inputMessageBuilder_;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> taskToDomain_;
private com.google.protobuf.MapField
internalGetTaskToDomain() {
if (taskToDomain_ == null) {
return com.google.protobuf.MapField.emptyMapField(
TaskToDomainDefaultEntryHolder.defaultEntry);
}
return taskToDomain_;
}
private com.google.protobuf.MapField
internalGetMutableTaskToDomain() {
if (taskToDomain_ == null) {
taskToDomain_ = com.google.protobuf.MapField.newMapField(
TaskToDomainDefaultEntryHolder.defaultEntry);
}
if (!taskToDomain_.isMutable()) {
taskToDomain_ = taskToDomain_.copy();
}
bitField0_ |= 0x00000020;
onChanged();
return taskToDomain_;
}
public int getTaskToDomainCount() {
return internalGetTaskToDomain().getMap().size();
}
/**
* map<string, string> task_to_domain = 6;
*/
@java.lang.Override
public boolean containsTaskToDomain(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetTaskToDomain().getMap().containsKey(key);
}
/**
* Use {@link #getTaskToDomainMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getTaskToDomain() {
return getTaskToDomainMap();
}
/**
* map<string, string> task_to_domain = 6;
*/
@java.lang.Override
public java.util.Map getTaskToDomainMap() {
return internalGetTaskToDomain().getMap();
}
/**
* map<string, string> task_to_domain = 6;
*/
@java.lang.Override
public /* nullable */
java.lang.String getTaskToDomainOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetTaskToDomain().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> task_to_domain = 6;
*/
@java.lang.Override
public java.lang.String getTaskToDomainOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetTaskToDomain().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearTaskToDomain() {
bitField0_ = (bitField0_ & ~0x00000020);
internalGetMutableTaskToDomain().getMutableMap()
.clear();
return this;
}
/**
* map<string, string> task_to_domain = 6;
*/
public Builder removeTaskToDomain(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableTaskToDomain().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableTaskToDomain() {
bitField0_ |= 0x00000020;
return internalGetMutableTaskToDomain().getMutableMap();
}
/**
* map<string, string> task_to_domain = 6;
*/
public Builder putTaskToDomain(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) { throw new NullPointerException("map value"); }
internalGetMutableTaskToDomain().getMutableMap()
.put(key, value);
bitField0_ |= 0x00000020;
return this;
}
/**
* map<string, string> task_to_domain = 6;
*/
public Builder putAllTaskToDomain(
java.util.Map values) {
internalGetMutableTaskToDomain().getMutableMap()
.putAll(values);
bitField0_ |= 0x00000020;
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:conductor.proto.EventHandler.StartWorkflow)
}
// @@protoc_insertion_point(class_scope:conductor.proto.EventHandler.StartWorkflow)
private static final com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow();
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StartWorkflow parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TaskDetailsOrBuilder extends
// @@protoc_insertion_point(interface_extends:conductor.proto.EventHandler.TaskDetails)
com.google.protobuf.MessageOrBuilder {
/**
* string workflow_id = 1;
* @return The workflowId.
*/
java.lang.String getWorkflowId();
/**
* string workflow_id = 1;
* @return The bytes for workflowId.
*/
com.google.protobuf.ByteString
getWorkflowIdBytes();
/**
* string task_ref_name = 2;
* @return The taskRefName.
*/
java.lang.String getTaskRefName();
/**
* string task_ref_name = 2;
* @return The bytes for taskRefName.
*/
com.google.protobuf.ByteString
getTaskRefNameBytes();
/**
* map<string, .google.protobuf.Value> output = 3;
*/
int getOutputCount();
/**
* map<string, .google.protobuf.Value> output = 3;
*/
boolean containsOutput(
java.lang.String key);
/**
* Use {@link #getOutputMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getOutput();
/**
* map<string, .google.protobuf.Value> output = 3;
*/
java.util.Map
getOutputMap();
/**
* map<string, .google.protobuf.Value> output = 3;
*/
/* nullable */
com.google.protobuf.Value getOutputOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Value defaultValue);
/**
* map<string, .google.protobuf.Value> output = 3;
*/
com.google.protobuf.Value getOutputOrThrow(
java.lang.String key);
/**
* .google.protobuf.Any output_message = 4;
* @return Whether the outputMessage field is set.
*/
boolean hasOutputMessage();
/**
* .google.protobuf.Any output_message = 4;
* @return The outputMessage.
*/
com.google.protobuf.Any getOutputMessage();
/**
* .google.protobuf.Any output_message = 4;
*/
com.google.protobuf.AnyOrBuilder getOutputMessageOrBuilder();
/**
* string task_id = 5;
* @return The taskId.
*/
java.lang.String getTaskId();
/**
* string task_id = 5;
* @return The bytes for taskId.
*/
com.google.protobuf.ByteString
getTaskIdBytes();
}
/**
* Protobuf type {@code conductor.proto.EventHandler.TaskDetails}
*/
public static final class TaskDetails extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:conductor.proto.EventHandler.TaskDetails)
TaskDetailsOrBuilder {
private static final long serialVersionUID = 0L;
// Use TaskDetails.newBuilder() to construct.
private TaskDetails(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TaskDetails() {
workflowId_ = "";
taskRefName_ = "";
taskId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TaskDetails();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_TaskDetails_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 3:
return internalGetOutput();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_TaskDetails_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.class, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.Builder.class);
}
public static final int WORKFLOW_ID_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object workflowId_ = "";
/**
* string workflow_id = 1;
* @return The workflowId.
*/
@java.lang.Override
public java.lang.String getWorkflowId() {
java.lang.Object ref = workflowId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workflowId_ = s;
return s;
}
}
/**
* string workflow_id = 1;
* @return The bytes for workflowId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkflowIdBytes() {
java.lang.Object ref = workflowId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workflowId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TASK_REF_NAME_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object taskRefName_ = "";
/**
* string task_ref_name = 2;
* @return The taskRefName.
*/
@java.lang.Override
public java.lang.String getTaskRefName() {
java.lang.Object ref = taskRefName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
taskRefName_ = s;
return s;
}
}
/**
* string task_ref_name = 2;
* @return The bytes for taskRefName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTaskRefNameBytes() {
java.lang.Object ref = taskRefName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
taskRefName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OUTPUT_FIELD_NUMBER = 3;
private static final class OutputDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.google.protobuf.Value> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_TaskDetails_OutputEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.protobuf.Value.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, com.google.protobuf.Value> output_;
private com.google.protobuf.MapField
internalGetOutput() {
if (output_ == null) {
return com.google.protobuf.MapField.emptyMapField(
OutputDefaultEntryHolder.defaultEntry);
}
return output_;
}
public int getOutputCount() {
return internalGetOutput().getMap().size();
}
/**
* map<string, .google.protobuf.Value> output = 3;
*/
@java.lang.Override
public boolean containsOutput(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetOutput().getMap().containsKey(key);
}
/**
* Use {@link #getOutputMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getOutput() {
return getOutputMap();
}
/**
* map<string, .google.protobuf.Value> output = 3;
*/
@java.lang.Override
public java.util.Map getOutputMap() {
return internalGetOutput().getMap();
}
/**
* map<string, .google.protobuf.Value> output = 3;
*/
@java.lang.Override
public /* nullable */
com.google.protobuf.Value getOutputOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Value defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetOutput().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .google.protobuf.Value> output = 3;
*/
@java.lang.Override
public com.google.protobuf.Value getOutputOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetOutput().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int OUTPUT_MESSAGE_FIELD_NUMBER = 4;
private com.google.protobuf.Any outputMessage_;
/**
* .google.protobuf.Any output_message = 4;
* @return Whether the outputMessage field is set.
*/
@java.lang.Override
public boolean hasOutputMessage() {
return outputMessage_ != null;
}
/**
* .google.protobuf.Any output_message = 4;
* @return The outputMessage.
*/
@java.lang.Override
public com.google.protobuf.Any getOutputMessage() {
return outputMessage_ == null ? com.google.protobuf.Any.getDefaultInstance() : outputMessage_;
}
/**
* .google.protobuf.Any output_message = 4;
*/
@java.lang.Override
public com.google.protobuf.AnyOrBuilder getOutputMessageOrBuilder() {
return outputMessage_ == null ? com.google.protobuf.Any.getDefaultInstance() : outputMessage_;
}
public static final int TASK_ID_FIELD_NUMBER = 5;
@SuppressWarnings("serial")
private volatile java.lang.Object taskId_ = "";
/**
* string task_id = 5;
* @return The taskId.
*/
@java.lang.Override
public java.lang.String getTaskId() {
java.lang.Object ref = taskId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
taskId_ = s;
return s;
}
}
/**
* string task_id = 5;
* @return The bytes for taskId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTaskIdBytes() {
java.lang.Object ref = taskId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
taskId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workflowId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, workflowId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(taskRefName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, taskRefName_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetOutput(),
OutputDefaultEntryHolder.defaultEntry,
3);
if (outputMessage_ != null) {
output.writeMessage(4, getOutputMessage());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(taskId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, taskId_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workflowId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, workflowId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(taskRefName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, taskRefName_);
}
for (java.util.Map.Entry entry
: internalGetOutput().getMap().entrySet()) {
com.google.protobuf.MapEntry
output__ = OutputDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, output__);
}
if (outputMessage_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getOutputMessage());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(taskId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, taskId_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails)) {
return super.equals(obj);
}
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails other = (com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails) obj;
if (!getWorkflowId()
.equals(other.getWorkflowId())) return false;
if (!getTaskRefName()
.equals(other.getTaskRefName())) return false;
if (!internalGetOutput().equals(
other.internalGetOutput())) return false;
if (hasOutputMessage() != other.hasOutputMessage()) return false;
if (hasOutputMessage()) {
if (!getOutputMessage()
.equals(other.getOutputMessage())) return false;
}
if (!getTaskId()
.equals(other.getTaskId())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + WORKFLOW_ID_FIELD_NUMBER;
hash = (53 * hash) + getWorkflowId().hashCode();
hash = (37 * hash) + TASK_REF_NAME_FIELD_NUMBER;
hash = (53 * hash) + getTaskRefName().hashCode();
if (!internalGetOutput().getMap().isEmpty()) {
hash = (37 * hash) + OUTPUT_FIELD_NUMBER;
hash = (53 * hash) + internalGetOutput().hashCode();
}
if (hasOutputMessage()) {
hash = (37 * hash) + OUTPUT_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getOutputMessage().hashCode();
}
hash = (37 * hash) + TASK_ID_FIELD_NUMBER;
hash = (53 * hash) + getTaskId().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code conductor.proto.EventHandler.TaskDetails}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:conductor.proto.EventHandler.TaskDetails)
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetailsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_TaskDetails_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 3:
return internalGetOutput();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 3:
return internalGetMutableOutput();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_TaskDetails_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.class, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.Builder.class);
}
// Construct using com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
workflowId_ = "";
taskRefName_ = "";
internalGetMutableOutput().clear();
outputMessage_ = null;
if (outputMessageBuilder_ != null) {
outputMessageBuilder_.dispose();
outputMessageBuilder_ = null;
}
taskId_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_TaskDetails_descriptor;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails getDefaultInstanceForType() {
return com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.getDefaultInstance();
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails build() {
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails buildPartial() {
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails result = new com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.workflowId_ = workflowId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.taskRefName_ = taskRefName_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.output_ = internalGetOutput();
result.output_.makeImmutable();
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.outputMessage_ = outputMessageBuilder_ == null
? outputMessage_
: outputMessageBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.taskId_ = taskId_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails) {
return mergeFrom((com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails other) {
if (other == com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.getDefaultInstance()) return this;
if (!other.getWorkflowId().isEmpty()) {
workflowId_ = other.workflowId_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getTaskRefName().isEmpty()) {
taskRefName_ = other.taskRefName_;
bitField0_ |= 0x00000002;
onChanged();
}
internalGetMutableOutput().mergeFrom(
other.internalGetOutput());
bitField0_ |= 0x00000004;
if (other.hasOutputMessage()) {
mergeOutputMessage(other.getOutputMessage());
}
if (!other.getTaskId().isEmpty()) {
taskId_ = other.taskId_;
bitField0_ |= 0x00000010;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
workflowId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
taskRefName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
com.google.protobuf.MapEntry
output__ = input.readMessage(
OutputDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
internalGetMutableOutput().getMutableMap().put(
output__.getKey(), output__.getValue());
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
input.readMessage(
getOutputMessageFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
case 42: {
taskId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 42
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object workflowId_ = "";
/**
* string workflow_id = 1;
* @return The workflowId.
*/
public java.lang.String getWorkflowId() {
java.lang.Object ref = workflowId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workflowId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string workflow_id = 1;
* @return The bytes for workflowId.
*/
public com.google.protobuf.ByteString
getWorkflowIdBytes() {
java.lang.Object ref = workflowId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workflowId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string workflow_id = 1;
* @param value The workflowId to set.
* @return This builder for chaining.
*/
public Builder setWorkflowId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
workflowId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string workflow_id = 1;
* @return This builder for chaining.
*/
public Builder clearWorkflowId() {
workflowId_ = getDefaultInstance().getWorkflowId();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string workflow_id = 1;
* @param value The bytes for workflowId to set.
* @return This builder for chaining.
*/
public Builder setWorkflowIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
workflowId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object taskRefName_ = "";
/**
* string task_ref_name = 2;
* @return The taskRefName.
*/
public java.lang.String getTaskRefName() {
java.lang.Object ref = taskRefName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
taskRefName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string task_ref_name = 2;
* @return The bytes for taskRefName.
*/
public com.google.protobuf.ByteString
getTaskRefNameBytes() {
java.lang.Object ref = taskRefName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
taskRefName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string task_ref_name = 2;
* @param value The taskRefName to set.
* @return This builder for chaining.
*/
public Builder setTaskRefName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
taskRefName_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string task_ref_name = 2;
* @return This builder for chaining.
*/
public Builder clearTaskRefName() {
taskRefName_ = getDefaultInstance().getTaskRefName();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string task_ref_name = 2;
* @param value The bytes for taskRefName to set.
* @return This builder for chaining.
*/
public Builder setTaskRefNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
taskRefName_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, com.google.protobuf.Value> output_;
private com.google.protobuf.MapField
internalGetOutput() {
if (output_ == null) {
return com.google.protobuf.MapField.emptyMapField(
OutputDefaultEntryHolder.defaultEntry);
}
return output_;
}
private com.google.protobuf.MapField
internalGetMutableOutput() {
if (output_ == null) {
output_ = com.google.protobuf.MapField.newMapField(
OutputDefaultEntryHolder.defaultEntry);
}
if (!output_.isMutable()) {
output_ = output_.copy();
}
bitField0_ |= 0x00000004;
onChanged();
return output_;
}
public int getOutputCount() {
return internalGetOutput().getMap().size();
}
/**
* map<string, .google.protobuf.Value> output = 3;
*/
@java.lang.Override
public boolean containsOutput(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetOutput().getMap().containsKey(key);
}
/**
* Use {@link #getOutputMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getOutput() {
return getOutputMap();
}
/**
* map<string, .google.protobuf.Value> output = 3;
*/
@java.lang.Override
public java.util.Map getOutputMap() {
return internalGetOutput().getMap();
}
/**
* map<string, .google.protobuf.Value> output = 3;
*/
@java.lang.Override
public /* nullable */
com.google.protobuf.Value getOutputOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Value defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetOutput().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .google.protobuf.Value> output = 3;
*/
@java.lang.Override
public com.google.protobuf.Value getOutputOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetOutput().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearOutput() {
bitField0_ = (bitField0_ & ~0x00000004);
internalGetMutableOutput().getMutableMap()
.clear();
return this;
}
/**
* map<string, .google.protobuf.Value> output = 3;
*/
public Builder removeOutput(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableOutput().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableOutput() {
bitField0_ |= 0x00000004;
return internalGetMutableOutput().getMutableMap();
}
/**
* map<string, .google.protobuf.Value> output = 3;
*/
public Builder putOutput(
java.lang.String key,
com.google.protobuf.Value value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) { throw new NullPointerException("map value"); }
internalGetMutableOutput().getMutableMap()
.put(key, value);
bitField0_ |= 0x00000004;
return this;
}
/**
* map<string, .google.protobuf.Value> output = 3;
*/
public Builder putAllOutput(
java.util.Map values) {
internalGetMutableOutput().getMutableMap()
.putAll(values);
bitField0_ |= 0x00000004;
return this;
}
private com.google.protobuf.Any outputMessage_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> outputMessageBuilder_;
/**
* .google.protobuf.Any output_message = 4;
* @return Whether the outputMessage field is set.
*/
public boolean hasOutputMessage() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* .google.protobuf.Any output_message = 4;
* @return The outputMessage.
*/
public com.google.protobuf.Any getOutputMessage() {
if (outputMessageBuilder_ == null) {
return outputMessage_ == null ? com.google.protobuf.Any.getDefaultInstance() : outputMessage_;
} else {
return outputMessageBuilder_.getMessage();
}
}
/**
* .google.protobuf.Any output_message = 4;
*/
public Builder setOutputMessage(com.google.protobuf.Any value) {
if (outputMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
outputMessage_ = value;
} else {
outputMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* .google.protobuf.Any output_message = 4;
*/
public Builder setOutputMessage(
com.google.protobuf.Any.Builder builderForValue) {
if (outputMessageBuilder_ == null) {
outputMessage_ = builderForValue.build();
} else {
outputMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* .google.protobuf.Any output_message = 4;
*/
public Builder mergeOutputMessage(com.google.protobuf.Any value) {
if (outputMessageBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
outputMessage_ != null &&
outputMessage_ != com.google.protobuf.Any.getDefaultInstance()) {
getOutputMessageBuilder().mergeFrom(value);
} else {
outputMessage_ = value;
}
} else {
outputMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* .google.protobuf.Any output_message = 4;
*/
public Builder clearOutputMessage() {
bitField0_ = (bitField0_ & ~0x00000008);
outputMessage_ = null;
if (outputMessageBuilder_ != null) {
outputMessageBuilder_.dispose();
outputMessageBuilder_ = null;
}
onChanged();
return this;
}
/**
* .google.protobuf.Any output_message = 4;
*/
public com.google.protobuf.Any.Builder getOutputMessageBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getOutputMessageFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Any output_message = 4;
*/
public com.google.protobuf.AnyOrBuilder getOutputMessageOrBuilder() {
if (outputMessageBuilder_ != null) {
return outputMessageBuilder_.getMessageOrBuilder();
} else {
return outputMessage_ == null ?
com.google.protobuf.Any.getDefaultInstance() : outputMessage_;
}
}
/**
* .google.protobuf.Any output_message = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getOutputMessageFieldBuilder() {
if (outputMessageBuilder_ == null) {
outputMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
getOutputMessage(),
getParentForChildren(),
isClean());
outputMessage_ = null;
}
return outputMessageBuilder_;
}
private java.lang.Object taskId_ = "";
/**
* string task_id = 5;
* @return The taskId.
*/
public java.lang.String getTaskId() {
java.lang.Object ref = taskId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
taskId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string task_id = 5;
* @return The bytes for taskId.
*/
public com.google.protobuf.ByteString
getTaskIdBytes() {
java.lang.Object ref = taskId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
taskId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string task_id = 5;
* @param value The taskId to set.
* @return This builder for chaining.
*/
public Builder setTaskId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
taskId_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* string task_id = 5;
* @return This builder for chaining.
*/
public Builder clearTaskId() {
taskId_ = getDefaultInstance().getTaskId();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
* string task_id = 5;
* @param value The bytes for taskId to set.
* @return This builder for chaining.
*/
public Builder setTaskIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
taskId_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:conductor.proto.EventHandler.TaskDetails)
}
// @@protoc_insertion_point(class_scope:conductor.proto.EventHandler.TaskDetails)
private static final com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails();
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TaskDetails parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ActionOrBuilder extends
// @@protoc_insertion_point(interface_extends:conductor.proto.EventHandler.Action)
com.google.protobuf.MessageOrBuilder {
/**
* .conductor.proto.EventHandler.Action.Type action = 1;
* @return The enum numeric value on the wire for action.
*/
int getActionValue();
/**
* .conductor.proto.EventHandler.Action.Type action = 1;
* @return The action.
*/
com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Type getAction();
/**
* .conductor.proto.EventHandler.StartWorkflow start_workflow = 2;
* @return Whether the startWorkflow field is set.
*/
boolean hasStartWorkflow();
/**
* .conductor.proto.EventHandler.StartWorkflow start_workflow = 2;
* @return The startWorkflow.
*/
com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow getStartWorkflow();
/**
* .conductor.proto.EventHandler.StartWorkflow start_workflow = 2;
*/
com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflowOrBuilder getStartWorkflowOrBuilder();
/**
* .conductor.proto.EventHandler.TaskDetails complete_task = 3;
* @return Whether the completeTask field is set.
*/
boolean hasCompleteTask();
/**
* .conductor.proto.EventHandler.TaskDetails complete_task = 3;
* @return The completeTask.
*/
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails getCompleteTask();
/**
* .conductor.proto.EventHandler.TaskDetails complete_task = 3;
*/
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetailsOrBuilder getCompleteTaskOrBuilder();
/**
* .conductor.proto.EventHandler.TaskDetails fail_task = 4;
* @return Whether the failTask field is set.
*/
boolean hasFailTask();
/**
* .conductor.proto.EventHandler.TaskDetails fail_task = 4;
* @return The failTask.
*/
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails getFailTask();
/**
* .conductor.proto.EventHandler.TaskDetails fail_task = 4;
*/
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetailsOrBuilder getFailTaskOrBuilder();
/**
* bool expand_inline_json = 5;
* @return The expandInlineJson.
*/
boolean getExpandInlineJson();
/**
* .conductor.proto.EventHandler.TerminateWorkflow terminate_workflow = 6;
* @return Whether the terminateWorkflow field is set.
*/
boolean hasTerminateWorkflow();
/**
* .conductor.proto.EventHandler.TerminateWorkflow terminate_workflow = 6;
* @return The terminateWorkflow.
*/
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow getTerminateWorkflow();
/**
* .conductor.proto.EventHandler.TerminateWorkflow terminate_workflow = 6;
*/
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflowOrBuilder getTerminateWorkflowOrBuilder();
/**
* .conductor.proto.EventHandler.UpdateWorkflowVariables update_workflow_variables = 7;
* @return Whether the updateWorkflowVariables field is set.
*/
boolean hasUpdateWorkflowVariables();
/**
* .conductor.proto.EventHandler.UpdateWorkflowVariables update_workflow_variables = 7;
* @return The updateWorkflowVariables.
*/
com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables getUpdateWorkflowVariables();
/**
* .conductor.proto.EventHandler.UpdateWorkflowVariables update_workflow_variables = 7;
*/
com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariablesOrBuilder getUpdateWorkflowVariablesOrBuilder();
}
/**
* Protobuf type {@code conductor.proto.EventHandler.Action}
*/
public static final class Action extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:conductor.proto.EventHandler.Action)
ActionOrBuilder {
private static final long serialVersionUID = 0L;
// Use Action.newBuilder() to construct.
private Action(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Action() {
action_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Action();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_Action_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_Action_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.class, com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Builder.class);
}
/**
* Protobuf enum {@code conductor.proto.EventHandler.Action.Type}
*/
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
/**
* START_WORKFLOW = 0;
*/
START_WORKFLOW(0),
/**
* COMPLETE_TASK = 1;
*/
COMPLETE_TASK(1),
/**
* FAIL_TASK = 2;
*/
FAIL_TASK(2),
/**
* TERMINATE_WORKFLOW = 3;
*/
TERMINATE_WORKFLOW(3),
/**
* UPDATE_WORKFLOW_VARIABLES = 4;
*/
UPDATE_WORKFLOW_VARIABLES(4),
UNRECOGNIZED(-1),
;
/**
* START_WORKFLOW = 0;
*/
public static final int START_WORKFLOW_VALUE = 0;
/**
* COMPLETE_TASK = 1;
*/
public static final int COMPLETE_TASK_VALUE = 1;
/**
* FAIL_TASK = 2;
*/
public static final int FAIL_TASK_VALUE = 2;
/**
* TERMINATE_WORKFLOW = 3;
*/
public static final int TERMINATE_WORKFLOW_VALUE = 3;
/**
* UPDATE_WORKFLOW_VARIABLES = 4;
*/
public static final int UPDATE_WORKFLOW_VARIABLES_VALUE = 4;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Type valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Type forNumber(int value) {
switch (value) {
case 0: return START_WORKFLOW;
case 1: return COMPLETE_TASK;
case 2: return FAIL_TASK;
case 3: return TERMINATE_WORKFLOW;
case 4: return UPDATE_WORKFLOW_VARIABLES;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Type> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = values();
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Type(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:conductor.proto.EventHandler.Action.Type)
}
public static final int ACTION_FIELD_NUMBER = 1;
private int action_ = 0;
/**
* .conductor.proto.EventHandler.Action.Type action = 1;
* @return The enum numeric value on the wire for action.
*/
@java.lang.Override public int getActionValue() {
return action_;
}
/**
* .conductor.proto.EventHandler.Action.Type action = 1;
* @return The action.
*/
@java.lang.Override public com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Type getAction() {
com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Type result = com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Type.forNumber(action_);
return result == null ? com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Type.UNRECOGNIZED : result;
}
public static final int START_WORKFLOW_FIELD_NUMBER = 2;
private com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow startWorkflow_;
/**
* .conductor.proto.EventHandler.StartWorkflow start_workflow = 2;
* @return Whether the startWorkflow field is set.
*/
@java.lang.Override
public boolean hasStartWorkflow() {
return startWorkflow_ != null;
}
/**
* .conductor.proto.EventHandler.StartWorkflow start_workflow = 2;
* @return The startWorkflow.
*/
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow getStartWorkflow() {
return startWorkflow_ == null ? com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow.getDefaultInstance() : startWorkflow_;
}
/**
* .conductor.proto.EventHandler.StartWorkflow start_workflow = 2;
*/
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflowOrBuilder getStartWorkflowOrBuilder() {
return startWorkflow_ == null ? com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow.getDefaultInstance() : startWorkflow_;
}
public static final int COMPLETE_TASK_FIELD_NUMBER = 3;
private com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails completeTask_;
/**
* .conductor.proto.EventHandler.TaskDetails complete_task = 3;
* @return Whether the completeTask field is set.
*/
@java.lang.Override
public boolean hasCompleteTask() {
return completeTask_ != null;
}
/**
* .conductor.proto.EventHandler.TaskDetails complete_task = 3;
* @return The completeTask.
*/
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails getCompleteTask() {
return completeTask_ == null ? com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.getDefaultInstance() : completeTask_;
}
/**
* .conductor.proto.EventHandler.TaskDetails complete_task = 3;
*/
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetailsOrBuilder getCompleteTaskOrBuilder() {
return completeTask_ == null ? com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.getDefaultInstance() : completeTask_;
}
public static final int FAIL_TASK_FIELD_NUMBER = 4;
private com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails failTask_;
/**
* .conductor.proto.EventHandler.TaskDetails fail_task = 4;
* @return Whether the failTask field is set.
*/
@java.lang.Override
public boolean hasFailTask() {
return failTask_ != null;
}
/**
* .conductor.proto.EventHandler.TaskDetails fail_task = 4;
* @return The failTask.
*/
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails getFailTask() {
return failTask_ == null ? com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.getDefaultInstance() : failTask_;
}
/**
* .conductor.proto.EventHandler.TaskDetails fail_task = 4;
*/
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetailsOrBuilder getFailTaskOrBuilder() {
return failTask_ == null ? com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.getDefaultInstance() : failTask_;
}
public static final int EXPAND_INLINE_JSON_FIELD_NUMBER = 5;
private boolean expandInlineJson_ = false;
/**
* bool expand_inline_json = 5;
* @return The expandInlineJson.
*/
@java.lang.Override
public boolean getExpandInlineJson() {
return expandInlineJson_;
}
public static final int TERMINATE_WORKFLOW_FIELD_NUMBER = 6;
private com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow terminateWorkflow_;
/**
* .conductor.proto.EventHandler.TerminateWorkflow terminate_workflow = 6;
* @return Whether the terminateWorkflow field is set.
*/
@java.lang.Override
public boolean hasTerminateWorkflow() {
return terminateWorkflow_ != null;
}
/**
* .conductor.proto.EventHandler.TerminateWorkflow terminate_workflow = 6;
* @return The terminateWorkflow.
*/
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow getTerminateWorkflow() {
return terminateWorkflow_ == null ? com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow.getDefaultInstance() : terminateWorkflow_;
}
/**
* .conductor.proto.EventHandler.TerminateWorkflow terminate_workflow = 6;
*/
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflowOrBuilder getTerminateWorkflowOrBuilder() {
return terminateWorkflow_ == null ? com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow.getDefaultInstance() : terminateWorkflow_;
}
public static final int UPDATE_WORKFLOW_VARIABLES_FIELD_NUMBER = 7;
private com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables updateWorkflowVariables_;
/**
* .conductor.proto.EventHandler.UpdateWorkflowVariables update_workflow_variables = 7;
* @return Whether the updateWorkflowVariables field is set.
*/
@java.lang.Override
public boolean hasUpdateWorkflowVariables() {
return updateWorkflowVariables_ != null;
}
/**
* .conductor.proto.EventHandler.UpdateWorkflowVariables update_workflow_variables = 7;
* @return The updateWorkflowVariables.
*/
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables getUpdateWorkflowVariables() {
return updateWorkflowVariables_ == null ? com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables.getDefaultInstance() : updateWorkflowVariables_;
}
/**
* .conductor.proto.EventHandler.UpdateWorkflowVariables update_workflow_variables = 7;
*/
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariablesOrBuilder getUpdateWorkflowVariablesOrBuilder() {
return updateWorkflowVariables_ == null ? com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables.getDefaultInstance() : updateWorkflowVariables_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (action_ != com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Type.START_WORKFLOW.getNumber()) {
output.writeEnum(1, action_);
}
if (startWorkflow_ != null) {
output.writeMessage(2, getStartWorkflow());
}
if (completeTask_ != null) {
output.writeMessage(3, getCompleteTask());
}
if (failTask_ != null) {
output.writeMessage(4, getFailTask());
}
if (expandInlineJson_ != false) {
output.writeBool(5, expandInlineJson_);
}
if (terminateWorkflow_ != null) {
output.writeMessage(6, getTerminateWorkflow());
}
if (updateWorkflowVariables_ != null) {
output.writeMessage(7, getUpdateWorkflowVariables());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (action_ != com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Type.START_WORKFLOW.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, action_);
}
if (startWorkflow_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getStartWorkflow());
}
if (completeTask_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getCompleteTask());
}
if (failTask_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getFailTask());
}
if (expandInlineJson_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, expandInlineJson_);
}
if (terminateWorkflow_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getTerminateWorkflow());
}
if (updateWorkflowVariables_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getUpdateWorkflowVariables());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action)) {
return super.equals(obj);
}
com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action other = (com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action) obj;
if (action_ != other.action_) return false;
if (hasStartWorkflow() != other.hasStartWorkflow()) return false;
if (hasStartWorkflow()) {
if (!getStartWorkflow()
.equals(other.getStartWorkflow())) return false;
}
if (hasCompleteTask() != other.hasCompleteTask()) return false;
if (hasCompleteTask()) {
if (!getCompleteTask()
.equals(other.getCompleteTask())) return false;
}
if (hasFailTask() != other.hasFailTask()) return false;
if (hasFailTask()) {
if (!getFailTask()
.equals(other.getFailTask())) return false;
}
if (getExpandInlineJson()
!= other.getExpandInlineJson()) return false;
if (hasTerminateWorkflow() != other.hasTerminateWorkflow()) return false;
if (hasTerminateWorkflow()) {
if (!getTerminateWorkflow()
.equals(other.getTerminateWorkflow())) return false;
}
if (hasUpdateWorkflowVariables() != other.hasUpdateWorkflowVariables()) return false;
if (hasUpdateWorkflowVariables()) {
if (!getUpdateWorkflowVariables()
.equals(other.getUpdateWorkflowVariables())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ACTION_FIELD_NUMBER;
hash = (53 * hash) + action_;
if (hasStartWorkflow()) {
hash = (37 * hash) + START_WORKFLOW_FIELD_NUMBER;
hash = (53 * hash) + getStartWorkflow().hashCode();
}
if (hasCompleteTask()) {
hash = (37 * hash) + COMPLETE_TASK_FIELD_NUMBER;
hash = (53 * hash) + getCompleteTask().hashCode();
}
if (hasFailTask()) {
hash = (37 * hash) + FAIL_TASK_FIELD_NUMBER;
hash = (53 * hash) + getFailTask().hashCode();
}
hash = (37 * hash) + EXPAND_INLINE_JSON_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getExpandInlineJson());
if (hasTerminateWorkflow()) {
hash = (37 * hash) + TERMINATE_WORKFLOW_FIELD_NUMBER;
hash = (53 * hash) + getTerminateWorkflow().hashCode();
}
if (hasUpdateWorkflowVariables()) {
hash = (37 * hash) + UPDATE_WORKFLOW_VARIABLES_FIELD_NUMBER;
hash = (53 * hash) + getUpdateWorkflowVariables().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code conductor.proto.EventHandler.Action}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:conductor.proto.EventHandler.Action)
com.netflix.conductor.proto.EventHandlerPb.EventHandler.ActionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_Action_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_Action_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.class, com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Builder.class);
}
// Construct using com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
action_ = 0;
startWorkflow_ = null;
if (startWorkflowBuilder_ != null) {
startWorkflowBuilder_.dispose();
startWorkflowBuilder_ = null;
}
completeTask_ = null;
if (completeTaskBuilder_ != null) {
completeTaskBuilder_.dispose();
completeTaskBuilder_ = null;
}
failTask_ = null;
if (failTaskBuilder_ != null) {
failTaskBuilder_.dispose();
failTaskBuilder_ = null;
}
expandInlineJson_ = false;
terminateWorkflow_ = null;
if (terminateWorkflowBuilder_ != null) {
terminateWorkflowBuilder_.dispose();
terminateWorkflowBuilder_ = null;
}
updateWorkflowVariables_ = null;
if (updateWorkflowVariablesBuilder_ != null) {
updateWorkflowVariablesBuilder_.dispose();
updateWorkflowVariablesBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_Action_descriptor;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action getDefaultInstanceForType() {
return com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.getDefaultInstance();
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action build() {
com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action buildPartial() {
com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action result = new com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.action_ = action_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.startWorkflow_ = startWorkflowBuilder_ == null
? startWorkflow_
: startWorkflowBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.completeTask_ = completeTaskBuilder_ == null
? completeTask_
: completeTaskBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.failTask_ = failTaskBuilder_ == null
? failTask_
: failTaskBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.expandInlineJson_ = expandInlineJson_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.terminateWorkflow_ = terminateWorkflowBuilder_ == null
? terminateWorkflow_
: terminateWorkflowBuilder_.build();
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.updateWorkflowVariables_ = updateWorkflowVariablesBuilder_ == null
? updateWorkflowVariables_
: updateWorkflowVariablesBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action) {
return mergeFrom((com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action other) {
if (other == com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.getDefaultInstance()) return this;
if (other.action_ != 0) {
setActionValue(other.getActionValue());
}
if (other.hasStartWorkflow()) {
mergeStartWorkflow(other.getStartWorkflow());
}
if (other.hasCompleteTask()) {
mergeCompleteTask(other.getCompleteTask());
}
if (other.hasFailTask()) {
mergeFailTask(other.getFailTask());
}
if (other.getExpandInlineJson() != false) {
setExpandInlineJson(other.getExpandInlineJson());
}
if (other.hasTerminateWorkflow()) {
mergeTerminateWorkflow(other.getTerminateWorkflow());
}
if (other.hasUpdateWorkflowVariables()) {
mergeUpdateWorkflowVariables(other.getUpdateWorkflowVariables());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
action_ = input.readEnum();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
input.readMessage(
getStartWorkflowFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
input.readMessage(
getCompleteTaskFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
input.readMessage(
getFailTaskFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
case 40: {
expandInlineJson_ = input.readBool();
bitField0_ |= 0x00000010;
break;
} // case 40
case 50: {
input.readMessage(
getTerminateWorkflowFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 50
case 58: {
input.readMessage(
getUpdateWorkflowVariablesFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000040;
break;
} // case 58
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int action_ = 0;
/**
* .conductor.proto.EventHandler.Action.Type action = 1;
* @return The enum numeric value on the wire for action.
*/
@java.lang.Override public int getActionValue() {
return action_;
}
/**
* .conductor.proto.EventHandler.Action.Type action = 1;
* @param value The enum numeric value on the wire for action to set.
* @return This builder for chaining.
*/
public Builder setActionValue(int value) {
action_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.Action.Type action = 1;
* @return The action.
*/
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Type getAction() {
com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Type result = com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Type.forNumber(action_);
return result == null ? com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Type.UNRECOGNIZED : result;
}
/**
* .conductor.proto.EventHandler.Action.Type action = 1;
* @param value The action to set.
* @return This builder for chaining.
*/
public Builder setAction(com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Type value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
action_ = value.getNumber();
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.Action.Type action = 1;
* @return This builder for chaining.
*/
public Builder clearAction() {
bitField0_ = (bitField0_ & ~0x00000001);
action_ = 0;
onChanged();
return this;
}
private com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow startWorkflow_;
private com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow, com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflowOrBuilder> startWorkflowBuilder_;
/**
* .conductor.proto.EventHandler.StartWorkflow start_workflow = 2;
* @return Whether the startWorkflow field is set.
*/
public boolean hasStartWorkflow() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* .conductor.proto.EventHandler.StartWorkflow start_workflow = 2;
* @return The startWorkflow.
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow getStartWorkflow() {
if (startWorkflowBuilder_ == null) {
return startWorkflow_ == null ? com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow.getDefaultInstance() : startWorkflow_;
} else {
return startWorkflowBuilder_.getMessage();
}
}
/**
* .conductor.proto.EventHandler.StartWorkflow start_workflow = 2;
*/
public Builder setStartWorkflow(com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow value) {
if (startWorkflowBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
startWorkflow_ = value;
} else {
startWorkflowBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.StartWorkflow start_workflow = 2;
*/
public Builder setStartWorkflow(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow.Builder builderForValue) {
if (startWorkflowBuilder_ == null) {
startWorkflow_ = builderForValue.build();
} else {
startWorkflowBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.StartWorkflow start_workflow = 2;
*/
public Builder mergeStartWorkflow(com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow value) {
if (startWorkflowBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
startWorkflow_ != null &&
startWorkflow_ != com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow.getDefaultInstance()) {
getStartWorkflowBuilder().mergeFrom(value);
} else {
startWorkflow_ = value;
}
} else {
startWorkflowBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.StartWorkflow start_workflow = 2;
*/
public Builder clearStartWorkflow() {
bitField0_ = (bitField0_ & ~0x00000002);
startWorkflow_ = null;
if (startWorkflowBuilder_ != null) {
startWorkflowBuilder_.dispose();
startWorkflowBuilder_ = null;
}
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.StartWorkflow start_workflow = 2;
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow.Builder getStartWorkflowBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getStartWorkflowFieldBuilder().getBuilder();
}
/**
* .conductor.proto.EventHandler.StartWorkflow start_workflow = 2;
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflowOrBuilder getStartWorkflowOrBuilder() {
if (startWorkflowBuilder_ != null) {
return startWorkflowBuilder_.getMessageOrBuilder();
} else {
return startWorkflow_ == null ?
com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow.getDefaultInstance() : startWorkflow_;
}
}
/**
* .conductor.proto.EventHandler.StartWorkflow start_workflow = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow, com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflowOrBuilder>
getStartWorkflowFieldBuilder() {
if (startWorkflowBuilder_ == null) {
startWorkflowBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow, com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflow.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.StartWorkflowOrBuilder>(
getStartWorkflow(),
getParentForChildren(),
isClean());
startWorkflow_ = null;
}
return startWorkflowBuilder_;
}
private com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails completeTask_;
private com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetailsOrBuilder> completeTaskBuilder_;
/**
* .conductor.proto.EventHandler.TaskDetails complete_task = 3;
* @return Whether the completeTask field is set.
*/
public boolean hasCompleteTask() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* .conductor.proto.EventHandler.TaskDetails complete_task = 3;
* @return The completeTask.
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails getCompleteTask() {
if (completeTaskBuilder_ == null) {
return completeTask_ == null ? com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.getDefaultInstance() : completeTask_;
} else {
return completeTaskBuilder_.getMessage();
}
}
/**
* .conductor.proto.EventHandler.TaskDetails complete_task = 3;
*/
public Builder setCompleteTask(com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails value) {
if (completeTaskBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
completeTask_ = value;
} else {
completeTaskBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.TaskDetails complete_task = 3;
*/
public Builder setCompleteTask(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.Builder builderForValue) {
if (completeTaskBuilder_ == null) {
completeTask_ = builderForValue.build();
} else {
completeTaskBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.TaskDetails complete_task = 3;
*/
public Builder mergeCompleteTask(com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails value) {
if (completeTaskBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
completeTask_ != null &&
completeTask_ != com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.getDefaultInstance()) {
getCompleteTaskBuilder().mergeFrom(value);
} else {
completeTask_ = value;
}
} else {
completeTaskBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.TaskDetails complete_task = 3;
*/
public Builder clearCompleteTask() {
bitField0_ = (bitField0_ & ~0x00000004);
completeTask_ = null;
if (completeTaskBuilder_ != null) {
completeTaskBuilder_.dispose();
completeTaskBuilder_ = null;
}
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.TaskDetails complete_task = 3;
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.Builder getCompleteTaskBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getCompleteTaskFieldBuilder().getBuilder();
}
/**
* .conductor.proto.EventHandler.TaskDetails complete_task = 3;
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetailsOrBuilder getCompleteTaskOrBuilder() {
if (completeTaskBuilder_ != null) {
return completeTaskBuilder_.getMessageOrBuilder();
} else {
return completeTask_ == null ?
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.getDefaultInstance() : completeTask_;
}
}
/**
* .conductor.proto.EventHandler.TaskDetails complete_task = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetailsOrBuilder>
getCompleteTaskFieldBuilder() {
if (completeTaskBuilder_ == null) {
completeTaskBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetailsOrBuilder>(
getCompleteTask(),
getParentForChildren(),
isClean());
completeTask_ = null;
}
return completeTaskBuilder_;
}
private com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails failTask_;
private com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetailsOrBuilder> failTaskBuilder_;
/**
* .conductor.proto.EventHandler.TaskDetails fail_task = 4;
* @return Whether the failTask field is set.
*/
public boolean hasFailTask() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* .conductor.proto.EventHandler.TaskDetails fail_task = 4;
* @return The failTask.
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails getFailTask() {
if (failTaskBuilder_ == null) {
return failTask_ == null ? com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.getDefaultInstance() : failTask_;
} else {
return failTaskBuilder_.getMessage();
}
}
/**
* .conductor.proto.EventHandler.TaskDetails fail_task = 4;
*/
public Builder setFailTask(com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails value) {
if (failTaskBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
failTask_ = value;
} else {
failTaskBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.TaskDetails fail_task = 4;
*/
public Builder setFailTask(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.Builder builderForValue) {
if (failTaskBuilder_ == null) {
failTask_ = builderForValue.build();
} else {
failTaskBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.TaskDetails fail_task = 4;
*/
public Builder mergeFailTask(com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails value) {
if (failTaskBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
failTask_ != null &&
failTask_ != com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.getDefaultInstance()) {
getFailTaskBuilder().mergeFrom(value);
} else {
failTask_ = value;
}
} else {
failTaskBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.TaskDetails fail_task = 4;
*/
public Builder clearFailTask() {
bitField0_ = (bitField0_ & ~0x00000008);
failTask_ = null;
if (failTaskBuilder_ != null) {
failTaskBuilder_.dispose();
failTaskBuilder_ = null;
}
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.TaskDetails fail_task = 4;
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.Builder getFailTaskBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getFailTaskFieldBuilder().getBuilder();
}
/**
* .conductor.proto.EventHandler.TaskDetails fail_task = 4;
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetailsOrBuilder getFailTaskOrBuilder() {
if (failTaskBuilder_ != null) {
return failTaskBuilder_.getMessageOrBuilder();
} else {
return failTask_ == null ?
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.getDefaultInstance() : failTask_;
}
}
/**
* .conductor.proto.EventHandler.TaskDetails fail_task = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetailsOrBuilder>
getFailTaskFieldBuilder() {
if (failTaskBuilder_ == null) {
failTaskBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetails.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TaskDetailsOrBuilder>(
getFailTask(),
getParentForChildren(),
isClean());
failTask_ = null;
}
return failTaskBuilder_;
}
private boolean expandInlineJson_ ;
/**
* bool expand_inline_json = 5;
* @return The expandInlineJson.
*/
@java.lang.Override
public boolean getExpandInlineJson() {
return expandInlineJson_;
}
/**
* bool expand_inline_json = 5;
* @param value The expandInlineJson to set.
* @return This builder for chaining.
*/
public Builder setExpandInlineJson(boolean value) {
expandInlineJson_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* bool expand_inline_json = 5;
* @return This builder for chaining.
*/
public Builder clearExpandInlineJson() {
bitField0_ = (bitField0_ & ~0x00000010);
expandInlineJson_ = false;
onChanged();
return this;
}
private com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow terminateWorkflow_;
private com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflowOrBuilder> terminateWorkflowBuilder_;
/**
* .conductor.proto.EventHandler.TerminateWorkflow terminate_workflow = 6;
* @return Whether the terminateWorkflow field is set.
*/
public boolean hasTerminateWorkflow() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* .conductor.proto.EventHandler.TerminateWorkflow terminate_workflow = 6;
* @return The terminateWorkflow.
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow getTerminateWorkflow() {
if (terminateWorkflowBuilder_ == null) {
return terminateWorkflow_ == null ? com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow.getDefaultInstance() : terminateWorkflow_;
} else {
return terminateWorkflowBuilder_.getMessage();
}
}
/**
* .conductor.proto.EventHandler.TerminateWorkflow terminate_workflow = 6;
*/
public Builder setTerminateWorkflow(com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow value) {
if (terminateWorkflowBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
terminateWorkflow_ = value;
} else {
terminateWorkflowBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.TerminateWorkflow terminate_workflow = 6;
*/
public Builder setTerminateWorkflow(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow.Builder builderForValue) {
if (terminateWorkflowBuilder_ == null) {
terminateWorkflow_ = builderForValue.build();
} else {
terminateWorkflowBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.TerminateWorkflow terminate_workflow = 6;
*/
public Builder mergeTerminateWorkflow(com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow value) {
if (terminateWorkflowBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0) &&
terminateWorkflow_ != null &&
terminateWorkflow_ != com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow.getDefaultInstance()) {
getTerminateWorkflowBuilder().mergeFrom(value);
} else {
terminateWorkflow_ = value;
}
} else {
terminateWorkflowBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.TerminateWorkflow terminate_workflow = 6;
*/
public Builder clearTerminateWorkflow() {
bitField0_ = (bitField0_ & ~0x00000020);
terminateWorkflow_ = null;
if (terminateWorkflowBuilder_ != null) {
terminateWorkflowBuilder_.dispose();
terminateWorkflowBuilder_ = null;
}
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.TerminateWorkflow terminate_workflow = 6;
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow.Builder getTerminateWorkflowBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getTerminateWorkflowFieldBuilder().getBuilder();
}
/**
* .conductor.proto.EventHandler.TerminateWorkflow terminate_workflow = 6;
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflowOrBuilder getTerminateWorkflowOrBuilder() {
if (terminateWorkflowBuilder_ != null) {
return terminateWorkflowBuilder_.getMessageOrBuilder();
} else {
return terminateWorkflow_ == null ?
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow.getDefaultInstance() : terminateWorkflow_;
}
}
/**
* .conductor.proto.EventHandler.TerminateWorkflow terminate_workflow = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflowOrBuilder>
getTerminateWorkflowFieldBuilder() {
if (terminateWorkflowBuilder_ == null) {
terminateWorkflowBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflow.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.TerminateWorkflowOrBuilder>(
getTerminateWorkflow(),
getParentForChildren(),
isClean());
terminateWorkflow_ = null;
}
return terminateWorkflowBuilder_;
}
private com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables updateWorkflowVariables_;
private com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables, com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariablesOrBuilder> updateWorkflowVariablesBuilder_;
/**
* .conductor.proto.EventHandler.UpdateWorkflowVariables update_workflow_variables = 7;
* @return Whether the updateWorkflowVariables field is set.
*/
public boolean hasUpdateWorkflowVariables() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* .conductor.proto.EventHandler.UpdateWorkflowVariables update_workflow_variables = 7;
* @return The updateWorkflowVariables.
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables getUpdateWorkflowVariables() {
if (updateWorkflowVariablesBuilder_ == null) {
return updateWorkflowVariables_ == null ? com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables.getDefaultInstance() : updateWorkflowVariables_;
} else {
return updateWorkflowVariablesBuilder_.getMessage();
}
}
/**
* .conductor.proto.EventHandler.UpdateWorkflowVariables update_workflow_variables = 7;
*/
public Builder setUpdateWorkflowVariables(com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables value) {
if (updateWorkflowVariablesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
updateWorkflowVariables_ = value;
} else {
updateWorkflowVariablesBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.UpdateWorkflowVariables update_workflow_variables = 7;
*/
public Builder setUpdateWorkflowVariables(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables.Builder builderForValue) {
if (updateWorkflowVariablesBuilder_ == null) {
updateWorkflowVariables_ = builderForValue.build();
} else {
updateWorkflowVariablesBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.UpdateWorkflowVariables update_workflow_variables = 7;
*/
public Builder mergeUpdateWorkflowVariables(com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables value) {
if (updateWorkflowVariablesBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0) &&
updateWorkflowVariables_ != null &&
updateWorkflowVariables_ != com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables.getDefaultInstance()) {
getUpdateWorkflowVariablesBuilder().mergeFrom(value);
} else {
updateWorkflowVariables_ = value;
}
} else {
updateWorkflowVariablesBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.UpdateWorkflowVariables update_workflow_variables = 7;
*/
public Builder clearUpdateWorkflowVariables() {
bitField0_ = (bitField0_ & ~0x00000040);
updateWorkflowVariables_ = null;
if (updateWorkflowVariablesBuilder_ != null) {
updateWorkflowVariablesBuilder_.dispose();
updateWorkflowVariablesBuilder_ = null;
}
onChanged();
return this;
}
/**
* .conductor.proto.EventHandler.UpdateWorkflowVariables update_workflow_variables = 7;
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables.Builder getUpdateWorkflowVariablesBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getUpdateWorkflowVariablesFieldBuilder().getBuilder();
}
/**
* .conductor.proto.EventHandler.UpdateWorkflowVariables update_workflow_variables = 7;
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariablesOrBuilder getUpdateWorkflowVariablesOrBuilder() {
if (updateWorkflowVariablesBuilder_ != null) {
return updateWorkflowVariablesBuilder_.getMessageOrBuilder();
} else {
return updateWorkflowVariables_ == null ?
com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables.getDefaultInstance() : updateWorkflowVariables_;
}
}
/**
* .conductor.proto.EventHandler.UpdateWorkflowVariables update_workflow_variables = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables, com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariablesOrBuilder>
getUpdateWorkflowVariablesFieldBuilder() {
if (updateWorkflowVariablesBuilder_ == null) {
updateWorkflowVariablesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables, com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariables.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.UpdateWorkflowVariablesOrBuilder>(
getUpdateWorkflowVariables(),
getParentForChildren(),
isClean());
updateWorkflowVariables_ = null;
}
return updateWorkflowVariablesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:conductor.proto.EventHandler.Action)
}
// @@protoc_insertion_point(class_scope:conductor.proto.EventHandler.Action)
private static final com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action();
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Action parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
* string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EVENT_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object event_ = "";
/**
* string event = 2;
* @return The event.
*/
@java.lang.Override
public java.lang.String getEvent() {
java.lang.Object ref = event_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
event_ = s;
return s;
}
}
/**
* string event = 2;
* @return The bytes for event.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getEventBytes() {
java.lang.Object ref = event_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
event_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONDITION_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object condition_ = "";
/**
* string condition = 3;
* @return The condition.
*/
@java.lang.Override
public java.lang.String getCondition() {
java.lang.Object ref = condition_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
condition_ = s;
return s;
}
}
/**
* string condition = 3;
* @return The bytes for condition.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getConditionBytes() {
java.lang.Object ref = condition_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
condition_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACTIONS_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private java.util.List actions_;
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
@java.lang.Override
public java.util.List getActionsList() {
return actions_;
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
@java.lang.Override
public java.util.List extends com.netflix.conductor.proto.EventHandlerPb.EventHandler.ActionOrBuilder>
getActionsOrBuilderList() {
return actions_;
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
@java.lang.Override
public int getActionsCount() {
return actions_.size();
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action getActions(int index) {
return actions_.get(index);
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.ActionOrBuilder getActionsOrBuilder(
int index) {
return actions_.get(index);
}
public static final int ACTIVE_FIELD_NUMBER = 5;
private boolean active_ = false;
/**
* bool active = 5;
* @return The active.
*/
@java.lang.Override
public boolean getActive() {
return active_;
}
public static final int EVALUATOR_TYPE_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private volatile java.lang.Object evaluatorType_ = "";
/**
* string evaluator_type = 6;
* @return The evaluatorType.
*/
@java.lang.Override
public java.lang.String getEvaluatorType() {
java.lang.Object ref = evaluatorType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
evaluatorType_ = s;
return s;
}
}
/**
* string evaluator_type = 6;
* @return The bytes for evaluatorType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getEvaluatorTypeBytes() {
java.lang.Object ref = evaluatorType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
evaluatorType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(event_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, event_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(condition_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, condition_);
}
for (int i = 0; i < actions_.size(); i++) {
output.writeMessage(4, actions_.get(i));
}
if (active_ != false) {
output.writeBool(5, active_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(evaluatorType_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, evaluatorType_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(event_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, event_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(condition_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, condition_);
}
for (int i = 0; i < actions_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, actions_.get(i));
}
if (active_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, active_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(evaluatorType_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, evaluatorType_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.netflix.conductor.proto.EventHandlerPb.EventHandler)) {
return super.equals(obj);
}
com.netflix.conductor.proto.EventHandlerPb.EventHandler other = (com.netflix.conductor.proto.EventHandlerPb.EventHandler) obj;
if (!getName()
.equals(other.getName())) return false;
if (!getEvent()
.equals(other.getEvent())) return false;
if (!getCondition()
.equals(other.getCondition())) return false;
if (!getActionsList()
.equals(other.getActionsList())) return false;
if (getActive()
!= other.getActive()) return false;
if (!getEvaluatorType()
.equals(other.getEvaluatorType())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + EVENT_FIELD_NUMBER;
hash = (53 * hash) + getEvent().hashCode();
hash = (37 * hash) + CONDITION_FIELD_NUMBER;
hash = (53 * hash) + getCondition().hashCode();
if (getActionsCount() > 0) {
hash = (37 * hash) + ACTIONS_FIELD_NUMBER;
hash = (53 * hash) + getActionsList().hashCode();
}
hash = (37 * hash) + ACTIVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getActive());
hash = (37 * hash) + EVALUATOR_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getEvaluatorType().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.netflix.conductor.proto.EventHandlerPb.EventHandler prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code conductor.proto.EventHandler}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:conductor.proto.EventHandler)
com.netflix.conductor.proto.EventHandlerPb.EventHandlerOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.class, com.netflix.conductor.proto.EventHandlerPb.EventHandler.Builder.class);
}
// Construct using com.netflix.conductor.proto.EventHandlerPb.EventHandler.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
name_ = "";
event_ = "";
condition_ = "";
if (actionsBuilder_ == null) {
actions_ = java.util.Collections.emptyList();
} else {
actions_ = null;
actionsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
active_ = false;
evaluatorType_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.netflix.conductor.proto.EventHandlerPb.internal_static_conductor_proto_EventHandler_descriptor;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler getDefaultInstanceForType() {
return com.netflix.conductor.proto.EventHandlerPb.EventHandler.getDefaultInstance();
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler build() {
com.netflix.conductor.proto.EventHandlerPb.EventHandler result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler buildPartial() {
com.netflix.conductor.proto.EventHandlerPb.EventHandler result = new com.netflix.conductor.proto.EventHandlerPb.EventHandler(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.netflix.conductor.proto.EventHandlerPb.EventHandler result) {
if (actionsBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)) {
actions_ = java.util.Collections.unmodifiableList(actions_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.actions_ = actions_;
} else {
result.actions_ = actionsBuilder_.build();
}
}
private void buildPartial0(com.netflix.conductor.proto.EventHandlerPb.EventHandler result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.name_ = name_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.event_ = event_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.condition_ = condition_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.active_ = active_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.evaluatorType_ = evaluatorType_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.netflix.conductor.proto.EventHandlerPb.EventHandler) {
return mergeFrom((com.netflix.conductor.proto.EventHandlerPb.EventHandler)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.netflix.conductor.proto.EventHandlerPb.EventHandler other) {
if (other == com.netflix.conductor.proto.EventHandlerPb.EventHandler.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getEvent().isEmpty()) {
event_ = other.event_;
bitField0_ |= 0x00000002;
onChanged();
}
if (!other.getCondition().isEmpty()) {
condition_ = other.condition_;
bitField0_ |= 0x00000004;
onChanged();
}
if (actionsBuilder_ == null) {
if (!other.actions_.isEmpty()) {
if (actions_.isEmpty()) {
actions_ = other.actions_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureActionsIsMutable();
actions_.addAll(other.actions_);
}
onChanged();
}
} else {
if (!other.actions_.isEmpty()) {
if (actionsBuilder_.isEmpty()) {
actionsBuilder_.dispose();
actionsBuilder_ = null;
actions_ = other.actions_;
bitField0_ = (bitField0_ & ~0x00000008);
actionsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getActionsFieldBuilder() : null;
} else {
actionsBuilder_.addAllMessages(other.actions_);
}
}
}
if (other.getActive() != false) {
setActive(other.getActive());
}
if (!other.getEvaluatorType().isEmpty()) {
evaluatorType_ = other.evaluatorType_;
bitField0_ |= 0x00000020;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
event_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
condition_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action m =
input.readMessage(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.parser(),
extensionRegistry);
if (actionsBuilder_ == null) {
ensureActionsIsMutable();
actions_.add(m);
} else {
actionsBuilder_.addMessage(m);
}
break;
} // case 34
case 40: {
active_ = input.readBool();
bitField0_ |= 0x00000010;
break;
} // case 40
case 50: {
evaluatorType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 50
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
* string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object event_ = "";
/**
* string event = 2;
* @return The event.
*/
public java.lang.String getEvent() {
java.lang.Object ref = event_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
event_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string event = 2;
* @return The bytes for event.
*/
public com.google.protobuf.ByteString
getEventBytes() {
java.lang.Object ref = event_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
event_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string event = 2;
* @param value The event to set.
* @return This builder for chaining.
*/
public Builder setEvent(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
event_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string event = 2;
* @return This builder for chaining.
*/
public Builder clearEvent() {
event_ = getDefaultInstance().getEvent();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string event = 2;
* @param value The bytes for event to set.
* @return This builder for chaining.
*/
public Builder setEventBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
event_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object condition_ = "";
/**
* string condition = 3;
* @return The condition.
*/
public java.lang.String getCondition() {
java.lang.Object ref = condition_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
condition_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string condition = 3;
* @return The bytes for condition.
*/
public com.google.protobuf.ByteString
getConditionBytes() {
java.lang.Object ref = condition_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
condition_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string condition = 3;
* @param value The condition to set.
* @return This builder for chaining.
*/
public Builder setCondition(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
condition_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* string condition = 3;
* @return This builder for chaining.
*/
public Builder clearCondition() {
condition_ = getDefaultInstance().getCondition();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
* string condition = 3;
* @param value The bytes for condition to set.
* @return This builder for chaining.
*/
public Builder setConditionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
condition_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private java.util.List actions_ =
java.util.Collections.emptyList();
private void ensureActionsIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
actions_ = new java.util.ArrayList(actions_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action, com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.ActionOrBuilder> actionsBuilder_;
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public java.util.List getActionsList() {
if (actionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(actions_);
} else {
return actionsBuilder_.getMessageList();
}
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public int getActionsCount() {
if (actionsBuilder_ == null) {
return actions_.size();
} else {
return actionsBuilder_.getCount();
}
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action getActions(int index) {
if (actionsBuilder_ == null) {
return actions_.get(index);
} else {
return actionsBuilder_.getMessage(index);
}
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public Builder setActions(
int index, com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action value) {
if (actionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureActionsIsMutable();
actions_.set(index, value);
onChanged();
} else {
actionsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public Builder setActions(
int index, com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Builder builderForValue) {
if (actionsBuilder_ == null) {
ensureActionsIsMutable();
actions_.set(index, builderForValue.build());
onChanged();
} else {
actionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public Builder addActions(com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action value) {
if (actionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureActionsIsMutable();
actions_.add(value);
onChanged();
} else {
actionsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public Builder addActions(
int index, com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action value) {
if (actionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureActionsIsMutable();
actions_.add(index, value);
onChanged();
} else {
actionsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public Builder addActions(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Builder builderForValue) {
if (actionsBuilder_ == null) {
ensureActionsIsMutable();
actions_.add(builderForValue.build());
onChanged();
} else {
actionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public Builder addActions(
int index, com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Builder builderForValue) {
if (actionsBuilder_ == null) {
ensureActionsIsMutable();
actions_.add(index, builderForValue.build());
onChanged();
} else {
actionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public Builder addAllActions(
java.lang.Iterable extends com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action> values) {
if (actionsBuilder_ == null) {
ensureActionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, actions_);
onChanged();
} else {
actionsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public Builder clearActions() {
if (actionsBuilder_ == null) {
actions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
actionsBuilder_.clear();
}
return this;
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public Builder removeActions(int index) {
if (actionsBuilder_ == null) {
ensureActionsIsMutable();
actions_.remove(index);
onChanged();
} else {
actionsBuilder_.remove(index);
}
return this;
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Builder getActionsBuilder(
int index) {
return getActionsFieldBuilder().getBuilder(index);
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.ActionOrBuilder getActionsOrBuilder(
int index) {
if (actionsBuilder_ == null) {
return actions_.get(index); } else {
return actionsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public java.util.List extends com.netflix.conductor.proto.EventHandlerPb.EventHandler.ActionOrBuilder>
getActionsOrBuilderList() {
if (actionsBuilder_ != null) {
return actionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(actions_);
}
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Builder addActionsBuilder() {
return getActionsFieldBuilder().addBuilder(
com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.getDefaultInstance());
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Builder addActionsBuilder(
int index) {
return getActionsFieldBuilder().addBuilder(
index, com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.getDefaultInstance());
}
/**
* repeated .conductor.proto.EventHandler.Action actions = 4;
*/
public java.util.List
getActionsBuilderList() {
return getActionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action, com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.ActionOrBuilder>
getActionsFieldBuilder() {
if (actionsBuilder_ == null) {
actionsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action, com.netflix.conductor.proto.EventHandlerPb.EventHandler.Action.Builder, com.netflix.conductor.proto.EventHandlerPb.EventHandler.ActionOrBuilder>(
actions_,
((bitField0_ & 0x00000008) != 0),
getParentForChildren(),
isClean());
actions_ = null;
}
return actionsBuilder_;
}
private boolean active_ ;
/**
* bool active = 5;
* @return The active.
*/
@java.lang.Override
public boolean getActive() {
return active_;
}
/**
* bool active = 5;
* @param value The active to set.
* @return This builder for chaining.
*/
public Builder setActive(boolean value) {
active_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* bool active = 5;
* @return This builder for chaining.
*/
public Builder clearActive() {
bitField0_ = (bitField0_ & ~0x00000010);
active_ = false;
onChanged();
return this;
}
private java.lang.Object evaluatorType_ = "";
/**
* string evaluator_type = 6;
* @return The evaluatorType.
*/
public java.lang.String getEvaluatorType() {
java.lang.Object ref = evaluatorType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
evaluatorType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string evaluator_type = 6;
* @return The bytes for evaluatorType.
*/
public com.google.protobuf.ByteString
getEvaluatorTypeBytes() {
java.lang.Object ref = evaluatorType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
evaluatorType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string evaluator_type = 6;
* @param value The evaluatorType to set.
* @return This builder for chaining.
*/
public Builder setEvaluatorType(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
evaluatorType_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* string evaluator_type = 6;
* @return This builder for chaining.
*/
public Builder clearEvaluatorType() {
evaluatorType_ = getDefaultInstance().getEvaluatorType();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
* string evaluator_type = 6;
* @param value The bytes for evaluatorType to set.
* @return This builder for chaining.
*/
public Builder setEvaluatorTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
evaluatorType_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:conductor.proto.EventHandler)
}
// @@protoc_insertion_point(class_scope:conductor.proto.EventHandler)
private static final com.netflix.conductor.proto.EventHandlerPb.EventHandler DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.netflix.conductor.proto.EventHandlerPb.EventHandler();
}
public static com.netflix.conductor.proto.EventHandlerPb.EventHandler getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public EventHandler parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.netflix.conductor.proto.EventHandlerPb.EventHandler getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_conductor_proto_EventHandler_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_conductor_proto_EventHandler_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_conductor_proto_EventHandler_UpdateWorkflowVariables_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_conductor_proto_EventHandler_UpdateWorkflowVariables_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_conductor_proto_EventHandler_UpdateWorkflowVariables_VariablesEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_conductor_proto_EventHandler_UpdateWorkflowVariables_VariablesEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_conductor_proto_EventHandler_TerminateWorkflow_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_conductor_proto_EventHandler_TerminateWorkflow_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_conductor_proto_EventHandler_StartWorkflow_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_conductor_proto_EventHandler_StartWorkflow_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_conductor_proto_EventHandler_StartWorkflow_InputEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_conductor_proto_EventHandler_StartWorkflow_InputEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_conductor_proto_EventHandler_StartWorkflow_TaskToDomainEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_conductor_proto_EventHandler_StartWorkflow_TaskToDomainEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_conductor_proto_EventHandler_TaskDetails_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_conductor_proto_EventHandler_TaskDetails_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_conductor_proto_EventHandler_TaskDetails_OutputEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_conductor_proto_EventHandler_TaskDetails_OutputEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_conductor_proto_EventHandler_Action_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_conductor_proto_EventHandler_Action_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\030model/eventhandler.proto\022\017conductor.pr" +
"oto\032\034google/protobuf/struct.proto\032\031googl" +
"e/protobuf/any.proto\"\250\r\n\014EventHandler\022\014\n" +
"\004name\030\001 \001(\t\022\r\n\005event\030\002 \001(\t\022\021\n\tcondition\030" +
"\003 \001(\t\0225\n\007actions\030\004 \003(\0132$.conductor.proto" +
".EventHandler.Action\022\016\n\006active\030\005 \001(\010\022\026\n\016" +
"evaluator_type\030\006 \001(\t\032\347\001\n\027UpdateWorkflowV" +
"ariables\022\023\n\013workflow_id\030\001 \001(\t\022W\n\tvariabl" +
"es\030\002 \003(\0132D.conductor.proto.EventHandler." +
"UpdateWorkflowVariables.VariablesEntry\022\024" +
"\n\014append_array\030\003 \001(\010\032H\n\016VariablesEntry\022\013" +
"\n\003key\030\001 \001(\t\022%\n\005value\030\002 \001(\0132\026.google.prot" +
"obuf.Value:\0028\001\032D\n\021TerminateWorkflow\022\023\n\013w" +
"orkflow_id\030\001 \001(\t\022\032\n\022termination_reason\030\002" +
" \001(\t\032\214\003\n\rStartWorkflow\022\014\n\004name\030\001 \001(\t\022\017\n\007" +
"version\030\002 \001(\005\022\026\n\016correlation_id\030\003 \001(\t\022E\n" +
"\005input\030\004 \003(\01326.conductor.proto.EventHand" +
"ler.StartWorkflow.InputEntry\022+\n\rinput_me" +
"ssage\030\005 \001(\0132\024.google.protobuf.Any\022U\n\016tas" +
"k_to_domain\030\006 \003(\0132=.conductor.proto.Even" +
"tHandler.StartWorkflow.TaskToDomainEntry" +
"\032D\n\nInputEntry\022\013\n\003key\030\001 \001(\t\022%\n\005value\030\002 \001" +
"(\0132\026.google.protobuf.Value:\0028\001\0323\n\021TaskTo" +
"DomainEntry\022\013\n\003key\030\001 \001(\t\022\r\n\005value\030\002 \001(\t:" +
"\0028\001\032\206\002\n\013TaskDetails\022\023\n\013workflow_id\030\001 \001(\t" +
"\022\025\n\rtask_ref_name\030\002 \001(\t\022E\n\006output\030\003 \003(\0132" +
"5.conductor.proto.EventHandler.TaskDetai" +
"ls.OutputEntry\022,\n\016output_message\030\004 \001(\0132\024" +
".google.protobuf.Any\022\017\n\007task_id\030\005 \001(\t\032E\n" +
"\013OutputEntry\022\013\n\003key\030\001 \001(\t\022%\n\005value\030\002 \001(\013" +
"2\026.google.protobuf.Value:\0028\001\032\300\004\n\006Action\022" +
"9\n\006action\030\001 \001(\0162).conductor.proto.EventH" +
"andler.Action.Type\022C\n\016start_workflow\030\002 \001" +
"(\0132+.conductor.proto.EventHandler.StartW" +
"orkflow\022@\n\rcomplete_task\030\003 \001(\0132).conduct" +
"or.proto.EventHandler.TaskDetails\022<\n\tfai" +
"l_task\030\004 \001(\0132).conductor.proto.EventHand" +
"ler.TaskDetails\022\032\n\022expand_inline_json\030\005 " +
"\001(\010\022K\n\022terminate_workflow\030\006 \001(\0132/.conduc" +
"tor.proto.EventHandler.TerminateWorkflow" +
"\022X\n\031update_workflow_variables\030\007 \001(\01325.co" +
"nductor.proto.EventHandler.UpdateWorkflo" +
"wVariables\"s\n\004Type\022\022\n\016START_WORKFLOW\020\000\022\021" +
"\n\rCOMPLETE_TASK\020\001\022\r\n\tFAIL_TASK\020\002\022\026\n\022TERM" +
"INATE_WORKFLOW\020\003\022\035\n\031UPDATE_WORKFLOW_VARI" +
"ABLES\020\004Bi\n\033com.netflix.conductor.protoB\016" +
"EventHandlerPbZ:github.com/netflix/condu" +
"ctor/client/gogrpc/conductor/modelb\006prot" +
"o3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.StructProto.getDescriptor(),
com.google.protobuf.AnyProto.getDescriptor(),
});
internal_static_conductor_proto_EventHandler_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_conductor_proto_EventHandler_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_conductor_proto_EventHandler_descriptor,
new java.lang.String[] { "Name", "Event", "Condition", "Actions", "Active", "EvaluatorType", });
internal_static_conductor_proto_EventHandler_UpdateWorkflowVariables_descriptor =
internal_static_conductor_proto_EventHandler_descriptor.getNestedTypes().get(0);
internal_static_conductor_proto_EventHandler_UpdateWorkflowVariables_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_conductor_proto_EventHandler_UpdateWorkflowVariables_descriptor,
new java.lang.String[] { "WorkflowId", "Variables", "AppendArray", });
internal_static_conductor_proto_EventHandler_UpdateWorkflowVariables_VariablesEntry_descriptor =
internal_static_conductor_proto_EventHandler_UpdateWorkflowVariables_descriptor.getNestedTypes().get(0);
internal_static_conductor_proto_EventHandler_UpdateWorkflowVariables_VariablesEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_conductor_proto_EventHandler_UpdateWorkflowVariables_VariablesEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_conductor_proto_EventHandler_TerminateWorkflow_descriptor =
internal_static_conductor_proto_EventHandler_descriptor.getNestedTypes().get(1);
internal_static_conductor_proto_EventHandler_TerminateWorkflow_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_conductor_proto_EventHandler_TerminateWorkflow_descriptor,
new java.lang.String[] { "WorkflowId", "TerminationReason", });
internal_static_conductor_proto_EventHandler_StartWorkflow_descriptor =
internal_static_conductor_proto_EventHandler_descriptor.getNestedTypes().get(2);
internal_static_conductor_proto_EventHandler_StartWorkflow_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_conductor_proto_EventHandler_StartWorkflow_descriptor,
new java.lang.String[] { "Name", "Version", "CorrelationId", "Input", "InputMessage", "TaskToDomain", });
internal_static_conductor_proto_EventHandler_StartWorkflow_InputEntry_descriptor =
internal_static_conductor_proto_EventHandler_StartWorkflow_descriptor.getNestedTypes().get(0);
internal_static_conductor_proto_EventHandler_StartWorkflow_InputEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_conductor_proto_EventHandler_StartWorkflow_InputEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_conductor_proto_EventHandler_StartWorkflow_TaskToDomainEntry_descriptor =
internal_static_conductor_proto_EventHandler_StartWorkflow_descriptor.getNestedTypes().get(1);
internal_static_conductor_proto_EventHandler_StartWorkflow_TaskToDomainEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_conductor_proto_EventHandler_StartWorkflow_TaskToDomainEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_conductor_proto_EventHandler_TaskDetails_descriptor =
internal_static_conductor_proto_EventHandler_descriptor.getNestedTypes().get(3);
internal_static_conductor_proto_EventHandler_TaskDetails_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_conductor_proto_EventHandler_TaskDetails_descriptor,
new java.lang.String[] { "WorkflowId", "TaskRefName", "Output", "OutputMessage", "TaskId", });
internal_static_conductor_proto_EventHandler_TaskDetails_OutputEntry_descriptor =
internal_static_conductor_proto_EventHandler_TaskDetails_descriptor.getNestedTypes().get(0);
internal_static_conductor_proto_EventHandler_TaskDetails_OutputEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_conductor_proto_EventHandler_TaskDetails_OutputEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_conductor_proto_EventHandler_Action_descriptor =
internal_static_conductor_proto_EventHandler_descriptor.getNestedTypes().get(4);
internal_static_conductor_proto_EventHandler_Action_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_conductor_proto_EventHandler_Action_descriptor,
new java.lang.String[] { "Action", "StartWorkflow", "CompleteTask", "FailTask", "ExpandInlineJson", "TerminateWorkflow", "UpdateWorkflowVariables", });
com.google.protobuf.StructProto.getDescriptor();
com.google.protobuf.AnyProto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy