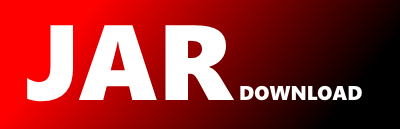
org.contextmapper.dsl.parser.antlr.internal.InternalContextMappingDSLParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of context-mapper-dsl Show documentation
Show all versions of context-mapper-dsl Show documentation
Use the ContextMapper DSL in your standalone application.
package org.contextmapper.dsl.parser.antlr.internal;
import org.eclipse.xtext.*;
import org.eclipse.xtext.parser.*;
import org.eclipse.xtext.parser.impl.*;
import org.eclipse.emf.ecore.util.EcoreUtil;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.emf.common.util.Enumerator;
import org.eclipse.xtext.parser.antlr.AbstractInternalAntlrParser;
import org.eclipse.xtext.parser.antlr.XtextTokenStream;
import org.eclipse.xtext.parser.antlr.XtextTokenStream.HiddenTokens;
import org.eclipse.xtext.parser.antlr.AntlrDatatypeRuleToken;
import org.contextmapper.dsl.services.ContextMappingDSLGrammarAccess;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
@SuppressWarnings("all")
public class InternalContextMappingDSLParser extends AbstractInternalAntlrParser {
public static final String[] tokenNames = new String[] {
"", "", "", "", "RULE_SL_COMMENT", "RULE_STRING", "RULE_ID", "RULE_OPEN", "RULE_CLOSE", "RULE_ML_COMMENT", "RULE_DELEGATE", "RULE_MAP_COLLECTION_TYPE", "RULE_NOT", "RULE_REF", "RULE_OPPOSITE", "RULE_INT", "RULE_WS", "RULE_ANY_OTHER", "'import'", "'ContextMap'", "'type'", "'='", "'state'", "'contains'", "','", "'BoundedContext'", "'implements'", "'realizes'", "'refines'", "'domainVisionStatement'", "'responsibilities'", "'implementationTechnology'", "'knowledgeLevel'", "'Domain'", "'Subdomain'", "'['", "'P'", "']'", "'<->'", "'Partnership'", "':'", "'SK'", "'Shared-Kernel'", "'U'", "'->'", "'D'", "'<-'", "'Upstream-Downstream'", "'Downstream-Upstream'", "'exposedAggregates'", "'downstreamRights'", "'S'", "'C'", "'Customer-Supplier'", "'Supplier-Customer'", "'Aggregate'", "'useCases'", "'userRequirements'", "'owner'", "'likelihoodForChange'", "'UseCase'", "'actor'", "'interactions'", "'benefit'", "'isLatencyCritical'", "'true'", "'reads'", "'writes'", "'UserStory'", "'As a'", "'As an'", "'I want to'", "'so that'", "'a'", "'an'", "'create'", "'read'", "'update'", "'delete'", "'Module'", "'external'", "'basePackage'", "'hint'", "'Application'", "'ApplicationPart'", "'Service'", "'gap'", "'nogap'", "'webservice'", "'Resource'", "'scaffold'", "'path'", "'Consumer'", "'unmarshall to'", "'@'", "'queueName'", "'topicName'", "'subscribe'", "'to'", "'eventBus'", "'publish'", "'void'", "'('", "')'", "'throws'", "';'", "'.'", "'return'", "'cache'", "'query'", "'condition'", "'select'", "'groupBy'", "'orderBy'", "'construct'", "'build'", "'map'", "'AccessObject'", "'<'", "'>'", "'abstract'", "'Entity'", "'extends'", "'with'", "'package'", "'optimisticLocking'", "'auditable'", "'databaseTable'", "'discriminatorValue'", "'discriminatorColumn'", "'discriminatorType'", "'discriminatorLength'", "'inheritanceType'", "'validate'", "'aggregateRoot'", "'belongsTo'", "'ValueObject'", "'immutable'", "'persistent'", "'DomainEvent'", "'CommandEvent'", "'Trait'", "'def'", "'*'", "'DataTransferObject'", "'BasicType'", "'key'", "'changeable'", "'required'", "'nullable'", "'index'", "'assertFalse'", "'assertTrue'", "'creditCardNumber'", "'digits'", "'email'", "'future'", "'past'", "'max'", "'min'", "'decimalMax'", "'decimalMin'", "'notEmpty'", "'notBlank'", "'pattern'", "'range'", "'size'", "'length'", "'scriptAssert'", "'url'", "'transient'", "'databaseColumn'", "'databaseType'", "'cascade'", "'fetch'", "'inverse'", "'databaseJoinTable'", "'databaseJoinColumn'", "'valid'", "'orderby'", "'orderColumn'", "'Repository'", "'inject'", "'enum'", "'ordinal'", "'String'", "'int'", "'Integer'", "'long'", "'Long'", "'boolean'", "'Boolean'", "'Date'", "'DateTime'", "'Timestamp'", "'BigDecimal'", "'BigInteger'", "'double'", "'Double'", "'float'", "'Float'", "'Key'", "'PagingParameter'", "'PagedResult'", "'Blob'", "'Clob'", "'Object[]'", "'/'", "'PL'", "'OHS'", "'ACL'", "'CF'", "'AS_IS'", "'TO_BE'", "'SYSTEM_LANDSCAPE'", "'ORGANIZATIONAL'", "'FEATURE'", "'APPLICATION'", "'SYSTEM'", "'TEAM'", "'CORE_DOMAIN'", "'SUPPORTING_DOMAIN'", "'GENERIC_SUBDOMAIN'", "'INFLUENCER'", "'OPINION_LEADER'", "'VETO_RIGHT'", "'DECISION_MAKER'", "'MONOPOLIST'", "'META'", "'CONCRETE'", "'NORMAL'", "'RARELY'", "'OFTEN'", "'None'", "'GET'", "'POST'", "'PUT'", "'DELETE'", "'JOINED'", "'SINGLE_TABLE'", "'STRING'", "'CHAR'", "'INTEGER'", "'Set'", "'List'", "'Bag'", "'Collection'", "'public'", "'protected'", "'private'"
};
public static final int T__144=144;
public static final int T__143=143;
public static final int T__146=146;
public static final int T__50=50;
public static final int T__145=145;
public static final int T__140=140;
public static final int T__142=142;
public static final int T__141=141;
public static final int T__59=59;
public static final int T__55=55;
public static final int T__56=56;
public static final int T__57=57;
public static final int T__58=58;
public static final int T__51=51;
public static final int T__137=137;
public static final int T__52=52;
public static final int T__136=136;
public static final int T__53=53;
public static final int T__139=139;
public static final int T__54=54;
public static final int T__138=138;
public static final int T__133=133;
public static final int T__132=132;
public static final int T__60=60;
public static final int T__135=135;
public static final int T__61=61;
public static final int T__134=134;
public static final int RULE_ID=6;
public static final int T__131=131;
public static final int T__130=130;
public static final int RULE_INT=15;
public static final int T__66=66;
public static final int T__67=67;
public static final int T__129=129;
public static final int T__68=68;
public static final int T__69=69;
public static final int T__62=62;
public static final int T__126=126;
public static final int T__247=247;
public static final int T__63=63;
public static final int T__125=125;
public static final int T__246=246;
public static final int T__64=64;
public static final int T__128=128;
public static final int T__249=249;
public static final int T__65=65;
public static final int T__127=127;
public static final int T__248=248;
public static final int T__166=166;
public static final int T__165=165;
public static final int T__168=168;
public static final int T__167=167;
public static final int T__162=162;
public static final int T__161=161;
public static final int T__164=164;
public static final int T__163=163;
public static final int T__160=160;
public static final int T__37=37;
public static final int T__38=38;
public static final int T__39=39;
public static final int T__33=33;
public static final int T__34=34;
public static final int T__35=35;
public static final int T__36=36;
public static final int T__159=159;
public static final int T__30=30;
public static final int T__158=158;
public static final int T__31=31;
public static final int T__32=32;
public static final int T__155=155;
public static final int T__154=154;
public static final int T__157=157;
public static final int T__156=156;
public static final int T__151=151;
public static final int T__150=150;
public static final int T__153=153;
public static final int T__152=152;
public static final int T__48=48;
public static final int T__49=49;
public static final int T__44=44;
public static final int T__45=45;
public static final int T__46=46;
public static final int T__47=47;
public static final int T__40=40;
public static final int T__148=148;
public static final int T__41=41;
public static final int T__147=147;
public static final int T__42=42;
public static final int T__43=43;
public static final int T__149=149;
public static final int T__100=100;
public static final int T__221=221;
public static final int T__220=220;
public static final int T__102=102;
public static final int T__223=223;
public static final int T__101=101;
public static final int T__222=222;
public static final int RULE_OPEN=7;
public static final int RULE_REF=13;
public static final int T__19=19;
public static final int T__18=18;
public static final int T__218=218;
public static final int T__217=217;
public static final int T__219=219;
public static final int T__214=214;
public static final int T__213=213;
public static final int T__216=216;
public static final int T__215=215;
public static final int T__210=210;
public static final int T__212=212;
public static final int T__211=211;
public static final int T__26=26;
public static final int T__27=27;
public static final int T__28=28;
public static final int RULE_OPPOSITE=14;
public static final int T__29=29;
public static final int T__22=22;
public static final int T__207=207;
public static final int T__23=23;
public static final int T__206=206;
public static final int T__24=24;
public static final int T__209=209;
public static final int T__25=25;
public static final int T__208=208;
public static final int T__203=203;
public static final int T__202=202;
public static final int T__20=20;
public static final int T__205=205;
public static final int T__21=21;
public static final int T__204=204;
public static final int T__122=122;
public static final int T__243=243;
public static final int T__121=121;
public static final int T__242=242;
public static final int T__124=124;
public static final int T__245=245;
public static final int T__123=123;
public static final int T__244=244;
public static final int T__120=120;
public static final int T__241=241;
public static final int T__240=240;
public static final int RULE_NOT=12;
public static final int RULE_SL_COMMENT=4;
public static final int T__119=119;
public static final int T__118=118;
public static final int T__239=239;
public static final int T__115=115;
public static final int T__236=236;
public static final int EOF=-1;
public static final int T__114=114;
public static final int T__235=235;
public static final int T__117=117;
public static final int T__238=238;
public static final int T__116=116;
public static final int T__237=237;
public static final int T__111=111;
public static final int T__232=232;
public static final int T__110=110;
public static final int T__231=231;
public static final int T__113=113;
public static final int T__234=234;
public static final int T__112=112;
public static final int T__233=233;
public static final int T__230=230;
public static final int T__108=108;
public static final int T__229=229;
public static final int T__107=107;
public static final int T__228=228;
public static final int T__109=109;
public static final int T__104=104;
public static final int T__225=225;
public static final int T__103=103;
public static final int T__224=224;
public static final int T__106=106;
public static final int T__227=227;
public static final int T__105=105;
public static final int T__226=226;
public static final int RULE_ML_COMMENT=9;
public static final int T__201=201;
public static final int T__200=200;
public static final int RULE_MAP_COLLECTION_TYPE=11;
public static final int T__91=91;
public static final int T__188=188;
public static final int T__92=92;
public static final int T__187=187;
public static final int T__93=93;
public static final int T__94=94;
public static final int T__189=189;
public static final int T__184=184;
public static final int T__183=183;
public static final int T__186=186;
public static final int T__90=90;
public static final int T__185=185;
public static final int T__180=180;
public static final int T__182=182;
public static final int T__181=181;
public static final int T__99=99;
public static final int T__95=95;
public static final int T__96=96;
public static final int T__97=97;
public static final int T__98=98;
public static final int T__177=177;
public static final int T__176=176;
public static final int T__179=179;
public static final int T__178=178;
public static final int T__173=173;
public static final int T__172=172;
public static final int T__175=175;
public static final int T__174=174;
public static final int T__171=171;
public static final int T__170=170;
public static final int T__169=169;
public static final int T__70=70;
public static final int T__71=71;
public static final int T__72=72;
public static final int RULE_CLOSE=8;
public static final int RULE_STRING=5;
public static final int T__77=77;
public static final int T__78=78;
public static final int T__79=79;
public static final int T__73=73;
public static final int T__74=74;
public static final int T__75=75;
public static final int T__76=76;
public static final int T__80=80;
public static final int T__199=199;
public static final int T__81=81;
public static final int T__198=198;
public static final int T__82=82;
public static final int T__83=83;
public static final int T__195=195;
public static final int T__194=194;
public static final int RULE_WS=16;
public static final int T__197=197;
public static final int T__196=196;
public static final int T__191=191;
public static final int T__190=190;
public static final int T__193=193;
public static final int T__192=192;
public static final int RULE_ANY_OTHER=17;
public static final int RULE_DELEGATE=10;
public static final int T__88=88;
public static final int T__89=89;
public static final int T__84=84;
public static final int T__85=85;
public static final int T__86=86;
public static final int T__87=87;
// delegates
// delegators
public InternalContextMappingDSLParser(TokenStream input) {
this(input, new RecognizerSharedState());
}
public InternalContextMappingDSLParser(TokenStream input, RecognizerSharedState state) {
super(input, state);
}
public String[] getTokenNames() { return InternalContextMappingDSLParser.tokenNames; }
public String getGrammarFileName() { return "InternalContextMappingDSL.g"; }
private ContextMappingDSLGrammarAccess grammarAccess;
public InternalContextMappingDSLParser(TokenStream input, ContextMappingDSLGrammarAccess grammarAccess) {
this(input);
this.grammarAccess = grammarAccess;
registerRules(grammarAccess.getGrammar());
}
@Override
protected String getFirstRuleName() {
return "ContextMappingModel";
}
@Override
protected ContextMappingDSLGrammarAccess getGrammarAccess() {
return grammarAccess;
}
// $ANTLR start "entryRuleContextMappingModel"
// InternalContextMappingDSL.g:65:1: entryRuleContextMappingModel returns [EObject current=null] : iv_ruleContextMappingModel= ruleContextMappingModel EOF ;
public final EObject entryRuleContextMappingModel() throws RecognitionException {
EObject current = null;
EObject iv_ruleContextMappingModel = null;
try {
// InternalContextMappingDSL.g:65:60: (iv_ruleContextMappingModel= ruleContextMappingModel EOF )
// InternalContextMappingDSL.g:66:2: iv_ruleContextMappingModel= ruleContextMappingModel EOF
{
newCompositeNode(grammarAccess.getContextMappingModelRule());
pushFollow(FOLLOW_1);
iv_ruleContextMappingModel=ruleContextMappingModel();
state._fsp--;
current =iv_ruleContextMappingModel;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleContextMappingModel"
// $ANTLR start "ruleContextMappingModel"
// InternalContextMappingDSL.g:72:1: ruleContextMappingModel returns [EObject current=null] : ( ( ( ( ({...}? => ( ({...}? => ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_imports_2_0= ruleImport ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_map_3_0= ruleContextMap ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_boundedContexts_4_0= ruleBoundedContext ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_domains_5_0= ruleDomain ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_userRequirements_6_0= ruleUserRequirement ) ) )+ ) ) )* ) ) ) ;
public final EObject ruleContextMappingModel() throws RecognitionException {
EObject current = null;
Token lv_firstLineComment_1_0=null;
EObject lv_imports_2_0 = null;
EObject lv_map_3_0 = null;
EObject lv_boundedContexts_4_0 = null;
EObject lv_domains_5_0 = null;
EObject lv_userRequirements_6_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:78:2: ( ( ( ( ( ({...}? => ( ({...}? => ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_imports_2_0= ruleImport ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_map_3_0= ruleContextMap ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_boundedContexts_4_0= ruleBoundedContext ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_domains_5_0= ruleDomain ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_userRequirements_6_0= ruleUserRequirement ) ) )+ ) ) )* ) ) ) )
// InternalContextMappingDSL.g:79:2: ( ( ( ( ({...}? => ( ({...}? => ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_imports_2_0= ruleImport ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_map_3_0= ruleContextMap ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_boundedContexts_4_0= ruleBoundedContext ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_domains_5_0= ruleDomain ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_userRequirements_6_0= ruleUserRequirement ) ) )+ ) ) )* ) ) )
{
// InternalContextMappingDSL.g:79:2: ( ( ( ( ({...}? => ( ({...}? => ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_imports_2_0= ruleImport ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_map_3_0= ruleContextMap ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_boundedContexts_4_0= ruleBoundedContext ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_domains_5_0= ruleDomain ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_userRequirements_6_0= ruleUserRequirement ) ) )+ ) ) )* ) ) )
// InternalContextMappingDSL.g:80:3: ( ( ( ({...}? => ( ({...}? => ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_imports_2_0= ruleImport ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_map_3_0= ruleContextMap ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_boundedContexts_4_0= ruleBoundedContext ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_domains_5_0= ruleDomain ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_userRequirements_6_0= ruleUserRequirement ) ) )+ ) ) )* ) )
{
// InternalContextMappingDSL.g:80:3: ( ( ( ({...}? => ( ({...}? => ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_imports_2_0= ruleImport ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_map_3_0= ruleContextMap ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_boundedContexts_4_0= ruleBoundedContext ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_domains_5_0= ruleDomain ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_userRequirements_6_0= ruleUserRequirement ) ) )+ ) ) )* ) )
// InternalContextMappingDSL.g:81:4: ( ( ({...}? => ( ({...}? => ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_imports_2_0= ruleImport ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_map_3_0= ruleContextMap ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_boundedContexts_4_0= ruleBoundedContext ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_domains_5_0= ruleDomain ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_userRequirements_6_0= ruleUserRequirement ) ) )+ ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getContextMappingModelAccess().getUnorderedGroup());
// InternalContextMappingDSL.g:84:4: ( ( ({...}? => ( ({...}? => ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_imports_2_0= ruleImport ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_map_3_0= ruleContextMap ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_boundedContexts_4_0= ruleBoundedContext ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_domains_5_0= ruleDomain ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_userRequirements_6_0= ruleUserRequirement ) ) )+ ) ) )* )
// InternalContextMappingDSL.g:85:5: ( ({...}? => ( ({...}? => ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_imports_2_0= ruleImport ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_map_3_0= ruleContextMap ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_boundedContexts_4_0= ruleBoundedContext ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_domains_5_0= ruleDomain ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_userRequirements_6_0= ruleUserRequirement ) ) )+ ) ) )*
{
// InternalContextMappingDSL.g:85:5: ( ({...}? => ( ({...}? => ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_imports_2_0= ruleImport ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_map_3_0= ruleContextMap ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_boundedContexts_4_0= ruleBoundedContext ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_domains_5_0= ruleDomain ) ) )+ ) ) | ({...}? => ( ({...}? => ( (lv_userRequirements_6_0= ruleUserRequirement ) ) )+ ) ) )*
loop5:
do {
int alt5=7;
int LA5_0 = input.LA(1);
if ( LA5_0 == RULE_SL_COMMENT && ( getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 0) || getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 3) ) ) {
int LA5_2 = input.LA(2);
if ( getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 0) ) {
alt5=1;
}
else if ( getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 3) ) {
alt5=4;
}
}
else if ( LA5_0 == 18 && getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 1) ) {
alt5=2;
}
else if ( LA5_0 == 19 && getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 2) ) {
alt5=3;
}
else if ( LA5_0 == 25 && getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 3) ) {
alt5=4;
}
else if ( LA5_0 == 33 && getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 4) ) {
alt5=5;
}
else if ( ( LA5_0 == 60 || LA5_0 == 68 ) && getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 5) ) {
alt5=6;
}
switch (alt5) {
case 1 :
// InternalContextMappingDSL.g:86:3: ({...}? => ( ({...}? => ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) ) ) ) )
{
// InternalContextMappingDSL.g:86:3: ({...}? => ( ({...}? => ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) ) ) ) )
// InternalContextMappingDSL.g:87:4: {...}? => ( ({...}? => ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 0) ) {
throw new FailedPredicateException(input, "ruleContextMappingModel", "getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 0)");
}
// InternalContextMappingDSL.g:87:113: ( ({...}? => ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) ) ) )
// InternalContextMappingDSL.g:88:5: ({...}? => ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 0);
// InternalContextMappingDSL.g:91:8: ({...}? => ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) ) )
// InternalContextMappingDSL.g:91:9: {...}? => ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleContextMappingModel", "true");
}
// InternalContextMappingDSL.g:91:18: ( (lv_firstLineComment_1_0= RULE_SL_COMMENT ) )
// InternalContextMappingDSL.g:91:19: (lv_firstLineComment_1_0= RULE_SL_COMMENT )
{
// InternalContextMappingDSL.g:91:19: (lv_firstLineComment_1_0= RULE_SL_COMMENT )
// InternalContextMappingDSL.g:92:9: lv_firstLineComment_1_0= RULE_SL_COMMENT
{
lv_firstLineComment_1_0=(Token)match(input,RULE_SL_COMMENT,FOLLOW_3);
newLeafNode(lv_firstLineComment_1_0, grammarAccess.getContextMappingModelAccess().getFirstLineCommentSL_COMMENTTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getContextMappingModelRule());
}
setWithLastConsumed(
current,
"firstLineComment",
lv_firstLineComment_1_0,
"org.eclipse.xtext.common.Terminals.SL_COMMENT");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getContextMappingModelAccess().getUnorderedGroup());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:113:3: ({...}? => ( ({...}? => ( (lv_imports_2_0= ruleImport ) ) )+ ) )
{
// InternalContextMappingDSL.g:113:3: ({...}? => ( ({...}? => ( (lv_imports_2_0= ruleImport ) ) )+ ) )
// InternalContextMappingDSL.g:114:4: {...}? => ( ({...}? => ( (lv_imports_2_0= ruleImport ) ) )+ )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 1) ) {
throw new FailedPredicateException(input, "ruleContextMappingModel", "getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 1)");
}
// InternalContextMappingDSL.g:114:113: ( ({...}? => ( (lv_imports_2_0= ruleImport ) ) )+ )
// InternalContextMappingDSL.g:115:5: ({...}? => ( (lv_imports_2_0= ruleImport ) ) )+
{
getUnorderedGroupHelper().select(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 1);
// InternalContextMappingDSL.g:118:8: ({...}? => ( (lv_imports_2_0= ruleImport ) ) )+
int cnt1=0;
loop1:
do {
int alt1=2;
int LA1_0 = input.LA(1);
if ( (LA1_0==18) ) {
int LA1_2 = input.LA(2);
if ( ((true)) ) {
alt1=1;
}
}
switch (alt1) {
case 1 :
// InternalContextMappingDSL.g:118:9: {...}? => ( (lv_imports_2_0= ruleImport ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleContextMappingModel", "true");
}
// InternalContextMappingDSL.g:118:18: ( (lv_imports_2_0= ruleImport ) )
// InternalContextMappingDSL.g:118:19: (lv_imports_2_0= ruleImport )
{
// InternalContextMappingDSL.g:118:19: (lv_imports_2_0= ruleImport )
// InternalContextMappingDSL.g:119:9: lv_imports_2_0= ruleImport
{
newCompositeNode(grammarAccess.getContextMappingModelAccess().getImportsImportParserRuleCall_1_0());
pushFollow(FOLLOW_3);
lv_imports_2_0=ruleImport();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getContextMappingModelRule());
}
add(
current,
"imports",
lv_imports_2_0,
"org.contextmapper.dsl.ContextMappingDSL.Import");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
if ( cnt1 >= 1 ) break loop1;
EarlyExitException eee =
new EarlyExitException(1, input);
throw eee;
}
cnt1++;
} while (true);
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getContextMappingModelAccess().getUnorderedGroup());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:141:3: ({...}? => ( ({...}? => ( (lv_map_3_0= ruleContextMap ) ) ) ) )
{
// InternalContextMappingDSL.g:141:3: ({...}? => ( ({...}? => ( (lv_map_3_0= ruleContextMap ) ) ) ) )
// InternalContextMappingDSL.g:142:4: {...}? => ( ({...}? => ( (lv_map_3_0= ruleContextMap ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 2) ) {
throw new FailedPredicateException(input, "ruleContextMappingModel", "getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 2)");
}
// InternalContextMappingDSL.g:142:113: ( ({...}? => ( (lv_map_3_0= ruleContextMap ) ) ) )
// InternalContextMappingDSL.g:143:5: ({...}? => ( (lv_map_3_0= ruleContextMap ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 2);
// InternalContextMappingDSL.g:146:8: ({...}? => ( (lv_map_3_0= ruleContextMap ) ) )
// InternalContextMappingDSL.g:146:9: {...}? => ( (lv_map_3_0= ruleContextMap ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleContextMappingModel", "true");
}
// InternalContextMappingDSL.g:146:18: ( (lv_map_3_0= ruleContextMap ) )
// InternalContextMappingDSL.g:146:19: (lv_map_3_0= ruleContextMap )
{
// InternalContextMappingDSL.g:146:19: (lv_map_3_0= ruleContextMap )
// InternalContextMappingDSL.g:147:9: lv_map_3_0= ruleContextMap
{
newCompositeNode(grammarAccess.getContextMappingModelAccess().getMapContextMapParserRuleCall_2_0());
pushFollow(FOLLOW_3);
lv_map_3_0=ruleContextMap();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getContextMappingModelRule());
}
set(
current,
"map",
lv_map_3_0,
"org.contextmapper.dsl.ContextMappingDSL.ContextMap");
afterParserOrEnumRuleCall();
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getContextMappingModelAccess().getUnorderedGroup());
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:169:3: ({...}? => ( ({...}? => ( (lv_boundedContexts_4_0= ruleBoundedContext ) ) )+ ) )
{
// InternalContextMappingDSL.g:169:3: ({...}? => ( ({...}? => ( (lv_boundedContexts_4_0= ruleBoundedContext ) ) )+ ) )
// InternalContextMappingDSL.g:170:4: {...}? => ( ({...}? => ( (lv_boundedContexts_4_0= ruleBoundedContext ) ) )+ )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 3) ) {
throw new FailedPredicateException(input, "ruleContextMappingModel", "getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 3)");
}
// InternalContextMappingDSL.g:170:113: ( ({...}? => ( (lv_boundedContexts_4_0= ruleBoundedContext ) ) )+ )
// InternalContextMappingDSL.g:171:5: ({...}? => ( (lv_boundedContexts_4_0= ruleBoundedContext ) ) )+
{
getUnorderedGroupHelper().select(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 3);
// InternalContextMappingDSL.g:174:8: ({...}? => ( (lv_boundedContexts_4_0= ruleBoundedContext ) ) )+
int cnt2=0;
loop2:
do {
int alt2=2;
int LA2_0 = input.LA(1);
if ( (LA2_0==RULE_SL_COMMENT) ) {
int LA2_2 = input.LA(2);
if ( ((true)) ) {
alt2=1;
}
}
else if ( (LA2_0==25) ) {
int LA2_3 = input.LA(2);
if ( ((true)) ) {
alt2=1;
}
}
switch (alt2) {
case 1 :
// InternalContextMappingDSL.g:174:9: {...}? => ( (lv_boundedContexts_4_0= ruleBoundedContext ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleContextMappingModel", "true");
}
// InternalContextMappingDSL.g:174:18: ( (lv_boundedContexts_4_0= ruleBoundedContext ) )
// InternalContextMappingDSL.g:174:19: (lv_boundedContexts_4_0= ruleBoundedContext )
{
// InternalContextMappingDSL.g:174:19: (lv_boundedContexts_4_0= ruleBoundedContext )
// InternalContextMappingDSL.g:175:9: lv_boundedContexts_4_0= ruleBoundedContext
{
newCompositeNode(grammarAccess.getContextMappingModelAccess().getBoundedContextsBoundedContextParserRuleCall_3_0());
pushFollow(FOLLOW_3);
lv_boundedContexts_4_0=ruleBoundedContext();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getContextMappingModelRule());
}
add(
current,
"boundedContexts",
lv_boundedContexts_4_0,
"org.contextmapper.dsl.ContextMappingDSL.BoundedContext");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
if ( cnt2 >= 1 ) break loop2;
EarlyExitException eee =
new EarlyExitException(2, input);
throw eee;
}
cnt2++;
} while (true);
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getContextMappingModelAccess().getUnorderedGroup());
}
}
}
break;
case 5 :
// InternalContextMappingDSL.g:197:3: ({...}? => ( ({...}? => ( (lv_domains_5_0= ruleDomain ) ) )+ ) )
{
// InternalContextMappingDSL.g:197:3: ({...}? => ( ({...}? => ( (lv_domains_5_0= ruleDomain ) ) )+ ) )
// InternalContextMappingDSL.g:198:4: {...}? => ( ({...}? => ( (lv_domains_5_0= ruleDomain ) ) )+ )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 4) ) {
throw new FailedPredicateException(input, "ruleContextMappingModel", "getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 4)");
}
// InternalContextMappingDSL.g:198:113: ( ({...}? => ( (lv_domains_5_0= ruleDomain ) ) )+ )
// InternalContextMappingDSL.g:199:5: ({...}? => ( (lv_domains_5_0= ruleDomain ) ) )+
{
getUnorderedGroupHelper().select(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 4);
// InternalContextMappingDSL.g:202:8: ({...}? => ( (lv_domains_5_0= ruleDomain ) ) )+
int cnt3=0;
loop3:
do {
int alt3=2;
int LA3_0 = input.LA(1);
if ( (LA3_0==33) ) {
int LA3_2 = input.LA(2);
if ( ((true)) ) {
alt3=1;
}
}
switch (alt3) {
case 1 :
// InternalContextMappingDSL.g:202:9: {...}? => ( (lv_domains_5_0= ruleDomain ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleContextMappingModel", "true");
}
// InternalContextMappingDSL.g:202:18: ( (lv_domains_5_0= ruleDomain ) )
// InternalContextMappingDSL.g:202:19: (lv_domains_5_0= ruleDomain )
{
// InternalContextMappingDSL.g:202:19: (lv_domains_5_0= ruleDomain )
// InternalContextMappingDSL.g:203:9: lv_domains_5_0= ruleDomain
{
newCompositeNode(grammarAccess.getContextMappingModelAccess().getDomainsDomainParserRuleCall_4_0());
pushFollow(FOLLOW_3);
lv_domains_5_0=ruleDomain();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getContextMappingModelRule());
}
add(
current,
"domains",
lv_domains_5_0,
"org.contextmapper.dsl.ContextMappingDSL.Domain");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
if ( cnt3 >= 1 ) break loop3;
EarlyExitException eee =
new EarlyExitException(3, input);
throw eee;
}
cnt3++;
} while (true);
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getContextMappingModelAccess().getUnorderedGroup());
}
}
}
break;
case 6 :
// InternalContextMappingDSL.g:225:3: ({...}? => ( ({...}? => ( (lv_userRequirements_6_0= ruleUserRequirement ) ) )+ ) )
{
// InternalContextMappingDSL.g:225:3: ({...}? => ( ({...}? => ( (lv_userRequirements_6_0= ruleUserRequirement ) ) )+ ) )
// InternalContextMappingDSL.g:226:4: {...}? => ( ({...}? => ( (lv_userRequirements_6_0= ruleUserRequirement ) ) )+ )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 5) ) {
throw new FailedPredicateException(input, "ruleContextMappingModel", "getUnorderedGroupHelper().canSelect(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 5)");
}
// InternalContextMappingDSL.g:226:113: ( ({...}? => ( (lv_userRequirements_6_0= ruleUserRequirement ) ) )+ )
// InternalContextMappingDSL.g:227:5: ({...}? => ( (lv_userRequirements_6_0= ruleUserRequirement ) ) )+
{
getUnorderedGroupHelper().select(grammarAccess.getContextMappingModelAccess().getUnorderedGroup(), 5);
// InternalContextMappingDSL.g:230:8: ({...}? => ( (lv_userRequirements_6_0= ruleUserRequirement ) ) )+
int cnt4=0;
loop4:
do {
int alt4=2;
int LA4_0 = input.LA(1);
if ( (LA4_0==60) ) {
int LA4_2 = input.LA(2);
if ( ((true)) ) {
alt4=1;
}
}
else if ( (LA4_0==68) ) {
int LA4_3 = input.LA(2);
if ( ((true)) ) {
alt4=1;
}
}
switch (alt4) {
case 1 :
// InternalContextMappingDSL.g:230:9: {...}? => ( (lv_userRequirements_6_0= ruleUserRequirement ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleContextMappingModel", "true");
}
// InternalContextMappingDSL.g:230:18: ( (lv_userRequirements_6_0= ruleUserRequirement ) )
// InternalContextMappingDSL.g:230:19: (lv_userRequirements_6_0= ruleUserRequirement )
{
// InternalContextMappingDSL.g:230:19: (lv_userRequirements_6_0= ruleUserRequirement )
// InternalContextMappingDSL.g:231:9: lv_userRequirements_6_0= ruleUserRequirement
{
newCompositeNode(grammarAccess.getContextMappingModelAccess().getUserRequirementsUserRequirementParserRuleCall_5_0());
pushFollow(FOLLOW_3);
lv_userRequirements_6_0=ruleUserRequirement();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getContextMappingModelRule());
}
add(
current,
"userRequirements",
lv_userRequirements_6_0,
"org.contextmapper.dsl.ContextMappingDSL.UserRequirement");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
if ( cnt4 >= 1 ) break loop4;
EarlyExitException eee =
new EarlyExitException(4, input);
throw eee;
}
cnt4++;
} while (true);
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getContextMappingModelAccess().getUnorderedGroup());
}
}
}
break;
default :
break loop5;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getContextMappingModelAccess().getUnorderedGroup());
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleContextMappingModel"
// $ANTLR start "entryRuleImport"
// InternalContextMappingDSL.g:263:1: entryRuleImport returns [EObject current=null] : iv_ruleImport= ruleImport EOF ;
public final EObject entryRuleImport() throws RecognitionException {
EObject current = null;
EObject iv_ruleImport = null;
try {
// InternalContextMappingDSL.g:263:47: (iv_ruleImport= ruleImport EOF )
// InternalContextMappingDSL.g:264:2: iv_ruleImport= ruleImport EOF
{
newCompositeNode(grammarAccess.getImportRule());
pushFollow(FOLLOW_1);
iv_ruleImport=ruleImport();
state._fsp--;
current =iv_ruleImport;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleImport"
// $ANTLR start "ruleImport"
// InternalContextMappingDSL.g:270:1: ruleImport returns [EObject current=null] : (otherlv_0= 'import' ( (lv_importURI_1_0= RULE_STRING ) ) ) ;
public final EObject ruleImport() throws RecognitionException {
EObject current = null;
Token otherlv_0=null;
Token lv_importURI_1_0=null;
enterRule();
try {
// InternalContextMappingDSL.g:276:2: ( (otherlv_0= 'import' ( (lv_importURI_1_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:277:2: (otherlv_0= 'import' ( (lv_importURI_1_0= RULE_STRING ) ) )
{
// InternalContextMappingDSL.g:277:2: (otherlv_0= 'import' ( (lv_importURI_1_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:278:3: otherlv_0= 'import' ( (lv_importURI_1_0= RULE_STRING ) )
{
otherlv_0=(Token)match(input,18,FOLLOW_4);
newLeafNode(otherlv_0, grammarAccess.getImportAccess().getImportKeyword_0());
// InternalContextMappingDSL.g:282:3: ( (lv_importURI_1_0= RULE_STRING ) )
// InternalContextMappingDSL.g:283:4: (lv_importURI_1_0= RULE_STRING )
{
// InternalContextMappingDSL.g:283:4: (lv_importURI_1_0= RULE_STRING )
// InternalContextMappingDSL.g:284:5: lv_importURI_1_0= RULE_STRING
{
lv_importURI_1_0=(Token)match(input,RULE_STRING,FOLLOW_2);
newLeafNode(lv_importURI_1_0, grammarAccess.getImportAccess().getImportURISTRINGTerminalRuleCall_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getImportRule());
}
setWithLastConsumed(
current,
"importURI",
lv_importURI_1_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleImport"
// $ANTLR start "entryRuleContextMap"
// InternalContextMappingDSL.g:304:1: entryRuleContextMap returns [EObject current=null] : iv_ruleContextMap= ruleContextMap EOF ;
public final EObject entryRuleContextMap() throws RecognitionException {
EObject current = null;
EObject iv_ruleContextMap = null;
try {
// InternalContextMappingDSL.g:304:51: (iv_ruleContextMap= ruleContextMap EOF )
// InternalContextMappingDSL.g:305:2: iv_ruleContextMap= ruleContextMap EOF
{
newCompositeNode(grammarAccess.getContextMapRule());
pushFollow(FOLLOW_1);
iv_ruleContextMap=ruleContextMap();
state._fsp--;
current =iv_ruleContextMap;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleContextMap"
// $ANTLR start "ruleContextMap"
// InternalContextMappingDSL.g:311:1: ruleContextMap returns [EObject current=null] : ( () otherlv_1= 'ContextMap' ( (lv_name_2_0= RULE_ID ) )? this_OPEN_3= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) ) ) ) )* ) ) ) (otherlv_11= 'contains' ( (otherlv_12= RULE_ID ) ) (otherlv_13= ',' ( (otherlv_14= RULE_ID ) ) )* )* ( (lv_relationships_15_0= ruleRelationship ) )* this_CLOSE_16= RULE_CLOSE ) ;
public final EObject ruleContextMap() throws RecognitionException {
EObject current = null;
Token otherlv_1=null;
Token lv_name_2_0=null;
Token this_OPEN_3=null;
Token otherlv_5=null;
Token otherlv_6=null;
Token otherlv_8=null;
Token otherlv_9=null;
Token otherlv_11=null;
Token otherlv_12=null;
Token otherlv_13=null;
Token otherlv_14=null;
Token this_CLOSE_16=null;
Enumerator lv_type_7_0 = null;
Enumerator lv_state_10_0 = null;
EObject lv_relationships_15_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:317:2: ( ( () otherlv_1= 'ContextMap' ( (lv_name_2_0= RULE_ID ) )? this_OPEN_3= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) ) ) ) )* ) ) ) (otherlv_11= 'contains' ( (otherlv_12= RULE_ID ) ) (otherlv_13= ',' ( (otherlv_14= RULE_ID ) ) )* )* ( (lv_relationships_15_0= ruleRelationship ) )* this_CLOSE_16= RULE_CLOSE ) )
// InternalContextMappingDSL.g:318:2: ( () otherlv_1= 'ContextMap' ( (lv_name_2_0= RULE_ID ) )? this_OPEN_3= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) ) ) ) )* ) ) ) (otherlv_11= 'contains' ( (otherlv_12= RULE_ID ) ) (otherlv_13= ',' ( (otherlv_14= RULE_ID ) ) )* )* ( (lv_relationships_15_0= ruleRelationship ) )* this_CLOSE_16= RULE_CLOSE )
{
// InternalContextMappingDSL.g:318:2: ( () otherlv_1= 'ContextMap' ( (lv_name_2_0= RULE_ID ) )? this_OPEN_3= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) ) ) ) )* ) ) ) (otherlv_11= 'contains' ( (otherlv_12= RULE_ID ) ) (otherlv_13= ',' ( (otherlv_14= RULE_ID ) ) )* )* ( (lv_relationships_15_0= ruleRelationship ) )* this_CLOSE_16= RULE_CLOSE )
// InternalContextMappingDSL.g:319:3: () otherlv_1= 'ContextMap' ( (lv_name_2_0= RULE_ID ) )? this_OPEN_3= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) ) ) ) )* ) ) ) (otherlv_11= 'contains' ( (otherlv_12= RULE_ID ) ) (otherlv_13= ',' ( (otherlv_14= RULE_ID ) ) )* )* ( (lv_relationships_15_0= ruleRelationship ) )* this_CLOSE_16= RULE_CLOSE
{
// InternalContextMappingDSL.g:319:3: ()
// InternalContextMappingDSL.g:320:4:
{
current = forceCreateModelElement(
grammarAccess.getContextMapAccess().getContextMapAction_0(),
current);
}
otherlv_1=(Token)match(input,19,FOLLOW_5);
newLeafNode(otherlv_1, grammarAccess.getContextMapAccess().getContextMapKeyword_1());
// InternalContextMappingDSL.g:330:3: ( (lv_name_2_0= RULE_ID ) )?
int alt6=2;
int LA6_0 = input.LA(1);
if ( (LA6_0==RULE_ID) ) {
alt6=1;
}
switch (alt6) {
case 1 :
// InternalContextMappingDSL.g:331:4: (lv_name_2_0= RULE_ID )
{
// InternalContextMappingDSL.g:331:4: (lv_name_2_0= RULE_ID )
// InternalContextMappingDSL.g:332:5: lv_name_2_0= RULE_ID
{
lv_name_2_0=(Token)match(input,RULE_ID,FOLLOW_6);
newLeafNode(lv_name_2_0, grammarAccess.getContextMapAccess().getNameIDTerminalRuleCall_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getContextMapRule());
}
setWithLastConsumed(
current,
"name",
lv_name_2_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
break;
}
this_OPEN_3=(Token)match(input,RULE_OPEN,FOLLOW_7);
newLeafNode(this_OPEN_3, grammarAccess.getContextMapAccess().getOPENTerminalRuleCall_3());
// InternalContextMappingDSL.g:352:3: ( ( ( ( ({...}? => ( ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:353:4: ( ( ( ({...}? => ( ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:353:4: ( ( ( ({...}? => ( ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:354:5: ( ( ({...}? => ( ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getContextMapAccess().getUnorderedGroup_4());
// InternalContextMappingDSL.g:357:5: ( ( ({...}? => ( ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:358:6: ( ({...}? => ( ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:358:6: ( ({...}? => ( ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) ) ) ) )*
loop9:
do {
int alt9=3;
int LA9_0 = input.LA(1);
if ( LA9_0 == 20 && getUnorderedGroupHelper().canSelect(grammarAccess.getContextMapAccess().getUnorderedGroup_4(), 0) ) {
alt9=1;
}
else if ( LA9_0 == 22 && getUnorderedGroupHelper().canSelect(grammarAccess.getContextMapAccess().getUnorderedGroup_4(), 1) ) {
alt9=2;
}
switch (alt9) {
case 1 :
// InternalContextMappingDSL.g:359:4: ({...}? => ( ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) ) ) )
{
// InternalContextMappingDSL.g:359:4: ({...}? => ( ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) ) ) )
// InternalContextMappingDSL.g:360:5: {...}? => ( ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getContextMapAccess().getUnorderedGroup_4(), 0) ) {
throw new FailedPredicateException(input, "ruleContextMap", "getUnorderedGroupHelper().canSelect(grammarAccess.getContextMapAccess().getUnorderedGroup_4(), 0)");
}
// InternalContextMappingDSL.g:360:107: ( ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) ) )
// InternalContextMappingDSL.g:361:6: ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getContextMapAccess().getUnorderedGroup_4(), 0);
// InternalContextMappingDSL.g:364:9: ({...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) ) )
// InternalContextMappingDSL.g:364:10: {...}? => (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleContextMap", "true");
}
// InternalContextMappingDSL.g:364:19: (otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) ) )
// InternalContextMappingDSL.g:364:20: otherlv_5= 'type' (otherlv_6= '=' )? ( (lv_type_7_0= ruleContextMapType ) )
{
otherlv_5=(Token)match(input,20,FOLLOW_8);
newLeafNode(otherlv_5, grammarAccess.getContextMapAccess().getTypeKeyword_4_0_0());
// InternalContextMappingDSL.g:368:9: (otherlv_6= '=' )?
int alt7=2;
int LA7_0 = input.LA(1);
if ( (LA7_0==21) ) {
alt7=1;
}
switch (alt7) {
case 1 :
// InternalContextMappingDSL.g:369:10: otherlv_6= '='
{
otherlv_6=(Token)match(input,21,FOLLOW_8);
newLeafNode(otherlv_6, grammarAccess.getContextMapAccess().getEqualsSignKeyword_4_0_1());
}
break;
}
// InternalContextMappingDSL.g:374:9: ( (lv_type_7_0= ruleContextMapType ) )
// InternalContextMappingDSL.g:375:10: (lv_type_7_0= ruleContextMapType )
{
// InternalContextMappingDSL.g:375:10: (lv_type_7_0= ruleContextMapType )
// InternalContextMappingDSL.g:376:11: lv_type_7_0= ruleContextMapType
{
newCompositeNode(grammarAccess.getContextMapAccess().getTypeContextMapTypeEnumRuleCall_4_0_2_0());
pushFollow(FOLLOW_7);
lv_type_7_0=ruleContextMapType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getContextMapRule());
}
set(
current,
"type",
lv_type_7_0,
"org.contextmapper.dsl.ContextMappingDSL.ContextMapType");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getContextMapAccess().getUnorderedGroup_4());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:399:4: ({...}? => ( ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) ) ) )
{
// InternalContextMappingDSL.g:399:4: ({...}? => ( ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) ) ) )
// InternalContextMappingDSL.g:400:5: {...}? => ( ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getContextMapAccess().getUnorderedGroup_4(), 1) ) {
throw new FailedPredicateException(input, "ruleContextMap", "getUnorderedGroupHelper().canSelect(grammarAccess.getContextMapAccess().getUnorderedGroup_4(), 1)");
}
// InternalContextMappingDSL.g:400:107: ( ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) ) )
// InternalContextMappingDSL.g:401:6: ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getContextMapAccess().getUnorderedGroup_4(), 1);
// InternalContextMappingDSL.g:404:9: ({...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) ) )
// InternalContextMappingDSL.g:404:10: {...}? => (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleContextMap", "true");
}
// InternalContextMappingDSL.g:404:19: (otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) ) )
// InternalContextMappingDSL.g:404:20: otherlv_8= 'state' (otherlv_9= '=' )? ( (lv_state_10_0= ruleContextMapState ) )
{
otherlv_8=(Token)match(input,22,FOLLOW_9);
newLeafNode(otherlv_8, grammarAccess.getContextMapAccess().getStateKeyword_4_1_0());
// InternalContextMappingDSL.g:408:9: (otherlv_9= '=' )?
int alt8=2;
int LA8_0 = input.LA(1);
if ( (LA8_0==21) ) {
alt8=1;
}
switch (alt8) {
case 1 :
// InternalContextMappingDSL.g:409:10: otherlv_9= '='
{
otherlv_9=(Token)match(input,21,FOLLOW_9);
newLeafNode(otherlv_9, grammarAccess.getContextMapAccess().getEqualsSignKeyword_4_1_1());
}
break;
}
// InternalContextMappingDSL.g:414:9: ( (lv_state_10_0= ruleContextMapState ) )
// InternalContextMappingDSL.g:415:10: (lv_state_10_0= ruleContextMapState )
{
// InternalContextMappingDSL.g:415:10: (lv_state_10_0= ruleContextMapState )
// InternalContextMappingDSL.g:416:11: lv_state_10_0= ruleContextMapState
{
newCompositeNode(grammarAccess.getContextMapAccess().getStateContextMapStateEnumRuleCall_4_1_2_0());
pushFollow(FOLLOW_7);
lv_state_10_0=ruleContextMapState();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getContextMapRule());
}
set(
current,
"state",
lv_state_10_0,
"org.contextmapper.dsl.ContextMappingDSL.ContextMapState");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getContextMapAccess().getUnorderedGroup_4());
}
}
}
break;
default :
break loop9;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getContextMapAccess().getUnorderedGroup_4());
}
// InternalContextMappingDSL.g:446:3: (otherlv_11= 'contains' ( (otherlv_12= RULE_ID ) ) (otherlv_13= ',' ( (otherlv_14= RULE_ID ) ) )* )*
loop11:
do {
int alt11=2;
int LA11_0 = input.LA(1);
if ( (LA11_0==23) ) {
alt11=1;
}
switch (alt11) {
case 1 :
// InternalContextMappingDSL.g:447:4: otherlv_11= 'contains' ( (otherlv_12= RULE_ID ) ) (otherlv_13= ',' ( (otherlv_14= RULE_ID ) ) )*
{
otherlv_11=(Token)match(input,23,FOLLOW_10);
newLeafNode(otherlv_11, grammarAccess.getContextMapAccess().getContainsKeyword_5_0());
// InternalContextMappingDSL.g:451:4: ( (otherlv_12= RULE_ID ) )
// InternalContextMappingDSL.g:452:5: (otherlv_12= RULE_ID )
{
// InternalContextMappingDSL.g:452:5: (otherlv_12= RULE_ID )
// InternalContextMappingDSL.g:453:6: otherlv_12= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getContextMapRule());
}
otherlv_12=(Token)match(input,RULE_ID,FOLLOW_11);
newLeafNode(otherlv_12, grammarAccess.getContextMapAccess().getBoundedContextsBoundedContextCrossReference_5_1_0());
}
}
// InternalContextMappingDSL.g:464:4: (otherlv_13= ',' ( (otherlv_14= RULE_ID ) ) )*
loop10:
do {
int alt10=2;
int LA10_0 = input.LA(1);
if ( (LA10_0==24) ) {
alt10=1;
}
switch (alt10) {
case 1 :
// InternalContextMappingDSL.g:465:5: otherlv_13= ',' ( (otherlv_14= RULE_ID ) )
{
otherlv_13=(Token)match(input,24,FOLLOW_10);
newLeafNode(otherlv_13, grammarAccess.getContextMapAccess().getCommaKeyword_5_2_0());
// InternalContextMappingDSL.g:469:5: ( (otherlv_14= RULE_ID ) )
// InternalContextMappingDSL.g:470:6: (otherlv_14= RULE_ID )
{
// InternalContextMappingDSL.g:470:6: (otherlv_14= RULE_ID )
// InternalContextMappingDSL.g:471:7: otherlv_14= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getContextMapRule());
}
otherlv_14=(Token)match(input,RULE_ID,FOLLOW_11);
newLeafNode(otherlv_14, grammarAccess.getContextMapAccess().getBoundedContextsBoundedContextCrossReference_5_2_1_0());
}
}
}
break;
default :
break loop10;
}
} while (true);
}
break;
default :
break loop11;
}
} while (true);
// InternalContextMappingDSL.g:484:3: ( (lv_relationships_15_0= ruleRelationship ) )*
loop12:
do {
int alt12=2;
int LA12_0 = input.LA(1);
if ( (LA12_0==RULE_ID||LA12_0==35) ) {
alt12=1;
}
switch (alt12) {
case 1 :
// InternalContextMappingDSL.g:485:4: (lv_relationships_15_0= ruleRelationship )
{
// InternalContextMappingDSL.g:485:4: (lv_relationships_15_0= ruleRelationship )
// InternalContextMappingDSL.g:486:5: lv_relationships_15_0= ruleRelationship
{
newCompositeNode(grammarAccess.getContextMapAccess().getRelationshipsRelationshipParserRuleCall_6_0());
pushFollow(FOLLOW_12);
lv_relationships_15_0=ruleRelationship();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getContextMapRule());
}
add(
current,
"relationships",
lv_relationships_15_0,
"org.contextmapper.dsl.ContextMappingDSL.Relationship");
afterParserOrEnumRuleCall();
}
}
break;
default :
break loop12;
}
} while (true);
this_CLOSE_16=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(this_CLOSE_16, grammarAccess.getContextMapAccess().getCLOSETerminalRuleCall_7());
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleContextMap"
// $ANTLR start "entryRuleBoundedContext"
// InternalContextMappingDSL.g:511:1: entryRuleBoundedContext returns [EObject current=null] : iv_ruleBoundedContext= ruleBoundedContext EOF ;
public final EObject entryRuleBoundedContext() throws RecognitionException {
EObject current = null;
EObject iv_ruleBoundedContext = null;
try {
// InternalContextMappingDSL.g:511:55: (iv_ruleBoundedContext= ruleBoundedContext EOF )
// InternalContextMappingDSL.g:512:2: iv_ruleBoundedContext= ruleBoundedContext EOF
{
newCompositeNode(grammarAccess.getBoundedContextRule());
pushFollow(FOLLOW_1);
iv_ruleBoundedContext=ruleBoundedContext();
state._fsp--;
current =iv_ruleBoundedContext;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleBoundedContext"
// $ANTLR start "ruleBoundedContext"
// InternalContextMappingDSL.g:518:1: ruleBoundedContext returns [EObject current=null] : ( ( (lv_comment_0_0= RULE_SL_COMMENT ) )? otherlv_1= 'BoundedContext' ( (lv_name_2_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) ) ) ) )* ) ) ) (this_OPEN_14= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) ) ) )* ) ) ) ( (lv_modules_33_0= ruleSculptorModule ) )* ( (lv_aggregates_34_0= ruleAggregate ) )* this_CLOSE_35= RULE_CLOSE )? ) ;
public final EObject ruleBoundedContext() throws RecognitionException {
EObject current = null;
Token lv_comment_0_0=null;
Token otherlv_1=null;
Token lv_name_2_0=null;
Token otherlv_4=null;
Token otherlv_5=null;
Token otherlv_6=null;
Token otherlv_7=null;
Token otherlv_8=null;
Token otherlv_9=null;
Token otherlv_10=null;
Token otherlv_11=null;
Token otherlv_12=null;
Token otherlv_13=null;
Token this_OPEN_14=null;
Token otherlv_16=null;
Token otherlv_17=null;
Token lv_domainVisionStatement_18_0=null;
Token otherlv_19=null;
Token otherlv_20=null;
Token otherlv_22=null;
Token otherlv_23=null;
Token lv_responsibilities_24_0=null;
Token otherlv_25=null;
Token lv_responsibilities_26_0=null;
Token otherlv_27=null;
Token otherlv_28=null;
Token lv_implementationTechnology_29_0=null;
Token otherlv_30=null;
Token otherlv_31=null;
Token this_CLOSE_35=null;
Enumerator lv_type_21_0 = null;
Enumerator lv_knowledgeLevel_32_0 = null;
EObject lv_modules_33_0 = null;
EObject lv_aggregates_34_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:524:2: ( ( ( (lv_comment_0_0= RULE_SL_COMMENT ) )? otherlv_1= 'BoundedContext' ( (lv_name_2_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) ) ) ) )* ) ) ) (this_OPEN_14= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) ) ) )* ) ) ) ( (lv_modules_33_0= ruleSculptorModule ) )* ( (lv_aggregates_34_0= ruleAggregate ) )* this_CLOSE_35= RULE_CLOSE )? ) )
// InternalContextMappingDSL.g:525:2: ( ( (lv_comment_0_0= RULE_SL_COMMENT ) )? otherlv_1= 'BoundedContext' ( (lv_name_2_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) ) ) ) )* ) ) ) (this_OPEN_14= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) ) ) )* ) ) ) ( (lv_modules_33_0= ruleSculptorModule ) )* ( (lv_aggregates_34_0= ruleAggregate ) )* this_CLOSE_35= RULE_CLOSE )? )
{
// InternalContextMappingDSL.g:525:2: ( ( (lv_comment_0_0= RULE_SL_COMMENT ) )? otherlv_1= 'BoundedContext' ( (lv_name_2_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) ) ) ) )* ) ) ) (this_OPEN_14= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) ) ) )* ) ) ) ( (lv_modules_33_0= ruleSculptorModule ) )* ( (lv_aggregates_34_0= ruleAggregate ) )* this_CLOSE_35= RULE_CLOSE )? )
// InternalContextMappingDSL.g:526:3: ( (lv_comment_0_0= RULE_SL_COMMENT ) )? otherlv_1= 'BoundedContext' ( (lv_name_2_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) ) ) ) )* ) ) ) (this_OPEN_14= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) ) ) )* ) ) ) ( (lv_modules_33_0= ruleSculptorModule ) )* ( (lv_aggregates_34_0= ruleAggregate ) )* this_CLOSE_35= RULE_CLOSE )?
{
// InternalContextMappingDSL.g:526:3: ( (lv_comment_0_0= RULE_SL_COMMENT ) )?
int alt13=2;
int LA13_0 = input.LA(1);
if ( (LA13_0==RULE_SL_COMMENT) ) {
alt13=1;
}
switch (alt13) {
case 1 :
// InternalContextMappingDSL.g:527:4: (lv_comment_0_0= RULE_SL_COMMENT )
{
// InternalContextMappingDSL.g:527:4: (lv_comment_0_0= RULE_SL_COMMENT )
// InternalContextMappingDSL.g:528:5: lv_comment_0_0= RULE_SL_COMMENT
{
lv_comment_0_0=(Token)match(input,RULE_SL_COMMENT,FOLLOW_13);
newLeafNode(lv_comment_0_0, grammarAccess.getBoundedContextAccess().getCommentSL_COMMENTTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getBoundedContextRule());
}
setWithLastConsumed(
current,
"comment",
lv_comment_0_0,
"org.eclipse.xtext.common.Terminals.SL_COMMENT");
}
}
break;
}
otherlv_1=(Token)match(input,25,FOLLOW_10);
newLeafNode(otherlv_1, grammarAccess.getBoundedContextAccess().getBoundedContextKeyword_1());
// InternalContextMappingDSL.g:548:3: ( (lv_name_2_0= RULE_ID ) )
// InternalContextMappingDSL.g:549:4: (lv_name_2_0= RULE_ID )
{
// InternalContextMappingDSL.g:549:4: (lv_name_2_0= RULE_ID )
// InternalContextMappingDSL.g:550:5: lv_name_2_0= RULE_ID
{
lv_name_2_0=(Token)match(input,RULE_ID,FOLLOW_14);
newLeafNode(lv_name_2_0, grammarAccess.getBoundedContextAccess().getNameIDTerminalRuleCall_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getBoundedContextRule());
}
setWithLastConsumed(
current,
"name",
lv_name_2_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:566:3: ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:567:4: ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:567:4: ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:568:5: ( ( ({...}? => ( ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getBoundedContextAccess().getUnorderedGroup_3());
// InternalContextMappingDSL.g:571:5: ( ( ({...}? => ( ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:572:6: ( ({...}? => ( ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:572:6: ( ({...}? => ( ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) ) ) ) )*
loop16:
do {
int alt16=4;
int LA16_0 = input.LA(1);
if ( LA16_0 == 26 && getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_3(), 0) ) {
alt16=1;
}
else if ( LA16_0 == 27 && getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_3(), 1) ) {
alt16=2;
}
else if ( LA16_0 == 28 && getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_3(), 2) ) {
alt16=3;
}
switch (alt16) {
case 1 :
// InternalContextMappingDSL.g:573:4: ({...}? => ( ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) ) ) )
{
// InternalContextMappingDSL.g:573:4: ({...}? => ( ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) ) ) )
// InternalContextMappingDSL.g:574:5: {...}? => ( ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_3(), 0) ) {
throw new FailedPredicateException(input, "ruleBoundedContext", "getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_3(), 0)");
}
// InternalContextMappingDSL.g:574:111: ( ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) ) )
// InternalContextMappingDSL.g:575:6: ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) )
{
getUnorderedGroupHelper().select(grammarAccess.getBoundedContextAccess().getUnorderedGroup_3(), 0);
// InternalContextMappingDSL.g:578:9: ({...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* ) )
// InternalContextMappingDSL.g:578:10: {...}? => (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleBoundedContext", "true");
}
// InternalContextMappingDSL.g:578:19: (otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )* )
// InternalContextMappingDSL.g:578:20: otherlv_4= 'implements' ( (otherlv_5= RULE_ID ) ) (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )*
{
otherlv_4=(Token)match(input,26,FOLLOW_10);
newLeafNode(otherlv_4, grammarAccess.getBoundedContextAccess().getImplementsKeyword_3_0_0());
// InternalContextMappingDSL.g:582:9: ( (otherlv_5= RULE_ID ) )
// InternalContextMappingDSL.g:583:10: (otherlv_5= RULE_ID )
{
// InternalContextMappingDSL.g:583:10: (otherlv_5= RULE_ID )
// InternalContextMappingDSL.g:584:11: otherlv_5= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getBoundedContextRule());
}
otherlv_5=(Token)match(input,RULE_ID,FOLLOW_15);
newLeafNode(otherlv_5, grammarAccess.getBoundedContextAccess().getImplementedDomainPartsDomainPartCrossReference_3_0_1_0());
}
}
// InternalContextMappingDSL.g:595:9: (otherlv_6= ',' ( (otherlv_7= RULE_ID ) ) )*
loop14:
do {
int alt14=2;
int LA14_0 = input.LA(1);
if ( (LA14_0==24) ) {
alt14=1;
}
switch (alt14) {
case 1 :
// InternalContextMappingDSL.g:596:10: otherlv_6= ',' ( (otherlv_7= RULE_ID ) )
{
otherlv_6=(Token)match(input,24,FOLLOW_10);
newLeafNode(otherlv_6, grammarAccess.getBoundedContextAccess().getCommaKeyword_3_0_2_0());
// InternalContextMappingDSL.g:600:10: ( (otherlv_7= RULE_ID ) )
// InternalContextMappingDSL.g:601:11: (otherlv_7= RULE_ID )
{
// InternalContextMappingDSL.g:601:11: (otherlv_7= RULE_ID )
// InternalContextMappingDSL.g:602:12: otherlv_7= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getBoundedContextRule());
}
otherlv_7=(Token)match(input,RULE_ID,FOLLOW_15);
newLeafNode(otherlv_7, grammarAccess.getBoundedContextAccess().getImplementedDomainPartsDomainPartCrossReference_3_0_2_1_0());
}
}
}
break;
default :
break loop14;
}
} while (true);
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getBoundedContextAccess().getUnorderedGroup_3());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:620:4: ({...}? => ( ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) ) ) )
{
// InternalContextMappingDSL.g:620:4: ({...}? => ( ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) ) ) )
// InternalContextMappingDSL.g:621:5: {...}? => ( ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_3(), 1) ) {
throw new FailedPredicateException(input, "ruleBoundedContext", "getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_3(), 1)");
}
// InternalContextMappingDSL.g:621:111: ( ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) ) )
// InternalContextMappingDSL.g:622:6: ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) )
{
getUnorderedGroupHelper().select(grammarAccess.getBoundedContextAccess().getUnorderedGroup_3(), 1);
// InternalContextMappingDSL.g:625:9: ({...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* ) )
// InternalContextMappingDSL.g:625:10: {...}? => (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleBoundedContext", "true");
}
// InternalContextMappingDSL.g:625:19: (otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )* )
// InternalContextMappingDSL.g:625:20: otherlv_8= 'realizes' ( (otherlv_9= RULE_ID ) ) (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )*
{
otherlv_8=(Token)match(input,27,FOLLOW_10);
newLeafNode(otherlv_8, grammarAccess.getBoundedContextAccess().getRealizesKeyword_3_1_0());
// InternalContextMappingDSL.g:629:9: ( (otherlv_9= RULE_ID ) )
// InternalContextMappingDSL.g:630:10: (otherlv_9= RULE_ID )
{
// InternalContextMappingDSL.g:630:10: (otherlv_9= RULE_ID )
// InternalContextMappingDSL.g:631:11: otherlv_9= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getBoundedContextRule());
}
otherlv_9=(Token)match(input,RULE_ID,FOLLOW_15);
newLeafNode(otherlv_9, grammarAccess.getBoundedContextAccess().getRealizedBoundedContextsBoundedContextCrossReference_3_1_1_0());
}
}
// InternalContextMappingDSL.g:642:9: (otherlv_10= ',' ( (otherlv_11= RULE_ID ) ) )*
loop15:
do {
int alt15=2;
int LA15_0 = input.LA(1);
if ( (LA15_0==24) ) {
alt15=1;
}
switch (alt15) {
case 1 :
// InternalContextMappingDSL.g:643:10: otherlv_10= ',' ( (otherlv_11= RULE_ID ) )
{
otherlv_10=(Token)match(input,24,FOLLOW_10);
newLeafNode(otherlv_10, grammarAccess.getBoundedContextAccess().getCommaKeyword_3_1_2_0());
// InternalContextMappingDSL.g:647:10: ( (otherlv_11= RULE_ID ) )
// InternalContextMappingDSL.g:648:11: (otherlv_11= RULE_ID )
{
// InternalContextMappingDSL.g:648:11: (otherlv_11= RULE_ID )
// InternalContextMappingDSL.g:649:12: otherlv_11= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getBoundedContextRule());
}
otherlv_11=(Token)match(input,RULE_ID,FOLLOW_15);
newLeafNode(otherlv_11, grammarAccess.getBoundedContextAccess().getRealizedBoundedContextsBoundedContextCrossReference_3_1_2_1_0());
}
}
}
break;
default :
break loop15;
}
} while (true);
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getBoundedContextAccess().getUnorderedGroup_3());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:667:4: ({...}? => ( ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) ) ) )
{
// InternalContextMappingDSL.g:667:4: ({...}? => ( ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) ) ) )
// InternalContextMappingDSL.g:668:5: {...}? => ( ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_3(), 2) ) {
throw new FailedPredicateException(input, "ruleBoundedContext", "getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_3(), 2)");
}
// InternalContextMappingDSL.g:668:111: ( ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) ) )
// InternalContextMappingDSL.g:669:6: ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getBoundedContextAccess().getUnorderedGroup_3(), 2);
// InternalContextMappingDSL.g:672:9: ({...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) ) )
// InternalContextMappingDSL.g:672:10: {...}? => (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleBoundedContext", "true");
}
// InternalContextMappingDSL.g:672:19: (otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) ) )
// InternalContextMappingDSL.g:672:20: otherlv_12= 'refines' ( (otherlv_13= RULE_ID ) )
{
otherlv_12=(Token)match(input,28,FOLLOW_10);
newLeafNode(otherlv_12, grammarAccess.getBoundedContextAccess().getRefinesKeyword_3_2_0());
// InternalContextMappingDSL.g:676:9: ( (otherlv_13= RULE_ID ) )
// InternalContextMappingDSL.g:677:10: (otherlv_13= RULE_ID )
{
// InternalContextMappingDSL.g:677:10: (otherlv_13= RULE_ID )
// InternalContextMappingDSL.g:678:11: otherlv_13= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getBoundedContextRule());
}
otherlv_13=(Token)match(input,RULE_ID,FOLLOW_14);
newLeafNode(otherlv_13, grammarAccess.getBoundedContextAccess().getRefinedBoundedContextBoundedContextCrossReference_3_2_1_0());
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getBoundedContextAccess().getUnorderedGroup_3());
}
}
}
break;
default :
break loop16;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getBoundedContextAccess().getUnorderedGroup_3());
}
// InternalContextMappingDSL.g:702:3: (this_OPEN_14= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) ) ) )* ) ) ) ( (lv_modules_33_0= ruleSculptorModule ) )* ( (lv_aggregates_34_0= ruleAggregate ) )* this_CLOSE_35= RULE_CLOSE )?
int alt26=2;
int LA26_0 = input.LA(1);
if ( (LA26_0==RULE_OPEN) ) {
alt26=1;
}
switch (alt26) {
case 1 :
// InternalContextMappingDSL.g:703:4: this_OPEN_14= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) ) ) )* ) ) ) ( (lv_modules_33_0= ruleSculptorModule ) )* ( (lv_aggregates_34_0= ruleAggregate ) )* this_CLOSE_35= RULE_CLOSE
{
this_OPEN_14=(Token)match(input,RULE_OPEN,FOLLOW_16);
newLeafNode(this_OPEN_14, grammarAccess.getBoundedContextAccess().getOPENTerminalRuleCall_4_0());
// InternalContextMappingDSL.g:707:4: ( ( ( ( ({...}? => ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:708:5: ( ( ( ({...}? => ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:708:5: ( ( ( ({...}? => ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:709:6: ( ( ({...}? => ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1());
// InternalContextMappingDSL.g:712:6: ( ( ({...}? => ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:713:7: ( ({...}? => ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:713:7: ( ({...}? => ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) ) ) )*
loop23:
do {
int alt23=6;
int LA23_0 = input.LA(1);
if ( LA23_0 == 29 && getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 0) ) {
alt23=1;
}
else if ( LA23_0 == 20 && getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 1) ) {
alt23=2;
}
else if ( LA23_0 == 30 && getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 2) ) {
alt23=3;
}
else if ( LA23_0 == 31 && getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 3) ) {
alt23=4;
}
else if ( LA23_0 == 32 && getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 4) ) {
alt23=5;
}
switch (alt23) {
case 1 :
// InternalContextMappingDSL.g:714:5: ({...}? => ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:714:5: ({...}? => ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:715:6: {...}? => ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 0) ) {
throw new FailedPredicateException(input, "ruleBoundedContext", "getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 0)");
}
// InternalContextMappingDSL.g:715:114: ( ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:716:7: ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 0);
// InternalContextMappingDSL.g:719:10: ({...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:719:11: {...}? => (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleBoundedContext", "true");
}
// InternalContextMappingDSL.g:719:20: (otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:719:21: otherlv_16= 'domainVisionStatement' (otherlv_17= '=' )? ( (lv_domainVisionStatement_18_0= RULE_STRING ) )
{
otherlv_16=(Token)match(input,29,FOLLOW_17);
newLeafNode(otherlv_16, grammarAccess.getBoundedContextAccess().getDomainVisionStatementKeyword_4_1_0_0());
// InternalContextMappingDSL.g:723:10: (otherlv_17= '=' )?
int alt17=2;
int LA17_0 = input.LA(1);
if ( (LA17_0==21) ) {
alt17=1;
}
switch (alt17) {
case 1 :
// InternalContextMappingDSL.g:724:11: otherlv_17= '='
{
otherlv_17=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_17, grammarAccess.getBoundedContextAccess().getEqualsSignKeyword_4_1_0_1());
}
break;
}
// InternalContextMappingDSL.g:729:10: ( (lv_domainVisionStatement_18_0= RULE_STRING ) )
// InternalContextMappingDSL.g:730:11: (lv_domainVisionStatement_18_0= RULE_STRING )
{
// InternalContextMappingDSL.g:730:11: (lv_domainVisionStatement_18_0= RULE_STRING )
// InternalContextMappingDSL.g:731:12: lv_domainVisionStatement_18_0= RULE_STRING
{
lv_domainVisionStatement_18_0=(Token)match(input,RULE_STRING,FOLLOW_16);
newLeafNode(lv_domainVisionStatement_18_0, grammarAccess.getBoundedContextAccess().getDomainVisionStatementSTRINGTerminalRuleCall_4_1_0_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getBoundedContextRule());
}
setWithLastConsumed(
current,
"domainVisionStatement",
lv_domainVisionStatement_18_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:753:5: ({...}? => ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) ) )
{
// InternalContextMappingDSL.g:753:5: ({...}? => ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) ) )
// InternalContextMappingDSL.g:754:6: {...}? => ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 1) ) {
throw new FailedPredicateException(input, "ruleBoundedContext", "getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 1)");
}
// InternalContextMappingDSL.g:754:114: ( ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) ) )
// InternalContextMappingDSL.g:755:7: ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 1);
// InternalContextMappingDSL.g:758:10: ({...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) ) )
// InternalContextMappingDSL.g:758:11: {...}? => (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleBoundedContext", "true");
}
// InternalContextMappingDSL.g:758:20: (otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) ) )
// InternalContextMappingDSL.g:758:21: otherlv_19= 'type' (otherlv_20= '=' )? ( (lv_type_21_0= ruleBoundedContextType ) )
{
otherlv_19=(Token)match(input,20,FOLLOW_18);
newLeafNode(otherlv_19, grammarAccess.getBoundedContextAccess().getTypeKeyword_4_1_1_0());
// InternalContextMappingDSL.g:762:10: (otherlv_20= '=' )?
int alt18=2;
int LA18_0 = input.LA(1);
if ( (LA18_0==21) ) {
alt18=1;
}
switch (alt18) {
case 1 :
// InternalContextMappingDSL.g:763:11: otherlv_20= '='
{
otherlv_20=(Token)match(input,21,FOLLOW_18);
newLeafNode(otherlv_20, grammarAccess.getBoundedContextAccess().getEqualsSignKeyword_4_1_1_1());
}
break;
}
// InternalContextMappingDSL.g:768:10: ( (lv_type_21_0= ruleBoundedContextType ) )
// InternalContextMappingDSL.g:769:11: (lv_type_21_0= ruleBoundedContextType )
{
// InternalContextMappingDSL.g:769:11: (lv_type_21_0= ruleBoundedContextType )
// InternalContextMappingDSL.g:770:12: lv_type_21_0= ruleBoundedContextType
{
newCompositeNode(grammarAccess.getBoundedContextAccess().getTypeBoundedContextTypeEnumRuleCall_4_1_1_2_0());
pushFollow(FOLLOW_16);
lv_type_21_0=ruleBoundedContextType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getBoundedContextRule());
}
set(
current,
"type",
lv_type_21_0,
"org.contextmapper.dsl.ContextMappingDSL.BoundedContextType");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:793:5: ({...}? => ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) ) )
{
// InternalContextMappingDSL.g:793:5: ({...}? => ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) ) )
// InternalContextMappingDSL.g:794:6: {...}? => ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 2) ) {
throw new FailedPredicateException(input, "ruleBoundedContext", "getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 2)");
}
// InternalContextMappingDSL.g:794:114: ( ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) ) )
// InternalContextMappingDSL.g:795:7: ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) )
{
getUnorderedGroupHelper().select(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 2);
// InternalContextMappingDSL.g:798:10: ({...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* ) )
// InternalContextMappingDSL.g:798:11: {...}? => ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleBoundedContext", "true");
}
// InternalContextMappingDSL.g:798:20: ( (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )* )
// InternalContextMappingDSL.g:798:21: (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) ) (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )*
{
// InternalContextMappingDSL.g:798:21: (otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:799:11: otherlv_22= 'responsibilities' (otherlv_23= '=' )? ( (lv_responsibilities_24_0= RULE_STRING ) )
{
otherlv_22=(Token)match(input,30,FOLLOW_17);
newLeafNode(otherlv_22, grammarAccess.getBoundedContextAccess().getResponsibilitiesKeyword_4_1_2_0_0());
// InternalContextMappingDSL.g:803:11: (otherlv_23= '=' )?
int alt19=2;
int LA19_0 = input.LA(1);
if ( (LA19_0==21) ) {
alt19=1;
}
switch (alt19) {
case 1 :
// InternalContextMappingDSL.g:804:12: otherlv_23= '='
{
otherlv_23=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_23, grammarAccess.getBoundedContextAccess().getEqualsSignKeyword_4_1_2_0_1());
}
break;
}
// InternalContextMappingDSL.g:809:11: ( (lv_responsibilities_24_0= RULE_STRING ) )
// InternalContextMappingDSL.g:810:12: (lv_responsibilities_24_0= RULE_STRING )
{
// InternalContextMappingDSL.g:810:12: (lv_responsibilities_24_0= RULE_STRING )
// InternalContextMappingDSL.g:811:13: lv_responsibilities_24_0= RULE_STRING
{
lv_responsibilities_24_0=(Token)match(input,RULE_STRING,FOLLOW_19);
newLeafNode(lv_responsibilities_24_0, grammarAccess.getBoundedContextAccess().getResponsibilitiesSTRINGTerminalRuleCall_4_1_2_0_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getBoundedContextRule());
}
addWithLastConsumed(
current,
"responsibilities",
lv_responsibilities_24_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
// InternalContextMappingDSL.g:828:10: (otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) ) )*
loop20:
do {
int alt20=2;
int LA20_0 = input.LA(1);
if ( (LA20_0==24) ) {
alt20=1;
}
switch (alt20) {
case 1 :
// InternalContextMappingDSL.g:829:11: otherlv_25= ',' ( (lv_responsibilities_26_0= RULE_STRING ) )
{
otherlv_25=(Token)match(input,24,FOLLOW_4);
newLeafNode(otherlv_25, grammarAccess.getBoundedContextAccess().getCommaKeyword_4_1_2_1_0());
// InternalContextMappingDSL.g:833:11: ( (lv_responsibilities_26_0= RULE_STRING ) )
// InternalContextMappingDSL.g:834:12: (lv_responsibilities_26_0= RULE_STRING )
{
// InternalContextMappingDSL.g:834:12: (lv_responsibilities_26_0= RULE_STRING )
// InternalContextMappingDSL.g:835:13: lv_responsibilities_26_0= RULE_STRING
{
lv_responsibilities_26_0=(Token)match(input,RULE_STRING,FOLLOW_19);
newLeafNode(lv_responsibilities_26_0, grammarAccess.getBoundedContextAccess().getResponsibilitiesSTRINGTerminalRuleCall_4_1_2_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getBoundedContextRule());
}
addWithLastConsumed(
current,
"responsibilities",
lv_responsibilities_26_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
default :
break loop20;
}
} while (true);
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1());
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:858:5: ({...}? => ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:858:5: ({...}? => ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:859:6: {...}? => ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 3) ) {
throw new FailedPredicateException(input, "ruleBoundedContext", "getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 3)");
}
// InternalContextMappingDSL.g:859:114: ( ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:860:7: ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 3);
// InternalContextMappingDSL.g:863:10: ({...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:863:11: {...}? => (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleBoundedContext", "true");
}
// InternalContextMappingDSL.g:863:20: (otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:863:21: otherlv_27= 'implementationTechnology' (otherlv_28= '=' )? ( (lv_implementationTechnology_29_0= RULE_STRING ) )
{
otherlv_27=(Token)match(input,31,FOLLOW_17);
newLeafNode(otherlv_27, grammarAccess.getBoundedContextAccess().getImplementationTechnologyKeyword_4_1_3_0());
// InternalContextMappingDSL.g:867:10: (otherlv_28= '=' )?
int alt21=2;
int LA21_0 = input.LA(1);
if ( (LA21_0==21) ) {
alt21=1;
}
switch (alt21) {
case 1 :
// InternalContextMappingDSL.g:868:11: otherlv_28= '='
{
otherlv_28=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_28, grammarAccess.getBoundedContextAccess().getEqualsSignKeyword_4_1_3_1());
}
break;
}
// InternalContextMappingDSL.g:873:10: ( (lv_implementationTechnology_29_0= RULE_STRING ) )
// InternalContextMappingDSL.g:874:11: (lv_implementationTechnology_29_0= RULE_STRING )
{
// InternalContextMappingDSL.g:874:11: (lv_implementationTechnology_29_0= RULE_STRING )
// InternalContextMappingDSL.g:875:12: lv_implementationTechnology_29_0= RULE_STRING
{
lv_implementationTechnology_29_0=(Token)match(input,RULE_STRING,FOLLOW_16);
newLeafNode(lv_implementationTechnology_29_0, grammarAccess.getBoundedContextAccess().getImplementationTechnologySTRINGTerminalRuleCall_4_1_3_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getBoundedContextRule());
}
setWithLastConsumed(
current,
"implementationTechnology",
lv_implementationTechnology_29_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1());
}
}
}
break;
case 5 :
// InternalContextMappingDSL.g:897:5: ({...}? => ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) ) )
{
// InternalContextMappingDSL.g:897:5: ({...}? => ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) ) )
// InternalContextMappingDSL.g:898:6: {...}? => ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 4) ) {
throw new FailedPredicateException(input, "ruleBoundedContext", "getUnorderedGroupHelper().canSelect(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 4)");
}
// InternalContextMappingDSL.g:898:114: ( ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) ) )
// InternalContextMappingDSL.g:899:7: ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1(), 4);
// InternalContextMappingDSL.g:902:10: ({...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) ) )
// InternalContextMappingDSL.g:902:11: {...}? => (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleBoundedContext", "true");
}
// InternalContextMappingDSL.g:902:20: (otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) ) )
// InternalContextMappingDSL.g:902:21: otherlv_30= 'knowledgeLevel' (otherlv_31= '=' )? ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) )
{
otherlv_30=(Token)match(input,32,FOLLOW_20);
newLeafNode(otherlv_30, grammarAccess.getBoundedContextAccess().getKnowledgeLevelKeyword_4_1_4_0());
// InternalContextMappingDSL.g:906:10: (otherlv_31= '=' )?
int alt22=2;
int LA22_0 = input.LA(1);
if ( (LA22_0==21) ) {
alt22=1;
}
switch (alt22) {
case 1 :
// InternalContextMappingDSL.g:907:11: otherlv_31= '='
{
otherlv_31=(Token)match(input,21,FOLLOW_20);
newLeafNode(otherlv_31, grammarAccess.getBoundedContextAccess().getEqualsSignKeyword_4_1_4_1());
}
break;
}
// InternalContextMappingDSL.g:912:10: ( (lv_knowledgeLevel_32_0= ruleKnowledgeLevel ) )
// InternalContextMappingDSL.g:913:11: (lv_knowledgeLevel_32_0= ruleKnowledgeLevel )
{
// InternalContextMappingDSL.g:913:11: (lv_knowledgeLevel_32_0= ruleKnowledgeLevel )
// InternalContextMappingDSL.g:914:12: lv_knowledgeLevel_32_0= ruleKnowledgeLevel
{
newCompositeNode(grammarAccess.getBoundedContextAccess().getKnowledgeLevelKnowledgeLevelEnumRuleCall_4_1_4_2_0());
pushFollow(FOLLOW_16);
lv_knowledgeLevel_32_0=ruleKnowledgeLevel();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getBoundedContextRule());
}
set(
current,
"knowledgeLevel",
lv_knowledgeLevel_32_0,
"org.contextmapper.dsl.ContextMappingDSL.KnowledgeLevel");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1());
}
}
}
break;
default :
break loop23;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getBoundedContextAccess().getUnorderedGroup_4_1());
}
// InternalContextMappingDSL.g:944:4: ( (lv_modules_33_0= ruleSculptorModule ) )*
loop24:
do {
int alt24=2;
int LA24_0 = input.LA(1);
if ( (LA24_0==RULE_STRING) ) {
int LA24_2 = input.LA(2);
if ( (LA24_2==79) ) {
alt24=1;
}
}
else if ( (LA24_0==79) ) {
alt24=1;
}
switch (alt24) {
case 1 :
// InternalContextMappingDSL.g:945:5: (lv_modules_33_0= ruleSculptorModule )
{
// InternalContextMappingDSL.g:945:5: (lv_modules_33_0= ruleSculptorModule )
// InternalContextMappingDSL.g:946:6: lv_modules_33_0= ruleSculptorModule
{
newCompositeNode(grammarAccess.getBoundedContextAccess().getModulesSculptorModuleParserRuleCall_4_2_0());
pushFollow(FOLLOW_21);
lv_modules_33_0=ruleSculptorModule();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getBoundedContextRule());
}
add(
current,
"modules",
lv_modules_33_0,
"org.contextmapper.dsl.ContextMappingDSL.SculptorModule");
afterParserOrEnumRuleCall();
}
}
break;
default :
break loop24;
}
} while (true);
// InternalContextMappingDSL.g:963:4: ( (lv_aggregates_34_0= ruleAggregate ) )*
loop25:
do {
int alt25=2;
int LA25_0 = input.LA(1);
if ( (LA25_0==RULE_STRING||LA25_0==RULE_ML_COMMENT||LA25_0==55) ) {
alt25=1;
}
switch (alt25) {
case 1 :
// InternalContextMappingDSL.g:964:5: (lv_aggregates_34_0= ruleAggregate )
{
// InternalContextMappingDSL.g:964:5: (lv_aggregates_34_0= ruleAggregate )
// InternalContextMappingDSL.g:965:6: lv_aggregates_34_0= ruleAggregate
{
newCompositeNode(grammarAccess.getBoundedContextAccess().getAggregatesAggregateParserRuleCall_4_3_0());
pushFollow(FOLLOW_22);
lv_aggregates_34_0=ruleAggregate();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getBoundedContextRule());
}
add(
current,
"aggregates",
lv_aggregates_34_0,
"org.contextmapper.dsl.ContextMappingDSL.Aggregate");
afterParserOrEnumRuleCall();
}
}
break;
default :
break loop25;
}
} while (true);
this_CLOSE_35=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(this_CLOSE_35, grammarAccess.getBoundedContextAccess().getCLOSETerminalRuleCall_4_4());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleBoundedContext"
// $ANTLR start "entryRuleDomain"
// InternalContextMappingDSL.g:991:1: entryRuleDomain returns [EObject current=null] : iv_ruleDomain= ruleDomain EOF ;
public final EObject entryRuleDomain() throws RecognitionException {
EObject current = null;
EObject iv_ruleDomain = null;
try {
// InternalContextMappingDSL.g:991:47: (iv_ruleDomain= ruleDomain EOF )
// InternalContextMappingDSL.g:992:2: iv_ruleDomain= ruleDomain EOF
{
newCompositeNode(grammarAccess.getDomainRule());
pushFollow(FOLLOW_1);
iv_ruleDomain=ruleDomain();
state._fsp--;
current =iv_ruleDomain;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleDomain"
// $ANTLR start "ruleDomain"
// InternalContextMappingDSL.g:998:1: ruleDomain returns [EObject current=null] : (otherlv_0= 'Domain' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ ) ) )* ) ) ) this_CLOSE_8= RULE_CLOSE )? ) ;
public final EObject ruleDomain() throws RecognitionException {
EObject current = null;
Token otherlv_0=null;
Token lv_name_1_0=null;
Token this_OPEN_2=null;
Token otherlv_4=null;
Token otherlv_5=null;
Token lv_domainVisionStatement_6_0=null;
Token this_CLOSE_8=null;
EObject lv_subdomains_7_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:1004:2: ( (otherlv_0= 'Domain' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ ) ) )* ) ) ) this_CLOSE_8= RULE_CLOSE )? ) )
// InternalContextMappingDSL.g:1005:2: (otherlv_0= 'Domain' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ ) ) )* ) ) ) this_CLOSE_8= RULE_CLOSE )? )
{
// InternalContextMappingDSL.g:1005:2: (otherlv_0= 'Domain' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ ) ) )* ) ) ) this_CLOSE_8= RULE_CLOSE )? )
// InternalContextMappingDSL.g:1006:3: otherlv_0= 'Domain' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ ) ) )* ) ) ) this_CLOSE_8= RULE_CLOSE )?
{
otherlv_0=(Token)match(input,33,FOLLOW_10);
newLeafNode(otherlv_0, grammarAccess.getDomainAccess().getDomainKeyword_0());
// InternalContextMappingDSL.g:1010:3: ( (lv_name_1_0= RULE_ID ) )
// InternalContextMappingDSL.g:1011:4: (lv_name_1_0= RULE_ID )
{
// InternalContextMappingDSL.g:1011:4: (lv_name_1_0= RULE_ID )
// InternalContextMappingDSL.g:1012:5: lv_name_1_0= RULE_ID
{
lv_name_1_0=(Token)match(input,RULE_ID,FOLLOW_23);
newLeafNode(lv_name_1_0, grammarAccess.getDomainAccess().getNameIDTerminalRuleCall_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainRule());
}
setWithLastConsumed(
current,
"name",
lv_name_1_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:1028:3: (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ ) ) )* ) ) ) this_CLOSE_8= RULE_CLOSE )?
int alt30=2;
int LA30_0 = input.LA(1);
if ( (LA30_0==RULE_OPEN) ) {
alt30=1;
}
switch (alt30) {
case 1 :
// InternalContextMappingDSL.g:1029:4: this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ ) ) )* ) ) ) this_CLOSE_8= RULE_CLOSE
{
this_OPEN_2=(Token)match(input,RULE_OPEN,FOLLOW_24);
newLeafNode(this_OPEN_2, grammarAccess.getDomainAccess().getOPENTerminalRuleCall_2_0());
// InternalContextMappingDSL.g:1033:4: ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ ) ) )* ) ) )
// InternalContextMappingDSL.g:1034:5: ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ ) ) )* ) )
{
// InternalContextMappingDSL.g:1034:5: ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ ) ) )* ) )
// InternalContextMappingDSL.g:1035:6: ( ( ({...}? => ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getDomainAccess().getUnorderedGroup_2_1());
// InternalContextMappingDSL.g:1038:6: ( ( ({...}? => ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ ) ) )* )
// InternalContextMappingDSL.g:1039:7: ( ({...}? => ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ ) ) )*
{
// InternalContextMappingDSL.g:1039:7: ( ({...}? => ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ ) ) )*
loop29:
do {
int alt29=3;
int LA29_0 = input.LA(1);
if ( LA29_0 == 29 && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainAccess().getUnorderedGroup_2_1(), 0) ) {
alt29=1;
}
else if ( LA29_0 == 34 && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainAccess().getUnorderedGroup_2_1(), 1) ) {
alt29=2;
}
switch (alt29) {
case 1 :
// InternalContextMappingDSL.g:1040:5: ({...}? => ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:1040:5: ({...}? => ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:1041:6: {...}? => ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainAccess().getUnorderedGroup_2_1(), 0) ) {
throw new FailedPredicateException(input, "ruleDomain", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainAccess().getUnorderedGroup_2_1(), 0)");
}
// InternalContextMappingDSL.g:1041:106: ( ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:1042:7: ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDomainAccess().getUnorderedGroup_2_1(), 0);
// InternalContextMappingDSL.g:1045:10: ({...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:1045:11: {...}? => (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomain", "true");
}
// InternalContextMappingDSL.g:1045:20: (otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:1045:21: otherlv_4= 'domainVisionStatement' (otherlv_5= '=' )? ( (lv_domainVisionStatement_6_0= RULE_STRING ) )
{
otherlv_4=(Token)match(input,29,FOLLOW_17);
newLeafNode(otherlv_4, grammarAccess.getDomainAccess().getDomainVisionStatementKeyword_2_1_0_0());
// InternalContextMappingDSL.g:1049:10: (otherlv_5= '=' )?
int alt27=2;
int LA27_0 = input.LA(1);
if ( (LA27_0==21) ) {
alt27=1;
}
switch (alt27) {
case 1 :
// InternalContextMappingDSL.g:1050:11: otherlv_5= '='
{
otherlv_5=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_5, grammarAccess.getDomainAccess().getEqualsSignKeyword_2_1_0_1());
}
break;
}
// InternalContextMappingDSL.g:1055:10: ( (lv_domainVisionStatement_6_0= RULE_STRING ) )
// InternalContextMappingDSL.g:1056:11: (lv_domainVisionStatement_6_0= RULE_STRING )
{
// InternalContextMappingDSL.g:1056:11: (lv_domainVisionStatement_6_0= RULE_STRING )
// InternalContextMappingDSL.g:1057:12: lv_domainVisionStatement_6_0= RULE_STRING
{
lv_domainVisionStatement_6_0=(Token)match(input,RULE_STRING,FOLLOW_24);
newLeafNode(lv_domainVisionStatement_6_0, grammarAccess.getDomainAccess().getDomainVisionStatementSTRINGTerminalRuleCall_2_1_0_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainRule());
}
setWithLastConsumed(
current,
"domainVisionStatement",
lv_domainVisionStatement_6_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainAccess().getUnorderedGroup_2_1());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:1079:5: ({...}? => ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ ) )
{
// InternalContextMappingDSL.g:1079:5: ({...}? => ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ ) )
// InternalContextMappingDSL.g:1080:6: {...}? => ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainAccess().getUnorderedGroup_2_1(), 1) ) {
throw new FailedPredicateException(input, "ruleDomain", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainAccess().getUnorderedGroup_2_1(), 1)");
}
// InternalContextMappingDSL.g:1080:106: ( ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+ )
// InternalContextMappingDSL.g:1081:7: ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+
{
getUnorderedGroupHelper().select(grammarAccess.getDomainAccess().getUnorderedGroup_2_1(), 1);
// InternalContextMappingDSL.g:1084:10: ({...}? => ( (lv_subdomains_7_0= ruleSubdomain ) ) )+
int cnt28=0;
loop28:
do {
int alt28=2;
int LA28_0 = input.LA(1);
if ( (LA28_0==34) ) {
int LA28_2 = input.LA(2);
if ( ((true)) ) {
alt28=1;
}
}
switch (alt28) {
case 1 :
// InternalContextMappingDSL.g:1084:11: {...}? => ( (lv_subdomains_7_0= ruleSubdomain ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomain", "true");
}
// InternalContextMappingDSL.g:1084:20: ( (lv_subdomains_7_0= ruleSubdomain ) )
// InternalContextMappingDSL.g:1084:21: (lv_subdomains_7_0= ruleSubdomain )
{
// InternalContextMappingDSL.g:1084:21: (lv_subdomains_7_0= ruleSubdomain )
// InternalContextMappingDSL.g:1085:11: lv_subdomains_7_0= ruleSubdomain
{
newCompositeNode(grammarAccess.getDomainAccess().getSubdomainsSubdomainParserRuleCall_2_1_1_0());
pushFollow(FOLLOW_24);
lv_subdomains_7_0=ruleSubdomain();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDomainRule());
}
add(
current,
"subdomains",
lv_subdomains_7_0,
"org.contextmapper.dsl.ContextMappingDSL.Subdomain");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
if ( cnt28 >= 1 ) break loop28;
EarlyExitException eee =
new EarlyExitException(28, input);
throw eee;
}
cnt28++;
} while (true);
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainAccess().getUnorderedGroup_2_1());
}
}
}
break;
default :
break loop29;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getDomainAccess().getUnorderedGroup_2_1());
}
this_CLOSE_8=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(this_CLOSE_8, grammarAccess.getDomainAccess().getCLOSETerminalRuleCall_2_2());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleDomain"
// $ANTLR start "entryRuleSubdomain"
// InternalContextMappingDSL.g:1123:1: entryRuleSubdomain returns [EObject current=null] : iv_ruleSubdomain= ruleSubdomain EOF ;
public final EObject entryRuleSubdomain() throws RecognitionException {
EObject current = null;
EObject iv_ruleSubdomain = null;
try {
// InternalContextMappingDSL.g:1123:50: (iv_ruleSubdomain= ruleSubdomain EOF )
// InternalContextMappingDSL.g:1124:2: iv_ruleSubdomain= ruleSubdomain EOF
{
newCompositeNode(grammarAccess.getSubdomainRule());
pushFollow(FOLLOW_1);
iv_ruleSubdomain=ruleSubdomain();
state._fsp--;
current =iv_ruleSubdomain;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleSubdomain"
// $ANTLR start "ruleSubdomain"
// InternalContextMappingDSL.g:1130:1: ruleSubdomain returns [EObject current=null] : (otherlv_0= 'Subdomain' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_entities_10_0= ruleEntity ) )* ( (lv_services_11_0= ruleService ) )* this_CLOSE_12= RULE_CLOSE )? ) ;
public final EObject ruleSubdomain() throws RecognitionException {
EObject current = null;
Token otherlv_0=null;
Token lv_name_1_0=null;
Token this_OPEN_2=null;
Token otherlv_4=null;
Token otherlv_5=null;
Token otherlv_7=null;
Token otherlv_8=null;
Token lv_domainVisionStatement_9_0=null;
Token this_CLOSE_12=null;
Enumerator lv_type_6_0 = null;
EObject lv_entities_10_0 = null;
EObject lv_services_11_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:1136:2: ( (otherlv_0= 'Subdomain' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_entities_10_0= ruleEntity ) )* ( (lv_services_11_0= ruleService ) )* this_CLOSE_12= RULE_CLOSE )? ) )
// InternalContextMappingDSL.g:1137:2: (otherlv_0= 'Subdomain' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_entities_10_0= ruleEntity ) )* ( (lv_services_11_0= ruleService ) )* this_CLOSE_12= RULE_CLOSE )? )
{
// InternalContextMappingDSL.g:1137:2: (otherlv_0= 'Subdomain' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_entities_10_0= ruleEntity ) )* ( (lv_services_11_0= ruleService ) )* this_CLOSE_12= RULE_CLOSE )? )
// InternalContextMappingDSL.g:1138:3: otherlv_0= 'Subdomain' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_entities_10_0= ruleEntity ) )* ( (lv_services_11_0= ruleService ) )* this_CLOSE_12= RULE_CLOSE )?
{
otherlv_0=(Token)match(input,34,FOLLOW_10);
newLeafNode(otherlv_0, grammarAccess.getSubdomainAccess().getSubdomainKeyword_0());
// InternalContextMappingDSL.g:1142:3: ( (lv_name_1_0= RULE_ID ) )
// InternalContextMappingDSL.g:1143:4: (lv_name_1_0= RULE_ID )
{
// InternalContextMappingDSL.g:1143:4: (lv_name_1_0= RULE_ID )
// InternalContextMappingDSL.g:1144:5: lv_name_1_0= RULE_ID
{
lv_name_1_0=(Token)match(input,RULE_ID,FOLLOW_23);
newLeafNode(lv_name_1_0, grammarAccess.getSubdomainAccess().getNameIDTerminalRuleCall_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getSubdomainRule());
}
setWithLastConsumed(
current,
"name",
lv_name_1_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:1160:3: (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_entities_10_0= ruleEntity ) )* ( (lv_services_11_0= ruleService ) )* this_CLOSE_12= RULE_CLOSE )?
int alt36=2;
int LA36_0 = input.LA(1);
if ( (LA36_0==RULE_OPEN) ) {
alt36=1;
}
switch (alt36) {
case 1 :
// InternalContextMappingDSL.g:1161:4: this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_entities_10_0= ruleEntity ) )* ( (lv_services_11_0= ruleService ) )* this_CLOSE_12= RULE_CLOSE
{
this_OPEN_2=(Token)match(input,RULE_OPEN,FOLLOW_25);
newLeafNode(this_OPEN_2, grammarAccess.getSubdomainAccess().getOPENTerminalRuleCall_2_0());
// InternalContextMappingDSL.g:1165:4: ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:1166:5: ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:1166:5: ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:1167:6: ( ( ({...}? => ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getSubdomainAccess().getUnorderedGroup_2_1());
// InternalContextMappingDSL.g:1170:6: ( ( ({...}? => ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:1171:7: ( ({...}? => ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:1171:7: ( ({...}? => ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) ) ) )*
loop33:
do {
int alt33=3;
int LA33_0 = input.LA(1);
if ( LA33_0 == 20 && getUnorderedGroupHelper().canSelect(grammarAccess.getSubdomainAccess().getUnorderedGroup_2_1(), 0) ) {
alt33=1;
}
else if ( LA33_0 == 29 && getUnorderedGroupHelper().canSelect(grammarAccess.getSubdomainAccess().getUnorderedGroup_2_1(), 1) ) {
alt33=2;
}
switch (alt33) {
case 1 :
// InternalContextMappingDSL.g:1172:5: ({...}? => ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) ) )
{
// InternalContextMappingDSL.g:1172:5: ({...}? => ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) ) )
// InternalContextMappingDSL.g:1173:6: {...}? => ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getSubdomainAccess().getUnorderedGroup_2_1(), 0) ) {
throw new FailedPredicateException(input, "ruleSubdomain", "getUnorderedGroupHelper().canSelect(grammarAccess.getSubdomainAccess().getUnorderedGroup_2_1(), 0)");
}
// InternalContextMappingDSL.g:1173:109: ( ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) ) )
// InternalContextMappingDSL.g:1174:7: ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getSubdomainAccess().getUnorderedGroup_2_1(), 0);
// InternalContextMappingDSL.g:1177:10: ({...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) ) )
// InternalContextMappingDSL.g:1177:11: {...}? => (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleSubdomain", "true");
}
// InternalContextMappingDSL.g:1177:20: (otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) ) )
// InternalContextMappingDSL.g:1177:21: otherlv_4= 'type' (otherlv_5= '=' )? ( (lv_type_6_0= ruleSubDomainType ) )
{
otherlv_4=(Token)match(input,20,FOLLOW_26);
newLeafNode(otherlv_4, grammarAccess.getSubdomainAccess().getTypeKeyword_2_1_0_0());
// InternalContextMappingDSL.g:1181:10: (otherlv_5= '=' )?
int alt31=2;
int LA31_0 = input.LA(1);
if ( (LA31_0==21) ) {
alt31=1;
}
switch (alt31) {
case 1 :
// InternalContextMappingDSL.g:1182:11: otherlv_5= '='
{
otherlv_5=(Token)match(input,21,FOLLOW_26);
newLeafNode(otherlv_5, grammarAccess.getSubdomainAccess().getEqualsSignKeyword_2_1_0_1());
}
break;
}
// InternalContextMappingDSL.g:1187:10: ( (lv_type_6_0= ruleSubDomainType ) )
// InternalContextMappingDSL.g:1188:11: (lv_type_6_0= ruleSubDomainType )
{
// InternalContextMappingDSL.g:1188:11: (lv_type_6_0= ruleSubDomainType )
// InternalContextMappingDSL.g:1189:12: lv_type_6_0= ruleSubDomainType
{
newCompositeNode(grammarAccess.getSubdomainAccess().getTypeSubDomainTypeEnumRuleCall_2_1_0_2_0());
pushFollow(FOLLOW_25);
lv_type_6_0=ruleSubDomainType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getSubdomainRule());
}
set(
current,
"type",
lv_type_6_0,
"org.contextmapper.dsl.ContextMappingDSL.SubDomainType");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getSubdomainAccess().getUnorderedGroup_2_1());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:1212:5: ({...}? => ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:1212:5: ({...}? => ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:1213:6: {...}? => ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getSubdomainAccess().getUnorderedGroup_2_1(), 1) ) {
throw new FailedPredicateException(input, "ruleSubdomain", "getUnorderedGroupHelper().canSelect(grammarAccess.getSubdomainAccess().getUnorderedGroup_2_1(), 1)");
}
// InternalContextMappingDSL.g:1213:109: ( ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:1214:7: ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getSubdomainAccess().getUnorderedGroup_2_1(), 1);
// InternalContextMappingDSL.g:1217:10: ({...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:1217:11: {...}? => (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleSubdomain", "true");
}
// InternalContextMappingDSL.g:1217:20: (otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:1217:21: otherlv_7= 'domainVisionStatement' (otherlv_8= '=' )? ( (lv_domainVisionStatement_9_0= RULE_STRING ) )
{
otherlv_7=(Token)match(input,29,FOLLOW_17);
newLeafNode(otherlv_7, grammarAccess.getSubdomainAccess().getDomainVisionStatementKeyword_2_1_1_0());
// InternalContextMappingDSL.g:1221:10: (otherlv_8= '=' )?
int alt32=2;
int LA32_0 = input.LA(1);
if ( (LA32_0==21) ) {
alt32=1;
}
switch (alt32) {
case 1 :
// InternalContextMappingDSL.g:1222:11: otherlv_8= '='
{
otherlv_8=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_8, grammarAccess.getSubdomainAccess().getEqualsSignKeyword_2_1_1_1());
}
break;
}
// InternalContextMappingDSL.g:1227:10: ( (lv_domainVisionStatement_9_0= RULE_STRING ) )
// InternalContextMappingDSL.g:1228:11: (lv_domainVisionStatement_9_0= RULE_STRING )
{
// InternalContextMappingDSL.g:1228:11: (lv_domainVisionStatement_9_0= RULE_STRING )
// InternalContextMappingDSL.g:1229:12: lv_domainVisionStatement_9_0= RULE_STRING
{
lv_domainVisionStatement_9_0=(Token)match(input,RULE_STRING,FOLLOW_25);
newLeafNode(lv_domainVisionStatement_9_0, grammarAccess.getSubdomainAccess().getDomainVisionStatementSTRINGTerminalRuleCall_2_1_1_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getSubdomainRule());
}
setWithLastConsumed(
current,
"domainVisionStatement",
lv_domainVisionStatement_9_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getSubdomainAccess().getUnorderedGroup_2_1());
}
}
}
break;
default :
break loop33;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getSubdomainAccess().getUnorderedGroup_2_1());
}
// InternalContextMappingDSL.g:1258:4: ( (lv_entities_10_0= ruleEntity ) )*
loop34:
do {
int alt34=2;
switch ( input.LA(1) ) {
case RULE_ML_COMMENT:
{
int LA34_1 = input.LA(2);
if ( (LA34_1==RULE_STRING) ) {
int LA34_2 = input.LA(3);
if ( ((LA34_2>=120 && LA34_2<=121)) ) {
alt34=1;
}
}
else if ( ((LA34_1>=120 && LA34_1<=121)) ) {
alt34=1;
}
}
break;
case RULE_STRING:
{
int LA34_2 = input.LA(2);
if ( ((LA34_2>=120 && LA34_2<=121)) ) {
alt34=1;
}
}
break;
case 120:
case 121:
{
alt34=1;
}
break;
}
switch (alt34) {
case 1 :
// InternalContextMappingDSL.g:1259:5: (lv_entities_10_0= ruleEntity )
{
// InternalContextMappingDSL.g:1259:5: (lv_entities_10_0= ruleEntity )
// InternalContextMappingDSL.g:1260:6: lv_entities_10_0= ruleEntity
{
newCompositeNode(grammarAccess.getSubdomainAccess().getEntitiesEntityParserRuleCall_2_2_0());
pushFollow(FOLLOW_27);
lv_entities_10_0=ruleEntity();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getSubdomainRule());
}
add(
current,
"entities",
lv_entities_10_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Entity");
afterParserOrEnumRuleCall();
}
}
break;
default :
break loop34;
}
} while (true);
// InternalContextMappingDSL.g:1277:4: ( (lv_services_11_0= ruleService ) )*
loop35:
do {
int alt35=2;
int LA35_0 = input.LA(1);
if ( (LA35_0==RULE_STRING||LA35_0==RULE_ML_COMMENT||LA35_0==85) ) {
alt35=1;
}
switch (alt35) {
case 1 :
// InternalContextMappingDSL.g:1278:5: (lv_services_11_0= ruleService )
{
// InternalContextMappingDSL.g:1278:5: (lv_services_11_0= ruleService )
// InternalContextMappingDSL.g:1279:6: lv_services_11_0= ruleService
{
newCompositeNode(grammarAccess.getSubdomainAccess().getServicesServiceParserRuleCall_2_3_0());
pushFollow(FOLLOW_28);
lv_services_11_0=ruleService();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getSubdomainRule());
}
add(
current,
"services",
lv_services_11_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Service");
afterParserOrEnumRuleCall();
}
}
break;
default :
break loop35;
}
} while (true);
this_CLOSE_12=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(this_CLOSE_12, grammarAccess.getSubdomainAccess().getCLOSETerminalRuleCall_2_4());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleSubdomain"
// $ANTLR start "entryRuleRelationship"
// InternalContextMappingDSL.g:1305:1: entryRuleRelationship returns [EObject current=null] : iv_ruleRelationship= ruleRelationship EOF ;
public final EObject entryRuleRelationship() throws RecognitionException {
EObject current = null;
EObject iv_ruleRelationship = null;
try {
// InternalContextMappingDSL.g:1305:53: (iv_ruleRelationship= ruleRelationship EOF )
// InternalContextMappingDSL.g:1306:2: iv_ruleRelationship= ruleRelationship EOF
{
newCompositeNode(grammarAccess.getRelationshipRule());
pushFollow(FOLLOW_1);
iv_ruleRelationship=ruleRelationship();
state._fsp--;
current =iv_ruleRelationship;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleRelationship"
// $ANTLR start "ruleRelationship"
// InternalContextMappingDSL.g:1312:1: ruleRelationship returns [EObject current=null] : (this_SymmetricRelationship_0= ruleSymmetricRelationship | this_UpstreamDownstreamRelationship_1= ruleUpstreamDownstreamRelationship ) ;
public final EObject ruleRelationship() throws RecognitionException {
EObject current = null;
EObject this_SymmetricRelationship_0 = null;
EObject this_UpstreamDownstreamRelationship_1 = null;
enterRule();
try {
// InternalContextMappingDSL.g:1318:2: ( (this_SymmetricRelationship_0= ruleSymmetricRelationship | this_UpstreamDownstreamRelationship_1= ruleUpstreamDownstreamRelationship ) )
// InternalContextMappingDSL.g:1319:2: (this_SymmetricRelationship_0= ruleSymmetricRelationship | this_UpstreamDownstreamRelationship_1= ruleUpstreamDownstreamRelationship )
{
// InternalContextMappingDSL.g:1319:2: (this_SymmetricRelationship_0= ruleSymmetricRelationship | this_UpstreamDownstreamRelationship_1= ruleUpstreamDownstreamRelationship )
int alt37=2;
int LA37_0 = input.LA(1);
if ( (LA37_0==RULE_ID) ) {
switch ( input.LA(2) ) {
case 35:
{
int LA37_3 = input.LA(3);
if ( (LA37_3==37||LA37_3==43||LA37_3==45||(LA37_3>=51 && LA37_3<=52)||(LA37_3>=208 && LA37_3<=211)) ) {
alt37=2;
}
else if ( (LA37_3==36||LA37_3==41) ) {
alt37=1;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 37, 3, input);
throw nvae;
}
}
break;
case 44:
case 46:
case 47:
case 48:
case 53:
case 54:
{
alt37=2;
}
break;
case 38:
case 39:
case 42:
{
alt37=1;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 37, 1, input);
throw nvae;
}
}
else if ( (LA37_0==35) ) {
alt37=1;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 37, 0, input);
throw nvae;
}
switch (alt37) {
case 1 :
// InternalContextMappingDSL.g:1320:3: this_SymmetricRelationship_0= ruleSymmetricRelationship
{
newCompositeNode(grammarAccess.getRelationshipAccess().getSymmetricRelationshipParserRuleCall_0());
pushFollow(FOLLOW_2);
this_SymmetricRelationship_0=ruleSymmetricRelationship();
state._fsp--;
current = this_SymmetricRelationship_0;
afterParserOrEnumRuleCall();
}
break;
case 2 :
// InternalContextMappingDSL.g:1329:3: this_UpstreamDownstreamRelationship_1= ruleUpstreamDownstreamRelationship
{
newCompositeNode(grammarAccess.getRelationshipAccess().getUpstreamDownstreamRelationshipParserRuleCall_1());
pushFollow(FOLLOW_2);
this_UpstreamDownstreamRelationship_1=ruleUpstreamDownstreamRelationship();
state._fsp--;
current = this_UpstreamDownstreamRelationship_1;
afterParserOrEnumRuleCall();
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleRelationship"
// $ANTLR start "entryRuleSymmetricRelationship"
// InternalContextMappingDSL.g:1341:1: entryRuleSymmetricRelationship returns [EObject current=null] : iv_ruleSymmetricRelationship= ruleSymmetricRelationship EOF ;
public final EObject entryRuleSymmetricRelationship() throws RecognitionException {
EObject current = null;
EObject iv_ruleSymmetricRelationship = null;
try {
// InternalContextMappingDSL.g:1341:62: (iv_ruleSymmetricRelationship= ruleSymmetricRelationship EOF )
// InternalContextMappingDSL.g:1342:2: iv_ruleSymmetricRelationship= ruleSymmetricRelationship EOF
{
newCompositeNode(grammarAccess.getSymmetricRelationshipRule());
pushFollow(FOLLOW_1);
iv_ruleSymmetricRelationship=ruleSymmetricRelationship();
state._fsp--;
current =iv_ruleSymmetricRelationship;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleSymmetricRelationship"
// $ANTLR start "ruleSymmetricRelationship"
// InternalContextMappingDSL.g:1348:1: ruleSymmetricRelationship returns [EObject current=null] : (this_Partnership_0= rulePartnership | this_SharedKernel_1= ruleSharedKernel ) ;
public final EObject ruleSymmetricRelationship() throws RecognitionException {
EObject current = null;
EObject this_Partnership_0 = null;
EObject this_SharedKernel_1 = null;
enterRule();
try {
// InternalContextMappingDSL.g:1354:2: ( (this_Partnership_0= rulePartnership | this_SharedKernel_1= ruleSharedKernel ) )
// InternalContextMappingDSL.g:1355:2: (this_Partnership_0= rulePartnership | this_SharedKernel_1= ruleSharedKernel )
{
// InternalContextMappingDSL.g:1355:2: (this_Partnership_0= rulePartnership | this_SharedKernel_1= ruleSharedKernel )
int alt38=2;
int LA38_0 = input.LA(1);
if ( (LA38_0==RULE_ID) ) {
switch ( input.LA(2) ) {
case 35:
{
int LA38_3 = input.LA(3);
if ( (LA38_3==36) ) {
alt38=1;
}
else if ( (LA38_3==41) ) {
alt38=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 38, 3, input);
throw nvae;
}
}
break;
case 39:
{
alt38=1;
}
break;
case 38:
case 42:
{
alt38=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 38, 1, input);
throw nvae;
}
}
else if ( (LA38_0==35) ) {
int LA38_2 = input.LA(2);
if ( (LA38_2==41) ) {
alt38=2;
}
else if ( (LA38_2==36) ) {
alt38=1;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 38, 2, input);
throw nvae;
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 38, 0, input);
throw nvae;
}
switch (alt38) {
case 1 :
// InternalContextMappingDSL.g:1356:3: this_Partnership_0= rulePartnership
{
newCompositeNode(grammarAccess.getSymmetricRelationshipAccess().getPartnershipParserRuleCall_0());
pushFollow(FOLLOW_2);
this_Partnership_0=rulePartnership();
state._fsp--;
current = this_Partnership_0;
afterParserOrEnumRuleCall();
}
break;
case 2 :
// InternalContextMappingDSL.g:1365:3: this_SharedKernel_1= ruleSharedKernel
{
newCompositeNode(grammarAccess.getSymmetricRelationshipAccess().getSharedKernelParserRuleCall_1());
pushFollow(FOLLOW_2);
this_SharedKernel_1=ruleSharedKernel();
state._fsp--;
current = this_SharedKernel_1;
afterParserOrEnumRuleCall();
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleSymmetricRelationship"
// $ANTLR start "entryRulePartnership"
// InternalContextMappingDSL.g:1377:1: entryRulePartnership returns [EObject current=null] : iv_rulePartnership= rulePartnership EOF ;
public final EObject entryRulePartnership() throws RecognitionException {
EObject current = null;
EObject iv_rulePartnership = null;
try {
// InternalContextMappingDSL.g:1377:52: (iv_rulePartnership= rulePartnership EOF )
// InternalContextMappingDSL.g:1378:2: iv_rulePartnership= rulePartnership EOF
{
newCompositeNode(grammarAccess.getPartnershipRule());
pushFollow(FOLLOW_1);
iv_rulePartnership=rulePartnership();
state._fsp--;
current =iv_rulePartnership;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRulePartnership"
// $ANTLR start "rulePartnership"
// InternalContextMappingDSL.g:1384:1: rulePartnership returns [EObject current=null] : ( ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'P' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'P' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) ) | (otherlv_9= '[' otherlv_10= 'P' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'P' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) ) | ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'P' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'P' otherlv_26= ']' ) | (otherlv_27= '[' otherlv_28= 'P' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'P' otherlv_35= ']' ) | ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Partnership' ( (otherlv_38= RULE_ID ) ) ) ) (otherlv_39= ':' ( (lv_name_40_0= RULE_ID ) ) )? (this_OPEN_41= RULE_OPEN (otherlv_42= 'implementationTechnology' (otherlv_43= '=' )? ( (lv_implementationTechnology_44_0= RULE_STRING ) ) )? this_CLOSE_45= RULE_CLOSE )? ) ;
public final EObject rulePartnership() throws RecognitionException {
EObject current = null;
Token otherlv_0=null;
Token otherlv_1=null;
Token otherlv_2=null;
Token otherlv_3=null;
Token otherlv_4=null;
Token otherlv_5=null;
Token otherlv_6=null;
Token otherlv_7=null;
Token otherlv_8=null;
Token otherlv_9=null;
Token otherlv_10=null;
Token otherlv_11=null;
Token otherlv_12=null;
Token otherlv_13=null;
Token otherlv_14=null;
Token otherlv_15=null;
Token otherlv_16=null;
Token otherlv_17=null;
Token otherlv_18=null;
Token otherlv_19=null;
Token otherlv_20=null;
Token otherlv_21=null;
Token otherlv_22=null;
Token otherlv_23=null;
Token otherlv_24=null;
Token otherlv_25=null;
Token otherlv_26=null;
Token otherlv_27=null;
Token otherlv_28=null;
Token otherlv_29=null;
Token otherlv_30=null;
Token otherlv_31=null;
Token otherlv_32=null;
Token otherlv_33=null;
Token otherlv_34=null;
Token otherlv_35=null;
Token otherlv_36=null;
Token otherlv_37=null;
Token otherlv_38=null;
Token otherlv_39=null;
Token lv_name_40_0=null;
Token this_OPEN_41=null;
Token otherlv_42=null;
Token otherlv_43=null;
Token lv_implementationTechnology_44_0=null;
Token this_CLOSE_45=null;
enterRule();
try {
// InternalContextMappingDSL.g:1390:2: ( ( ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'P' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'P' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) ) | (otherlv_9= '[' otherlv_10= 'P' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'P' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) ) | ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'P' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'P' otherlv_26= ']' ) | (otherlv_27= '[' otherlv_28= 'P' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'P' otherlv_35= ']' ) | ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Partnership' ( (otherlv_38= RULE_ID ) ) ) ) (otherlv_39= ':' ( (lv_name_40_0= RULE_ID ) ) )? (this_OPEN_41= RULE_OPEN (otherlv_42= 'implementationTechnology' (otherlv_43= '=' )? ( (lv_implementationTechnology_44_0= RULE_STRING ) ) )? this_CLOSE_45= RULE_CLOSE )? ) )
// InternalContextMappingDSL.g:1391:2: ( ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'P' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'P' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) ) | (otherlv_9= '[' otherlv_10= 'P' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'P' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) ) | ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'P' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'P' otherlv_26= ']' ) | (otherlv_27= '[' otherlv_28= 'P' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'P' otherlv_35= ']' ) | ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Partnership' ( (otherlv_38= RULE_ID ) ) ) ) (otherlv_39= ':' ( (lv_name_40_0= RULE_ID ) ) )? (this_OPEN_41= RULE_OPEN (otherlv_42= 'implementationTechnology' (otherlv_43= '=' )? ( (lv_implementationTechnology_44_0= RULE_STRING ) ) )? this_CLOSE_45= RULE_CLOSE )? )
{
// InternalContextMappingDSL.g:1391:2: ( ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'P' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'P' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) ) | (otherlv_9= '[' otherlv_10= 'P' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'P' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) ) | ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'P' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'P' otherlv_26= ']' ) | (otherlv_27= '[' otherlv_28= 'P' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'P' otherlv_35= ']' ) | ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Partnership' ( (otherlv_38= RULE_ID ) ) ) ) (otherlv_39= ':' ( (lv_name_40_0= RULE_ID ) ) )? (this_OPEN_41= RULE_OPEN (otherlv_42= 'implementationTechnology' (otherlv_43= '=' )? ( (lv_implementationTechnology_44_0= RULE_STRING ) ) )? this_CLOSE_45= RULE_CLOSE )? )
// InternalContextMappingDSL.g:1392:3: ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'P' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'P' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) ) | (otherlv_9= '[' otherlv_10= 'P' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'P' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) ) | ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'P' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'P' otherlv_26= ']' ) | (otherlv_27= '[' otherlv_28= 'P' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'P' otherlv_35= ']' ) | ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Partnership' ( (otherlv_38= RULE_ID ) ) ) ) (otherlv_39= ':' ( (lv_name_40_0= RULE_ID ) ) )? (this_OPEN_41= RULE_OPEN (otherlv_42= 'implementationTechnology' (otherlv_43= '=' )? ( (lv_implementationTechnology_44_0= RULE_STRING ) ) )? this_CLOSE_45= RULE_CLOSE )?
{
// InternalContextMappingDSL.g:1392:3: ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'P' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'P' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) ) | (otherlv_9= '[' otherlv_10= 'P' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'P' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) ) | ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'P' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'P' otherlv_26= ']' ) | (otherlv_27= '[' otherlv_28= 'P' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'P' otherlv_35= ']' ) | ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Partnership' ( (otherlv_38= RULE_ID ) ) ) )
int alt39=5;
alt39 = dfa39.predict(input);
switch (alt39) {
case 1 :
// InternalContextMappingDSL.g:1393:4: ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'P' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'P' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:1393:4: ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'P' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'P' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) )
// InternalContextMappingDSL.g:1394:5: ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'P' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'P' otherlv_7= ']' ( (otherlv_8= RULE_ID ) )
{
// InternalContextMappingDSL.g:1394:5: ( (otherlv_0= RULE_ID ) )
// InternalContextMappingDSL.g:1395:6: (otherlv_0= RULE_ID )
{
// InternalContextMappingDSL.g:1395:6: (otherlv_0= RULE_ID )
// InternalContextMappingDSL.g:1396:7: otherlv_0= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getPartnershipRule());
}
otherlv_0=(Token)match(input,RULE_ID,FOLLOW_29);
newLeafNode(otherlv_0, grammarAccess.getPartnershipAccess().getParticipant1BoundedContextCrossReference_0_0_0_0());
}
}
otherlv_1=(Token)match(input,35,FOLLOW_30);
newLeafNode(otherlv_1, grammarAccess.getPartnershipAccess().getLeftSquareBracketKeyword_0_0_1());
otherlv_2=(Token)match(input,36,FOLLOW_31);
newLeafNode(otherlv_2, grammarAccess.getPartnershipAccess().getPKeyword_0_0_2());
otherlv_3=(Token)match(input,37,FOLLOW_32);
newLeafNode(otherlv_3, grammarAccess.getPartnershipAccess().getRightSquareBracketKeyword_0_0_3());
otherlv_4=(Token)match(input,38,FOLLOW_29);
newLeafNode(otherlv_4, grammarAccess.getPartnershipAccess().getLessThanSignHyphenMinusGreaterThanSignKeyword_0_0_4());
otherlv_5=(Token)match(input,35,FOLLOW_30);
newLeafNode(otherlv_5, grammarAccess.getPartnershipAccess().getLeftSquareBracketKeyword_0_0_5());
otherlv_6=(Token)match(input,36,FOLLOW_31);
newLeafNode(otherlv_6, grammarAccess.getPartnershipAccess().getPKeyword_0_0_6());
otherlv_7=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_7, grammarAccess.getPartnershipAccess().getRightSquareBracketKeyword_0_0_7());
// InternalContextMappingDSL.g:1435:5: ( (otherlv_8= RULE_ID ) )
// InternalContextMappingDSL.g:1436:6: (otherlv_8= RULE_ID )
{
// InternalContextMappingDSL.g:1436:6: (otherlv_8= RULE_ID )
// InternalContextMappingDSL.g:1437:7: otherlv_8= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getPartnershipRule());
}
otherlv_8=(Token)match(input,RULE_ID,FOLLOW_33);
newLeafNode(otherlv_8, grammarAccess.getPartnershipAccess().getParticipant2BoundedContextCrossReference_0_0_8_0());
}
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:1450:4: (otherlv_9= '[' otherlv_10= 'P' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'P' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:1450:4: (otherlv_9= '[' otherlv_10= 'P' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'P' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) )
// InternalContextMappingDSL.g:1451:5: otherlv_9= '[' otherlv_10= 'P' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'P' otherlv_16= ']' ( (otherlv_17= RULE_ID ) )
{
otherlv_9=(Token)match(input,35,FOLLOW_30);
newLeafNode(otherlv_9, grammarAccess.getPartnershipAccess().getLeftSquareBracketKeyword_0_1_0());
otherlv_10=(Token)match(input,36,FOLLOW_31);
newLeafNode(otherlv_10, grammarAccess.getPartnershipAccess().getPKeyword_0_1_1());
otherlv_11=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_11, grammarAccess.getPartnershipAccess().getRightSquareBracketKeyword_0_1_2());
// InternalContextMappingDSL.g:1463:5: ( (otherlv_12= RULE_ID ) )
// InternalContextMappingDSL.g:1464:6: (otherlv_12= RULE_ID )
{
// InternalContextMappingDSL.g:1464:6: (otherlv_12= RULE_ID )
// InternalContextMappingDSL.g:1465:7: otherlv_12= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getPartnershipRule());
}
otherlv_12=(Token)match(input,RULE_ID,FOLLOW_32);
newLeafNode(otherlv_12, grammarAccess.getPartnershipAccess().getParticipant1BoundedContextCrossReference_0_1_3_0());
}
}
otherlv_13=(Token)match(input,38,FOLLOW_29);
newLeafNode(otherlv_13, grammarAccess.getPartnershipAccess().getLessThanSignHyphenMinusGreaterThanSignKeyword_0_1_4());
otherlv_14=(Token)match(input,35,FOLLOW_30);
newLeafNode(otherlv_14, grammarAccess.getPartnershipAccess().getLeftSquareBracketKeyword_0_1_5());
otherlv_15=(Token)match(input,36,FOLLOW_31);
newLeafNode(otherlv_15, grammarAccess.getPartnershipAccess().getPKeyword_0_1_6());
otherlv_16=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_16, grammarAccess.getPartnershipAccess().getRightSquareBracketKeyword_0_1_7());
// InternalContextMappingDSL.g:1492:5: ( (otherlv_17= RULE_ID ) )
// InternalContextMappingDSL.g:1493:6: (otherlv_17= RULE_ID )
{
// InternalContextMappingDSL.g:1493:6: (otherlv_17= RULE_ID )
// InternalContextMappingDSL.g:1494:7: otherlv_17= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getPartnershipRule());
}
otherlv_17=(Token)match(input,RULE_ID,FOLLOW_33);
newLeafNode(otherlv_17, grammarAccess.getPartnershipAccess().getParticipant2BoundedContextCrossReference_0_1_8_0());
}
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:1507:4: ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'P' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'P' otherlv_26= ']' )
{
// InternalContextMappingDSL.g:1507:4: ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'P' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'P' otherlv_26= ']' )
// InternalContextMappingDSL.g:1508:5: ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'P' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'P' otherlv_26= ']'
{
// InternalContextMappingDSL.g:1508:5: ( (otherlv_18= RULE_ID ) )
// InternalContextMappingDSL.g:1509:6: (otherlv_18= RULE_ID )
{
// InternalContextMappingDSL.g:1509:6: (otherlv_18= RULE_ID )
// InternalContextMappingDSL.g:1510:7: otherlv_18= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getPartnershipRule());
}
otherlv_18=(Token)match(input,RULE_ID,FOLLOW_29);
newLeafNode(otherlv_18, grammarAccess.getPartnershipAccess().getParticipant1BoundedContextCrossReference_0_2_0_0());
}
}
otherlv_19=(Token)match(input,35,FOLLOW_30);
newLeafNode(otherlv_19, grammarAccess.getPartnershipAccess().getLeftSquareBracketKeyword_0_2_1());
otherlv_20=(Token)match(input,36,FOLLOW_31);
newLeafNode(otherlv_20, grammarAccess.getPartnershipAccess().getPKeyword_0_2_2());
otherlv_21=(Token)match(input,37,FOLLOW_32);
newLeafNode(otherlv_21, grammarAccess.getPartnershipAccess().getRightSquareBracketKeyword_0_2_3());
otherlv_22=(Token)match(input,38,FOLLOW_10);
newLeafNode(otherlv_22, grammarAccess.getPartnershipAccess().getLessThanSignHyphenMinusGreaterThanSignKeyword_0_2_4());
// InternalContextMappingDSL.g:1537:5: ( (otherlv_23= RULE_ID ) )
// InternalContextMappingDSL.g:1538:6: (otherlv_23= RULE_ID )
{
// InternalContextMappingDSL.g:1538:6: (otherlv_23= RULE_ID )
// InternalContextMappingDSL.g:1539:7: otherlv_23= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getPartnershipRule());
}
otherlv_23=(Token)match(input,RULE_ID,FOLLOW_29);
newLeafNode(otherlv_23, grammarAccess.getPartnershipAccess().getParticipant2BoundedContextCrossReference_0_2_5_0());
}
}
otherlv_24=(Token)match(input,35,FOLLOW_30);
newLeafNode(otherlv_24, grammarAccess.getPartnershipAccess().getLeftSquareBracketKeyword_0_2_6());
otherlv_25=(Token)match(input,36,FOLLOW_31);
newLeafNode(otherlv_25, grammarAccess.getPartnershipAccess().getPKeyword_0_2_7());
otherlv_26=(Token)match(input,37,FOLLOW_33);
newLeafNode(otherlv_26, grammarAccess.getPartnershipAccess().getRightSquareBracketKeyword_0_2_8());
}
}
break;
case 4 :
// InternalContextMappingDSL.g:1564:4: (otherlv_27= '[' otherlv_28= 'P' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'P' otherlv_35= ']' )
{
// InternalContextMappingDSL.g:1564:4: (otherlv_27= '[' otherlv_28= 'P' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'P' otherlv_35= ']' )
// InternalContextMappingDSL.g:1565:5: otherlv_27= '[' otherlv_28= 'P' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'P' otherlv_35= ']'
{
otherlv_27=(Token)match(input,35,FOLLOW_30);
newLeafNode(otherlv_27, grammarAccess.getPartnershipAccess().getLeftSquareBracketKeyword_0_3_0());
otherlv_28=(Token)match(input,36,FOLLOW_31);
newLeafNode(otherlv_28, grammarAccess.getPartnershipAccess().getPKeyword_0_3_1());
otherlv_29=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_29, grammarAccess.getPartnershipAccess().getRightSquareBracketKeyword_0_3_2());
// InternalContextMappingDSL.g:1577:5: ( (otherlv_30= RULE_ID ) )
// InternalContextMappingDSL.g:1578:6: (otherlv_30= RULE_ID )
{
// InternalContextMappingDSL.g:1578:6: (otherlv_30= RULE_ID )
// InternalContextMappingDSL.g:1579:7: otherlv_30= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getPartnershipRule());
}
otherlv_30=(Token)match(input,RULE_ID,FOLLOW_32);
newLeafNode(otherlv_30, grammarAccess.getPartnershipAccess().getParticipant1BoundedContextCrossReference_0_3_3_0());
}
}
otherlv_31=(Token)match(input,38,FOLLOW_10);
newLeafNode(otherlv_31, grammarAccess.getPartnershipAccess().getLessThanSignHyphenMinusGreaterThanSignKeyword_0_3_4());
// InternalContextMappingDSL.g:1594:5: ( (otherlv_32= RULE_ID ) )
// InternalContextMappingDSL.g:1595:6: (otherlv_32= RULE_ID )
{
// InternalContextMappingDSL.g:1595:6: (otherlv_32= RULE_ID )
// InternalContextMappingDSL.g:1596:7: otherlv_32= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getPartnershipRule());
}
otherlv_32=(Token)match(input,RULE_ID,FOLLOW_29);
newLeafNode(otherlv_32, grammarAccess.getPartnershipAccess().getParticipant2BoundedContextCrossReference_0_3_5_0());
}
}
otherlv_33=(Token)match(input,35,FOLLOW_30);
newLeafNode(otherlv_33, grammarAccess.getPartnershipAccess().getLeftSquareBracketKeyword_0_3_6());
otherlv_34=(Token)match(input,36,FOLLOW_31);
newLeafNode(otherlv_34, grammarAccess.getPartnershipAccess().getPKeyword_0_3_7());
otherlv_35=(Token)match(input,37,FOLLOW_33);
newLeafNode(otherlv_35, grammarAccess.getPartnershipAccess().getRightSquareBracketKeyword_0_3_8());
}
}
break;
case 5 :
// InternalContextMappingDSL.g:1621:4: ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Partnership' ( (otherlv_38= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:1621:4: ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Partnership' ( (otherlv_38= RULE_ID ) ) )
// InternalContextMappingDSL.g:1622:5: ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Partnership' ( (otherlv_38= RULE_ID ) )
{
// InternalContextMappingDSL.g:1622:5: ( (otherlv_36= RULE_ID ) )
// InternalContextMappingDSL.g:1623:6: (otherlv_36= RULE_ID )
{
// InternalContextMappingDSL.g:1623:6: (otherlv_36= RULE_ID )
// InternalContextMappingDSL.g:1624:7: otherlv_36= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getPartnershipRule());
}
otherlv_36=(Token)match(input,RULE_ID,FOLLOW_34);
newLeafNode(otherlv_36, grammarAccess.getPartnershipAccess().getParticipant1BoundedContextCrossReference_0_4_0_0());
}
}
otherlv_37=(Token)match(input,39,FOLLOW_10);
newLeafNode(otherlv_37, grammarAccess.getPartnershipAccess().getPartnershipKeyword_0_4_1());
// InternalContextMappingDSL.g:1639:5: ( (otherlv_38= RULE_ID ) )
// InternalContextMappingDSL.g:1640:6: (otherlv_38= RULE_ID )
{
// InternalContextMappingDSL.g:1640:6: (otherlv_38= RULE_ID )
// InternalContextMappingDSL.g:1641:7: otherlv_38= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getPartnershipRule());
}
otherlv_38=(Token)match(input,RULE_ID,FOLLOW_33);
newLeafNode(otherlv_38, grammarAccess.getPartnershipAccess().getParticipant2BoundedContextCrossReference_0_4_2_0());
}
}
}
}
break;
}
// InternalContextMappingDSL.g:1654:3: (otherlv_39= ':' ( (lv_name_40_0= RULE_ID ) ) )?
int alt40=2;
int LA40_0 = input.LA(1);
if ( (LA40_0==40) ) {
alt40=1;
}
switch (alt40) {
case 1 :
// InternalContextMappingDSL.g:1655:4: otherlv_39= ':' ( (lv_name_40_0= RULE_ID ) )
{
otherlv_39=(Token)match(input,40,FOLLOW_10);
newLeafNode(otherlv_39, grammarAccess.getPartnershipAccess().getColonKeyword_1_0());
// InternalContextMappingDSL.g:1659:4: ( (lv_name_40_0= RULE_ID ) )
// InternalContextMappingDSL.g:1660:5: (lv_name_40_0= RULE_ID )
{
// InternalContextMappingDSL.g:1660:5: (lv_name_40_0= RULE_ID )
// InternalContextMappingDSL.g:1661:6: lv_name_40_0= RULE_ID
{
lv_name_40_0=(Token)match(input,RULE_ID,FOLLOW_23);
newLeafNode(lv_name_40_0, grammarAccess.getPartnershipAccess().getNameIDTerminalRuleCall_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getPartnershipRule());
}
setWithLastConsumed(
current,
"name",
lv_name_40_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
}
break;
}
// InternalContextMappingDSL.g:1678:3: (this_OPEN_41= RULE_OPEN (otherlv_42= 'implementationTechnology' (otherlv_43= '=' )? ( (lv_implementationTechnology_44_0= RULE_STRING ) ) )? this_CLOSE_45= RULE_CLOSE )?
int alt43=2;
int LA43_0 = input.LA(1);
if ( (LA43_0==RULE_OPEN) ) {
alt43=1;
}
switch (alt43) {
case 1 :
// InternalContextMappingDSL.g:1679:4: this_OPEN_41= RULE_OPEN (otherlv_42= 'implementationTechnology' (otherlv_43= '=' )? ( (lv_implementationTechnology_44_0= RULE_STRING ) ) )? this_CLOSE_45= RULE_CLOSE
{
this_OPEN_41=(Token)match(input,RULE_OPEN,FOLLOW_35);
newLeafNode(this_OPEN_41, grammarAccess.getPartnershipAccess().getOPENTerminalRuleCall_2_0());
// InternalContextMappingDSL.g:1683:4: (otherlv_42= 'implementationTechnology' (otherlv_43= '=' )? ( (lv_implementationTechnology_44_0= RULE_STRING ) ) )?
int alt42=2;
int LA42_0 = input.LA(1);
if ( (LA42_0==31) ) {
alt42=1;
}
switch (alt42) {
case 1 :
// InternalContextMappingDSL.g:1684:5: otherlv_42= 'implementationTechnology' (otherlv_43= '=' )? ( (lv_implementationTechnology_44_0= RULE_STRING ) )
{
otherlv_42=(Token)match(input,31,FOLLOW_17);
newLeafNode(otherlv_42, grammarAccess.getPartnershipAccess().getImplementationTechnologyKeyword_2_1_0());
// InternalContextMappingDSL.g:1688:5: (otherlv_43= '=' )?
int alt41=2;
int LA41_0 = input.LA(1);
if ( (LA41_0==21) ) {
alt41=1;
}
switch (alt41) {
case 1 :
// InternalContextMappingDSL.g:1689:6: otherlv_43= '='
{
otherlv_43=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_43, grammarAccess.getPartnershipAccess().getEqualsSignKeyword_2_1_1());
}
break;
}
// InternalContextMappingDSL.g:1694:5: ( (lv_implementationTechnology_44_0= RULE_STRING ) )
// InternalContextMappingDSL.g:1695:6: (lv_implementationTechnology_44_0= RULE_STRING )
{
// InternalContextMappingDSL.g:1695:6: (lv_implementationTechnology_44_0= RULE_STRING )
// InternalContextMappingDSL.g:1696:7: lv_implementationTechnology_44_0= RULE_STRING
{
lv_implementationTechnology_44_0=(Token)match(input,RULE_STRING,FOLLOW_36);
newLeafNode(lv_implementationTechnology_44_0, grammarAccess.getPartnershipAccess().getImplementationTechnologySTRINGTerminalRuleCall_2_1_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getPartnershipRule());
}
setWithLastConsumed(
current,
"implementationTechnology",
lv_implementationTechnology_44_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
this_CLOSE_45=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(this_CLOSE_45, grammarAccess.getPartnershipAccess().getCLOSETerminalRuleCall_2_2());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "rulePartnership"
// $ANTLR start "entryRuleSharedKernel"
// InternalContextMappingDSL.g:1722:1: entryRuleSharedKernel returns [EObject current=null] : iv_ruleSharedKernel= ruleSharedKernel EOF ;
public final EObject entryRuleSharedKernel() throws RecognitionException {
EObject current = null;
EObject iv_ruleSharedKernel = null;
try {
// InternalContextMappingDSL.g:1722:53: (iv_ruleSharedKernel= ruleSharedKernel EOF )
// InternalContextMappingDSL.g:1723:2: iv_ruleSharedKernel= ruleSharedKernel EOF
{
newCompositeNode(grammarAccess.getSharedKernelRule());
pushFollow(FOLLOW_1);
iv_ruleSharedKernel=ruleSharedKernel();
state._fsp--;
current =iv_ruleSharedKernel;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleSharedKernel"
// $ANTLR start "ruleSharedKernel"
// InternalContextMappingDSL.g:1729:1: ruleSharedKernel returns [EObject current=null] : ( ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'SK' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'SK' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) ) | (otherlv_9= '[' otherlv_10= 'SK' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'SK' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) ) | ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'SK' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'SK' otherlv_26= ']' ) | (otherlv_27= '[' otherlv_28= 'SK' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'SK' otherlv_35= ']' ) | ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Shared-Kernel' ( (otherlv_38= RULE_ID ) ) ) | ( ( (otherlv_39= RULE_ID ) ) otherlv_40= '<->' ( (otherlv_41= RULE_ID ) ) ) ) (otherlv_42= ':' ( (lv_name_43_0= RULE_ID ) ) )? (this_OPEN_44= RULE_OPEN (otherlv_45= 'implementationTechnology' (otherlv_46= '=' )? ( (lv_implementationTechnology_47_0= RULE_STRING ) ) )? this_CLOSE_48= RULE_CLOSE )? ) ;
public final EObject ruleSharedKernel() throws RecognitionException {
EObject current = null;
Token otherlv_0=null;
Token otherlv_1=null;
Token otherlv_2=null;
Token otherlv_3=null;
Token otherlv_4=null;
Token otherlv_5=null;
Token otherlv_6=null;
Token otherlv_7=null;
Token otherlv_8=null;
Token otherlv_9=null;
Token otherlv_10=null;
Token otherlv_11=null;
Token otherlv_12=null;
Token otherlv_13=null;
Token otherlv_14=null;
Token otherlv_15=null;
Token otherlv_16=null;
Token otherlv_17=null;
Token otherlv_18=null;
Token otherlv_19=null;
Token otherlv_20=null;
Token otherlv_21=null;
Token otherlv_22=null;
Token otherlv_23=null;
Token otherlv_24=null;
Token otherlv_25=null;
Token otherlv_26=null;
Token otherlv_27=null;
Token otherlv_28=null;
Token otherlv_29=null;
Token otherlv_30=null;
Token otherlv_31=null;
Token otherlv_32=null;
Token otherlv_33=null;
Token otherlv_34=null;
Token otherlv_35=null;
Token otherlv_36=null;
Token otherlv_37=null;
Token otherlv_38=null;
Token otherlv_39=null;
Token otherlv_40=null;
Token otherlv_41=null;
Token otherlv_42=null;
Token lv_name_43_0=null;
Token this_OPEN_44=null;
Token otherlv_45=null;
Token otherlv_46=null;
Token lv_implementationTechnology_47_0=null;
Token this_CLOSE_48=null;
enterRule();
try {
// InternalContextMappingDSL.g:1735:2: ( ( ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'SK' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'SK' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) ) | (otherlv_9= '[' otherlv_10= 'SK' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'SK' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) ) | ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'SK' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'SK' otherlv_26= ']' ) | (otherlv_27= '[' otherlv_28= 'SK' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'SK' otherlv_35= ']' ) | ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Shared-Kernel' ( (otherlv_38= RULE_ID ) ) ) | ( ( (otherlv_39= RULE_ID ) ) otherlv_40= '<->' ( (otherlv_41= RULE_ID ) ) ) ) (otherlv_42= ':' ( (lv_name_43_0= RULE_ID ) ) )? (this_OPEN_44= RULE_OPEN (otherlv_45= 'implementationTechnology' (otherlv_46= '=' )? ( (lv_implementationTechnology_47_0= RULE_STRING ) ) )? this_CLOSE_48= RULE_CLOSE )? ) )
// InternalContextMappingDSL.g:1736:2: ( ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'SK' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'SK' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) ) | (otherlv_9= '[' otherlv_10= 'SK' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'SK' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) ) | ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'SK' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'SK' otherlv_26= ']' ) | (otherlv_27= '[' otherlv_28= 'SK' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'SK' otherlv_35= ']' ) | ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Shared-Kernel' ( (otherlv_38= RULE_ID ) ) ) | ( ( (otherlv_39= RULE_ID ) ) otherlv_40= '<->' ( (otherlv_41= RULE_ID ) ) ) ) (otherlv_42= ':' ( (lv_name_43_0= RULE_ID ) ) )? (this_OPEN_44= RULE_OPEN (otherlv_45= 'implementationTechnology' (otherlv_46= '=' )? ( (lv_implementationTechnology_47_0= RULE_STRING ) ) )? this_CLOSE_48= RULE_CLOSE )? )
{
// InternalContextMappingDSL.g:1736:2: ( ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'SK' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'SK' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) ) | (otherlv_9= '[' otherlv_10= 'SK' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'SK' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) ) | ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'SK' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'SK' otherlv_26= ']' ) | (otherlv_27= '[' otherlv_28= 'SK' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'SK' otherlv_35= ']' ) | ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Shared-Kernel' ( (otherlv_38= RULE_ID ) ) ) | ( ( (otherlv_39= RULE_ID ) ) otherlv_40= '<->' ( (otherlv_41= RULE_ID ) ) ) ) (otherlv_42= ':' ( (lv_name_43_0= RULE_ID ) ) )? (this_OPEN_44= RULE_OPEN (otherlv_45= 'implementationTechnology' (otherlv_46= '=' )? ( (lv_implementationTechnology_47_0= RULE_STRING ) ) )? this_CLOSE_48= RULE_CLOSE )? )
// InternalContextMappingDSL.g:1737:3: ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'SK' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'SK' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) ) | (otherlv_9= '[' otherlv_10= 'SK' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'SK' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) ) | ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'SK' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'SK' otherlv_26= ']' ) | (otherlv_27= '[' otherlv_28= 'SK' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'SK' otherlv_35= ']' ) | ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Shared-Kernel' ( (otherlv_38= RULE_ID ) ) ) | ( ( (otherlv_39= RULE_ID ) ) otherlv_40= '<->' ( (otherlv_41= RULE_ID ) ) ) ) (otherlv_42= ':' ( (lv_name_43_0= RULE_ID ) ) )? (this_OPEN_44= RULE_OPEN (otherlv_45= 'implementationTechnology' (otherlv_46= '=' )? ( (lv_implementationTechnology_47_0= RULE_STRING ) ) )? this_CLOSE_48= RULE_CLOSE )?
{
// InternalContextMappingDSL.g:1737:3: ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'SK' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'SK' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) ) | (otherlv_9= '[' otherlv_10= 'SK' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'SK' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) ) | ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'SK' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'SK' otherlv_26= ']' ) | (otherlv_27= '[' otherlv_28= 'SK' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'SK' otherlv_35= ']' ) | ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Shared-Kernel' ( (otherlv_38= RULE_ID ) ) ) | ( ( (otherlv_39= RULE_ID ) ) otherlv_40= '<->' ( (otherlv_41= RULE_ID ) ) ) )
int alt44=6;
alt44 = dfa44.predict(input);
switch (alt44) {
case 1 :
// InternalContextMappingDSL.g:1738:4: ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'SK' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'SK' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:1738:4: ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'SK' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'SK' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) )
// InternalContextMappingDSL.g:1739:5: ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'SK' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'SK' otherlv_7= ']' ( (otherlv_8= RULE_ID ) )
{
// InternalContextMappingDSL.g:1739:5: ( (otherlv_0= RULE_ID ) )
// InternalContextMappingDSL.g:1740:6: (otherlv_0= RULE_ID )
{
// InternalContextMappingDSL.g:1740:6: (otherlv_0= RULE_ID )
// InternalContextMappingDSL.g:1741:7: otherlv_0= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getSharedKernelRule());
}
otherlv_0=(Token)match(input,RULE_ID,FOLLOW_29);
newLeafNode(otherlv_0, grammarAccess.getSharedKernelAccess().getParticipant1BoundedContextCrossReference_0_0_0_0());
}
}
otherlv_1=(Token)match(input,35,FOLLOW_37);
newLeafNode(otherlv_1, grammarAccess.getSharedKernelAccess().getLeftSquareBracketKeyword_0_0_1());
otherlv_2=(Token)match(input,41,FOLLOW_31);
newLeafNode(otherlv_2, grammarAccess.getSharedKernelAccess().getSKKeyword_0_0_2());
otherlv_3=(Token)match(input,37,FOLLOW_32);
newLeafNode(otherlv_3, grammarAccess.getSharedKernelAccess().getRightSquareBracketKeyword_0_0_3());
otherlv_4=(Token)match(input,38,FOLLOW_29);
newLeafNode(otherlv_4, grammarAccess.getSharedKernelAccess().getLessThanSignHyphenMinusGreaterThanSignKeyword_0_0_4());
otherlv_5=(Token)match(input,35,FOLLOW_37);
newLeafNode(otherlv_5, grammarAccess.getSharedKernelAccess().getLeftSquareBracketKeyword_0_0_5());
otherlv_6=(Token)match(input,41,FOLLOW_31);
newLeafNode(otherlv_6, grammarAccess.getSharedKernelAccess().getSKKeyword_0_0_6());
otherlv_7=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_7, grammarAccess.getSharedKernelAccess().getRightSquareBracketKeyword_0_0_7());
// InternalContextMappingDSL.g:1780:5: ( (otherlv_8= RULE_ID ) )
// InternalContextMappingDSL.g:1781:6: (otherlv_8= RULE_ID )
{
// InternalContextMappingDSL.g:1781:6: (otherlv_8= RULE_ID )
// InternalContextMappingDSL.g:1782:7: otherlv_8= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getSharedKernelRule());
}
otherlv_8=(Token)match(input,RULE_ID,FOLLOW_33);
newLeafNode(otherlv_8, grammarAccess.getSharedKernelAccess().getParticipant2BoundedContextCrossReference_0_0_8_0());
}
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:1795:4: (otherlv_9= '[' otherlv_10= 'SK' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'SK' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:1795:4: (otherlv_9= '[' otherlv_10= 'SK' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'SK' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) )
// InternalContextMappingDSL.g:1796:5: otherlv_9= '[' otherlv_10= 'SK' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'SK' otherlv_16= ']' ( (otherlv_17= RULE_ID ) )
{
otherlv_9=(Token)match(input,35,FOLLOW_37);
newLeafNode(otherlv_9, grammarAccess.getSharedKernelAccess().getLeftSquareBracketKeyword_0_1_0());
otherlv_10=(Token)match(input,41,FOLLOW_31);
newLeafNode(otherlv_10, grammarAccess.getSharedKernelAccess().getSKKeyword_0_1_1());
otherlv_11=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_11, grammarAccess.getSharedKernelAccess().getRightSquareBracketKeyword_0_1_2());
// InternalContextMappingDSL.g:1808:5: ( (otherlv_12= RULE_ID ) )
// InternalContextMappingDSL.g:1809:6: (otherlv_12= RULE_ID )
{
// InternalContextMappingDSL.g:1809:6: (otherlv_12= RULE_ID )
// InternalContextMappingDSL.g:1810:7: otherlv_12= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getSharedKernelRule());
}
otherlv_12=(Token)match(input,RULE_ID,FOLLOW_32);
newLeafNode(otherlv_12, grammarAccess.getSharedKernelAccess().getParticipant1BoundedContextCrossReference_0_1_3_0());
}
}
otherlv_13=(Token)match(input,38,FOLLOW_29);
newLeafNode(otherlv_13, grammarAccess.getSharedKernelAccess().getLessThanSignHyphenMinusGreaterThanSignKeyword_0_1_4());
otherlv_14=(Token)match(input,35,FOLLOW_37);
newLeafNode(otherlv_14, grammarAccess.getSharedKernelAccess().getLeftSquareBracketKeyword_0_1_5());
otherlv_15=(Token)match(input,41,FOLLOW_31);
newLeafNode(otherlv_15, grammarAccess.getSharedKernelAccess().getSKKeyword_0_1_6());
otherlv_16=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_16, grammarAccess.getSharedKernelAccess().getRightSquareBracketKeyword_0_1_7());
// InternalContextMappingDSL.g:1837:5: ( (otherlv_17= RULE_ID ) )
// InternalContextMappingDSL.g:1838:6: (otherlv_17= RULE_ID )
{
// InternalContextMappingDSL.g:1838:6: (otherlv_17= RULE_ID )
// InternalContextMappingDSL.g:1839:7: otherlv_17= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getSharedKernelRule());
}
otherlv_17=(Token)match(input,RULE_ID,FOLLOW_33);
newLeafNode(otherlv_17, grammarAccess.getSharedKernelAccess().getParticipant2BoundedContextCrossReference_0_1_8_0());
}
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:1852:4: ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'SK' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'SK' otherlv_26= ']' )
{
// InternalContextMappingDSL.g:1852:4: ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'SK' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'SK' otherlv_26= ']' )
// InternalContextMappingDSL.g:1853:5: ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'SK' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'SK' otherlv_26= ']'
{
// InternalContextMappingDSL.g:1853:5: ( (otherlv_18= RULE_ID ) )
// InternalContextMappingDSL.g:1854:6: (otherlv_18= RULE_ID )
{
// InternalContextMappingDSL.g:1854:6: (otherlv_18= RULE_ID )
// InternalContextMappingDSL.g:1855:7: otherlv_18= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getSharedKernelRule());
}
otherlv_18=(Token)match(input,RULE_ID,FOLLOW_29);
newLeafNode(otherlv_18, grammarAccess.getSharedKernelAccess().getParticipant1BoundedContextCrossReference_0_2_0_0());
}
}
otherlv_19=(Token)match(input,35,FOLLOW_37);
newLeafNode(otherlv_19, grammarAccess.getSharedKernelAccess().getLeftSquareBracketKeyword_0_2_1());
otherlv_20=(Token)match(input,41,FOLLOW_31);
newLeafNode(otherlv_20, grammarAccess.getSharedKernelAccess().getSKKeyword_0_2_2());
otherlv_21=(Token)match(input,37,FOLLOW_32);
newLeafNode(otherlv_21, grammarAccess.getSharedKernelAccess().getRightSquareBracketKeyword_0_2_3());
otherlv_22=(Token)match(input,38,FOLLOW_10);
newLeafNode(otherlv_22, grammarAccess.getSharedKernelAccess().getLessThanSignHyphenMinusGreaterThanSignKeyword_0_2_4());
// InternalContextMappingDSL.g:1882:5: ( (otherlv_23= RULE_ID ) )
// InternalContextMappingDSL.g:1883:6: (otherlv_23= RULE_ID )
{
// InternalContextMappingDSL.g:1883:6: (otherlv_23= RULE_ID )
// InternalContextMappingDSL.g:1884:7: otherlv_23= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getSharedKernelRule());
}
otherlv_23=(Token)match(input,RULE_ID,FOLLOW_29);
newLeafNode(otherlv_23, grammarAccess.getSharedKernelAccess().getParticipant2BoundedContextCrossReference_0_2_5_0());
}
}
otherlv_24=(Token)match(input,35,FOLLOW_37);
newLeafNode(otherlv_24, grammarAccess.getSharedKernelAccess().getLeftSquareBracketKeyword_0_2_6());
otherlv_25=(Token)match(input,41,FOLLOW_31);
newLeafNode(otherlv_25, grammarAccess.getSharedKernelAccess().getSKKeyword_0_2_7());
otherlv_26=(Token)match(input,37,FOLLOW_33);
newLeafNode(otherlv_26, grammarAccess.getSharedKernelAccess().getRightSquareBracketKeyword_0_2_8());
}
}
break;
case 4 :
// InternalContextMappingDSL.g:1909:4: (otherlv_27= '[' otherlv_28= 'SK' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'SK' otherlv_35= ']' )
{
// InternalContextMappingDSL.g:1909:4: (otherlv_27= '[' otherlv_28= 'SK' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'SK' otherlv_35= ']' )
// InternalContextMappingDSL.g:1910:5: otherlv_27= '[' otherlv_28= 'SK' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'SK' otherlv_35= ']'
{
otherlv_27=(Token)match(input,35,FOLLOW_37);
newLeafNode(otherlv_27, grammarAccess.getSharedKernelAccess().getLeftSquareBracketKeyword_0_3_0());
otherlv_28=(Token)match(input,41,FOLLOW_31);
newLeafNode(otherlv_28, grammarAccess.getSharedKernelAccess().getSKKeyword_0_3_1());
otherlv_29=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_29, grammarAccess.getSharedKernelAccess().getRightSquareBracketKeyword_0_3_2());
// InternalContextMappingDSL.g:1922:5: ( (otherlv_30= RULE_ID ) )
// InternalContextMappingDSL.g:1923:6: (otherlv_30= RULE_ID )
{
// InternalContextMappingDSL.g:1923:6: (otherlv_30= RULE_ID )
// InternalContextMappingDSL.g:1924:7: otherlv_30= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getSharedKernelRule());
}
otherlv_30=(Token)match(input,RULE_ID,FOLLOW_32);
newLeafNode(otherlv_30, grammarAccess.getSharedKernelAccess().getParticipant1BoundedContextCrossReference_0_3_3_0());
}
}
otherlv_31=(Token)match(input,38,FOLLOW_10);
newLeafNode(otherlv_31, grammarAccess.getSharedKernelAccess().getLessThanSignHyphenMinusGreaterThanSignKeyword_0_3_4());
// InternalContextMappingDSL.g:1939:5: ( (otherlv_32= RULE_ID ) )
// InternalContextMappingDSL.g:1940:6: (otherlv_32= RULE_ID )
{
// InternalContextMappingDSL.g:1940:6: (otherlv_32= RULE_ID )
// InternalContextMappingDSL.g:1941:7: otherlv_32= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getSharedKernelRule());
}
otherlv_32=(Token)match(input,RULE_ID,FOLLOW_29);
newLeafNode(otherlv_32, grammarAccess.getSharedKernelAccess().getParticipant2BoundedContextCrossReference_0_3_5_0());
}
}
otherlv_33=(Token)match(input,35,FOLLOW_37);
newLeafNode(otherlv_33, grammarAccess.getSharedKernelAccess().getLeftSquareBracketKeyword_0_3_6());
otherlv_34=(Token)match(input,41,FOLLOW_31);
newLeafNode(otherlv_34, grammarAccess.getSharedKernelAccess().getSKKeyword_0_3_7());
otherlv_35=(Token)match(input,37,FOLLOW_33);
newLeafNode(otherlv_35, grammarAccess.getSharedKernelAccess().getRightSquareBracketKeyword_0_3_8());
}
}
break;
case 5 :
// InternalContextMappingDSL.g:1966:4: ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Shared-Kernel' ( (otherlv_38= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:1966:4: ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Shared-Kernel' ( (otherlv_38= RULE_ID ) ) )
// InternalContextMappingDSL.g:1967:5: ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Shared-Kernel' ( (otherlv_38= RULE_ID ) )
{
// InternalContextMappingDSL.g:1967:5: ( (otherlv_36= RULE_ID ) )
// InternalContextMappingDSL.g:1968:6: (otherlv_36= RULE_ID )
{
// InternalContextMappingDSL.g:1968:6: (otherlv_36= RULE_ID )
// InternalContextMappingDSL.g:1969:7: otherlv_36= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getSharedKernelRule());
}
otherlv_36=(Token)match(input,RULE_ID,FOLLOW_38);
newLeafNode(otherlv_36, grammarAccess.getSharedKernelAccess().getParticipant1BoundedContextCrossReference_0_4_0_0());
}
}
otherlv_37=(Token)match(input,42,FOLLOW_10);
newLeafNode(otherlv_37, grammarAccess.getSharedKernelAccess().getSharedKernelKeyword_0_4_1());
// InternalContextMappingDSL.g:1984:5: ( (otherlv_38= RULE_ID ) )
// InternalContextMappingDSL.g:1985:6: (otherlv_38= RULE_ID )
{
// InternalContextMappingDSL.g:1985:6: (otherlv_38= RULE_ID )
// InternalContextMappingDSL.g:1986:7: otherlv_38= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getSharedKernelRule());
}
otherlv_38=(Token)match(input,RULE_ID,FOLLOW_33);
newLeafNode(otherlv_38, grammarAccess.getSharedKernelAccess().getParticipant2BoundedContextCrossReference_0_4_2_0());
}
}
}
}
break;
case 6 :
// InternalContextMappingDSL.g:1999:4: ( ( (otherlv_39= RULE_ID ) ) otherlv_40= '<->' ( (otherlv_41= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:1999:4: ( ( (otherlv_39= RULE_ID ) ) otherlv_40= '<->' ( (otherlv_41= RULE_ID ) ) )
// InternalContextMappingDSL.g:2000:5: ( (otherlv_39= RULE_ID ) ) otherlv_40= '<->' ( (otherlv_41= RULE_ID ) )
{
// InternalContextMappingDSL.g:2000:5: ( (otherlv_39= RULE_ID ) )
// InternalContextMappingDSL.g:2001:6: (otherlv_39= RULE_ID )
{
// InternalContextMappingDSL.g:2001:6: (otherlv_39= RULE_ID )
// InternalContextMappingDSL.g:2002:7: otherlv_39= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getSharedKernelRule());
}
otherlv_39=(Token)match(input,RULE_ID,FOLLOW_32);
newLeafNode(otherlv_39, grammarAccess.getSharedKernelAccess().getParticipant1BoundedContextCrossReference_0_5_0_0());
}
}
otherlv_40=(Token)match(input,38,FOLLOW_10);
newLeafNode(otherlv_40, grammarAccess.getSharedKernelAccess().getLessThanSignHyphenMinusGreaterThanSignKeyword_0_5_1());
// InternalContextMappingDSL.g:2017:5: ( (otherlv_41= RULE_ID ) )
// InternalContextMappingDSL.g:2018:6: (otherlv_41= RULE_ID )
{
// InternalContextMappingDSL.g:2018:6: (otherlv_41= RULE_ID )
// InternalContextMappingDSL.g:2019:7: otherlv_41= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getSharedKernelRule());
}
otherlv_41=(Token)match(input,RULE_ID,FOLLOW_33);
newLeafNode(otherlv_41, grammarAccess.getSharedKernelAccess().getParticipant2BoundedContextCrossReference_0_5_2_0());
}
}
}
}
break;
}
// InternalContextMappingDSL.g:2032:3: (otherlv_42= ':' ( (lv_name_43_0= RULE_ID ) ) )?
int alt45=2;
int LA45_0 = input.LA(1);
if ( (LA45_0==40) ) {
alt45=1;
}
switch (alt45) {
case 1 :
// InternalContextMappingDSL.g:2033:4: otherlv_42= ':' ( (lv_name_43_0= RULE_ID ) )
{
otherlv_42=(Token)match(input,40,FOLLOW_10);
newLeafNode(otherlv_42, grammarAccess.getSharedKernelAccess().getColonKeyword_1_0());
// InternalContextMappingDSL.g:2037:4: ( (lv_name_43_0= RULE_ID ) )
// InternalContextMappingDSL.g:2038:5: (lv_name_43_0= RULE_ID )
{
// InternalContextMappingDSL.g:2038:5: (lv_name_43_0= RULE_ID )
// InternalContextMappingDSL.g:2039:6: lv_name_43_0= RULE_ID
{
lv_name_43_0=(Token)match(input,RULE_ID,FOLLOW_23);
newLeafNode(lv_name_43_0, grammarAccess.getSharedKernelAccess().getNameIDTerminalRuleCall_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getSharedKernelRule());
}
setWithLastConsumed(
current,
"name",
lv_name_43_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
}
break;
}
// InternalContextMappingDSL.g:2056:3: (this_OPEN_44= RULE_OPEN (otherlv_45= 'implementationTechnology' (otherlv_46= '=' )? ( (lv_implementationTechnology_47_0= RULE_STRING ) ) )? this_CLOSE_48= RULE_CLOSE )?
int alt48=2;
int LA48_0 = input.LA(1);
if ( (LA48_0==RULE_OPEN) ) {
alt48=1;
}
switch (alt48) {
case 1 :
// InternalContextMappingDSL.g:2057:4: this_OPEN_44= RULE_OPEN (otherlv_45= 'implementationTechnology' (otherlv_46= '=' )? ( (lv_implementationTechnology_47_0= RULE_STRING ) ) )? this_CLOSE_48= RULE_CLOSE
{
this_OPEN_44=(Token)match(input,RULE_OPEN,FOLLOW_35);
newLeafNode(this_OPEN_44, grammarAccess.getSharedKernelAccess().getOPENTerminalRuleCall_2_0());
// InternalContextMappingDSL.g:2061:4: (otherlv_45= 'implementationTechnology' (otherlv_46= '=' )? ( (lv_implementationTechnology_47_0= RULE_STRING ) ) )?
int alt47=2;
int LA47_0 = input.LA(1);
if ( (LA47_0==31) ) {
alt47=1;
}
switch (alt47) {
case 1 :
// InternalContextMappingDSL.g:2062:5: otherlv_45= 'implementationTechnology' (otherlv_46= '=' )? ( (lv_implementationTechnology_47_0= RULE_STRING ) )
{
otherlv_45=(Token)match(input,31,FOLLOW_17);
newLeafNode(otherlv_45, grammarAccess.getSharedKernelAccess().getImplementationTechnologyKeyword_2_1_0());
// InternalContextMappingDSL.g:2066:5: (otherlv_46= '=' )?
int alt46=2;
int LA46_0 = input.LA(1);
if ( (LA46_0==21) ) {
alt46=1;
}
switch (alt46) {
case 1 :
// InternalContextMappingDSL.g:2067:6: otherlv_46= '='
{
otherlv_46=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_46, grammarAccess.getSharedKernelAccess().getEqualsSignKeyword_2_1_1());
}
break;
}
// InternalContextMappingDSL.g:2072:5: ( (lv_implementationTechnology_47_0= RULE_STRING ) )
// InternalContextMappingDSL.g:2073:6: (lv_implementationTechnology_47_0= RULE_STRING )
{
// InternalContextMappingDSL.g:2073:6: (lv_implementationTechnology_47_0= RULE_STRING )
// InternalContextMappingDSL.g:2074:7: lv_implementationTechnology_47_0= RULE_STRING
{
lv_implementationTechnology_47_0=(Token)match(input,RULE_STRING,FOLLOW_36);
newLeafNode(lv_implementationTechnology_47_0, grammarAccess.getSharedKernelAccess().getImplementationTechnologySTRINGTerminalRuleCall_2_1_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getSharedKernelRule());
}
setWithLastConsumed(
current,
"implementationTechnology",
lv_implementationTechnology_47_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
this_CLOSE_48=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(this_CLOSE_48, grammarAccess.getSharedKernelAccess().getCLOSETerminalRuleCall_2_2());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleSharedKernel"
// $ANTLR start "entryRuleUpstreamDownstreamRelationship"
// InternalContextMappingDSL.g:2100:1: entryRuleUpstreamDownstreamRelationship returns [EObject current=null] : iv_ruleUpstreamDownstreamRelationship= ruleUpstreamDownstreamRelationship EOF ;
public final EObject entryRuleUpstreamDownstreamRelationship() throws RecognitionException {
EObject current = null;
EObject iv_ruleUpstreamDownstreamRelationship = null;
try {
// InternalContextMappingDSL.g:2100:71: (iv_ruleUpstreamDownstreamRelationship= ruleUpstreamDownstreamRelationship EOF )
// InternalContextMappingDSL.g:2101:2: iv_ruleUpstreamDownstreamRelationship= ruleUpstreamDownstreamRelationship EOF
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipRule());
pushFollow(FOLLOW_1);
iv_ruleUpstreamDownstreamRelationship=ruleUpstreamDownstreamRelationship();
state._fsp--;
current =iv_ruleUpstreamDownstreamRelationship;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleUpstreamDownstreamRelationship"
// $ANTLR start "ruleUpstreamDownstreamRelationship"
// InternalContextMappingDSL.g:2107:1: ruleUpstreamDownstreamRelationship returns [EObject current=null] : (this_CustomerSupplierRelationship_0= ruleCustomerSupplierRelationship | ( ( ( ( (otherlv_1= RULE_ID ) ) ( (otherlv_2= '[' otherlv_3= 'U' otherlv_4= ']' ) | ( (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* ) otherlv_11= ']' ) )? otherlv_12= '->' ( (otherlv_13= '[' otherlv_14= 'D' otherlv_15= ']' ) | ( (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* ) otherlv_22= ']' ) )? ( (otherlv_23= RULE_ID ) ) ) | ( ( (otherlv_24= RULE_ID ) ) ( (otherlv_25= '[' otherlv_26= 'D' otherlv_27= ']' ) | ( (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* ) otherlv_34= ']' ) )? otherlv_35= '<-' ( (otherlv_36= '[' otherlv_37= 'U' otherlv_38= ']' ) | ( (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* ) otherlv_45= ']' ) )? ( (otherlv_46= RULE_ID ) ) ) | ( ( (otherlv_47= RULE_ID ) ) (otherlv_48= '[' ( ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) ) (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )* )? otherlv_52= ']' )? otherlv_53= 'Upstream-Downstream' (otherlv_54= '[' ( ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) ) (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )* )? otherlv_58= ']' )? ( (otherlv_59= RULE_ID ) ) ) | ( ( (otherlv_60= RULE_ID ) ) (otherlv_61= '[' ( ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) ) (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )* )? otherlv_65= ']' )? otherlv_66= 'Downstream-Upstream' (otherlv_67= '[' ( ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) ) (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )* )? otherlv_71= ']' )? ( (otherlv_72= RULE_ID ) ) ) ) (otherlv_73= ':' ( (lv_name_74_0= RULE_ID ) ) )? (this_OPEN_75= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) ) this_CLOSE_89= RULE_CLOSE )? ) ) ;
public final EObject ruleUpstreamDownstreamRelationship() throws RecognitionException {
EObject current = null;
Token otherlv_1=null;
Token otherlv_2=null;
Token otherlv_3=null;
Token otherlv_4=null;
Token otherlv_5=null;
Token otherlv_6=null;
Token otherlv_7=null;
Token otherlv_9=null;
Token otherlv_11=null;
Token otherlv_12=null;
Token otherlv_13=null;
Token otherlv_14=null;
Token otherlv_15=null;
Token otherlv_16=null;
Token otherlv_17=null;
Token otherlv_18=null;
Token otherlv_20=null;
Token otherlv_22=null;
Token otherlv_23=null;
Token otherlv_24=null;
Token otherlv_25=null;
Token otherlv_26=null;
Token otherlv_27=null;
Token otherlv_28=null;
Token otherlv_29=null;
Token otherlv_30=null;
Token otherlv_32=null;
Token otherlv_34=null;
Token otherlv_35=null;
Token otherlv_36=null;
Token otherlv_37=null;
Token otherlv_38=null;
Token otherlv_39=null;
Token otherlv_40=null;
Token otherlv_41=null;
Token otherlv_43=null;
Token otherlv_45=null;
Token otherlv_46=null;
Token otherlv_47=null;
Token otherlv_48=null;
Token otherlv_50=null;
Token otherlv_52=null;
Token otherlv_53=null;
Token otherlv_54=null;
Token otherlv_56=null;
Token otherlv_58=null;
Token otherlv_59=null;
Token otherlv_60=null;
Token otherlv_61=null;
Token otherlv_63=null;
Token otherlv_65=null;
Token otherlv_66=null;
Token otherlv_67=null;
Token otherlv_69=null;
Token otherlv_71=null;
Token otherlv_72=null;
Token otherlv_73=null;
Token lv_name_74_0=null;
Token this_OPEN_75=null;
Token otherlv_77=null;
Token otherlv_78=null;
Token lv_implementationTechnology_79_0=null;
Token otherlv_80=null;
Token otherlv_81=null;
Token otherlv_82=null;
Token otherlv_83=null;
Token otherlv_84=null;
Token lv_exposedAggregatesComment_85_0=null;
Token otherlv_86=null;
Token otherlv_87=null;
Token this_CLOSE_89=null;
EObject this_CustomerSupplierRelationship_0 = null;
Enumerator lv_upstreamRoles_8_0 = null;
Enumerator lv_upstreamRoles_10_0 = null;
Enumerator lv_downstreamRoles_19_0 = null;
Enumerator lv_downstreamRoles_21_0 = null;
Enumerator lv_downstreamRoles_31_0 = null;
Enumerator lv_downstreamRoles_33_0 = null;
Enumerator lv_upstreamRoles_42_0 = null;
Enumerator lv_upstreamRoles_44_0 = null;
Enumerator lv_upstreamRoles_49_0 = null;
Enumerator lv_upstreamRoles_51_0 = null;
Enumerator lv_downstreamRoles_55_0 = null;
Enumerator lv_downstreamRoles_57_0 = null;
Enumerator lv_downstreamRoles_62_0 = null;
Enumerator lv_downstreamRoles_64_0 = null;
Enumerator lv_upstreamRoles_68_0 = null;
Enumerator lv_upstreamRoles_70_0 = null;
Enumerator lv_downstreamGovernanceRights_88_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:2113:2: ( (this_CustomerSupplierRelationship_0= ruleCustomerSupplierRelationship | ( ( ( ( (otherlv_1= RULE_ID ) ) ( (otherlv_2= '[' otherlv_3= 'U' otherlv_4= ']' ) | ( (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* ) otherlv_11= ']' ) )? otherlv_12= '->' ( (otherlv_13= '[' otherlv_14= 'D' otherlv_15= ']' ) | ( (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* ) otherlv_22= ']' ) )? ( (otherlv_23= RULE_ID ) ) ) | ( ( (otherlv_24= RULE_ID ) ) ( (otherlv_25= '[' otherlv_26= 'D' otherlv_27= ']' ) | ( (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* ) otherlv_34= ']' ) )? otherlv_35= '<-' ( (otherlv_36= '[' otherlv_37= 'U' otherlv_38= ']' ) | ( (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* ) otherlv_45= ']' ) )? ( (otherlv_46= RULE_ID ) ) ) | ( ( (otherlv_47= RULE_ID ) ) (otherlv_48= '[' ( ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) ) (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )* )? otherlv_52= ']' )? otherlv_53= 'Upstream-Downstream' (otherlv_54= '[' ( ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) ) (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )* )? otherlv_58= ']' )? ( (otherlv_59= RULE_ID ) ) ) | ( ( (otherlv_60= RULE_ID ) ) (otherlv_61= '[' ( ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) ) (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )* )? otherlv_65= ']' )? otherlv_66= 'Downstream-Upstream' (otherlv_67= '[' ( ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) ) (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )* )? otherlv_71= ']' )? ( (otherlv_72= RULE_ID ) ) ) ) (otherlv_73= ':' ( (lv_name_74_0= RULE_ID ) ) )? (this_OPEN_75= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) ) this_CLOSE_89= RULE_CLOSE )? ) ) )
// InternalContextMappingDSL.g:2114:2: (this_CustomerSupplierRelationship_0= ruleCustomerSupplierRelationship | ( ( ( ( (otherlv_1= RULE_ID ) ) ( (otherlv_2= '[' otherlv_3= 'U' otherlv_4= ']' ) | ( (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* ) otherlv_11= ']' ) )? otherlv_12= '->' ( (otherlv_13= '[' otherlv_14= 'D' otherlv_15= ']' ) | ( (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* ) otherlv_22= ']' ) )? ( (otherlv_23= RULE_ID ) ) ) | ( ( (otherlv_24= RULE_ID ) ) ( (otherlv_25= '[' otherlv_26= 'D' otherlv_27= ']' ) | ( (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* ) otherlv_34= ']' ) )? otherlv_35= '<-' ( (otherlv_36= '[' otherlv_37= 'U' otherlv_38= ']' ) | ( (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* ) otherlv_45= ']' ) )? ( (otherlv_46= RULE_ID ) ) ) | ( ( (otherlv_47= RULE_ID ) ) (otherlv_48= '[' ( ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) ) (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )* )? otherlv_52= ']' )? otherlv_53= 'Upstream-Downstream' (otherlv_54= '[' ( ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) ) (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )* )? otherlv_58= ']' )? ( (otherlv_59= RULE_ID ) ) ) | ( ( (otherlv_60= RULE_ID ) ) (otherlv_61= '[' ( ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) ) (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )* )? otherlv_65= ']' )? otherlv_66= 'Downstream-Upstream' (otherlv_67= '[' ( ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) ) (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )* )? otherlv_71= ']' )? ( (otherlv_72= RULE_ID ) ) ) ) (otherlv_73= ':' ( (lv_name_74_0= RULE_ID ) ) )? (this_OPEN_75= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) ) this_CLOSE_89= RULE_CLOSE )? ) )
{
// InternalContextMappingDSL.g:2114:2: (this_CustomerSupplierRelationship_0= ruleCustomerSupplierRelationship | ( ( ( ( (otherlv_1= RULE_ID ) ) ( (otherlv_2= '[' otherlv_3= 'U' otherlv_4= ']' ) | ( (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* ) otherlv_11= ']' ) )? otherlv_12= '->' ( (otherlv_13= '[' otherlv_14= 'D' otherlv_15= ']' ) | ( (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* ) otherlv_22= ']' ) )? ( (otherlv_23= RULE_ID ) ) ) | ( ( (otherlv_24= RULE_ID ) ) ( (otherlv_25= '[' otherlv_26= 'D' otherlv_27= ']' ) | ( (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* ) otherlv_34= ']' ) )? otherlv_35= '<-' ( (otherlv_36= '[' otherlv_37= 'U' otherlv_38= ']' ) | ( (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* ) otherlv_45= ']' ) )? ( (otherlv_46= RULE_ID ) ) ) | ( ( (otherlv_47= RULE_ID ) ) (otherlv_48= '[' ( ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) ) (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )* )? otherlv_52= ']' )? otherlv_53= 'Upstream-Downstream' (otherlv_54= '[' ( ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) ) (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )* )? otherlv_58= ']' )? ( (otherlv_59= RULE_ID ) ) ) | ( ( (otherlv_60= RULE_ID ) ) (otherlv_61= '[' ( ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) ) (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )* )? otherlv_65= ']' )? otherlv_66= 'Downstream-Upstream' (otherlv_67= '[' ( ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) ) (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )* )? otherlv_71= ']' )? ( (otherlv_72= RULE_ID ) ) ) ) (otherlv_73= ':' ( (lv_name_74_0= RULE_ID ) ) )? (this_OPEN_75= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) ) this_CLOSE_89= RULE_CLOSE )? ) )
int alt82=2;
alt82 = dfa82.predict(input);
switch (alt82) {
case 1 :
// InternalContextMappingDSL.g:2115:3: this_CustomerSupplierRelationship_0= ruleCustomerSupplierRelationship
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getCustomerSupplierRelationshipParserRuleCall_0());
pushFollow(FOLLOW_2);
this_CustomerSupplierRelationship_0=ruleCustomerSupplierRelationship();
state._fsp--;
current = this_CustomerSupplierRelationship_0;
afterParserOrEnumRuleCall();
}
break;
case 2 :
// InternalContextMappingDSL.g:2124:3: ( ( ( ( (otherlv_1= RULE_ID ) ) ( (otherlv_2= '[' otherlv_3= 'U' otherlv_4= ']' ) | ( (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* ) otherlv_11= ']' ) )? otherlv_12= '->' ( (otherlv_13= '[' otherlv_14= 'D' otherlv_15= ']' ) | ( (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* ) otherlv_22= ']' ) )? ( (otherlv_23= RULE_ID ) ) ) | ( ( (otherlv_24= RULE_ID ) ) ( (otherlv_25= '[' otherlv_26= 'D' otherlv_27= ']' ) | ( (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* ) otherlv_34= ']' ) )? otherlv_35= '<-' ( (otherlv_36= '[' otherlv_37= 'U' otherlv_38= ']' ) | ( (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* ) otherlv_45= ']' ) )? ( (otherlv_46= RULE_ID ) ) ) | ( ( (otherlv_47= RULE_ID ) ) (otherlv_48= '[' ( ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) ) (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )* )? otherlv_52= ']' )? otherlv_53= 'Upstream-Downstream' (otherlv_54= '[' ( ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) ) (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )* )? otherlv_58= ']' )? ( (otherlv_59= RULE_ID ) ) ) | ( ( (otherlv_60= RULE_ID ) ) (otherlv_61= '[' ( ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) ) (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )* )? otherlv_65= ']' )? otherlv_66= 'Downstream-Upstream' (otherlv_67= '[' ( ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) ) (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )* )? otherlv_71= ']' )? ( (otherlv_72= RULE_ID ) ) ) ) (otherlv_73= ':' ( (lv_name_74_0= RULE_ID ) ) )? (this_OPEN_75= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) ) this_CLOSE_89= RULE_CLOSE )? )
{
// InternalContextMappingDSL.g:2124:3: ( ( ( ( (otherlv_1= RULE_ID ) ) ( (otherlv_2= '[' otherlv_3= 'U' otherlv_4= ']' ) | ( (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* ) otherlv_11= ']' ) )? otherlv_12= '->' ( (otherlv_13= '[' otherlv_14= 'D' otherlv_15= ']' ) | ( (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* ) otherlv_22= ']' ) )? ( (otherlv_23= RULE_ID ) ) ) | ( ( (otherlv_24= RULE_ID ) ) ( (otherlv_25= '[' otherlv_26= 'D' otherlv_27= ']' ) | ( (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* ) otherlv_34= ']' ) )? otherlv_35= '<-' ( (otherlv_36= '[' otherlv_37= 'U' otherlv_38= ']' ) | ( (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* ) otherlv_45= ']' ) )? ( (otherlv_46= RULE_ID ) ) ) | ( ( (otherlv_47= RULE_ID ) ) (otherlv_48= '[' ( ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) ) (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )* )? otherlv_52= ']' )? otherlv_53= 'Upstream-Downstream' (otherlv_54= '[' ( ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) ) (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )* )? otherlv_58= ']' )? ( (otherlv_59= RULE_ID ) ) ) | ( ( (otherlv_60= RULE_ID ) ) (otherlv_61= '[' ( ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) ) (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )* )? otherlv_65= ']' )? otherlv_66= 'Downstream-Upstream' (otherlv_67= '[' ( ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) ) (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )* )? otherlv_71= ']' )? ( (otherlv_72= RULE_ID ) ) ) ) (otherlv_73= ':' ( (lv_name_74_0= RULE_ID ) ) )? (this_OPEN_75= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) ) this_CLOSE_89= RULE_CLOSE )? )
// InternalContextMappingDSL.g:2125:4: ( ( ( (otherlv_1= RULE_ID ) ) ( (otherlv_2= '[' otherlv_3= 'U' otherlv_4= ']' ) | ( (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* ) otherlv_11= ']' ) )? otherlv_12= '->' ( (otherlv_13= '[' otherlv_14= 'D' otherlv_15= ']' ) | ( (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* ) otherlv_22= ']' ) )? ( (otherlv_23= RULE_ID ) ) ) | ( ( (otherlv_24= RULE_ID ) ) ( (otherlv_25= '[' otherlv_26= 'D' otherlv_27= ']' ) | ( (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* ) otherlv_34= ']' ) )? otherlv_35= '<-' ( (otherlv_36= '[' otherlv_37= 'U' otherlv_38= ']' ) | ( (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* ) otherlv_45= ']' ) )? ( (otherlv_46= RULE_ID ) ) ) | ( ( (otherlv_47= RULE_ID ) ) (otherlv_48= '[' ( ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) ) (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )* )? otherlv_52= ']' )? otherlv_53= 'Upstream-Downstream' (otherlv_54= '[' ( ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) ) (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )* )? otherlv_58= ']' )? ( (otherlv_59= RULE_ID ) ) ) | ( ( (otherlv_60= RULE_ID ) ) (otherlv_61= '[' ( ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) ) (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )* )? otherlv_65= ']' )? otherlv_66= 'Downstream-Upstream' (otherlv_67= '[' ( ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) ) (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )* )? otherlv_71= ']' )? ( (otherlv_72= RULE_ID ) ) ) ) (otherlv_73= ':' ( (lv_name_74_0= RULE_ID ) ) )? (this_OPEN_75= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) ) this_CLOSE_89= RULE_CLOSE )?
{
// InternalContextMappingDSL.g:2125:4: ( ( ( (otherlv_1= RULE_ID ) ) ( (otherlv_2= '[' otherlv_3= 'U' otherlv_4= ']' ) | ( (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* ) otherlv_11= ']' ) )? otherlv_12= '->' ( (otherlv_13= '[' otherlv_14= 'D' otherlv_15= ']' ) | ( (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* ) otherlv_22= ']' ) )? ( (otherlv_23= RULE_ID ) ) ) | ( ( (otherlv_24= RULE_ID ) ) ( (otherlv_25= '[' otherlv_26= 'D' otherlv_27= ']' ) | ( (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* ) otherlv_34= ']' ) )? otherlv_35= '<-' ( (otherlv_36= '[' otherlv_37= 'U' otherlv_38= ']' ) | ( (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* ) otherlv_45= ']' ) )? ( (otherlv_46= RULE_ID ) ) ) | ( ( (otherlv_47= RULE_ID ) ) (otherlv_48= '[' ( ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) ) (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )* )? otherlv_52= ']' )? otherlv_53= 'Upstream-Downstream' (otherlv_54= '[' ( ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) ) (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )* )? otherlv_58= ']' )? ( (otherlv_59= RULE_ID ) ) ) | ( ( (otherlv_60= RULE_ID ) ) (otherlv_61= '[' ( ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) ) (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )* )? otherlv_65= ']' )? otherlv_66= 'Downstream-Upstream' (otherlv_67= '[' ( ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) ) (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )* )? otherlv_71= ']' )? ( (otherlv_72= RULE_ID ) ) ) )
int alt73=4;
alt73 = dfa73.predict(input);
switch (alt73) {
case 1 :
// InternalContextMappingDSL.g:2126:5: ( ( (otherlv_1= RULE_ID ) ) ( (otherlv_2= '[' otherlv_3= 'U' otherlv_4= ']' ) | ( (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* ) otherlv_11= ']' ) )? otherlv_12= '->' ( (otherlv_13= '[' otherlv_14= 'D' otherlv_15= ']' ) | ( (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* ) otherlv_22= ']' ) )? ( (otherlv_23= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:2126:5: ( ( (otherlv_1= RULE_ID ) ) ( (otherlv_2= '[' otherlv_3= 'U' otherlv_4= ']' ) | ( (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* ) otherlv_11= ']' ) )? otherlv_12= '->' ( (otherlv_13= '[' otherlv_14= 'D' otherlv_15= ']' ) | ( (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* ) otherlv_22= ']' ) )? ( (otherlv_23= RULE_ID ) ) )
// InternalContextMappingDSL.g:2127:6: ( (otherlv_1= RULE_ID ) ) ( (otherlv_2= '[' otherlv_3= 'U' otherlv_4= ']' ) | ( (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* ) otherlv_11= ']' ) )? otherlv_12= '->' ( (otherlv_13= '[' otherlv_14= 'D' otherlv_15= ']' ) | ( (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* ) otherlv_22= ']' ) )? ( (otherlv_23= RULE_ID ) )
{
// InternalContextMappingDSL.g:2127:6: ( (otherlv_1= RULE_ID ) )
// InternalContextMappingDSL.g:2128:7: (otherlv_1= RULE_ID )
{
// InternalContextMappingDSL.g:2128:7: (otherlv_1= RULE_ID )
// InternalContextMappingDSL.g:2129:8: otherlv_1= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
otherlv_1=(Token)match(input,RULE_ID,FOLLOW_39);
newLeafNode(otherlv_1, grammarAccess.getUpstreamDownstreamRelationshipAccess().getUpstreamBoundedContextCrossReference_1_0_0_0_0());
}
}
// InternalContextMappingDSL.g:2140:6: ( (otherlv_2= '[' otherlv_3= 'U' otherlv_4= ']' ) | ( (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* ) otherlv_11= ']' ) )?
int alt51=3;
int LA51_0 = input.LA(1);
if ( (LA51_0==35) ) {
int LA51_1 = input.LA(2);
if ( (LA51_1==43) ) {
int LA51_3 = input.LA(3);
if ( (LA51_3==24) ) {
alt51=2;
}
else if ( (LA51_3==37) ) {
alt51=1;
}
}
else if ( ((LA51_1>=208 && LA51_1<=209)) ) {
alt51=2;
}
}
switch (alt51) {
case 1 :
// InternalContextMappingDSL.g:2141:7: (otherlv_2= '[' otherlv_3= 'U' otherlv_4= ']' )
{
// InternalContextMappingDSL.g:2141:7: (otherlv_2= '[' otherlv_3= 'U' otherlv_4= ']' )
// InternalContextMappingDSL.g:2142:8: otherlv_2= '[' otherlv_3= 'U' otherlv_4= ']'
{
otherlv_2=(Token)match(input,35,FOLLOW_40);
newLeafNode(otherlv_2, grammarAccess.getUpstreamDownstreamRelationshipAccess().getLeftSquareBracketKeyword_1_0_0_1_0_0());
otherlv_3=(Token)match(input,43,FOLLOW_31);
newLeafNode(otherlv_3, grammarAccess.getUpstreamDownstreamRelationshipAccess().getUKeyword_1_0_0_1_0_1());
otherlv_4=(Token)match(input,37,FOLLOW_41);
newLeafNode(otherlv_4, grammarAccess.getUpstreamDownstreamRelationshipAccess().getRightSquareBracketKeyword_1_0_0_1_0_2());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:2156:7: ( (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* ) otherlv_11= ']' )
{
// InternalContextMappingDSL.g:2156:7: ( (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* ) otherlv_11= ']' )
// InternalContextMappingDSL.g:2157:8: (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* ) otherlv_11= ']'
{
// InternalContextMappingDSL.g:2157:8: (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* )
// InternalContextMappingDSL.g:2158:9: otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )*
{
otherlv_5=(Token)match(input,35,FOLLOW_42);
newLeafNode(otherlv_5, grammarAccess.getUpstreamDownstreamRelationshipAccess().getLeftSquareBracketKeyword_1_0_0_1_1_0_0());
// InternalContextMappingDSL.g:2162:9: (otherlv_6= 'U' otherlv_7= ',' )?
int alt49=2;
int LA49_0 = input.LA(1);
if ( (LA49_0==43) ) {
alt49=1;
}
switch (alt49) {
case 1 :
// InternalContextMappingDSL.g:2163:10: otherlv_6= 'U' otherlv_7= ','
{
otherlv_6=(Token)match(input,43,FOLLOW_43);
newLeafNode(otherlv_6, grammarAccess.getUpstreamDownstreamRelationshipAccess().getUKeyword_1_0_0_1_1_0_1_0());
otherlv_7=(Token)match(input,24,FOLLOW_42);
newLeafNode(otherlv_7, grammarAccess.getUpstreamDownstreamRelationshipAccess().getCommaKeyword_1_0_0_1_1_0_1_1());
}
break;
}
// InternalContextMappingDSL.g:2172:9: ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) )
// InternalContextMappingDSL.g:2173:10: (lv_upstreamRoles_8_0= ruleUpstreamRole )
{
// InternalContextMappingDSL.g:2173:10: (lv_upstreamRoles_8_0= ruleUpstreamRole )
// InternalContextMappingDSL.g:2174:11: lv_upstreamRoles_8_0= ruleUpstreamRole
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUpstreamRolesUpstreamRoleEnumRuleCall_1_0_0_1_1_0_2_0());
pushFollow(FOLLOW_44);
lv_upstreamRoles_8_0=ruleUpstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
add(
current,
"upstreamRoles",
lv_upstreamRoles_8_0,
"org.contextmapper.dsl.ContextMappingDSL.UpstreamRole");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:2191:9: (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )*
loop50:
do {
int alt50=2;
int LA50_0 = input.LA(1);
if ( (LA50_0==24) ) {
alt50=1;
}
switch (alt50) {
case 1 :
// InternalContextMappingDSL.g:2192:10: otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) )
{
otherlv_9=(Token)match(input,24,FOLLOW_42);
newLeafNode(otherlv_9, grammarAccess.getUpstreamDownstreamRelationshipAccess().getCommaKeyword_1_0_0_1_1_0_3_0());
// InternalContextMappingDSL.g:2196:10: ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) )
// InternalContextMappingDSL.g:2197:11: (lv_upstreamRoles_10_0= ruleUpstreamRole )
{
// InternalContextMappingDSL.g:2197:11: (lv_upstreamRoles_10_0= ruleUpstreamRole )
// InternalContextMappingDSL.g:2198:12: lv_upstreamRoles_10_0= ruleUpstreamRole
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUpstreamRolesUpstreamRoleEnumRuleCall_1_0_0_1_1_0_3_1_0());
pushFollow(FOLLOW_44);
lv_upstreamRoles_10_0=ruleUpstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
add(
current,
"upstreamRoles",
lv_upstreamRoles_10_0,
"org.contextmapper.dsl.ContextMappingDSL.UpstreamRole");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop50;
}
} while (true);
}
otherlv_11=(Token)match(input,37,FOLLOW_41);
newLeafNode(otherlv_11, grammarAccess.getUpstreamDownstreamRelationshipAccess().getRightSquareBracketKeyword_1_0_0_1_1_1());
}
}
break;
}
otherlv_12=(Token)match(input,44,FOLLOW_45);
newLeafNode(otherlv_12, grammarAccess.getUpstreamDownstreamRelationshipAccess().getHyphenMinusGreaterThanSignKeyword_1_0_0_2());
// InternalContextMappingDSL.g:2227:6: ( (otherlv_13= '[' otherlv_14= 'D' otherlv_15= ']' ) | ( (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* ) otherlv_22= ']' ) )?
int alt54=3;
int LA54_0 = input.LA(1);
if ( (LA54_0==35) ) {
int LA54_1 = input.LA(2);
if ( (LA54_1==45) ) {
int LA54_3 = input.LA(3);
if ( (LA54_3==24) ) {
alt54=2;
}
else if ( (LA54_3==37) ) {
alt54=1;
}
}
else if ( ((LA54_1>=210 && LA54_1<=211)) ) {
alt54=2;
}
}
switch (alt54) {
case 1 :
// InternalContextMappingDSL.g:2228:7: (otherlv_13= '[' otherlv_14= 'D' otherlv_15= ']' )
{
// InternalContextMappingDSL.g:2228:7: (otherlv_13= '[' otherlv_14= 'D' otherlv_15= ']' )
// InternalContextMappingDSL.g:2229:8: otherlv_13= '[' otherlv_14= 'D' otherlv_15= ']'
{
otherlv_13=(Token)match(input,35,FOLLOW_46);
newLeafNode(otherlv_13, grammarAccess.getUpstreamDownstreamRelationshipAccess().getLeftSquareBracketKeyword_1_0_0_3_0_0());
otherlv_14=(Token)match(input,45,FOLLOW_31);
newLeafNode(otherlv_14, grammarAccess.getUpstreamDownstreamRelationshipAccess().getDKeyword_1_0_0_3_0_1());
otherlv_15=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_15, grammarAccess.getUpstreamDownstreamRelationshipAccess().getRightSquareBracketKeyword_1_0_0_3_0_2());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:2243:7: ( (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* ) otherlv_22= ']' )
{
// InternalContextMappingDSL.g:2243:7: ( (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* ) otherlv_22= ']' )
// InternalContextMappingDSL.g:2244:8: (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* ) otherlv_22= ']'
{
// InternalContextMappingDSL.g:2244:8: (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* )
// InternalContextMappingDSL.g:2245:9: otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )*
{
otherlv_16=(Token)match(input,35,FOLLOW_47);
newLeafNode(otherlv_16, grammarAccess.getUpstreamDownstreamRelationshipAccess().getLeftSquareBracketKeyword_1_0_0_3_1_0_0());
// InternalContextMappingDSL.g:2249:9: (otherlv_17= 'D' otherlv_18= ',' )?
int alt52=2;
int LA52_0 = input.LA(1);
if ( (LA52_0==45) ) {
alt52=1;
}
switch (alt52) {
case 1 :
// InternalContextMappingDSL.g:2250:10: otherlv_17= 'D' otherlv_18= ','
{
otherlv_17=(Token)match(input,45,FOLLOW_43);
newLeafNode(otherlv_17, grammarAccess.getUpstreamDownstreamRelationshipAccess().getDKeyword_1_0_0_3_1_0_1_0());
otherlv_18=(Token)match(input,24,FOLLOW_47);
newLeafNode(otherlv_18, grammarAccess.getUpstreamDownstreamRelationshipAccess().getCommaKeyword_1_0_0_3_1_0_1_1());
}
break;
}
// InternalContextMappingDSL.g:2259:9: ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) )
// InternalContextMappingDSL.g:2260:10: (lv_downstreamRoles_19_0= ruleDownstreamRole )
{
// InternalContextMappingDSL.g:2260:10: (lv_downstreamRoles_19_0= ruleDownstreamRole )
// InternalContextMappingDSL.g:2261:11: lv_downstreamRoles_19_0= ruleDownstreamRole
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getDownstreamRolesDownstreamRoleEnumRuleCall_1_0_0_3_1_0_2_0());
pushFollow(FOLLOW_44);
lv_downstreamRoles_19_0=ruleDownstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
add(
current,
"downstreamRoles",
lv_downstreamRoles_19_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamRole");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:2278:9: (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )*
loop53:
do {
int alt53=2;
int LA53_0 = input.LA(1);
if ( (LA53_0==24) ) {
alt53=1;
}
switch (alt53) {
case 1 :
// InternalContextMappingDSL.g:2279:10: otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) )
{
otherlv_20=(Token)match(input,24,FOLLOW_47);
newLeafNode(otherlv_20, grammarAccess.getUpstreamDownstreamRelationshipAccess().getCommaKeyword_1_0_0_3_1_0_3_0());
// InternalContextMappingDSL.g:2283:10: ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) )
// InternalContextMappingDSL.g:2284:11: (lv_downstreamRoles_21_0= ruleDownstreamRole )
{
// InternalContextMappingDSL.g:2284:11: (lv_downstreamRoles_21_0= ruleDownstreamRole )
// InternalContextMappingDSL.g:2285:12: lv_downstreamRoles_21_0= ruleDownstreamRole
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getDownstreamRolesDownstreamRoleEnumRuleCall_1_0_0_3_1_0_3_1_0());
pushFollow(FOLLOW_44);
lv_downstreamRoles_21_0=ruleDownstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
add(
current,
"downstreamRoles",
lv_downstreamRoles_21_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamRole");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop53;
}
} while (true);
}
otherlv_22=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_22, grammarAccess.getUpstreamDownstreamRelationshipAccess().getRightSquareBracketKeyword_1_0_0_3_1_1());
}
}
break;
}
// InternalContextMappingDSL.g:2310:6: ( (otherlv_23= RULE_ID ) )
// InternalContextMappingDSL.g:2311:7: (otherlv_23= RULE_ID )
{
// InternalContextMappingDSL.g:2311:7: (otherlv_23= RULE_ID )
// InternalContextMappingDSL.g:2312:8: otherlv_23= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
otherlv_23=(Token)match(input,RULE_ID,FOLLOW_33);
newLeafNode(otherlv_23, grammarAccess.getUpstreamDownstreamRelationshipAccess().getDownstreamBoundedContextCrossReference_1_0_0_4_0());
}
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:2325:5: ( ( (otherlv_24= RULE_ID ) ) ( (otherlv_25= '[' otherlv_26= 'D' otherlv_27= ']' ) | ( (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* ) otherlv_34= ']' ) )? otherlv_35= '<-' ( (otherlv_36= '[' otherlv_37= 'U' otherlv_38= ']' ) | ( (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* ) otherlv_45= ']' ) )? ( (otherlv_46= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:2325:5: ( ( (otherlv_24= RULE_ID ) ) ( (otherlv_25= '[' otherlv_26= 'D' otherlv_27= ']' ) | ( (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* ) otherlv_34= ']' ) )? otherlv_35= '<-' ( (otherlv_36= '[' otherlv_37= 'U' otherlv_38= ']' ) | ( (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* ) otherlv_45= ']' ) )? ( (otherlv_46= RULE_ID ) ) )
// InternalContextMappingDSL.g:2326:6: ( (otherlv_24= RULE_ID ) ) ( (otherlv_25= '[' otherlv_26= 'D' otherlv_27= ']' ) | ( (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* ) otherlv_34= ']' ) )? otherlv_35= '<-' ( (otherlv_36= '[' otherlv_37= 'U' otherlv_38= ']' ) | ( (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* ) otherlv_45= ']' ) )? ( (otherlv_46= RULE_ID ) )
{
// InternalContextMappingDSL.g:2326:6: ( (otherlv_24= RULE_ID ) )
// InternalContextMappingDSL.g:2327:7: (otherlv_24= RULE_ID )
{
// InternalContextMappingDSL.g:2327:7: (otherlv_24= RULE_ID )
// InternalContextMappingDSL.g:2328:8: otherlv_24= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
otherlv_24=(Token)match(input,RULE_ID,FOLLOW_48);
newLeafNode(otherlv_24, grammarAccess.getUpstreamDownstreamRelationshipAccess().getDownstreamBoundedContextCrossReference_1_0_1_0_0());
}
}
// InternalContextMappingDSL.g:2339:6: ( (otherlv_25= '[' otherlv_26= 'D' otherlv_27= ']' ) | ( (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* ) otherlv_34= ']' ) )?
int alt57=3;
int LA57_0 = input.LA(1);
if ( (LA57_0==35) ) {
int LA57_1 = input.LA(2);
if ( (LA57_1==45) ) {
int LA57_3 = input.LA(3);
if ( (LA57_3==24) ) {
alt57=2;
}
else if ( (LA57_3==37) ) {
alt57=1;
}
}
else if ( ((LA57_1>=210 && LA57_1<=211)) ) {
alt57=2;
}
}
switch (alt57) {
case 1 :
// InternalContextMappingDSL.g:2340:7: (otherlv_25= '[' otherlv_26= 'D' otherlv_27= ']' )
{
// InternalContextMappingDSL.g:2340:7: (otherlv_25= '[' otherlv_26= 'D' otherlv_27= ']' )
// InternalContextMappingDSL.g:2341:8: otherlv_25= '[' otherlv_26= 'D' otherlv_27= ']'
{
otherlv_25=(Token)match(input,35,FOLLOW_46);
newLeafNode(otherlv_25, grammarAccess.getUpstreamDownstreamRelationshipAccess().getLeftSquareBracketKeyword_1_0_1_1_0_0());
otherlv_26=(Token)match(input,45,FOLLOW_31);
newLeafNode(otherlv_26, grammarAccess.getUpstreamDownstreamRelationshipAccess().getDKeyword_1_0_1_1_0_1());
otherlv_27=(Token)match(input,37,FOLLOW_49);
newLeafNode(otherlv_27, grammarAccess.getUpstreamDownstreamRelationshipAccess().getRightSquareBracketKeyword_1_0_1_1_0_2());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:2355:7: ( (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* ) otherlv_34= ']' )
{
// InternalContextMappingDSL.g:2355:7: ( (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* ) otherlv_34= ']' )
// InternalContextMappingDSL.g:2356:8: (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* ) otherlv_34= ']'
{
// InternalContextMappingDSL.g:2356:8: (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* )
// InternalContextMappingDSL.g:2357:9: otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )*
{
otherlv_28=(Token)match(input,35,FOLLOW_47);
newLeafNode(otherlv_28, grammarAccess.getUpstreamDownstreamRelationshipAccess().getLeftSquareBracketKeyword_1_0_1_1_1_0_0());
// InternalContextMappingDSL.g:2361:9: (otherlv_29= 'D' otherlv_30= ',' )?
int alt55=2;
int LA55_0 = input.LA(1);
if ( (LA55_0==45) ) {
alt55=1;
}
switch (alt55) {
case 1 :
// InternalContextMappingDSL.g:2362:10: otherlv_29= 'D' otherlv_30= ','
{
otherlv_29=(Token)match(input,45,FOLLOW_43);
newLeafNode(otherlv_29, grammarAccess.getUpstreamDownstreamRelationshipAccess().getDKeyword_1_0_1_1_1_0_1_0());
otherlv_30=(Token)match(input,24,FOLLOW_47);
newLeafNode(otherlv_30, grammarAccess.getUpstreamDownstreamRelationshipAccess().getCommaKeyword_1_0_1_1_1_0_1_1());
}
break;
}
// InternalContextMappingDSL.g:2371:9: ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) )
// InternalContextMappingDSL.g:2372:10: (lv_downstreamRoles_31_0= ruleDownstreamRole )
{
// InternalContextMappingDSL.g:2372:10: (lv_downstreamRoles_31_0= ruleDownstreamRole )
// InternalContextMappingDSL.g:2373:11: lv_downstreamRoles_31_0= ruleDownstreamRole
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getDownstreamRolesDownstreamRoleEnumRuleCall_1_0_1_1_1_0_2_0());
pushFollow(FOLLOW_44);
lv_downstreamRoles_31_0=ruleDownstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
add(
current,
"downstreamRoles",
lv_downstreamRoles_31_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamRole");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:2390:9: (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )*
loop56:
do {
int alt56=2;
int LA56_0 = input.LA(1);
if ( (LA56_0==24) ) {
alt56=1;
}
switch (alt56) {
case 1 :
// InternalContextMappingDSL.g:2391:10: otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) )
{
otherlv_32=(Token)match(input,24,FOLLOW_47);
newLeafNode(otherlv_32, grammarAccess.getUpstreamDownstreamRelationshipAccess().getCommaKeyword_1_0_1_1_1_0_3_0());
// InternalContextMappingDSL.g:2395:10: ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) )
// InternalContextMappingDSL.g:2396:11: (lv_downstreamRoles_33_0= ruleDownstreamRole )
{
// InternalContextMappingDSL.g:2396:11: (lv_downstreamRoles_33_0= ruleDownstreamRole )
// InternalContextMappingDSL.g:2397:12: lv_downstreamRoles_33_0= ruleDownstreamRole
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getDownstreamRolesDownstreamRoleEnumRuleCall_1_0_1_1_1_0_3_1_0());
pushFollow(FOLLOW_44);
lv_downstreamRoles_33_0=ruleDownstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
add(
current,
"downstreamRoles",
lv_downstreamRoles_33_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamRole");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop56;
}
} while (true);
}
otherlv_34=(Token)match(input,37,FOLLOW_49);
newLeafNode(otherlv_34, grammarAccess.getUpstreamDownstreamRelationshipAccess().getRightSquareBracketKeyword_1_0_1_1_1_1());
}
}
break;
}
otherlv_35=(Token)match(input,46,FOLLOW_45);
newLeafNode(otherlv_35, grammarAccess.getUpstreamDownstreamRelationshipAccess().getLessThanSignHyphenMinusKeyword_1_0_1_2());
// InternalContextMappingDSL.g:2426:6: ( (otherlv_36= '[' otherlv_37= 'U' otherlv_38= ']' ) | ( (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* ) otherlv_45= ']' ) )?
int alt60=3;
int LA60_0 = input.LA(1);
if ( (LA60_0==35) ) {
int LA60_1 = input.LA(2);
if ( (LA60_1==43) ) {
int LA60_3 = input.LA(3);
if ( (LA60_3==24) ) {
alt60=2;
}
else if ( (LA60_3==37) ) {
alt60=1;
}
}
else if ( ((LA60_1>=208 && LA60_1<=209)) ) {
alt60=2;
}
}
switch (alt60) {
case 1 :
// InternalContextMappingDSL.g:2427:7: (otherlv_36= '[' otherlv_37= 'U' otherlv_38= ']' )
{
// InternalContextMappingDSL.g:2427:7: (otherlv_36= '[' otherlv_37= 'U' otherlv_38= ']' )
// InternalContextMappingDSL.g:2428:8: otherlv_36= '[' otherlv_37= 'U' otherlv_38= ']'
{
otherlv_36=(Token)match(input,35,FOLLOW_40);
newLeafNode(otherlv_36, grammarAccess.getUpstreamDownstreamRelationshipAccess().getLeftSquareBracketKeyword_1_0_1_3_0_0());
otherlv_37=(Token)match(input,43,FOLLOW_31);
newLeafNode(otherlv_37, grammarAccess.getUpstreamDownstreamRelationshipAccess().getUKeyword_1_0_1_3_0_1());
otherlv_38=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_38, grammarAccess.getUpstreamDownstreamRelationshipAccess().getRightSquareBracketKeyword_1_0_1_3_0_2());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:2442:7: ( (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* ) otherlv_45= ']' )
{
// InternalContextMappingDSL.g:2442:7: ( (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* ) otherlv_45= ']' )
// InternalContextMappingDSL.g:2443:8: (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* ) otherlv_45= ']'
{
// InternalContextMappingDSL.g:2443:8: (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* )
// InternalContextMappingDSL.g:2444:9: otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )*
{
otherlv_39=(Token)match(input,35,FOLLOW_42);
newLeafNode(otherlv_39, grammarAccess.getUpstreamDownstreamRelationshipAccess().getLeftSquareBracketKeyword_1_0_1_3_1_0_0());
// InternalContextMappingDSL.g:2448:9: (otherlv_40= 'U' otherlv_41= ',' )?
int alt58=2;
int LA58_0 = input.LA(1);
if ( (LA58_0==43) ) {
alt58=1;
}
switch (alt58) {
case 1 :
// InternalContextMappingDSL.g:2449:10: otherlv_40= 'U' otherlv_41= ','
{
otherlv_40=(Token)match(input,43,FOLLOW_43);
newLeafNode(otherlv_40, grammarAccess.getUpstreamDownstreamRelationshipAccess().getUKeyword_1_0_1_3_1_0_1_0());
otherlv_41=(Token)match(input,24,FOLLOW_42);
newLeafNode(otherlv_41, grammarAccess.getUpstreamDownstreamRelationshipAccess().getCommaKeyword_1_0_1_3_1_0_1_1());
}
break;
}
// InternalContextMappingDSL.g:2458:9: ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) )
// InternalContextMappingDSL.g:2459:10: (lv_upstreamRoles_42_0= ruleUpstreamRole )
{
// InternalContextMappingDSL.g:2459:10: (lv_upstreamRoles_42_0= ruleUpstreamRole )
// InternalContextMappingDSL.g:2460:11: lv_upstreamRoles_42_0= ruleUpstreamRole
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUpstreamRolesUpstreamRoleEnumRuleCall_1_0_1_3_1_0_2_0());
pushFollow(FOLLOW_44);
lv_upstreamRoles_42_0=ruleUpstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
add(
current,
"upstreamRoles",
lv_upstreamRoles_42_0,
"org.contextmapper.dsl.ContextMappingDSL.UpstreamRole");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:2477:9: (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )*
loop59:
do {
int alt59=2;
int LA59_0 = input.LA(1);
if ( (LA59_0==24) ) {
alt59=1;
}
switch (alt59) {
case 1 :
// InternalContextMappingDSL.g:2478:10: otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) )
{
otherlv_43=(Token)match(input,24,FOLLOW_42);
newLeafNode(otherlv_43, grammarAccess.getUpstreamDownstreamRelationshipAccess().getCommaKeyword_1_0_1_3_1_0_3_0());
// InternalContextMappingDSL.g:2482:10: ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) )
// InternalContextMappingDSL.g:2483:11: (lv_upstreamRoles_44_0= ruleUpstreamRole )
{
// InternalContextMappingDSL.g:2483:11: (lv_upstreamRoles_44_0= ruleUpstreamRole )
// InternalContextMappingDSL.g:2484:12: lv_upstreamRoles_44_0= ruleUpstreamRole
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUpstreamRolesUpstreamRoleEnumRuleCall_1_0_1_3_1_0_3_1_0());
pushFollow(FOLLOW_44);
lv_upstreamRoles_44_0=ruleUpstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
add(
current,
"upstreamRoles",
lv_upstreamRoles_44_0,
"org.contextmapper.dsl.ContextMappingDSL.UpstreamRole");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop59;
}
} while (true);
}
otherlv_45=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_45, grammarAccess.getUpstreamDownstreamRelationshipAccess().getRightSquareBracketKeyword_1_0_1_3_1_1());
}
}
break;
}
// InternalContextMappingDSL.g:2509:6: ( (otherlv_46= RULE_ID ) )
// InternalContextMappingDSL.g:2510:7: (otherlv_46= RULE_ID )
{
// InternalContextMappingDSL.g:2510:7: (otherlv_46= RULE_ID )
// InternalContextMappingDSL.g:2511:8: otherlv_46= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
otherlv_46=(Token)match(input,RULE_ID,FOLLOW_33);
newLeafNode(otherlv_46, grammarAccess.getUpstreamDownstreamRelationshipAccess().getUpstreamBoundedContextCrossReference_1_0_1_4_0());
}
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:2524:5: ( ( (otherlv_47= RULE_ID ) ) (otherlv_48= '[' ( ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) ) (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )* )? otherlv_52= ']' )? otherlv_53= 'Upstream-Downstream' (otherlv_54= '[' ( ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) ) (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )* )? otherlv_58= ']' )? ( (otherlv_59= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:2524:5: ( ( (otherlv_47= RULE_ID ) ) (otherlv_48= '[' ( ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) ) (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )* )? otherlv_52= ']' )? otherlv_53= 'Upstream-Downstream' (otherlv_54= '[' ( ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) ) (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )* )? otherlv_58= ']' )? ( (otherlv_59= RULE_ID ) ) )
// InternalContextMappingDSL.g:2525:6: ( (otherlv_47= RULE_ID ) ) (otherlv_48= '[' ( ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) ) (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )* )? otherlv_52= ']' )? otherlv_53= 'Upstream-Downstream' (otherlv_54= '[' ( ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) ) (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )* )? otherlv_58= ']' )? ( (otherlv_59= RULE_ID ) )
{
// InternalContextMappingDSL.g:2525:6: ( (otherlv_47= RULE_ID ) )
// InternalContextMappingDSL.g:2526:7: (otherlv_47= RULE_ID )
{
// InternalContextMappingDSL.g:2526:7: (otherlv_47= RULE_ID )
// InternalContextMappingDSL.g:2527:8: otherlv_47= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
otherlv_47=(Token)match(input,RULE_ID,FOLLOW_50);
newLeafNode(otherlv_47, grammarAccess.getUpstreamDownstreamRelationshipAccess().getUpstreamBoundedContextCrossReference_1_0_2_0_0());
}
}
// InternalContextMappingDSL.g:2538:6: (otherlv_48= '[' ( ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) ) (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )* )? otherlv_52= ']' )?
int alt63=2;
int LA63_0 = input.LA(1);
if ( (LA63_0==35) ) {
alt63=1;
}
switch (alt63) {
case 1 :
// InternalContextMappingDSL.g:2539:7: otherlv_48= '[' ( ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) ) (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )* )? otherlv_52= ']'
{
otherlv_48=(Token)match(input,35,FOLLOW_51);
newLeafNode(otherlv_48, grammarAccess.getUpstreamDownstreamRelationshipAccess().getLeftSquareBracketKeyword_1_0_2_1_0());
// InternalContextMappingDSL.g:2543:7: ( ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) ) (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )* )?
int alt62=2;
int LA62_0 = input.LA(1);
if ( ((LA62_0>=208 && LA62_0<=209)) ) {
alt62=1;
}
switch (alt62) {
case 1 :
// InternalContextMappingDSL.g:2544:8: ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) ) (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )*
{
// InternalContextMappingDSL.g:2544:8: ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) )
// InternalContextMappingDSL.g:2545:9: (lv_upstreamRoles_49_0= ruleUpstreamRole )
{
// InternalContextMappingDSL.g:2545:9: (lv_upstreamRoles_49_0= ruleUpstreamRole )
// InternalContextMappingDSL.g:2546:10: lv_upstreamRoles_49_0= ruleUpstreamRole
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUpstreamRolesUpstreamRoleEnumRuleCall_1_0_2_1_1_0_0());
pushFollow(FOLLOW_44);
lv_upstreamRoles_49_0=ruleUpstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
add(
current,
"upstreamRoles",
lv_upstreamRoles_49_0,
"org.contextmapper.dsl.ContextMappingDSL.UpstreamRole");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:2563:8: (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )*
loop61:
do {
int alt61=2;
int LA61_0 = input.LA(1);
if ( (LA61_0==24) ) {
alt61=1;
}
switch (alt61) {
case 1 :
// InternalContextMappingDSL.g:2564:9: otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) )
{
otherlv_50=(Token)match(input,24,FOLLOW_42);
newLeafNode(otherlv_50, grammarAccess.getUpstreamDownstreamRelationshipAccess().getCommaKeyword_1_0_2_1_1_1_0());
// InternalContextMappingDSL.g:2568:9: ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) )
// InternalContextMappingDSL.g:2569:10: (lv_upstreamRoles_51_0= ruleUpstreamRole )
{
// InternalContextMappingDSL.g:2569:10: (lv_upstreamRoles_51_0= ruleUpstreamRole )
// InternalContextMappingDSL.g:2570:11: lv_upstreamRoles_51_0= ruleUpstreamRole
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUpstreamRolesUpstreamRoleEnumRuleCall_1_0_2_1_1_1_1_0());
pushFollow(FOLLOW_44);
lv_upstreamRoles_51_0=ruleUpstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
add(
current,
"upstreamRoles",
lv_upstreamRoles_51_0,
"org.contextmapper.dsl.ContextMappingDSL.UpstreamRole");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop61;
}
} while (true);
}
break;
}
otherlv_52=(Token)match(input,37,FOLLOW_52);
newLeafNode(otherlv_52, grammarAccess.getUpstreamDownstreamRelationshipAccess().getRightSquareBracketKeyword_1_0_2_1_2());
}
break;
}
otherlv_53=(Token)match(input,47,FOLLOW_45);
newLeafNode(otherlv_53, grammarAccess.getUpstreamDownstreamRelationshipAccess().getUpstreamDownstreamKeyword_1_0_2_2());
// InternalContextMappingDSL.g:2598:6: (otherlv_54= '[' ( ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) ) (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )* )? otherlv_58= ']' )?
int alt66=2;
int LA66_0 = input.LA(1);
if ( (LA66_0==35) ) {
alt66=1;
}
switch (alt66) {
case 1 :
// InternalContextMappingDSL.g:2599:7: otherlv_54= '[' ( ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) ) (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )* )? otherlv_58= ']'
{
otherlv_54=(Token)match(input,35,FOLLOW_53);
newLeafNode(otherlv_54, grammarAccess.getUpstreamDownstreamRelationshipAccess().getLeftSquareBracketKeyword_1_0_2_3_0());
// InternalContextMappingDSL.g:2603:7: ( ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) ) (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )* )?
int alt65=2;
int LA65_0 = input.LA(1);
if ( ((LA65_0>=210 && LA65_0<=211)) ) {
alt65=1;
}
switch (alt65) {
case 1 :
// InternalContextMappingDSL.g:2604:8: ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) ) (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )*
{
// InternalContextMappingDSL.g:2604:8: ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) )
// InternalContextMappingDSL.g:2605:9: (lv_downstreamRoles_55_0= ruleDownstreamRole )
{
// InternalContextMappingDSL.g:2605:9: (lv_downstreamRoles_55_0= ruleDownstreamRole )
// InternalContextMappingDSL.g:2606:10: lv_downstreamRoles_55_0= ruleDownstreamRole
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getDownstreamRolesDownstreamRoleEnumRuleCall_1_0_2_3_1_0_0());
pushFollow(FOLLOW_44);
lv_downstreamRoles_55_0=ruleDownstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
add(
current,
"downstreamRoles",
lv_downstreamRoles_55_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamRole");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:2623:8: (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )*
loop64:
do {
int alt64=2;
int LA64_0 = input.LA(1);
if ( (LA64_0==24) ) {
alt64=1;
}
switch (alt64) {
case 1 :
// InternalContextMappingDSL.g:2624:9: otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) )
{
otherlv_56=(Token)match(input,24,FOLLOW_47);
newLeafNode(otherlv_56, grammarAccess.getUpstreamDownstreamRelationshipAccess().getCommaKeyword_1_0_2_3_1_1_0());
// InternalContextMappingDSL.g:2628:9: ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) )
// InternalContextMappingDSL.g:2629:10: (lv_downstreamRoles_57_0= ruleDownstreamRole )
{
// InternalContextMappingDSL.g:2629:10: (lv_downstreamRoles_57_0= ruleDownstreamRole )
// InternalContextMappingDSL.g:2630:11: lv_downstreamRoles_57_0= ruleDownstreamRole
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getDownstreamRolesDownstreamRoleEnumRuleCall_1_0_2_3_1_1_1_0());
pushFollow(FOLLOW_44);
lv_downstreamRoles_57_0=ruleDownstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
add(
current,
"downstreamRoles",
lv_downstreamRoles_57_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamRole");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop64;
}
} while (true);
}
break;
}
otherlv_58=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_58, grammarAccess.getUpstreamDownstreamRelationshipAccess().getRightSquareBracketKeyword_1_0_2_3_2());
}
break;
}
// InternalContextMappingDSL.g:2654:6: ( (otherlv_59= RULE_ID ) )
// InternalContextMappingDSL.g:2655:7: (otherlv_59= RULE_ID )
{
// InternalContextMappingDSL.g:2655:7: (otherlv_59= RULE_ID )
// InternalContextMappingDSL.g:2656:8: otherlv_59= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
otherlv_59=(Token)match(input,RULE_ID,FOLLOW_33);
newLeafNode(otherlv_59, grammarAccess.getUpstreamDownstreamRelationshipAccess().getDownstreamBoundedContextCrossReference_1_0_2_4_0());
}
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:2669:5: ( ( (otherlv_60= RULE_ID ) ) (otherlv_61= '[' ( ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) ) (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )* )? otherlv_65= ']' )? otherlv_66= 'Downstream-Upstream' (otherlv_67= '[' ( ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) ) (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )* )? otherlv_71= ']' )? ( (otherlv_72= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:2669:5: ( ( (otherlv_60= RULE_ID ) ) (otherlv_61= '[' ( ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) ) (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )* )? otherlv_65= ']' )? otherlv_66= 'Downstream-Upstream' (otherlv_67= '[' ( ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) ) (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )* )? otherlv_71= ']' )? ( (otherlv_72= RULE_ID ) ) )
// InternalContextMappingDSL.g:2670:6: ( (otherlv_60= RULE_ID ) ) (otherlv_61= '[' ( ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) ) (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )* )? otherlv_65= ']' )? otherlv_66= 'Downstream-Upstream' (otherlv_67= '[' ( ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) ) (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )* )? otherlv_71= ']' )? ( (otherlv_72= RULE_ID ) )
{
// InternalContextMappingDSL.g:2670:6: ( (otherlv_60= RULE_ID ) )
// InternalContextMappingDSL.g:2671:7: (otherlv_60= RULE_ID )
{
// InternalContextMappingDSL.g:2671:7: (otherlv_60= RULE_ID )
// InternalContextMappingDSL.g:2672:8: otherlv_60= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
otherlv_60=(Token)match(input,RULE_ID,FOLLOW_54);
newLeafNode(otherlv_60, grammarAccess.getUpstreamDownstreamRelationshipAccess().getDownstreamBoundedContextCrossReference_1_0_3_0_0());
}
}
// InternalContextMappingDSL.g:2683:6: (otherlv_61= '[' ( ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) ) (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )* )? otherlv_65= ']' )?
int alt69=2;
int LA69_0 = input.LA(1);
if ( (LA69_0==35) ) {
alt69=1;
}
switch (alt69) {
case 1 :
// InternalContextMappingDSL.g:2684:7: otherlv_61= '[' ( ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) ) (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )* )? otherlv_65= ']'
{
otherlv_61=(Token)match(input,35,FOLLOW_53);
newLeafNode(otherlv_61, grammarAccess.getUpstreamDownstreamRelationshipAccess().getLeftSquareBracketKeyword_1_0_3_1_0());
// InternalContextMappingDSL.g:2688:7: ( ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) ) (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )* )?
int alt68=2;
int LA68_0 = input.LA(1);
if ( ((LA68_0>=210 && LA68_0<=211)) ) {
alt68=1;
}
switch (alt68) {
case 1 :
// InternalContextMappingDSL.g:2689:8: ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) ) (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )*
{
// InternalContextMappingDSL.g:2689:8: ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) )
// InternalContextMappingDSL.g:2690:9: (lv_downstreamRoles_62_0= ruleDownstreamRole )
{
// InternalContextMappingDSL.g:2690:9: (lv_downstreamRoles_62_0= ruleDownstreamRole )
// InternalContextMappingDSL.g:2691:10: lv_downstreamRoles_62_0= ruleDownstreamRole
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getDownstreamRolesDownstreamRoleEnumRuleCall_1_0_3_1_1_0_0());
pushFollow(FOLLOW_44);
lv_downstreamRoles_62_0=ruleDownstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
add(
current,
"downstreamRoles",
lv_downstreamRoles_62_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamRole");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:2708:8: (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )*
loop67:
do {
int alt67=2;
int LA67_0 = input.LA(1);
if ( (LA67_0==24) ) {
alt67=1;
}
switch (alt67) {
case 1 :
// InternalContextMappingDSL.g:2709:9: otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) )
{
otherlv_63=(Token)match(input,24,FOLLOW_47);
newLeafNode(otherlv_63, grammarAccess.getUpstreamDownstreamRelationshipAccess().getCommaKeyword_1_0_3_1_1_1_0());
// InternalContextMappingDSL.g:2713:9: ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) )
// InternalContextMappingDSL.g:2714:10: (lv_downstreamRoles_64_0= ruleDownstreamRole )
{
// InternalContextMappingDSL.g:2714:10: (lv_downstreamRoles_64_0= ruleDownstreamRole )
// InternalContextMappingDSL.g:2715:11: lv_downstreamRoles_64_0= ruleDownstreamRole
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getDownstreamRolesDownstreamRoleEnumRuleCall_1_0_3_1_1_1_1_0());
pushFollow(FOLLOW_44);
lv_downstreamRoles_64_0=ruleDownstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
add(
current,
"downstreamRoles",
lv_downstreamRoles_64_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamRole");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop67;
}
} while (true);
}
break;
}
otherlv_65=(Token)match(input,37,FOLLOW_55);
newLeafNode(otherlv_65, grammarAccess.getUpstreamDownstreamRelationshipAccess().getRightSquareBracketKeyword_1_0_3_1_2());
}
break;
}
otherlv_66=(Token)match(input,48,FOLLOW_45);
newLeafNode(otherlv_66, grammarAccess.getUpstreamDownstreamRelationshipAccess().getDownstreamUpstreamKeyword_1_0_3_2());
// InternalContextMappingDSL.g:2743:6: (otherlv_67= '[' ( ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) ) (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )* )? otherlv_71= ']' )?
int alt72=2;
int LA72_0 = input.LA(1);
if ( (LA72_0==35) ) {
alt72=1;
}
switch (alt72) {
case 1 :
// InternalContextMappingDSL.g:2744:7: otherlv_67= '[' ( ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) ) (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )* )? otherlv_71= ']'
{
otherlv_67=(Token)match(input,35,FOLLOW_51);
newLeafNode(otherlv_67, grammarAccess.getUpstreamDownstreamRelationshipAccess().getLeftSquareBracketKeyword_1_0_3_3_0());
// InternalContextMappingDSL.g:2748:7: ( ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) ) (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )* )?
int alt71=2;
int LA71_0 = input.LA(1);
if ( ((LA71_0>=208 && LA71_0<=209)) ) {
alt71=1;
}
switch (alt71) {
case 1 :
// InternalContextMappingDSL.g:2749:8: ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) ) (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )*
{
// InternalContextMappingDSL.g:2749:8: ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) )
// InternalContextMappingDSL.g:2750:9: (lv_upstreamRoles_68_0= ruleUpstreamRole )
{
// InternalContextMappingDSL.g:2750:9: (lv_upstreamRoles_68_0= ruleUpstreamRole )
// InternalContextMappingDSL.g:2751:10: lv_upstreamRoles_68_0= ruleUpstreamRole
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUpstreamRolesUpstreamRoleEnumRuleCall_1_0_3_3_1_0_0());
pushFollow(FOLLOW_44);
lv_upstreamRoles_68_0=ruleUpstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
add(
current,
"upstreamRoles",
lv_upstreamRoles_68_0,
"org.contextmapper.dsl.ContextMappingDSL.UpstreamRole");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:2768:8: (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )*
loop70:
do {
int alt70=2;
int LA70_0 = input.LA(1);
if ( (LA70_0==24) ) {
alt70=1;
}
switch (alt70) {
case 1 :
// InternalContextMappingDSL.g:2769:9: otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) )
{
otherlv_69=(Token)match(input,24,FOLLOW_42);
newLeafNode(otherlv_69, grammarAccess.getUpstreamDownstreamRelationshipAccess().getCommaKeyword_1_0_3_3_1_1_0());
// InternalContextMappingDSL.g:2773:9: ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) )
// InternalContextMappingDSL.g:2774:10: (lv_upstreamRoles_70_0= ruleUpstreamRole )
{
// InternalContextMappingDSL.g:2774:10: (lv_upstreamRoles_70_0= ruleUpstreamRole )
// InternalContextMappingDSL.g:2775:11: lv_upstreamRoles_70_0= ruleUpstreamRole
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUpstreamRolesUpstreamRoleEnumRuleCall_1_0_3_3_1_1_1_0());
pushFollow(FOLLOW_44);
lv_upstreamRoles_70_0=ruleUpstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
add(
current,
"upstreamRoles",
lv_upstreamRoles_70_0,
"org.contextmapper.dsl.ContextMappingDSL.UpstreamRole");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop70;
}
} while (true);
}
break;
}
otherlv_71=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_71, grammarAccess.getUpstreamDownstreamRelationshipAccess().getRightSquareBracketKeyword_1_0_3_3_2());
}
break;
}
// InternalContextMappingDSL.g:2799:6: ( (otherlv_72= RULE_ID ) )
// InternalContextMappingDSL.g:2800:7: (otherlv_72= RULE_ID )
{
// InternalContextMappingDSL.g:2800:7: (otherlv_72= RULE_ID )
// InternalContextMappingDSL.g:2801:8: otherlv_72= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
otherlv_72=(Token)match(input,RULE_ID,FOLLOW_33);
newLeafNode(otherlv_72, grammarAccess.getUpstreamDownstreamRelationshipAccess().getUpstreamBoundedContextCrossReference_1_0_3_4_0());
}
}
}
}
break;
}
// InternalContextMappingDSL.g:2814:4: (otherlv_73= ':' ( (lv_name_74_0= RULE_ID ) ) )?
int alt74=2;
int LA74_0 = input.LA(1);
if ( (LA74_0==40) ) {
alt74=1;
}
switch (alt74) {
case 1 :
// InternalContextMappingDSL.g:2815:5: otherlv_73= ':' ( (lv_name_74_0= RULE_ID ) )
{
otherlv_73=(Token)match(input,40,FOLLOW_10);
newLeafNode(otherlv_73, grammarAccess.getUpstreamDownstreamRelationshipAccess().getColonKeyword_1_1_0());
// InternalContextMappingDSL.g:2819:5: ( (lv_name_74_0= RULE_ID ) )
// InternalContextMappingDSL.g:2820:6: (lv_name_74_0= RULE_ID )
{
// InternalContextMappingDSL.g:2820:6: (lv_name_74_0= RULE_ID )
// InternalContextMappingDSL.g:2821:7: lv_name_74_0= RULE_ID
{
lv_name_74_0=(Token)match(input,RULE_ID,FOLLOW_23);
newLeafNode(lv_name_74_0, grammarAccess.getUpstreamDownstreamRelationshipAccess().getNameIDTerminalRuleCall_1_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
setWithLastConsumed(
current,
"name",
lv_name_74_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
}
break;
}
// InternalContextMappingDSL.g:2838:4: (this_OPEN_75= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) ) this_CLOSE_89= RULE_CLOSE )?
int alt81=2;
int LA81_0 = input.LA(1);
if ( (LA81_0==RULE_OPEN) ) {
alt81=1;
}
switch (alt81) {
case 1 :
// InternalContextMappingDSL.g:2839:5: this_OPEN_75= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) ) this_CLOSE_89= RULE_CLOSE
{
this_OPEN_75=(Token)match(input,RULE_OPEN,FOLLOW_56);
newLeafNode(this_OPEN_75, grammarAccess.getUpstreamDownstreamRelationshipAccess().getOPENTerminalRuleCall_1_2_0());
// InternalContextMappingDSL.g:2843:5: ( ( ( ( ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:2844:6: ( ( ( ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:2844:6: ( ( ( ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:2845:7: ( ( ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUnorderedGroup_1_2_1());
// InternalContextMappingDSL.g:2848:7: ( ( ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:2849:8: ( ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:2849:8: ( ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )*
loop80:
do {
int alt80=4;
int LA80_0 = input.LA(1);
if ( LA80_0 == 31 && getUnorderedGroupHelper().canSelect(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUnorderedGroup_1_2_1(), 0) ) {
alt80=1;
}
else if ( LA80_0 == 49 && getUnorderedGroupHelper().canSelect(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUnorderedGroup_1_2_1(), 1) ) {
alt80=2;
}
else if ( LA80_0 == 50 && getUnorderedGroupHelper().canSelect(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUnorderedGroup_1_2_1(), 2) ) {
alt80=3;
}
switch (alt80) {
case 1 :
// InternalContextMappingDSL.g:2850:6: ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:2850:6: ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:2851:7: {...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUnorderedGroup_1_2_1(), 0) ) {
throw new FailedPredicateException(input, "ruleUpstreamDownstreamRelationship", "getUnorderedGroupHelper().canSelect(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUnorderedGroup_1_2_1(), 0)");
}
// InternalContextMappingDSL.g:2851:133: ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:2852:8: ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUnorderedGroup_1_2_1(), 0);
// InternalContextMappingDSL.g:2855:11: ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:2855:12: {...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleUpstreamDownstreamRelationship", "true");
}
// InternalContextMappingDSL.g:2855:21: (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:2855:22: otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) )
{
otherlv_77=(Token)match(input,31,FOLLOW_17);
newLeafNode(otherlv_77, grammarAccess.getUpstreamDownstreamRelationshipAccess().getImplementationTechnologyKeyword_1_2_1_0_0());
// InternalContextMappingDSL.g:2859:11: (otherlv_78= '=' )?
int alt75=2;
int LA75_0 = input.LA(1);
if ( (LA75_0==21) ) {
alt75=1;
}
switch (alt75) {
case 1 :
// InternalContextMappingDSL.g:2860:12: otherlv_78= '='
{
otherlv_78=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_78, grammarAccess.getUpstreamDownstreamRelationshipAccess().getEqualsSignKeyword_1_2_1_0_1());
}
break;
}
// InternalContextMappingDSL.g:2865:11: ( (lv_implementationTechnology_79_0= RULE_STRING ) )
// InternalContextMappingDSL.g:2866:12: (lv_implementationTechnology_79_0= RULE_STRING )
{
// InternalContextMappingDSL.g:2866:12: (lv_implementationTechnology_79_0= RULE_STRING )
// InternalContextMappingDSL.g:2867:13: lv_implementationTechnology_79_0= RULE_STRING
{
lv_implementationTechnology_79_0=(Token)match(input,RULE_STRING,FOLLOW_56);
newLeafNode(lv_implementationTechnology_79_0, grammarAccess.getUpstreamDownstreamRelationshipAccess().getImplementationTechnologySTRINGTerminalRuleCall_1_2_1_0_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
setWithLastConsumed(
current,
"implementationTechnology",
lv_implementationTechnology_79_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUnorderedGroup_1_2_1());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:2889:6: ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) )
{
// InternalContextMappingDSL.g:2889:6: ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) )
// InternalContextMappingDSL.g:2890:7: {...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUnorderedGroup_1_2_1(), 1) ) {
throw new FailedPredicateException(input, "ruleUpstreamDownstreamRelationship", "getUnorderedGroupHelper().canSelect(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUnorderedGroup_1_2_1(), 1)");
}
// InternalContextMappingDSL.g:2890:133: ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) )
// InternalContextMappingDSL.g:2891:8: ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUnorderedGroup_1_2_1(), 1);
// InternalContextMappingDSL.g:2894:11: ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) )
// InternalContextMappingDSL.g:2894:12: {...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleUpstreamDownstreamRelationship", "true");
}
// InternalContextMappingDSL.g:2894:21: ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? )
// InternalContextMappingDSL.g:2894:22: (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )?
{
// InternalContextMappingDSL.g:2894:22: (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) )
// InternalContextMappingDSL.g:2895:12: otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) )
{
otherlv_80=(Token)match(input,49,FOLLOW_57);
newLeafNode(otherlv_80, grammarAccess.getUpstreamDownstreamRelationshipAccess().getExposedAggregatesKeyword_1_2_1_1_0_0());
// InternalContextMappingDSL.g:2899:12: (otherlv_81= '=' )?
int alt76=2;
int LA76_0 = input.LA(1);
if ( (LA76_0==21) ) {
alt76=1;
}
switch (alt76) {
case 1 :
// InternalContextMappingDSL.g:2900:13: otherlv_81= '='
{
otherlv_81=(Token)match(input,21,FOLLOW_10);
newLeafNode(otherlv_81, grammarAccess.getUpstreamDownstreamRelationshipAccess().getEqualsSignKeyword_1_2_1_1_0_1());
}
break;
}
// InternalContextMappingDSL.g:2905:12: ( (otherlv_82= RULE_ID ) )
// InternalContextMappingDSL.g:2906:13: (otherlv_82= RULE_ID )
{
// InternalContextMappingDSL.g:2906:13: (otherlv_82= RULE_ID )
// InternalContextMappingDSL.g:2907:14: otherlv_82= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
otherlv_82=(Token)match(input,RULE_ID,FOLLOW_58);
newLeafNode(otherlv_82, grammarAccess.getUpstreamDownstreamRelationshipAccess().getUpstreamExposedAggregatesAggregateCrossReference_1_2_1_1_0_2_0());
}
}
}
// InternalContextMappingDSL.g:2919:11: (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )*
loop77:
do {
int alt77=2;
int LA77_0 = input.LA(1);
if ( (LA77_0==24) ) {
alt77=1;
}
switch (alt77) {
case 1 :
// InternalContextMappingDSL.g:2920:12: otherlv_83= ',' ( (otherlv_84= RULE_ID ) )
{
otherlv_83=(Token)match(input,24,FOLLOW_10);
newLeafNode(otherlv_83, grammarAccess.getUpstreamDownstreamRelationshipAccess().getCommaKeyword_1_2_1_1_1_0());
// InternalContextMappingDSL.g:2924:12: ( (otherlv_84= RULE_ID ) )
// InternalContextMappingDSL.g:2925:13: (otherlv_84= RULE_ID )
{
// InternalContextMappingDSL.g:2925:13: (otherlv_84= RULE_ID )
// InternalContextMappingDSL.g:2926:14: otherlv_84= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
otherlv_84=(Token)match(input,RULE_ID,FOLLOW_58);
newLeafNode(otherlv_84, grammarAccess.getUpstreamDownstreamRelationshipAccess().getUpstreamExposedAggregatesAggregateCrossReference_1_2_1_1_1_1_0());
}
}
}
break;
default :
break loop77;
}
} while (true);
// InternalContextMappingDSL.g:2938:11: ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )?
int alt78=2;
int LA78_0 = input.LA(1);
if ( (LA78_0==RULE_SL_COMMENT) ) {
alt78=1;
}
switch (alt78) {
case 1 :
// InternalContextMappingDSL.g:2939:12: (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT )
{
// InternalContextMappingDSL.g:2939:12: (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT )
// InternalContextMappingDSL.g:2940:13: lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT
{
lv_exposedAggregatesComment_85_0=(Token)match(input,RULE_SL_COMMENT,FOLLOW_56);
newLeafNode(lv_exposedAggregatesComment_85_0, grammarAccess.getUpstreamDownstreamRelationshipAccess().getExposedAggregatesCommentSL_COMMENTTerminalRuleCall_1_2_1_1_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
setWithLastConsumed(
current,
"exposedAggregatesComment",
lv_exposedAggregatesComment_85_0,
"org.eclipse.xtext.common.Terminals.SL_COMMENT");
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUnorderedGroup_1_2_1());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:2962:6: ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) )
{
// InternalContextMappingDSL.g:2962:6: ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) )
// InternalContextMappingDSL.g:2963:7: {...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUnorderedGroup_1_2_1(), 2) ) {
throw new FailedPredicateException(input, "ruleUpstreamDownstreamRelationship", "getUnorderedGroupHelper().canSelect(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUnorderedGroup_1_2_1(), 2)");
}
// InternalContextMappingDSL.g:2963:133: ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) )
// InternalContextMappingDSL.g:2964:8: ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUnorderedGroup_1_2_1(), 2);
// InternalContextMappingDSL.g:2967:11: ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) )
// InternalContextMappingDSL.g:2967:12: {...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleUpstreamDownstreamRelationship", "true");
}
// InternalContextMappingDSL.g:2967:21: (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) )
// InternalContextMappingDSL.g:2967:22: otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) )
{
otherlv_86=(Token)match(input,50,FOLLOW_59);
newLeafNode(otherlv_86, grammarAccess.getUpstreamDownstreamRelationshipAccess().getDownstreamRightsKeyword_1_2_1_2_0());
// InternalContextMappingDSL.g:2971:11: (otherlv_87= '=' )?
int alt79=2;
int LA79_0 = input.LA(1);
if ( (LA79_0==21) ) {
alt79=1;
}
switch (alt79) {
case 1 :
// InternalContextMappingDSL.g:2972:12: otherlv_87= '='
{
otherlv_87=(Token)match(input,21,FOLLOW_59);
newLeafNode(otherlv_87, grammarAccess.getUpstreamDownstreamRelationshipAccess().getEqualsSignKeyword_1_2_1_2_1());
}
break;
}
// InternalContextMappingDSL.g:2977:11: ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) )
// InternalContextMappingDSL.g:2978:12: (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights )
{
// InternalContextMappingDSL.g:2978:12: (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights )
// InternalContextMappingDSL.g:2979:13: lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights
{
newCompositeNode(grammarAccess.getUpstreamDownstreamRelationshipAccess().getDownstreamGovernanceRightsDownstreamGovernanceRightsEnumRuleCall_1_2_1_2_2_0());
pushFollow(FOLLOW_56);
lv_downstreamGovernanceRights_88_0=ruleDownstreamGovernanceRights();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUpstreamDownstreamRelationshipRule());
}
set(
current,
"downstreamGovernanceRights",
lv_downstreamGovernanceRights_88_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamGovernanceRights");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUnorderedGroup_1_2_1());
}
}
}
break;
default :
break loop80;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getUpstreamDownstreamRelationshipAccess().getUnorderedGroup_1_2_1());
}
this_CLOSE_89=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(this_CLOSE_89, grammarAccess.getUpstreamDownstreamRelationshipAccess().getCLOSETerminalRuleCall_1_2_2());
}
break;
}
}
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleUpstreamDownstreamRelationship"
// $ANTLR start "entryRuleCustomerSupplierRelationship"
// InternalContextMappingDSL.g:3019:1: entryRuleCustomerSupplierRelationship returns [EObject current=null] : iv_ruleCustomerSupplierRelationship= ruleCustomerSupplierRelationship EOF ;
public final EObject entryRuleCustomerSupplierRelationship() throws RecognitionException {
EObject current = null;
EObject iv_ruleCustomerSupplierRelationship = null;
try {
// InternalContextMappingDSL.g:3019:69: (iv_ruleCustomerSupplierRelationship= ruleCustomerSupplierRelationship EOF )
// InternalContextMappingDSL.g:3020:2: iv_ruleCustomerSupplierRelationship= ruleCustomerSupplierRelationship EOF
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipRule());
pushFollow(FOLLOW_1);
iv_ruleCustomerSupplierRelationship=ruleCustomerSupplierRelationship();
state._fsp--;
current =iv_ruleCustomerSupplierRelationship;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleCustomerSupplierRelationship"
// $ANTLR start "ruleCustomerSupplierRelationship"
// InternalContextMappingDSL.g:3026:1: ruleCustomerSupplierRelationship returns [EObject current=null] : ( ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' (otherlv_2= 'U' otherlv_3= ',' )? otherlv_4= 'S' (otherlv_5= ',' ( (lv_upstreamRoles_6_0= ruleUpstreamRole ) ) (otherlv_7= ',' ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) )* )? otherlv_9= ']' otherlv_10= '->' otherlv_11= '[' (otherlv_12= 'D' otherlv_13= ',' )? otherlv_14= 'C' (otherlv_15= ',' ( (lv_downstreamRoles_16_0= ruleDownstreamRole ) ) (otherlv_17= ',' ( (lv_downstreamRoles_18_0= ruleDownstreamRole ) ) )* )? otherlv_19= ']' ( (otherlv_20= RULE_ID ) ) ) | ( ( (otherlv_21= RULE_ID ) ) otherlv_22= '[' (otherlv_23= 'D' otherlv_24= ',' )? otherlv_25= 'C' (otherlv_26= ',' ( (lv_downstreamRoles_27_0= ruleDownstreamRole ) ) (otherlv_28= ',' ( (lv_downstreamRoles_29_0= ruleDownstreamRole ) ) )* )? otherlv_30= ']' otherlv_31= '<-' otherlv_32= '[' (otherlv_33= 'U' otherlv_34= ',' )? otherlv_35= 'S' (otherlv_36= ',' ( (lv_upstreamRoles_37_0= ruleUpstreamRole ) ) (otherlv_38= ',' ( (lv_upstreamRoles_39_0= ruleUpstreamRole ) ) )* )? otherlv_40= ']' ( (otherlv_41= RULE_ID ) ) ) | ( ( (otherlv_42= RULE_ID ) ) (otherlv_43= '[' ( ( (lv_downstreamRoles_44_0= ruleDownstreamRole ) ) (otherlv_45= ',' ( (lv_downstreamRoles_46_0= ruleDownstreamRole ) ) )* )? otherlv_47= ']' )? otherlv_48= 'Customer-Supplier' (otherlv_49= '[' ( ( (lv_upstreamRoles_50_0= ruleUpstreamRole ) ) (otherlv_51= ',' ( (lv_upstreamRoles_52_0= ruleUpstreamRole ) ) )* )? otherlv_53= ']' )? ( (otherlv_54= RULE_ID ) ) ) | ( ( (otherlv_55= RULE_ID ) ) (otherlv_56= '[' ( ( (lv_upstreamRoles_57_0= ruleUpstreamRole ) ) (otherlv_58= ',' ( (lv_upstreamRoles_59_0= ruleUpstreamRole ) ) )* )? otherlv_60= ']' )? otherlv_61= 'Supplier-Customer' (otherlv_62= '[' ( ( (lv_downstreamRoles_63_0= ruleDownstreamRole ) ) (otherlv_64= ',' ( (lv_downstreamRoles_65_0= ruleDownstreamRole ) ) )* )? otherlv_66= ']' )? ( (otherlv_67= RULE_ID ) ) ) ) (otherlv_68= ':' ( (lv_name_69_0= RULE_ID ) ) )? (this_OPEN_70= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) ) this_CLOSE_84= RULE_CLOSE )? ) ;
public final EObject ruleCustomerSupplierRelationship() throws RecognitionException {
EObject current = null;
Token otherlv_0=null;
Token otherlv_1=null;
Token otherlv_2=null;
Token otherlv_3=null;
Token otherlv_4=null;
Token otherlv_5=null;
Token otherlv_7=null;
Token otherlv_9=null;
Token otherlv_10=null;
Token otherlv_11=null;
Token otherlv_12=null;
Token otherlv_13=null;
Token otherlv_14=null;
Token otherlv_15=null;
Token otherlv_17=null;
Token otherlv_19=null;
Token otherlv_20=null;
Token otherlv_21=null;
Token otherlv_22=null;
Token otherlv_23=null;
Token otherlv_24=null;
Token otherlv_25=null;
Token otherlv_26=null;
Token otherlv_28=null;
Token otherlv_30=null;
Token otherlv_31=null;
Token otherlv_32=null;
Token otherlv_33=null;
Token otherlv_34=null;
Token otherlv_35=null;
Token otherlv_36=null;
Token otherlv_38=null;
Token otherlv_40=null;
Token otherlv_41=null;
Token otherlv_42=null;
Token otherlv_43=null;
Token otherlv_45=null;
Token otherlv_47=null;
Token otherlv_48=null;
Token otherlv_49=null;
Token otherlv_51=null;
Token otherlv_53=null;
Token otherlv_54=null;
Token otherlv_55=null;
Token otherlv_56=null;
Token otherlv_58=null;
Token otherlv_60=null;
Token otherlv_61=null;
Token otherlv_62=null;
Token otherlv_64=null;
Token otherlv_66=null;
Token otherlv_67=null;
Token otherlv_68=null;
Token lv_name_69_0=null;
Token this_OPEN_70=null;
Token otherlv_72=null;
Token otherlv_73=null;
Token lv_implementationTechnology_74_0=null;
Token otherlv_75=null;
Token otherlv_76=null;
Token otherlv_77=null;
Token otherlv_78=null;
Token otherlv_79=null;
Token lv_exposedAggregatesComment_80_0=null;
Token otherlv_81=null;
Token otherlv_82=null;
Token this_CLOSE_84=null;
Enumerator lv_upstreamRoles_6_0 = null;
Enumerator lv_upstreamRoles_8_0 = null;
Enumerator lv_downstreamRoles_16_0 = null;
Enumerator lv_downstreamRoles_18_0 = null;
Enumerator lv_downstreamRoles_27_0 = null;
Enumerator lv_downstreamRoles_29_0 = null;
Enumerator lv_upstreamRoles_37_0 = null;
Enumerator lv_upstreamRoles_39_0 = null;
Enumerator lv_downstreamRoles_44_0 = null;
Enumerator lv_downstreamRoles_46_0 = null;
Enumerator lv_upstreamRoles_50_0 = null;
Enumerator lv_upstreamRoles_52_0 = null;
Enumerator lv_upstreamRoles_57_0 = null;
Enumerator lv_upstreamRoles_59_0 = null;
Enumerator lv_downstreamRoles_63_0 = null;
Enumerator lv_downstreamRoles_65_0 = null;
Enumerator lv_downstreamGovernanceRights_83_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:3032:2: ( ( ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' (otherlv_2= 'U' otherlv_3= ',' )? otherlv_4= 'S' (otherlv_5= ',' ( (lv_upstreamRoles_6_0= ruleUpstreamRole ) ) (otherlv_7= ',' ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) )* )? otherlv_9= ']' otherlv_10= '->' otherlv_11= '[' (otherlv_12= 'D' otherlv_13= ',' )? otherlv_14= 'C' (otherlv_15= ',' ( (lv_downstreamRoles_16_0= ruleDownstreamRole ) ) (otherlv_17= ',' ( (lv_downstreamRoles_18_0= ruleDownstreamRole ) ) )* )? otherlv_19= ']' ( (otherlv_20= RULE_ID ) ) ) | ( ( (otherlv_21= RULE_ID ) ) otherlv_22= '[' (otherlv_23= 'D' otherlv_24= ',' )? otherlv_25= 'C' (otherlv_26= ',' ( (lv_downstreamRoles_27_0= ruleDownstreamRole ) ) (otherlv_28= ',' ( (lv_downstreamRoles_29_0= ruleDownstreamRole ) ) )* )? otherlv_30= ']' otherlv_31= '<-' otherlv_32= '[' (otherlv_33= 'U' otherlv_34= ',' )? otherlv_35= 'S' (otherlv_36= ',' ( (lv_upstreamRoles_37_0= ruleUpstreamRole ) ) (otherlv_38= ',' ( (lv_upstreamRoles_39_0= ruleUpstreamRole ) ) )* )? otherlv_40= ']' ( (otherlv_41= RULE_ID ) ) ) | ( ( (otherlv_42= RULE_ID ) ) (otherlv_43= '[' ( ( (lv_downstreamRoles_44_0= ruleDownstreamRole ) ) (otherlv_45= ',' ( (lv_downstreamRoles_46_0= ruleDownstreamRole ) ) )* )? otherlv_47= ']' )? otherlv_48= 'Customer-Supplier' (otherlv_49= '[' ( ( (lv_upstreamRoles_50_0= ruleUpstreamRole ) ) (otherlv_51= ',' ( (lv_upstreamRoles_52_0= ruleUpstreamRole ) ) )* )? otherlv_53= ']' )? ( (otherlv_54= RULE_ID ) ) ) | ( ( (otherlv_55= RULE_ID ) ) (otherlv_56= '[' ( ( (lv_upstreamRoles_57_0= ruleUpstreamRole ) ) (otherlv_58= ',' ( (lv_upstreamRoles_59_0= ruleUpstreamRole ) ) )* )? otherlv_60= ']' )? otherlv_61= 'Supplier-Customer' (otherlv_62= '[' ( ( (lv_downstreamRoles_63_0= ruleDownstreamRole ) ) (otherlv_64= ',' ( (lv_downstreamRoles_65_0= ruleDownstreamRole ) ) )* )? otherlv_66= ']' )? ( (otherlv_67= RULE_ID ) ) ) ) (otherlv_68= ':' ( (lv_name_69_0= RULE_ID ) ) )? (this_OPEN_70= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) ) this_CLOSE_84= RULE_CLOSE )? ) )
// InternalContextMappingDSL.g:3033:2: ( ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' (otherlv_2= 'U' otherlv_3= ',' )? otherlv_4= 'S' (otherlv_5= ',' ( (lv_upstreamRoles_6_0= ruleUpstreamRole ) ) (otherlv_7= ',' ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) )* )? otherlv_9= ']' otherlv_10= '->' otherlv_11= '[' (otherlv_12= 'D' otherlv_13= ',' )? otherlv_14= 'C' (otherlv_15= ',' ( (lv_downstreamRoles_16_0= ruleDownstreamRole ) ) (otherlv_17= ',' ( (lv_downstreamRoles_18_0= ruleDownstreamRole ) ) )* )? otherlv_19= ']' ( (otherlv_20= RULE_ID ) ) ) | ( ( (otherlv_21= RULE_ID ) ) otherlv_22= '[' (otherlv_23= 'D' otherlv_24= ',' )? otherlv_25= 'C' (otherlv_26= ',' ( (lv_downstreamRoles_27_0= ruleDownstreamRole ) ) (otherlv_28= ',' ( (lv_downstreamRoles_29_0= ruleDownstreamRole ) ) )* )? otherlv_30= ']' otherlv_31= '<-' otherlv_32= '[' (otherlv_33= 'U' otherlv_34= ',' )? otherlv_35= 'S' (otherlv_36= ',' ( (lv_upstreamRoles_37_0= ruleUpstreamRole ) ) (otherlv_38= ',' ( (lv_upstreamRoles_39_0= ruleUpstreamRole ) ) )* )? otherlv_40= ']' ( (otherlv_41= RULE_ID ) ) ) | ( ( (otherlv_42= RULE_ID ) ) (otherlv_43= '[' ( ( (lv_downstreamRoles_44_0= ruleDownstreamRole ) ) (otherlv_45= ',' ( (lv_downstreamRoles_46_0= ruleDownstreamRole ) ) )* )? otherlv_47= ']' )? otherlv_48= 'Customer-Supplier' (otherlv_49= '[' ( ( (lv_upstreamRoles_50_0= ruleUpstreamRole ) ) (otherlv_51= ',' ( (lv_upstreamRoles_52_0= ruleUpstreamRole ) ) )* )? otherlv_53= ']' )? ( (otherlv_54= RULE_ID ) ) ) | ( ( (otherlv_55= RULE_ID ) ) (otherlv_56= '[' ( ( (lv_upstreamRoles_57_0= ruleUpstreamRole ) ) (otherlv_58= ',' ( (lv_upstreamRoles_59_0= ruleUpstreamRole ) ) )* )? otherlv_60= ']' )? otherlv_61= 'Supplier-Customer' (otherlv_62= '[' ( ( (lv_downstreamRoles_63_0= ruleDownstreamRole ) ) (otherlv_64= ',' ( (lv_downstreamRoles_65_0= ruleDownstreamRole ) ) )* )? otherlv_66= ']' )? ( (otherlv_67= RULE_ID ) ) ) ) (otherlv_68= ':' ( (lv_name_69_0= RULE_ID ) ) )? (this_OPEN_70= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) ) this_CLOSE_84= RULE_CLOSE )? )
{
// InternalContextMappingDSL.g:3033:2: ( ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' (otherlv_2= 'U' otherlv_3= ',' )? otherlv_4= 'S' (otherlv_5= ',' ( (lv_upstreamRoles_6_0= ruleUpstreamRole ) ) (otherlv_7= ',' ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) )* )? otherlv_9= ']' otherlv_10= '->' otherlv_11= '[' (otherlv_12= 'D' otherlv_13= ',' )? otherlv_14= 'C' (otherlv_15= ',' ( (lv_downstreamRoles_16_0= ruleDownstreamRole ) ) (otherlv_17= ',' ( (lv_downstreamRoles_18_0= ruleDownstreamRole ) ) )* )? otherlv_19= ']' ( (otherlv_20= RULE_ID ) ) ) | ( ( (otherlv_21= RULE_ID ) ) otherlv_22= '[' (otherlv_23= 'D' otherlv_24= ',' )? otherlv_25= 'C' (otherlv_26= ',' ( (lv_downstreamRoles_27_0= ruleDownstreamRole ) ) (otherlv_28= ',' ( (lv_downstreamRoles_29_0= ruleDownstreamRole ) ) )* )? otherlv_30= ']' otherlv_31= '<-' otherlv_32= '[' (otherlv_33= 'U' otherlv_34= ',' )? otherlv_35= 'S' (otherlv_36= ',' ( (lv_upstreamRoles_37_0= ruleUpstreamRole ) ) (otherlv_38= ',' ( (lv_upstreamRoles_39_0= ruleUpstreamRole ) ) )* )? otherlv_40= ']' ( (otherlv_41= RULE_ID ) ) ) | ( ( (otherlv_42= RULE_ID ) ) (otherlv_43= '[' ( ( (lv_downstreamRoles_44_0= ruleDownstreamRole ) ) (otherlv_45= ',' ( (lv_downstreamRoles_46_0= ruleDownstreamRole ) ) )* )? otherlv_47= ']' )? otherlv_48= 'Customer-Supplier' (otherlv_49= '[' ( ( (lv_upstreamRoles_50_0= ruleUpstreamRole ) ) (otherlv_51= ',' ( (lv_upstreamRoles_52_0= ruleUpstreamRole ) ) )* )? otherlv_53= ']' )? ( (otherlv_54= RULE_ID ) ) ) | ( ( (otherlv_55= RULE_ID ) ) (otherlv_56= '[' ( ( (lv_upstreamRoles_57_0= ruleUpstreamRole ) ) (otherlv_58= ',' ( (lv_upstreamRoles_59_0= ruleUpstreamRole ) ) )* )? otherlv_60= ']' )? otherlv_61= 'Supplier-Customer' (otherlv_62= '[' ( ( (lv_downstreamRoles_63_0= ruleDownstreamRole ) ) (otherlv_64= ',' ( (lv_downstreamRoles_65_0= ruleDownstreamRole ) ) )* )? otherlv_66= ']' )? ( (otherlv_67= RULE_ID ) ) ) ) (otherlv_68= ':' ( (lv_name_69_0= RULE_ID ) ) )? (this_OPEN_70= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) ) this_CLOSE_84= RULE_CLOSE )? )
// InternalContextMappingDSL.g:3034:3: ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' (otherlv_2= 'U' otherlv_3= ',' )? otherlv_4= 'S' (otherlv_5= ',' ( (lv_upstreamRoles_6_0= ruleUpstreamRole ) ) (otherlv_7= ',' ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) )* )? otherlv_9= ']' otherlv_10= '->' otherlv_11= '[' (otherlv_12= 'D' otherlv_13= ',' )? otherlv_14= 'C' (otherlv_15= ',' ( (lv_downstreamRoles_16_0= ruleDownstreamRole ) ) (otherlv_17= ',' ( (lv_downstreamRoles_18_0= ruleDownstreamRole ) ) )* )? otherlv_19= ']' ( (otherlv_20= RULE_ID ) ) ) | ( ( (otherlv_21= RULE_ID ) ) otherlv_22= '[' (otherlv_23= 'D' otherlv_24= ',' )? otherlv_25= 'C' (otherlv_26= ',' ( (lv_downstreamRoles_27_0= ruleDownstreamRole ) ) (otherlv_28= ',' ( (lv_downstreamRoles_29_0= ruleDownstreamRole ) ) )* )? otherlv_30= ']' otherlv_31= '<-' otherlv_32= '[' (otherlv_33= 'U' otherlv_34= ',' )? otherlv_35= 'S' (otherlv_36= ',' ( (lv_upstreamRoles_37_0= ruleUpstreamRole ) ) (otherlv_38= ',' ( (lv_upstreamRoles_39_0= ruleUpstreamRole ) ) )* )? otherlv_40= ']' ( (otherlv_41= RULE_ID ) ) ) | ( ( (otherlv_42= RULE_ID ) ) (otherlv_43= '[' ( ( (lv_downstreamRoles_44_0= ruleDownstreamRole ) ) (otherlv_45= ',' ( (lv_downstreamRoles_46_0= ruleDownstreamRole ) ) )* )? otherlv_47= ']' )? otherlv_48= 'Customer-Supplier' (otherlv_49= '[' ( ( (lv_upstreamRoles_50_0= ruleUpstreamRole ) ) (otherlv_51= ',' ( (lv_upstreamRoles_52_0= ruleUpstreamRole ) ) )* )? otherlv_53= ']' )? ( (otherlv_54= RULE_ID ) ) ) | ( ( (otherlv_55= RULE_ID ) ) (otherlv_56= '[' ( ( (lv_upstreamRoles_57_0= ruleUpstreamRole ) ) (otherlv_58= ',' ( (lv_upstreamRoles_59_0= ruleUpstreamRole ) ) )* )? otherlv_60= ']' )? otherlv_61= 'Supplier-Customer' (otherlv_62= '[' ( ( (lv_downstreamRoles_63_0= ruleDownstreamRole ) ) (otherlv_64= ',' ( (lv_downstreamRoles_65_0= ruleDownstreamRole ) ) )* )? otherlv_66= ']' )? ( (otherlv_67= RULE_ID ) ) ) ) (otherlv_68= ':' ( (lv_name_69_0= RULE_ID ) ) )? (this_OPEN_70= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) ) this_CLOSE_84= RULE_CLOSE )?
{
// InternalContextMappingDSL.g:3034:3: ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' (otherlv_2= 'U' otherlv_3= ',' )? otherlv_4= 'S' (otherlv_5= ',' ( (lv_upstreamRoles_6_0= ruleUpstreamRole ) ) (otherlv_7= ',' ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) )* )? otherlv_9= ']' otherlv_10= '->' otherlv_11= '[' (otherlv_12= 'D' otherlv_13= ',' )? otherlv_14= 'C' (otherlv_15= ',' ( (lv_downstreamRoles_16_0= ruleDownstreamRole ) ) (otherlv_17= ',' ( (lv_downstreamRoles_18_0= ruleDownstreamRole ) ) )* )? otherlv_19= ']' ( (otherlv_20= RULE_ID ) ) ) | ( ( (otherlv_21= RULE_ID ) ) otherlv_22= '[' (otherlv_23= 'D' otherlv_24= ',' )? otherlv_25= 'C' (otherlv_26= ',' ( (lv_downstreamRoles_27_0= ruleDownstreamRole ) ) (otherlv_28= ',' ( (lv_downstreamRoles_29_0= ruleDownstreamRole ) ) )* )? otherlv_30= ']' otherlv_31= '<-' otherlv_32= '[' (otherlv_33= 'U' otherlv_34= ',' )? otherlv_35= 'S' (otherlv_36= ',' ( (lv_upstreamRoles_37_0= ruleUpstreamRole ) ) (otherlv_38= ',' ( (lv_upstreamRoles_39_0= ruleUpstreamRole ) ) )* )? otherlv_40= ']' ( (otherlv_41= RULE_ID ) ) ) | ( ( (otherlv_42= RULE_ID ) ) (otherlv_43= '[' ( ( (lv_downstreamRoles_44_0= ruleDownstreamRole ) ) (otherlv_45= ',' ( (lv_downstreamRoles_46_0= ruleDownstreamRole ) ) )* )? otherlv_47= ']' )? otherlv_48= 'Customer-Supplier' (otherlv_49= '[' ( ( (lv_upstreamRoles_50_0= ruleUpstreamRole ) ) (otherlv_51= ',' ( (lv_upstreamRoles_52_0= ruleUpstreamRole ) ) )* )? otherlv_53= ']' )? ( (otherlv_54= RULE_ID ) ) ) | ( ( (otherlv_55= RULE_ID ) ) (otherlv_56= '[' ( ( (lv_upstreamRoles_57_0= ruleUpstreamRole ) ) (otherlv_58= ',' ( (lv_upstreamRoles_59_0= ruleUpstreamRole ) ) )* )? otherlv_60= ']' )? otherlv_61= 'Supplier-Customer' (otherlv_62= '[' ( ( (lv_downstreamRoles_63_0= ruleDownstreamRole ) ) (otherlv_64= ',' ( (lv_downstreamRoles_65_0= ruleDownstreamRole ) ) )* )? otherlv_66= ']' )? ( (otherlv_67= RULE_ID ) ) ) )
int alt107=4;
int LA107_0 = input.LA(1);
if ( (LA107_0==RULE_ID) ) {
switch ( input.LA(2) ) {
case 35:
{
switch ( input.LA(3) ) {
case 210:
case 211:
{
alt107=3;
}
break;
case 37:
{
int LA107_5 = input.LA(4);
if ( (LA107_5==53) ) {
alt107=3;
}
else if ( (LA107_5==54) ) {
alt107=4;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 107, 5, input);
throw nvae;
}
}
break;
case 43:
case 51:
{
alt107=1;
}
break;
case 208:
case 209:
{
alt107=4;
}
break;
case 45:
case 52:
{
alt107=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 107, 2, input);
throw nvae;
}
}
break;
case 54:
{
alt107=4;
}
break;
case 53:
{
alt107=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 107, 1, input);
throw nvae;
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 107, 0, input);
throw nvae;
}
switch (alt107) {
case 1 :
// InternalContextMappingDSL.g:3035:4: ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' (otherlv_2= 'U' otherlv_3= ',' )? otherlv_4= 'S' (otherlv_5= ',' ( (lv_upstreamRoles_6_0= ruleUpstreamRole ) ) (otherlv_7= ',' ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) )* )? otherlv_9= ']' otherlv_10= '->' otherlv_11= '[' (otherlv_12= 'D' otherlv_13= ',' )? otherlv_14= 'C' (otherlv_15= ',' ( (lv_downstreamRoles_16_0= ruleDownstreamRole ) ) (otherlv_17= ',' ( (lv_downstreamRoles_18_0= ruleDownstreamRole ) ) )* )? otherlv_19= ']' ( (otherlv_20= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:3035:4: ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' (otherlv_2= 'U' otherlv_3= ',' )? otherlv_4= 'S' (otherlv_5= ',' ( (lv_upstreamRoles_6_0= ruleUpstreamRole ) ) (otherlv_7= ',' ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) )* )? otherlv_9= ']' otherlv_10= '->' otherlv_11= '[' (otherlv_12= 'D' otherlv_13= ',' )? otherlv_14= 'C' (otherlv_15= ',' ( (lv_downstreamRoles_16_0= ruleDownstreamRole ) ) (otherlv_17= ',' ( (lv_downstreamRoles_18_0= ruleDownstreamRole ) ) )* )? otherlv_19= ']' ( (otherlv_20= RULE_ID ) ) )
// InternalContextMappingDSL.g:3036:5: ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' (otherlv_2= 'U' otherlv_3= ',' )? otherlv_4= 'S' (otherlv_5= ',' ( (lv_upstreamRoles_6_0= ruleUpstreamRole ) ) (otherlv_7= ',' ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) )* )? otherlv_9= ']' otherlv_10= '->' otherlv_11= '[' (otherlv_12= 'D' otherlv_13= ',' )? otherlv_14= 'C' (otherlv_15= ',' ( (lv_downstreamRoles_16_0= ruleDownstreamRole ) ) (otherlv_17= ',' ( (lv_downstreamRoles_18_0= ruleDownstreamRole ) ) )* )? otherlv_19= ']' ( (otherlv_20= RULE_ID ) )
{
// InternalContextMappingDSL.g:3036:5: ( (otherlv_0= RULE_ID ) )
// InternalContextMappingDSL.g:3037:6: (otherlv_0= RULE_ID )
{
// InternalContextMappingDSL.g:3037:6: (otherlv_0= RULE_ID )
// InternalContextMappingDSL.g:3038:7: otherlv_0= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getCustomerSupplierRelationshipRule());
}
otherlv_0=(Token)match(input,RULE_ID,FOLLOW_29);
newLeafNode(otherlv_0, grammarAccess.getCustomerSupplierRelationshipAccess().getUpstreamBoundedContextCrossReference_0_0_0_0());
}
}
otherlv_1=(Token)match(input,35,FOLLOW_60);
newLeafNode(otherlv_1, grammarAccess.getCustomerSupplierRelationshipAccess().getLeftSquareBracketKeyword_0_0_1());
// InternalContextMappingDSL.g:3053:5: (otherlv_2= 'U' otherlv_3= ',' )?
int alt83=2;
int LA83_0 = input.LA(1);
if ( (LA83_0==43) ) {
alt83=1;
}
switch (alt83) {
case 1 :
// InternalContextMappingDSL.g:3054:6: otherlv_2= 'U' otherlv_3= ','
{
otherlv_2=(Token)match(input,43,FOLLOW_43);
newLeafNode(otherlv_2, grammarAccess.getCustomerSupplierRelationshipAccess().getUKeyword_0_0_2_0());
otherlv_3=(Token)match(input,24,FOLLOW_61);
newLeafNode(otherlv_3, grammarAccess.getCustomerSupplierRelationshipAccess().getCommaKeyword_0_0_2_1());
}
break;
}
otherlv_4=(Token)match(input,51,FOLLOW_44);
newLeafNode(otherlv_4, grammarAccess.getCustomerSupplierRelationshipAccess().getSKeyword_0_0_3());
// InternalContextMappingDSL.g:3067:5: (otherlv_5= ',' ( (lv_upstreamRoles_6_0= ruleUpstreamRole ) ) (otherlv_7= ',' ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) )* )?
int alt85=2;
int LA85_0 = input.LA(1);
if ( (LA85_0==24) ) {
alt85=1;
}
switch (alt85) {
case 1 :
// InternalContextMappingDSL.g:3068:6: otherlv_5= ',' ( (lv_upstreamRoles_6_0= ruleUpstreamRole ) ) (otherlv_7= ',' ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) )*
{
otherlv_5=(Token)match(input,24,FOLLOW_42);
newLeafNode(otherlv_5, grammarAccess.getCustomerSupplierRelationshipAccess().getCommaKeyword_0_0_4_0());
// InternalContextMappingDSL.g:3072:6: ( (lv_upstreamRoles_6_0= ruleUpstreamRole ) )
// InternalContextMappingDSL.g:3073:7: (lv_upstreamRoles_6_0= ruleUpstreamRole )
{
// InternalContextMappingDSL.g:3073:7: (lv_upstreamRoles_6_0= ruleUpstreamRole )
// InternalContextMappingDSL.g:3074:8: lv_upstreamRoles_6_0= ruleUpstreamRole
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipAccess().getUpstreamRolesUpstreamRoleEnumRuleCall_0_0_4_1_0());
pushFollow(FOLLOW_44);
lv_upstreamRoles_6_0=ruleUpstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCustomerSupplierRelationshipRule());
}
add(
current,
"upstreamRoles",
lv_upstreamRoles_6_0,
"org.contextmapper.dsl.ContextMappingDSL.UpstreamRole");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:3091:6: (otherlv_7= ',' ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) )*
loop84:
do {
int alt84=2;
int LA84_0 = input.LA(1);
if ( (LA84_0==24) ) {
alt84=1;
}
switch (alt84) {
case 1 :
// InternalContextMappingDSL.g:3092:7: otherlv_7= ',' ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) )
{
otherlv_7=(Token)match(input,24,FOLLOW_42);
newLeafNode(otherlv_7, grammarAccess.getCustomerSupplierRelationshipAccess().getCommaKeyword_0_0_4_2_0());
// InternalContextMappingDSL.g:3096:7: ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) )
// InternalContextMappingDSL.g:3097:8: (lv_upstreamRoles_8_0= ruleUpstreamRole )
{
// InternalContextMappingDSL.g:3097:8: (lv_upstreamRoles_8_0= ruleUpstreamRole )
// InternalContextMappingDSL.g:3098:9: lv_upstreamRoles_8_0= ruleUpstreamRole
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipAccess().getUpstreamRolesUpstreamRoleEnumRuleCall_0_0_4_2_1_0());
pushFollow(FOLLOW_44);
lv_upstreamRoles_8_0=ruleUpstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCustomerSupplierRelationshipRule());
}
add(
current,
"upstreamRoles",
lv_upstreamRoles_8_0,
"org.contextmapper.dsl.ContextMappingDSL.UpstreamRole");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop84;
}
} while (true);
}
break;
}
otherlv_9=(Token)match(input,37,FOLLOW_41);
newLeafNode(otherlv_9, grammarAccess.getCustomerSupplierRelationshipAccess().getRightSquareBracketKeyword_0_0_5());
otherlv_10=(Token)match(input,44,FOLLOW_29);
newLeafNode(otherlv_10, grammarAccess.getCustomerSupplierRelationshipAccess().getHyphenMinusGreaterThanSignKeyword_0_0_6());
otherlv_11=(Token)match(input,35,FOLLOW_62);
newLeafNode(otherlv_11, grammarAccess.getCustomerSupplierRelationshipAccess().getLeftSquareBracketKeyword_0_0_7());
// InternalContextMappingDSL.g:3129:5: (otherlv_12= 'D' otherlv_13= ',' )?
int alt86=2;
int LA86_0 = input.LA(1);
if ( (LA86_0==45) ) {
alt86=1;
}
switch (alt86) {
case 1 :
// InternalContextMappingDSL.g:3130:6: otherlv_12= 'D' otherlv_13= ','
{
otherlv_12=(Token)match(input,45,FOLLOW_43);
newLeafNode(otherlv_12, grammarAccess.getCustomerSupplierRelationshipAccess().getDKeyword_0_0_8_0());
otherlv_13=(Token)match(input,24,FOLLOW_63);
newLeafNode(otherlv_13, grammarAccess.getCustomerSupplierRelationshipAccess().getCommaKeyword_0_0_8_1());
}
break;
}
otherlv_14=(Token)match(input,52,FOLLOW_44);
newLeafNode(otherlv_14, grammarAccess.getCustomerSupplierRelationshipAccess().getCKeyword_0_0_9());
// InternalContextMappingDSL.g:3143:5: (otherlv_15= ',' ( (lv_downstreamRoles_16_0= ruleDownstreamRole ) ) (otherlv_17= ',' ( (lv_downstreamRoles_18_0= ruleDownstreamRole ) ) )* )?
int alt88=2;
int LA88_0 = input.LA(1);
if ( (LA88_0==24) ) {
alt88=1;
}
switch (alt88) {
case 1 :
// InternalContextMappingDSL.g:3144:6: otherlv_15= ',' ( (lv_downstreamRoles_16_0= ruleDownstreamRole ) ) (otherlv_17= ',' ( (lv_downstreamRoles_18_0= ruleDownstreamRole ) ) )*
{
otherlv_15=(Token)match(input,24,FOLLOW_47);
newLeafNode(otherlv_15, grammarAccess.getCustomerSupplierRelationshipAccess().getCommaKeyword_0_0_10_0());
// InternalContextMappingDSL.g:3148:6: ( (lv_downstreamRoles_16_0= ruleDownstreamRole ) )
// InternalContextMappingDSL.g:3149:7: (lv_downstreamRoles_16_0= ruleDownstreamRole )
{
// InternalContextMappingDSL.g:3149:7: (lv_downstreamRoles_16_0= ruleDownstreamRole )
// InternalContextMappingDSL.g:3150:8: lv_downstreamRoles_16_0= ruleDownstreamRole
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipAccess().getDownstreamRolesDownstreamRoleEnumRuleCall_0_0_10_1_0());
pushFollow(FOLLOW_44);
lv_downstreamRoles_16_0=ruleDownstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCustomerSupplierRelationshipRule());
}
add(
current,
"downstreamRoles",
lv_downstreamRoles_16_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamRole");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:3167:6: (otherlv_17= ',' ( (lv_downstreamRoles_18_0= ruleDownstreamRole ) ) )*
loop87:
do {
int alt87=2;
int LA87_0 = input.LA(1);
if ( (LA87_0==24) ) {
alt87=1;
}
switch (alt87) {
case 1 :
// InternalContextMappingDSL.g:3168:7: otherlv_17= ',' ( (lv_downstreamRoles_18_0= ruleDownstreamRole ) )
{
otherlv_17=(Token)match(input,24,FOLLOW_47);
newLeafNode(otherlv_17, grammarAccess.getCustomerSupplierRelationshipAccess().getCommaKeyword_0_0_10_2_0());
// InternalContextMappingDSL.g:3172:7: ( (lv_downstreamRoles_18_0= ruleDownstreamRole ) )
// InternalContextMappingDSL.g:3173:8: (lv_downstreamRoles_18_0= ruleDownstreamRole )
{
// InternalContextMappingDSL.g:3173:8: (lv_downstreamRoles_18_0= ruleDownstreamRole )
// InternalContextMappingDSL.g:3174:9: lv_downstreamRoles_18_0= ruleDownstreamRole
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipAccess().getDownstreamRolesDownstreamRoleEnumRuleCall_0_0_10_2_1_0());
pushFollow(FOLLOW_44);
lv_downstreamRoles_18_0=ruleDownstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCustomerSupplierRelationshipRule());
}
add(
current,
"downstreamRoles",
lv_downstreamRoles_18_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamRole");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop87;
}
} while (true);
}
break;
}
otherlv_19=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_19, grammarAccess.getCustomerSupplierRelationshipAccess().getRightSquareBracketKeyword_0_0_11());
// InternalContextMappingDSL.g:3197:5: ( (otherlv_20= RULE_ID ) )
// InternalContextMappingDSL.g:3198:6: (otherlv_20= RULE_ID )
{
// InternalContextMappingDSL.g:3198:6: (otherlv_20= RULE_ID )
// InternalContextMappingDSL.g:3199:7: otherlv_20= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getCustomerSupplierRelationshipRule());
}
otherlv_20=(Token)match(input,RULE_ID,FOLLOW_33);
newLeafNode(otherlv_20, grammarAccess.getCustomerSupplierRelationshipAccess().getDownstreamBoundedContextCrossReference_0_0_12_0());
}
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:3212:4: ( ( (otherlv_21= RULE_ID ) ) otherlv_22= '[' (otherlv_23= 'D' otherlv_24= ',' )? otherlv_25= 'C' (otherlv_26= ',' ( (lv_downstreamRoles_27_0= ruleDownstreamRole ) ) (otherlv_28= ',' ( (lv_downstreamRoles_29_0= ruleDownstreamRole ) ) )* )? otherlv_30= ']' otherlv_31= '<-' otherlv_32= '[' (otherlv_33= 'U' otherlv_34= ',' )? otherlv_35= 'S' (otherlv_36= ',' ( (lv_upstreamRoles_37_0= ruleUpstreamRole ) ) (otherlv_38= ',' ( (lv_upstreamRoles_39_0= ruleUpstreamRole ) ) )* )? otherlv_40= ']' ( (otherlv_41= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:3212:4: ( ( (otherlv_21= RULE_ID ) ) otherlv_22= '[' (otherlv_23= 'D' otherlv_24= ',' )? otherlv_25= 'C' (otherlv_26= ',' ( (lv_downstreamRoles_27_0= ruleDownstreamRole ) ) (otherlv_28= ',' ( (lv_downstreamRoles_29_0= ruleDownstreamRole ) ) )* )? otherlv_30= ']' otherlv_31= '<-' otherlv_32= '[' (otherlv_33= 'U' otherlv_34= ',' )? otherlv_35= 'S' (otherlv_36= ',' ( (lv_upstreamRoles_37_0= ruleUpstreamRole ) ) (otherlv_38= ',' ( (lv_upstreamRoles_39_0= ruleUpstreamRole ) ) )* )? otherlv_40= ']' ( (otherlv_41= RULE_ID ) ) )
// InternalContextMappingDSL.g:3213:5: ( (otherlv_21= RULE_ID ) ) otherlv_22= '[' (otherlv_23= 'D' otherlv_24= ',' )? otherlv_25= 'C' (otherlv_26= ',' ( (lv_downstreamRoles_27_0= ruleDownstreamRole ) ) (otherlv_28= ',' ( (lv_downstreamRoles_29_0= ruleDownstreamRole ) ) )* )? otherlv_30= ']' otherlv_31= '<-' otherlv_32= '[' (otherlv_33= 'U' otherlv_34= ',' )? otherlv_35= 'S' (otherlv_36= ',' ( (lv_upstreamRoles_37_0= ruleUpstreamRole ) ) (otherlv_38= ',' ( (lv_upstreamRoles_39_0= ruleUpstreamRole ) ) )* )? otherlv_40= ']' ( (otherlv_41= RULE_ID ) )
{
// InternalContextMappingDSL.g:3213:5: ( (otherlv_21= RULE_ID ) )
// InternalContextMappingDSL.g:3214:6: (otherlv_21= RULE_ID )
{
// InternalContextMappingDSL.g:3214:6: (otherlv_21= RULE_ID )
// InternalContextMappingDSL.g:3215:7: otherlv_21= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getCustomerSupplierRelationshipRule());
}
otherlv_21=(Token)match(input,RULE_ID,FOLLOW_29);
newLeafNode(otherlv_21, grammarAccess.getCustomerSupplierRelationshipAccess().getDownstreamBoundedContextCrossReference_0_1_0_0());
}
}
otherlv_22=(Token)match(input,35,FOLLOW_62);
newLeafNode(otherlv_22, grammarAccess.getCustomerSupplierRelationshipAccess().getLeftSquareBracketKeyword_0_1_1());
// InternalContextMappingDSL.g:3230:5: (otherlv_23= 'D' otherlv_24= ',' )?
int alt89=2;
int LA89_0 = input.LA(1);
if ( (LA89_0==45) ) {
alt89=1;
}
switch (alt89) {
case 1 :
// InternalContextMappingDSL.g:3231:6: otherlv_23= 'D' otherlv_24= ','
{
otherlv_23=(Token)match(input,45,FOLLOW_43);
newLeafNode(otherlv_23, grammarAccess.getCustomerSupplierRelationshipAccess().getDKeyword_0_1_2_0());
otherlv_24=(Token)match(input,24,FOLLOW_63);
newLeafNode(otherlv_24, grammarAccess.getCustomerSupplierRelationshipAccess().getCommaKeyword_0_1_2_1());
}
break;
}
otherlv_25=(Token)match(input,52,FOLLOW_44);
newLeafNode(otherlv_25, grammarAccess.getCustomerSupplierRelationshipAccess().getCKeyword_0_1_3());
// InternalContextMappingDSL.g:3244:5: (otherlv_26= ',' ( (lv_downstreamRoles_27_0= ruleDownstreamRole ) ) (otherlv_28= ',' ( (lv_downstreamRoles_29_0= ruleDownstreamRole ) ) )* )?
int alt91=2;
int LA91_0 = input.LA(1);
if ( (LA91_0==24) ) {
alt91=1;
}
switch (alt91) {
case 1 :
// InternalContextMappingDSL.g:3245:6: otherlv_26= ',' ( (lv_downstreamRoles_27_0= ruleDownstreamRole ) ) (otherlv_28= ',' ( (lv_downstreamRoles_29_0= ruleDownstreamRole ) ) )*
{
otherlv_26=(Token)match(input,24,FOLLOW_47);
newLeafNode(otherlv_26, grammarAccess.getCustomerSupplierRelationshipAccess().getCommaKeyword_0_1_4_0());
// InternalContextMappingDSL.g:3249:6: ( (lv_downstreamRoles_27_0= ruleDownstreamRole ) )
// InternalContextMappingDSL.g:3250:7: (lv_downstreamRoles_27_0= ruleDownstreamRole )
{
// InternalContextMappingDSL.g:3250:7: (lv_downstreamRoles_27_0= ruleDownstreamRole )
// InternalContextMappingDSL.g:3251:8: lv_downstreamRoles_27_0= ruleDownstreamRole
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipAccess().getDownstreamRolesDownstreamRoleEnumRuleCall_0_1_4_1_0());
pushFollow(FOLLOW_44);
lv_downstreamRoles_27_0=ruleDownstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCustomerSupplierRelationshipRule());
}
add(
current,
"downstreamRoles",
lv_downstreamRoles_27_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamRole");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:3268:6: (otherlv_28= ',' ( (lv_downstreamRoles_29_0= ruleDownstreamRole ) ) )*
loop90:
do {
int alt90=2;
int LA90_0 = input.LA(1);
if ( (LA90_0==24) ) {
alt90=1;
}
switch (alt90) {
case 1 :
// InternalContextMappingDSL.g:3269:7: otherlv_28= ',' ( (lv_downstreamRoles_29_0= ruleDownstreamRole ) )
{
otherlv_28=(Token)match(input,24,FOLLOW_47);
newLeafNode(otherlv_28, grammarAccess.getCustomerSupplierRelationshipAccess().getCommaKeyword_0_1_4_2_0());
// InternalContextMappingDSL.g:3273:7: ( (lv_downstreamRoles_29_0= ruleDownstreamRole ) )
// InternalContextMappingDSL.g:3274:8: (lv_downstreamRoles_29_0= ruleDownstreamRole )
{
// InternalContextMappingDSL.g:3274:8: (lv_downstreamRoles_29_0= ruleDownstreamRole )
// InternalContextMappingDSL.g:3275:9: lv_downstreamRoles_29_0= ruleDownstreamRole
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipAccess().getDownstreamRolesDownstreamRoleEnumRuleCall_0_1_4_2_1_0());
pushFollow(FOLLOW_44);
lv_downstreamRoles_29_0=ruleDownstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCustomerSupplierRelationshipRule());
}
add(
current,
"downstreamRoles",
lv_downstreamRoles_29_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamRole");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop90;
}
} while (true);
}
break;
}
otherlv_30=(Token)match(input,37,FOLLOW_49);
newLeafNode(otherlv_30, grammarAccess.getCustomerSupplierRelationshipAccess().getRightSquareBracketKeyword_0_1_5());
otherlv_31=(Token)match(input,46,FOLLOW_29);
newLeafNode(otherlv_31, grammarAccess.getCustomerSupplierRelationshipAccess().getLessThanSignHyphenMinusKeyword_0_1_6());
otherlv_32=(Token)match(input,35,FOLLOW_60);
newLeafNode(otherlv_32, grammarAccess.getCustomerSupplierRelationshipAccess().getLeftSquareBracketKeyword_0_1_7());
// InternalContextMappingDSL.g:3306:5: (otherlv_33= 'U' otherlv_34= ',' )?
int alt92=2;
int LA92_0 = input.LA(1);
if ( (LA92_0==43) ) {
alt92=1;
}
switch (alt92) {
case 1 :
// InternalContextMappingDSL.g:3307:6: otherlv_33= 'U' otherlv_34= ','
{
otherlv_33=(Token)match(input,43,FOLLOW_43);
newLeafNode(otherlv_33, grammarAccess.getCustomerSupplierRelationshipAccess().getUKeyword_0_1_8_0());
otherlv_34=(Token)match(input,24,FOLLOW_61);
newLeafNode(otherlv_34, grammarAccess.getCustomerSupplierRelationshipAccess().getCommaKeyword_0_1_8_1());
}
break;
}
otherlv_35=(Token)match(input,51,FOLLOW_44);
newLeafNode(otherlv_35, grammarAccess.getCustomerSupplierRelationshipAccess().getSKeyword_0_1_9());
// InternalContextMappingDSL.g:3320:5: (otherlv_36= ',' ( (lv_upstreamRoles_37_0= ruleUpstreamRole ) ) (otherlv_38= ',' ( (lv_upstreamRoles_39_0= ruleUpstreamRole ) ) )* )?
int alt94=2;
int LA94_0 = input.LA(1);
if ( (LA94_0==24) ) {
alt94=1;
}
switch (alt94) {
case 1 :
// InternalContextMappingDSL.g:3321:6: otherlv_36= ',' ( (lv_upstreamRoles_37_0= ruleUpstreamRole ) ) (otherlv_38= ',' ( (lv_upstreamRoles_39_0= ruleUpstreamRole ) ) )*
{
otherlv_36=(Token)match(input,24,FOLLOW_42);
newLeafNode(otherlv_36, grammarAccess.getCustomerSupplierRelationshipAccess().getCommaKeyword_0_1_10_0());
// InternalContextMappingDSL.g:3325:6: ( (lv_upstreamRoles_37_0= ruleUpstreamRole ) )
// InternalContextMappingDSL.g:3326:7: (lv_upstreamRoles_37_0= ruleUpstreamRole )
{
// InternalContextMappingDSL.g:3326:7: (lv_upstreamRoles_37_0= ruleUpstreamRole )
// InternalContextMappingDSL.g:3327:8: lv_upstreamRoles_37_0= ruleUpstreamRole
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipAccess().getUpstreamRolesUpstreamRoleEnumRuleCall_0_1_10_1_0());
pushFollow(FOLLOW_44);
lv_upstreamRoles_37_0=ruleUpstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCustomerSupplierRelationshipRule());
}
add(
current,
"upstreamRoles",
lv_upstreamRoles_37_0,
"org.contextmapper.dsl.ContextMappingDSL.UpstreamRole");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:3344:6: (otherlv_38= ',' ( (lv_upstreamRoles_39_0= ruleUpstreamRole ) ) )*
loop93:
do {
int alt93=2;
int LA93_0 = input.LA(1);
if ( (LA93_0==24) ) {
alt93=1;
}
switch (alt93) {
case 1 :
// InternalContextMappingDSL.g:3345:7: otherlv_38= ',' ( (lv_upstreamRoles_39_0= ruleUpstreamRole ) )
{
otherlv_38=(Token)match(input,24,FOLLOW_42);
newLeafNode(otherlv_38, grammarAccess.getCustomerSupplierRelationshipAccess().getCommaKeyword_0_1_10_2_0());
// InternalContextMappingDSL.g:3349:7: ( (lv_upstreamRoles_39_0= ruleUpstreamRole ) )
// InternalContextMappingDSL.g:3350:8: (lv_upstreamRoles_39_0= ruleUpstreamRole )
{
// InternalContextMappingDSL.g:3350:8: (lv_upstreamRoles_39_0= ruleUpstreamRole )
// InternalContextMappingDSL.g:3351:9: lv_upstreamRoles_39_0= ruleUpstreamRole
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipAccess().getUpstreamRolesUpstreamRoleEnumRuleCall_0_1_10_2_1_0());
pushFollow(FOLLOW_44);
lv_upstreamRoles_39_0=ruleUpstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCustomerSupplierRelationshipRule());
}
add(
current,
"upstreamRoles",
lv_upstreamRoles_39_0,
"org.contextmapper.dsl.ContextMappingDSL.UpstreamRole");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop93;
}
} while (true);
}
break;
}
otherlv_40=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_40, grammarAccess.getCustomerSupplierRelationshipAccess().getRightSquareBracketKeyword_0_1_11());
// InternalContextMappingDSL.g:3374:5: ( (otherlv_41= RULE_ID ) )
// InternalContextMappingDSL.g:3375:6: (otherlv_41= RULE_ID )
{
// InternalContextMappingDSL.g:3375:6: (otherlv_41= RULE_ID )
// InternalContextMappingDSL.g:3376:7: otherlv_41= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getCustomerSupplierRelationshipRule());
}
otherlv_41=(Token)match(input,RULE_ID,FOLLOW_33);
newLeafNode(otherlv_41, grammarAccess.getCustomerSupplierRelationshipAccess().getUpstreamBoundedContextCrossReference_0_1_12_0());
}
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:3389:4: ( ( (otherlv_42= RULE_ID ) ) (otherlv_43= '[' ( ( (lv_downstreamRoles_44_0= ruleDownstreamRole ) ) (otherlv_45= ',' ( (lv_downstreamRoles_46_0= ruleDownstreamRole ) ) )* )? otherlv_47= ']' )? otherlv_48= 'Customer-Supplier' (otherlv_49= '[' ( ( (lv_upstreamRoles_50_0= ruleUpstreamRole ) ) (otherlv_51= ',' ( (lv_upstreamRoles_52_0= ruleUpstreamRole ) ) )* )? otherlv_53= ']' )? ( (otherlv_54= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:3389:4: ( ( (otherlv_42= RULE_ID ) ) (otherlv_43= '[' ( ( (lv_downstreamRoles_44_0= ruleDownstreamRole ) ) (otherlv_45= ',' ( (lv_downstreamRoles_46_0= ruleDownstreamRole ) ) )* )? otherlv_47= ']' )? otherlv_48= 'Customer-Supplier' (otherlv_49= '[' ( ( (lv_upstreamRoles_50_0= ruleUpstreamRole ) ) (otherlv_51= ',' ( (lv_upstreamRoles_52_0= ruleUpstreamRole ) ) )* )? otherlv_53= ']' )? ( (otherlv_54= RULE_ID ) ) )
// InternalContextMappingDSL.g:3390:5: ( (otherlv_42= RULE_ID ) ) (otherlv_43= '[' ( ( (lv_downstreamRoles_44_0= ruleDownstreamRole ) ) (otherlv_45= ',' ( (lv_downstreamRoles_46_0= ruleDownstreamRole ) ) )* )? otherlv_47= ']' )? otherlv_48= 'Customer-Supplier' (otherlv_49= '[' ( ( (lv_upstreamRoles_50_0= ruleUpstreamRole ) ) (otherlv_51= ',' ( (lv_upstreamRoles_52_0= ruleUpstreamRole ) ) )* )? otherlv_53= ']' )? ( (otherlv_54= RULE_ID ) )
{
// InternalContextMappingDSL.g:3390:5: ( (otherlv_42= RULE_ID ) )
// InternalContextMappingDSL.g:3391:6: (otherlv_42= RULE_ID )
{
// InternalContextMappingDSL.g:3391:6: (otherlv_42= RULE_ID )
// InternalContextMappingDSL.g:3392:7: otherlv_42= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getCustomerSupplierRelationshipRule());
}
otherlv_42=(Token)match(input,RULE_ID,FOLLOW_64);
newLeafNode(otherlv_42, grammarAccess.getCustomerSupplierRelationshipAccess().getDownstreamBoundedContextCrossReference_0_2_0_0());
}
}
// InternalContextMappingDSL.g:3403:5: (otherlv_43= '[' ( ( (lv_downstreamRoles_44_0= ruleDownstreamRole ) ) (otherlv_45= ',' ( (lv_downstreamRoles_46_0= ruleDownstreamRole ) ) )* )? otherlv_47= ']' )?
int alt97=2;
int LA97_0 = input.LA(1);
if ( (LA97_0==35) ) {
alt97=1;
}
switch (alt97) {
case 1 :
// InternalContextMappingDSL.g:3404:6: otherlv_43= '[' ( ( (lv_downstreamRoles_44_0= ruleDownstreamRole ) ) (otherlv_45= ',' ( (lv_downstreamRoles_46_0= ruleDownstreamRole ) ) )* )? otherlv_47= ']'
{
otherlv_43=(Token)match(input,35,FOLLOW_53);
newLeafNode(otherlv_43, grammarAccess.getCustomerSupplierRelationshipAccess().getLeftSquareBracketKeyword_0_2_1_0());
// InternalContextMappingDSL.g:3408:6: ( ( (lv_downstreamRoles_44_0= ruleDownstreamRole ) ) (otherlv_45= ',' ( (lv_downstreamRoles_46_0= ruleDownstreamRole ) ) )* )?
int alt96=2;
int LA96_0 = input.LA(1);
if ( ((LA96_0>=210 && LA96_0<=211)) ) {
alt96=1;
}
switch (alt96) {
case 1 :
// InternalContextMappingDSL.g:3409:7: ( (lv_downstreamRoles_44_0= ruleDownstreamRole ) ) (otherlv_45= ',' ( (lv_downstreamRoles_46_0= ruleDownstreamRole ) ) )*
{
// InternalContextMappingDSL.g:3409:7: ( (lv_downstreamRoles_44_0= ruleDownstreamRole ) )
// InternalContextMappingDSL.g:3410:8: (lv_downstreamRoles_44_0= ruleDownstreamRole )
{
// InternalContextMappingDSL.g:3410:8: (lv_downstreamRoles_44_0= ruleDownstreamRole )
// InternalContextMappingDSL.g:3411:9: lv_downstreamRoles_44_0= ruleDownstreamRole
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipAccess().getDownstreamRolesDownstreamRoleEnumRuleCall_0_2_1_1_0_0());
pushFollow(FOLLOW_44);
lv_downstreamRoles_44_0=ruleDownstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCustomerSupplierRelationshipRule());
}
add(
current,
"downstreamRoles",
lv_downstreamRoles_44_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamRole");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:3428:7: (otherlv_45= ',' ( (lv_downstreamRoles_46_0= ruleDownstreamRole ) ) )*
loop95:
do {
int alt95=2;
int LA95_0 = input.LA(1);
if ( (LA95_0==24) ) {
alt95=1;
}
switch (alt95) {
case 1 :
// InternalContextMappingDSL.g:3429:8: otherlv_45= ',' ( (lv_downstreamRoles_46_0= ruleDownstreamRole ) )
{
otherlv_45=(Token)match(input,24,FOLLOW_47);
newLeafNode(otherlv_45, grammarAccess.getCustomerSupplierRelationshipAccess().getCommaKeyword_0_2_1_1_1_0());
// InternalContextMappingDSL.g:3433:8: ( (lv_downstreamRoles_46_0= ruleDownstreamRole ) )
// InternalContextMappingDSL.g:3434:9: (lv_downstreamRoles_46_0= ruleDownstreamRole )
{
// InternalContextMappingDSL.g:3434:9: (lv_downstreamRoles_46_0= ruleDownstreamRole )
// InternalContextMappingDSL.g:3435:10: lv_downstreamRoles_46_0= ruleDownstreamRole
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipAccess().getDownstreamRolesDownstreamRoleEnumRuleCall_0_2_1_1_1_1_0());
pushFollow(FOLLOW_44);
lv_downstreamRoles_46_0=ruleDownstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCustomerSupplierRelationshipRule());
}
add(
current,
"downstreamRoles",
lv_downstreamRoles_46_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamRole");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop95;
}
} while (true);
}
break;
}
otherlv_47=(Token)match(input,37,FOLLOW_65);
newLeafNode(otherlv_47, grammarAccess.getCustomerSupplierRelationshipAccess().getRightSquareBracketKeyword_0_2_1_2());
}
break;
}
otherlv_48=(Token)match(input,53,FOLLOW_45);
newLeafNode(otherlv_48, grammarAccess.getCustomerSupplierRelationshipAccess().getCustomerSupplierKeyword_0_2_2());
// InternalContextMappingDSL.g:3463:5: (otherlv_49= '[' ( ( (lv_upstreamRoles_50_0= ruleUpstreamRole ) ) (otherlv_51= ',' ( (lv_upstreamRoles_52_0= ruleUpstreamRole ) ) )* )? otherlv_53= ']' )?
int alt100=2;
int LA100_0 = input.LA(1);
if ( (LA100_0==35) ) {
alt100=1;
}
switch (alt100) {
case 1 :
// InternalContextMappingDSL.g:3464:6: otherlv_49= '[' ( ( (lv_upstreamRoles_50_0= ruleUpstreamRole ) ) (otherlv_51= ',' ( (lv_upstreamRoles_52_0= ruleUpstreamRole ) ) )* )? otherlv_53= ']'
{
otherlv_49=(Token)match(input,35,FOLLOW_51);
newLeafNode(otherlv_49, grammarAccess.getCustomerSupplierRelationshipAccess().getLeftSquareBracketKeyword_0_2_3_0());
// InternalContextMappingDSL.g:3468:6: ( ( (lv_upstreamRoles_50_0= ruleUpstreamRole ) ) (otherlv_51= ',' ( (lv_upstreamRoles_52_0= ruleUpstreamRole ) ) )* )?
int alt99=2;
int LA99_0 = input.LA(1);
if ( ((LA99_0>=208 && LA99_0<=209)) ) {
alt99=1;
}
switch (alt99) {
case 1 :
// InternalContextMappingDSL.g:3469:7: ( (lv_upstreamRoles_50_0= ruleUpstreamRole ) ) (otherlv_51= ',' ( (lv_upstreamRoles_52_0= ruleUpstreamRole ) ) )*
{
// InternalContextMappingDSL.g:3469:7: ( (lv_upstreamRoles_50_0= ruleUpstreamRole ) )
// InternalContextMappingDSL.g:3470:8: (lv_upstreamRoles_50_0= ruleUpstreamRole )
{
// InternalContextMappingDSL.g:3470:8: (lv_upstreamRoles_50_0= ruleUpstreamRole )
// InternalContextMappingDSL.g:3471:9: lv_upstreamRoles_50_0= ruleUpstreamRole
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipAccess().getUpstreamRolesUpstreamRoleEnumRuleCall_0_2_3_1_0_0());
pushFollow(FOLLOW_44);
lv_upstreamRoles_50_0=ruleUpstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCustomerSupplierRelationshipRule());
}
add(
current,
"upstreamRoles",
lv_upstreamRoles_50_0,
"org.contextmapper.dsl.ContextMappingDSL.UpstreamRole");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:3488:7: (otherlv_51= ',' ( (lv_upstreamRoles_52_0= ruleUpstreamRole ) ) )*
loop98:
do {
int alt98=2;
int LA98_0 = input.LA(1);
if ( (LA98_0==24) ) {
alt98=1;
}
switch (alt98) {
case 1 :
// InternalContextMappingDSL.g:3489:8: otherlv_51= ',' ( (lv_upstreamRoles_52_0= ruleUpstreamRole ) )
{
otherlv_51=(Token)match(input,24,FOLLOW_42);
newLeafNode(otherlv_51, grammarAccess.getCustomerSupplierRelationshipAccess().getCommaKeyword_0_2_3_1_1_0());
// InternalContextMappingDSL.g:3493:8: ( (lv_upstreamRoles_52_0= ruleUpstreamRole ) )
// InternalContextMappingDSL.g:3494:9: (lv_upstreamRoles_52_0= ruleUpstreamRole )
{
// InternalContextMappingDSL.g:3494:9: (lv_upstreamRoles_52_0= ruleUpstreamRole )
// InternalContextMappingDSL.g:3495:10: lv_upstreamRoles_52_0= ruleUpstreamRole
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipAccess().getUpstreamRolesUpstreamRoleEnumRuleCall_0_2_3_1_1_1_0());
pushFollow(FOLLOW_44);
lv_upstreamRoles_52_0=ruleUpstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCustomerSupplierRelationshipRule());
}
add(
current,
"upstreamRoles",
lv_upstreamRoles_52_0,
"org.contextmapper.dsl.ContextMappingDSL.UpstreamRole");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop98;
}
} while (true);
}
break;
}
otherlv_53=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_53, grammarAccess.getCustomerSupplierRelationshipAccess().getRightSquareBracketKeyword_0_2_3_2());
}
break;
}
// InternalContextMappingDSL.g:3519:5: ( (otherlv_54= RULE_ID ) )
// InternalContextMappingDSL.g:3520:6: (otherlv_54= RULE_ID )
{
// InternalContextMappingDSL.g:3520:6: (otherlv_54= RULE_ID )
// InternalContextMappingDSL.g:3521:7: otherlv_54= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getCustomerSupplierRelationshipRule());
}
otherlv_54=(Token)match(input,RULE_ID,FOLLOW_33);
newLeafNode(otherlv_54, grammarAccess.getCustomerSupplierRelationshipAccess().getUpstreamBoundedContextCrossReference_0_2_4_0());
}
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:3534:4: ( ( (otherlv_55= RULE_ID ) ) (otherlv_56= '[' ( ( (lv_upstreamRoles_57_0= ruleUpstreamRole ) ) (otherlv_58= ',' ( (lv_upstreamRoles_59_0= ruleUpstreamRole ) ) )* )? otherlv_60= ']' )? otherlv_61= 'Supplier-Customer' (otherlv_62= '[' ( ( (lv_downstreamRoles_63_0= ruleDownstreamRole ) ) (otherlv_64= ',' ( (lv_downstreamRoles_65_0= ruleDownstreamRole ) ) )* )? otherlv_66= ']' )? ( (otherlv_67= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:3534:4: ( ( (otherlv_55= RULE_ID ) ) (otherlv_56= '[' ( ( (lv_upstreamRoles_57_0= ruleUpstreamRole ) ) (otherlv_58= ',' ( (lv_upstreamRoles_59_0= ruleUpstreamRole ) ) )* )? otherlv_60= ']' )? otherlv_61= 'Supplier-Customer' (otherlv_62= '[' ( ( (lv_downstreamRoles_63_0= ruleDownstreamRole ) ) (otherlv_64= ',' ( (lv_downstreamRoles_65_0= ruleDownstreamRole ) ) )* )? otherlv_66= ']' )? ( (otherlv_67= RULE_ID ) ) )
// InternalContextMappingDSL.g:3535:5: ( (otherlv_55= RULE_ID ) ) (otherlv_56= '[' ( ( (lv_upstreamRoles_57_0= ruleUpstreamRole ) ) (otherlv_58= ',' ( (lv_upstreamRoles_59_0= ruleUpstreamRole ) ) )* )? otherlv_60= ']' )? otherlv_61= 'Supplier-Customer' (otherlv_62= '[' ( ( (lv_downstreamRoles_63_0= ruleDownstreamRole ) ) (otherlv_64= ',' ( (lv_downstreamRoles_65_0= ruleDownstreamRole ) ) )* )? otherlv_66= ']' )? ( (otherlv_67= RULE_ID ) )
{
// InternalContextMappingDSL.g:3535:5: ( (otherlv_55= RULE_ID ) )
// InternalContextMappingDSL.g:3536:6: (otherlv_55= RULE_ID )
{
// InternalContextMappingDSL.g:3536:6: (otherlv_55= RULE_ID )
// InternalContextMappingDSL.g:3537:7: otherlv_55= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getCustomerSupplierRelationshipRule());
}
otherlv_55=(Token)match(input,RULE_ID,FOLLOW_66);
newLeafNode(otherlv_55, grammarAccess.getCustomerSupplierRelationshipAccess().getUpstreamBoundedContextCrossReference_0_3_0_0());
}
}
// InternalContextMappingDSL.g:3548:5: (otherlv_56= '[' ( ( (lv_upstreamRoles_57_0= ruleUpstreamRole ) ) (otherlv_58= ',' ( (lv_upstreamRoles_59_0= ruleUpstreamRole ) ) )* )? otherlv_60= ']' )?
int alt103=2;
int LA103_0 = input.LA(1);
if ( (LA103_0==35) ) {
alt103=1;
}
switch (alt103) {
case 1 :
// InternalContextMappingDSL.g:3549:6: otherlv_56= '[' ( ( (lv_upstreamRoles_57_0= ruleUpstreamRole ) ) (otherlv_58= ',' ( (lv_upstreamRoles_59_0= ruleUpstreamRole ) ) )* )? otherlv_60= ']'
{
otherlv_56=(Token)match(input,35,FOLLOW_51);
newLeafNode(otherlv_56, grammarAccess.getCustomerSupplierRelationshipAccess().getLeftSquareBracketKeyword_0_3_1_0());
// InternalContextMappingDSL.g:3553:6: ( ( (lv_upstreamRoles_57_0= ruleUpstreamRole ) ) (otherlv_58= ',' ( (lv_upstreamRoles_59_0= ruleUpstreamRole ) ) )* )?
int alt102=2;
int LA102_0 = input.LA(1);
if ( ((LA102_0>=208 && LA102_0<=209)) ) {
alt102=1;
}
switch (alt102) {
case 1 :
// InternalContextMappingDSL.g:3554:7: ( (lv_upstreamRoles_57_0= ruleUpstreamRole ) ) (otherlv_58= ',' ( (lv_upstreamRoles_59_0= ruleUpstreamRole ) ) )*
{
// InternalContextMappingDSL.g:3554:7: ( (lv_upstreamRoles_57_0= ruleUpstreamRole ) )
// InternalContextMappingDSL.g:3555:8: (lv_upstreamRoles_57_0= ruleUpstreamRole )
{
// InternalContextMappingDSL.g:3555:8: (lv_upstreamRoles_57_0= ruleUpstreamRole )
// InternalContextMappingDSL.g:3556:9: lv_upstreamRoles_57_0= ruleUpstreamRole
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipAccess().getUpstreamRolesUpstreamRoleEnumRuleCall_0_3_1_1_0_0());
pushFollow(FOLLOW_44);
lv_upstreamRoles_57_0=ruleUpstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCustomerSupplierRelationshipRule());
}
add(
current,
"upstreamRoles",
lv_upstreamRoles_57_0,
"org.contextmapper.dsl.ContextMappingDSL.UpstreamRole");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:3573:7: (otherlv_58= ',' ( (lv_upstreamRoles_59_0= ruleUpstreamRole ) ) )*
loop101:
do {
int alt101=2;
int LA101_0 = input.LA(1);
if ( (LA101_0==24) ) {
alt101=1;
}
switch (alt101) {
case 1 :
// InternalContextMappingDSL.g:3574:8: otherlv_58= ',' ( (lv_upstreamRoles_59_0= ruleUpstreamRole ) )
{
otherlv_58=(Token)match(input,24,FOLLOW_42);
newLeafNode(otherlv_58, grammarAccess.getCustomerSupplierRelationshipAccess().getCommaKeyword_0_3_1_1_1_0());
// InternalContextMappingDSL.g:3578:8: ( (lv_upstreamRoles_59_0= ruleUpstreamRole ) )
// InternalContextMappingDSL.g:3579:9: (lv_upstreamRoles_59_0= ruleUpstreamRole )
{
// InternalContextMappingDSL.g:3579:9: (lv_upstreamRoles_59_0= ruleUpstreamRole )
// InternalContextMappingDSL.g:3580:10: lv_upstreamRoles_59_0= ruleUpstreamRole
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipAccess().getUpstreamRolesUpstreamRoleEnumRuleCall_0_3_1_1_1_1_0());
pushFollow(FOLLOW_44);
lv_upstreamRoles_59_0=ruleUpstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCustomerSupplierRelationshipRule());
}
add(
current,
"upstreamRoles",
lv_upstreamRoles_59_0,
"org.contextmapper.dsl.ContextMappingDSL.UpstreamRole");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop101;
}
} while (true);
}
break;
}
otherlv_60=(Token)match(input,37,FOLLOW_67);
newLeafNode(otherlv_60, grammarAccess.getCustomerSupplierRelationshipAccess().getRightSquareBracketKeyword_0_3_1_2());
}
break;
}
otherlv_61=(Token)match(input,54,FOLLOW_45);
newLeafNode(otherlv_61, grammarAccess.getCustomerSupplierRelationshipAccess().getSupplierCustomerKeyword_0_3_2());
// InternalContextMappingDSL.g:3608:5: (otherlv_62= '[' ( ( (lv_downstreamRoles_63_0= ruleDownstreamRole ) ) (otherlv_64= ',' ( (lv_downstreamRoles_65_0= ruleDownstreamRole ) ) )* )? otherlv_66= ']' )?
int alt106=2;
int LA106_0 = input.LA(1);
if ( (LA106_0==35) ) {
alt106=1;
}
switch (alt106) {
case 1 :
// InternalContextMappingDSL.g:3609:6: otherlv_62= '[' ( ( (lv_downstreamRoles_63_0= ruleDownstreamRole ) ) (otherlv_64= ',' ( (lv_downstreamRoles_65_0= ruleDownstreamRole ) ) )* )? otherlv_66= ']'
{
otherlv_62=(Token)match(input,35,FOLLOW_53);
newLeafNode(otherlv_62, grammarAccess.getCustomerSupplierRelationshipAccess().getLeftSquareBracketKeyword_0_3_3_0());
// InternalContextMappingDSL.g:3613:6: ( ( (lv_downstreamRoles_63_0= ruleDownstreamRole ) ) (otherlv_64= ',' ( (lv_downstreamRoles_65_0= ruleDownstreamRole ) ) )* )?
int alt105=2;
int LA105_0 = input.LA(1);
if ( ((LA105_0>=210 && LA105_0<=211)) ) {
alt105=1;
}
switch (alt105) {
case 1 :
// InternalContextMappingDSL.g:3614:7: ( (lv_downstreamRoles_63_0= ruleDownstreamRole ) ) (otherlv_64= ',' ( (lv_downstreamRoles_65_0= ruleDownstreamRole ) ) )*
{
// InternalContextMappingDSL.g:3614:7: ( (lv_downstreamRoles_63_0= ruleDownstreamRole ) )
// InternalContextMappingDSL.g:3615:8: (lv_downstreamRoles_63_0= ruleDownstreamRole )
{
// InternalContextMappingDSL.g:3615:8: (lv_downstreamRoles_63_0= ruleDownstreamRole )
// InternalContextMappingDSL.g:3616:9: lv_downstreamRoles_63_0= ruleDownstreamRole
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipAccess().getDownstreamRolesDownstreamRoleEnumRuleCall_0_3_3_1_0_0());
pushFollow(FOLLOW_44);
lv_downstreamRoles_63_0=ruleDownstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCustomerSupplierRelationshipRule());
}
add(
current,
"downstreamRoles",
lv_downstreamRoles_63_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamRole");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:3633:7: (otherlv_64= ',' ( (lv_downstreamRoles_65_0= ruleDownstreamRole ) ) )*
loop104:
do {
int alt104=2;
int LA104_0 = input.LA(1);
if ( (LA104_0==24) ) {
alt104=1;
}
switch (alt104) {
case 1 :
// InternalContextMappingDSL.g:3634:8: otherlv_64= ',' ( (lv_downstreamRoles_65_0= ruleDownstreamRole ) )
{
otherlv_64=(Token)match(input,24,FOLLOW_47);
newLeafNode(otherlv_64, grammarAccess.getCustomerSupplierRelationshipAccess().getCommaKeyword_0_3_3_1_1_0());
// InternalContextMappingDSL.g:3638:8: ( (lv_downstreamRoles_65_0= ruleDownstreamRole ) )
// InternalContextMappingDSL.g:3639:9: (lv_downstreamRoles_65_0= ruleDownstreamRole )
{
// InternalContextMappingDSL.g:3639:9: (lv_downstreamRoles_65_0= ruleDownstreamRole )
// InternalContextMappingDSL.g:3640:10: lv_downstreamRoles_65_0= ruleDownstreamRole
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipAccess().getDownstreamRolesDownstreamRoleEnumRuleCall_0_3_3_1_1_1_0());
pushFollow(FOLLOW_44);
lv_downstreamRoles_65_0=ruleDownstreamRole();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCustomerSupplierRelationshipRule());
}
add(
current,
"downstreamRoles",
lv_downstreamRoles_65_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamRole");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop104;
}
} while (true);
}
break;
}
otherlv_66=(Token)match(input,37,FOLLOW_10);
newLeafNode(otherlv_66, grammarAccess.getCustomerSupplierRelationshipAccess().getRightSquareBracketKeyword_0_3_3_2());
}
break;
}
// InternalContextMappingDSL.g:3664:5: ( (otherlv_67= RULE_ID ) )
// InternalContextMappingDSL.g:3665:6: (otherlv_67= RULE_ID )
{
// InternalContextMappingDSL.g:3665:6: (otherlv_67= RULE_ID )
// InternalContextMappingDSL.g:3666:7: otherlv_67= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getCustomerSupplierRelationshipRule());
}
otherlv_67=(Token)match(input,RULE_ID,FOLLOW_33);
newLeafNode(otherlv_67, grammarAccess.getCustomerSupplierRelationshipAccess().getDownstreamBoundedContextCrossReference_0_3_4_0());
}
}
}
}
break;
}
// InternalContextMappingDSL.g:3679:3: (otherlv_68= ':' ( (lv_name_69_0= RULE_ID ) ) )?
int alt108=2;
int LA108_0 = input.LA(1);
if ( (LA108_0==40) ) {
alt108=1;
}
switch (alt108) {
case 1 :
// InternalContextMappingDSL.g:3680:4: otherlv_68= ':' ( (lv_name_69_0= RULE_ID ) )
{
otherlv_68=(Token)match(input,40,FOLLOW_10);
newLeafNode(otherlv_68, grammarAccess.getCustomerSupplierRelationshipAccess().getColonKeyword_1_0());
// InternalContextMappingDSL.g:3684:4: ( (lv_name_69_0= RULE_ID ) )
// InternalContextMappingDSL.g:3685:5: (lv_name_69_0= RULE_ID )
{
// InternalContextMappingDSL.g:3685:5: (lv_name_69_0= RULE_ID )
// InternalContextMappingDSL.g:3686:6: lv_name_69_0= RULE_ID
{
lv_name_69_0=(Token)match(input,RULE_ID,FOLLOW_23);
newLeafNode(lv_name_69_0, grammarAccess.getCustomerSupplierRelationshipAccess().getNameIDTerminalRuleCall_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getCustomerSupplierRelationshipRule());
}
setWithLastConsumed(
current,
"name",
lv_name_69_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
}
break;
}
// InternalContextMappingDSL.g:3703:3: (this_OPEN_70= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) ) this_CLOSE_84= RULE_CLOSE )?
int alt115=2;
int LA115_0 = input.LA(1);
if ( (LA115_0==RULE_OPEN) ) {
alt115=1;
}
switch (alt115) {
case 1 :
// InternalContextMappingDSL.g:3704:4: this_OPEN_70= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) ) this_CLOSE_84= RULE_CLOSE
{
this_OPEN_70=(Token)match(input,RULE_OPEN,FOLLOW_56);
newLeafNode(this_OPEN_70, grammarAccess.getCustomerSupplierRelationshipAccess().getOPENTerminalRuleCall_2_0());
// InternalContextMappingDSL.g:3708:4: ( ( ( ( ({...}? => ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:3709:5: ( ( ( ({...}? => ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:3709:5: ( ( ( ({...}? => ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:3710:6: ( ( ({...}? => ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getCustomerSupplierRelationshipAccess().getUnorderedGroup_2_1());
// InternalContextMappingDSL.g:3713:6: ( ( ({...}? => ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:3714:7: ( ({...}? => ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:3714:7: ( ({...}? => ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )*
loop114:
do {
int alt114=4;
int LA114_0 = input.LA(1);
if ( LA114_0 == 31 && getUnorderedGroupHelper().canSelect(grammarAccess.getCustomerSupplierRelationshipAccess().getUnorderedGroup_2_1(), 0) ) {
alt114=1;
}
else if ( LA114_0 == 49 && getUnorderedGroupHelper().canSelect(grammarAccess.getCustomerSupplierRelationshipAccess().getUnorderedGroup_2_1(), 1) ) {
alt114=2;
}
else if ( LA114_0 == 50 && getUnorderedGroupHelper().canSelect(grammarAccess.getCustomerSupplierRelationshipAccess().getUnorderedGroup_2_1(), 2) ) {
alt114=3;
}
switch (alt114) {
case 1 :
// InternalContextMappingDSL.g:3715:5: ({...}? => ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:3715:5: ({...}? => ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:3716:6: {...}? => ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getCustomerSupplierRelationshipAccess().getUnorderedGroup_2_1(), 0) ) {
throw new FailedPredicateException(input, "ruleCustomerSupplierRelationship", "getUnorderedGroupHelper().canSelect(grammarAccess.getCustomerSupplierRelationshipAccess().getUnorderedGroup_2_1(), 0)");
}
// InternalContextMappingDSL.g:3716:128: ( ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:3717:7: ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getCustomerSupplierRelationshipAccess().getUnorderedGroup_2_1(), 0);
// InternalContextMappingDSL.g:3720:10: ({...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:3720:11: {...}? => (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleCustomerSupplierRelationship", "true");
}
// InternalContextMappingDSL.g:3720:20: (otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:3720:21: otherlv_72= 'implementationTechnology' (otherlv_73= '=' )? ( (lv_implementationTechnology_74_0= RULE_STRING ) )
{
otherlv_72=(Token)match(input,31,FOLLOW_17);
newLeafNode(otherlv_72, grammarAccess.getCustomerSupplierRelationshipAccess().getImplementationTechnologyKeyword_2_1_0_0());
// InternalContextMappingDSL.g:3724:10: (otherlv_73= '=' )?
int alt109=2;
int LA109_0 = input.LA(1);
if ( (LA109_0==21) ) {
alt109=1;
}
switch (alt109) {
case 1 :
// InternalContextMappingDSL.g:3725:11: otherlv_73= '='
{
otherlv_73=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_73, grammarAccess.getCustomerSupplierRelationshipAccess().getEqualsSignKeyword_2_1_0_1());
}
break;
}
// InternalContextMappingDSL.g:3730:10: ( (lv_implementationTechnology_74_0= RULE_STRING ) )
// InternalContextMappingDSL.g:3731:11: (lv_implementationTechnology_74_0= RULE_STRING )
{
// InternalContextMappingDSL.g:3731:11: (lv_implementationTechnology_74_0= RULE_STRING )
// InternalContextMappingDSL.g:3732:12: lv_implementationTechnology_74_0= RULE_STRING
{
lv_implementationTechnology_74_0=(Token)match(input,RULE_STRING,FOLLOW_56);
newLeafNode(lv_implementationTechnology_74_0, grammarAccess.getCustomerSupplierRelationshipAccess().getImplementationTechnologySTRINGTerminalRuleCall_2_1_0_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getCustomerSupplierRelationshipRule());
}
setWithLastConsumed(
current,
"implementationTechnology",
lv_implementationTechnology_74_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getCustomerSupplierRelationshipAccess().getUnorderedGroup_2_1());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:3754:5: ({...}? => ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) ) )
{
// InternalContextMappingDSL.g:3754:5: ({...}? => ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) ) )
// InternalContextMappingDSL.g:3755:6: {...}? => ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getCustomerSupplierRelationshipAccess().getUnorderedGroup_2_1(), 1) ) {
throw new FailedPredicateException(input, "ruleCustomerSupplierRelationship", "getUnorderedGroupHelper().canSelect(grammarAccess.getCustomerSupplierRelationshipAccess().getUnorderedGroup_2_1(), 1)");
}
// InternalContextMappingDSL.g:3755:128: ( ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) ) )
// InternalContextMappingDSL.g:3756:7: ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getCustomerSupplierRelationshipAccess().getUnorderedGroup_2_1(), 1);
// InternalContextMappingDSL.g:3759:10: ({...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? ) )
// InternalContextMappingDSL.g:3759:11: {...}? => ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleCustomerSupplierRelationship", "true");
}
// InternalContextMappingDSL.g:3759:20: ( (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )? )
// InternalContextMappingDSL.g:3759:21: (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) ) (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )?
{
// InternalContextMappingDSL.g:3759:21: (otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) ) )
// InternalContextMappingDSL.g:3760:11: otherlv_75= 'exposedAggregates' (otherlv_76= '=' )? ( (otherlv_77= RULE_ID ) )
{
otherlv_75=(Token)match(input,49,FOLLOW_57);
newLeafNode(otherlv_75, grammarAccess.getCustomerSupplierRelationshipAccess().getExposedAggregatesKeyword_2_1_1_0_0());
// InternalContextMappingDSL.g:3764:11: (otherlv_76= '=' )?
int alt110=2;
int LA110_0 = input.LA(1);
if ( (LA110_0==21) ) {
alt110=1;
}
switch (alt110) {
case 1 :
// InternalContextMappingDSL.g:3765:12: otherlv_76= '='
{
otherlv_76=(Token)match(input,21,FOLLOW_10);
newLeafNode(otherlv_76, grammarAccess.getCustomerSupplierRelationshipAccess().getEqualsSignKeyword_2_1_1_0_1());
}
break;
}
// InternalContextMappingDSL.g:3770:11: ( (otherlv_77= RULE_ID ) )
// InternalContextMappingDSL.g:3771:12: (otherlv_77= RULE_ID )
{
// InternalContextMappingDSL.g:3771:12: (otherlv_77= RULE_ID )
// InternalContextMappingDSL.g:3772:13: otherlv_77= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getCustomerSupplierRelationshipRule());
}
otherlv_77=(Token)match(input,RULE_ID,FOLLOW_58);
newLeafNode(otherlv_77, grammarAccess.getCustomerSupplierRelationshipAccess().getUpstreamExposedAggregatesAggregateCrossReference_2_1_1_0_2_0());
}
}
}
// InternalContextMappingDSL.g:3784:10: (otherlv_78= ',' ( (otherlv_79= RULE_ID ) ) )*
loop111:
do {
int alt111=2;
int LA111_0 = input.LA(1);
if ( (LA111_0==24) ) {
alt111=1;
}
switch (alt111) {
case 1 :
// InternalContextMappingDSL.g:3785:11: otherlv_78= ',' ( (otherlv_79= RULE_ID ) )
{
otherlv_78=(Token)match(input,24,FOLLOW_10);
newLeafNode(otherlv_78, grammarAccess.getCustomerSupplierRelationshipAccess().getCommaKeyword_2_1_1_1_0());
// InternalContextMappingDSL.g:3789:11: ( (otherlv_79= RULE_ID ) )
// InternalContextMappingDSL.g:3790:12: (otherlv_79= RULE_ID )
{
// InternalContextMappingDSL.g:3790:12: (otherlv_79= RULE_ID )
// InternalContextMappingDSL.g:3791:13: otherlv_79= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getCustomerSupplierRelationshipRule());
}
otherlv_79=(Token)match(input,RULE_ID,FOLLOW_58);
newLeafNode(otherlv_79, grammarAccess.getCustomerSupplierRelationshipAccess().getUpstreamExposedAggregatesAggregateCrossReference_2_1_1_1_1_0());
}
}
}
break;
default :
break loop111;
}
} while (true);
// InternalContextMappingDSL.g:3803:10: ( (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT ) )?
int alt112=2;
int LA112_0 = input.LA(1);
if ( (LA112_0==RULE_SL_COMMENT) ) {
alt112=1;
}
switch (alt112) {
case 1 :
// InternalContextMappingDSL.g:3804:11: (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT )
{
// InternalContextMappingDSL.g:3804:11: (lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT )
// InternalContextMappingDSL.g:3805:12: lv_exposedAggregatesComment_80_0= RULE_SL_COMMENT
{
lv_exposedAggregatesComment_80_0=(Token)match(input,RULE_SL_COMMENT,FOLLOW_56);
newLeafNode(lv_exposedAggregatesComment_80_0, grammarAccess.getCustomerSupplierRelationshipAccess().getExposedAggregatesCommentSL_COMMENTTerminalRuleCall_2_1_1_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getCustomerSupplierRelationshipRule());
}
setWithLastConsumed(
current,
"exposedAggregatesComment",
lv_exposedAggregatesComment_80_0,
"org.eclipse.xtext.common.Terminals.SL_COMMENT");
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getCustomerSupplierRelationshipAccess().getUnorderedGroup_2_1());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:3827:5: ({...}? => ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) ) )
{
// InternalContextMappingDSL.g:3827:5: ({...}? => ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) ) )
// InternalContextMappingDSL.g:3828:6: {...}? => ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getCustomerSupplierRelationshipAccess().getUnorderedGroup_2_1(), 2) ) {
throw new FailedPredicateException(input, "ruleCustomerSupplierRelationship", "getUnorderedGroupHelper().canSelect(grammarAccess.getCustomerSupplierRelationshipAccess().getUnorderedGroup_2_1(), 2)");
}
// InternalContextMappingDSL.g:3828:128: ( ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) ) )
// InternalContextMappingDSL.g:3829:7: ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getCustomerSupplierRelationshipAccess().getUnorderedGroup_2_1(), 2);
// InternalContextMappingDSL.g:3832:10: ({...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) ) )
// InternalContextMappingDSL.g:3832:11: {...}? => (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleCustomerSupplierRelationship", "true");
}
// InternalContextMappingDSL.g:3832:20: (otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) ) )
// InternalContextMappingDSL.g:3832:21: otherlv_81= 'downstreamRights' (otherlv_82= '=' )? ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) )
{
otherlv_81=(Token)match(input,50,FOLLOW_59);
newLeafNode(otherlv_81, grammarAccess.getCustomerSupplierRelationshipAccess().getDownstreamRightsKeyword_2_1_2_0());
// InternalContextMappingDSL.g:3836:10: (otherlv_82= '=' )?
int alt113=2;
int LA113_0 = input.LA(1);
if ( (LA113_0==21) ) {
alt113=1;
}
switch (alt113) {
case 1 :
// InternalContextMappingDSL.g:3837:11: otherlv_82= '='
{
otherlv_82=(Token)match(input,21,FOLLOW_59);
newLeafNode(otherlv_82, grammarAccess.getCustomerSupplierRelationshipAccess().getEqualsSignKeyword_2_1_2_1());
}
break;
}
// InternalContextMappingDSL.g:3842:10: ( (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights ) )
// InternalContextMappingDSL.g:3843:11: (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights )
{
// InternalContextMappingDSL.g:3843:11: (lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights )
// InternalContextMappingDSL.g:3844:12: lv_downstreamGovernanceRights_83_0= ruleDownstreamGovernanceRights
{
newCompositeNode(grammarAccess.getCustomerSupplierRelationshipAccess().getDownstreamGovernanceRightsDownstreamGovernanceRightsEnumRuleCall_2_1_2_2_0());
pushFollow(FOLLOW_56);
lv_downstreamGovernanceRights_83_0=ruleDownstreamGovernanceRights();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCustomerSupplierRelationshipRule());
}
set(
current,
"downstreamGovernanceRights",
lv_downstreamGovernanceRights_83_0,
"org.contextmapper.dsl.ContextMappingDSL.DownstreamGovernanceRights");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getCustomerSupplierRelationshipAccess().getUnorderedGroup_2_1());
}
}
}
break;
default :
break loop114;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getCustomerSupplierRelationshipAccess().getUnorderedGroup_2_1());
}
this_CLOSE_84=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(this_CLOSE_84, grammarAccess.getCustomerSupplierRelationshipAccess().getCLOSETerminalRuleCall_2_2());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleCustomerSupplierRelationship"
// $ANTLR start "entryRuleAggregate"
// InternalContextMappingDSL.g:3883:1: entryRuleAggregate returns [EObject current=null] : iv_ruleAggregate= ruleAggregate EOF ;
public final EObject entryRuleAggregate() throws RecognitionException {
EObject current = null;
EObject iv_ruleAggregate = null;
try {
// InternalContextMappingDSL.g:3883:50: (iv_ruleAggregate= ruleAggregate EOF )
// InternalContextMappingDSL.g:3884:2: iv_ruleAggregate= ruleAggregate EOF
{
newCompositeNode(grammarAccess.getAggregateRule());
pushFollow(FOLLOW_1);
iv_ruleAggregate=ruleAggregate();
state._fsp--;
current =iv_ruleAggregate;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleAggregate"
// $ANTLR start "ruleAggregate"
// InternalContextMappingDSL.g:3890:1: ruleAggregate returns [EObject current=null] : ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? otherlv_2= 'Aggregate' ( (lv_name_3_0= RULE_ID ) ) (this_OPEN_4= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) ) ) )* ) ) ) ( ( (lv_services_26_0= ruleService ) ) | ( (lv_resources_27_0= ruleResource ) ) | ( (lv_consumers_28_0= ruleConsumer ) ) | ( (lv_domainObjects_29_0= ruleSimpleDomainObject ) ) )* this_CLOSE_30= RULE_CLOSE )? ) ;
public final EObject ruleAggregate() throws RecognitionException {
EObject current = null;
Token lv_comment_0_0=null;
Token lv_doc_1_0=null;
Token otherlv_2=null;
Token lv_name_3_0=null;
Token this_OPEN_4=null;
Token otherlv_6=null;
Token otherlv_7=null;
Token lv_responsibilities_8_0=null;
Token otherlv_9=null;
Token lv_responsibilities_10_0=null;
Token otherlv_11=null;
Token otherlv_12=null;
Token otherlv_13=null;
Token otherlv_14=null;
Token otherlv_15=null;
Token otherlv_16=null;
Token otherlv_17=null;
Token otherlv_18=null;
Token otherlv_19=null;
Token otherlv_20=null;
Token otherlv_21=null;
Token otherlv_23=null;
Token otherlv_24=null;
Token this_CLOSE_30=null;
Enumerator lv_knowledgeLevel_22_0 = null;
Enumerator lv_likelihoodForChange_25_0 = null;
EObject lv_services_26_0 = null;
EObject lv_resources_27_0 = null;
EObject lv_consumers_28_0 = null;
EObject lv_domainObjects_29_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:3896:2: ( ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? otherlv_2= 'Aggregate' ( (lv_name_3_0= RULE_ID ) ) (this_OPEN_4= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) ) ) )* ) ) ) ( ( (lv_services_26_0= ruleService ) ) | ( (lv_resources_27_0= ruleResource ) ) | ( (lv_consumers_28_0= ruleConsumer ) ) | ( (lv_domainObjects_29_0= ruleSimpleDomainObject ) ) )* this_CLOSE_30= RULE_CLOSE )? ) )
// InternalContextMappingDSL.g:3897:2: ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? otherlv_2= 'Aggregate' ( (lv_name_3_0= RULE_ID ) ) (this_OPEN_4= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) ) ) )* ) ) ) ( ( (lv_services_26_0= ruleService ) ) | ( (lv_resources_27_0= ruleResource ) ) | ( (lv_consumers_28_0= ruleConsumer ) ) | ( (lv_domainObjects_29_0= ruleSimpleDomainObject ) ) )* this_CLOSE_30= RULE_CLOSE )? )
{
// InternalContextMappingDSL.g:3897:2: ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? otherlv_2= 'Aggregate' ( (lv_name_3_0= RULE_ID ) ) (this_OPEN_4= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) ) ) )* ) ) ) ( ( (lv_services_26_0= ruleService ) ) | ( (lv_resources_27_0= ruleResource ) ) | ( (lv_consumers_28_0= ruleConsumer ) ) | ( (lv_domainObjects_29_0= ruleSimpleDomainObject ) ) )* this_CLOSE_30= RULE_CLOSE )? )
// InternalContextMappingDSL.g:3898:3: ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? otherlv_2= 'Aggregate' ( (lv_name_3_0= RULE_ID ) ) (this_OPEN_4= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) ) ) )* ) ) ) ( ( (lv_services_26_0= ruleService ) ) | ( (lv_resources_27_0= ruleResource ) ) | ( (lv_consumers_28_0= ruleConsumer ) ) | ( (lv_domainObjects_29_0= ruleSimpleDomainObject ) ) )* this_CLOSE_30= RULE_CLOSE )?
{
// InternalContextMappingDSL.g:3898:3: ( (lv_comment_0_0= RULE_ML_COMMENT ) )?
int alt116=2;
int LA116_0 = input.LA(1);
if ( (LA116_0==RULE_ML_COMMENT) ) {
alt116=1;
}
switch (alt116) {
case 1 :
// InternalContextMappingDSL.g:3899:4: (lv_comment_0_0= RULE_ML_COMMENT )
{
// InternalContextMappingDSL.g:3899:4: (lv_comment_0_0= RULE_ML_COMMENT )
// InternalContextMappingDSL.g:3900:5: lv_comment_0_0= RULE_ML_COMMENT
{
lv_comment_0_0=(Token)match(input,RULE_ML_COMMENT,FOLLOW_68);
newLeafNode(lv_comment_0_0, grammarAccess.getAggregateAccess().getCommentML_COMMENTTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getAggregateRule());
}
setWithLastConsumed(
current,
"comment",
lv_comment_0_0,
"org.eclipse.xtext.common.Terminals.ML_COMMENT");
}
}
break;
}
// InternalContextMappingDSL.g:3916:3: ( (lv_doc_1_0= RULE_STRING ) )?
int alt117=2;
int LA117_0 = input.LA(1);
if ( (LA117_0==RULE_STRING) ) {
alt117=1;
}
switch (alt117) {
case 1 :
// InternalContextMappingDSL.g:3917:4: (lv_doc_1_0= RULE_STRING )
{
// InternalContextMappingDSL.g:3917:4: (lv_doc_1_0= RULE_STRING )
// InternalContextMappingDSL.g:3918:5: lv_doc_1_0= RULE_STRING
{
lv_doc_1_0=(Token)match(input,RULE_STRING,FOLLOW_69);
newLeafNode(lv_doc_1_0, grammarAccess.getAggregateAccess().getDocSTRINGTerminalRuleCall_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getAggregateRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_1_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
otherlv_2=(Token)match(input,55,FOLLOW_10);
newLeafNode(otherlv_2, grammarAccess.getAggregateAccess().getAggregateKeyword_2());
// InternalContextMappingDSL.g:3938:3: ( (lv_name_3_0= RULE_ID ) )
// InternalContextMappingDSL.g:3939:4: (lv_name_3_0= RULE_ID )
{
// InternalContextMappingDSL.g:3939:4: (lv_name_3_0= RULE_ID )
// InternalContextMappingDSL.g:3940:5: lv_name_3_0= RULE_ID
{
lv_name_3_0=(Token)match(input,RULE_ID,FOLLOW_23);
newLeafNode(lv_name_3_0, grammarAccess.getAggregateAccess().getNameIDTerminalRuleCall_3_0());
if (current==null) {
current = createModelElement(grammarAccess.getAggregateRule());
}
setWithLastConsumed(
current,
"name",
lv_name_3_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:3956:3: (this_OPEN_4= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) ) ) )* ) ) ) ( ( (lv_services_26_0= ruleService ) ) | ( (lv_resources_27_0= ruleResource ) ) | ( (lv_consumers_28_0= ruleConsumer ) ) | ( (lv_domainObjects_29_0= ruleSimpleDomainObject ) ) )* this_CLOSE_30= RULE_CLOSE )?
int alt128=2;
int LA128_0 = input.LA(1);
if ( (LA128_0==RULE_OPEN) ) {
alt128=1;
}
switch (alt128) {
case 1 :
// InternalContextMappingDSL.g:3957:4: this_OPEN_4= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) ) ) )* ) ) ) ( ( (lv_services_26_0= ruleService ) ) | ( (lv_resources_27_0= ruleResource ) ) | ( (lv_consumers_28_0= ruleConsumer ) ) | ( (lv_domainObjects_29_0= ruleSimpleDomainObject ) ) )* this_CLOSE_30= RULE_CLOSE
{
this_OPEN_4=(Token)match(input,RULE_OPEN,FOLLOW_70);
newLeafNode(this_OPEN_4, grammarAccess.getAggregateAccess().getOPENTerminalRuleCall_4_0());
// InternalContextMappingDSL.g:3961:4: ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:3962:5: ( ( ( ({...}? => ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:3962:5: ( ( ( ({...}? => ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:3963:6: ( ( ({...}? => ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1());
// InternalContextMappingDSL.g:3966:6: ( ( ({...}? => ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:3967:7: ( ({...}? => ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:3967:7: ( ({...}? => ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) ) ) )*
loop126:
do {
int alt126=6;
int LA126_0 = input.LA(1);
if ( LA126_0 == 30 && getUnorderedGroupHelper().canSelect(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 0) ) {
alt126=1;
}
else if ( LA126_0 >= 56 && LA126_0 <= 57 && getUnorderedGroupHelper().canSelect(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 1) ) {
alt126=2;
}
else if ( LA126_0 == 58 && getUnorderedGroupHelper().canSelect(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 2) ) {
alt126=3;
}
else if ( LA126_0 == 32 && getUnorderedGroupHelper().canSelect(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 3) ) {
alt126=4;
}
else if ( LA126_0 == 59 && getUnorderedGroupHelper().canSelect(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 4) ) {
alt126=5;
}
switch (alt126) {
case 1 :
// InternalContextMappingDSL.g:3968:5: ({...}? => ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) ) )
{
// InternalContextMappingDSL.g:3968:5: ({...}? => ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) ) )
// InternalContextMappingDSL.g:3969:6: {...}? => ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 0) ) {
throw new FailedPredicateException(input, "ruleAggregate", "getUnorderedGroupHelper().canSelect(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 0)");
}
// InternalContextMappingDSL.g:3969:109: ( ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) ) )
// InternalContextMappingDSL.g:3970:7: ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 0);
// InternalContextMappingDSL.g:3973:10: ({...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* ) )
// InternalContextMappingDSL.g:3973:11: {...}? => ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAggregate", "true");
}
// InternalContextMappingDSL.g:3973:20: ( (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )* )
// InternalContextMappingDSL.g:3973:21: (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) ) (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )*
{
// InternalContextMappingDSL.g:3973:21: (otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:3974:11: otherlv_6= 'responsibilities' (otherlv_7= '=' )? ( (lv_responsibilities_8_0= RULE_STRING ) )
{
otherlv_6=(Token)match(input,30,FOLLOW_17);
newLeafNode(otherlv_6, grammarAccess.getAggregateAccess().getResponsibilitiesKeyword_4_1_0_0_0());
// InternalContextMappingDSL.g:3978:11: (otherlv_7= '=' )?
int alt118=2;
int LA118_0 = input.LA(1);
if ( (LA118_0==21) ) {
alt118=1;
}
switch (alt118) {
case 1 :
// InternalContextMappingDSL.g:3979:12: otherlv_7= '='
{
otherlv_7=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_7, grammarAccess.getAggregateAccess().getEqualsSignKeyword_4_1_0_0_1());
}
break;
}
// InternalContextMappingDSL.g:3984:11: ( (lv_responsibilities_8_0= RULE_STRING ) )
// InternalContextMappingDSL.g:3985:12: (lv_responsibilities_8_0= RULE_STRING )
{
// InternalContextMappingDSL.g:3985:12: (lv_responsibilities_8_0= RULE_STRING )
// InternalContextMappingDSL.g:3986:13: lv_responsibilities_8_0= RULE_STRING
{
lv_responsibilities_8_0=(Token)match(input,RULE_STRING,FOLLOW_71);
newLeafNode(lv_responsibilities_8_0, grammarAccess.getAggregateAccess().getResponsibilitiesSTRINGTerminalRuleCall_4_1_0_0_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getAggregateRule());
}
addWithLastConsumed(
current,
"responsibilities",
lv_responsibilities_8_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
// InternalContextMappingDSL.g:4003:10: (otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) ) )*
loop119:
do {
int alt119=2;
int LA119_0 = input.LA(1);
if ( (LA119_0==24) ) {
alt119=1;
}
switch (alt119) {
case 1 :
// InternalContextMappingDSL.g:4004:11: otherlv_9= ',' ( (lv_responsibilities_10_0= RULE_STRING ) )
{
otherlv_9=(Token)match(input,24,FOLLOW_4);
newLeafNode(otherlv_9, grammarAccess.getAggregateAccess().getCommaKeyword_4_1_0_1_0());
// InternalContextMappingDSL.g:4008:11: ( (lv_responsibilities_10_0= RULE_STRING ) )
// InternalContextMappingDSL.g:4009:12: (lv_responsibilities_10_0= RULE_STRING )
{
// InternalContextMappingDSL.g:4009:12: (lv_responsibilities_10_0= RULE_STRING )
// InternalContextMappingDSL.g:4010:13: lv_responsibilities_10_0= RULE_STRING
{
lv_responsibilities_10_0=(Token)match(input,RULE_STRING,FOLLOW_71);
newLeafNode(lv_responsibilities_10_0, grammarAccess.getAggregateAccess().getResponsibilitiesSTRINGTerminalRuleCall_4_1_0_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getAggregateRule());
}
addWithLastConsumed(
current,
"responsibilities",
lv_responsibilities_10_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
default :
break loop119;
}
} while (true);
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:4033:5: ({...}? => ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) ) )
{
// InternalContextMappingDSL.g:4033:5: ({...}? => ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) ) )
// InternalContextMappingDSL.g:4034:6: {...}? => ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 1) ) {
throw new FailedPredicateException(input, "ruleAggregate", "getUnorderedGroupHelper().canSelect(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 1)");
}
// InternalContextMappingDSL.g:4034:109: ( ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) ) )
// InternalContextMappingDSL.g:4035:7: ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 1);
// InternalContextMappingDSL.g:4038:10: ({...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* ) )
// InternalContextMappingDSL.g:4038:11: {...}? => ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAggregate", "true");
}
// InternalContextMappingDSL.g:4038:20: ( ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )* )
// InternalContextMappingDSL.g:4038:21: ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) ) (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )*
{
// InternalContextMappingDSL.g:4038:21: ( (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) ) )
// InternalContextMappingDSL.g:4039:11: (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' ) (otherlv_13= '=' )? ( (otherlv_14= RULE_ID ) )
{
// InternalContextMappingDSL.g:4039:11: (otherlv_11= 'useCases' | otherlv_12= 'userRequirements' )
int alt120=2;
int LA120_0 = input.LA(1);
if ( (LA120_0==56) ) {
alt120=1;
}
else if ( (LA120_0==57) ) {
alt120=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 120, 0, input);
throw nvae;
}
switch (alt120) {
case 1 :
// InternalContextMappingDSL.g:4040:12: otherlv_11= 'useCases'
{
otherlv_11=(Token)match(input,56,FOLLOW_57);
newLeafNode(otherlv_11, grammarAccess.getAggregateAccess().getUseCasesKeyword_4_1_1_0_0_0());
}
break;
case 2 :
// InternalContextMappingDSL.g:4045:12: otherlv_12= 'userRequirements'
{
otherlv_12=(Token)match(input,57,FOLLOW_57);
newLeafNode(otherlv_12, grammarAccess.getAggregateAccess().getUserRequirementsKeyword_4_1_1_0_0_1());
}
break;
}
// InternalContextMappingDSL.g:4050:11: (otherlv_13= '=' )?
int alt121=2;
int LA121_0 = input.LA(1);
if ( (LA121_0==21) ) {
alt121=1;
}
switch (alt121) {
case 1 :
// InternalContextMappingDSL.g:4051:12: otherlv_13= '='
{
otherlv_13=(Token)match(input,21,FOLLOW_10);
newLeafNode(otherlv_13, grammarAccess.getAggregateAccess().getEqualsSignKeyword_4_1_1_0_1());
}
break;
}
// InternalContextMappingDSL.g:4056:11: ( (otherlv_14= RULE_ID ) )
// InternalContextMappingDSL.g:4057:12: (otherlv_14= RULE_ID )
{
// InternalContextMappingDSL.g:4057:12: (otherlv_14= RULE_ID )
// InternalContextMappingDSL.g:4058:13: otherlv_14= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getAggregateRule());
}
otherlv_14=(Token)match(input,RULE_ID,FOLLOW_71);
newLeafNode(otherlv_14, grammarAccess.getAggregateAccess().getUserRequirementsUserRequirementCrossReference_4_1_1_0_2_0());
}
}
}
// InternalContextMappingDSL.g:4070:10: (otherlv_15= ',' ( (otherlv_16= RULE_ID ) ) )*
loop122:
do {
int alt122=2;
int LA122_0 = input.LA(1);
if ( (LA122_0==24) ) {
alt122=1;
}
switch (alt122) {
case 1 :
// InternalContextMappingDSL.g:4071:11: otherlv_15= ',' ( (otherlv_16= RULE_ID ) )
{
otherlv_15=(Token)match(input,24,FOLLOW_10);
newLeafNode(otherlv_15, grammarAccess.getAggregateAccess().getCommaKeyword_4_1_1_1_0());
// InternalContextMappingDSL.g:4075:11: ( (otherlv_16= RULE_ID ) )
// InternalContextMappingDSL.g:4076:12: (otherlv_16= RULE_ID )
{
// InternalContextMappingDSL.g:4076:12: (otherlv_16= RULE_ID )
// InternalContextMappingDSL.g:4077:13: otherlv_16= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getAggregateRule());
}
otherlv_16=(Token)match(input,RULE_ID,FOLLOW_71);
newLeafNode(otherlv_16, grammarAccess.getAggregateAccess().getUserRequirementsUserRequirementCrossReference_4_1_1_1_1_0());
}
}
}
break;
default :
break loop122;
}
} while (true);
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:4095:5: ({...}? => ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) ) )
{
// InternalContextMappingDSL.g:4095:5: ({...}? => ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) ) )
// InternalContextMappingDSL.g:4096:6: {...}? => ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 2) ) {
throw new FailedPredicateException(input, "ruleAggregate", "getUnorderedGroupHelper().canSelect(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 2)");
}
// InternalContextMappingDSL.g:4096:109: ( ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) ) )
// InternalContextMappingDSL.g:4097:7: ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 2);
// InternalContextMappingDSL.g:4100:10: ({...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) ) )
// InternalContextMappingDSL.g:4100:11: {...}? => (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAggregate", "true");
}
// InternalContextMappingDSL.g:4100:20: (otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) ) )
// InternalContextMappingDSL.g:4100:21: otherlv_17= 'owner' (otherlv_18= '=' )? ( (otherlv_19= RULE_ID ) )
{
otherlv_17=(Token)match(input,58,FOLLOW_57);
newLeafNode(otherlv_17, grammarAccess.getAggregateAccess().getOwnerKeyword_4_1_2_0());
// InternalContextMappingDSL.g:4104:10: (otherlv_18= '=' )?
int alt123=2;
int LA123_0 = input.LA(1);
if ( (LA123_0==21) ) {
alt123=1;
}
switch (alt123) {
case 1 :
// InternalContextMappingDSL.g:4105:11: otherlv_18= '='
{
otherlv_18=(Token)match(input,21,FOLLOW_10);
newLeafNode(otherlv_18, grammarAccess.getAggregateAccess().getEqualsSignKeyword_4_1_2_1());
}
break;
}
// InternalContextMappingDSL.g:4110:10: ( (otherlv_19= RULE_ID ) )
// InternalContextMappingDSL.g:4111:11: (otherlv_19= RULE_ID )
{
// InternalContextMappingDSL.g:4111:11: (otherlv_19= RULE_ID )
// InternalContextMappingDSL.g:4112:12: otherlv_19= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getAggregateRule());
}
otherlv_19=(Token)match(input,RULE_ID,FOLLOW_70);
newLeafNode(otherlv_19, grammarAccess.getAggregateAccess().getOwnerBoundedContextCrossReference_4_1_2_2_0());
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1());
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:4129:5: ({...}? => ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) ) )
{
// InternalContextMappingDSL.g:4129:5: ({...}? => ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) ) )
// InternalContextMappingDSL.g:4130:6: {...}? => ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 3) ) {
throw new FailedPredicateException(input, "ruleAggregate", "getUnorderedGroupHelper().canSelect(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 3)");
}
// InternalContextMappingDSL.g:4130:109: ( ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) ) )
// InternalContextMappingDSL.g:4131:7: ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 3);
// InternalContextMappingDSL.g:4134:10: ({...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) ) )
// InternalContextMappingDSL.g:4134:11: {...}? => (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAggregate", "true");
}
// InternalContextMappingDSL.g:4134:20: (otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) ) )
// InternalContextMappingDSL.g:4134:21: otherlv_20= 'knowledgeLevel' (otherlv_21= '=' )? ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) )
{
otherlv_20=(Token)match(input,32,FOLLOW_20);
newLeafNode(otherlv_20, grammarAccess.getAggregateAccess().getKnowledgeLevelKeyword_4_1_3_0());
// InternalContextMappingDSL.g:4138:10: (otherlv_21= '=' )?
int alt124=2;
int LA124_0 = input.LA(1);
if ( (LA124_0==21) ) {
alt124=1;
}
switch (alt124) {
case 1 :
// InternalContextMappingDSL.g:4139:11: otherlv_21= '='
{
otherlv_21=(Token)match(input,21,FOLLOW_20);
newLeafNode(otherlv_21, grammarAccess.getAggregateAccess().getEqualsSignKeyword_4_1_3_1());
}
break;
}
// InternalContextMappingDSL.g:4144:10: ( (lv_knowledgeLevel_22_0= ruleKnowledgeLevel ) )
// InternalContextMappingDSL.g:4145:11: (lv_knowledgeLevel_22_0= ruleKnowledgeLevel )
{
// InternalContextMappingDSL.g:4145:11: (lv_knowledgeLevel_22_0= ruleKnowledgeLevel )
// InternalContextMappingDSL.g:4146:12: lv_knowledgeLevel_22_0= ruleKnowledgeLevel
{
newCompositeNode(grammarAccess.getAggregateAccess().getKnowledgeLevelKnowledgeLevelEnumRuleCall_4_1_3_2_0());
pushFollow(FOLLOW_70);
lv_knowledgeLevel_22_0=ruleKnowledgeLevel();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getAggregateRule());
}
set(
current,
"knowledgeLevel",
lv_knowledgeLevel_22_0,
"org.contextmapper.dsl.ContextMappingDSL.KnowledgeLevel");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1());
}
}
}
break;
case 5 :
// InternalContextMappingDSL.g:4169:5: ({...}? => ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) ) )
{
// InternalContextMappingDSL.g:4169:5: ({...}? => ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) ) )
// InternalContextMappingDSL.g:4170:6: {...}? => ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 4) ) {
throw new FailedPredicateException(input, "ruleAggregate", "getUnorderedGroupHelper().canSelect(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 4)");
}
// InternalContextMappingDSL.g:4170:109: ( ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) ) )
// InternalContextMappingDSL.g:4171:7: ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1(), 4);
// InternalContextMappingDSL.g:4174:10: ({...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) ) )
// InternalContextMappingDSL.g:4174:11: {...}? => (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAggregate", "true");
}
// InternalContextMappingDSL.g:4174:20: (otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) ) )
// InternalContextMappingDSL.g:4174:21: otherlv_23= 'likelihoodForChange' (otherlv_24= '=' )? ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) )
{
otherlv_23=(Token)match(input,59,FOLLOW_72);
newLeafNode(otherlv_23, grammarAccess.getAggregateAccess().getLikelihoodForChangeKeyword_4_1_4_0());
// InternalContextMappingDSL.g:4178:10: (otherlv_24= '=' )?
int alt125=2;
int LA125_0 = input.LA(1);
if ( (LA125_0==21) ) {
alt125=1;
}
switch (alt125) {
case 1 :
// InternalContextMappingDSL.g:4179:11: otherlv_24= '='
{
otherlv_24=(Token)match(input,21,FOLLOW_72);
newLeafNode(otherlv_24, grammarAccess.getAggregateAccess().getEqualsSignKeyword_4_1_4_1());
}
break;
}
// InternalContextMappingDSL.g:4184:10: ( (lv_likelihoodForChange_25_0= ruleLikelihoodForChange ) )
// InternalContextMappingDSL.g:4185:11: (lv_likelihoodForChange_25_0= ruleLikelihoodForChange )
{
// InternalContextMappingDSL.g:4185:11: (lv_likelihoodForChange_25_0= ruleLikelihoodForChange )
// InternalContextMappingDSL.g:4186:12: lv_likelihoodForChange_25_0= ruleLikelihoodForChange
{
newCompositeNode(grammarAccess.getAggregateAccess().getLikelihoodForChangeLikelihoodForChangeEnumRuleCall_4_1_4_2_0());
pushFollow(FOLLOW_70);
lv_likelihoodForChange_25_0=ruleLikelihoodForChange();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getAggregateRule());
}
set(
current,
"likelihoodForChange",
lv_likelihoodForChange_25_0,
"org.contextmapper.dsl.ContextMappingDSL.LikelihoodForChange");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1());
}
}
}
break;
default :
break loop126;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getAggregateAccess().getUnorderedGroup_4_1());
}
// InternalContextMappingDSL.g:4216:4: ( ( (lv_services_26_0= ruleService ) ) | ( (lv_resources_27_0= ruleResource ) ) | ( (lv_consumers_28_0= ruleConsumer ) ) | ( (lv_domainObjects_29_0= ruleSimpleDomainObject ) ) )*
loop127:
do {
int alt127=5;
switch ( input.LA(1) ) {
case RULE_ML_COMMENT:
{
switch ( input.LA(2) ) {
case RULE_STRING:
{
int LA127_8 = input.LA(3);
if ( (LA127_8==85) ) {
alt127=1;
}
else if ( ((LA127_8>=120 && LA127_8<=121)||LA127_8==136||(LA127_8>=139 && LA127_8<=140)||LA127_8==144) ) {
alt127=4;
}
}
break;
case 120:
case 121:
case 136:
case 139:
case 140:
case 144:
{
alt127=4;
}
break;
case 85:
{
alt127=1;
}
break;
}
}
break;
case RULE_STRING:
{
switch ( input.LA(2) ) {
case 89:
{
alt127=2;
}
break;
case 85:
{
alt127=1;
}
break;
case 92:
{
alt127=3;
}
break;
case 120:
case 121:
case 136:
case 139:
case 140:
case 141:
case 144:
case 145:
case 183:
{
alt127=4;
}
break;
}
}
break;
case 85:
{
alt127=1;
}
break;
case 89:
{
alt127=2;
}
break;
case 92:
{
alt127=3;
}
break;
case 120:
case 121:
case 136:
case 139:
case 140:
case 141:
case 144:
case 145:
case 183:
{
alt127=4;
}
break;
}
switch (alt127) {
case 1 :
// InternalContextMappingDSL.g:4217:5: ( (lv_services_26_0= ruleService ) )
{
// InternalContextMappingDSL.g:4217:5: ( (lv_services_26_0= ruleService ) )
// InternalContextMappingDSL.g:4218:6: (lv_services_26_0= ruleService )
{
// InternalContextMappingDSL.g:4218:6: (lv_services_26_0= ruleService )
// InternalContextMappingDSL.g:4219:7: lv_services_26_0= ruleService
{
newCompositeNode(grammarAccess.getAggregateAccess().getServicesServiceParserRuleCall_4_2_0_0());
pushFollow(FOLLOW_73);
lv_services_26_0=ruleService();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getAggregateRule());
}
add(
current,
"services",
lv_services_26_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Service");
afterParserOrEnumRuleCall();
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:4237:5: ( (lv_resources_27_0= ruleResource ) )
{
// InternalContextMappingDSL.g:4237:5: ( (lv_resources_27_0= ruleResource ) )
// InternalContextMappingDSL.g:4238:6: (lv_resources_27_0= ruleResource )
{
// InternalContextMappingDSL.g:4238:6: (lv_resources_27_0= ruleResource )
// InternalContextMappingDSL.g:4239:7: lv_resources_27_0= ruleResource
{
newCompositeNode(grammarAccess.getAggregateAccess().getResourcesResourceParserRuleCall_4_2_1_0());
pushFollow(FOLLOW_73);
lv_resources_27_0=ruleResource();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getAggregateRule());
}
add(
current,
"resources",
lv_resources_27_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Resource");
afterParserOrEnumRuleCall();
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:4257:5: ( (lv_consumers_28_0= ruleConsumer ) )
{
// InternalContextMappingDSL.g:4257:5: ( (lv_consumers_28_0= ruleConsumer ) )
// InternalContextMappingDSL.g:4258:6: (lv_consumers_28_0= ruleConsumer )
{
// InternalContextMappingDSL.g:4258:6: (lv_consumers_28_0= ruleConsumer )
// InternalContextMappingDSL.g:4259:7: lv_consumers_28_0= ruleConsumer
{
newCompositeNode(grammarAccess.getAggregateAccess().getConsumersConsumerParserRuleCall_4_2_2_0());
pushFollow(FOLLOW_73);
lv_consumers_28_0=ruleConsumer();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getAggregateRule());
}
add(
current,
"consumers",
lv_consumers_28_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Consumer");
afterParserOrEnumRuleCall();
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:4277:5: ( (lv_domainObjects_29_0= ruleSimpleDomainObject ) )
{
// InternalContextMappingDSL.g:4277:5: ( (lv_domainObjects_29_0= ruleSimpleDomainObject ) )
// InternalContextMappingDSL.g:4278:6: (lv_domainObjects_29_0= ruleSimpleDomainObject )
{
// InternalContextMappingDSL.g:4278:6: (lv_domainObjects_29_0= ruleSimpleDomainObject )
// InternalContextMappingDSL.g:4279:7: lv_domainObjects_29_0= ruleSimpleDomainObject
{
newCompositeNode(grammarAccess.getAggregateAccess().getDomainObjectsSimpleDomainObjectParserRuleCall_4_2_3_0());
pushFollow(FOLLOW_73);
lv_domainObjects_29_0=ruleSimpleDomainObject();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getAggregateRule());
}
add(
current,
"domainObjects",
lv_domainObjects_29_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.SimpleDomainObject");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop127;
}
} while (true);
this_CLOSE_30=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(this_CLOSE_30, grammarAccess.getAggregateAccess().getCLOSETerminalRuleCall_4_3());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleAggregate"
// $ANTLR start "entryRuleUserRequirement"
// InternalContextMappingDSL.g:4306:1: entryRuleUserRequirement returns [EObject current=null] : iv_ruleUserRequirement= ruleUserRequirement EOF ;
public final EObject entryRuleUserRequirement() throws RecognitionException {
EObject current = null;
EObject iv_ruleUserRequirement = null;
try {
// InternalContextMappingDSL.g:4306:56: (iv_ruleUserRequirement= ruleUserRequirement EOF )
// InternalContextMappingDSL.g:4307:2: iv_ruleUserRequirement= ruleUserRequirement EOF
{
newCompositeNode(grammarAccess.getUserRequirementRule());
pushFollow(FOLLOW_1);
iv_ruleUserRequirement=ruleUserRequirement();
state._fsp--;
current =iv_ruleUserRequirement;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleUserRequirement"
// $ANTLR start "ruleUserRequirement"
// InternalContextMappingDSL.g:4313:1: ruleUserRequirement returns [EObject current=null] : (this_UseCase_0= ruleUseCase | this_UserStory_1= ruleUserStory ) ;
public final EObject ruleUserRequirement() throws RecognitionException {
EObject current = null;
EObject this_UseCase_0 = null;
EObject this_UserStory_1 = null;
enterRule();
try {
// InternalContextMappingDSL.g:4319:2: ( (this_UseCase_0= ruleUseCase | this_UserStory_1= ruleUserStory ) )
// InternalContextMappingDSL.g:4320:2: (this_UseCase_0= ruleUseCase | this_UserStory_1= ruleUserStory )
{
// InternalContextMappingDSL.g:4320:2: (this_UseCase_0= ruleUseCase | this_UserStory_1= ruleUserStory )
int alt129=2;
int LA129_0 = input.LA(1);
if ( (LA129_0==60) ) {
alt129=1;
}
else if ( (LA129_0==68) ) {
alt129=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 129, 0, input);
throw nvae;
}
switch (alt129) {
case 1 :
// InternalContextMappingDSL.g:4321:3: this_UseCase_0= ruleUseCase
{
newCompositeNode(grammarAccess.getUserRequirementAccess().getUseCaseParserRuleCall_0());
pushFollow(FOLLOW_2);
this_UseCase_0=ruleUseCase();
state._fsp--;
current = this_UseCase_0;
afterParserOrEnumRuleCall();
}
break;
case 2 :
// InternalContextMappingDSL.g:4330:3: this_UserStory_1= ruleUserStory
{
newCompositeNode(grammarAccess.getUserRequirementAccess().getUserStoryParserRuleCall_1());
pushFollow(FOLLOW_2);
this_UserStory_1=ruleUserStory();
state._fsp--;
current = this_UserStory_1;
afterParserOrEnumRuleCall();
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleUserRequirement"
// $ANTLR start "entryRuleUseCase"
// InternalContextMappingDSL.g:4342:1: entryRuleUseCase returns [EObject current=null] : iv_ruleUseCase= ruleUseCase EOF ;
public final EObject entryRuleUseCase() throws RecognitionException {
EObject current = null;
EObject iv_ruleUseCase = null;
try {
// InternalContextMappingDSL.g:4342:48: (iv_ruleUseCase= ruleUseCase EOF )
// InternalContextMappingDSL.g:4343:2: iv_ruleUseCase= ruleUseCase EOF
{
newCompositeNode(grammarAccess.getUseCaseRule());
pushFollow(FOLLOW_1);
iv_ruleUseCase=ruleUseCase();
state._fsp--;
current =iv_ruleUseCase;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleUseCase"
// $ANTLR start "ruleUseCase"
// InternalContextMappingDSL.g:4349:1: ruleUseCase returns [EObject current=null] : (otherlv_0= 'UseCase' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) ) ) )* ) ) ) this_CLOSE_26= RULE_CLOSE )? ) ;
public final EObject ruleUseCase() throws RecognitionException {
EObject current = null;
Token otherlv_0=null;
Token lv_name_1_0=null;
Token this_OPEN_2=null;
Token otherlv_4=null;
Token otherlv_5=null;
Token lv_role_6_0=null;
Token otherlv_7=null;
Token otherlv_8=null;
Token otherlv_10=null;
Token otherlv_12=null;
Token otherlv_13=null;
Token lv_benefit_14_0=null;
Token otherlv_15=null;
Token otherlv_16=null;
Token lv_isLatencyCritical_17_0=null;
Token otherlv_18=null;
Token lv_nanoentitiesRead_19_0=null;
Token otherlv_20=null;
Token lv_nanoentitiesRead_21_0=null;
Token otherlv_22=null;
Token lv_nanoentitiesWritten_23_0=null;
Token otherlv_24=null;
Token lv_nanoentitiesWritten_25_0=null;
Token this_CLOSE_26=null;
EObject lv_features_9_0 = null;
EObject lv_features_11_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:4355:2: ( (otherlv_0= 'UseCase' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) ) ) )* ) ) ) this_CLOSE_26= RULE_CLOSE )? ) )
// InternalContextMappingDSL.g:4356:2: (otherlv_0= 'UseCase' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) ) ) )* ) ) ) this_CLOSE_26= RULE_CLOSE )? )
{
// InternalContextMappingDSL.g:4356:2: (otherlv_0= 'UseCase' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) ) ) )* ) ) ) this_CLOSE_26= RULE_CLOSE )? )
// InternalContextMappingDSL.g:4357:3: otherlv_0= 'UseCase' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) ) ) )* ) ) ) this_CLOSE_26= RULE_CLOSE )?
{
otherlv_0=(Token)match(input,60,FOLLOW_10);
newLeafNode(otherlv_0, grammarAccess.getUseCaseAccess().getUseCaseKeyword_0());
// InternalContextMappingDSL.g:4361:3: ( (lv_name_1_0= RULE_ID ) )
// InternalContextMappingDSL.g:4362:4: (lv_name_1_0= RULE_ID )
{
// InternalContextMappingDSL.g:4362:4: (lv_name_1_0= RULE_ID )
// InternalContextMappingDSL.g:4363:5: lv_name_1_0= RULE_ID
{
lv_name_1_0=(Token)match(input,RULE_ID,FOLLOW_23);
newLeafNode(lv_name_1_0, grammarAccess.getUseCaseAccess().getNameIDTerminalRuleCall_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getUseCaseRule());
}
setWithLastConsumed(
current,
"name",
lv_name_1_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:4379:3: (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) ) ) )* ) ) ) this_CLOSE_26= RULE_CLOSE )?
int alt140=2;
int LA140_0 = input.LA(1);
if ( (LA140_0==RULE_OPEN) ) {
alt140=1;
}
switch (alt140) {
case 1 :
// InternalContextMappingDSL.g:4380:4: this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) ) ) )* ) ) ) this_CLOSE_26= RULE_CLOSE
{
this_OPEN_2=(Token)match(input,RULE_OPEN,FOLLOW_74);
newLeafNode(this_OPEN_2, grammarAccess.getUseCaseAccess().getOPENTerminalRuleCall_2_0());
// InternalContextMappingDSL.g:4384:4: ( ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:4385:5: ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:4385:5: ( ( ( ({...}? => ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) ) ) )* ) )
// InternalContextMappingDSL.g:4386:6: ( ( ({...}? => ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1());
// InternalContextMappingDSL.g:4389:6: ( ( ({...}? => ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) ) ) )* )
// InternalContextMappingDSL.g:4390:7: ( ({...}? => ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) ) ) )*
{
// InternalContextMappingDSL.g:4390:7: ( ({...}? => ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) ) ) )*
loop139:
do {
int alt139=7;
int LA139_0 = input.LA(1);
if ( LA139_0 == 61 && getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 0) ) {
alt139=1;
}
else if ( LA139_0 == 62 && getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 1) ) {
alt139=2;
}
else if ( LA139_0 == 63 && getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 2) ) {
alt139=3;
}
else if ( LA139_0 == 64 && getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 3) ) {
alt139=4;
}
else if ( LA139_0 == 66 && getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 4) ) {
alt139=5;
}
else if ( LA139_0 == 67 && getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 5) ) {
alt139=6;
}
switch (alt139) {
case 1 :
// InternalContextMappingDSL.g:4391:5: ({...}? => ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:4391:5: ({...}? => ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:4392:6: {...}? => ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 0) ) {
throw new FailedPredicateException(input, "ruleUseCase", "getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 0)");
}
// InternalContextMappingDSL.g:4392:107: ( ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:4393:7: ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 0);
// InternalContextMappingDSL.g:4396:10: ({...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:4396:11: {...}? => (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleUseCase", "true");
}
// InternalContextMappingDSL.g:4396:20: (otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:4396:21: otherlv_4= 'actor' (otherlv_5= '=' )? ( (lv_role_6_0= RULE_STRING ) )
{
otherlv_4=(Token)match(input,61,FOLLOW_17);
newLeafNode(otherlv_4, grammarAccess.getUseCaseAccess().getActorKeyword_2_1_0_0());
// InternalContextMappingDSL.g:4400:10: (otherlv_5= '=' )?
int alt130=2;
int LA130_0 = input.LA(1);
if ( (LA130_0==21) ) {
alt130=1;
}
switch (alt130) {
case 1 :
// InternalContextMappingDSL.g:4401:11: otherlv_5= '='
{
otherlv_5=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_5, grammarAccess.getUseCaseAccess().getEqualsSignKeyword_2_1_0_1());
}
break;
}
// InternalContextMappingDSL.g:4406:10: ( (lv_role_6_0= RULE_STRING ) )
// InternalContextMappingDSL.g:4407:11: (lv_role_6_0= RULE_STRING )
{
// InternalContextMappingDSL.g:4407:11: (lv_role_6_0= RULE_STRING )
// InternalContextMappingDSL.g:4408:12: lv_role_6_0= RULE_STRING
{
lv_role_6_0=(Token)match(input,RULE_STRING,FOLLOW_74);
newLeafNode(lv_role_6_0, grammarAccess.getUseCaseAccess().getRoleSTRINGTerminalRuleCall_2_1_0_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getUseCaseRule());
}
setWithLastConsumed(
current,
"role",
lv_role_6_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:4430:5: ({...}? => ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) ) )
{
// InternalContextMappingDSL.g:4430:5: ({...}? => ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) ) )
// InternalContextMappingDSL.g:4431:6: {...}? => ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 1) ) {
throw new FailedPredicateException(input, "ruleUseCase", "getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 1)");
}
// InternalContextMappingDSL.g:4431:107: ( ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) ) )
// InternalContextMappingDSL.g:4432:7: ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) )
{
getUnorderedGroupHelper().select(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 1);
// InternalContextMappingDSL.g:4435:10: ({...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* ) )
// InternalContextMappingDSL.g:4435:11: {...}? => (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleUseCase", "true");
}
// InternalContextMappingDSL.g:4435:20: (otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )* )
// InternalContextMappingDSL.g:4435:21: otherlv_7= 'interactions' (otherlv_8= '=' )? ( (lv_features_9_0= ruleFeature ) ) (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )*
{
otherlv_7=(Token)match(input,62,FOLLOW_75);
newLeafNode(otherlv_7, grammarAccess.getUseCaseAccess().getInteractionsKeyword_2_1_1_0());
// InternalContextMappingDSL.g:4439:10: (otherlv_8= '=' )?
int alt131=2;
int LA131_0 = input.LA(1);
if ( (LA131_0==21) ) {
alt131=1;
}
switch (alt131) {
case 1 :
// InternalContextMappingDSL.g:4440:11: otherlv_8= '='
{
otherlv_8=(Token)match(input,21,FOLLOW_75);
newLeafNode(otherlv_8, grammarAccess.getUseCaseAccess().getEqualsSignKeyword_2_1_1_1());
}
break;
}
// InternalContextMappingDSL.g:4445:10: ( (lv_features_9_0= ruleFeature ) )
// InternalContextMappingDSL.g:4446:11: (lv_features_9_0= ruleFeature )
{
// InternalContextMappingDSL.g:4446:11: (lv_features_9_0= ruleFeature )
// InternalContextMappingDSL.g:4447:12: lv_features_9_0= ruleFeature
{
newCompositeNode(grammarAccess.getUseCaseAccess().getFeaturesFeatureParserRuleCall_2_1_1_2_0());
pushFollow(FOLLOW_76);
lv_features_9_0=ruleFeature();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUseCaseRule());
}
add(
current,
"features",
lv_features_9_0,
"org.contextmapper.dsl.ContextMappingDSL.Feature");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:4464:10: (otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) ) )*
loop132:
do {
int alt132=2;
int LA132_0 = input.LA(1);
if ( (LA132_0==24) ) {
alt132=1;
}
switch (alt132) {
case 1 :
// InternalContextMappingDSL.g:4465:11: otherlv_10= ',' ( (lv_features_11_0= ruleFeature ) )
{
otherlv_10=(Token)match(input,24,FOLLOW_75);
newLeafNode(otherlv_10, grammarAccess.getUseCaseAccess().getCommaKeyword_2_1_1_3_0());
// InternalContextMappingDSL.g:4469:11: ( (lv_features_11_0= ruleFeature ) )
// InternalContextMappingDSL.g:4470:12: (lv_features_11_0= ruleFeature )
{
// InternalContextMappingDSL.g:4470:12: (lv_features_11_0= ruleFeature )
// InternalContextMappingDSL.g:4471:13: lv_features_11_0= ruleFeature
{
newCompositeNode(grammarAccess.getUseCaseAccess().getFeaturesFeatureParserRuleCall_2_1_1_3_1_0());
pushFollow(FOLLOW_76);
lv_features_11_0=ruleFeature();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUseCaseRule());
}
add(
current,
"features",
lv_features_11_0,
"org.contextmapper.dsl.ContextMappingDSL.Feature");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop132;
}
} while (true);
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:4495:5: ({...}? => ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:4495:5: ({...}? => ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:4496:6: {...}? => ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 2) ) {
throw new FailedPredicateException(input, "ruleUseCase", "getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 2)");
}
// InternalContextMappingDSL.g:4496:107: ( ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:4497:7: ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 2);
// InternalContextMappingDSL.g:4500:10: ({...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:4500:11: {...}? => (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleUseCase", "true");
}
// InternalContextMappingDSL.g:4500:20: (otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:4500:21: otherlv_12= 'benefit' (otherlv_13= '=' )? ( (lv_benefit_14_0= RULE_STRING ) )
{
otherlv_12=(Token)match(input,63,FOLLOW_17);
newLeafNode(otherlv_12, grammarAccess.getUseCaseAccess().getBenefitKeyword_2_1_2_0());
// InternalContextMappingDSL.g:4504:10: (otherlv_13= '=' )?
int alt133=2;
int LA133_0 = input.LA(1);
if ( (LA133_0==21) ) {
alt133=1;
}
switch (alt133) {
case 1 :
// InternalContextMappingDSL.g:4505:11: otherlv_13= '='
{
otherlv_13=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_13, grammarAccess.getUseCaseAccess().getEqualsSignKeyword_2_1_2_1());
}
break;
}
// InternalContextMappingDSL.g:4510:10: ( (lv_benefit_14_0= RULE_STRING ) )
// InternalContextMappingDSL.g:4511:11: (lv_benefit_14_0= RULE_STRING )
{
// InternalContextMappingDSL.g:4511:11: (lv_benefit_14_0= RULE_STRING )
// InternalContextMappingDSL.g:4512:12: lv_benefit_14_0= RULE_STRING
{
lv_benefit_14_0=(Token)match(input,RULE_STRING,FOLLOW_74);
newLeafNode(lv_benefit_14_0, grammarAccess.getUseCaseAccess().getBenefitSTRINGTerminalRuleCall_2_1_2_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getUseCaseRule());
}
setWithLastConsumed(
current,
"benefit",
lv_benefit_14_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1());
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:4534:5: ({...}? => ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) ) )
{
// InternalContextMappingDSL.g:4534:5: ({...}? => ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) ) )
// InternalContextMappingDSL.g:4535:6: {...}? => ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 3) ) {
throw new FailedPredicateException(input, "ruleUseCase", "getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 3)");
}
// InternalContextMappingDSL.g:4535:107: ( ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) ) )
// InternalContextMappingDSL.g:4536:7: ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 3);
// InternalContextMappingDSL.g:4539:10: ({...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) ) )
// InternalContextMappingDSL.g:4539:11: {...}? => (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleUseCase", "true");
}
// InternalContextMappingDSL.g:4539:20: (otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) ) )
// InternalContextMappingDSL.g:4539:21: otherlv_15= 'isLatencyCritical' (otherlv_16= '=' )? ( (lv_isLatencyCritical_17_0= 'true' ) )
{
otherlv_15=(Token)match(input,64,FOLLOW_77);
newLeafNode(otherlv_15, grammarAccess.getUseCaseAccess().getIsLatencyCriticalKeyword_2_1_3_0());
// InternalContextMappingDSL.g:4543:10: (otherlv_16= '=' )?
int alt134=2;
int LA134_0 = input.LA(1);
if ( (LA134_0==21) ) {
alt134=1;
}
switch (alt134) {
case 1 :
// InternalContextMappingDSL.g:4544:11: otherlv_16= '='
{
otherlv_16=(Token)match(input,21,FOLLOW_78);
newLeafNode(otherlv_16, grammarAccess.getUseCaseAccess().getEqualsSignKeyword_2_1_3_1());
}
break;
}
// InternalContextMappingDSL.g:4549:10: ( (lv_isLatencyCritical_17_0= 'true' ) )
// InternalContextMappingDSL.g:4550:11: (lv_isLatencyCritical_17_0= 'true' )
{
// InternalContextMappingDSL.g:4550:11: (lv_isLatencyCritical_17_0= 'true' )
// InternalContextMappingDSL.g:4551:12: lv_isLatencyCritical_17_0= 'true'
{
lv_isLatencyCritical_17_0=(Token)match(input,65,FOLLOW_74);
newLeafNode(lv_isLatencyCritical_17_0, grammarAccess.getUseCaseAccess().getIsLatencyCriticalTrueKeyword_2_1_3_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getUseCaseRule());
}
setWithLastConsumed(current, "isLatencyCritical", true, "true");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1());
}
}
}
break;
case 5 :
// InternalContextMappingDSL.g:4569:5: ({...}? => ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) ) )
{
// InternalContextMappingDSL.g:4569:5: ({...}? => ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) ) )
// InternalContextMappingDSL.g:4570:6: {...}? => ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 4) ) {
throw new FailedPredicateException(input, "ruleUseCase", "getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 4)");
}
// InternalContextMappingDSL.g:4570:107: ( ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) ) )
// InternalContextMappingDSL.g:4571:7: ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) )
{
getUnorderedGroupHelper().select(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 4);
// InternalContextMappingDSL.g:4574:10: ({...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* ) )
// InternalContextMappingDSL.g:4574:11: {...}? => ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleUseCase", "true");
}
// InternalContextMappingDSL.g:4574:20: ( (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )* )
// InternalContextMappingDSL.g:4574:21: (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )*
{
// InternalContextMappingDSL.g:4574:21: (otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )* )
// InternalContextMappingDSL.g:4575:11: otherlv_18= 'reads' ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )*
{
otherlv_18=(Token)match(input,66,FOLLOW_79);
newLeafNode(otherlv_18, grammarAccess.getUseCaseAccess().getReadsKeyword_2_1_4_0_0());
// InternalContextMappingDSL.g:4579:11: ( (lv_nanoentitiesRead_19_0= RULE_STRING ) )*
loop135:
do {
int alt135=2;
int LA135_0 = input.LA(1);
if ( (LA135_0==RULE_STRING) ) {
alt135=1;
}
switch (alt135) {
case 1 :
// InternalContextMappingDSL.g:4580:12: (lv_nanoentitiesRead_19_0= RULE_STRING )
{
// InternalContextMappingDSL.g:4580:12: (lv_nanoentitiesRead_19_0= RULE_STRING )
// InternalContextMappingDSL.g:4581:13: lv_nanoentitiesRead_19_0= RULE_STRING
{
lv_nanoentitiesRead_19_0=(Token)match(input,RULE_STRING,FOLLOW_79);
newLeafNode(lv_nanoentitiesRead_19_0, grammarAccess.getUseCaseAccess().getNanoentitiesReadSTRINGTerminalRuleCall_2_1_4_0_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getUseCaseRule());
}
addWithLastConsumed(
current,
"nanoentitiesRead",
lv_nanoentitiesRead_19_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
default :
break loop135;
}
} while (true);
}
// InternalContextMappingDSL.g:4598:10: (otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) ) )*
loop136:
do {
int alt136=2;
int LA136_0 = input.LA(1);
if ( (LA136_0==24) ) {
alt136=1;
}
switch (alt136) {
case 1 :
// InternalContextMappingDSL.g:4599:11: otherlv_20= ',' ( (lv_nanoentitiesRead_21_0= RULE_STRING ) )
{
otherlv_20=(Token)match(input,24,FOLLOW_4);
newLeafNode(otherlv_20, grammarAccess.getUseCaseAccess().getCommaKeyword_2_1_4_1_0());
// InternalContextMappingDSL.g:4603:11: ( (lv_nanoentitiesRead_21_0= RULE_STRING ) )
// InternalContextMappingDSL.g:4604:12: (lv_nanoentitiesRead_21_0= RULE_STRING )
{
// InternalContextMappingDSL.g:4604:12: (lv_nanoentitiesRead_21_0= RULE_STRING )
// InternalContextMappingDSL.g:4605:13: lv_nanoentitiesRead_21_0= RULE_STRING
{
lv_nanoentitiesRead_21_0=(Token)match(input,RULE_STRING,FOLLOW_76);
newLeafNode(lv_nanoentitiesRead_21_0, grammarAccess.getUseCaseAccess().getNanoentitiesReadSTRINGTerminalRuleCall_2_1_4_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getUseCaseRule());
}
addWithLastConsumed(
current,
"nanoentitiesRead",
lv_nanoentitiesRead_21_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
default :
break loop136;
}
} while (true);
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1());
}
}
}
break;
case 6 :
// InternalContextMappingDSL.g:4628:5: ({...}? => ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) ) )
{
// InternalContextMappingDSL.g:4628:5: ({...}? => ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) ) )
// InternalContextMappingDSL.g:4629:6: {...}? => ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 5) ) {
throw new FailedPredicateException(input, "ruleUseCase", "getUnorderedGroupHelper().canSelect(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 5)");
}
// InternalContextMappingDSL.g:4629:107: ( ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) ) )
// InternalContextMappingDSL.g:4630:7: ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) )
{
getUnorderedGroupHelper().select(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1(), 5);
// InternalContextMappingDSL.g:4633:10: ({...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* ) )
// InternalContextMappingDSL.g:4633:11: {...}? => ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleUseCase", "true");
}
// InternalContextMappingDSL.g:4633:20: ( (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )* )
// InternalContextMappingDSL.g:4633:21: (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* ) (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )*
{
// InternalContextMappingDSL.g:4633:21: (otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )* )
// InternalContextMappingDSL.g:4634:11: otherlv_22= 'writes' ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )*
{
otherlv_22=(Token)match(input,67,FOLLOW_79);
newLeafNode(otherlv_22, grammarAccess.getUseCaseAccess().getWritesKeyword_2_1_5_0_0());
// InternalContextMappingDSL.g:4638:11: ( (lv_nanoentitiesWritten_23_0= RULE_STRING ) )*
loop137:
do {
int alt137=2;
int LA137_0 = input.LA(1);
if ( (LA137_0==RULE_STRING) ) {
alt137=1;
}
switch (alt137) {
case 1 :
// InternalContextMappingDSL.g:4639:12: (lv_nanoentitiesWritten_23_0= RULE_STRING )
{
// InternalContextMappingDSL.g:4639:12: (lv_nanoentitiesWritten_23_0= RULE_STRING )
// InternalContextMappingDSL.g:4640:13: lv_nanoentitiesWritten_23_0= RULE_STRING
{
lv_nanoentitiesWritten_23_0=(Token)match(input,RULE_STRING,FOLLOW_79);
newLeafNode(lv_nanoentitiesWritten_23_0, grammarAccess.getUseCaseAccess().getNanoentitiesWrittenSTRINGTerminalRuleCall_2_1_5_0_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getUseCaseRule());
}
addWithLastConsumed(
current,
"nanoentitiesWritten",
lv_nanoentitiesWritten_23_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
default :
break loop137;
}
} while (true);
}
// InternalContextMappingDSL.g:4657:10: (otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) ) )*
loop138:
do {
int alt138=2;
int LA138_0 = input.LA(1);
if ( (LA138_0==24) ) {
alt138=1;
}
switch (alt138) {
case 1 :
// InternalContextMappingDSL.g:4658:11: otherlv_24= ',' ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) )
{
otherlv_24=(Token)match(input,24,FOLLOW_4);
newLeafNode(otherlv_24, grammarAccess.getUseCaseAccess().getCommaKeyword_2_1_5_1_0());
// InternalContextMappingDSL.g:4662:11: ( (lv_nanoentitiesWritten_25_0= RULE_STRING ) )
// InternalContextMappingDSL.g:4663:12: (lv_nanoentitiesWritten_25_0= RULE_STRING )
{
// InternalContextMappingDSL.g:4663:12: (lv_nanoentitiesWritten_25_0= RULE_STRING )
// InternalContextMappingDSL.g:4664:13: lv_nanoentitiesWritten_25_0= RULE_STRING
{
lv_nanoentitiesWritten_25_0=(Token)match(input,RULE_STRING,FOLLOW_76);
newLeafNode(lv_nanoentitiesWritten_25_0, grammarAccess.getUseCaseAccess().getNanoentitiesWrittenSTRINGTerminalRuleCall_2_1_5_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getUseCaseRule());
}
addWithLastConsumed(
current,
"nanoentitiesWritten",
lv_nanoentitiesWritten_25_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
default :
break loop138;
}
} while (true);
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1());
}
}
}
break;
default :
break loop139;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getUseCaseAccess().getUnorderedGroup_2_1());
}
this_CLOSE_26=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(this_CLOSE_26, grammarAccess.getUseCaseAccess().getCLOSETerminalRuleCall_2_2());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleUseCase"
// $ANTLR start "entryRuleUserStory"
// InternalContextMappingDSL.g:4703:1: entryRuleUserStory returns [EObject current=null] : iv_ruleUserStory= ruleUserStory EOF ;
public final EObject entryRuleUserStory() throws RecognitionException {
EObject current = null;
EObject iv_ruleUserStory = null;
try {
// InternalContextMappingDSL.g:4703:50: (iv_ruleUserStory= ruleUserStory EOF )
// InternalContextMappingDSL.g:4704:2: iv_ruleUserStory= ruleUserStory EOF
{
newCompositeNode(grammarAccess.getUserStoryRule());
pushFollow(FOLLOW_1);
iv_ruleUserStory=ruleUserStory();
state._fsp--;
current =iv_ruleUserStory;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleUserStory"
// $ANTLR start "ruleUserStory"
// InternalContextMappingDSL.g:4710:1: ruleUserStory returns [EObject current=null] : (otherlv_0= 'UserStory' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) ) ) )* ) ) ) this_CLOSE_22= RULE_CLOSE )? ) ;
public final EObject ruleUserStory() throws RecognitionException {
EObject current = null;
Token otherlv_0=null;
Token lv_name_1_0=null;
Token this_OPEN_2=null;
Token otherlv_4=null;
Token otherlv_5=null;
Token lv_role_6_0=null;
Token otherlv_7=null;
Token otherlv_9=null;
Token lv_benefit_10_0=null;
Token otherlv_11=null;
Token otherlv_12=null;
Token lv_isLatencyCritical_13_0=null;
Token otherlv_14=null;
Token lv_nanoentitiesRead_15_0=null;
Token otherlv_16=null;
Token lv_nanoentitiesRead_17_0=null;
Token otherlv_18=null;
Token lv_nanoentitiesWritten_19_0=null;
Token otherlv_20=null;
Token lv_nanoentitiesWritten_21_0=null;
Token this_CLOSE_22=null;
EObject lv_features_8_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:4716:2: ( (otherlv_0= 'UserStory' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) ) ) )* ) ) ) this_CLOSE_22= RULE_CLOSE )? ) )
// InternalContextMappingDSL.g:4717:2: (otherlv_0= 'UserStory' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) ) ) )* ) ) ) this_CLOSE_22= RULE_CLOSE )? )
{
// InternalContextMappingDSL.g:4717:2: (otherlv_0= 'UserStory' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) ) ) )* ) ) ) this_CLOSE_22= RULE_CLOSE )? )
// InternalContextMappingDSL.g:4718:3: otherlv_0= 'UserStory' ( (lv_name_1_0= RULE_ID ) ) (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) ) ) )* ) ) ) this_CLOSE_22= RULE_CLOSE )?
{
otherlv_0=(Token)match(input,68,FOLLOW_10);
newLeafNode(otherlv_0, grammarAccess.getUserStoryAccess().getUserStoryKeyword_0());
// InternalContextMappingDSL.g:4722:3: ( (lv_name_1_0= RULE_ID ) )
// InternalContextMappingDSL.g:4723:4: (lv_name_1_0= RULE_ID )
{
// InternalContextMappingDSL.g:4723:4: (lv_name_1_0= RULE_ID )
// InternalContextMappingDSL.g:4724:5: lv_name_1_0= RULE_ID
{
lv_name_1_0=(Token)match(input,RULE_ID,FOLLOW_23);
newLeafNode(lv_name_1_0, grammarAccess.getUserStoryAccess().getNameIDTerminalRuleCall_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getUserStoryRule());
}
setWithLastConsumed(
current,
"name",
lv_name_1_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:4740:3: (this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) ) ) )* ) ) ) this_CLOSE_22= RULE_CLOSE )?
int alt149=2;
int LA149_0 = input.LA(1);
if ( (LA149_0==RULE_OPEN) ) {
alt149=1;
}
switch (alt149) {
case 1 :
// InternalContextMappingDSL.g:4741:4: this_OPEN_2= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) ) ) )* ) ) ) this_CLOSE_22= RULE_CLOSE
{
this_OPEN_2=(Token)match(input,RULE_OPEN,FOLLOW_80);
newLeafNode(this_OPEN_2, grammarAccess.getUserStoryAccess().getOPENTerminalRuleCall_2_0());
// InternalContextMappingDSL.g:4745:4: ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:4746:5: ( ( ( ({...}? => ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:4746:5: ( ( ( ({...}? => ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) ) ) )* ) )
// InternalContextMappingDSL.g:4747:6: ( ( ({...}? => ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1());
// InternalContextMappingDSL.g:4750:6: ( ( ({...}? => ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) ) ) )* )
// InternalContextMappingDSL.g:4751:7: ( ({...}? => ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) ) ) )*
{
// InternalContextMappingDSL.g:4751:7: ( ({...}? => ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) ) ) )*
loop148:
do {
int alt148=5;
int LA148_0 = input.LA(1);
if ( LA148_0 >= 69 && LA148_0 <= 70 && getUnorderedGroupHelper().canSelect(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1(), 0) ) {
alt148=1;
}
else if ( LA148_0 == 64 && getUnorderedGroupHelper().canSelect(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1(), 1) ) {
alt148=2;
}
else if ( LA148_0 == 66 && getUnorderedGroupHelper().canSelect(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1(), 2) ) {
alt148=3;
}
else if ( LA148_0 == 67 && getUnorderedGroupHelper().canSelect(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1(), 3) ) {
alt148=4;
}
switch (alt148) {
case 1 :
// InternalContextMappingDSL.g:4752:5: ({...}? => ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:4752:5: ({...}? => ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:4753:6: {...}? => ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1(), 0) ) {
throw new FailedPredicateException(input, "ruleUserStory", "getUnorderedGroupHelper().canSelect(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1(), 0)");
}
// InternalContextMappingDSL.g:4753:109: ( ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:4754:7: ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1(), 0);
// InternalContextMappingDSL.g:4757:10: ({...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:4757:11: {...}? => ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleUserStory", "true");
}
// InternalContextMappingDSL.g:4757:20: ( (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:4757:21: (otherlv_4= 'As a' | otherlv_5= 'As an' ) ( (lv_role_6_0= RULE_STRING ) ) (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+ otherlv_9= 'so that' ( (lv_benefit_10_0= RULE_STRING ) )
{
// InternalContextMappingDSL.g:4757:21: (otherlv_4= 'As a' | otherlv_5= 'As an' )
int alt141=2;
int LA141_0 = input.LA(1);
if ( (LA141_0==69) ) {
alt141=1;
}
else if ( (LA141_0==70) ) {
alt141=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 141, 0, input);
throw nvae;
}
switch (alt141) {
case 1 :
// InternalContextMappingDSL.g:4758:11: otherlv_4= 'As a'
{
otherlv_4=(Token)match(input,69,FOLLOW_4);
newLeafNode(otherlv_4, grammarAccess.getUserStoryAccess().getAsAKeyword_2_1_0_0_0());
}
break;
case 2 :
// InternalContextMappingDSL.g:4763:11: otherlv_5= 'As an'
{
otherlv_5=(Token)match(input,70,FOLLOW_4);
newLeafNode(otherlv_5, grammarAccess.getUserStoryAccess().getAsAnKeyword_2_1_0_0_1());
}
break;
}
// InternalContextMappingDSL.g:4768:10: ( (lv_role_6_0= RULE_STRING ) )
// InternalContextMappingDSL.g:4769:11: (lv_role_6_0= RULE_STRING )
{
// InternalContextMappingDSL.g:4769:11: (lv_role_6_0= RULE_STRING )
// InternalContextMappingDSL.g:4770:12: lv_role_6_0= RULE_STRING
{
lv_role_6_0=(Token)match(input,RULE_STRING,FOLLOW_81);
newLeafNode(lv_role_6_0, grammarAccess.getUserStoryAccess().getRoleSTRINGTerminalRuleCall_2_1_0_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getUserStoryRule());
}
setWithLastConsumed(
current,
"role",
lv_role_6_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
// InternalContextMappingDSL.g:4786:10: (otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) ) )+
int cnt142=0;
loop142:
do {
int alt142=2;
int LA142_0 = input.LA(1);
if ( (LA142_0==71) ) {
alt142=1;
}
switch (alt142) {
case 1 :
// InternalContextMappingDSL.g:4787:11: otherlv_7= 'I want to' ( (lv_features_8_0= ruleFeature ) )
{
otherlv_7=(Token)match(input,71,FOLLOW_75);
newLeafNode(otherlv_7, grammarAccess.getUserStoryAccess().getIWantToKeyword_2_1_0_2_0());
// InternalContextMappingDSL.g:4791:11: ( (lv_features_8_0= ruleFeature ) )
// InternalContextMappingDSL.g:4792:12: (lv_features_8_0= ruleFeature )
{
// InternalContextMappingDSL.g:4792:12: (lv_features_8_0= ruleFeature )
// InternalContextMappingDSL.g:4793:13: lv_features_8_0= ruleFeature
{
newCompositeNode(grammarAccess.getUserStoryAccess().getFeaturesFeatureParserRuleCall_2_1_0_2_1_0());
pushFollow(FOLLOW_82);
lv_features_8_0=ruleFeature();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getUserStoryRule());
}
add(
current,
"features",
lv_features_8_0,
"org.contextmapper.dsl.ContextMappingDSL.Feature");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
if ( cnt142 >= 1 ) break loop142;
EarlyExitException eee =
new EarlyExitException(142, input);
throw eee;
}
cnt142++;
} while (true);
otherlv_9=(Token)match(input,72,FOLLOW_4);
newLeafNode(otherlv_9, grammarAccess.getUserStoryAccess().getSoThatKeyword_2_1_0_3());
// InternalContextMappingDSL.g:4815:10: ( (lv_benefit_10_0= RULE_STRING ) )
// InternalContextMappingDSL.g:4816:11: (lv_benefit_10_0= RULE_STRING )
{
// InternalContextMappingDSL.g:4816:11: (lv_benefit_10_0= RULE_STRING )
// InternalContextMappingDSL.g:4817:12: lv_benefit_10_0= RULE_STRING
{
lv_benefit_10_0=(Token)match(input,RULE_STRING,FOLLOW_80);
newLeafNode(lv_benefit_10_0, grammarAccess.getUserStoryAccess().getBenefitSTRINGTerminalRuleCall_2_1_0_4_0());
if (current==null) {
current = createModelElement(grammarAccess.getUserStoryRule());
}
setWithLastConsumed(
current,
"benefit",
lv_benefit_10_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:4839:5: ({...}? => ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) ) )
{
// InternalContextMappingDSL.g:4839:5: ({...}? => ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) ) )
// InternalContextMappingDSL.g:4840:6: {...}? => ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1(), 1) ) {
throw new FailedPredicateException(input, "ruleUserStory", "getUnorderedGroupHelper().canSelect(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1(), 1)");
}
// InternalContextMappingDSL.g:4840:109: ( ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) ) )
// InternalContextMappingDSL.g:4841:7: ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1(), 1);
// InternalContextMappingDSL.g:4844:10: ({...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) ) )
// InternalContextMappingDSL.g:4844:11: {...}? => (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleUserStory", "true");
}
// InternalContextMappingDSL.g:4844:20: (otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) ) )
// InternalContextMappingDSL.g:4844:21: otherlv_11= 'isLatencyCritical' (otherlv_12= '=' )? ( (lv_isLatencyCritical_13_0= 'true' ) )
{
otherlv_11=(Token)match(input,64,FOLLOW_77);
newLeafNode(otherlv_11, grammarAccess.getUserStoryAccess().getIsLatencyCriticalKeyword_2_1_1_0());
// InternalContextMappingDSL.g:4848:10: (otherlv_12= '=' )?
int alt143=2;
int LA143_0 = input.LA(1);
if ( (LA143_0==21) ) {
alt143=1;
}
switch (alt143) {
case 1 :
// InternalContextMappingDSL.g:4849:11: otherlv_12= '='
{
otherlv_12=(Token)match(input,21,FOLLOW_78);
newLeafNode(otherlv_12, grammarAccess.getUserStoryAccess().getEqualsSignKeyword_2_1_1_1());
}
break;
}
// InternalContextMappingDSL.g:4854:10: ( (lv_isLatencyCritical_13_0= 'true' ) )
// InternalContextMappingDSL.g:4855:11: (lv_isLatencyCritical_13_0= 'true' )
{
// InternalContextMappingDSL.g:4855:11: (lv_isLatencyCritical_13_0= 'true' )
// InternalContextMappingDSL.g:4856:12: lv_isLatencyCritical_13_0= 'true'
{
lv_isLatencyCritical_13_0=(Token)match(input,65,FOLLOW_80);
newLeafNode(lv_isLatencyCritical_13_0, grammarAccess.getUserStoryAccess().getIsLatencyCriticalTrueKeyword_2_1_1_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getUserStoryRule());
}
setWithLastConsumed(current, "isLatencyCritical", true, "true");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:4874:5: ({...}? => ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) ) )
{
// InternalContextMappingDSL.g:4874:5: ({...}? => ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) ) )
// InternalContextMappingDSL.g:4875:6: {...}? => ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1(), 2) ) {
throw new FailedPredicateException(input, "ruleUserStory", "getUnorderedGroupHelper().canSelect(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1(), 2)");
}
// InternalContextMappingDSL.g:4875:109: ( ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) ) )
// InternalContextMappingDSL.g:4876:7: ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) )
{
getUnorderedGroupHelper().select(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1(), 2);
// InternalContextMappingDSL.g:4879:10: ({...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* ) )
// InternalContextMappingDSL.g:4879:11: {...}? => ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleUserStory", "true");
}
// InternalContextMappingDSL.g:4879:20: ( (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )* )
// InternalContextMappingDSL.g:4879:21: (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* ) (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )*
{
// InternalContextMappingDSL.g:4879:21: (otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )* )
// InternalContextMappingDSL.g:4880:11: otherlv_14= 'reads' ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )*
{
otherlv_14=(Token)match(input,66,FOLLOW_83);
newLeafNode(otherlv_14, grammarAccess.getUserStoryAccess().getReadsKeyword_2_1_2_0_0());
// InternalContextMappingDSL.g:4884:11: ( (lv_nanoentitiesRead_15_0= RULE_STRING ) )*
loop144:
do {
int alt144=2;
int LA144_0 = input.LA(1);
if ( (LA144_0==RULE_STRING) ) {
alt144=1;
}
switch (alt144) {
case 1 :
// InternalContextMappingDSL.g:4885:12: (lv_nanoentitiesRead_15_0= RULE_STRING )
{
// InternalContextMappingDSL.g:4885:12: (lv_nanoentitiesRead_15_0= RULE_STRING )
// InternalContextMappingDSL.g:4886:13: lv_nanoentitiesRead_15_0= RULE_STRING
{
lv_nanoentitiesRead_15_0=(Token)match(input,RULE_STRING,FOLLOW_83);
newLeafNode(lv_nanoentitiesRead_15_0, grammarAccess.getUserStoryAccess().getNanoentitiesReadSTRINGTerminalRuleCall_2_1_2_0_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getUserStoryRule());
}
addWithLastConsumed(
current,
"nanoentitiesRead",
lv_nanoentitiesRead_15_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
default :
break loop144;
}
} while (true);
}
// InternalContextMappingDSL.g:4903:10: (otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) ) )*
loop145:
do {
int alt145=2;
int LA145_0 = input.LA(1);
if ( (LA145_0==24) ) {
alt145=1;
}
switch (alt145) {
case 1 :
// InternalContextMappingDSL.g:4904:11: otherlv_16= ',' ( (lv_nanoentitiesRead_17_0= RULE_STRING ) )
{
otherlv_16=(Token)match(input,24,FOLLOW_4);
newLeafNode(otherlv_16, grammarAccess.getUserStoryAccess().getCommaKeyword_2_1_2_1_0());
// InternalContextMappingDSL.g:4908:11: ( (lv_nanoentitiesRead_17_0= RULE_STRING ) )
// InternalContextMappingDSL.g:4909:12: (lv_nanoentitiesRead_17_0= RULE_STRING )
{
// InternalContextMappingDSL.g:4909:12: (lv_nanoentitiesRead_17_0= RULE_STRING )
// InternalContextMappingDSL.g:4910:13: lv_nanoentitiesRead_17_0= RULE_STRING
{
lv_nanoentitiesRead_17_0=(Token)match(input,RULE_STRING,FOLLOW_84);
newLeafNode(lv_nanoentitiesRead_17_0, grammarAccess.getUserStoryAccess().getNanoentitiesReadSTRINGTerminalRuleCall_2_1_2_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getUserStoryRule());
}
addWithLastConsumed(
current,
"nanoentitiesRead",
lv_nanoentitiesRead_17_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
default :
break loop145;
}
} while (true);
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1());
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:4933:5: ({...}? => ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) ) )
{
// InternalContextMappingDSL.g:4933:5: ({...}? => ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) ) )
// InternalContextMappingDSL.g:4934:6: {...}? => ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1(), 3) ) {
throw new FailedPredicateException(input, "ruleUserStory", "getUnorderedGroupHelper().canSelect(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1(), 3)");
}
// InternalContextMappingDSL.g:4934:109: ( ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) ) )
// InternalContextMappingDSL.g:4935:7: ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) )
{
getUnorderedGroupHelper().select(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1(), 3);
// InternalContextMappingDSL.g:4938:10: ({...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* ) )
// InternalContextMappingDSL.g:4938:11: {...}? => ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleUserStory", "true");
}
// InternalContextMappingDSL.g:4938:20: ( (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )* )
// InternalContextMappingDSL.g:4938:21: (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* ) (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )*
{
// InternalContextMappingDSL.g:4938:21: (otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )* )
// InternalContextMappingDSL.g:4939:11: otherlv_18= 'writes' ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )*
{
otherlv_18=(Token)match(input,67,FOLLOW_83);
newLeafNode(otherlv_18, grammarAccess.getUserStoryAccess().getWritesKeyword_2_1_3_0_0());
// InternalContextMappingDSL.g:4943:11: ( (lv_nanoentitiesWritten_19_0= RULE_STRING ) )*
loop146:
do {
int alt146=2;
int LA146_0 = input.LA(1);
if ( (LA146_0==RULE_STRING) ) {
alt146=1;
}
switch (alt146) {
case 1 :
// InternalContextMappingDSL.g:4944:12: (lv_nanoentitiesWritten_19_0= RULE_STRING )
{
// InternalContextMappingDSL.g:4944:12: (lv_nanoentitiesWritten_19_0= RULE_STRING )
// InternalContextMappingDSL.g:4945:13: lv_nanoentitiesWritten_19_0= RULE_STRING
{
lv_nanoentitiesWritten_19_0=(Token)match(input,RULE_STRING,FOLLOW_83);
newLeafNode(lv_nanoentitiesWritten_19_0, grammarAccess.getUserStoryAccess().getNanoentitiesWrittenSTRINGTerminalRuleCall_2_1_3_0_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getUserStoryRule());
}
addWithLastConsumed(
current,
"nanoentitiesWritten",
lv_nanoentitiesWritten_19_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
default :
break loop146;
}
} while (true);
}
// InternalContextMappingDSL.g:4962:10: (otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) ) )*
loop147:
do {
int alt147=2;
int LA147_0 = input.LA(1);
if ( (LA147_0==24) ) {
alt147=1;
}
switch (alt147) {
case 1 :
// InternalContextMappingDSL.g:4963:11: otherlv_20= ',' ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) )
{
otherlv_20=(Token)match(input,24,FOLLOW_4);
newLeafNode(otherlv_20, grammarAccess.getUserStoryAccess().getCommaKeyword_2_1_3_1_0());
// InternalContextMappingDSL.g:4967:11: ( (lv_nanoentitiesWritten_21_0= RULE_STRING ) )
// InternalContextMappingDSL.g:4968:12: (lv_nanoentitiesWritten_21_0= RULE_STRING )
{
// InternalContextMappingDSL.g:4968:12: (lv_nanoentitiesWritten_21_0= RULE_STRING )
// InternalContextMappingDSL.g:4969:13: lv_nanoentitiesWritten_21_0= RULE_STRING
{
lv_nanoentitiesWritten_21_0=(Token)match(input,RULE_STRING,FOLLOW_84);
newLeafNode(lv_nanoentitiesWritten_21_0, grammarAccess.getUserStoryAccess().getNanoentitiesWrittenSTRINGTerminalRuleCall_2_1_3_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getUserStoryRule());
}
addWithLastConsumed(
current,
"nanoentitiesWritten",
lv_nanoentitiesWritten_21_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
default :
break loop147;
}
} while (true);
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1());
}
}
}
break;
default :
break loop148;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getUserStoryAccess().getUnorderedGroup_2_1());
}
this_CLOSE_22=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(this_CLOSE_22, grammarAccess.getUserStoryAccess().getCLOSETerminalRuleCall_2_2());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleUserStory"
// $ANTLR start "entryRuleFeature"
// InternalContextMappingDSL.g:5008:1: entryRuleFeature returns [EObject current=null] : iv_ruleFeature= ruleFeature EOF ;
public final EObject entryRuleFeature() throws RecognitionException {
EObject current = null;
EObject iv_ruleFeature = null;
try {
// InternalContextMappingDSL.g:5008:48: (iv_ruleFeature= ruleFeature EOF )
// InternalContextMappingDSL.g:5009:2: iv_ruleFeature= ruleFeature EOF
{
newCompositeNode(grammarAccess.getFeatureRule());
pushFollow(FOLLOW_1);
iv_ruleFeature=ruleFeature();
state._fsp--;
current =iv_ruleFeature;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleFeature"
// $ANTLR start "ruleFeature"
// InternalContextMappingDSL.g:5015:1: ruleFeature returns [EObject current=null] : ( ( ( (lv_verb_0_1= ruleUserActivityDefaultVerb | lv_verb_0_2= RULE_STRING ) ) ) (otherlv_1= 'a' | otherlv_2= 'an' )? ( (lv_entity_3_0= RULE_STRING ) ) ) ;
public final EObject ruleFeature() throws RecognitionException {
EObject current = null;
Token lv_verb_0_2=null;
Token otherlv_1=null;
Token otherlv_2=null;
Token lv_entity_3_0=null;
AntlrDatatypeRuleToken lv_verb_0_1 = null;
enterRule();
try {
// InternalContextMappingDSL.g:5021:2: ( ( ( ( (lv_verb_0_1= ruleUserActivityDefaultVerb | lv_verb_0_2= RULE_STRING ) ) ) (otherlv_1= 'a' | otherlv_2= 'an' )? ( (lv_entity_3_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:5022:2: ( ( ( (lv_verb_0_1= ruleUserActivityDefaultVerb | lv_verb_0_2= RULE_STRING ) ) ) (otherlv_1= 'a' | otherlv_2= 'an' )? ( (lv_entity_3_0= RULE_STRING ) ) )
{
// InternalContextMappingDSL.g:5022:2: ( ( ( (lv_verb_0_1= ruleUserActivityDefaultVerb | lv_verb_0_2= RULE_STRING ) ) ) (otherlv_1= 'a' | otherlv_2= 'an' )? ( (lv_entity_3_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:5023:3: ( ( (lv_verb_0_1= ruleUserActivityDefaultVerb | lv_verb_0_2= RULE_STRING ) ) ) (otherlv_1= 'a' | otherlv_2= 'an' )? ( (lv_entity_3_0= RULE_STRING ) )
{
// InternalContextMappingDSL.g:5023:3: ( ( (lv_verb_0_1= ruleUserActivityDefaultVerb | lv_verb_0_2= RULE_STRING ) ) )
// InternalContextMappingDSL.g:5024:4: ( (lv_verb_0_1= ruleUserActivityDefaultVerb | lv_verb_0_2= RULE_STRING ) )
{
// InternalContextMappingDSL.g:5024:4: ( (lv_verb_0_1= ruleUserActivityDefaultVerb | lv_verb_0_2= RULE_STRING ) )
// InternalContextMappingDSL.g:5025:5: (lv_verb_0_1= ruleUserActivityDefaultVerb | lv_verb_0_2= RULE_STRING )
{
// InternalContextMappingDSL.g:5025:5: (lv_verb_0_1= ruleUserActivityDefaultVerb | lv_verb_0_2= RULE_STRING )
int alt150=2;
int LA150_0 = input.LA(1);
if ( ((LA150_0>=75 && LA150_0<=78)) ) {
alt150=1;
}
else if ( (LA150_0==RULE_STRING) ) {
alt150=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 150, 0, input);
throw nvae;
}
switch (alt150) {
case 1 :
// InternalContextMappingDSL.g:5026:6: lv_verb_0_1= ruleUserActivityDefaultVerb
{
newCompositeNode(grammarAccess.getFeatureAccess().getVerbUserActivityDefaultVerbParserRuleCall_0_0_0());
pushFollow(FOLLOW_85);
lv_verb_0_1=ruleUserActivityDefaultVerb();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getFeatureRule());
}
set(
current,
"verb",
lv_verb_0_1,
"org.contextmapper.dsl.ContextMappingDSL.UserActivityDefaultVerb");
afterParserOrEnumRuleCall();
}
break;
case 2 :
// InternalContextMappingDSL.g:5042:6: lv_verb_0_2= RULE_STRING
{
lv_verb_0_2=(Token)match(input,RULE_STRING,FOLLOW_85);
newLeafNode(lv_verb_0_2, grammarAccess.getFeatureAccess().getVerbSTRINGTerminalRuleCall_0_0_1());
if (current==null) {
current = createModelElement(grammarAccess.getFeatureRule());
}
setWithLastConsumed(
current,
"verb",
lv_verb_0_2,
"org.eclipse.xtext.common.Terminals.STRING");
}
break;
}
}
}
// InternalContextMappingDSL.g:5059:3: (otherlv_1= 'a' | otherlv_2= 'an' )?
int alt151=3;
int LA151_0 = input.LA(1);
if ( (LA151_0==73) ) {
alt151=1;
}
else if ( (LA151_0==74) ) {
alt151=2;
}
switch (alt151) {
case 1 :
// InternalContextMappingDSL.g:5060:4: otherlv_1= 'a'
{
otherlv_1=(Token)match(input,73,FOLLOW_4);
newLeafNode(otherlv_1, grammarAccess.getFeatureAccess().getAKeyword_1_0());
}
break;
case 2 :
// InternalContextMappingDSL.g:5065:4: otherlv_2= 'an'
{
otherlv_2=(Token)match(input,74,FOLLOW_4);
newLeafNode(otherlv_2, grammarAccess.getFeatureAccess().getAnKeyword_1_1());
}
break;
}
// InternalContextMappingDSL.g:5070:3: ( (lv_entity_3_0= RULE_STRING ) )
// InternalContextMappingDSL.g:5071:4: (lv_entity_3_0= RULE_STRING )
{
// InternalContextMappingDSL.g:5071:4: (lv_entity_3_0= RULE_STRING )
// InternalContextMappingDSL.g:5072:5: lv_entity_3_0= RULE_STRING
{
lv_entity_3_0=(Token)match(input,RULE_STRING,FOLLOW_2);
newLeafNode(lv_entity_3_0, grammarAccess.getFeatureAccess().getEntitySTRINGTerminalRuleCall_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getFeatureRule());
}
setWithLastConsumed(
current,
"entity",
lv_entity_3_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleFeature"
// $ANTLR start "entryRuleUserActivityDefaultVerb"
// InternalContextMappingDSL.g:5092:1: entryRuleUserActivityDefaultVerb returns [String current=null] : iv_ruleUserActivityDefaultVerb= ruleUserActivityDefaultVerb EOF ;
public final String entryRuleUserActivityDefaultVerb() throws RecognitionException {
String current = null;
AntlrDatatypeRuleToken iv_ruleUserActivityDefaultVerb = null;
try {
// InternalContextMappingDSL.g:5092:63: (iv_ruleUserActivityDefaultVerb= ruleUserActivityDefaultVerb EOF )
// InternalContextMappingDSL.g:5093:2: iv_ruleUserActivityDefaultVerb= ruleUserActivityDefaultVerb EOF
{
newCompositeNode(grammarAccess.getUserActivityDefaultVerbRule());
pushFollow(FOLLOW_1);
iv_ruleUserActivityDefaultVerb=ruleUserActivityDefaultVerb();
state._fsp--;
current =iv_ruleUserActivityDefaultVerb.getText();
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleUserActivityDefaultVerb"
// $ANTLR start "ruleUserActivityDefaultVerb"
// InternalContextMappingDSL.g:5099:1: ruleUserActivityDefaultVerb returns [AntlrDatatypeRuleToken current=new AntlrDatatypeRuleToken()] : (kw= 'create' | kw= 'read' | kw= 'update' | kw= 'delete' ) ;
public final AntlrDatatypeRuleToken ruleUserActivityDefaultVerb() throws RecognitionException {
AntlrDatatypeRuleToken current = new AntlrDatatypeRuleToken();
Token kw=null;
enterRule();
try {
// InternalContextMappingDSL.g:5105:2: ( (kw= 'create' | kw= 'read' | kw= 'update' | kw= 'delete' ) )
// InternalContextMappingDSL.g:5106:2: (kw= 'create' | kw= 'read' | kw= 'update' | kw= 'delete' )
{
// InternalContextMappingDSL.g:5106:2: (kw= 'create' | kw= 'read' | kw= 'update' | kw= 'delete' )
int alt152=4;
switch ( input.LA(1) ) {
case 75:
{
alt152=1;
}
break;
case 76:
{
alt152=2;
}
break;
case 77:
{
alt152=3;
}
break;
case 78:
{
alt152=4;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 152, 0, input);
throw nvae;
}
switch (alt152) {
case 1 :
// InternalContextMappingDSL.g:5107:3: kw= 'create'
{
kw=(Token)match(input,75,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getUserActivityDefaultVerbAccess().getCreateKeyword_0());
}
break;
case 2 :
// InternalContextMappingDSL.g:5113:3: kw= 'read'
{
kw=(Token)match(input,76,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getUserActivityDefaultVerbAccess().getReadKeyword_1());
}
break;
case 3 :
// InternalContextMappingDSL.g:5119:3: kw= 'update'
{
kw=(Token)match(input,77,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getUserActivityDefaultVerbAccess().getUpdateKeyword_2());
}
break;
case 4 :
// InternalContextMappingDSL.g:5125:3: kw= 'delete'
{
kw=(Token)match(input,78,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getUserActivityDefaultVerbAccess().getDeleteKeyword_3());
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleUserActivityDefaultVerb"
// $ANTLR start "entryRuleSculptorModule"
// InternalContextMappingDSL.g:5134:1: entryRuleSculptorModule returns [EObject current=null] : iv_ruleSculptorModule= ruleSculptorModule EOF ;
public final EObject entryRuleSculptorModule() throws RecognitionException {
EObject current = null;
EObject iv_ruleSculptorModule = null;
try {
// InternalContextMappingDSL.g:5134:55: (iv_ruleSculptorModule= ruleSculptorModule EOF )
// InternalContextMappingDSL.g:5135:2: iv_ruleSculptorModule= ruleSculptorModule EOF
{
newCompositeNode(grammarAccess.getSculptorModuleRule());
pushFollow(FOLLOW_1);
iv_ruleSculptorModule=ruleSculptorModule();
state._fsp--;
current =iv_ruleSculptorModule;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleSculptorModule"
// $ANTLR start "ruleSculptorModule"
// InternalContextMappingDSL.g:5141:1: ruleSculptorModule returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Module' ( (lv_name_2_0= RULE_ID ) ) (this_OPEN_3= RULE_OPEN ( (lv_external_4_0= 'external' ) )? (otherlv_5= 'basePackage' otherlv_6= '=' ( (lv_basePackage_7_0= ruleJavaIdentifier ) ) )? (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) )? ( ( (lv_services_11_0= ruleService ) ) | ( (lv_resources_12_0= ruleResource ) ) | ( (lv_consumers_13_0= ruleConsumer ) ) | ( (lv_domainObjects_14_0= ruleSimpleDomainObject ) ) | ( (lv_aggregates_15_0= ruleAggregate ) ) )* this_CLOSE_16= RULE_CLOSE )? ) ;
public final EObject ruleSculptorModule() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token otherlv_1=null;
Token lv_name_2_0=null;
Token this_OPEN_3=null;
Token lv_external_4_0=null;
Token otherlv_5=null;
Token otherlv_6=null;
Token otherlv_8=null;
Token otherlv_9=null;
Token lv_hint_10_0=null;
Token this_CLOSE_16=null;
AntlrDatatypeRuleToken lv_basePackage_7_0 = null;
EObject lv_services_11_0 = null;
EObject lv_resources_12_0 = null;
EObject lv_consumers_13_0 = null;
EObject lv_domainObjects_14_0 = null;
EObject lv_aggregates_15_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:5147:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Module' ( (lv_name_2_0= RULE_ID ) ) (this_OPEN_3= RULE_OPEN ( (lv_external_4_0= 'external' ) )? (otherlv_5= 'basePackage' otherlv_6= '=' ( (lv_basePackage_7_0= ruleJavaIdentifier ) ) )? (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) )? ( ( (lv_services_11_0= ruleService ) ) | ( (lv_resources_12_0= ruleResource ) ) | ( (lv_consumers_13_0= ruleConsumer ) ) | ( (lv_domainObjects_14_0= ruleSimpleDomainObject ) ) | ( (lv_aggregates_15_0= ruleAggregate ) ) )* this_CLOSE_16= RULE_CLOSE )? ) )
// InternalContextMappingDSL.g:5148:2: ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Module' ( (lv_name_2_0= RULE_ID ) ) (this_OPEN_3= RULE_OPEN ( (lv_external_4_0= 'external' ) )? (otherlv_5= 'basePackage' otherlv_6= '=' ( (lv_basePackage_7_0= ruleJavaIdentifier ) ) )? (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) )? ( ( (lv_services_11_0= ruleService ) ) | ( (lv_resources_12_0= ruleResource ) ) | ( (lv_consumers_13_0= ruleConsumer ) ) | ( (lv_domainObjects_14_0= ruleSimpleDomainObject ) ) | ( (lv_aggregates_15_0= ruleAggregate ) ) )* this_CLOSE_16= RULE_CLOSE )? )
{
// InternalContextMappingDSL.g:5148:2: ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Module' ( (lv_name_2_0= RULE_ID ) ) (this_OPEN_3= RULE_OPEN ( (lv_external_4_0= 'external' ) )? (otherlv_5= 'basePackage' otherlv_6= '=' ( (lv_basePackage_7_0= ruleJavaIdentifier ) ) )? (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) )? ( ( (lv_services_11_0= ruleService ) ) | ( (lv_resources_12_0= ruleResource ) ) | ( (lv_consumers_13_0= ruleConsumer ) ) | ( (lv_domainObjects_14_0= ruleSimpleDomainObject ) ) | ( (lv_aggregates_15_0= ruleAggregate ) ) )* this_CLOSE_16= RULE_CLOSE )? )
// InternalContextMappingDSL.g:5149:3: ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Module' ( (lv_name_2_0= RULE_ID ) ) (this_OPEN_3= RULE_OPEN ( (lv_external_4_0= 'external' ) )? (otherlv_5= 'basePackage' otherlv_6= '=' ( (lv_basePackage_7_0= ruleJavaIdentifier ) ) )? (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) )? ( ( (lv_services_11_0= ruleService ) ) | ( (lv_resources_12_0= ruleResource ) ) | ( (lv_consumers_13_0= ruleConsumer ) ) | ( (lv_domainObjects_14_0= ruleSimpleDomainObject ) ) | ( (lv_aggregates_15_0= ruleAggregate ) ) )* this_CLOSE_16= RULE_CLOSE )?
{
// InternalContextMappingDSL.g:5149:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt153=2;
int LA153_0 = input.LA(1);
if ( (LA153_0==RULE_STRING) ) {
alt153=1;
}
switch (alt153) {
case 1 :
// InternalContextMappingDSL.g:5150:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:5150:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:5151:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_86);
newLeafNode(lv_doc_0_0, grammarAccess.getSculptorModuleAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getSculptorModuleRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
otherlv_1=(Token)match(input,79,FOLLOW_10);
newLeafNode(otherlv_1, grammarAccess.getSculptorModuleAccess().getModuleKeyword_1());
// InternalContextMappingDSL.g:5171:3: ( (lv_name_2_0= RULE_ID ) )
// InternalContextMappingDSL.g:5172:4: (lv_name_2_0= RULE_ID )
{
// InternalContextMappingDSL.g:5172:4: (lv_name_2_0= RULE_ID )
// InternalContextMappingDSL.g:5173:5: lv_name_2_0= RULE_ID
{
lv_name_2_0=(Token)match(input,RULE_ID,FOLLOW_23);
newLeafNode(lv_name_2_0, grammarAccess.getSculptorModuleAccess().getNameIDTerminalRuleCall_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getSculptorModuleRule());
}
setWithLastConsumed(
current,
"name",
lv_name_2_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:5189:3: (this_OPEN_3= RULE_OPEN ( (lv_external_4_0= 'external' ) )? (otherlv_5= 'basePackage' otherlv_6= '=' ( (lv_basePackage_7_0= ruleJavaIdentifier ) ) )? (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) )? ( ( (lv_services_11_0= ruleService ) ) | ( (lv_resources_12_0= ruleResource ) ) | ( (lv_consumers_13_0= ruleConsumer ) ) | ( (lv_domainObjects_14_0= ruleSimpleDomainObject ) ) | ( (lv_aggregates_15_0= ruleAggregate ) ) )* this_CLOSE_16= RULE_CLOSE )?
int alt158=2;
int LA158_0 = input.LA(1);
if ( (LA158_0==RULE_OPEN) ) {
alt158=1;
}
switch (alt158) {
case 1 :
// InternalContextMappingDSL.g:5190:4: this_OPEN_3= RULE_OPEN ( (lv_external_4_0= 'external' ) )? (otherlv_5= 'basePackage' otherlv_6= '=' ( (lv_basePackage_7_0= ruleJavaIdentifier ) ) )? (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) )? ( ( (lv_services_11_0= ruleService ) ) | ( (lv_resources_12_0= ruleResource ) ) | ( (lv_consumers_13_0= ruleConsumer ) ) | ( (lv_domainObjects_14_0= ruleSimpleDomainObject ) ) | ( (lv_aggregates_15_0= ruleAggregate ) ) )* this_CLOSE_16= RULE_CLOSE
{
this_OPEN_3=(Token)match(input,RULE_OPEN,FOLLOW_87);
newLeafNode(this_OPEN_3, grammarAccess.getSculptorModuleAccess().getOPENTerminalRuleCall_3_0());
// InternalContextMappingDSL.g:5194:4: ( (lv_external_4_0= 'external' ) )?
int alt154=2;
int LA154_0 = input.LA(1);
if ( (LA154_0==80) ) {
alt154=1;
}
switch (alt154) {
case 1 :
// InternalContextMappingDSL.g:5195:5: (lv_external_4_0= 'external' )
{
// InternalContextMappingDSL.g:5195:5: (lv_external_4_0= 'external' )
// InternalContextMappingDSL.g:5196:6: lv_external_4_0= 'external'
{
lv_external_4_0=(Token)match(input,80,FOLLOW_88);
newLeafNode(lv_external_4_0, grammarAccess.getSculptorModuleAccess().getExternalExternalKeyword_3_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getSculptorModuleRule());
}
setWithLastConsumed(current, "external", true, "external");
}
}
break;
}
// InternalContextMappingDSL.g:5208:4: (otherlv_5= 'basePackage' otherlv_6= '=' ( (lv_basePackage_7_0= ruleJavaIdentifier ) ) )?
int alt155=2;
int LA155_0 = input.LA(1);
if ( (LA155_0==81) ) {
alt155=1;
}
switch (alt155) {
case 1 :
// InternalContextMappingDSL.g:5209:5: otherlv_5= 'basePackage' otherlv_6= '=' ( (lv_basePackage_7_0= ruleJavaIdentifier ) )
{
otherlv_5=(Token)match(input,81,FOLLOW_89);
newLeafNode(otherlv_5, grammarAccess.getSculptorModuleAccess().getBasePackageKeyword_3_2_0());
otherlv_6=(Token)match(input,21,FOLLOW_10);
newLeafNode(otherlv_6, grammarAccess.getSculptorModuleAccess().getEqualsSignKeyword_3_2_1());
// InternalContextMappingDSL.g:5217:5: ( (lv_basePackage_7_0= ruleJavaIdentifier ) )
// InternalContextMappingDSL.g:5218:6: (lv_basePackage_7_0= ruleJavaIdentifier )
{
// InternalContextMappingDSL.g:5218:6: (lv_basePackage_7_0= ruleJavaIdentifier )
// InternalContextMappingDSL.g:5219:7: lv_basePackage_7_0= ruleJavaIdentifier
{
newCompositeNode(grammarAccess.getSculptorModuleAccess().getBasePackageJavaIdentifierParserRuleCall_3_2_2_0());
pushFollow(FOLLOW_90);
lv_basePackage_7_0=ruleJavaIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getSculptorModuleRule());
}
set(
current,
"basePackage",
lv_basePackage_7_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.JavaIdentifier");
afterParserOrEnumRuleCall();
}
}
}
break;
}
// InternalContextMappingDSL.g:5237:4: (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) )?
int alt156=2;
int LA156_0 = input.LA(1);
if ( (LA156_0==82) ) {
alt156=1;
}
switch (alt156) {
case 1 :
// InternalContextMappingDSL.g:5238:5: otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) )
{
otherlv_8=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_8, grammarAccess.getSculptorModuleAccess().getHintKeyword_3_3_0());
otherlv_9=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_9, grammarAccess.getSculptorModuleAccess().getEqualsSignKeyword_3_3_1());
// InternalContextMappingDSL.g:5246:5: ( (lv_hint_10_0= RULE_STRING ) )
// InternalContextMappingDSL.g:5247:6: (lv_hint_10_0= RULE_STRING )
{
// InternalContextMappingDSL.g:5247:6: (lv_hint_10_0= RULE_STRING )
// InternalContextMappingDSL.g:5248:7: lv_hint_10_0= RULE_STRING
{
lv_hint_10_0=(Token)match(input,RULE_STRING,FOLLOW_91);
newLeafNode(lv_hint_10_0, grammarAccess.getSculptorModuleAccess().getHintSTRINGTerminalRuleCall_3_3_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getSculptorModuleRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_10_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
// InternalContextMappingDSL.g:5265:4: ( ( (lv_services_11_0= ruleService ) ) | ( (lv_resources_12_0= ruleResource ) ) | ( (lv_consumers_13_0= ruleConsumer ) ) | ( (lv_domainObjects_14_0= ruleSimpleDomainObject ) ) | ( (lv_aggregates_15_0= ruleAggregate ) ) )*
loop157:
do {
int alt157=6;
alt157 = dfa157.predict(input);
switch (alt157) {
case 1 :
// InternalContextMappingDSL.g:5266:5: ( (lv_services_11_0= ruleService ) )
{
// InternalContextMappingDSL.g:5266:5: ( (lv_services_11_0= ruleService ) )
// InternalContextMappingDSL.g:5267:6: (lv_services_11_0= ruleService )
{
// InternalContextMappingDSL.g:5267:6: (lv_services_11_0= ruleService )
// InternalContextMappingDSL.g:5268:7: lv_services_11_0= ruleService
{
newCompositeNode(grammarAccess.getSculptorModuleAccess().getServicesServiceParserRuleCall_3_4_0_0());
pushFollow(FOLLOW_91);
lv_services_11_0=ruleService();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getSculptorModuleRule());
}
add(
current,
"services",
lv_services_11_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Service");
afterParserOrEnumRuleCall();
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:5286:5: ( (lv_resources_12_0= ruleResource ) )
{
// InternalContextMappingDSL.g:5286:5: ( (lv_resources_12_0= ruleResource ) )
// InternalContextMappingDSL.g:5287:6: (lv_resources_12_0= ruleResource )
{
// InternalContextMappingDSL.g:5287:6: (lv_resources_12_0= ruleResource )
// InternalContextMappingDSL.g:5288:7: lv_resources_12_0= ruleResource
{
newCompositeNode(grammarAccess.getSculptorModuleAccess().getResourcesResourceParserRuleCall_3_4_1_0());
pushFollow(FOLLOW_91);
lv_resources_12_0=ruleResource();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getSculptorModuleRule());
}
add(
current,
"resources",
lv_resources_12_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Resource");
afterParserOrEnumRuleCall();
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:5306:5: ( (lv_consumers_13_0= ruleConsumer ) )
{
// InternalContextMappingDSL.g:5306:5: ( (lv_consumers_13_0= ruleConsumer ) )
// InternalContextMappingDSL.g:5307:6: (lv_consumers_13_0= ruleConsumer )
{
// InternalContextMappingDSL.g:5307:6: (lv_consumers_13_0= ruleConsumer )
// InternalContextMappingDSL.g:5308:7: lv_consumers_13_0= ruleConsumer
{
newCompositeNode(grammarAccess.getSculptorModuleAccess().getConsumersConsumerParserRuleCall_3_4_2_0());
pushFollow(FOLLOW_91);
lv_consumers_13_0=ruleConsumer();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getSculptorModuleRule());
}
add(
current,
"consumers",
lv_consumers_13_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Consumer");
afterParserOrEnumRuleCall();
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:5326:5: ( (lv_domainObjects_14_0= ruleSimpleDomainObject ) )
{
// InternalContextMappingDSL.g:5326:5: ( (lv_domainObjects_14_0= ruleSimpleDomainObject ) )
// InternalContextMappingDSL.g:5327:6: (lv_domainObjects_14_0= ruleSimpleDomainObject )
{
// InternalContextMappingDSL.g:5327:6: (lv_domainObjects_14_0= ruleSimpleDomainObject )
// InternalContextMappingDSL.g:5328:7: lv_domainObjects_14_0= ruleSimpleDomainObject
{
newCompositeNode(grammarAccess.getSculptorModuleAccess().getDomainObjectsSimpleDomainObjectParserRuleCall_3_4_3_0());
pushFollow(FOLLOW_91);
lv_domainObjects_14_0=ruleSimpleDomainObject();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getSculptorModuleRule());
}
add(
current,
"domainObjects",
lv_domainObjects_14_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.SimpleDomainObject");
afterParserOrEnumRuleCall();
}
}
}
break;
case 5 :
// InternalContextMappingDSL.g:5346:5: ( (lv_aggregates_15_0= ruleAggregate ) )
{
// InternalContextMappingDSL.g:5346:5: ( (lv_aggregates_15_0= ruleAggregate ) )
// InternalContextMappingDSL.g:5347:6: (lv_aggregates_15_0= ruleAggregate )
{
// InternalContextMappingDSL.g:5347:6: (lv_aggregates_15_0= ruleAggregate )
// InternalContextMappingDSL.g:5348:7: lv_aggregates_15_0= ruleAggregate
{
newCompositeNode(grammarAccess.getSculptorModuleAccess().getAggregatesAggregateParserRuleCall_3_4_4_0());
pushFollow(FOLLOW_91);
lv_aggregates_15_0=ruleAggregate();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getSculptorModuleRule());
}
add(
current,
"aggregates",
lv_aggregates_15_0,
"org.contextmapper.dsl.ContextMappingDSL.Aggregate");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop157;
}
} while (true);
this_CLOSE_16=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(this_CLOSE_16, grammarAccess.getSculptorModuleAccess().getCLOSETerminalRuleCall_3_5());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleSculptorModule"
// $ANTLR start "entryRuleApplication"
// InternalContextMappingDSL.g:5375:1: entryRuleApplication returns [EObject current=null] : iv_ruleApplication= ruleApplication EOF ;
public final EObject entryRuleApplication() throws RecognitionException {
EObject current = null;
EObject iv_ruleApplication = null;
try {
// InternalContextMappingDSL.g:5375:52: (iv_ruleApplication= ruleApplication EOF )
// InternalContextMappingDSL.g:5376:2: iv_ruleApplication= ruleApplication EOF
{
newCompositeNode(grammarAccess.getApplicationRule());
pushFollow(FOLLOW_1);
iv_ruleApplication=ruleApplication();
state._fsp--;
current =iv_ruleApplication;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleApplication"
// $ANTLR start "ruleApplication"
// InternalContextMappingDSL.g:5382:1: ruleApplication returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? ( (otherlv_1= 'Application' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' otherlv_4= 'basePackage' otherlv_5= '=' ( (lv_basePackage_6_0= ruleJavaIdentifier ) ) ) | (otherlv_7= 'ApplicationPart' ( (lv_name_8_0= RULE_ID ) ) otherlv_9= '{' ) ) ( ( (lv_services_10_0= ruleService ) ) | ( (lv_resources_11_0= ruleResource ) ) | ( (lv_consumers_12_0= ruleConsumer ) ) | ( (lv_domainObjects_13_0= ruleSimpleDomainObject ) ) )* otherlv_14= '}' ) ;
public final EObject ruleApplication() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token otherlv_1=null;
Token lv_name_2_0=null;
Token otherlv_3=null;
Token otherlv_4=null;
Token otherlv_5=null;
Token otherlv_7=null;
Token lv_name_8_0=null;
Token otherlv_9=null;
Token otherlv_14=null;
AntlrDatatypeRuleToken lv_basePackage_6_0 = null;
EObject lv_services_10_0 = null;
EObject lv_resources_11_0 = null;
EObject lv_consumers_12_0 = null;
EObject lv_domainObjects_13_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:5388:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? ( (otherlv_1= 'Application' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' otherlv_4= 'basePackage' otherlv_5= '=' ( (lv_basePackage_6_0= ruleJavaIdentifier ) ) ) | (otherlv_7= 'ApplicationPart' ( (lv_name_8_0= RULE_ID ) ) otherlv_9= '{' ) ) ( ( (lv_services_10_0= ruleService ) ) | ( (lv_resources_11_0= ruleResource ) ) | ( (lv_consumers_12_0= ruleConsumer ) ) | ( (lv_domainObjects_13_0= ruleSimpleDomainObject ) ) )* otherlv_14= '}' ) )
// InternalContextMappingDSL.g:5389:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (otherlv_1= 'Application' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' otherlv_4= 'basePackage' otherlv_5= '=' ( (lv_basePackage_6_0= ruleJavaIdentifier ) ) ) | (otherlv_7= 'ApplicationPart' ( (lv_name_8_0= RULE_ID ) ) otherlv_9= '{' ) ) ( ( (lv_services_10_0= ruleService ) ) | ( (lv_resources_11_0= ruleResource ) ) | ( (lv_consumers_12_0= ruleConsumer ) ) | ( (lv_domainObjects_13_0= ruleSimpleDomainObject ) ) )* otherlv_14= '}' )
{
// InternalContextMappingDSL.g:5389:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (otherlv_1= 'Application' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' otherlv_4= 'basePackage' otherlv_5= '=' ( (lv_basePackage_6_0= ruleJavaIdentifier ) ) ) | (otherlv_7= 'ApplicationPart' ( (lv_name_8_0= RULE_ID ) ) otherlv_9= '{' ) ) ( ( (lv_services_10_0= ruleService ) ) | ( (lv_resources_11_0= ruleResource ) ) | ( (lv_consumers_12_0= ruleConsumer ) ) | ( (lv_domainObjects_13_0= ruleSimpleDomainObject ) ) )* otherlv_14= '}' )
// InternalContextMappingDSL.g:5390:3: ( (lv_doc_0_0= RULE_STRING ) )? ( (otherlv_1= 'Application' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' otherlv_4= 'basePackage' otherlv_5= '=' ( (lv_basePackage_6_0= ruleJavaIdentifier ) ) ) | (otherlv_7= 'ApplicationPart' ( (lv_name_8_0= RULE_ID ) ) otherlv_9= '{' ) ) ( ( (lv_services_10_0= ruleService ) ) | ( (lv_resources_11_0= ruleResource ) ) | ( (lv_consumers_12_0= ruleConsumer ) ) | ( (lv_domainObjects_13_0= ruleSimpleDomainObject ) ) )* otherlv_14= '}'
{
// InternalContextMappingDSL.g:5390:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt159=2;
int LA159_0 = input.LA(1);
if ( (LA159_0==RULE_STRING) ) {
alt159=1;
}
switch (alt159) {
case 1 :
// InternalContextMappingDSL.g:5391:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:5391:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:5392:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_92);
newLeafNode(lv_doc_0_0, grammarAccess.getApplicationAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getApplicationRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
// InternalContextMappingDSL.g:5408:3: ( (otherlv_1= 'Application' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' otherlv_4= 'basePackage' otherlv_5= '=' ( (lv_basePackage_6_0= ruleJavaIdentifier ) ) ) | (otherlv_7= 'ApplicationPart' ( (lv_name_8_0= RULE_ID ) ) otherlv_9= '{' ) )
int alt160=2;
int LA160_0 = input.LA(1);
if ( (LA160_0==83) ) {
alt160=1;
}
else if ( (LA160_0==84) ) {
alt160=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 160, 0, input);
throw nvae;
}
switch (alt160) {
case 1 :
// InternalContextMappingDSL.g:5409:4: (otherlv_1= 'Application' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' otherlv_4= 'basePackage' otherlv_5= '=' ( (lv_basePackage_6_0= ruleJavaIdentifier ) ) )
{
// InternalContextMappingDSL.g:5409:4: (otherlv_1= 'Application' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' otherlv_4= 'basePackage' otherlv_5= '=' ( (lv_basePackage_6_0= ruleJavaIdentifier ) ) )
// InternalContextMappingDSL.g:5410:5: otherlv_1= 'Application' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' otherlv_4= 'basePackage' otherlv_5= '=' ( (lv_basePackage_6_0= ruleJavaIdentifier ) )
{
otherlv_1=(Token)match(input,83,FOLLOW_10);
newLeafNode(otherlv_1, grammarAccess.getApplicationAccess().getApplicationKeyword_1_0_0());
// InternalContextMappingDSL.g:5414:5: ( (lv_name_2_0= RULE_ID ) )
// InternalContextMappingDSL.g:5415:6: (lv_name_2_0= RULE_ID )
{
// InternalContextMappingDSL.g:5415:6: (lv_name_2_0= RULE_ID )
// InternalContextMappingDSL.g:5416:7: lv_name_2_0= RULE_ID
{
lv_name_2_0=(Token)match(input,RULE_ID,FOLLOW_6);
newLeafNode(lv_name_2_0, grammarAccess.getApplicationAccess().getNameIDTerminalRuleCall_1_0_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getApplicationRule());
}
setWithLastConsumed(
current,
"name",
lv_name_2_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
otherlv_3=(Token)match(input,RULE_OPEN,FOLLOW_93);
newLeafNode(otherlv_3, grammarAccess.getApplicationAccess().getLeftCurlyBracketKeyword_1_0_2());
otherlv_4=(Token)match(input,81,FOLLOW_89);
newLeafNode(otherlv_4, grammarAccess.getApplicationAccess().getBasePackageKeyword_1_0_3());
otherlv_5=(Token)match(input,21,FOLLOW_10);
newLeafNode(otherlv_5, grammarAccess.getApplicationAccess().getEqualsSignKeyword_1_0_4());
// InternalContextMappingDSL.g:5444:5: ( (lv_basePackage_6_0= ruleJavaIdentifier ) )
// InternalContextMappingDSL.g:5445:6: (lv_basePackage_6_0= ruleJavaIdentifier )
{
// InternalContextMappingDSL.g:5445:6: (lv_basePackage_6_0= ruleJavaIdentifier )
// InternalContextMappingDSL.g:5446:7: lv_basePackage_6_0= ruleJavaIdentifier
{
newCompositeNode(grammarAccess.getApplicationAccess().getBasePackageJavaIdentifierParserRuleCall_1_0_5_0());
pushFollow(FOLLOW_73);
lv_basePackage_6_0=ruleJavaIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getApplicationRule());
}
set(
current,
"basePackage",
lv_basePackage_6_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.JavaIdentifier");
afterParserOrEnumRuleCall();
}
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:5465:4: (otherlv_7= 'ApplicationPart' ( (lv_name_8_0= RULE_ID ) ) otherlv_9= '{' )
{
// InternalContextMappingDSL.g:5465:4: (otherlv_7= 'ApplicationPart' ( (lv_name_8_0= RULE_ID ) ) otherlv_9= '{' )
// InternalContextMappingDSL.g:5466:5: otherlv_7= 'ApplicationPart' ( (lv_name_8_0= RULE_ID ) ) otherlv_9= '{'
{
otherlv_7=(Token)match(input,84,FOLLOW_10);
newLeafNode(otherlv_7, grammarAccess.getApplicationAccess().getApplicationPartKeyword_1_1_0());
// InternalContextMappingDSL.g:5470:5: ( (lv_name_8_0= RULE_ID ) )
// InternalContextMappingDSL.g:5471:6: (lv_name_8_0= RULE_ID )
{
// InternalContextMappingDSL.g:5471:6: (lv_name_8_0= RULE_ID )
// InternalContextMappingDSL.g:5472:7: lv_name_8_0= RULE_ID
{
lv_name_8_0=(Token)match(input,RULE_ID,FOLLOW_6);
newLeafNode(lv_name_8_0, grammarAccess.getApplicationAccess().getNameIDTerminalRuleCall_1_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getApplicationRule());
}
setWithLastConsumed(
current,
"name",
lv_name_8_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
otherlv_9=(Token)match(input,RULE_OPEN,FOLLOW_73);
newLeafNode(otherlv_9, grammarAccess.getApplicationAccess().getLeftCurlyBracketKeyword_1_1_2());
}
}
break;
}
// InternalContextMappingDSL.g:5494:3: ( ( (lv_services_10_0= ruleService ) ) | ( (lv_resources_11_0= ruleResource ) ) | ( (lv_consumers_12_0= ruleConsumer ) ) | ( (lv_domainObjects_13_0= ruleSimpleDomainObject ) ) )*
loop161:
do {
int alt161=5;
switch ( input.LA(1) ) {
case RULE_ML_COMMENT:
{
switch ( input.LA(2) ) {
case RULE_STRING:
{
int LA161_8 = input.LA(3);
if ( ((LA161_8>=120 && LA161_8<=121)||LA161_8==136||(LA161_8>=139 && LA161_8<=140)||LA161_8==144) ) {
alt161=4;
}
else if ( (LA161_8==85) ) {
alt161=1;
}
}
break;
case 85:
{
alt161=1;
}
break;
case 120:
case 121:
case 136:
case 139:
case 140:
case 144:
{
alt161=4;
}
break;
}
}
break;
case RULE_STRING:
{
switch ( input.LA(2) ) {
case 120:
case 121:
case 136:
case 139:
case 140:
case 141:
case 144:
case 145:
case 183:
{
alt161=4;
}
break;
case 89:
{
alt161=2;
}
break;
case 85:
{
alt161=1;
}
break;
case 92:
{
alt161=3;
}
break;
}
}
break;
case 85:
{
alt161=1;
}
break;
case 89:
{
alt161=2;
}
break;
case 92:
{
alt161=3;
}
break;
case 120:
case 121:
case 136:
case 139:
case 140:
case 141:
case 144:
case 145:
case 183:
{
alt161=4;
}
break;
}
switch (alt161) {
case 1 :
// InternalContextMappingDSL.g:5495:4: ( (lv_services_10_0= ruleService ) )
{
// InternalContextMappingDSL.g:5495:4: ( (lv_services_10_0= ruleService ) )
// InternalContextMappingDSL.g:5496:5: (lv_services_10_0= ruleService )
{
// InternalContextMappingDSL.g:5496:5: (lv_services_10_0= ruleService )
// InternalContextMappingDSL.g:5497:6: lv_services_10_0= ruleService
{
newCompositeNode(grammarAccess.getApplicationAccess().getServicesServiceParserRuleCall_2_0_0());
pushFollow(FOLLOW_73);
lv_services_10_0=ruleService();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getApplicationRule());
}
add(
current,
"services",
lv_services_10_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Service");
afterParserOrEnumRuleCall();
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:5515:4: ( (lv_resources_11_0= ruleResource ) )
{
// InternalContextMappingDSL.g:5515:4: ( (lv_resources_11_0= ruleResource ) )
// InternalContextMappingDSL.g:5516:5: (lv_resources_11_0= ruleResource )
{
// InternalContextMappingDSL.g:5516:5: (lv_resources_11_0= ruleResource )
// InternalContextMappingDSL.g:5517:6: lv_resources_11_0= ruleResource
{
newCompositeNode(grammarAccess.getApplicationAccess().getResourcesResourceParserRuleCall_2_1_0());
pushFollow(FOLLOW_73);
lv_resources_11_0=ruleResource();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getApplicationRule());
}
add(
current,
"resources",
lv_resources_11_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Resource");
afterParserOrEnumRuleCall();
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:5535:4: ( (lv_consumers_12_0= ruleConsumer ) )
{
// InternalContextMappingDSL.g:5535:4: ( (lv_consumers_12_0= ruleConsumer ) )
// InternalContextMappingDSL.g:5536:5: (lv_consumers_12_0= ruleConsumer )
{
// InternalContextMappingDSL.g:5536:5: (lv_consumers_12_0= ruleConsumer )
// InternalContextMappingDSL.g:5537:6: lv_consumers_12_0= ruleConsumer
{
newCompositeNode(grammarAccess.getApplicationAccess().getConsumersConsumerParserRuleCall_2_2_0());
pushFollow(FOLLOW_73);
lv_consumers_12_0=ruleConsumer();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getApplicationRule());
}
add(
current,
"consumers",
lv_consumers_12_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Consumer");
afterParserOrEnumRuleCall();
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:5555:4: ( (lv_domainObjects_13_0= ruleSimpleDomainObject ) )
{
// InternalContextMappingDSL.g:5555:4: ( (lv_domainObjects_13_0= ruleSimpleDomainObject ) )
// InternalContextMappingDSL.g:5556:5: (lv_domainObjects_13_0= ruleSimpleDomainObject )
{
// InternalContextMappingDSL.g:5556:5: (lv_domainObjects_13_0= ruleSimpleDomainObject )
// InternalContextMappingDSL.g:5557:6: lv_domainObjects_13_0= ruleSimpleDomainObject
{
newCompositeNode(grammarAccess.getApplicationAccess().getDomainObjectsSimpleDomainObjectParserRuleCall_2_3_0());
pushFollow(FOLLOW_73);
lv_domainObjects_13_0=ruleSimpleDomainObject();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getApplicationRule());
}
add(
current,
"domainObjects",
lv_domainObjects_13_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.SimpleDomainObject");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop161;
}
} while (true);
otherlv_14=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(otherlv_14, grammarAccess.getApplicationAccess().getRightCurlyBracketKeyword_3());
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleApplication"
// $ANTLR start "entryRuleService"
// InternalContextMappingDSL.g:5583:1: entryRuleService returns [EObject current=null] : iv_ruleService= ruleService EOF ;
public final EObject entryRuleService() throws RecognitionException {
EObject current = null;
EObject iv_ruleService = null;
try {
// InternalContextMappingDSL.g:5583:48: (iv_ruleService= ruleService EOF )
// InternalContextMappingDSL.g:5584:2: iv_ruleService= ruleService EOF
{
newCompositeNode(grammarAccess.getServiceRule());
pushFollow(FOLLOW_1);
iv_ruleService=ruleService();
state._fsp--;
current =iv_ruleService;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleService"
// $ANTLR start "ruleService"
// InternalContextMappingDSL.g:5590:1: ruleService returns [EObject current=null] : ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? otherlv_2= 'Service' ( (lv_name_3_0= RULE_ID ) ) (otherlv_4= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) ) ) )* ) ) ) ( (lv_dependencies_13_0= ruleDependency ) )* ( (lv_operations_14_0= ruleServiceOperation ) )* otherlv_15= '}' )? ) ;
public final EObject ruleService() throws RecognitionException {
EObject current = null;
Token lv_comment_0_0=null;
Token lv_doc_1_0=null;
Token otherlv_2=null;
Token lv_name_3_0=null;
Token otherlv_4=null;
Token lv_gapClass_6_0=null;
Token lv_noGapClass_7_0=null;
Token otherlv_8=null;
Token otherlv_9=null;
Token lv_hint_10_0=null;
Token lv_webService_11_0=null;
Token otherlv_15=null;
EObject lv_subscribe_12_0 = null;
EObject lv_dependencies_13_0 = null;
EObject lv_operations_14_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:5596:2: ( ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? otherlv_2= 'Service' ( (lv_name_3_0= RULE_ID ) ) (otherlv_4= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) ) ) )* ) ) ) ( (lv_dependencies_13_0= ruleDependency ) )* ( (lv_operations_14_0= ruleServiceOperation ) )* otherlv_15= '}' )? ) )
// InternalContextMappingDSL.g:5597:2: ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? otherlv_2= 'Service' ( (lv_name_3_0= RULE_ID ) ) (otherlv_4= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) ) ) )* ) ) ) ( (lv_dependencies_13_0= ruleDependency ) )* ( (lv_operations_14_0= ruleServiceOperation ) )* otherlv_15= '}' )? )
{
// InternalContextMappingDSL.g:5597:2: ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? otherlv_2= 'Service' ( (lv_name_3_0= RULE_ID ) ) (otherlv_4= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) ) ) )* ) ) ) ( (lv_dependencies_13_0= ruleDependency ) )* ( (lv_operations_14_0= ruleServiceOperation ) )* otherlv_15= '}' )? )
// InternalContextMappingDSL.g:5598:3: ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? otherlv_2= 'Service' ( (lv_name_3_0= RULE_ID ) ) (otherlv_4= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) ) ) )* ) ) ) ( (lv_dependencies_13_0= ruleDependency ) )* ( (lv_operations_14_0= ruleServiceOperation ) )* otherlv_15= '}' )?
{
// InternalContextMappingDSL.g:5598:3: ( (lv_comment_0_0= RULE_ML_COMMENT ) )?
int alt162=2;
int LA162_0 = input.LA(1);
if ( (LA162_0==RULE_ML_COMMENT) ) {
alt162=1;
}
switch (alt162) {
case 1 :
// InternalContextMappingDSL.g:5599:4: (lv_comment_0_0= RULE_ML_COMMENT )
{
// InternalContextMappingDSL.g:5599:4: (lv_comment_0_0= RULE_ML_COMMENT )
// InternalContextMappingDSL.g:5600:5: lv_comment_0_0= RULE_ML_COMMENT
{
lv_comment_0_0=(Token)match(input,RULE_ML_COMMENT,FOLLOW_94);
newLeafNode(lv_comment_0_0, grammarAccess.getServiceAccess().getCommentML_COMMENTTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getServiceRule());
}
setWithLastConsumed(
current,
"comment",
lv_comment_0_0,
"org.eclipse.xtext.common.Terminals.ML_COMMENT");
}
}
break;
}
// InternalContextMappingDSL.g:5616:3: ( (lv_doc_1_0= RULE_STRING ) )?
int alt163=2;
int LA163_0 = input.LA(1);
if ( (LA163_0==RULE_STRING) ) {
alt163=1;
}
switch (alt163) {
case 1 :
// InternalContextMappingDSL.g:5617:4: (lv_doc_1_0= RULE_STRING )
{
// InternalContextMappingDSL.g:5617:4: (lv_doc_1_0= RULE_STRING )
// InternalContextMappingDSL.g:5618:5: lv_doc_1_0= RULE_STRING
{
lv_doc_1_0=(Token)match(input,RULE_STRING,FOLLOW_95);
newLeafNode(lv_doc_1_0, grammarAccess.getServiceAccess().getDocSTRINGTerminalRuleCall_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getServiceRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_1_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
otherlv_2=(Token)match(input,85,FOLLOW_10);
newLeafNode(otherlv_2, grammarAccess.getServiceAccess().getServiceKeyword_2());
// InternalContextMappingDSL.g:5638:3: ( (lv_name_3_0= RULE_ID ) )
// InternalContextMappingDSL.g:5639:4: (lv_name_3_0= RULE_ID )
{
// InternalContextMappingDSL.g:5639:4: (lv_name_3_0= RULE_ID )
// InternalContextMappingDSL.g:5640:5: lv_name_3_0= RULE_ID
{
lv_name_3_0=(Token)match(input,RULE_ID,FOLLOW_23);
newLeafNode(lv_name_3_0, grammarAccess.getServiceAccess().getNameIDTerminalRuleCall_3_0());
if (current==null) {
current = createModelElement(grammarAccess.getServiceRule());
}
setWithLastConsumed(
current,
"name",
lv_name_3_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:5656:3: (otherlv_4= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) ) ) )* ) ) ) ( (lv_dependencies_13_0= ruleDependency ) )* ( (lv_operations_14_0= ruleServiceOperation ) )* otherlv_15= '}' )?
int alt168=2;
int LA168_0 = input.LA(1);
if ( (LA168_0==RULE_OPEN) ) {
alt168=1;
}
switch (alt168) {
case 1 :
// InternalContextMappingDSL.g:5657:4: otherlv_4= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) ) ) )* ) ) ) ( (lv_dependencies_13_0= ruleDependency ) )* ( (lv_operations_14_0= ruleServiceOperation ) )* otherlv_15= '}'
{
otherlv_4=(Token)match(input,RULE_OPEN,FOLLOW_96);
newLeafNode(otherlv_4, grammarAccess.getServiceAccess().getLeftCurlyBracketKeyword_4_0());
// InternalContextMappingDSL.g:5661:4: ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:5662:5: ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:5662:5: ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:5663:6: ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getServiceAccess().getUnorderedGroup_4_1());
// InternalContextMappingDSL.g:5666:6: ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) ) ) )* )
// InternalContextMappingDSL.g:5667:7: ( ({...}? => ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:5667:7: ( ({...}? => ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) ) ) )*
loop165:
do {
int alt165=5;
int LA165_0 = input.LA(1);
if ( LA165_0 >= 86 && LA165_0 <= 87 && getUnorderedGroupHelper().canSelect(grammarAccess.getServiceAccess().getUnorderedGroup_4_1(), 0) ) {
alt165=1;
}
else if ( LA165_0 == 82 && getUnorderedGroupHelper().canSelect(grammarAccess.getServiceAccess().getUnorderedGroup_4_1(), 1) ) {
alt165=2;
}
else if ( LA165_0 == 88 && getUnorderedGroupHelper().canSelect(grammarAccess.getServiceAccess().getUnorderedGroup_4_1(), 2) ) {
alt165=3;
}
else if ( LA165_0 == 97 && getUnorderedGroupHelper().canSelect(grammarAccess.getServiceAccess().getUnorderedGroup_4_1(), 3) ) {
alt165=4;
}
switch (alt165) {
case 1 :
// InternalContextMappingDSL.g:5668:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) ) )
{
// InternalContextMappingDSL.g:5668:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) ) )
// InternalContextMappingDSL.g:5669:6: {...}? => ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getServiceAccess().getUnorderedGroup_4_1(), 0) ) {
throw new FailedPredicateException(input, "ruleService", "getUnorderedGroupHelper().canSelect(grammarAccess.getServiceAccess().getUnorderedGroup_4_1(), 0)");
}
// InternalContextMappingDSL.g:5669:107: ( ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) ) )
// InternalContextMappingDSL.g:5670:7: ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getServiceAccess().getUnorderedGroup_4_1(), 0);
// InternalContextMappingDSL.g:5673:10: ({...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) ) )
// InternalContextMappingDSL.g:5673:11: {...}? => ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleService", "true");
}
// InternalContextMappingDSL.g:5673:20: ( ( (lv_gapClass_6_0= 'gap' ) ) | ( (lv_noGapClass_7_0= 'nogap' ) ) )
int alt164=2;
int LA164_0 = input.LA(1);
if ( (LA164_0==86) ) {
alt164=1;
}
else if ( (LA164_0==87) ) {
alt164=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 164, 0, input);
throw nvae;
}
switch (alt164) {
case 1 :
// InternalContextMappingDSL.g:5673:21: ( (lv_gapClass_6_0= 'gap' ) )
{
// InternalContextMappingDSL.g:5673:21: ( (lv_gapClass_6_0= 'gap' ) )
// InternalContextMappingDSL.g:5674:11: (lv_gapClass_6_0= 'gap' )
{
// InternalContextMappingDSL.g:5674:11: (lv_gapClass_6_0= 'gap' )
// InternalContextMappingDSL.g:5675:12: lv_gapClass_6_0= 'gap'
{
lv_gapClass_6_0=(Token)match(input,86,FOLLOW_96);
newLeafNode(lv_gapClass_6_0, grammarAccess.getServiceAccess().getGapClassGapKeyword_4_1_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getServiceRule());
}
setWithLastConsumed(current, "gapClass", true, "gap");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:5688:10: ( (lv_noGapClass_7_0= 'nogap' ) )
{
// InternalContextMappingDSL.g:5688:10: ( (lv_noGapClass_7_0= 'nogap' ) )
// InternalContextMappingDSL.g:5689:11: (lv_noGapClass_7_0= 'nogap' )
{
// InternalContextMappingDSL.g:5689:11: (lv_noGapClass_7_0= 'nogap' )
// InternalContextMappingDSL.g:5690:12: lv_noGapClass_7_0= 'nogap'
{
lv_noGapClass_7_0=(Token)match(input,87,FOLLOW_96);
newLeafNode(lv_noGapClass_7_0, grammarAccess.getServiceAccess().getNoGapClassNogapKeyword_4_1_0_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getServiceRule());
}
setWithLastConsumed(current, "noGapClass", true, "nogap");
}
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getServiceAccess().getUnorderedGroup_4_1());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:5708:5: ({...}? => ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:5708:5: ({...}? => ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:5709:6: {...}? => ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getServiceAccess().getUnorderedGroup_4_1(), 1) ) {
throw new FailedPredicateException(input, "ruleService", "getUnorderedGroupHelper().canSelect(grammarAccess.getServiceAccess().getUnorderedGroup_4_1(), 1)");
}
// InternalContextMappingDSL.g:5709:107: ( ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:5710:7: ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getServiceAccess().getUnorderedGroup_4_1(), 1);
// InternalContextMappingDSL.g:5713:10: ({...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:5713:11: {...}? => (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleService", "true");
}
// InternalContextMappingDSL.g:5713:20: (otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:5713:21: otherlv_8= 'hint' otherlv_9= '=' ( (lv_hint_10_0= RULE_STRING ) )
{
otherlv_8=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_8, grammarAccess.getServiceAccess().getHintKeyword_4_1_1_0());
otherlv_9=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_9, grammarAccess.getServiceAccess().getEqualsSignKeyword_4_1_1_1());
// InternalContextMappingDSL.g:5721:10: ( (lv_hint_10_0= RULE_STRING ) )
// InternalContextMappingDSL.g:5722:11: (lv_hint_10_0= RULE_STRING )
{
// InternalContextMappingDSL.g:5722:11: (lv_hint_10_0= RULE_STRING )
// InternalContextMappingDSL.g:5723:12: lv_hint_10_0= RULE_STRING
{
lv_hint_10_0=(Token)match(input,RULE_STRING,FOLLOW_96);
newLeafNode(lv_hint_10_0, grammarAccess.getServiceAccess().getHintSTRINGTerminalRuleCall_4_1_1_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getServiceRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_10_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getServiceAccess().getUnorderedGroup_4_1());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:5745:5: ({...}? => ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) ) )
{
// InternalContextMappingDSL.g:5745:5: ({...}? => ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) ) )
// InternalContextMappingDSL.g:5746:6: {...}? => ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getServiceAccess().getUnorderedGroup_4_1(), 2) ) {
throw new FailedPredicateException(input, "ruleService", "getUnorderedGroupHelper().canSelect(grammarAccess.getServiceAccess().getUnorderedGroup_4_1(), 2)");
}
// InternalContextMappingDSL.g:5746:107: ( ({...}? => ( (lv_webService_11_0= 'webservice' ) ) ) )
// InternalContextMappingDSL.g:5747:7: ({...}? => ( (lv_webService_11_0= 'webservice' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getServiceAccess().getUnorderedGroup_4_1(), 2);
// InternalContextMappingDSL.g:5750:10: ({...}? => ( (lv_webService_11_0= 'webservice' ) ) )
// InternalContextMappingDSL.g:5750:11: {...}? => ( (lv_webService_11_0= 'webservice' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleService", "true");
}
// InternalContextMappingDSL.g:5750:20: ( (lv_webService_11_0= 'webservice' ) )
// InternalContextMappingDSL.g:5750:21: (lv_webService_11_0= 'webservice' )
{
// InternalContextMappingDSL.g:5750:21: (lv_webService_11_0= 'webservice' )
// InternalContextMappingDSL.g:5751:11: lv_webService_11_0= 'webservice'
{
lv_webService_11_0=(Token)match(input,88,FOLLOW_96);
newLeafNode(lv_webService_11_0, grammarAccess.getServiceAccess().getWebServiceWebserviceKeyword_4_1_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getServiceRule());
}
setWithLastConsumed(current, "webService", true, "webservice");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getServiceAccess().getUnorderedGroup_4_1());
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:5768:5: ({...}? => ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) ) )
{
// InternalContextMappingDSL.g:5768:5: ({...}? => ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) ) )
// InternalContextMappingDSL.g:5769:6: {...}? => ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getServiceAccess().getUnorderedGroup_4_1(), 3) ) {
throw new FailedPredicateException(input, "ruleService", "getUnorderedGroupHelper().canSelect(grammarAccess.getServiceAccess().getUnorderedGroup_4_1(), 3)");
}
// InternalContextMappingDSL.g:5769:107: ( ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) ) )
// InternalContextMappingDSL.g:5770:7: ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getServiceAccess().getUnorderedGroup_4_1(), 3);
// InternalContextMappingDSL.g:5773:10: ({...}? => ( (lv_subscribe_12_0= ruleSubscribe ) ) )
// InternalContextMappingDSL.g:5773:11: {...}? => ( (lv_subscribe_12_0= ruleSubscribe ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleService", "true");
}
// InternalContextMappingDSL.g:5773:20: ( (lv_subscribe_12_0= ruleSubscribe ) )
// InternalContextMappingDSL.g:5773:21: (lv_subscribe_12_0= ruleSubscribe )
{
// InternalContextMappingDSL.g:5773:21: (lv_subscribe_12_0= ruleSubscribe )
// InternalContextMappingDSL.g:5774:11: lv_subscribe_12_0= ruleSubscribe
{
newCompositeNode(grammarAccess.getServiceAccess().getSubscribeSubscribeParserRuleCall_4_1_3_0());
pushFollow(FOLLOW_96);
lv_subscribe_12_0=ruleSubscribe();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getServiceRule());
}
set(
current,
"subscribe",
lv_subscribe_12_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Subscribe");
afterParserOrEnumRuleCall();
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getServiceAccess().getUnorderedGroup_4_1());
}
}
}
break;
default :
break loop165;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getServiceAccess().getUnorderedGroup_4_1());
}
// InternalContextMappingDSL.g:5803:4: ( (lv_dependencies_13_0= ruleDependency ) )*
loop166:
do {
int alt166=2;
int LA166_0 = input.LA(1);
if ( (LA166_0==119||LA166_0==182) ) {
alt166=1;
}
switch (alt166) {
case 1 :
// InternalContextMappingDSL.g:5804:5: (lv_dependencies_13_0= ruleDependency )
{
// InternalContextMappingDSL.g:5804:5: (lv_dependencies_13_0= ruleDependency )
// InternalContextMappingDSL.g:5805:6: lv_dependencies_13_0= ruleDependency
{
newCompositeNode(grammarAccess.getServiceAccess().getDependenciesDependencyParserRuleCall_4_2_0());
pushFollow(FOLLOW_97);
lv_dependencies_13_0=ruleDependency();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getServiceRule());
}
add(
current,
"dependencies",
lv_dependencies_13_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Dependency");
afterParserOrEnumRuleCall();
}
}
break;
default :
break loop166;
}
} while (true);
// InternalContextMappingDSL.g:5822:4: ( (lv_operations_14_0= ruleServiceOperation ) )*
loop167:
do {
int alt167=2;
int LA167_0 = input.LA(1);
if ( ((LA167_0>=RULE_STRING && LA167_0<=RULE_ID)||LA167_0==RULE_MAP_COLLECTION_TYPE||LA167_0==94||LA167_0==101||LA167_0==124||(LA167_0>=185 && LA167_0<=206)||LA167_0==233||(LA167_0>=243 && LA167_0<=249)) ) {
alt167=1;
}
switch (alt167) {
case 1 :
// InternalContextMappingDSL.g:5823:5: (lv_operations_14_0= ruleServiceOperation )
{
// InternalContextMappingDSL.g:5823:5: (lv_operations_14_0= ruleServiceOperation )
// InternalContextMappingDSL.g:5824:6: lv_operations_14_0= ruleServiceOperation
{
newCompositeNode(grammarAccess.getServiceAccess().getOperationsServiceOperationParserRuleCall_4_3_0());
pushFollow(FOLLOW_98);
lv_operations_14_0=ruleServiceOperation();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getServiceRule());
}
add(
current,
"operations",
lv_operations_14_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.ServiceOperation");
afterParserOrEnumRuleCall();
}
}
break;
default :
break loop167;
}
} while (true);
otherlv_15=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(otherlv_15, grammarAccess.getServiceAccess().getRightCurlyBracketKeyword_4_4());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleService"
// $ANTLR start "entryRuleResource"
// InternalContextMappingDSL.g:5850:1: entryRuleResource returns [EObject current=null] : iv_ruleResource= ruleResource EOF ;
public final EObject entryRuleResource() throws RecognitionException {
EObject current = null;
EObject iv_ruleResource = null;
try {
// InternalContextMappingDSL.g:5850:49: (iv_ruleResource= ruleResource EOF )
// InternalContextMappingDSL.g:5851:2: iv_ruleResource= ruleResource EOF
{
newCompositeNode(grammarAccess.getResourceRule());
pushFollow(FOLLOW_1);
iv_ruleResource=ruleResource();
state._fsp--;
current =iv_ruleResource;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleResource"
// $ANTLR start "ruleResource"
// InternalContextMappingDSL.g:5857:1: ruleResource returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Resource' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_dependencies_14_0= ruleServiceDependency ) )* ( (lv_operations_15_0= ruleResourceOperation ) )* otherlv_16= '}' )? ) ;
public final EObject ruleResource() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token otherlv_1=null;
Token lv_name_2_0=null;
Token otherlv_3=null;
Token lv_gapClass_5_0=null;
Token lv_noGapClass_6_0=null;
Token otherlv_7=null;
Token otherlv_8=null;
Token lv_hint_9_0=null;
Token lv_scaffold_10_0=null;
Token otherlv_11=null;
Token otherlv_12=null;
Token lv_path_13_0=null;
Token otherlv_16=null;
EObject lv_dependencies_14_0 = null;
EObject lv_operations_15_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:5863:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Resource' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_dependencies_14_0= ruleServiceDependency ) )* ( (lv_operations_15_0= ruleResourceOperation ) )* otherlv_16= '}' )? ) )
// InternalContextMappingDSL.g:5864:2: ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Resource' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_dependencies_14_0= ruleServiceDependency ) )* ( (lv_operations_15_0= ruleResourceOperation ) )* otherlv_16= '}' )? )
{
// InternalContextMappingDSL.g:5864:2: ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Resource' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_dependencies_14_0= ruleServiceDependency ) )* ( (lv_operations_15_0= ruleResourceOperation ) )* otherlv_16= '}' )? )
// InternalContextMappingDSL.g:5865:3: ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Resource' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_dependencies_14_0= ruleServiceDependency ) )* ( (lv_operations_15_0= ruleResourceOperation ) )* otherlv_16= '}' )?
{
// InternalContextMappingDSL.g:5865:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt169=2;
int LA169_0 = input.LA(1);
if ( (LA169_0==RULE_STRING) ) {
alt169=1;
}
switch (alt169) {
case 1 :
// InternalContextMappingDSL.g:5866:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:5866:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:5867:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_99);
newLeafNode(lv_doc_0_0, grammarAccess.getResourceAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getResourceRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
otherlv_1=(Token)match(input,89,FOLLOW_10);
newLeafNode(otherlv_1, grammarAccess.getResourceAccess().getResourceKeyword_1());
// InternalContextMappingDSL.g:5887:3: ( (lv_name_2_0= RULE_ID ) )
// InternalContextMappingDSL.g:5888:4: (lv_name_2_0= RULE_ID )
{
// InternalContextMappingDSL.g:5888:4: (lv_name_2_0= RULE_ID )
// InternalContextMappingDSL.g:5889:5: lv_name_2_0= RULE_ID
{
lv_name_2_0=(Token)match(input,RULE_ID,FOLLOW_23);
newLeafNode(lv_name_2_0, grammarAccess.getResourceAccess().getNameIDTerminalRuleCall_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getResourceRule());
}
setWithLastConsumed(
current,
"name",
lv_name_2_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:5905:3: (otherlv_3= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_dependencies_14_0= ruleServiceDependency ) )* ( (lv_operations_15_0= ruleResourceOperation ) )* otherlv_16= '}' )?
int alt174=2;
int LA174_0 = input.LA(1);
if ( (LA174_0==RULE_OPEN) ) {
alt174=1;
}
switch (alt174) {
case 1 :
// InternalContextMappingDSL.g:5906:4: otherlv_3= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_dependencies_14_0= ruleServiceDependency ) )* ( (lv_operations_15_0= ruleResourceOperation ) )* otherlv_16= '}'
{
otherlv_3=(Token)match(input,RULE_OPEN,FOLLOW_100);
newLeafNode(otherlv_3, grammarAccess.getResourceAccess().getLeftCurlyBracketKeyword_3_0());
// InternalContextMappingDSL.g:5910:4: ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:5911:5: ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:5911:5: ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:5912:6: ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getResourceAccess().getUnorderedGroup_3_1());
// InternalContextMappingDSL.g:5915:6: ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:5916:7: ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:5916:7: ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) ) ) )*
loop171:
do {
int alt171=5;
int LA171_0 = input.LA(1);
if ( LA171_0 >= 86 && LA171_0 <= 87 && getUnorderedGroupHelper().canSelect(grammarAccess.getResourceAccess().getUnorderedGroup_3_1(), 0) ) {
alt171=1;
}
else if ( LA171_0 == 82 && getUnorderedGroupHelper().canSelect(grammarAccess.getResourceAccess().getUnorderedGroup_3_1(), 1) ) {
alt171=2;
}
else if ( LA171_0 == 90 && getUnorderedGroupHelper().canSelect(grammarAccess.getResourceAccess().getUnorderedGroup_3_1(), 2) ) {
alt171=3;
}
else if ( LA171_0 == 91 && getUnorderedGroupHelper().canSelect(grammarAccess.getResourceAccess().getUnorderedGroup_3_1(), 3) ) {
alt171=4;
}
switch (alt171) {
case 1 :
// InternalContextMappingDSL.g:5917:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) )
{
// InternalContextMappingDSL.g:5917:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) )
// InternalContextMappingDSL.g:5918:6: {...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getResourceAccess().getUnorderedGroup_3_1(), 0) ) {
throw new FailedPredicateException(input, "ruleResource", "getUnorderedGroupHelper().canSelect(grammarAccess.getResourceAccess().getUnorderedGroup_3_1(), 0)");
}
// InternalContextMappingDSL.g:5918:108: ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) )
// InternalContextMappingDSL.g:5919:7: ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getResourceAccess().getUnorderedGroup_3_1(), 0);
// InternalContextMappingDSL.g:5922:10: ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) )
// InternalContextMappingDSL.g:5922:11: {...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleResource", "true");
}
// InternalContextMappingDSL.g:5922:20: ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) )
int alt170=2;
int LA170_0 = input.LA(1);
if ( (LA170_0==86) ) {
alt170=1;
}
else if ( (LA170_0==87) ) {
alt170=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 170, 0, input);
throw nvae;
}
switch (alt170) {
case 1 :
// InternalContextMappingDSL.g:5922:21: ( (lv_gapClass_5_0= 'gap' ) )
{
// InternalContextMappingDSL.g:5922:21: ( (lv_gapClass_5_0= 'gap' ) )
// InternalContextMappingDSL.g:5923:11: (lv_gapClass_5_0= 'gap' )
{
// InternalContextMappingDSL.g:5923:11: (lv_gapClass_5_0= 'gap' )
// InternalContextMappingDSL.g:5924:12: lv_gapClass_5_0= 'gap'
{
lv_gapClass_5_0=(Token)match(input,86,FOLLOW_100);
newLeafNode(lv_gapClass_5_0, grammarAccess.getResourceAccess().getGapClassGapKeyword_3_1_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getResourceRule());
}
setWithLastConsumed(current, "gapClass", true, "gap");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:5937:10: ( (lv_noGapClass_6_0= 'nogap' ) )
{
// InternalContextMappingDSL.g:5937:10: ( (lv_noGapClass_6_0= 'nogap' ) )
// InternalContextMappingDSL.g:5938:11: (lv_noGapClass_6_0= 'nogap' )
{
// InternalContextMappingDSL.g:5938:11: (lv_noGapClass_6_0= 'nogap' )
// InternalContextMappingDSL.g:5939:12: lv_noGapClass_6_0= 'nogap'
{
lv_noGapClass_6_0=(Token)match(input,87,FOLLOW_100);
newLeafNode(lv_noGapClass_6_0, grammarAccess.getResourceAccess().getNoGapClassNogapKeyword_3_1_0_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getResourceRule());
}
setWithLastConsumed(current, "noGapClass", true, "nogap");
}
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getResourceAccess().getUnorderedGroup_3_1());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:5957:5: ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:5957:5: ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:5958:6: {...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getResourceAccess().getUnorderedGroup_3_1(), 1) ) {
throw new FailedPredicateException(input, "ruleResource", "getUnorderedGroupHelper().canSelect(grammarAccess.getResourceAccess().getUnorderedGroup_3_1(), 1)");
}
// InternalContextMappingDSL.g:5958:108: ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:5959:7: ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getResourceAccess().getUnorderedGroup_3_1(), 1);
// InternalContextMappingDSL.g:5962:10: ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:5962:11: {...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleResource", "true");
}
// InternalContextMappingDSL.g:5962:20: (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:5962:21: otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) )
{
otherlv_7=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_7, grammarAccess.getResourceAccess().getHintKeyword_3_1_1_0());
otherlv_8=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_8, grammarAccess.getResourceAccess().getEqualsSignKeyword_3_1_1_1());
// InternalContextMappingDSL.g:5970:10: ( (lv_hint_9_0= RULE_STRING ) )
// InternalContextMappingDSL.g:5971:11: (lv_hint_9_0= RULE_STRING )
{
// InternalContextMappingDSL.g:5971:11: (lv_hint_9_0= RULE_STRING )
// InternalContextMappingDSL.g:5972:12: lv_hint_9_0= RULE_STRING
{
lv_hint_9_0=(Token)match(input,RULE_STRING,FOLLOW_100);
newLeafNode(lv_hint_9_0, grammarAccess.getResourceAccess().getHintSTRINGTerminalRuleCall_3_1_1_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getResourceRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_9_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getResourceAccess().getUnorderedGroup_3_1());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:5994:5: ({...}? => ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) ) )
{
// InternalContextMappingDSL.g:5994:5: ({...}? => ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) ) )
// InternalContextMappingDSL.g:5995:6: {...}? => ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getResourceAccess().getUnorderedGroup_3_1(), 2) ) {
throw new FailedPredicateException(input, "ruleResource", "getUnorderedGroupHelper().canSelect(grammarAccess.getResourceAccess().getUnorderedGroup_3_1(), 2)");
}
// InternalContextMappingDSL.g:5995:108: ( ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) ) )
// InternalContextMappingDSL.g:5996:7: ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getResourceAccess().getUnorderedGroup_3_1(), 2);
// InternalContextMappingDSL.g:5999:10: ({...}? => ( (lv_scaffold_10_0= 'scaffold' ) ) )
// InternalContextMappingDSL.g:5999:11: {...}? => ( (lv_scaffold_10_0= 'scaffold' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleResource", "true");
}
// InternalContextMappingDSL.g:5999:20: ( (lv_scaffold_10_0= 'scaffold' ) )
// InternalContextMappingDSL.g:5999:21: (lv_scaffold_10_0= 'scaffold' )
{
// InternalContextMappingDSL.g:5999:21: (lv_scaffold_10_0= 'scaffold' )
// InternalContextMappingDSL.g:6000:11: lv_scaffold_10_0= 'scaffold'
{
lv_scaffold_10_0=(Token)match(input,90,FOLLOW_100);
newLeafNode(lv_scaffold_10_0, grammarAccess.getResourceAccess().getScaffoldScaffoldKeyword_3_1_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getResourceRule());
}
setWithLastConsumed(current, "scaffold", true, "scaffold");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getResourceAccess().getUnorderedGroup_3_1());
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:6017:5: ({...}? => ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:6017:5: ({...}? => ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:6018:6: {...}? => ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getResourceAccess().getUnorderedGroup_3_1(), 3) ) {
throw new FailedPredicateException(input, "ruleResource", "getUnorderedGroupHelper().canSelect(grammarAccess.getResourceAccess().getUnorderedGroup_3_1(), 3)");
}
// InternalContextMappingDSL.g:6018:108: ( ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:6019:7: ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getResourceAccess().getUnorderedGroup_3_1(), 3);
// InternalContextMappingDSL.g:6022:10: ({...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:6022:11: {...}? => (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleResource", "true");
}
// InternalContextMappingDSL.g:6022:20: (otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:6022:21: otherlv_11= 'path' otherlv_12= '=' ( (lv_path_13_0= RULE_STRING ) )
{
otherlv_11=(Token)match(input,91,FOLLOW_89);
newLeafNode(otherlv_11, grammarAccess.getResourceAccess().getPathKeyword_3_1_3_0());
otherlv_12=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_12, grammarAccess.getResourceAccess().getEqualsSignKeyword_3_1_3_1());
// InternalContextMappingDSL.g:6030:10: ( (lv_path_13_0= RULE_STRING ) )
// InternalContextMappingDSL.g:6031:11: (lv_path_13_0= RULE_STRING )
{
// InternalContextMappingDSL.g:6031:11: (lv_path_13_0= RULE_STRING )
// InternalContextMappingDSL.g:6032:12: lv_path_13_0= RULE_STRING
{
lv_path_13_0=(Token)match(input,RULE_STRING,FOLLOW_100);
newLeafNode(lv_path_13_0, grammarAccess.getResourceAccess().getPathSTRINGTerminalRuleCall_3_1_3_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getResourceRule());
}
setWithLastConsumed(
current,
"path",
lv_path_13_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getResourceAccess().getUnorderedGroup_3_1());
}
}
}
break;
default :
break loop171;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getResourceAccess().getUnorderedGroup_3_1());
}
// InternalContextMappingDSL.g:6061:4: ( (lv_dependencies_14_0= ruleServiceDependency ) )*
loop172:
do {
int alt172=2;
int LA172_0 = input.LA(1);
if ( (LA172_0==119||LA172_0==182) ) {
alt172=1;
}
switch (alt172) {
case 1 :
// InternalContextMappingDSL.g:6062:5: (lv_dependencies_14_0= ruleServiceDependency )
{
// InternalContextMappingDSL.g:6062:5: (lv_dependencies_14_0= ruleServiceDependency )
// InternalContextMappingDSL.g:6063:6: lv_dependencies_14_0= ruleServiceDependency
{
newCompositeNode(grammarAccess.getResourceAccess().getDependenciesServiceDependencyParserRuleCall_3_2_0());
pushFollow(FOLLOW_101);
lv_dependencies_14_0=ruleServiceDependency();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getResourceRule());
}
add(
current,
"dependencies",
lv_dependencies_14_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.ServiceDependency");
afterParserOrEnumRuleCall();
}
}
break;
default :
break loop172;
}
} while (true);
// InternalContextMappingDSL.g:6080:4: ( (lv_operations_15_0= ruleResourceOperation ) )*
loop173:
do {
int alt173=2;
int LA173_0 = input.LA(1);
if ( ((LA173_0>=RULE_STRING && LA173_0<=RULE_ID)||LA173_0==RULE_MAP_COLLECTION_TYPE||LA173_0==94||LA173_0==124||(LA173_0>=185 && LA173_0<=206)||LA173_0==233||(LA173_0>=243 && LA173_0<=249)) ) {
alt173=1;
}
switch (alt173) {
case 1 :
// InternalContextMappingDSL.g:6081:5: (lv_operations_15_0= ruleResourceOperation )
{
// InternalContextMappingDSL.g:6081:5: (lv_operations_15_0= ruleResourceOperation )
// InternalContextMappingDSL.g:6082:6: lv_operations_15_0= ruleResourceOperation
{
newCompositeNode(grammarAccess.getResourceAccess().getOperationsResourceOperationParserRuleCall_3_3_0());
pushFollow(FOLLOW_102);
lv_operations_15_0=ruleResourceOperation();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getResourceRule());
}
add(
current,
"operations",
lv_operations_15_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.ResourceOperation");
afterParserOrEnumRuleCall();
}
}
break;
default :
break loop173;
}
} while (true);
otherlv_16=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(otherlv_16, grammarAccess.getResourceAccess().getRightCurlyBracketKeyword_3_4());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleResource"
// $ANTLR start "entryRuleConsumer"
// InternalContextMappingDSL.g:6108:1: entryRuleConsumer returns [EObject current=null] : iv_ruleConsumer= ruleConsumer EOF ;
public final EObject entryRuleConsumer() throws RecognitionException {
EObject current = null;
EObject iv_ruleConsumer = null;
try {
// InternalContextMappingDSL.g:6108:49: (iv_ruleConsumer= ruleConsumer EOF )
// InternalContextMappingDSL.g:6109:2: iv_ruleConsumer= ruleConsumer EOF
{
newCompositeNode(grammarAccess.getConsumerRule());
pushFollow(FOLLOW_1);
iv_ruleConsumer=ruleConsumer();
state._fsp--;
current =iv_ruleConsumer;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleConsumer"
// $ANTLR start "ruleConsumer"
// InternalContextMappingDSL.g:6115:1: ruleConsumer returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Consumer' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' (otherlv_4= 'hint' otherlv_5= '=' ( (lv_hint_6_0= RULE_STRING ) ) )? ( (lv_dependencies_7_0= ruleDependency ) )* (otherlv_8= 'unmarshall to' (otherlv_9= '@' )? ( (otherlv_10= RULE_ID ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) ) ) ) )* ) ) ) otherlv_17= '}' ) ;
public final EObject ruleConsumer() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token otherlv_1=null;
Token lv_name_2_0=null;
Token otherlv_3=null;
Token otherlv_4=null;
Token otherlv_5=null;
Token lv_hint_6_0=null;
Token otherlv_8=null;
Token otherlv_9=null;
Token otherlv_10=null;
Token otherlv_12=null;
Token otherlv_13=null;
Token otherlv_14=null;
Token otherlv_17=null;
EObject lv_dependencies_7_0 = null;
AntlrDatatypeRuleToken lv_channel_15_0 = null;
EObject lv_subscribe_16_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:6121:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Consumer' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' (otherlv_4= 'hint' otherlv_5= '=' ( (lv_hint_6_0= RULE_STRING ) ) )? ( (lv_dependencies_7_0= ruleDependency ) )* (otherlv_8= 'unmarshall to' (otherlv_9= '@' )? ( (otherlv_10= RULE_ID ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) ) ) ) )* ) ) ) otherlv_17= '}' ) )
// InternalContextMappingDSL.g:6122:2: ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Consumer' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' (otherlv_4= 'hint' otherlv_5= '=' ( (lv_hint_6_0= RULE_STRING ) ) )? ( (lv_dependencies_7_0= ruleDependency ) )* (otherlv_8= 'unmarshall to' (otherlv_9= '@' )? ( (otherlv_10= RULE_ID ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) ) ) ) )* ) ) ) otherlv_17= '}' )
{
// InternalContextMappingDSL.g:6122:2: ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Consumer' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' (otherlv_4= 'hint' otherlv_5= '=' ( (lv_hint_6_0= RULE_STRING ) ) )? ( (lv_dependencies_7_0= ruleDependency ) )* (otherlv_8= 'unmarshall to' (otherlv_9= '@' )? ( (otherlv_10= RULE_ID ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) ) ) ) )* ) ) ) otherlv_17= '}' )
// InternalContextMappingDSL.g:6123:3: ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Consumer' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' (otherlv_4= 'hint' otherlv_5= '=' ( (lv_hint_6_0= RULE_STRING ) ) )? ( (lv_dependencies_7_0= ruleDependency ) )* (otherlv_8= 'unmarshall to' (otherlv_9= '@' )? ( (otherlv_10= RULE_ID ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) ) ) ) )* ) ) ) otherlv_17= '}'
{
// InternalContextMappingDSL.g:6123:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt175=2;
int LA175_0 = input.LA(1);
if ( (LA175_0==RULE_STRING) ) {
alt175=1;
}
switch (alt175) {
case 1 :
// InternalContextMappingDSL.g:6124:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:6124:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:6125:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_103);
newLeafNode(lv_doc_0_0, grammarAccess.getConsumerAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getConsumerRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
otherlv_1=(Token)match(input,92,FOLLOW_10);
newLeafNode(otherlv_1, grammarAccess.getConsumerAccess().getConsumerKeyword_1());
// InternalContextMappingDSL.g:6145:3: ( (lv_name_2_0= RULE_ID ) )
// InternalContextMappingDSL.g:6146:4: (lv_name_2_0= RULE_ID )
{
// InternalContextMappingDSL.g:6146:4: (lv_name_2_0= RULE_ID )
// InternalContextMappingDSL.g:6147:5: lv_name_2_0= RULE_ID
{
lv_name_2_0=(Token)match(input,RULE_ID,FOLLOW_6);
newLeafNode(lv_name_2_0, grammarAccess.getConsumerAccess().getNameIDTerminalRuleCall_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getConsumerRule());
}
setWithLastConsumed(
current,
"name",
lv_name_2_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
otherlv_3=(Token)match(input,RULE_OPEN,FOLLOW_104);
newLeafNode(otherlv_3, grammarAccess.getConsumerAccess().getLeftCurlyBracketKeyword_3());
// InternalContextMappingDSL.g:6167:3: (otherlv_4= 'hint' otherlv_5= '=' ( (lv_hint_6_0= RULE_STRING ) ) )?
int alt176=2;
int LA176_0 = input.LA(1);
if ( (LA176_0==82) ) {
alt176=1;
}
switch (alt176) {
case 1 :
// InternalContextMappingDSL.g:6168:4: otherlv_4= 'hint' otherlv_5= '=' ( (lv_hint_6_0= RULE_STRING ) )
{
otherlv_4=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_4, grammarAccess.getConsumerAccess().getHintKeyword_4_0());
otherlv_5=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_5, grammarAccess.getConsumerAccess().getEqualsSignKeyword_4_1());
// InternalContextMappingDSL.g:6176:4: ( (lv_hint_6_0= RULE_STRING ) )
// InternalContextMappingDSL.g:6177:5: (lv_hint_6_0= RULE_STRING )
{
// InternalContextMappingDSL.g:6177:5: (lv_hint_6_0= RULE_STRING )
// InternalContextMappingDSL.g:6178:6: lv_hint_6_0= RULE_STRING
{
lv_hint_6_0=(Token)match(input,RULE_STRING,FOLLOW_104);
newLeafNode(lv_hint_6_0, grammarAccess.getConsumerAccess().getHintSTRINGTerminalRuleCall_4_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getConsumerRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_6_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
// InternalContextMappingDSL.g:6195:3: ( (lv_dependencies_7_0= ruleDependency ) )*
loop177:
do {
int alt177=2;
int LA177_0 = input.LA(1);
if ( (LA177_0==119||LA177_0==182) ) {
alt177=1;
}
switch (alt177) {
case 1 :
// InternalContextMappingDSL.g:6196:4: (lv_dependencies_7_0= ruleDependency )
{
// InternalContextMappingDSL.g:6196:4: (lv_dependencies_7_0= ruleDependency )
// InternalContextMappingDSL.g:6197:5: lv_dependencies_7_0= ruleDependency
{
newCompositeNode(grammarAccess.getConsumerAccess().getDependenciesDependencyParserRuleCall_5_0());
pushFollow(FOLLOW_104);
lv_dependencies_7_0=ruleDependency();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getConsumerRule());
}
add(
current,
"dependencies",
lv_dependencies_7_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Dependency");
afterParserOrEnumRuleCall();
}
}
break;
default :
break loop177;
}
} while (true);
// InternalContextMappingDSL.g:6214:3: (otherlv_8= 'unmarshall to' (otherlv_9= '@' )? ( (otherlv_10= RULE_ID ) ) )?
int alt179=2;
int LA179_0 = input.LA(1);
if ( (LA179_0==93) ) {
alt179=1;
}
switch (alt179) {
case 1 :
// InternalContextMappingDSL.g:6215:4: otherlv_8= 'unmarshall to' (otherlv_9= '@' )? ( (otherlv_10= RULE_ID ) )
{
otherlv_8=(Token)match(input,93,FOLLOW_105);
newLeafNode(otherlv_8, grammarAccess.getConsumerAccess().getUnmarshallToKeyword_6_0());
// InternalContextMappingDSL.g:6219:4: (otherlv_9= '@' )?
int alt178=2;
int LA178_0 = input.LA(1);
if ( (LA178_0==94) ) {
alt178=1;
}
switch (alt178) {
case 1 :
// InternalContextMappingDSL.g:6220:5: otherlv_9= '@'
{
otherlv_9=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_9, grammarAccess.getConsumerAccess().getCommercialAtKeyword_6_1());
}
break;
}
// InternalContextMappingDSL.g:6225:4: ( (otherlv_10= RULE_ID ) )
// InternalContextMappingDSL.g:6226:5: (otherlv_10= RULE_ID )
{
// InternalContextMappingDSL.g:6226:5: (otherlv_10= RULE_ID )
// InternalContextMappingDSL.g:6227:6: otherlv_10= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getConsumerRule());
}
otherlv_10=(Token)match(input,RULE_ID,FOLLOW_106);
newLeafNode(otherlv_10, grammarAccess.getConsumerAccess().getMessageRootDomainObjectCrossReference_6_2_0());
}
}
}
break;
}
// InternalContextMappingDSL.g:6239:3: ( ( ( ( ({...}? => ( ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:6240:4: ( ( ( ({...}? => ( ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:6240:4: ( ( ( ({...}? => ( ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:6241:5: ( ( ({...}? => ( ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getConsumerAccess().getUnorderedGroup_7());
// InternalContextMappingDSL.g:6244:5: ( ( ({...}? => ( ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) ) ) ) )* )
// InternalContextMappingDSL.g:6245:6: ( ({...}? => ( ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:6245:6: ( ({...}? => ( ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) ) ) ) )*
loop181:
do {
int alt181=3;
int LA181_0 = input.LA(1);
if ( LA181_0 >= 95 && LA181_0 <= 96 && getUnorderedGroupHelper().canSelect(grammarAccess.getConsumerAccess().getUnorderedGroup_7(), 0) ) {
alt181=1;
}
else if ( LA181_0 == 97 && getUnorderedGroupHelper().canSelect(grammarAccess.getConsumerAccess().getUnorderedGroup_7(), 1) ) {
alt181=2;
}
switch (alt181) {
case 1 :
// InternalContextMappingDSL.g:6246:4: ({...}? => ( ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) ) ) )
{
// InternalContextMappingDSL.g:6246:4: ({...}? => ( ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) ) ) )
// InternalContextMappingDSL.g:6247:5: {...}? => ( ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getConsumerAccess().getUnorderedGroup_7(), 0) ) {
throw new FailedPredicateException(input, "ruleConsumer", "getUnorderedGroupHelper().canSelect(grammarAccess.getConsumerAccess().getUnorderedGroup_7(), 0)");
}
// InternalContextMappingDSL.g:6247:105: ( ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) ) )
// InternalContextMappingDSL.g:6248:6: ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getConsumerAccess().getUnorderedGroup_7(), 0);
// InternalContextMappingDSL.g:6251:9: ({...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) ) )
// InternalContextMappingDSL.g:6251:10: {...}? => ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleConsumer", "true");
}
// InternalContextMappingDSL.g:6251:19: ( (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) ) )
// InternalContextMappingDSL.g:6251:20: (otherlv_12= 'queueName' | otherlv_13= 'topicName' ) otherlv_14= '=' ( (lv_channel_15_0= ruleChannelIdentifier ) )
{
// InternalContextMappingDSL.g:6251:20: (otherlv_12= 'queueName' | otherlv_13= 'topicName' )
int alt180=2;
int LA180_0 = input.LA(1);
if ( (LA180_0==95) ) {
alt180=1;
}
else if ( (LA180_0==96) ) {
alt180=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 180, 0, input);
throw nvae;
}
switch (alt180) {
case 1 :
// InternalContextMappingDSL.g:6252:10: otherlv_12= 'queueName'
{
otherlv_12=(Token)match(input,95,FOLLOW_89);
newLeafNode(otherlv_12, grammarAccess.getConsumerAccess().getQueueNameKeyword_7_0_0_0());
}
break;
case 2 :
// InternalContextMappingDSL.g:6257:10: otherlv_13= 'topicName'
{
otherlv_13=(Token)match(input,96,FOLLOW_89);
newLeafNode(otherlv_13, grammarAccess.getConsumerAccess().getTopicNameKeyword_7_0_0_1());
}
break;
}
otherlv_14=(Token)match(input,21,FOLLOW_10);
newLeafNode(otherlv_14, grammarAccess.getConsumerAccess().getEqualsSignKeyword_7_0_1());
// InternalContextMappingDSL.g:6266:9: ( (lv_channel_15_0= ruleChannelIdentifier ) )
// InternalContextMappingDSL.g:6267:10: (lv_channel_15_0= ruleChannelIdentifier )
{
// InternalContextMappingDSL.g:6267:10: (lv_channel_15_0= ruleChannelIdentifier )
// InternalContextMappingDSL.g:6268:11: lv_channel_15_0= ruleChannelIdentifier
{
newCompositeNode(grammarAccess.getConsumerAccess().getChannelChannelIdentifierParserRuleCall_7_0_2_0());
pushFollow(FOLLOW_106);
lv_channel_15_0=ruleChannelIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getConsumerRule());
}
set(
current,
"channel",
lv_channel_15_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.ChannelIdentifier");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getConsumerAccess().getUnorderedGroup_7());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:6291:4: ({...}? => ( ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) ) ) )
{
// InternalContextMappingDSL.g:6291:4: ({...}? => ( ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) ) ) )
// InternalContextMappingDSL.g:6292:5: {...}? => ( ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getConsumerAccess().getUnorderedGroup_7(), 1) ) {
throw new FailedPredicateException(input, "ruleConsumer", "getUnorderedGroupHelper().canSelect(grammarAccess.getConsumerAccess().getUnorderedGroup_7(), 1)");
}
// InternalContextMappingDSL.g:6292:105: ( ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) ) )
// InternalContextMappingDSL.g:6293:6: ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getConsumerAccess().getUnorderedGroup_7(), 1);
// InternalContextMappingDSL.g:6296:9: ({...}? => ( (lv_subscribe_16_0= ruleSubscribe ) ) )
// InternalContextMappingDSL.g:6296:10: {...}? => ( (lv_subscribe_16_0= ruleSubscribe ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleConsumer", "true");
}
// InternalContextMappingDSL.g:6296:19: ( (lv_subscribe_16_0= ruleSubscribe ) )
// InternalContextMappingDSL.g:6296:20: (lv_subscribe_16_0= ruleSubscribe )
{
// InternalContextMappingDSL.g:6296:20: (lv_subscribe_16_0= ruleSubscribe )
// InternalContextMappingDSL.g:6297:10: lv_subscribe_16_0= ruleSubscribe
{
newCompositeNode(grammarAccess.getConsumerAccess().getSubscribeSubscribeParserRuleCall_7_1_0());
pushFollow(FOLLOW_106);
lv_subscribe_16_0=ruleSubscribe();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getConsumerRule());
}
set(
current,
"subscribe",
lv_subscribe_16_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Subscribe");
afterParserOrEnumRuleCall();
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getConsumerAccess().getUnorderedGroup_7());
}
}
}
break;
default :
break loop181;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getConsumerAccess().getUnorderedGroup_7());
}
otherlv_17=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(otherlv_17, grammarAccess.getConsumerAccess().getRightCurlyBracketKeyword_8());
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleConsumer"
// $ANTLR start "entryRuleSubscribe"
// InternalContextMappingDSL.g:6334:1: entryRuleSubscribe returns [EObject current=null] : iv_ruleSubscribe= ruleSubscribe EOF ;
public final EObject entryRuleSubscribe() throws RecognitionException {
EObject current = null;
EObject iv_ruleSubscribe = null;
try {
// InternalContextMappingDSL.g:6334:50: (iv_ruleSubscribe= ruleSubscribe EOF )
// InternalContextMappingDSL.g:6335:2: iv_ruleSubscribe= ruleSubscribe EOF
{
newCompositeNode(grammarAccess.getSubscribeRule());
pushFollow(FOLLOW_1);
iv_ruleSubscribe=ruleSubscribe();
state._fsp--;
current =iv_ruleSubscribe;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleSubscribe"
// $ANTLR start "ruleSubscribe"
// InternalContextMappingDSL.g:6341:1: ruleSubscribe returns [EObject current=null] : (otherlv_0= 'subscribe' otherlv_1= 'to' ( (lv_topic_2_0= ruleChannelIdentifier ) ) (otherlv_3= 'eventBus' otherlv_4= '=' ( (lv_eventBus_5_0= RULE_ID ) ) )? ) ;
public final EObject ruleSubscribe() throws RecognitionException {
EObject current = null;
Token otherlv_0=null;
Token otherlv_1=null;
Token otherlv_3=null;
Token otherlv_4=null;
Token lv_eventBus_5_0=null;
AntlrDatatypeRuleToken lv_topic_2_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:6347:2: ( (otherlv_0= 'subscribe' otherlv_1= 'to' ( (lv_topic_2_0= ruleChannelIdentifier ) ) (otherlv_3= 'eventBus' otherlv_4= '=' ( (lv_eventBus_5_0= RULE_ID ) ) )? ) )
// InternalContextMappingDSL.g:6348:2: (otherlv_0= 'subscribe' otherlv_1= 'to' ( (lv_topic_2_0= ruleChannelIdentifier ) ) (otherlv_3= 'eventBus' otherlv_4= '=' ( (lv_eventBus_5_0= RULE_ID ) ) )? )
{
// InternalContextMappingDSL.g:6348:2: (otherlv_0= 'subscribe' otherlv_1= 'to' ( (lv_topic_2_0= ruleChannelIdentifier ) ) (otherlv_3= 'eventBus' otherlv_4= '=' ( (lv_eventBus_5_0= RULE_ID ) ) )? )
// InternalContextMappingDSL.g:6349:3: otherlv_0= 'subscribe' otherlv_1= 'to' ( (lv_topic_2_0= ruleChannelIdentifier ) ) (otherlv_3= 'eventBus' otherlv_4= '=' ( (lv_eventBus_5_0= RULE_ID ) ) )?
{
otherlv_0=(Token)match(input,97,FOLLOW_107);
newLeafNode(otherlv_0, grammarAccess.getSubscribeAccess().getSubscribeKeyword_0());
otherlv_1=(Token)match(input,98,FOLLOW_10);
newLeafNode(otherlv_1, grammarAccess.getSubscribeAccess().getToKeyword_1());
// InternalContextMappingDSL.g:6357:3: ( (lv_topic_2_0= ruleChannelIdentifier ) )
// InternalContextMappingDSL.g:6358:4: (lv_topic_2_0= ruleChannelIdentifier )
{
// InternalContextMappingDSL.g:6358:4: (lv_topic_2_0= ruleChannelIdentifier )
// InternalContextMappingDSL.g:6359:5: lv_topic_2_0= ruleChannelIdentifier
{
newCompositeNode(grammarAccess.getSubscribeAccess().getTopicChannelIdentifierParserRuleCall_2_0());
pushFollow(FOLLOW_108);
lv_topic_2_0=ruleChannelIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getSubscribeRule());
}
set(
current,
"topic",
lv_topic_2_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.ChannelIdentifier");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:6376:3: (otherlv_3= 'eventBus' otherlv_4= '=' ( (lv_eventBus_5_0= RULE_ID ) ) )?
int alt182=2;
int LA182_0 = input.LA(1);
if ( (LA182_0==99) ) {
alt182=1;
}
switch (alt182) {
case 1 :
// InternalContextMappingDSL.g:6377:4: otherlv_3= 'eventBus' otherlv_4= '=' ( (lv_eventBus_5_0= RULE_ID ) )
{
otherlv_3=(Token)match(input,99,FOLLOW_89);
newLeafNode(otherlv_3, grammarAccess.getSubscribeAccess().getEventBusKeyword_3_0());
otherlv_4=(Token)match(input,21,FOLLOW_10);
newLeafNode(otherlv_4, grammarAccess.getSubscribeAccess().getEqualsSignKeyword_3_1());
// InternalContextMappingDSL.g:6385:4: ( (lv_eventBus_5_0= RULE_ID ) )
// InternalContextMappingDSL.g:6386:5: (lv_eventBus_5_0= RULE_ID )
{
// InternalContextMappingDSL.g:6386:5: (lv_eventBus_5_0= RULE_ID )
// InternalContextMappingDSL.g:6387:6: lv_eventBus_5_0= RULE_ID
{
lv_eventBus_5_0=(Token)match(input,RULE_ID,FOLLOW_2);
newLeafNode(lv_eventBus_5_0, grammarAccess.getSubscribeAccess().getEventBusIDTerminalRuleCall_3_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getSubscribeRule());
}
setWithLastConsumed(
current,
"eventBus",
lv_eventBus_5_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleSubscribe"
// $ANTLR start "entryRulePublish"
// InternalContextMappingDSL.g:6408:1: entryRulePublish returns [EObject current=null] : iv_rulePublish= rulePublish EOF ;
public final EObject entryRulePublish() throws RecognitionException {
EObject current = null;
EObject iv_rulePublish = null;
try {
// InternalContextMappingDSL.g:6408:48: (iv_rulePublish= rulePublish EOF )
// InternalContextMappingDSL.g:6409:2: iv_rulePublish= rulePublish EOF
{
newCompositeNode(grammarAccess.getPublishRule());
pushFollow(FOLLOW_1);
iv_rulePublish=rulePublish();
state._fsp--;
current =iv_rulePublish;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRulePublish"
// $ANTLR start "rulePublish"
// InternalContextMappingDSL.g:6415:1: rulePublish returns [EObject current=null] : (otherlv_0= 'publish' ( (otherlv_1= '@' )? ( (otherlv_2= RULE_ID ) ) )? otherlv_3= 'to' ( (lv_topic_4_0= ruleChannelIdentifier ) ) (otherlv_5= 'eventBus' otherlv_6= '=' ( (lv_eventBus_7_0= RULE_ID ) ) )? ) ;
public final EObject rulePublish() throws RecognitionException {
EObject current = null;
Token otherlv_0=null;
Token otherlv_1=null;
Token otherlv_2=null;
Token otherlv_3=null;
Token otherlv_5=null;
Token otherlv_6=null;
Token lv_eventBus_7_0=null;
AntlrDatatypeRuleToken lv_topic_4_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:6421:2: ( (otherlv_0= 'publish' ( (otherlv_1= '@' )? ( (otherlv_2= RULE_ID ) ) )? otherlv_3= 'to' ( (lv_topic_4_0= ruleChannelIdentifier ) ) (otherlv_5= 'eventBus' otherlv_6= '=' ( (lv_eventBus_7_0= RULE_ID ) ) )? ) )
// InternalContextMappingDSL.g:6422:2: (otherlv_0= 'publish' ( (otherlv_1= '@' )? ( (otherlv_2= RULE_ID ) ) )? otherlv_3= 'to' ( (lv_topic_4_0= ruleChannelIdentifier ) ) (otherlv_5= 'eventBus' otherlv_6= '=' ( (lv_eventBus_7_0= RULE_ID ) ) )? )
{
// InternalContextMappingDSL.g:6422:2: (otherlv_0= 'publish' ( (otherlv_1= '@' )? ( (otherlv_2= RULE_ID ) ) )? otherlv_3= 'to' ( (lv_topic_4_0= ruleChannelIdentifier ) ) (otherlv_5= 'eventBus' otherlv_6= '=' ( (lv_eventBus_7_0= RULE_ID ) ) )? )
// InternalContextMappingDSL.g:6423:3: otherlv_0= 'publish' ( (otherlv_1= '@' )? ( (otherlv_2= RULE_ID ) ) )? otherlv_3= 'to' ( (lv_topic_4_0= ruleChannelIdentifier ) ) (otherlv_5= 'eventBus' otherlv_6= '=' ( (lv_eventBus_7_0= RULE_ID ) ) )?
{
otherlv_0=(Token)match(input,100,FOLLOW_109);
newLeafNode(otherlv_0, grammarAccess.getPublishAccess().getPublishKeyword_0());
// InternalContextMappingDSL.g:6427:3: ( (otherlv_1= '@' )? ( (otherlv_2= RULE_ID ) ) )?
int alt184=2;
int LA184_0 = input.LA(1);
if ( (LA184_0==RULE_ID||LA184_0==94) ) {
alt184=1;
}
switch (alt184) {
case 1 :
// InternalContextMappingDSL.g:6428:4: (otherlv_1= '@' )? ( (otherlv_2= RULE_ID ) )
{
// InternalContextMappingDSL.g:6428:4: (otherlv_1= '@' )?
int alt183=2;
int LA183_0 = input.LA(1);
if ( (LA183_0==94) ) {
alt183=1;
}
switch (alt183) {
case 1 :
// InternalContextMappingDSL.g:6429:5: otherlv_1= '@'
{
otherlv_1=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_1, grammarAccess.getPublishAccess().getCommercialAtKeyword_1_0());
}
break;
}
// InternalContextMappingDSL.g:6434:4: ( (otherlv_2= RULE_ID ) )
// InternalContextMappingDSL.g:6435:5: (otherlv_2= RULE_ID )
{
// InternalContextMappingDSL.g:6435:5: (otherlv_2= RULE_ID )
// InternalContextMappingDSL.g:6436:6: otherlv_2= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getPublishRule());
}
otherlv_2=(Token)match(input,RULE_ID,FOLLOW_107);
newLeafNode(otherlv_2, grammarAccess.getPublishAccess().getEventTypeEventCrossReference_1_1_0());
}
}
}
break;
}
otherlv_3=(Token)match(input,98,FOLLOW_10);
newLeafNode(otherlv_3, grammarAccess.getPublishAccess().getToKeyword_2());
// InternalContextMappingDSL.g:6452:3: ( (lv_topic_4_0= ruleChannelIdentifier ) )
// InternalContextMappingDSL.g:6453:4: (lv_topic_4_0= ruleChannelIdentifier )
{
// InternalContextMappingDSL.g:6453:4: (lv_topic_4_0= ruleChannelIdentifier )
// InternalContextMappingDSL.g:6454:5: lv_topic_4_0= ruleChannelIdentifier
{
newCompositeNode(grammarAccess.getPublishAccess().getTopicChannelIdentifierParserRuleCall_3_0());
pushFollow(FOLLOW_108);
lv_topic_4_0=ruleChannelIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getPublishRule());
}
set(
current,
"topic",
lv_topic_4_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.ChannelIdentifier");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:6471:3: (otherlv_5= 'eventBus' otherlv_6= '=' ( (lv_eventBus_7_0= RULE_ID ) ) )?
int alt185=2;
int LA185_0 = input.LA(1);
if ( (LA185_0==99) ) {
alt185=1;
}
switch (alt185) {
case 1 :
// InternalContextMappingDSL.g:6472:4: otherlv_5= 'eventBus' otherlv_6= '=' ( (lv_eventBus_7_0= RULE_ID ) )
{
otherlv_5=(Token)match(input,99,FOLLOW_89);
newLeafNode(otherlv_5, grammarAccess.getPublishAccess().getEventBusKeyword_4_0());
otherlv_6=(Token)match(input,21,FOLLOW_10);
newLeafNode(otherlv_6, grammarAccess.getPublishAccess().getEqualsSignKeyword_4_1());
// InternalContextMappingDSL.g:6480:4: ( (lv_eventBus_7_0= RULE_ID ) )
// InternalContextMappingDSL.g:6481:5: (lv_eventBus_7_0= RULE_ID )
{
// InternalContextMappingDSL.g:6481:5: (lv_eventBus_7_0= RULE_ID )
// InternalContextMappingDSL.g:6482:6: lv_eventBus_7_0= RULE_ID
{
lv_eventBus_7_0=(Token)match(input,RULE_ID,FOLLOW_2);
newLeafNode(lv_eventBus_7_0, grammarAccess.getPublishAccess().getEventBusIDTerminalRuleCall_4_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getPublishRule());
}
setWithLastConsumed(
current,
"eventBus",
lv_eventBus_7_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "rulePublish"
// $ANTLR start "entryRuleEvent"
// InternalContextMappingDSL.g:6503:1: entryRuleEvent returns [EObject current=null] : iv_ruleEvent= ruleEvent EOF ;
public final EObject entryRuleEvent() throws RecognitionException {
EObject current = null;
EObject iv_ruleEvent = null;
try {
// InternalContextMappingDSL.g:6503:46: (iv_ruleEvent= ruleEvent EOF )
// InternalContextMappingDSL.g:6504:2: iv_ruleEvent= ruleEvent EOF
{
newCompositeNode(grammarAccess.getEventRule());
pushFollow(FOLLOW_1);
iv_ruleEvent=ruleEvent();
state._fsp--;
current =iv_ruleEvent;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleEvent"
// $ANTLR start "ruleEvent"
// InternalContextMappingDSL.g:6510:1: ruleEvent returns [EObject current=null] : (this_DomainEvent_0= ruleDomainEvent | this_CommandEvent_1= ruleCommandEvent ) ;
public final EObject ruleEvent() throws RecognitionException {
EObject current = null;
EObject this_DomainEvent_0 = null;
EObject this_CommandEvent_1 = null;
enterRule();
try {
// InternalContextMappingDSL.g:6516:2: ( (this_DomainEvent_0= ruleDomainEvent | this_CommandEvent_1= ruleCommandEvent ) )
// InternalContextMappingDSL.g:6517:2: (this_DomainEvent_0= ruleDomainEvent | this_CommandEvent_1= ruleCommandEvent )
{
// InternalContextMappingDSL.g:6517:2: (this_DomainEvent_0= ruleDomainEvent | this_CommandEvent_1= ruleCommandEvent )
int alt186=2;
switch ( input.LA(1) ) {
case RULE_ML_COMMENT:
{
switch ( input.LA(2) ) {
case RULE_STRING:
{
switch ( input.LA(3) ) {
case 120:
{
int LA186_3 = input.LA(4);
if ( (LA186_3==139) ) {
alt186=1;
}
else if ( (LA186_3==140) ) {
alt186=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 186, 3, input);
throw nvae;
}
}
break;
case 139:
{
alt186=1;
}
break;
case 140:
{
alt186=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 186, 2, input);
throw nvae;
}
}
break;
case 120:
{
int LA186_3 = input.LA(3);
if ( (LA186_3==139) ) {
alt186=1;
}
else if ( (LA186_3==140) ) {
alt186=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 186, 3, input);
throw nvae;
}
}
break;
case 139:
{
alt186=1;
}
break;
case 140:
{
alt186=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 186, 1, input);
throw nvae;
}
}
break;
case RULE_STRING:
{
switch ( input.LA(2) ) {
case 120:
{
int LA186_3 = input.LA(3);
if ( (LA186_3==139) ) {
alt186=1;
}
else if ( (LA186_3==140) ) {
alt186=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 186, 3, input);
throw nvae;
}
}
break;
case 139:
{
alt186=1;
}
break;
case 140:
{
alt186=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 186, 2, input);
throw nvae;
}
}
break;
case 120:
{
int LA186_3 = input.LA(2);
if ( (LA186_3==139) ) {
alt186=1;
}
else if ( (LA186_3==140) ) {
alt186=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 186, 3, input);
throw nvae;
}
}
break;
case 139:
{
alt186=1;
}
break;
case 140:
{
alt186=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 186, 0, input);
throw nvae;
}
switch (alt186) {
case 1 :
// InternalContextMappingDSL.g:6518:3: this_DomainEvent_0= ruleDomainEvent
{
newCompositeNode(grammarAccess.getEventAccess().getDomainEventParserRuleCall_0());
pushFollow(FOLLOW_2);
this_DomainEvent_0=ruleDomainEvent();
state._fsp--;
current = this_DomainEvent_0;
afterParserOrEnumRuleCall();
}
break;
case 2 :
// InternalContextMappingDSL.g:6527:3: this_CommandEvent_1= ruleCommandEvent
{
newCompositeNode(grammarAccess.getEventAccess().getCommandEventParserRuleCall_1());
pushFollow(FOLLOW_2);
this_CommandEvent_1=ruleCommandEvent();
state._fsp--;
current = this_CommandEvent_1;
afterParserOrEnumRuleCall();
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleEvent"
// $ANTLR start "entryRuleServiceOperation"
// InternalContextMappingDSL.g:6539:1: entryRuleServiceOperation returns [EObject current=null] : iv_ruleServiceOperation= ruleServiceOperation EOF ;
public final EObject entryRuleServiceOperation() throws RecognitionException {
EObject current = null;
EObject iv_ruleServiceOperation = null;
try {
// InternalContextMappingDSL.g:6539:57: (iv_ruleServiceOperation= ruleServiceOperation EOF )
// InternalContextMappingDSL.g:6540:2: iv_ruleServiceOperation= ruleServiceOperation EOF
{
newCompositeNode(grammarAccess.getServiceOperationRule());
pushFollow(FOLLOW_1);
iv_ruleServiceOperation=ruleServiceOperation();
state._fsp--;
current =iv_ruleServiceOperation;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleServiceOperation"
// $ANTLR start "ruleServiceOperation"
// InternalContextMappingDSL.g:6546:1: ruleServiceOperation returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( (lv_returnType_2_0= ruleComplexType ) ) | otherlv_3= 'void' )? ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= '(' ( (lv_parameters_6_0= ruleParameter ) )? (otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) ) )* otherlv_9= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_16_0= rulePublish ) ) ) ) ) )* ) ) ) ( (lv_delegateHolder_17_0= ruleServiceOperationDelegate ) )? otherlv_18= ';' ) ;
public final EObject ruleServiceOperation() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token otherlv_3=null;
Token lv_name_4_0=null;
Token otherlv_5=null;
Token otherlv_7=null;
Token otherlv_9=null;
Token otherlv_11=null;
Token otherlv_13=null;
Token otherlv_14=null;
Token lv_hint_15_0=null;
Token otherlv_18=null;
Enumerator lv_visibility_1_0 = null;
EObject lv_returnType_2_0 = null;
EObject lv_parameters_6_0 = null;
EObject lv_parameters_8_0 = null;
AntlrDatatypeRuleToken lv_throws_12_0 = null;
EObject lv_publish_16_0 = null;
EObject lv_delegateHolder_17_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:6552:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( (lv_returnType_2_0= ruleComplexType ) ) | otherlv_3= 'void' )? ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= '(' ( (lv_parameters_6_0= ruleParameter ) )? (otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) ) )* otherlv_9= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_16_0= rulePublish ) ) ) ) ) )* ) ) ) ( (lv_delegateHolder_17_0= ruleServiceOperationDelegate ) )? otherlv_18= ';' ) )
// InternalContextMappingDSL.g:6553:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( (lv_returnType_2_0= ruleComplexType ) ) | otherlv_3= 'void' )? ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= '(' ( (lv_parameters_6_0= ruleParameter ) )? (otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) ) )* otherlv_9= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_16_0= rulePublish ) ) ) ) ) )* ) ) ) ( (lv_delegateHolder_17_0= ruleServiceOperationDelegate ) )? otherlv_18= ';' )
{
// InternalContextMappingDSL.g:6553:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( (lv_returnType_2_0= ruleComplexType ) ) | otherlv_3= 'void' )? ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= '(' ( (lv_parameters_6_0= ruleParameter ) )? (otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) ) )* otherlv_9= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_16_0= rulePublish ) ) ) ) ) )* ) ) ) ( (lv_delegateHolder_17_0= ruleServiceOperationDelegate ) )? otherlv_18= ';' )
// InternalContextMappingDSL.g:6554:3: ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( (lv_returnType_2_0= ruleComplexType ) ) | otherlv_3= 'void' )? ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= '(' ( (lv_parameters_6_0= ruleParameter ) )? (otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) ) )* otherlv_9= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_16_0= rulePublish ) ) ) ) ) )* ) ) ) ( (lv_delegateHolder_17_0= ruleServiceOperationDelegate ) )? otherlv_18= ';'
{
// InternalContextMappingDSL.g:6554:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt187=2;
int LA187_0 = input.LA(1);
if ( (LA187_0==RULE_STRING) ) {
alt187=1;
}
switch (alt187) {
case 1 :
// InternalContextMappingDSL.g:6555:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:6555:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:6556:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_110);
newLeafNode(lv_doc_0_0, grammarAccess.getServiceOperationAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getServiceOperationRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
// InternalContextMappingDSL.g:6572:3: ( (lv_visibility_1_0= ruleVisibility ) )?
int alt188=2;
int LA188_0 = input.LA(1);
if ( (LA188_0==124||(LA188_0>=247 && LA188_0<=249)) ) {
alt188=1;
}
switch (alt188) {
case 1 :
// InternalContextMappingDSL.g:6573:4: (lv_visibility_1_0= ruleVisibility )
{
// InternalContextMappingDSL.g:6573:4: (lv_visibility_1_0= ruleVisibility )
// InternalContextMappingDSL.g:6574:5: lv_visibility_1_0= ruleVisibility
{
newCompositeNode(grammarAccess.getServiceOperationAccess().getVisibilityVisibilityEnumRuleCall_1_0());
pushFollow(FOLLOW_111);
lv_visibility_1_0=ruleVisibility();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getServiceOperationRule());
}
set(
current,
"visibility",
lv_visibility_1_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Visibility");
afterParserOrEnumRuleCall();
}
}
break;
}
// InternalContextMappingDSL.g:6591:3: ( ( (lv_returnType_2_0= ruleComplexType ) ) | otherlv_3= 'void' )?
int alt189=3;
switch ( input.LA(1) ) {
case RULE_MAP_COLLECTION_TYPE:
case 94:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
{
alt189=1;
}
break;
case RULE_ID:
{
int LA189_2 = input.LA(2);
if ( (LA189_2==RULE_ID||LA189_2==106||LA189_2==118) ) {
alt189=1;
}
}
break;
case 101:
{
alt189=2;
}
break;
}
switch (alt189) {
case 1 :
// InternalContextMappingDSL.g:6592:4: ( (lv_returnType_2_0= ruleComplexType ) )
{
// InternalContextMappingDSL.g:6592:4: ( (lv_returnType_2_0= ruleComplexType ) )
// InternalContextMappingDSL.g:6593:5: (lv_returnType_2_0= ruleComplexType )
{
// InternalContextMappingDSL.g:6593:5: (lv_returnType_2_0= ruleComplexType )
// InternalContextMappingDSL.g:6594:6: lv_returnType_2_0= ruleComplexType
{
newCompositeNode(grammarAccess.getServiceOperationAccess().getReturnTypeComplexTypeParserRuleCall_2_0_0());
pushFollow(FOLLOW_10);
lv_returnType_2_0=ruleComplexType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getServiceOperationRule());
}
set(
current,
"returnType",
lv_returnType_2_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.ComplexType");
afterParserOrEnumRuleCall();
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:6612:4: otherlv_3= 'void'
{
otherlv_3=(Token)match(input,101,FOLLOW_10);
newLeafNode(otherlv_3, grammarAccess.getServiceOperationAccess().getVoidKeyword_2_1());
}
break;
}
// InternalContextMappingDSL.g:6617:3: ( (lv_name_4_0= RULE_ID ) )
// InternalContextMappingDSL.g:6618:4: (lv_name_4_0= RULE_ID )
{
// InternalContextMappingDSL.g:6618:4: (lv_name_4_0= RULE_ID )
// InternalContextMappingDSL.g:6619:5: lv_name_4_0= RULE_ID
{
lv_name_4_0=(Token)match(input,RULE_ID,FOLLOW_112);
newLeafNode(lv_name_4_0, grammarAccess.getServiceOperationAccess().getNameIDTerminalRuleCall_3_0());
if (current==null) {
current = createModelElement(grammarAccess.getServiceOperationRule());
}
setWithLastConsumed(
current,
"name",
lv_name_4_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:6635:3: (otherlv_5= '(' ( (lv_parameters_6_0= ruleParameter ) )? (otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) ) )* otherlv_9= ')' )?
int alt192=2;
int LA192_0 = input.LA(1);
if ( (LA192_0==102) ) {
alt192=1;
}
switch (alt192) {
case 1 :
// InternalContextMappingDSL.g:6636:4: otherlv_5= '(' ( (lv_parameters_6_0= ruleParameter ) )? (otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) ) )* otherlv_9= ')'
{
otherlv_5=(Token)match(input,102,FOLLOW_113);
newLeafNode(otherlv_5, grammarAccess.getServiceOperationAccess().getLeftParenthesisKeyword_4_0());
// InternalContextMappingDSL.g:6640:4: ( (lv_parameters_6_0= ruleParameter ) )?
int alt190=2;
int LA190_0 = input.LA(1);
if ( ((LA190_0>=RULE_STRING && LA190_0<=RULE_ID)||LA190_0==RULE_MAP_COLLECTION_TYPE||LA190_0==94||(LA190_0>=185 && LA190_0<=206)||LA190_0==233||(LA190_0>=243 && LA190_0<=246)) ) {
alt190=1;
}
switch (alt190) {
case 1 :
// InternalContextMappingDSL.g:6641:5: (lv_parameters_6_0= ruleParameter )
{
// InternalContextMappingDSL.g:6641:5: (lv_parameters_6_0= ruleParameter )
// InternalContextMappingDSL.g:6642:6: lv_parameters_6_0= ruleParameter
{
newCompositeNode(grammarAccess.getServiceOperationAccess().getParametersParameterParserRuleCall_4_1_0());
pushFollow(FOLLOW_114);
lv_parameters_6_0=ruleParameter();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getServiceOperationRule());
}
add(
current,
"parameters",
lv_parameters_6_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Parameter");
afterParserOrEnumRuleCall();
}
}
break;
}
// InternalContextMappingDSL.g:6659:4: (otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) ) )*
loop191:
do {
int alt191=2;
int LA191_0 = input.LA(1);
if ( (LA191_0==24) ) {
alt191=1;
}
switch (alt191) {
case 1 :
// InternalContextMappingDSL.g:6660:5: otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) )
{
otherlv_7=(Token)match(input,24,FOLLOW_115);
newLeafNode(otherlv_7, grammarAccess.getServiceOperationAccess().getCommaKeyword_4_2_0());
// InternalContextMappingDSL.g:6664:5: ( (lv_parameters_8_0= ruleParameter ) )
// InternalContextMappingDSL.g:6665:6: (lv_parameters_8_0= ruleParameter )
{
// InternalContextMappingDSL.g:6665:6: (lv_parameters_8_0= ruleParameter )
// InternalContextMappingDSL.g:6666:7: lv_parameters_8_0= ruleParameter
{
newCompositeNode(grammarAccess.getServiceOperationAccess().getParametersParameterParserRuleCall_4_2_1_0());
pushFollow(FOLLOW_114);
lv_parameters_8_0=ruleParameter();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getServiceOperationRule());
}
add(
current,
"parameters",
lv_parameters_8_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Parameter");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop191;
}
} while (true);
otherlv_9=(Token)match(input,103,FOLLOW_116);
newLeafNode(otherlv_9, grammarAccess.getServiceOperationAccess().getRightParenthesisKeyword_4_3());
}
break;
}
// InternalContextMappingDSL.g:6689:3: ( ( ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_16_0= rulePublish ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:6690:4: ( ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_16_0= rulePublish ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:6690:4: ( ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_16_0= rulePublish ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:6691:5: ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_16_0= rulePublish ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getServiceOperationAccess().getUnorderedGroup_5());
// InternalContextMappingDSL.g:6694:5: ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_16_0= rulePublish ) ) ) ) ) )* )
// InternalContextMappingDSL.g:6695:6: ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_16_0= rulePublish ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:6695:6: ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_16_0= rulePublish ) ) ) ) ) )*
loop193:
do {
int alt193=4;
int LA193_0 = input.LA(1);
if ( LA193_0 == 104 && getUnorderedGroupHelper().canSelect(grammarAccess.getServiceOperationAccess().getUnorderedGroup_5(), 0) ) {
alt193=1;
}
else if ( LA193_0 == 82 && getUnorderedGroupHelper().canSelect(grammarAccess.getServiceOperationAccess().getUnorderedGroup_5(), 1) ) {
alt193=2;
}
else if ( LA193_0 == 100 && getUnorderedGroupHelper().canSelect(grammarAccess.getServiceOperationAccess().getUnorderedGroup_5(), 2) ) {
alt193=3;
}
switch (alt193) {
case 1 :
// InternalContextMappingDSL.g:6696:4: ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) )
{
// InternalContextMappingDSL.g:6696:4: ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) )
// InternalContextMappingDSL.g:6697:5: {...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getServiceOperationAccess().getUnorderedGroup_5(), 0) ) {
throw new FailedPredicateException(input, "ruleServiceOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getServiceOperationAccess().getUnorderedGroup_5(), 0)");
}
// InternalContextMappingDSL.g:6697:113: ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) )
// InternalContextMappingDSL.g:6698:6: ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getServiceOperationAccess().getUnorderedGroup_5(), 0);
// InternalContextMappingDSL.g:6701:9: ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) )
// InternalContextMappingDSL.g:6701:10: {...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleServiceOperation", "true");
}
// InternalContextMappingDSL.g:6701:19: (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) )
// InternalContextMappingDSL.g:6701:20: otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) )
{
otherlv_11=(Token)match(input,104,FOLLOW_10);
newLeafNode(otherlv_11, grammarAccess.getServiceOperationAccess().getThrowsKeyword_5_0_0());
// InternalContextMappingDSL.g:6705:9: ( (lv_throws_12_0= ruleThrowsIdentifier ) )
// InternalContextMappingDSL.g:6706:10: (lv_throws_12_0= ruleThrowsIdentifier )
{
// InternalContextMappingDSL.g:6706:10: (lv_throws_12_0= ruleThrowsIdentifier )
// InternalContextMappingDSL.g:6707:11: lv_throws_12_0= ruleThrowsIdentifier
{
newCompositeNode(grammarAccess.getServiceOperationAccess().getThrowsThrowsIdentifierParserRuleCall_5_0_1_0());
pushFollow(FOLLOW_116);
lv_throws_12_0=ruleThrowsIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getServiceOperationRule());
}
set(
current,
"throws",
lv_throws_12_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.ThrowsIdentifier");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getServiceOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:6730:4: ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:6730:4: ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:6731:5: {...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getServiceOperationAccess().getUnorderedGroup_5(), 1) ) {
throw new FailedPredicateException(input, "ruleServiceOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getServiceOperationAccess().getUnorderedGroup_5(), 1)");
}
// InternalContextMappingDSL.g:6731:113: ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:6732:6: ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getServiceOperationAccess().getUnorderedGroup_5(), 1);
// InternalContextMappingDSL.g:6735:9: ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:6735:10: {...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleServiceOperation", "true");
}
// InternalContextMappingDSL.g:6735:19: (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:6735:20: otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) )
{
otherlv_13=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_13, grammarAccess.getServiceOperationAccess().getHintKeyword_5_1_0());
otherlv_14=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_14, grammarAccess.getServiceOperationAccess().getEqualsSignKeyword_5_1_1());
// InternalContextMappingDSL.g:6743:9: ( (lv_hint_15_0= RULE_STRING ) )
// InternalContextMappingDSL.g:6744:10: (lv_hint_15_0= RULE_STRING )
{
// InternalContextMappingDSL.g:6744:10: (lv_hint_15_0= RULE_STRING )
// InternalContextMappingDSL.g:6745:11: lv_hint_15_0= RULE_STRING
{
lv_hint_15_0=(Token)match(input,RULE_STRING,FOLLOW_116);
newLeafNode(lv_hint_15_0, grammarAccess.getServiceOperationAccess().getHintSTRINGTerminalRuleCall_5_1_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getServiceOperationRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_15_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getServiceOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:6767:4: ({...}? => ( ({...}? => ( (lv_publish_16_0= rulePublish ) ) ) ) )
{
// InternalContextMappingDSL.g:6767:4: ({...}? => ( ({...}? => ( (lv_publish_16_0= rulePublish ) ) ) ) )
// InternalContextMappingDSL.g:6768:5: {...}? => ( ({...}? => ( (lv_publish_16_0= rulePublish ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getServiceOperationAccess().getUnorderedGroup_5(), 2) ) {
throw new FailedPredicateException(input, "ruleServiceOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getServiceOperationAccess().getUnorderedGroup_5(), 2)");
}
// InternalContextMappingDSL.g:6768:113: ( ({...}? => ( (lv_publish_16_0= rulePublish ) ) ) )
// InternalContextMappingDSL.g:6769:6: ({...}? => ( (lv_publish_16_0= rulePublish ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getServiceOperationAccess().getUnorderedGroup_5(), 2);
// InternalContextMappingDSL.g:6772:9: ({...}? => ( (lv_publish_16_0= rulePublish ) ) )
// InternalContextMappingDSL.g:6772:10: {...}? => ( (lv_publish_16_0= rulePublish ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleServiceOperation", "true");
}
// InternalContextMappingDSL.g:6772:19: ( (lv_publish_16_0= rulePublish ) )
// InternalContextMappingDSL.g:6772:20: (lv_publish_16_0= rulePublish )
{
// InternalContextMappingDSL.g:6772:20: (lv_publish_16_0= rulePublish )
// InternalContextMappingDSL.g:6773:10: lv_publish_16_0= rulePublish
{
newCompositeNode(grammarAccess.getServiceOperationAccess().getPublishPublishParserRuleCall_5_2_0());
pushFollow(FOLLOW_116);
lv_publish_16_0=rulePublish();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getServiceOperationRule());
}
set(
current,
"publish",
lv_publish_16_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Publish");
afterParserOrEnumRuleCall();
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getServiceOperationAccess().getUnorderedGroup_5());
}
}
}
break;
default :
break loop193;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getServiceOperationAccess().getUnorderedGroup_5());
}
// InternalContextMappingDSL.g:6802:3: ( (lv_delegateHolder_17_0= ruleServiceOperationDelegate ) )?
int alt194=2;
int LA194_0 = input.LA(1);
if ( (LA194_0==RULE_DELEGATE) ) {
alt194=1;
}
switch (alt194) {
case 1 :
// InternalContextMappingDSL.g:6803:4: (lv_delegateHolder_17_0= ruleServiceOperationDelegate )
{
// InternalContextMappingDSL.g:6803:4: (lv_delegateHolder_17_0= ruleServiceOperationDelegate )
// InternalContextMappingDSL.g:6804:5: lv_delegateHolder_17_0= ruleServiceOperationDelegate
{
newCompositeNode(grammarAccess.getServiceOperationAccess().getDelegateHolderServiceOperationDelegateParserRuleCall_6_0());
pushFollow(FOLLOW_117);
lv_delegateHolder_17_0=ruleServiceOperationDelegate();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getServiceOperationRule());
}
set(
current,
"delegateHolder",
lv_delegateHolder_17_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.ServiceOperationDelegate");
afterParserOrEnumRuleCall();
}
}
break;
}
otherlv_18=(Token)match(input,105,FOLLOW_2);
newLeafNode(otherlv_18, grammarAccess.getServiceOperationAccess().getSemicolonKeyword_7());
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleServiceOperation"
// $ANTLR start "entryRuleServiceOperationDelegate"
// InternalContextMappingDSL.g:6829:1: entryRuleServiceOperationDelegate returns [EObject current=null] : iv_ruleServiceOperationDelegate= ruleServiceOperationDelegate EOF ;
public final EObject entryRuleServiceOperationDelegate() throws RecognitionException {
EObject current = null;
EObject iv_ruleServiceOperationDelegate = null;
try {
// InternalContextMappingDSL.g:6829:65: (iv_ruleServiceOperationDelegate= ruleServiceOperationDelegate EOF )
// InternalContextMappingDSL.g:6830:2: iv_ruleServiceOperationDelegate= ruleServiceOperationDelegate EOF
{
newCompositeNode(grammarAccess.getServiceOperationDelegateRule());
pushFollow(FOLLOW_1);
iv_ruleServiceOperationDelegate=ruleServiceOperationDelegate();
state._fsp--;
current =iv_ruleServiceOperationDelegate;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleServiceOperationDelegate"
// $ANTLR start "ruleServiceOperationDelegate"
// InternalContextMappingDSL.g:6836:1: ruleServiceOperationDelegate returns [EObject current=null] : (this_DELEGATE_0= RULE_DELEGATE (otherlv_1= '@' )? ( (otherlv_2= RULE_ID ) ) otherlv_3= '.' ( (otherlv_4= RULE_ID ) ) ) ;
public final EObject ruleServiceOperationDelegate() throws RecognitionException {
EObject current = null;
Token this_DELEGATE_0=null;
Token otherlv_1=null;
Token otherlv_2=null;
Token otherlv_3=null;
Token otherlv_4=null;
enterRule();
try {
// InternalContextMappingDSL.g:6842:2: ( (this_DELEGATE_0= RULE_DELEGATE (otherlv_1= '@' )? ( (otherlv_2= RULE_ID ) ) otherlv_3= '.' ( (otherlv_4= RULE_ID ) ) ) )
// InternalContextMappingDSL.g:6843:2: (this_DELEGATE_0= RULE_DELEGATE (otherlv_1= '@' )? ( (otherlv_2= RULE_ID ) ) otherlv_3= '.' ( (otherlv_4= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:6843:2: (this_DELEGATE_0= RULE_DELEGATE (otherlv_1= '@' )? ( (otherlv_2= RULE_ID ) ) otherlv_3= '.' ( (otherlv_4= RULE_ID ) ) )
// InternalContextMappingDSL.g:6844:3: this_DELEGATE_0= RULE_DELEGATE (otherlv_1= '@' )? ( (otherlv_2= RULE_ID ) ) otherlv_3= '.' ( (otherlv_4= RULE_ID ) )
{
this_DELEGATE_0=(Token)match(input,RULE_DELEGATE,FOLLOW_105);
newLeafNode(this_DELEGATE_0, grammarAccess.getServiceOperationDelegateAccess().getDELEGATETerminalRuleCall_0());
// InternalContextMappingDSL.g:6848:3: (otherlv_1= '@' )?
int alt195=2;
int LA195_0 = input.LA(1);
if ( (LA195_0==94) ) {
alt195=1;
}
switch (alt195) {
case 1 :
// InternalContextMappingDSL.g:6849:4: otherlv_1= '@'
{
otherlv_1=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_1, grammarAccess.getServiceOperationDelegateAccess().getCommercialAtKeyword_1());
}
break;
}
// InternalContextMappingDSL.g:6854:3: ( (otherlv_2= RULE_ID ) )
// InternalContextMappingDSL.g:6855:4: (otherlv_2= RULE_ID )
{
// InternalContextMappingDSL.g:6855:4: (otherlv_2= RULE_ID )
// InternalContextMappingDSL.g:6856:5: otherlv_2= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getServiceOperationDelegateRule());
}
otherlv_2=(Token)match(input,RULE_ID,FOLLOW_118);
newLeafNode(otherlv_2, grammarAccess.getServiceOperationDelegateAccess().getDelegateServiceRepositoryOptionCrossReference_2_0());
}
}
otherlv_3=(Token)match(input,106,FOLLOW_10);
newLeafNode(otherlv_3, grammarAccess.getServiceOperationDelegateAccess().getFullStopKeyword_3());
// InternalContextMappingDSL.g:6871:3: ( (otherlv_4= RULE_ID ) )
// InternalContextMappingDSL.g:6872:4: (otherlv_4= RULE_ID )
{
// InternalContextMappingDSL.g:6872:4: (otherlv_4= RULE_ID )
// InternalContextMappingDSL.g:6873:5: otherlv_4= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getServiceOperationDelegateRule());
}
otherlv_4=(Token)match(input,RULE_ID,FOLLOW_2);
newLeafNode(otherlv_4, grammarAccess.getServiceOperationDelegateAccess().getDelegateOperationServiceRepositoryOperationOptionCrossReference_4_0());
}
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleServiceOperationDelegate"
// $ANTLR start "entryRuleResourceOperation"
// InternalContextMappingDSL.g:6888:1: entryRuleResourceOperation returns [EObject current=null] : iv_ruleResourceOperation= ruleResourceOperation EOF ;
public final EObject entryRuleResourceOperation() throws RecognitionException {
EObject current = null;
EObject iv_ruleResourceOperation = null;
try {
// InternalContextMappingDSL.g:6888:58: (iv_ruleResourceOperation= ruleResourceOperation EOF )
// InternalContextMappingDSL.g:6889:2: iv_ruleResourceOperation= ruleResourceOperation EOF
{
newCompositeNode(grammarAccess.getResourceOperationRule());
pushFollow(FOLLOW_1);
iv_ruleResourceOperation=ruleResourceOperation();
state._fsp--;
current =iv_ruleResourceOperation;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleResourceOperation"
// $ANTLR start "ruleResourceOperation"
// InternalContextMappingDSL.g:6895:1: ruleResourceOperation returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( (lv_returnType_2_0= ruleComplexType ) )? ( (lv_name_3_0= RULE_ID ) ) (otherlv_4= '(' ( (lv_parameters_5_0= ruleParameter ) )? (otherlv_6= ',' ( (lv_parameters_7_0= ruleParameter ) ) )* otherlv_8= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_delegateHolder_22_0= ruleResourceOperationDelegate ) )? otherlv_23= ';' ) ;
public final EObject ruleResourceOperation() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token lv_name_3_0=null;
Token otherlv_4=null;
Token otherlv_6=null;
Token otherlv_8=null;
Token otherlv_10=null;
Token otherlv_12=null;
Token otherlv_13=null;
Token lv_hint_14_0=null;
Token otherlv_16=null;
Token otherlv_17=null;
Token lv_path_18_0=null;
Token otherlv_19=null;
Token otherlv_20=null;
Token lv_returnString_21_0=null;
Token otherlv_23=null;
Enumerator lv_visibility_1_0 = null;
EObject lv_returnType_2_0 = null;
EObject lv_parameters_5_0 = null;
EObject lv_parameters_7_0 = null;
AntlrDatatypeRuleToken lv_throws_11_0 = null;
Enumerator lv_httpMethod_15_0 = null;
EObject lv_delegateHolder_22_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:6901:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( (lv_returnType_2_0= ruleComplexType ) )? ( (lv_name_3_0= RULE_ID ) ) (otherlv_4= '(' ( (lv_parameters_5_0= ruleParameter ) )? (otherlv_6= ',' ( (lv_parameters_7_0= ruleParameter ) ) )* otherlv_8= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_delegateHolder_22_0= ruleResourceOperationDelegate ) )? otherlv_23= ';' ) )
// InternalContextMappingDSL.g:6902:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( (lv_returnType_2_0= ruleComplexType ) )? ( (lv_name_3_0= RULE_ID ) ) (otherlv_4= '(' ( (lv_parameters_5_0= ruleParameter ) )? (otherlv_6= ',' ( (lv_parameters_7_0= ruleParameter ) ) )* otherlv_8= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_delegateHolder_22_0= ruleResourceOperationDelegate ) )? otherlv_23= ';' )
{
// InternalContextMappingDSL.g:6902:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( (lv_returnType_2_0= ruleComplexType ) )? ( (lv_name_3_0= RULE_ID ) ) (otherlv_4= '(' ( (lv_parameters_5_0= ruleParameter ) )? (otherlv_6= ',' ( (lv_parameters_7_0= ruleParameter ) ) )* otherlv_8= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_delegateHolder_22_0= ruleResourceOperationDelegate ) )? otherlv_23= ';' )
// InternalContextMappingDSL.g:6903:3: ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( (lv_returnType_2_0= ruleComplexType ) )? ( (lv_name_3_0= RULE_ID ) ) (otherlv_4= '(' ( (lv_parameters_5_0= ruleParameter ) )? (otherlv_6= ',' ( (lv_parameters_7_0= ruleParameter ) ) )* otherlv_8= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( (lv_delegateHolder_22_0= ruleResourceOperationDelegate ) )? otherlv_23= ';'
{
// InternalContextMappingDSL.g:6903:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt196=2;
int LA196_0 = input.LA(1);
if ( (LA196_0==RULE_STRING) ) {
alt196=1;
}
switch (alt196) {
case 1 :
// InternalContextMappingDSL.g:6904:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:6904:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:6905:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_119);
newLeafNode(lv_doc_0_0, grammarAccess.getResourceOperationAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getResourceOperationRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
// InternalContextMappingDSL.g:6921:3: ( (lv_visibility_1_0= ruleVisibility ) )?
int alt197=2;
int LA197_0 = input.LA(1);
if ( (LA197_0==124||(LA197_0>=247 && LA197_0<=249)) ) {
alt197=1;
}
switch (alt197) {
case 1 :
// InternalContextMappingDSL.g:6922:4: (lv_visibility_1_0= ruleVisibility )
{
// InternalContextMappingDSL.g:6922:4: (lv_visibility_1_0= ruleVisibility )
// InternalContextMappingDSL.g:6923:5: lv_visibility_1_0= ruleVisibility
{
newCompositeNode(grammarAccess.getResourceOperationAccess().getVisibilityVisibilityEnumRuleCall_1_0());
pushFollow(FOLLOW_120);
lv_visibility_1_0=ruleVisibility();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getResourceOperationRule());
}
set(
current,
"visibility",
lv_visibility_1_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Visibility");
afterParserOrEnumRuleCall();
}
}
break;
}
// InternalContextMappingDSL.g:6940:3: ( (lv_returnType_2_0= ruleComplexType ) )?
int alt198=2;
int LA198_0 = input.LA(1);
if ( (LA198_0==RULE_MAP_COLLECTION_TYPE||LA198_0==94||(LA198_0>=185 && LA198_0<=206)||LA198_0==233||(LA198_0>=243 && LA198_0<=246)) ) {
alt198=1;
}
else if ( (LA198_0==RULE_ID) ) {
int LA198_2 = input.LA(2);
if ( (LA198_2==RULE_ID||LA198_2==106||LA198_2==118) ) {
alt198=1;
}
}
switch (alt198) {
case 1 :
// InternalContextMappingDSL.g:6941:4: (lv_returnType_2_0= ruleComplexType )
{
// InternalContextMappingDSL.g:6941:4: (lv_returnType_2_0= ruleComplexType )
// InternalContextMappingDSL.g:6942:5: lv_returnType_2_0= ruleComplexType
{
newCompositeNode(grammarAccess.getResourceOperationAccess().getReturnTypeComplexTypeParserRuleCall_2_0());
pushFollow(FOLLOW_10);
lv_returnType_2_0=ruleComplexType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getResourceOperationRule());
}
set(
current,
"returnType",
lv_returnType_2_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.ComplexType");
afterParserOrEnumRuleCall();
}
}
break;
}
// InternalContextMappingDSL.g:6959:3: ( (lv_name_3_0= RULE_ID ) )
// InternalContextMappingDSL.g:6960:4: (lv_name_3_0= RULE_ID )
{
// InternalContextMappingDSL.g:6960:4: (lv_name_3_0= RULE_ID )
// InternalContextMappingDSL.g:6961:5: lv_name_3_0= RULE_ID
{
lv_name_3_0=(Token)match(input,RULE_ID,FOLLOW_121);
newLeafNode(lv_name_3_0, grammarAccess.getResourceOperationAccess().getNameIDTerminalRuleCall_3_0());
if (current==null) {
current = createModelElement(grammarAccess.getResourceOperationRule());
}
setWithLastConsumed(
current,
"name",
lv_name_3_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:6977:3: (otherlv_4= '(' ( (lv_parameters_5_0= ruleParameter ) )? (otherlv_6= ',' ( (lv_parameters_7_0= ruleParameter ) ) )* otherlv_8= ')' )?
int alt201=2;
int LA201_0 = input.LA(1);
if ( (LA201_0==102) ) {
alt201=1;
}
switch (alt201) {
case 1 :
// InternalContextMappingDSL.g:6978:4: otherlv_4= '(' ( (lv_parameters_5_0= ruleParameter ) )? (otherlv_6= ',' ( (lv_parameters_7_0= ruleParameter ) ) )* otherlv_8= ')'
{
otherlv_4=(Token)match(input,102,FOLLOW_113);
newLeafNode(otherlv_4, grammarAccess.getResourceOperationAccess().getLeftParenthesisKeyword_4_0());
// InternalContextMappingDSL.g:6982:4: ( (lv_parameters_5_0= ruleParameter ) )?
int alt199=2;
int LA199_0 = input.LA(1);
if ( ((LA199_0>=RULE_STRING && LA199_0<=RULE_ID)||LA199_0==RULE_MAP_COLLECTION_TYPE||LA199_0==94||(LA199_0>=185 && LA199_0<=206)||LA199_0==233||(LA199_0>=243 && LA199_0<=246)) ) {
alt199=1;
}
switch (alt199) {
case 1 :
// InternalContextMappingDSL.g:6983:5: (lv_parameters_5_0= ruleParameter )
{
// InternalContextMappingDSL.g:6983:5: (lv_parameters_5_0= ruleParameter )
// InternalContextMappingDSL.g:6984:6: lv_parameters_5_0= ruleParameter
{
newCompositeNode(grammarAccess.getResourceOperationAccess().getParametersParameterParserRuleCall_4_1_0());
pushFollow(FOLLOW_114);
lv_parameters_5_0=ruleParameter();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getResourceOperationRule());
}
add(
current,
"parameters",
lv_parameters_5_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Parameter");
afterParserOrEnumRuleCall();
}
}
break;
}
// InternalContextMappingDSL.g:7001:4: (otherlv_6= ',' ( (lv_parameters_7_0= ruleParameter ) ) )*
loop200:
do {
int alt200=2;
int LA200_0 = input.LA(1);
if ( (LA200_0==24) ) {
alt200=1;
}
switch (alt200) {
case 1 :
// InternalContextMappingDSL.g:7002:5: otherlv_6= ',' ( (lv_parameters_7_0= ruleParameter ) )
{
otherlv_6=(Token)match(input,24,FOLLOW_115);
newLeafNode(otherlv_6, grammarAccess.getResourceOperationAccess().getCommaKeyword_4_2_0());
// InternalContextMappingDSL.g:7006:5: ( (lv_parameters_7_0= ruleParameter ) )
// InternalContextMappingDSL.g:7007:6: (lv_parameters_7_0= ruleParameter )
{
// InternalContextMappingDSL.g:7007:6: (lv_parameters_7_0= ruleParameter )
// InternalContextMappingDSL.g:7008:7: lv_parameters_7_0= ruleParameter
{
newCompositeNode(grammarAccess.getResourceOperationAccess().getParametersParameterParserRuleCall_4_2_1_0());
pushFollow(FOLLOW_114);
lv_parameters_7_0=ruleParameter();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getResourceOperationRule());
}
add(
current,
"parameters",
lv_parameters_7_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Parameter");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop200;
}
} while (true);
otherlv_8=(Token)match(input,103,FOLLOW_122);
newLeafNode(otherlv_8, grammarAccess.getResourceOperationAccess().getRightParenthesisKeyword_4_3());
}
break;
}
// InternalContextMappingDSL.g:7031:3: ( ( ( ( ({...}? => ( ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:7032:4: ( ( ( ({...}? => ( ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:7032:4: ( ( ( ({...}? => ( ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:7033:5: ( ( ({...}? => ( ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5());
// InternalContextMappingDSL.g:7036:5: ( ( ({...}? => ( ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:7037:6: ( ({...}? => ( ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:7037:6: ( ({...}? => ( ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) ) ) ) )*
loop202:
do {
int alt202=6;
int LA202_0 = input.LA(1);
if ( LA202_0 == 104 && getUnorderedGroupHelper().canSelect(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 0) ) {
alt202=1;
}
else if ( LA202_0 == 82 && getUnorderedGroupHelper().canSelect(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 1) ) {
alt202=2;
}
else if ( LA202_0 >= 233 && LA202_0 <= 237 && getUnorderedGroupHelper().canSelect(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 2) ) {
alt202=3;
}
else if ( LA202_0 == 91 && getUnorderedGroupHelper().canSelect(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 3) ) {
alt202=4;
}
else if ( LA202_0 == 107 && getUnorderedGroupHelper().canSelect(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 4) ) {
alt202=5;
}
switch (alt202) {
case 1 :
// InternalContextMappingDSL.g:7038:4: ({...}? => ( ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) ) ) )
{
// InternalContextMappingDSL.g:7038:4: ({...}? => ( ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) ) ) )
// InternalContextMappingDSL.g:7039:5: {...}? => ( ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 0) ) {
throw new FailedPredicateException(input, "ruleResourceOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 0)");
}
// InternalContextMappingDSL.g:7039:114: ( ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) ) )
// InternalContextMappingDSL.g:7040:6: ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 0);
// InternalContextMappingDSL.g:7043:9: ({...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) ) )
// InternalContextMappingDSL.g:7043:10: {...}? => (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleResourceOperation", "true");
}
// InternalContextMappingDSL.g:7043:19: (otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) ) )
// InternalContextMappingDSL.g:7043:20: otherlv_10= 'throws' ( (lv_throws_11_0= ruleThrowsIdentifier ) )
{
otherlv_10=(Token)match(input,104,FOLLOW_10);
newLeafNode(otherlv_10, grammarAccess.getResourceOperationAccess().getThrowsKeyword_5_0_0());
// InternalContextMappingDSL.g:7047:9: ( (lv_throws_11_0= ruleThrowsIdentifier ) )
// InternalContextMappingDSL.g:7048:10: (lv_throws_11_0= ruleThrowsIdentifier )
{
// InternalContextMappingDSL.g:7048:10: (lv_throws_11_0= ruleThrowsIdentifier )
// InternalContextMappingDSL.g:7049:11: lv_throws_11_0= ruleThrowsIdentifier
{
newCompositeNode(grammarAccess.getResourceOperationAccess().getThrowsThrowsIdentifierParserRuleCall_5_0_1_0());
pushFollow(FOLLOW_122);
lv_throws_11_0=ruleThrowsIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getResourceOperationRule());
}
set(
current,
"throws",
lv_throws_11_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.ThrowsIdentifier");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:7072:4: ({...}? => ( ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:7072:4: ({...}? => ( ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:7073:5: {...}? => ( ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 1) ) {
throw new FailedPredicateException(input, "ruleResourceOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 1)");
}
// InternalContextMappingDSL.g:7073:114: ( ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:7074:6: ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 1);
// InternalContextMappingDSL.g:7077:9: ({...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:7077:10: {...}? => (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleResourceOperation", "true");
}
// InternalContextMappingDSL.g:7077:19: (otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:7077:20: otherlv_12= 'hint' otherlv_13= '=' ( (lv_hint_14_0= RULE_STRING ) )
{
otherlv_12=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_12, grammarAccess.getResourceOperationAccess().getHintKeyword_5_1_0());
otherlv_13=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_13, grammarAccess.getResourceOperationAccess().getEqualsSignKeyword_5_1_1());
// InternalContextMappingDSL.g:7085:9: ( (lv_hint_14_0= RULE_STRING ) )
// InternalContextMappingDSL.g:7086:10: (lv_hint_14_0= RULE_STRING )
{
// InternalContextMappingDSL.g:7086:10: (lv_hint_14_0= RULE_STRING )
// InternalContextMappingDSL.g:7087:11: lv_hint_14_0= RULE_STRING
{
lv_hint_14_0=(Token)match(input,RULE_STRING,FOLLOW_122);
newLeafNode(lv_hint_14_0, grammarAccess.getResourceOperationAccess().getHintSTRINGTerminalRuleCall_5_1_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getResourceOperationRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_14_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:7109:4: ({...}? => ( ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) ) ) )
{
// InternalContextMappingDSL.g:7109:4: ({...}? => ( ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) ) ) )
// InternalContextMappingDSL.g:7110:5: {...}? => ( ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 2) ) {
throw new FailedPredicateException(input, "ruleResourceOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 2)");
}
// InternalContextMappingDSL.g:7110:114: ( ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) ) )
// InternalContextMappingDSL.g:7111:6: ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 2);
// InternalContextMappingDSL.g:7114:9: ({...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) ) )
// InternalContextMappingDSL.g:7114:10: {...}? => ( (lv_httpMethod_15_0= ruleHttpMethod ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleResourceOperation", "true");
}
// InternalContextMappingDSL.g:7114:19: ( (lv_httpMethod_15_0= ruleHttpMethod ) )
// InternalContextMappingDSL.g:7114:20: (lv_httpMethod_15_0= ruleHttpMethod )
{
// InternalContextMappingDSL.g:7114:20: (lv_httpMethod_15_0= ruleHttpMethod )
// InternalContextMappingDSL.g:7115:10: lv_httpMethod_15_0= ruleHttpMethod
{
newCompositeNode(grammarAccess.getResourceOperationAccess().getHttpMethodHttpMethodEnumRuleCall_5_2_0());
pushFollow(FOLLOW_122);
lv_httpMethod_15_0=ruleHttpMethod();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getResourceOperationRule());
}
set(
current,
"httpMethod",
lv_httpMethod_15_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.HttpMethod");
afterParserOrEnumRuleCall();
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:7137:4: ({...}? => ( ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:7137:4: ({...}? => ( ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:7138:5: {...}? => ( ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 3) ) {
throw new FailedPredicateException(input, "ruleResourceOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 3)");
}
// InternalContextMappingDSL.g:7138:114: ( ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:7139:6: ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 3);
// InternalContextMappingDSL.g:7142:9: ({...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:7142:10: {...}? => (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleResourceOperation", "true");
}
// InternalContextMappingDSL.g:7142:19: (otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:7142:20: otherlv_16= 'path' otherlv_17= '=' ( (lv_path_18_0= RULE_STRING ) )
{
otherlv_16=(Token)match(input,91,FOLLOW_89);
newLeafNode(otherlv_16, grammarAccess.getResourceOperationAccess().getPathKeyword_5_3_0());
otherlv_17=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_17, grammarAccess.getResourceOperationAccess().getEqualsSignKeyword_5_3_1());
// InternalContextMappingDSL.g:7150:9: ( (lv_path_18_0= RULE_STRING ) )
// InternalContextMappingDSL.g:7151:10: (lv_path_18_0= RULE_STRING )
{
// InternalContextMappingDSL.g:7151:10: (lv_path_18_0= RULE_STRING )
// InternalContextMappingDSL.g:7152:11: lv_path_18_0= RULE_STRING
{
lv_path_18_0=(Token)match(input,RULE_STRING,FOLLOW_122);
newLeafNode(lv_path_18_0, grammarAccess.getResourceOperationAccess().getPathSTRINGTerminalRuleCall_5_3_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getResourceOperationRule());
}
setWithLastConsumed(
current,
"path",
lv_path_18_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 5 :
// InternalContextMappingDSL.g:7174:4: ({...}? => ( ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:7174:4: ({...}? => ( ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:7175:5: {...}? => ( ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 4) ) {
throw new FailedPredicateException(input, "ruleResourceOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 4)");
}
// InternalContextMappingDSL.g:7175:114: ( ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:7176:6: ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5(), 4);
// InternalContextMappingDSL.g:7179:9: ({...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:7179:10: {...}? => (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleResourceOperation", "true");
}
// InternalContextMappingDSL.g:7179:19: (otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:7179:20: otherlv_19= 'return' otherlv_20= '=' ( (lv_returnString_21_0= RULE_STRING ) )
{
otherlv_19=(Token)match(input,107,FOLLOW_89);
newLeafNode(otherlv_19, grammarAccess.getResourceOperationAccess().getReturnKeyword_5_4_0());
otherlv_20=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_20, grammarAccess.getResourceOperationAccess().getEqualsSignKeyword_5_4_1());
// InternalContextMappingDSL.g:7187:9: ( (lv_returnString_21_0= RULE_STRING ) )
// InternalContextMappingDSL.g:7188:10: (lv_returnString_21_0= RULE_STRING )
{
// InternalContextMappingDSL.g:7188:10: (lv_returnString_21_0= RULE_STRING )
// InternalContextMappingDSL.g:7189:11: lv_returnString_21_0= RULE_STRING
{
lv_returnString_21_0=(Token)match(input,RULE_STRING,FOLLOW_122);
newLeafNode(lv_returnString_21_0, grammarAccess.getResourceOperationAccess().getReturnStringSTRINGTerminalRuleCall_5_4_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getResourceOperationRule());
}
setWithLastConsumed(
current,
"returnString",
lv_returnString_21_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5());
}
}
}
break;
default :
break loop202;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getResourceOperationAccess().getUnorderedGroup_5());
}
// InternalContextMappingDSL.g:7218:3: ( (lv_delegateHolder_22_0= ruleResourceOperationDelegate ) )?
int alt203=2;
int LA203_0 = input.LA(1);
if ( (LA203_0==RULE_DELEGATE) ) {
alt203=1;
}
switch (alt203) {
case 1 :
// InternalContextMappingDSL.g:7219:4: (lv_delegateHolder_22_0= ruleResourceOperationDelegate )
{
// InternalContextMappingDSL.g:7219:4: (lv_delegateHolder_22_0= ruleResourceOperationDelegate )
// InternalContextMappingDSL.g:7220:5: lv_delegateHolder_22_0= ruleResourceOperationDelegate
{
newCompositeNode(grammarAccess.getResourceOperationAccess().getDelegateHolderResourceOperationDelegateParserRuleCall_6_0());
pushFollow(FOLLOW_117);
lv_delegateHolder_22_0=ruleResourceOperationDelegate();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getResourceOperationRule());
}
set(
current,
"delegateHolder",
lv_delegateHolder_22_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.ResourceOperationDelegate");
afterParserOrEnumRuleCall();
}
}
break;
}
otherlv_23=(Token)match(input,105,FOLLOW_2);
newLeafNode(otherlv_23, grammarAccess.getResourceOperationAccess().getSemicolonKeyword_7());
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleResourceOperation"
// $ANTLR start "entryRuleResourceOperationDelegate"
// InternalContextMappingDSL.g:7245:1: entryRuleResourceOperationDelegate returns [EObject current=null] : iv_ruleResourceOperationDelegate= ruleResourceOperationDelegate EOF ;
public final EObject entryRuleResourceOperationDelegate() throws RecognitionException {
EObject current = null;
EObject iv_ruleResourceOperationDelegate = null;
try {
// InternalContextMappingDSL.g:7245:66: (iv_ruleResourceOperationDelegate= ruleResourceOperationDelegate EOF )
// InternalContextMappingDSL.g:7246:2: iv_ruleResourceOperationDelegate= ruleResourceOperationDelegate EOF
{
newCompositeNode(grammarAccess.getResourceOperationDelegateRule());
pushFollow(FOLLOW_1);
iv_ruleResourceOperationDelegate=ruleResourceOperationDelegate();
state._fsp--;
current =iv_ruleResourceOperationDelegate;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleResourceOperationDelegate"
// $ANTLR start "ruleResourceOperationDelegate"
// InternalContextMappingDSL.g:7252:1: ruleResourceOperationDelegate returns [EObject current=null] : (this_DELEGATE_0= RULE_DELEGATE (otherlv_1= '@' )? ( (otherlv_2= RULE_ID ) ) otherlv_3= '.' ( (otherlv_4= RULE_ID ) ) ) ;
public final EObject ruleResourceOperationDelegate() throws RecognitionException {
EObject current = null;
Token this_DELEGATE_0=null;
Token otherlv_1=null;
Token otherlv_2=null;
Token otherlv_3=null;
Token otherlv_4=null;
enterRule();
try {
// InternalContextMappingDSL.g:7258:2: ( (this_DELEGATE_0= RULE_DELEGATE (otherlv_1= '@' )? ( (otherlv_2= RULE_ID ) ) otherlv_3= '.' ( (otherlv_4= RULE_ID ) ) ) )
// InternalContextMappingDSL.g:7259:2: (this_DELEGATE_0= RULE_DELEGATE (otherlv_1= '@' )? ( (otherlv_2= RULE_ID ) ) otherlv_3= '.' ( (otherlv_4= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:7259:2: (this_DELEGATE_0= RULE_DELEGATE (otherlv_1= '@' )? ( (otherlv_2= RULE_ID ) ) otherlv_3= '.' ( (otherlv_4= RULE_ID ) ) )
// InternalContextMappingDSL.g:7260:3: this_DELEGATE_0= RULE_DELEGATE (otherlv_1= '@' )? ( (otherlv_2= RULE_ID ) ) otherlv_3= '.' ( (otherlv_4= RULE_ID ) )
{
this_DELEGATE_0=(Token)match(input,RULE_DELEGATE,FOLLOW_105);
newLeafNode(this_DELEGATE_0, grammarAccess.getResourceOperationDelegateAccess().getDELEGATETerminalRuleCall_0());
// InternalContextMappingDSL.g:7264:3: (otherlv_1= '@' )?
int alt204=2;
int LA204_0 = input.LA(1);
if ( (LA204_0==94) ) {
alt204=1;
}
switch (alt204) {
case 1 :
// InternalContextMappingDSL.g:7265:4: otherlv_1= '@'
{
otherlv_1=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_1, grammarAccess.getResourceOperationDelegateAccess().getCommercialAtKeyword_1());
}
break;
}
// InternalContextMappingDSL.g:7270:3: ( (otherlv_2= RULE_ID ) )
// InternalContextMappingDSL.g:7271:4: (otherlv_2= RULE_ID )
{
// InternalContextMappingDSL.g:7271:4: (otherlv_2= RULE_ID )
// InternalContextMappingDSL.g:7272:5: otherlv_2= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getResourceOperationDelegateRule());
}
otherlv_2=(Token)match(input,RULE_ID,FOLLOW_118);
newLeafNode(otherlv_2, grammarAccess.getResourceOperationDelegateAccess().getDelegateServiceCrossReference_2_0());
}
}
otherlv_3=(Token)match(input,106,FOLLOW_10);
newLeafNode(otherlv_3, grammarAccess.getResourceOperationDelegateAccess().getFullStopKeyword_3());
// InternalContextMappingDSL.g:7287:3: ( (otherlv_4= RULE_ID ) )
// InternalContextMappingDSL.g:7288:4: (otherlv_4= RULE_ID )
{
// InternalContextMappingDSL.g:7288:4: (otherlv_4= RULE_ID )
// InternalContextMappingDSL.g:7289:5: otherlv_4= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getResourceOperationDelegateRule());
}
otherlv_4=(Token)match(input,RULE_ID,FOLLOW_2);
newLeafNode(otherlv_4, grammarAccess.getResourceOperationDelegateAccess().getDelegateOperationServiceOperationCrossReference_4_0());
}
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleResourceOperationDelegate"
// $ANTLR start "entryRuleRepositoryOperation"
// InternalContextMappingDSL.g:7304:1: entryRuleRepositoryOperation returns [EObject current=null] : iv_ruleRepositoryOperation= ruleRepositoryOperation EOF ;
public final EObject entryRuleRepositoryOperation() throws RecognitionException {
EObject current = null;
EObject iv_ruleRepositoryOperation = null;
try {
// InternalContextMappingDSL.g:7304:60: (iv_ruleRepositoryOperation= ruleRepositoryOperation EOF )
// InternalContextMappingDSL.g:7305:2: iv_ruleRepositoryOperation= ruleRepositoryOperation EOF
{
newCompositeNode(grammarAccess.getRepositoryOperationRule());
pushFollow(FOLLOW_1);
iv_ruleRepositoryOperation=ruleRepositoryOperation();
state._fsp--;
current =iv_ruleRepositoryOperation;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleRepositoryOperation"
// $ANTLR start "ruleRepositoryOperation"
// InternalContextMappingDSL.g:7311:1: ruleRepositoryOperation returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( (lv_returnType_2_0= ruleComplexType ) ) | otherlv_3= 'void' )? ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= '(' ( (lv_parameters_6_0= ruleParameter ) )? (otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) ) )* otherlv_9= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_cache_16_0= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_construct_34_0= 'construct' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_build_35_0= 'build' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_map_36_0= 'map' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_37_0= rulePublish ) ) ) ) ) )* ) ) ) ( ( (lv_delegateToAccessObject_38_0= RULE_DELEGATE ) ) (otherlv_39= 'AccessObject' | ( (lv_accessObjectName_40_0= RULE_ID ) ) ) )? otherlv_41= ';' ) ;
public final EObject ruleRepositoryOperation() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token otherlv_3=null;
Token lv_name_4_0=null;
Token otherlv_5=null;
Token otherlv_7=null;
Token otherlv_9=null;
Token otherlv_11=null;
Token otherlv_13=null;
Token otherlv_14=null;
Token lv_hint_15_0=null;
Token lv_cache_16_0=null;
Token lv_gapOperation_17_0=null;
Token lv_noGapOperation_18_0=null;
Token otherlv_19=null;
Token otherlv_20=null;
Token lv_query_21_0=null;
Token otherlv_22=null;
Token otherlv_23=null;
Token lv_condition_24_0=null;
Token otherlv_25=null;
Token otherlv_26=null;
Token lv_select_27_0=null;
Token otherlv_28=null;
Token otherlv_29=null;
Token lv_groupBy_30_0=null;
Token otherlv_31=null;
Token otherlv_32=null;
Token lv_orderBy_33_0=null;
Token lv_construct_34_0=null;
Token lv_build_35_0=null;
Token lv_map_36_0=null;
Token lv_delegateToAccessObject_38_0=null;
Token otherlv_39=null;
Token lv_accessObjectName_40_0=null;
Token otherlv_41=null;
Enumerator lv_visibility_1_0 = null;
EObject lv_returnType_2_0 = null;
EObject lv_parameters_6_0 = null;
EObject lv_parameters_8_0 = null;
AntlrDatatypeRuleToken lv_throws_12_0 = null;
EObject lv_publish_37_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:7317:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( (lv_returnType_2_0= ruleComplexType ) ) | otherlv_3= 'void' )? ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= '(' ( (lv_parameters_6_0= ruleParameter ) )? (otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) ) )* otherlv_9= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_cache_16_0= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_construct_34_0= 'construct' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_build_35_0= 'build' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_map_36_0= 'map' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_37_0= rulePublish ) ) ) ) ) )* ) ) ) ( ( (lv_delegateToAccessObject_38_0= RULE_DELEGATE ) ) (otherlv_39= 'AccessObject' | ( (lv_accessObjectName_40_0= RULE_ID ) ) ) )? otherlv_41= ';' ) )
// InternalContextMappingDSL.g:7318:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( (lv_returnType_2_0= ruleComplexType ) ) | otherlv_3= 'void' )? ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= '(' ( (lv_parameters_6_0= ruleParameter ) )? (otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) ) )* otherlv_9= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_cache_16_0= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_construct_34_0= 'construct' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_build_35_0= 'build' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_map_36_0= 'map' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_37_0= rulePublish ) ) ) ) ) )* ) ) ) ( ( (lv_delegateToAccessObject_38_0= RULE_DELEGATE ) ) (otherlv_39= 'AccessObject' | ( (lv_accessObjectName_40_0= RULE_ID ) ) ) )? otherlv_41= ';' )
{
// InternalContextMappingDSL.g:7318:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( (lv_returnType_2_0= ruleComplexType ) ) | otherlv_3= 'void' )? ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= '(' ( (lv_parameters_6_0= ruleParameter ) )? (otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) ) )* otherlv_9= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_cache_16_0= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_construct_34_0= 'construct' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_build_35_0= 'build' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_map_36_0= 'map' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_37_0= rulePublish ) ) ) ) ) )* ) ) ) ( ( (lv_delegateToAccessObject_38_0= RULE_DELEGATE ) ) (otherlv_39= 'AccessObject' | ( (lv_accessObjectName_40_0= RULE_ID ) ) ) )? otherlv_41= ';' )
// InternalContextMappingDSL.g:7319:3: ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( (lv_returnType_2_0= ruleComplexType ) ) | otherlv_3= 'void' )? ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= '(' ( (lv_parameters_6_0= ruleParameter ) )? (otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) ) )* otherlv_9= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_cache_16_0= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_construct_34_0= 'construct' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_build_35_0= 'build' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_map_36_0= 'map' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_37_0= rulePublish ) ) ) ) ) )* ) ) ) ( ( (lv_delegateToAccessObject_38_0= RULE_DELEGATE ) ) (otherlv_39= 'AccessObject' | ( (lv_accessObjectName_40_0= RULE_ID ) ) ) )? otherlv_41= ';'
{
// InternalContextMappingDSL.g:7319:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt205=2;
int LA205_0 = input.LA(1);
if ( (LA205_0==RULE_STRING) ) {
alt205=1;
}
switch (alt205) {
case 1 :
// InternalContextMappingDSL.g:7320:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:7320:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:7321:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_110);
newLeafNode(lv_doc_0_0, grammarAccess.getRepositoryOperationAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryOperationRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
// InternalContextMappingDSL.g:7337:3: ( (lv_visibility_1_0= ruleVisibility ) )?
int alt206=2;
int LA206_0 = input.LA(1);
if ( (LA206_0==124||(LA206_0>=247 && LA206_0<=249)) ) {
alt206=1;
}
switch (alt206) {
case 1 :
// InternalContextMappingDSL.g:7338:4: (lv_visibility_1_0= ruleVisibility )
{
// InternalContextMappingDSL.g:7338:4: (lv_visibility_1_0= ruleVisibility )
// InternalContextMappingDSL.g:7339:5: lv_visibility_1_0= ruleVisibility
{
newCompositeNode(grammarAccess.getRepositoryOperationAccess().getVisibilityVisibilityEnumRuleCall_1_0());
pushFollow(FOLLOW_111);
lv_visibility_1_0=ruleVisibility();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getRepositoryOperationRule());
}
set(
current,
"visibility",
lv_visibility_1_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Visibility");
afterParserOrEnumRuleCall();
}
}
break;
}
// InternalContextMappingDSL.g:7356:3: ( ( (lv_returnType_2_0= ruleComplexType ) ) | otherlv_3= 'void' )?
int alt207=3;
switch ( input.LA(1) ) {
case RULE_MAP_COLLECTION_TYPE:
case 94:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
{
alt207=1;
}
break;
case RULE_ID:
{
int LA207_2 = input.LA(2);
if ( (LA207_2==RULE_ID||LA207_2==106||LA207_2==118) ) {
alt207=1;
}
}
break;
case 101:
{
alt207=2;
}
break;
}
switch (alt207) {
case 1 :
// InternalContextMappingDSL.g:7357:4: ( (lv_returnType_2_0= ruleComplexType ) )
{
// InternalContextMappingDSL.g:7357:4: ( (lv_returnType_2_0= ruleComplexType ) )
// InternalContextMappingDSL.g:7358:5: (lv_returnType_2_0= ruleComplexType )
{
// InternalContextMappingDSL.g:7358:5: (lv_returnType_2_0= ruleComplexType )
// InternalContextMappingDSL.g:7359:6: lv_returnType_2_0= ruleComplexType
{
newCompositeNode(grammarAccess.getRepositoryOperationAccess().getReturnTypeComplexTypeParserRuleCall_2_0_0());
pushFollow(FOLLOW_10);
lv_returnType_2_0=ruleComplexType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getRepositoryOperationRule());
}
set(
current,
"returnType",
lv_returnType_2_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.ComplexType");
afterParserOrEnumRuleCall();
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:7377:4: otherlv_3= 'void'
{
otherlv_3=(Token)match(input,101,FOLLOW_10);
newLeafNode(otherlv_3, grammarAccess.getRepositoryOperationAccess().getVoidKeyword_2_1());
}
break;
}
// InternalContextMappingDSL.g:7382:3: ( (lv_name_4_0= RULE_ID ) )
// InternalContextMappingDSL.g:7383:4: (lv_name_4_0= RULE_ID )
{
// InternalContextMappingDSL.g:7383:4: (lv_name_4_0= RULE_ID )
// InternalContextMappingDSL.g:7384:5: lv_name_4_0= RULE_ID
{
lv_name_4_0=(Token)match(input,RULE_ID,FOLLOW_123);
newLeafNode(lv_name_4_0, grammarAccess.getRepositoryOperationAccess().getNameIDTerminalRuleCall_3_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryOperationRule());
}
setWithLastConsumed(
current,
"name",
lv_name_4_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:7400:3: (otherlv_5= '(' ( (lv_parameters_6_0= ruleParameter ) )? (otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) ) )* otherlv_9= ')' )?
int alt210=2;
int LA210_0 = input.LA(1);
if ( (LA210_0==102) ) {
alt210=1;
}
switch (alt210) {
case 1 :
// InternalContextMappingDSL.g:7401:4: otherlv_5= '(' ( (lv_parameters_6_0= ruleParameter ) )? (otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) ) )* otherlv_9= ')'
{
otherlv_5=(Token)match(input,102,FOLLOW_113);
newLeafNode(otherlv_5, grammarAccess.getRepositoryOperationAccess().getLeftParenthesisKeyword_4_0());
// InternalContextMappingDSL.g:7405:4: ( (lv_parameters_6_0= ruleParameter ) )?
int alt208=2;
int LA208_0 = input.LA(1);
if ( ((LA208_0>=RULE_STRING && LA208_0<=RULE_ID)||LA208_0==RULE_MAP_COLLECTION_TYPE||LA208_0==94||(LA208_0>=185 && LA208_0<=206)||LA208_0==233||(LA208_0>=243 && LA208_0<=246)) ) {
alt208=1;
}
switch (alt208) {
case 1 :
// InternalContextMappingDSL.g:7406:5: (lv_parameters_6_0= ruleParameter )
{
// InternalContextMappingDSL.g:7406:5: (lv_parameters_6_0= ruleParameter )
// InternalContextMappingDSL.g:7407:6: lv_parameters_6_0= ruleParameter
{
newCompositeNode(grammarAccess.getRepositoryOperationAccess().getParametersParameterParserRuleCall_4_1_0());
pushFollow(FOLLOW_114);
lv_parameters_6_0=ruleParameter();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getRepositoryOperationRule());
}
add(
current,
"parameters",
lv_parameters_6_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Parameter");
afterParserOrEnumRuleCall();
}
}
break;
}
// InternalContextMappingDSL.g:7424:4: (otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) ) )*
loop209:
do {
int alt209=2;
int LA209_0 = input.LA(1);
if ( (LA209_0==24) ) {
alt209=1;
}
switch (alt209) {
case 1 :
// InternalContextMappingDSL.g:7425:5: otherlv_7= ',' ( (lv_parameters_8_0= ruleParameter ) )
{
otherlv_7=(Token)match(input,24,FOLLOW_115);
newLeafNode(otherlv_7, grammarAccess.getRepositoryOperationAccess().getCommaKeyword_4_2_0());
// InternalContextMappingDSL.g:7429:5: ( (lv_parameters_8_0= ruleParameter ) )
// InternalContextMappingDSL.g:7430:6: (lv_parameters_8_0= ruleParameter )
{
// InternalContextMappingDSL.g:7430:6: (lv_parameters_8_0= ruleParameter )
// InternalContextMappingDSL.g:7431:7: lv_parameters_8_0= ruleParameter
{
newCompositeNode(grammarAccess.getRepositoryOperationAccess().getParametersParameterParserRuleCall_4_2_1_0());
pushFollow(FOLLOW_114);
lv_parameters_8_0=ruleParameter();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getRepositoryOperationRule());
}
add(
current,
"parameters",
lv_parameters_8_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Parameter");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop209;
}
} while (true);
otherlv_9=(Token)match(input,103,FOLLOW_124);
newLeafNode(otherlv_9, grammarAccess.getRepositoryOperationAccess().getRightParenthesisKeyword_4_3());
}
break;
}
// InternalContextMappingDSL.g:7454:3: ( ( ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_cache_16_0= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_construct_34_0= 'construct' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_build_35_0= 'build' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_map_36_0= 'map' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_37_0= rulePublish ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:7455:4: ( ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_cache_16_0= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_construct_34_0= 'construct' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_build_35_0= 'build' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_map_36_0= 'map' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_37_0= rulePublish ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:7455:4: ( ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_cache_16_0= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_construct_34_0= 'construct' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_build_35_0= 'build' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_map_36_0= 'map' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_37_0= rulePublish ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:7456:5: ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_cache_16_0= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_construct_34_0= 'construct' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_build_35_0= 'build' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_map_36_0= 'map' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_37_0= rulePublish ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5());
// InternalContextMappingDSL.g:7459:5: ( ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_cache_16_0= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_construct_34_0= 'construct' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_build_35_0= 'build' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_map_36_0= 'map' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_37_0= rulePublish ) ) ) ) ) )* )
// InternalContextMappingDSL.g:7460:6: ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_cache_16_0= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_construct_34_0= 'construct' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_build_35_0= 'build' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_map_36_0= 'map' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_37_0= rulePublish ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:7460:6: ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_cache_16_0= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_construct_34_0= 'construct' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_build_35_0= 'build' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_map_36_0= 'map' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_37_0= rulePublish ) ) ) ) ) )*
loop212:
do {
int alt212=14;
alt212 = dfa212.predict(input);
switch (alt212) {
case 1 :
// InternalContextMappingDSL.g:7461:4: ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) )
{
// InternalContextMappingDSL.g:7461:4: ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) )
// InternalContextMappingDSL.g:7462:5: {...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 0) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 0)");
}
// InternalContextMappingDSL.g:7462:116: ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) )
// InternalContextMappingDSL.g:7463:6: ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 0);
// InternalContextMappingDSL.g:7466:9: ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) )
// InternalContextMappingDSL.g:7466:10: {...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "true");
}
// InternalContextMappingDSL.g:7466:19: (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) )
// InternalContextMappingDSL.g:7466:20: otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) )
{
otherlv_11=(Token)match(input,104,FOLLOW_10);
newLeafNode(otherlv_11, grammarAccess.getRepositoryOperationAccess().getThrowsKeyword_5_0_0());
// InternalContextMappingDSL.g:7470:9: ( (lv_throws_12_0= ruleThrowsIdentifier ) )
// InternalContextMappingDSL.g:7471:10: (lv_throws_12_0= ruleThrowsIdentifier )
{
// InternalContextMappingDSL.g:7471:10: (lv_throws_12_0= ruleThrowsIdentifier )
// InternalContextMappingDSL.g:7472:11: lv_throws_12_0= ruleThrowsIdentifier
{
newCompositeNode(grammarAccess.getRepositoryOperationAccess().getThrowsThrowsIdentifierParserRuleCall_5_0_1_0());
pushFollow(FOLLOW_124);
lv_throws_12_0=ruleThrowsIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getRepositoryOperationRule());
}
set(
current,
"throws",
lv_throws_12_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.ThrowsIdentifier");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:7495:4: ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:7495:4: ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:7496:5: {...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 1) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 1)");
}
// InternalContextMappingDSL.g:7496:116: ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:7497:6: ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 1);
// InternalContextMappingDSL.g:7500:9: ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:7500:10: {...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "true");
}
// InternalContextMappingDSL.g:7500:19: (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:7500:20: otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) )
{
otherlv_13=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_13, grammarAccess.getRepositoryOperationAccess().getHintKeyword_5_1_0());
otherlv_14=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_14, grammarAccess.getRepositoryOperationAccess().getEqualsSignKeyword_5_1_1());
// InternalContextMappingDSL.g:7508:9: ( (lv_hint_15_0= RULE_STRING ) )
// InternalContextMappingDSL.g:7509:10: (lv_hint_15_0= RULE_STRING )
{
// InternalContextMappingDSL.g:7509:10: (lv_hint_15_0= RULE_STRING )
// InternalContextMappingDSL.g:7510:11: lv_hint_15_0= RULE_STRING
{
lv_hint_15_0=(Token)match(input,RULE_STRING,FOLLOW_124);
newLeafNode(lv_hint_15_0, grammarAccess.getRepositoryOperationAccess().getHintSTRINGTerminalRuleCall_5_1_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryOperationRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_15_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:7532:4: ({...}? => ( ({...}? => ( (lv_cache_16_0= 'cache' ) ) ) ) )
{
// InternalContextMappingDSL.g:7532:4: ({...}? => ( ({...}? => ( (lv_cache_16_0= 'cache' ) ) ) ) )
// InternalContextMappingDSL.g:7533:5: {...}? => ( ({...}? => ( (lv_cache_16_0= 'cache' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 2) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 2)");
}
// InternalContextMappingDSL.g:7533:116: ( ({...}? => ( (lv_cache_16_0= 'cache' ) ) ) )
// InternalContextMappingDSL.g:7534:6: ({...}? => ( (lv_cache_16_0= 'cache' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 2);
// InternalContextMappingDSL.g:7537:9: ({...}? => ( (lv_cache_16_0= 'cache' ) ) )
// InternalContextMappingDSL.g:7537:10: {...}? => ( (lv_cache_16_0= 'cache' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "true");
}
// InternalContextMappingDSL.g:7537:19: ( (lv_cache_16_0= 'cache' ) )
// InternalContextMappingDSL.g:7537:20: (lv_cache_16_0= 'cache' )
{
// InternalContextMappingDSL.g:7537:20: (lv_cache_16_0= 'cache' )
// InternalContextMappingDSL.g:7538:10: lv_cache_16_0= 'cache'
{
lv_cache_16_0=(Token)match(input,108,FOLLOW_124);
newLeafNode(lv_cache_16_0, grammarAccess.getRepositoryOperationAccess().getCacheCacheKeyword_5_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryOperationRule());
}
setWithLastConsumed(current, "cache", true, "cache");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:7555:4: ({...}? => ( ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) ) ) )
{
// InternalContextMappingDSL.g:7555:4: ({...}? => ( ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) ) ) )
// InternalContextMappingDSL.g:7556:5: {...}? => ( ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 3) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 3)");
}
// InternalContextMappingDSL.g:7556:116: ( ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) ) )
// InternalContextMappingDSL.g:7557:6: ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 3);
// InternalContextMappingDSL.g:7560:9: ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) )
// InternalContextMappingDSL.g:7560:10: {...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "true");
}
// InternalContextMappingDSL.g:7560:19: ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) )
int alt211=2;
int LA211_0 = input.LA(1);
if ( (LA211_0==86) ) {
alt211=1;
}
else if ( (LA211_0==87) ) {
alt211=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 211, 0, input);
throw nvae;
}
switch (alt211) {
case 1 :
// InternalContextMappingDSL.g:7560:20: ( (lv_gapOperation_17_0= 'gap' ) )
{
// InternalContextMappingDSL.g:7560:20: ( (lv_gapOperation_17_0= 'gap' ) )
// InternalContextMappingDSL.g:7561:10: (lv_gapOperation_17_0= 'gap' )
{
// InternalContextMappingDSL.g:7561:10: (lv_gapOperation_17_0= 'gap' )
// InternalContextMappingDSL.g:7562:11: lv_gapOperation_17_0= 'gap'
{
lv_gapOperation_17_0=(Token)match(input,86,FOLLOW_124);
newLeafNode(lv_gapOperation_17_0, grammarAccess.getRepositoryOperationAccess().getGapOperationGapKeyword_5_3_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryOperationRule());
}
setWithLastConsumed(current, "gapOperation", true, "gap");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:7575:9: ( (lv_noGapOperation_18_0= 'nogap' ) )
{
// InternalContextMappingDSL.g:7575:9: ( (lv_noGapOperation_18_0= 'nogap' ) )
// InternalContextMappingDSL.g:7576:10: (lv_noGapOperation_18_0= 'nogap' )
{
// InternalContextMappingDSL.g:7576:10: (lv_noGapOperation_18_0= 'nogap' )
// InternalContextMappingDSL.g:7577:11: lv_noGapOperation_18_0= 'nogap'
{
lv_noGapOperation_18_0=(Token)match(input,87,FOLLOW_124);
newLeafNode(lv_noGapOperation_18_0, grammarAccess.getRepositoryOperationAccess().getNoGapOperationNogapKeyword_5_3_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryOperationRule());
}
setWithLastConsumed(current, "noGapOperation", true, "nogap");
}
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 5 :
// InternalContextMappingDSL.g:7595:4: ({...}? => ( ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:7595:4: ({...}? => ( ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:7596:5: {...}? => ( ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 4) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 4)");
}
// InternalContextMappingDSL.g:7596:116: ( ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:7597:6: ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 4);
// InternalContextMappingDSL.g:7600:9: ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:7600:10: {...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "true");
}
// InternalContextMappingDSL.g:7600:19: (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:7600:20: otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) )
{
otherlv_19=(Token)match(input,109,FOLLOW_89);
newLeafNode(otherlv_19, grammarAccess.getRepositoryOperationAccess().getQueryKeyword_5_4_0());
otherlv_20=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_20, grammarAccess.getRepositoryOperationAccess().getEqualsSignKeyword_5_4_1());
// InternalContextMappingDSL.g:7608:9: ( (lv_query_21_0= RULE_STRING ) )
// InternalContextMappingDSL.g:7609:10: (lv_query_21_0= RULE_STRING )
{
// InternalContextMappingDSL.g:7609:10: (lv_query_21_0= RULE_STRING )
// InternalContextMappingDSL.g:7610:11: lv_query_21_0= RULE_STRING
{
lv_query_21_0=(Token)match(input,RULE_STRING,FOLLOW_124);
newLeafNode(lv_query_21_0, grammarAccess.getRepositoryOperationAccess().getQuerySTRINGTerminalRuleCall_5_4_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryOperationRule());
}
setWithLastConsumed(
current,
"query",
lv_query_21_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 6 :
// InternalContextMappingDSL.g:7632:4: ({...}? => ( ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:7632:4: ({...}? => ( ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:7633:5: {...}? => ( ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 5) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 5)");
}
// InternalContextMappingDSL.g:7633:116: ( ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:7634:6: ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 5);
// InternalContextMappingDSL.g:7637:9: ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:7637:10: {...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "true");
}
// InternalContextMappingDSL.g:7637:19: (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:7637:20: otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) )
{
otherlv_22=(Token)match(input,110,FOLLOW_89);
newLeafNode(otherlv_22, grammarAccess.getRepositoryOperationAccess().getConditionKeyword_5_5_0());
otherlv_23=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_23, grammarAccess.getRepositoryOperationAccess().getEqualsSignKeyword_5_5_1());
// InternalContextMappingDSL.g:7645:9: ( (lv_condition_24_0= RULE_STRING ) )
// InternalContextMappingDSL.g:7646:10: (lv_condition_24_0= RULE_STRING )
{
// InternalContextMappingDSL.g:7646:10: (lv_condition_24_0= RULE_STRING )
// InternalContextMappingDSL.g:7647:11: lv_condition_24_0= RULE_STRING
{
lv_condition_24_0=(Token)match(input,RULE_STRING,FOLLOW_124);
newLeafNode(lv_condition_24_0, grammarAccess.getRepositoryOperationAccess().getConditionSTRINGTerminalRuleCall_5_5_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryOperationRule());
}
setWithLastConsumed(
current,
"condition",
lv_condition_24_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 7 :
// InternalContextMappingDSL.g:7669:4: ({...}? => ( ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:7669:4: ({...}? => ( ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:7670:5: {...}? => ( ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 6) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 6)");
}
// InternalContextMappingDSL.g:7670:116: ( ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:7671:6: ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 6);
// InternalContextMappingDSL.g:7674:9: ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:7674:10: {...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "true");
}
// InternalContextMappingDSL.g:7674:19: (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:7674:20: otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) )
{
otherlv_25=(Token)match(input,111,FOLLOW_89);
newLeafNode(otherlv_25, grammarAccess.getRepositoryOperationAccess().getSelectKeyword_5_6_0());
otherlv_26=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_26, grammarAccess.getRepositoryOperationAccess().getEqualsSignKeyword_5_6_1());
// InternalContextMappingDSL.g:7682:9: ( (lv_select_27_0= RULE_STRING ) )
// InternalContextMappingDSL.g:7683:10: (lv_select_27_0= RULE_STRING )
{
// InternalContextMappingDSL.g:7683:10: (lv_select_27_0= RULE_STRING )
// InternalContextMappingDSL.g:7684:11: lv_select_27_0= RULE_STRING
{
lv_select_27_0=(Token)match(input,RULE_STRING,FOLLOW_124);
newLeafNode(lv_select_27_0, grammarAccess.getRepositoryOperationAccess().getSelectSTRINGTerminalRuleCall_5_6_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryOperationRule());
}
setWithLastConsumed(
current,
"select",
lv_select_27_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 8 :
// InternalContextMappingDSL.g:7706:4: ({...}? => ( ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:7706:4: ({...}? => ( ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:7707:5: {...}? => ( ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 7) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 7)");
}
// InternalContextMappingDSL.g:7707:116: ( ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:7708:6: ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 7);
// InternalContextMappingDSL.g:7711:9: ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:7711:10: {...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "true");
}
// InternalContextMappingDSL.g:7711:19: (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:7711:20: otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) )
{
otherlv_28=(Token)match(input,112,FOLLOW_89);
newLeafNode(otherlv_28, grammarAccess.getRepositoryOperationAccess().getGroupByKeyword_5_7_0());
otherlv_29=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_29, grammarAccess.getRepositoryOperationAccess().getEqualsSignKeyword_5_7_1());
// InternalContextMappingDSL.g:7719:9: ( (lv_groupBy_30_0= RULE_STRING ) )
// InternalContextMappingDSL.g:7720:10: (lv_groupBy_30_0= RULE_STRING )
{
// InternalContextMappingDSL.g:7720:10: (lv_groupBy_30_0= RULE_STRING )
// InternalContextMappingDSL.g:7721:11: lv_groupBy_30_0= RULE_STRING
{
lv_groupBy_30_0=(Token)match(input,RULE_STRING,FOLLOW_124);
newLeafNode(lv_groupBy_30_0, grammarAccess.getRepositoryOperationAccess().getGroupBySTRINGTerminalRuleCall_5_7_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryOperationRule());
}
setWithLastConsumed(
current,
"groupBy",
lv_groupBy_30_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 9 :
// InternalContextMappingDSL.g:7743:4: ({...}? => ( ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:7743:4: ({...}? => ( ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:7744:5: {...}? => ( ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 8) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 8)");
}
// InternalContextMappingDSL.g:7744:116: ( ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:7745:6: ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 8);
// InternalContextMappingDSL.g:7748:9: ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:7748:10: {...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "true");
}
// InternalContextMappingDSL.g:7748:19: (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:7748:20: otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) )
{
otherlv_31=(Token)match(input,113,FOLLOW_89);
newLeafNode(otherlv_31, grammarAccess.getRepositoryOperationAccess().getOrderByKeyword_5_8_0());
otherlv_32=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_32, grammarAccess.getRepositoryOperationAccess().getEqualsSignKeyword_5_8_1());
// InternalContextMappingDSL.g:7756:9: ( (lv_orderBy_33_0= RULE_STRING ) )
// InternalContextMappingDSL.g:7757:10: (lv_orderBy_33_0= RULE_STRING )
{
// InternalContextMappingDSL.g:7757:10: (lv_orderBy_33_0= RULE_STRING )
// InternalContextMappingDSL.g:7758:11: lv_orderBy_33_0= RULE_STRING
{
lv_orderBy_33_0=(Token)match(input,RULE_STRING,FOLLOW_124);
newLeafNode(lv_orderBy_33_0, grammarAccess.getRepositoryOperationAccess().getOrderBySTRINGTerminalRuleCall_5_8_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryOperationRule());
}
setWithLastConsumed(
current,
"orderBy",
lv_orderBy_33_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 10 :
// InternalContextMappingDSL.g:7780:4: ({...}? => ( ({...}? => ( (lv_construct_34_0= 'construct' ) ) ) ) )
{
// InternalContextMappingDSL.g:7780:4: ({...}? => ( ({...}? => ( (lv_construct_34_0= 'construct' ) ) ) ) )
// InternalContextMappingDSL.g:7781:5: {...}? => ( ({...}? => ( (lv_construct_34_0= 'construct' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 9) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 9)");
}
// InternalContextMappingDSL.g:7781:116: ( ({...}? => ( (lv_construct_34_0= 'construct' ) ) ) )
// InternalContextMappingDSL.g:7782:6: ({...}? => ( (lv_construct_34_0= 'construct' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 9);
// InternalContextMappingDSL.g:7785:9: ({...}? => ( (lv_construct_34_0= 'construct' ) ) )
// InternalContextMappingDSL.g:7785:10: {...}? => ( (lv_construct_34_0= 'construct' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "true");
}
// InternalContextMappingDSL.g:7785:19: ( (lv_construct_34_0= 'construct' ) )
// InternalContextMappingDSL.g:7785:20: (lv_construct_34_0= 'construct' )
{
// InternalContextMappingDSL.g:7785:20: (lv_construct_34_0= 'construct' )
// InternalContextMappingDSL.g:7786:10: lv_construct_34_0= 'construct'
{
lv_construct_34_0=(Token)match(input,114,FOLLOW_124);
newLeafNode(lv_construct_34_0, grammarAccess.getRepositoryOperationAccess().getConstructConstructKeyword_5_9_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryOperationRule());
}
setWithLastConsumed(current, "construct", true, "construct");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 11 :
// InternalContextMappingDSL.g:7803:4: ({...}? => ( ({...}? => ( (lv_build_35_0= 'build' ) ) ) ) )
{
// InternalContextMappingDSL.g:7803:4: ({...}? => ( ({...}? => ( (lv_build_35_0= 'build' ) ) ) ) )
// InternalContextMappingDSL.g:7804:5: {...}? => ( ({...}? => ( (lv_build_35_0= 'build' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 10) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 10)");
}
// InternalContextMappingDSL.g:7804:117: ( ({...}? => ( (lv_build_35_0= 'build' ) ) ) )
// InternalContextMappingDSL.g:7805:6: ({...}? => ( (lv_build_35_0= 'build' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 10);
// InternalContextMappingDSL.g:7808:9: ({...}? => ( (lv_build_35_0= 'build' ) ) )
// InternalContextMappingDSL.g:7808:10: {...}? => ( (lv_build_35_0= 'build' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "true");
}
// InternalContextMappingDSL.g:7808:19: ( (lv_build_35_0= 'build' ) )
// InternalContextMappingDSL.g:7808:20: (lv_build_35_0= 'build' )
{
// InternalContextMappingDSL.g:7808:20: (lv_build_35_0= 'build' )
// InternalContextMappingDSL.g:7809:10: lv_build_35_0= 'build'
{
lv_build_35_0=(Token)match(input,115,FOLLOW_124);
newLeafNode(lv_build_35_0, grammarAccess.getRepositoryOperationAccess().getBuildBuildKeyword_5_10_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryOperationRule());
}
setWithLastConsumed(current, "build", true, "build");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 12 :
// InternalContextMappingDSL.g:7826:4: ({...}? => ( ({...}? => ( (lv_map_36_0= 'map' ) ) ) ) )
{
// InternalContextMappingDSL.g:7826:4: ({...}? => ( ({...}? => ( (lv_map_36_0= 'map' ) ) ) ) )
// InternalContextMappingDSL.g:7827:5: {...}? => ( ({...}? => ( (lv_map_36_0= 'map' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 11) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 11)");
}
// InternalContextMappingDSL.g:7827:117: ( ({...}? => ( (lv_map_36_0= 'map' ) ) ) )
// InternalContextMappingDSL.g:7828:6: ({...}? => ( (lv_map_36_0= 'map' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 11);
// InternalContextMappingDSL.g:7831:9: ({...}? => ( (lv_map_36_0= 'map' ) ) )
// InternalContextMappingDSL.g:7831:10: {...}? => ( (lv_map_36_0= 'map' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "true");
}
// InternalContextMappingDSL.g:7831:19: ( (lv_map_36_0= 'map' ) )
// InternalContextMappingDSL.g:7831:20: (lv_map_36_0= 'map' )
{
// InternalContextMappingDSL.g:7831:20: (lv_map_36_0= 'map' )
// InternalContextMappingDSL.g:7832:10: lv_map_36_0= 'map'
{
lv_map_36_0=(Token)match(input,116,FOLLOW_124);
newLeafNode(lv_map_36_0, grammarAccess.getRepositoryOperationAccess().getMapMapKeyword_5_11_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryOperationRule());
}
setWithLastConsumed(current, "map", true, "map");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5());
}
}
}
break;
case 13 :
// InternalContextMappingDSL.g:7849:4: ({...}? => ( ({...}? => ( (lv_publish_37_0= rulePublish ) ) ) ) )
{
// InternalContextMappingDSL.g:7849:4: ({...}? => ( ({...}? => ( (lv_publish_37_0= rulePublish ) ) ) ) )
// InternalContextMappingDSL.g:7850:5: {...}? => ( ({...}? => ( (lv_publish_37_0= rulePublish ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 12) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 12)");
}
// InternalContextMappingDSL.g:7850:117: ( ({...}? => ( (lv_publish_37_0= rulePublish ) ) ) )
// InternalContextMappingDSL.g:7851:6: ({...}? => ( (lv_publish_37_0= rulePublish ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 12);
// InternalContextMappingDSL.g:7854:9: ({...}? => ( (lv_publish_37_0= rulePublish ) ) )
// InternalContextMappingDSL.g:7854:10: {...}? => ( (lv_publish_37_0= rulePublish ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleRepositoryOperation", "true");
}
// InternalContextMappingDSL.g:7854:19: ( (lv_publish_37_0= rulePublish ) )
// InternalContextMappingDSL.g:7854:20: (lv_publish_37_0= rulePublish )
{
// InternalContextMappingDSL.g:7854:20: (lv_publish_37_0= rulePublish )
// InternalContextMappingDSL.g:7855:10: lv_publish_37_0= rulePublish
{
newCompositeNode(grammarAccess.getRepositoryOperationAccess().getPublishPublishParserRuleCall_5_12_0());
pushFollow(FOLLOW_124);
lv_publish_37_0=rulePublish();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getRepositoryOperationRule());
}
set(
current,
"publish",
lv_publish_37_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Publish");
afterParserOrEnumRuleCall();
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5());
}
}
}
break;
default :
break loop212;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5());
}
// InternalContextMappingDSL.g:7884:3: ( ( (lv_delegateToAccessObject_38_0= RULE_DELEGATE ) ) (otherlv_39= 'AccessObject' | ( (lv_accessObjectName_40_0= RULE_ID ) ) ) )?
int alt214=2;
int LA214_0 = input.LA(1);
if ( (LA214_0==RULE_DELEGATE) ) {
alt214=1;
}
switch (alt214) {
case 1 :
// InternalContextMappingDSL.g:7885:4: ( (lv_delegateToAccessObject_38_0= RULE_DELEGATE ) ) (otherlv_39= 'AccessObject' | ( (lv_accessObjectName_40_0= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:7885:4: ( (lv_delegateToAccessObject_38_0= RULE_DELEGATE ) )
// InternalContextMappingDSL.g:7886:5: (lv_delegateToAccessObject_38_0= RULE_DELEGATE )
{
// InternalContextMappingDSL.g:7886:5: (lv_delegateToAccessObject_38_0= RULE_DELEGATE )
// InternalContextMappingDSL.g:7887:6: lv_delegateToAccessObject_38_0= RULE_DELEGATE
{
lv_delegateToAccessObject_38_0=(Token)match(input,RULE_DELEGATE,FOLLOW_125);
newLeafNode(lv_delegateToAccessObject_38_0, grammarAccess.getRepositoryOperationAccess().getDelegateToAccessObjectDELEGATETerminalRuleCall_6_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryOperationRule());
}
setWithLastConsumed(
current,
"delegateToAccessObject",
true,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.DELEGATE");
}
}
// InternalContextMappingDSL.g:7903:4: (otherlv_39= 'AccessObject' | ( (lv_accessObjectName_40_0= RULE_ID ) ) )
int alt213=2;
int LA213_0 = input.LA(1);
if ( (LA213_0==117) ) {
alt213=1;
}
else if ( (LA213_0==RULE_ID) ) {
alt213=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 213, 0, input);
throw nvae;
}
switch (alt213) {
case 1 :
// InternalContextMappingDSL.g:7904:5: otherlv_39= 'AccessObject'
{
otherlv_39=(Token)match(input,117,FOLLOW_117);
newLeafNode(otherlv_39, grammarAccess.getRepositoryOperationAccess().getAccessObjectKeyword_6_1_0());
}
break;
case 2 :
// InternalContextMappingDSL.g:7909:5: ( (lv_accessObjectName_40_0= RULE_ID ) )
{
// InternalContextMappingDSL.g:7909:5: ( (lv_accessObjectName_40_0= RULE_ID ) )
// InternalContextMappingDSL.g:7910:6: (lv_accessObjectName_40_0= RULE_ID )
{
// InternalContextMappingDSL.g:7910:6: (lv_accessObjectName_40_0= RULE_ID )
// InternalContextMappingDSL.g:7911:7: lv_accessObjectName_40_0= RULE_ID
{
lv_accessObjectName_40_0=(Token)match(input,RULE_ID,FOLLOW_117);
newLeafNode(lv_accessObjectName_40_0, grammarAccess.getRepositoryOperationAccess().getAccessObjectNameIDTerminalRuleCall_6_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryOperationRule());
}
setWithLastConsumed(
current,
"accessObjectName",
lv_accessObjectName_40_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
}
break;
}
}
break;
}
otherlv_41=(Token)match(input,105,FOLLOW_2);
newLeafNode(otherlv_41, grammarAccess.getRepositoryOperationAccess().getSemicolonKeyword_7());
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleRepositoryOperation"
// $ANTLR start "entryRuleParameter"
// InternalContextMappingDSL.g:7937:1: entryRuleParameter returns [EObject current=null] : iv_ruleParameter= ruleParameter EOF ;
public final EObject entryRuleParameter() throws RecognitionException {
EObject current = null;
EObject iv_ruleParameter = null;
try {
// InternalContextMappingDSL.g:7937:50: (iv_ruleParameter= ruleParameter EOF )
// InternalContextMappingDSL.g:7938:2: iv_ruleParameter= ruleParameter EOF
{
newCompositeNode(grammarAccess.getParameterRule());
pushFollow(FOLLOW_1);
iv_ruleParameter=ruleParameter();
state._fsp--;
current =iv_ruleParameter;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleParameter"
// $ANTLR start "ruleParameter"
// InternalContextMappingDSL.g:7944:1: ruleParameter returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_parameterType_1_0= ruleComplexType ) ) ( (lv_name_2_0= RULE_ID ) ) ) ;
public final EObject ruleParameter() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token lv_name_2_0=null;
EObject lv_parameterType_1_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:7950:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_parameterType_1_0= ruleComplexType ) ) ( (lv_name_2_0= RULE_ID ) ) ) )
// InternalContextMappingDSL.g:7951:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_parameterType_1_0= ruleComplexType ) ) ( (lv_name_2_0= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:7951:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_parameterType_1_0= ruleComplexType ) ) ( (lv_name_2_0= RULE_ID ) ) )
// InternalContextMappingDSL.g:7952:3: ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_parameterType_1_0= ruleComplexType ) ) ( (lv_name_2_0= RULE_ID ) )
{
// InternalContextMappingDSL.g:7952:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt215=2;
int LA215_0 = input.LA(1);
if ( (LA215_0==RULE_STRING) ) {
alt215=1;
}
switch (alt215) {
case 1 :
// InternalContextMappingDSL.g:7953:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:7953:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:7954:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_120);
newLeafNode(lv_doc_0_0, grammarAccess.getParameterAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getParameterRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
// InternalContextMappingDSL.g:7970:3: ( (lv_parameterType_1_0= ruleComplexType ) )
// InternalContextMappingDSL.g:7971:4: (lv_parameterType_1_0= ruleComplexType )
{
// InternalContextMappingDSL.g:7971:4: (lv_parameterType_1_0= ruleComplexType )
// InternalContextMappingDSL.g:7972:5: lv_parameterType_1_0= ruleComplexType
{
newCompositeNode(grammarAccess.getParameterAccess().getParameterTypeComplexTypeParserRuleCall_1_0());
pushFollow(FOLLOW_10);
lv_parameterType_1_0=ruleComplexType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getParameterRule());
}
set(
current,
"parameterType",
lv_parameterType_1_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.ComplexType");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:7989:3: ( (lv_name_2_0= RULE_ID ) )
// InternalContextMappingDSL.g:7990:4: (lv_name_2_0= RULE_ID )
{
// InternalContextMappingDSL.g:7990:4: (lv_name_2_0= RULE_ID )
// InternalContextMappingDSL.g:7991:5: lv_name_2_0= RULE_ID
{
lv_name_2_0=(Token)match(input,RULE_ID,FOLLOW_2);
newLeafNode(lv_name_2_0, grammarAccess.getParameterAccess().getNameIDTerminalRuleCall_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getParameterRule());
}
setWithLastConsumed(
current,
"name",
lv_name_2_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleParameter"
// $ANTLR start "entryRuleComplexType"
// InternalContextMappingDSL.g:8011:1: entryRuleComplexType returns [EObject current=null] : iv_ruleComplexType= ruleComplexType EOF ;
public final EObject entryRuleComplexType() throws RecognitionException {
EObject current = null;
EObject iv_ruleComplexType = null;
try {
// InternalContextMappingDSL.g:8011:52: (iv_ruleComplexType= ruleComplexType EOF )
// InternalContextMappingDSL.g:8012:2: iv_ruleComplexType= ruleComplexType EOF
{
newCompositeNode(grammarAccess.getComplexTypeRule());
pushFollow(FOLLOW_1);
iv_ruleComplexType=ruleComplexType();
state._fsp--;
current =iv_ruleComplexType;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleComplexType"
// $ANTLR start "ruleComplexType"
// InternalContextMappingDSL.g:8018:1: ruleComplexType returns [EObject current=null] : ( ( (lv_type_0_0= ruleType ) ) | ( ( (lv_type_1_0= ruleType ) ) otherlv_2= '<' otherlv_3= '@' ( (otherlv_4= RULE_ID ) ) otherlv_5= '>' ) | (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( ( (lv_collectionType_8_0= ruleCollectionType ) ) otherlv_9= '<' ( (otherlv_10= '@' ( (otherlv_11= RULE_ID ) ) ) | ( (lv_type_12_0= ruleType ) ) | ( ( (lv_type_13_0= ruleType ) ) otherlv_14= '<' otherlv_15= '@' ( (otherlv_16= RULE_ID ) ) otherlv_17= '>' ) ) otherlv_18= '>' ) | ( ( (lv_mapCollectionType_19_0= RULE_MAP_COLLECTION_TYPE ) ) otherlv_20= '<' ( ( (lv_mapKeyType_21_0= ruleType ) ) | (otherlv_22= '@' ( (otherlv_23= RULE_ID ) ) ) ) otherlv_24= ',' ( (otherlv_25= '@' ( (otherlv_26= RULE_ID ) ) ) | ( (lv_type_27_0= ruleType ) ) | ( ( (lv_type_28_0= ruleType ) ) otherlv_29= '<' otherlv_30= '@' ( (otherlv_31= RULE_ID ) ) otherlv_32= '>' ) ) otherlv_33= '>' ) ) ;
public final EObject ruleComplexType() throws RecognitionException {
EObject current = null;
Token otherlv_2=null;
Token otherlv_3=null;
Token otherlv_4=null;
Token otherlv_5=null;
Token otherlv_6=null;
Token otherlv_7=null;
Token otherlv_9=null;
Token otherlv_10=null;
Token otherlv_11=null;
Token otherlv_14=null;
Token otherlv_15=null;
Token otherlv_16=null;
Token otherlv_17=null;
Token otherlv_18=null;
Token lv_mapCollectionType_19_0=null;
Token otherlv_20=null;
Token otherlv_22=null;
Token otherlv_23=null;
Token otherlv_24=null;
Token otherlv_25=null;
Token otherlv_26=null;
Token otherlv_29=null;
Token otherlv_30=null;
Token otherlv_31=null;
Token otherlv_32=null;
Token otherlv_33=null;
AntlrDatatypeRuleToken lv_type_0_0 = null;
AntlrDatatypeRuleToken lv_type_1_0 = null;
Enumerator lv_collectionType_8_0 = null;
AntlrDatatypeRuleToken lv_type_12_0 = null;
AntlrDatatypeRuleToken lv_type_13_0 = null;
AntlrDatatypeRuleToken lv_mapKeyType_21_0 = null;
AntlrDatatypeRuleToken lv_type_27_0 = null;
AntlrDatatypeRuleToken lv_type_28_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:8024:2: ( ( ( (lv_type_0_0= ruleType ) ) | ( ( (lv_type_1_0= ruleType ) ) otherlv_2= '<' otherlv_3= '@' ( (otherlv_4= RULE_ID ) ) otherlv_5= '>' ) | (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( ( (lv_collectionType_8_0= ruleCollectionType ) ) otherlv_9= '<' ( (otherlv_10= '@' ( (otherlv_11= RULE_ID ) ) ) | ( (lv_type_12_0= ruleType ) ) | ( ( (lv_type_13_0= ruleType ) ) otherlv_14= '<' otherlv_15= '@' ( (otherlv_16= RULE_ID ) ) otherlv_17= '>' ) ) otherlv_18= '>' ) | ( ( (lv_mapCollectionType_19_0= RULE_MAP_COLLECTION_TYPE ) ) otherlv_20= '<' ( ( (lv_mapKeyType_21_0= ruleType ) ) | (otherlv_22= '@' ( (otherlv_23= RULE_ID ) ) ) ) otherlv_24= ',' ( (otherlv_25= '@' ( (otherlv_26= RULE_ID ) ) ) | ( (lv_type_27_0= ruleType ) ) | ( ( (lv_type_28_0= ruleType ) ) otherlv_29= '<' otherlv_30= '@' ( (otherlv_31= RULE_ID ) ) otherlv_32= '>' ) ) otherlv_33= '>' ) ) )
// InternalContextMappingDSL.g:8025:2: ( ( (lv_type_0_0= ruleType ) ) | ( ( (lv_type_1_0= ruleType ) ) otherlv_2= '<' otherlv_3= '@' ( (otherlv_4= RULE_ID ) ) otherlv_5= '>' ) | (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( ( (lv_collectionType_8_0= ruleCollectionType ) ) otherlv_9= '<' ( (otherlv_10= '@' ( (otherlv_11= RULE_ID ) ) ) | ( (lv_type_12_0= ruleType ) ) | ( ( (lv_type_13_0= ruleType ) ) otherlv_14= '<' otherlv_15= '@' ( (otherlv_16= RULE_ID ) ) otherlv_17= '>' ) ) otherlv_18= '>' ) | ( ( (lv_mapCollectionType_19_0= RULE_MAP_COLLECTION_TYPE ) ) otherlv_20= '<' ( ( (lv_mapKeyType_21_0= ruleType ) ) | (otherlv_22= '@' ( (otherlv_23= RULE_ID ) ) ) ) otherlv_24= ',' ( (otherlv_25= '@' ( (otherlv_26= RULE_ID ) ) ) | ( (lv_type_27_0= ruleType ) ) | ( ( (lv_type_28_0= ruleType ) ) otherlv_29= '<' otherlv_30= '@' ( (otherlv_31= RULE_ID ) ) otherlv_32= '>' ) ) otherlv_33= '>' ) )
{
// InternalContextMappingDSL.g:8025:2: ( ( (lv_type_0_0= ruleType ) ) | ( ( (lv_type_1_0= ruleType ) ) otherlv_2= '<' otherlv_3= '@' ( (otherlv_4= RULE_ID ) ) otherlv_5= '>' ) | (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( ( (lv_collectionType_8_0= ruleCollectionType ) ) otherlv_9= '<' ( (otherlv_10= '@' ( (otherlv_11= RULE_ID ) ) ) | ( (lv_type_12_0= ruleType ) ) | ( ( (lv_type_13_0= ruleType ) ) otherlv_14= '<' otherlv_15= '@' ( (otherlv_16= RULE_ID ) ) otherlv_17= '>' ) ) otherlv_18= '>' ) | ( ( (lv_mapCollectionType_19_0= RULE_MAP_COLLECTION_TYPE ) ) otherlv_20= '<' ( ( (lv_mapKeyType_21_0= ruleType ) ) | (otherlv_22= '@' ( (otherlv_23= RULE_ID ) ) ) ) otherlv_24= ',' ( (otherlv_25= '@' ( (otherlv_26= RULE_ID ) ) ) | ( (lv_type_27_0= ruleType ) ) | ( ( (lv_type_28_0= ruleType ) ) otherlv_29= '<' otherlv_30= '@' ( (otherlv_31= RULE_ID ) ) otherlv_32= '>' ) ) otherlv_33= '>' ) )
int alt219=5;
alt219 = dfa219.predict(input);
switch (alt219) {
case 1 :
// InternalContextMappingDSL.g:8026:3: ( (lv_type_0_0= ruleType ) )
{
// InternalContextMappingDSL.g:8026:3: ( (lv_type_0_0= ruleType ) )
// InternalContextMappingDSL.g:8027:4: (lv_type_0_0= ruleType )
{
// InternalContextMappingDSL.g:8027:4: (lv_type_0_0= ruleType )
// InternalContextMappingDSL.g:8028:5: lv_type_0_0= ruleType
{
newCompositeNode(grammarAccess.getComplexTypeAccess().getTypeTypeParserRuleCall_0_0());
pushFollow(FOLLOW_2);
lv_type_0_0=ruleType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getComplexTypeRule());
}
set(
current,
"type",
lv_type_0_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Type");
afterParserOrEnumRuleCall();
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:8046:3: ( ( (lv_type_1_0= ruleType ) ) otherlv_2= '<' otherlv_3= '@' ( (otherlv_4= RULE_ID ) ) otherlv_5= '>' )
{
// InternalContextMappingDSL.g:8046:3: ( ( (lv_type_1_0= ruleType ) ) otherlv_2= '<' otherlv_3= '@' ( (otherlv_4= RULE_ID ) ) otherlv_5= '>' )
// InternalContextMappingDSL.g:8047:4: ( (lv_type_1_0= ruleType ) ) otherlv_2= '<' otherlv_3= '@' ( (otherlv_4= RULE_ID ) ) otherlv_5= '>'
{
// InternalContextMappingDSL.g:8047:4: ( (lv_type_1_0= ruleType ) )
// InternalContextMappingDSL.g:8048:5: (lv_type_1_0= ruleType )
{
// InternalContextMappingDSL.g:8048:5: (lv_type_1_0= ruleType )
// InternalContextMappingDSL.g:8049:6: lv_type_1_0= ruleType
{
newCompositeNode(grammarAccess.getComplexTypeAccess().getTypeTypeParserRuleCall_1_0_0());
pushFollow(FOLLOW_126);
lv_type_1_0=ruleType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getComplexTypeRule());
}
set(
current,
"type",
lv_type_1_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Type");
afterParserOrEnumRuleCall();
}
}
otherlv_2=(Token)match(input,118,FOLLOW_127);
newLeafNode(otherlv_2, grammarAccess.getComplexTypeAccess().getLessThanSignKeyword_1_1());
otherlv_3=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_3, grammarAccess.getComplexTypeAccess().getCommercialAtKeyword_1_2());
// InternalContextMappingDSL.g:8074:4: ( (otherlv_4= RULE_ID ) )
// InternalContextMappingDSL.g:8075:5: (otherlv_4= RULE_ID )
{
// InternalContextMappingDSL.g:8075:5: (otherlv_4= RULE_ID )
// InternalContextMappingDSL.g:8076:6: otherlv_4= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getComplexTypeRule());
}
otherlv_4=(Token)match(input,RULE_ID,FOLLOW_128);
newLeafNode(otherlv_4, grammarAccess.getComplexTypeAccess().getDomainObjectTypeSimpleDomainObjectCrossReference_1_3_0());
}
}
otherlv_5=(Token)match(input,119,FOLLOW_2);
newLeafNode(otherlv_5, grammarAccess.getComplexTypeAccess().getGreaterThanSignKeyword_1_4());
}
}
break;
case 3 :
// InternalContextMappingDSL.g:8093:3: (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:8093:3: (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) )
// InternalContextMappingDSL.g:8094:4: otherlv_6= '@' ( (otherlv_7= RULE_ID ) )
{
otherlv_6=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_6, grammarAccess.getComplexTypeAccess().getCommercialAtKeyword_2_0());
// InternalContextMappingDSL.g:8098:4: ( (otherlv_7= RULE_ID ) )
// InternalContextMappingDSL.g:8099:5: (otherlv_7= RULE_ID )
{
// InternalContextMappingDSL.g:8099:5: (otherlv_7= RULE_ID )
// InternalContextMappingDSL.g:8100:6: otherlv_7= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getComplexTypeRule());
}
otherlv_7=(Token)match(input,RULE_ID,FOLLOW_2);
newLeafNode(otherlv_7, grammarAccess.getComplexTypeAccess().getDomainObjectTypeSimpleDomainObjectCrossReference_2_1_0());
}
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:8113:3: ( ( (lv_collectionType_8_0= ruleCollectionType ) ) otherlv_9= '<' ( (otherlv_10= '@' ( (otherlv_11= RULE_ID ) ) ) | ( (lv_type_12_0= ruleType ) ) | ( ( (lv_type_13_0= ruleType ) ) otherlv_14= '<' otherlv_15= '@' ( (otherlv_16= RULE_ID ) ) otherlv_17= '>' ) ) otherlv_18= '>' )
{
// InternalContextMappingDSL.g:8113:3: ( ( (lv_collectionType_8_0= ruleCollectionType ) ) otherlv_9= '<' ( (otherlv_10= '@' ( (otherlv_11= RULE_ID ) ) ) | ( (lv_type_12_0= ruleType ) ) | ( ( (lv_type_13_0= ruleType ) ) otherlv_14= '<' otherlv_15= '@' ( (otherlv_16= RULE_ID ) ) otherlv_17= '>' ) ) otherlv_18= '>' )
// InternalContextMappingDSL.g:8114:4: ( (lv_collectionType_8_0= ruleCollectionType ) ) otherlv_9= '<' ( (otherlv_10= '@' ( (otherlv_11= RULE_ID ) ) ) | ( (lv_type_12_0= ruleType ) ) | ( ( (lv_type_13_0= ruleType ) ) otherlv_14= '<' otherlv_15= '@' ( (otherlv_16= RULE_ID ) ) otherlv_17= '>' ) ) otherlv_18= '>'
{
// InternalContextMappingDSL.g:8114:4: ( (lv_collectionType_8_0= ruleCollectionType ) )
// InternalContextMappingDSL.g:8115:5: (lv_collectionType_8_0= ruleCollectionType )
{
// InternalContextMappingDSL.g:8115:5: (lv_collectionType_8_0= ruleCollectionType )
// InternalContextMappingDSL.g:8116:6: lv_collectionType_8_0= ruleCollectionType
{
newCompositeNode(grammarAccess.getComplexTypeAccess().getCollectionTypeCollectionTypeEnumRuleCall_3_0_0());
pushFollow(FOLLOW_126);
lv_collectionType_8_0=ruleCollectionType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getComplexTypeRule());
}
set(
current,
"collectionType",
lv_collectionType_8_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.CollectionType");
afterParserOrEnumRuleCall();
}
}
otherlv_9=(Token)match(input,118,FOLLOW_129);
newLeafNode(otherlv_9, grammarAccess.getComplexTypeAccess().getLessThanSignKeyword_3_1());
// InternalContextMappingDSL.g:8137:4: ( (otherlv_10= '@' ( (otherlv_11= RULE_ID ) ) ) | ( (lv_type_12_0= ruleType ) ) | ( ( (lv_type_13_0= ruleType ) ) otherlv_14= '<' otherlv_15= '@' ( (otherlv_16= RULE_ID ) ) otherlv_17= '>' ) )
int alt216=3;
alt216 = dfa216.predict(input);
switch (alt216) {
case 1 :
// InternalContextMappingDSL.g:8138:5: (otherlv_10= '@' ( (otherlv_11= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:8138:5: (otherlv_10= '@' ( (otherlv_11= RULE_ID ) ) )
// InternalContextMappingDSL.g:8139:6: otherlv_10= '@' ( (otherlv_11= RULE_ID ) )
{
otherlv_10=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_10, grammarAccess.getComplexTypeAccess().getCommercialAtKeyword_3_2_0_0());
// InternalContextMappingDSL.g:8143:6: ( (otherlv_11= RULE_ID ) )
// InternalContextMappingDSL.g:8144:7: (otherlv_11= RULE_ID )
{
// InternalContextMappingDSL.g:8144:7: (otherlv_11= RULE_ID )
// InternalContextMappingDSL.g:8145:8: otherlv_11= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getComplexTypeRule());
}
otherlv_11=(Token)match(input,RULE_ID,FOLLOW_128);
newLeafNode(otherlv_11, grammarAccess.getComplexTypeAccess().getDomainObjectTypeSimpleDomainObjectCrossReference_3_2_0_1_0());
}
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:8158:5: ( (lv_type_12_0= ruleType ) )
{
// InternalContextMappingDSL.g:8158:5: ( (lv_type_12_0= ruleType ) )
// InternalContextMappingDSL.g:8159:6: (lv_type_12_0= ruleType )
{
// InternalContextMappingDSL.g:8159:6: (lv_type_12_0= ruleType )
// InternalContextMappingDSL.g:8160:7: lv_type_12_0= ruleType
{
newCompositeNode(grammarAccess.getComplexTypeAccess().getTypeTypeParserRuleCall_3_2_1_0());
pushFollow(FOLLOW_128);
lv_type_12_0=ruleType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getComplexTypeRule());
}
set(
current,
"type",
lv_type_12_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Type");
afterParserOrEnumRuleCall();
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:8178:5: ( ( (lv_type_13_0= ruleType ) ) otherlv_14= '<' otherlv_15= '@' ( (otherlv_16= RULE_ID ) ) otherlv_17= '>' )
{
// InternalContextMappingDSL.g:8178:5: ( ( (lv_type_13_0= ruleType ) ) otherlv_14= '<' otherlv_15= '@' ( (otherlv_16= RULE_ID ) ) otherlv_17= '>' )
// InternalContextMappingDSL.g:8179:6: ( (lv_type_13_0= ruleType ) ) otherlv_14= '<' otherlv_15= '@' ( (otherlv_16= RULE_ID ) ) otherlv_17= '>'
{
// InternalContextMappingDSL.g:8179:6: ( (lv_type_13_0= ruleType ) )
// InternalContextMappingDSL.g:8180:7: (lv_type_13_0= ruleType )
{
// InternalContextMappingDSL.g:8180:7: (lv_type_13_0= ruleType )
// InternalContextMappingDSL.g:8181:8: lv_type_13_0= ruleType
{
newCompositeNode(grammarAccess.getComplexTypeAccess().getTypeTypeParserRuleCall_3_2_2_0_0());
pushFollow(FOLLOW_126);
lv_type_13_0=ruleType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getComplexTypeRule());
}
set(
current,
"type",
lv_type_13_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Type");
afterParserOrEnumRuleCall();
}
}
otherlv_14=(Token)match(input,118,FOLLOW_127);
newLeafNode(otherlv_14, grammarAccess.getComplexTypeAccess().getLessThanSignKeyword_3_2_2_1());
otherlv_15=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_15, grammarAccess.getComplexTypeAccess().getCommercialAtKeyword_3_2_2_2());
// InternalContextMappingDSL.g:8206:6: ( (otherlv_16= RULE_ID ) )
// InternalContextMappingDSL.g:8207:7: (otherlv_16= RULE_ID )
{
// InternalContextMappingDSL.g:8207:7: (otherlv_16= RULE_ID )
// InternalContextMappingDSL.g:8208:8: otherlv_16= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getComplexTypeRule());
}
otherlv_16=(Token)match(input,RULE_ID,FOLLOW_128);
newLeafNode(otherlv_16, grammarAccess.getComplexTypeAccess().getDomainObjectTypeSimpleDomainObjectCrossReference_3_2_2_3_0());
}
}
otherlv_17=(Token)match(input,119,FOLLOW_128);
newLeafNode(otherlv_17, grammarAccess.getComplexTypeAccess().getGreaterThanSignKeyword_3_2_2_4());
}
}
break;
}
otherlv_18=(Token)match(input,119,FOLLOW_2);
newLeafNode(otherlv_18, grammarAccess.getComplexTypeAccess().getGreaterThanSignKeyword_3_3());
}
}
break;
case 5 :
// InternalContextMappingDSL.g:8231:3: ( ( (lv_mapCollectionType_19_0= RULE_MAP_COLLECTION_TYPE ) ) otherlv_20= '<' ( ( (lv_mapKeyType_21_0= ruleType ) ) | (otherlv_22= '@' ( (otherlv_23= RULE_ID ) ) ) ) otherlv_24= ',' ( (otherlv_25= '@' ( (otherlv_26= RULE_ID ) ) ) | ( (lv_type_27_0= ruleType ) ) | ( ( (lv_type_28_0= ruleType ) ) otherlv_29= '<' otherlv_30= '@' ( (otherlv_31= RULE_ID ) ) otherlv_32= '>' ) ) otherlv_33= '>' )
{
// InternalContextMappingDSL.g:8231:3: ( ( (lv_mapCollectionType_19_0= RULE_MAP_COLLECTION_TYPE ) ) otherlv_20= '<' ( ( (lv_mapKeyType_21_0= ruleType ) ) | (otherlv_22= '@' ( (otherlv_23= RULE_ID ) ) ) ) otherlv_24= ',' ( (otherlv_25= '@' ( (otherlv_26= RULE_ID ) ) ) | ( (lv_type_27_0= ruleType ) ) | ( ( (lv_type_28_0= ruleType ) ) otherlv_29= '<' otherlv_30= '@' ( (otherlv_31= RULE_ID ) ) otherlv_32= '>' ) ) otherlv_33= '>' )
// InternalContextMappingDSL.g:8232:4: ( (lv_mapCollectionType_19_0= RULE_MAP_COLLECTION_TYPE ) ) otherlv_20= '<' ( ( (lv_mapKeyType_21_0= ruleType ) ) | (otherlv_22= '@' ( (otherlv_23= RULE_ID ) ) ) ) otherlv_24= ',' ( (otherlv_25= '@' ( (otherlv_26= RULE_ID ) ) ) | ( (lv_type_27_0= ruleType ) ) | ( ( (lv_type_28_0= ruleType ) ) otherlv_29= '<' otherlv_30= '@' ( (otherlv_31= RULE_ID ) ) otherlv_32= '>' ) ) otherlv_33= '>'
{
// InternalContextMappingDSL.g:8232:4: ( (lv_mapCollectionType_19_0= RULE_MAP_COLLECTION_TYPE ) )
// InternalContextMappingDSL.g:8233:5: (lv_mapCollectionType_19_0= RULE_MAP_COLLECTION_TYPE )
{
// InternalContextMappingDSL.g:8233:5: (lv_mapCollectionType_19_0= RULE_MAP_COLLECTION_TYPE )
// InternalContextMappingDSL.g:8234:6: lv_mapCollectionType_19_0= RULE_MAP_COLLECTION_TYPE
{
lv_mapCollectionType_19_0=(Token)match(input,RULE_MAP_COLLECTION_TYPE,FOLLOW_126);
newLeafNode(lv_mapCollectionType_19_0, grammarAccess.getComplexTypeAccess().getMapCollectionTypeMAP_COLLECTION_TYPETerminalRuleCall_4_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getComplexTypeRule());
}
setWithLastConsumed(
current,
"mapCollectionType",
lv_mapCollectionType_19_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.MAP_COLLECTION_TYPE");
}
}
otherlv_20=(Token)match(input,118,FOLLOW_129);
newLeafNode(otherlv_20, grammarAccess.getComplexTypeAccess().getLessThanSignKeyword_4_1());
// InternalContextMappingDSL.g:8254:4: ( ( (lv_mapKeyType_21_0= ruleType ) ) | (otherlv_22= '@' ( (otherlv_23= RULE_ID ) ) ) )
int alt217=2;
int LA217_0 = input.LA(1);
if ( (LA217_0==RULE_ID||(LA217_0>=185 && LA217_0<=206)) ) {
alt217=1;
}
else if ( (LA217_0==94) ) {
alt217=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 217, 0, input);
throw nvae;
}
switch (alt217) {
case 1 :
// InternalContextMappingDSL.g:8255:5: ( (lv_mapKeyType_21_0= ruleType ) )
{
// InternalContextMappingDSL.g:8255:5: ( (lv_mapKeyType_21_0= ruleType ) )
// InternalContextMappingDSL.g:8256:6: (lv_mapKeyType_21_0= ruleType )
{
// InternalContextMappingDSL.g:8256:6: (lv_mapKeyType_21_0= ruleType )
// InternalContextMappingDSL.g:8257:7: lv_mapKeyType_21_0= ruleType
{
newCompositeNode(grammarAccess.getComplexTypeAccess().getMapKeyTypeTypeParserRuleCall_4_2_0_0());
pushFollow(FOLLOW_43);
lv_mapKeyType_21_0=ruleType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getComplexTypeRule());
}
set(
current,
"mapKeyType",
lv_mapKeyType_21_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Type");
afterParserOrEnumRuleCall();
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:8275:5: (otherlv_22= '@' ( (otherlv_23= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:8275:5: (otherlv_22= '@' ( (otherlv_23= RULE_ID ) ) )
// InternalContextMappingDSL.g:8276:6: otherlv_22= '@' ( (otherlv_23= RULE_ID ) )
{
otherlv_22=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_22, grammarAccess.getComplexTypeAccess().getCommercialAtKeyword_4_2_1_0());
// InternalContextMappingDSL.g:8280:6: ( (otherlv_23= RULE_ID ) )
// InternalContextMappingDSL.g:8281:7: (otherlv_23= RULE_ID )
{
// InternalContextMappingDSL.g:8281:7: (otherlv_23= RULE_ID )
// InternalContextMappingDSL.g:8282:8: otherlv_23= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getComplexTypeRule());
}
otherlv_23=(Token)match(input,RULE_ID,FOLLOW_43);
newLeafNode(otherlv_23, grammarAccess.getComplexTypeAccess().getMapKeyDomainObjectTypeSimpleDomainObjectCrossReference_4_2_1_1_0());
}
}
}
}
break;
}
otherlv_24=(Token)match(input,24,FOLLOW_129);
newLeafNode(otherlv_24, grammarAccess.getComplexTypeAccess().getCommaKeyword_4_3());
// InternalContextMappingDSL.g:8299:4: ( (otherlv_25= '@' ( (otherlv_26= RULE_ID ) ) ) | ( (lv_type_27_0= ruleType ) ) | ( ( (lv_type_28_0= ruleType ) ) otherlv_29= '<' otherlv_30= '@' ( (otherlv_31= RULE_ID ) ) otherlv_32= '>' ) )
int alt218=3;
alt218 = dfa218.predict(input);
switch (alt218) {
case 1 :
// InternalContextMappingDSL.g:8300:5: (otherlv_25= '@' ( (otherlv_26= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:8300:5: (otherlv_25= '@' ( (otherlv_26= RULE_ID ) ) )
// InternalContextMappingDSL.g:8301:6: otherlv_25= '@' ( (otherlv_26= RULE_ID ) )
{
otherlv_25=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_25, grammarAccess.getComplexTypeAccess().getCommercialAtKeyword_4_4_0_0());
// InternalContextMappingDSL.g:8305:6: ( (otherlv_26= RULE_ID ) )
// InternalContextMappingDSL.g:8306:7: (otherlv_26= RULE_ID )
{
// InternalContextMappingDSL.g:8306:7: (otherlv_26= RULE_ID )
// InternalContextMappingDSL.g:8307:8: otherlv_26= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getComplexTypeRule());
}
otherlv_26=(Token)match(input,RULE_ID,FOLLOW_128);
newLeafNode(otherlv_26, grammarAccess.getComplexTypeAccess().getDomainObjectTypeSimpleDomainObjectCrossReference_4_4_0_1_0());
}
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:8320:5: ( (lv_type_27_0= ruleType ) )
{
// InternalContextMappingDSL.g:8320:5: ( (lv_type_27_0= ruleType ) )
// InternalContextMappingDSL.g:8321:6: (lv_type_27_0= ruleType )
{
// InternalContextMappingDSL.g:8321:6: (lv_type_27_0= ruleType )
// InternalContextMappingDSL.g:8322:7: lv_type_27_0= ruleType
{
newCompositeNode(grammarAccess.getComplexTypeAccess().getTypeTypeParserRuleCall_4_4_1_0());
pushFollow(FOLLOW_128);
lv_type_27_0=ruleType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getComplexTypeRule());
}
set(
current,
"type",
lv_type_27_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Type");
afterParserOrEnumRuleCall();
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:8340:5: ( ( (lv_type_28_0= ruleType ) ) otherlv_29= '<' otherlv_30= '@' ( (otherlv_31= RULE_ID ) ) otherlv_32= '>' )
{
// InternalContextMappingDSL.g:8340:5: ( ( (lv_type_28_0= ruleType ) ) otherlv_29= '<' otherlv_30= '@' ( (otherlv_31= RULE_ID ) ) otherlv_32= '>' )
// InternalContextMappingDSL.g:8341:6: ( (lv_type_28_0= ruleType ) ) otherlv_29= '<' otherlv_30= '@' ( (otherlv_31= RULE_ID ) ) otherlv_32= '>'
{
// InternalContextMappingDSL.g:8341:6: ( (lv_type_28_0= ruleType ) )
// InternalContextMappingDSL.g:8342:7: (lv_type_28_0= ruleType )
{
// InternalContextMappingDSL.g:8342:7: (lv_type_28_0= ruleType )
// InternalContextMappingDSL.g:8343:8: lv_type_28_0= ruleType
{
newCompositeNode(grammarAccess.getComplexTypeAccess().getTypeTypeParserRuleCall_4_4_2_0_0());
pushFollow(FOLLOW_126);
lv_type_28_0=ruleType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getComplexTypeRule());
}
set(
current,
"type",
lv_type_28_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Type");
afterParserOrEnumRuleCall();
}
}
otherlv_29=(Token)match(input,118,FOLLOW_127);
newLeafNode(otherlv_29, grammarAccess.getComplexTypeAccess().getLessThanSignKeyword_4_4_2_1());
otherlv_30=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_30, grammarAccess.getComplexTypeAccess().getCommercialAtKeyword_4_4_2_2());
// InternalContextMappingDSL.g:8368:6: ( (otherlv_31= RULE_ID ) )
// InternalContextMappingDSL.g:8369:7: (otherlv_31= RULE_ID )
{
// InternalContextMappingDSL.g:8369:7: (otherlv_31= RULE_ID )
// InternalContextMappingDSL.g:8370:8: otherlv_31= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getComplexTypeRule());
}
otherlv_31=(Token)match(input,RULE_ID,FOLLOW_128);
newLeafNode(otherlv_31, grammarAccess.getComplexTypeAccess().getDomainObjectTypeSimpleDomainObjectCrossReference_4_4_2_3_0());
}
}
otherlv_32=(Token)match(input,119,FOLLOW_128);
newLeafNode(otherlv_32, grammarAccess.getComplexTypeAccess().getGreaterThanSignKeyword_4_4_2_4());
}
}
break;
}
otherlv_33=(Token)match(input,119,FOLLOW_2);
newLeafNode(otherlv_33, grammarAccess.getComplexTypeAccess().getGreaterThanSignKeyword_4_5());
}
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleComplexType"
// $ANTLR start "entryRuleSimpleDomainObject"
// InternalContextMappingDSL.g:8396:1: entryRuleSimpleDomainObject returns [EObject current=null] : iv_ruleSimpleDomainObject= ruleSimpleDomainObject EOF ;
public final EObject entryRuleSimpleDomainObject() throws RecognitionException {
EObject current = null;
EObject iv_ruleSimpleDomainObject = null;
try {
// InternalContextMappingDSL.g:8396:59: (iv_ruleSimpleDomainObject= ruleSimpleDomainObject EOF )
// InternalContextMappingDSL.g:8397:2: iv_ruleSimpleDomainObject= ruleSimpleDomainObject EOF
{
newCompositeNode(grammarAccess.getSimpleDomainObjectRule());
pushFollow(FOLLOW_1);
iv_ruleSimpleDomainObject=ruleSimpleDomainObject();
state._fsp--;
current =iv_ruleSimpleDomainObject;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleSimpleDomainObject"
// $ANTLR start "ruleSimpleDomainObject"
// InternalContextMappingDSL.g:8403:1: ruleSimpleDomainObject returns [EObject current=null] : (this_BasicType_0= ruleBasicType | this_Enum_1= ruleEnum | this_DomainObject_2= ruleDomainObject | this_DataTransferObject_3= ruleDataTransferObject | this_Trait_4= ruleTrait ) ;
public final EObject ruleSimpleDomainObject() throws RecognitionException {
EObject current = null;
EObject this_BasicType_0 = null;
EObject this_Enum_1 = null;
EObject this_DomainObject_2 = null;
EObject this_DataTransferObject_3 = null;
EObject this_Trait_4 = null;
enterRule();
try {
// InternalContextMappingDSL.g:8409:2: ( (this_BasicType_0= ruleBasicType | this_Enum_1= ruleEnum | this_DomainObject_2= ruleDomainObject | this_DataTransferObject_3= ruleDataTransferObject | this_Trait_4= ruleTrait ) )
// InternalContextMappingDSL.g:8410:2: (this_BasicType_0= ruleBasicType | this_Enum_1= ruleEnum | this_DomainObject_2= ruleDomainObject | this_DataTransferObject_3= ruleDataTransferObject | this_Trait_4= ruleTrait )
{
// InternalContextMappingDSL.g:8410:2: (this_BasicType_0= ruleBasicType | this_Enum_1= ruleEnum | this_DomainObject_2= ruleDomainObject | this_DataTransferObject_3= ruleDataTransferObject | this_Trait_4= ruleTrait )
int alt220=5;
alt220 = dfa220.predict(input);
switch (alt220) {
case 1 :
// InternalContextMappingDSL.g:8411:3: this_BasicType_0= ruleBasicType
{
newCompositeNode(grammarAccess.getSimpleDomainObjectAccess().getBasicTypeParserRuleCall_0());
pushFollow(FOLLOW_2);
this_BasicType_0=ruleBasicType();
state._fsp--;
current = this_BasicType_0;
afterParserOrEnumRuleCall();
}
break;
case 2 :
// InternalContextMappingDSL.g:8420:3: this_Enum_1= ruleEnum
{
newCompositeNode(grammarAccess.getSimpleDomainObjectAccess().getEnumParserRuleCall_1());
pushFollow(FOLLOW_2);
this_Enum_1=ruleEnum();
state._fsp--;
current = this_Enum_1;
afterParserOrEnumRuleCall();
}
break;
case 3 :
// InternalContextMappingDSL.g:8429:3: this_DomainObject_2= ruleDomainObject
{
newCompositeNode(grammarAccess.getSimpleDomainObjectAccess().getDomainObjectParserRuleCall_2());
pushFollow(FOLLOW_2);
this_DomainObject_2=ruleDomainObject();
state._fsp--;
current = this_DomainObject_2;
afterParserOrEnumRuleCall();
}
break;
case 4 :
// InternalContextMappingDSL.g:8438:3: this_DataTransferObject_3= ruleDataTransferObject
{
newCompositeNode(grammarAccess.getSimpleDomainObjectAccess().getDataTransferObjectParserRuleCall_3());
pushFollow(FOLLOW_2);
this_DataTransferObject_3=ruleDataTransferObject();
state._fsp--;
current = this_DataTransferObject_3;
afterParserOrEnumRuleCall();
}
break;
case 5 :
// InternalContextMappingDSL.g:8447:3: this_Trait_4= ruleTrait
{
newCompositeNode(grammarAccess.getSimpleDomainObjectAccess().getTraitParserRuleCall_4());
pushFollow(FOLLOW_2);
this_Trait_4=ruleTrait();
state._fsp--;
current = this_Trait_4;
afterParserOrEnumRuleCall();
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleSimpleDomainObject"
// $ANTLR start "entryRuleDomainObject"
// InternalContextMappingDSL.g:8459:1: entryRuleDomainObject returns [EObject current=null] : iv_ruleDomainObject= ruleDomainObject EOF ;
public final EObject entryRuleDomainObject() throws RecognitionException {
EObject current = null;
EObject iv_ruleDomainObject = null;
try {
// InternalContextMappingDSL.g:8459:53: (iv_ruleDomainObject= ruleDomainObject EOF )
// InternalContextMappingDSL.g:8460:2: iv_ruleDomainObject= ruleDomainObject EOF
{
newCompositeNode(grammarAccess.getDomainObjectRule());
pushFollow(FOLLOW_1);
iv_ruleDomainObject=ruleDomainObject();
state._fsp--;
current =iv_ruleDomainObject;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleDomainObject"
// $ANTLR start "ruleDomainObject"
// InternalContextMappingDSL.g:8466:1: ruleDomainObject returns [EObject current=null] : (this_Entity_0= ruleEntity | this_ValueObject_1= ruleValueObject | this_Event_2= ruleEvent ) ;
public final EObject ruleDomainObject() throws RecognitionException {
EObject current = null;
EObject this_Entity_0 = null;
EObject this_ValueObject_1 = null;
EObject this_Event_2 = null;
enterRule();
try {
// InternalContextMappingDSL.g:8472:2: ( (this_Entity_0= ruleEntity | this_ValueObject_1= ruleValueObject | this_Event_2= ruleEvent ) )
// InternalContextMappingDSL.g:8473:2: (this_Entity_0= ruleEntity | this_ValueObject_1= ruleValueObject | this_Event_2= ruleEvent )
{
// InternalContextMappingDSL.g:8473:2: (this_Entity_0= ruleEntity | this_ValueObject_1= ruleValueObject | this_Event_2= ruleEvent )
int alt221=3;
switch ( input.LA(1) ) {
case RULE_ML_COMMENT:
{
switch ( input.LA(2) ) {
case RULE_STRING:
{
switch ( input.LA(3) ) {
case 120:
{
switch ( input.LA(4) ) {
case 136:
{
alt221=2;
}
break;
case 121:
{
alt221=1;
}
break;
case 139:
case 140:
{
alt221=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 221, 3, input);
throw nvae;
}
}
break;
case 121:
{
alt221=1;
}
break;
case 139:
case 140:
{
alt221=3;
}
break;
case 136:
{
alt221=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 221, 2, input);
throw nvae;
}
}
break;
case 120:
{
switch ( input.LA(3) ) {
case 136:
{
alt221=2;
}
break;
case 121:
{
alt221=1;
}
break;
case 139:
case 140:
{
alt221=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 221, 3, input);
throw nvae;
}
}
break;
case 121:
{
alt221=1;
}
break;
case 139:
case 140:
{
alt221=3;
}
break;
case 136:
{
alt221=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 221, 1, input);
throw nvae;
}
}
break;
case RULE_STRING:
{
switch ( input.LA(2) ) {
case 120:
{
switch ( input.LA(3) ) {
case 136:
{
alt221=2;
}
break;
case 121:
{
alt221=1;
}
break;
case 139:
case 140:
{
alt221=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 221, 3, input);
throw nvae;
}
}
break;
case 121:
{
alt221=1;
}
break;
case 139:
case 140:
{
alt221=3;
}
break;
case 136:
{
alt221=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 221, 2, input);
throw nvae;
}
}
break;
case 120:
{
switch ( input.LA(2) ) {
case 136:
{
alt221=2;
}
break;
case 121:
{
alt221=1;
}
break;
case 139:
case 140:
{
alt221=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 221, 3, input);
throw nvae;
}
}
break;
case 121:
{
alt221=1;
}
break;
case 136:
{
alt221=2;
}
break;
case 139:
case 140:
{
alt221=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 221, 0, input);
throw nvae;
}
switch (alt221) {
case 1 :
// InternalContextMappingDSL.g:8474:3: this_Entity_0= ruleEntity
{
newCompositeNode(grammarAccess.getDomainObjectAccess().getEntityParserRuleCall_0());
pushFollow(FOLLOW_2);
this_Entity_0=ruleEntity();
state._fsp--;
current = this_Entity_0;
afterParserOrEnumRuleCall();
}
break;
case 2 :
// InternalContextMappingDSL.g:8483:3: this_ValueObject_1= ruleValueObject
{
newCompositeNode(grammarAccess.getDomainObjectAccess().getValueObjectParserRuleCall_1());
pushFollow(FOLLOW_2);
this_ValueObject_1=ruleValueObject();
state._fsp--;
current = this_ValueObject_1;
afterParserOrEnumRuleCall();
}
break;
case 3 :
// InternalContextMappingDSL.g:8492:3: this_Event_2= ruleEvent
{
newCompositeNode(grammarAccess.getDomainObjectAccess().getEventParserRuleCall_2());
pushFollow(FOLLOW_2);
this_Event_2=ruleEvent();
state._fsp--;
current = this_Event_2;
afterParserOrEnumRuleCall();
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleDomainObject"
// $ANTLR start "entryRuleEntity"
// InternalContextMappingDSL.g:8504:1: entryRuleEntity returns [EObject current=null] : iv_ruleEntity= ruleEntity EOF ;
public final EObject entryRuleEntity() throws RecognitionException {
EObject current = null;
EObject iv_ruleEntity = null;
try {
// InternalContextMappingDSL.g:8504:47: (iv_ruleEntity= ruleEntity EOF )
// InternalContextMappingDSL.g:8505:2: iv_ruleEntity= ruleEntity EOF
{
newCompositeNode(grammarAccess.getEntityRule());
pushFollow(FOLLOW_1);
iv_ruleEntity=ruleEntity();
state._fsp--;
current =iv_ruleEntity;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleEntity"
// $ANTLR start "ruleEntity"
// InternalContextMappingDSL.g:8511:1: ruleEntity returns [EObject current=null] : ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'Entity' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_57_0= ruleAttribute ) ) | ( (lv_references_58_0= ruleReference ) ) | ( (lv_operations_59_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_60_0= ruleRepository ) )? otherlv_61= '}' )? ) ;
public final EObject ruleEntity() throws RecognitionException {
EObject current = null;
Token lv_comment_0_0=null;
Token lv_doc_1_0=null;
Token lv_abstract_2_0=null;
Token otherlv_3=null;
Token lv_name_4_0=null;
Token otherlv_5=null;
Token otherlv_6=null;
Token otherlv_7=null;
Token otherlv_9=null;
Token otherlv_10=null;
Token otherlv_11=null;
Token otherlv_12=null;
Token otherlv_13=null;
Token otherlv_14=null;
Token lv_notOptimisticLocking_17_0=null;
Token otherlv_18=null;
Token otherlv_19=null;
Token lv_notAuditable_20_0=null;
Token otherlv_21=null;
Token otherlv_22=null;
Token lv_cache_23_0=null;
Token this_NOT_24=null;
Token otherlv_25=null;
Token lv_gapClass_26_0=null;
Token lv_noGapClass_27_0=null;
Token lv_scaffold_28_0=null;
Token otherlv_29=null;
Token otherlv_30=null;
Token lv_hint_31_0=null;
Token otherlv_32=null;
Token otherlv_33=null;
Token lv_databaseTable_34_0=null;
Token otherlv_35=null;
Token otherlv_36=null;
Token lv_discriminatorValue_37_0=null;
Token otherlv_38=null;
Token otherlv_39=null;
Token lv_discriminatorColumn_40_0=null;
Token otherlv_41=null;
Token otherlv_42=null;
Token otherlv_44=null;
Token otherlv_45=null;
Token lv_discriminatorLength_46_0=null;
Token otherlv_47=null;
Token otherlv_48=null;
Token otherlv_50=null;
Token otherlv_51=null;
Token lv_validate_52_0=null;
Token lv_aggregateRoot_53_0=null;
Token otherlv_54=null;
Token otherlv_55=null;
Token otherlv_56=null;
Token otherlv_61=null;
AntlrDatatypeRuleToken lv_extendsName_8_0 = null;
AntlrDatatypeRuleToken lv_package_15_0 = null;
Enumerator lv_discriminatorType_43_0 = null;
Enumerator lv_inheritanceType_49_0 = null;
EObject lv_attributes_57_0 = null;
EObject lv_references_58_0 = null;
EObject lv_operations_59_0 = null;
EObject lv_repository_60_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:8517:2: ( ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'Entity' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_57_0= ruleAttribute ) ) | ( (lv_references_58_0= ruleReference ) ) | ( (lv_operations_59_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_60_0= ruleRepository ) )? otherlv_61= '}' )? ) )
// InternalContextMappingDSL.g:8518:2: ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'Entity' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_57_0= ruleAttribute ) ) | ( (lv_references_58_0= ruleReference ) ) | ( (lv_operations_59_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_60_0= ruleRepository ) )? otherlv_61= '}' )? )
{
// InternalContextMappingDSL.g:8518:2: ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'Entity' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_57_0= ruleAttribute ) ) | ( (lv_references_58_0= ruleReference ) ) | ( (lv_operations_59_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_60_0= ruleRepository ) )? otherlv_61= '}' )? )
// InternalContextMappingDSL.g:8519:3: ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'Entity' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_57_0= ruleAttribute ) ) | ( (lv_references_58_0= ruleReference ) ) | ( (lv_operations_59_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_60_0= ruleRepository ) )? otherlv_61= '}' )?
{
// InternalContextMappingDSL.g:8519:3: ( (lv_comment_0_0= RULE_ML_COMMENT ) )?
int alt222=2;
int LA222_0 = input.LA(1);
if ( (LA222_0==RULE_ML_COMMENT) ) {
alt222=1;
}
switch (alt222) {
case 1 :
// InternalContextMappingDSL.g:8520:4: (lv_comment_0_0= RULE_ML_COMMENT )
{
// InternalContextMappingDSL.g:8520:4: (lv_comment_0_0= RULE_ML_COMMENT )
// InternalContextMappingDSL.g:8521:5: lv_comment_0_0= RULE_ML_COMMENT
{
lv_comment_0_0=(Token)match(input,RULE_ML_COMMENT,FOLLOW_130);
newLeafNode(lv_comment_0_0, grammarAccess.getEntityAccess().getCommentML_COMMENTTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
setWithLastConsumed(
current,
"comment",
lv_comment_0_0,
"org.eclipse.xtext.common.Terminals.ML_COMMENT");
}
}
break;
}
// InternalContextMappingDSL.g:8537:3: ( (lv_doc_1_0= RULE_STRING ) )?
int alt223=2;
int LA223_0 = input.LA(1);
if ( (LA223_0==RULE_STRING) ) {
alt223=1;
}
switch (alt223) {
case 1 :
// InternalContextMappingDSL.g:8538:4: (lv_doc_1_0= RULE_STRING )
{
// InternalContextMappingDSL.g:8538:4: (lv_doc_1_0= RULE_STRING )
// InternalContextMappingDSL.g:8539:5: lv_doc_1_0= RULE_STRING
{
lv_doc_1_0=(Token)match(input,RULE_STRING,FOLLOW_131);
newLeafNode(lv_doc_1_0, grammarAccess.getEntityAccess().getDocSTRINGTerminalRuleCall_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_1_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
// InternalContextMappingDSL.g:8555:3: ( (lv_abstract_2_0= 'abstract' ) )?
int alt224=2;
int LA224_0 = input.LA(1);
if ( (LA224_0==120) ) {
alt224=1;
}
switch (alt224) {
case 1 :
// InternalContextMappingDSL.g:8556:4: (lv_abstract_2_0= 'abstract' )
{
// InternalContextMappingDSL.g:8556:4: (lv_abstract_2_0= 'abstract' )
// InternalContextMappingDSL.g:8557:5: lv_abstract_2_0= 'abstract'
{
lv_abstract_2_0=(Token)match(input,120,FOLLOW_132);
newLeafNode(lv_abstract_2_0, grammarAccess.getEntityAccess().getAbstractAbstractKeyword_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
setWithLastConsumed(current, "abstract", true, "abstract");
}
}
break;
}
otherlv_3=(Token)match(input,121,FOLLOW_10);
newLeafNode(otherlv_3, grammarAccess.getEntityAccess().getEntityKeyword_3());
// InternalContextMappingDSL.g:8573:3: ( (lv_name_4_0= RULE_ID ) )
// InternalContextMappingDSL.g:8574:4: (lv_name_4_0= RULE_ID )
{
// InternalContextMappingDSL.g:8574:4: (lv_name_4_0= RULE_ID )
// InternalContextMappingDSL.g:8575:5: lv_name_4_0= RULE_ID
{
lv_name_4_0=(Token)match(input,RULE_ID,FOLLOW_133);
newLeafNode(lv_name_4_0, grammarAccess.getEntityAccess().getNameIDTerminalRuleCall_4_0());
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
setWithLastConsumed(
current,
"name",
lv_name_4_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:8591:3: (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )?
int alt226=2;
int LA226_0 = input.LA(1);
if ( (LA226_0==122) ) {
alt226=1;
}
switch (alt226) {
case 1 :
// InternalContextMappingDSL.g:8592:4: otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) )
{
otherlv_5=(Token)match(input,122,FOLLOW_105);
newLeafNode(otherlv_5, grammarAccess.getEntityAccess().getExtendsKeyword_5_0());
// InternalContextMappingDSL.g:8596:4: ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) )
int alt225=2;
int LA225_0 = input.LA(1);
if ( (LA225_0==94) ) {
alt225=1;
}
else if ( (LA225_0==RULE_ID) ) {
alt225=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 225, 0, input);
throw nvae;
}
switch (alt225) {
case 1 :
// InternalContextMappingDSL.g:8597:5: (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:8597:5: (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) )
// InternalContextMappingDSL.g:8598:6: otherlv_6= '@' ( (otherlv_7= RULE_ID ) )
{
otherlv_6=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_6, grammarAccess.getEntityAccess().getCommercialAtKeyword_5_1_0_0());
// InternalContextMappingDSL.g:8602:6: ( (otherlv_7= RULE_ID ) )
// InternalContextMappingDSL.g:8603:7: (otherlv_7= RULE_ID )
{
// InternalContextMappingDSL.g:8603:7: (otherlv_7= RULE_ID )
// InternalContextMappingDSL.g:8604:8: otherlv_7= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
otherlv_7=(Token)match(input,RULE_ID,FOLLOW_134);
newLeafNode(otherlv_7, grammarAccess.getEntityAccess().getExtendsEntityCrossReference_5_1_0_1_0());
}
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:8617:5: ( (lv_extendsName_8_0= ruleJavaIdentifier ) )
{
// InternalContextMappingDSL.g:8617:5: ( (lv_extendsName_8_0= ruleJavaIdentifier ) )
// InternalContextMappingDSL.g:8618:6: (lv_extendsName_8_0= ruleJavaIdentifier )
{
// InternalContextMappingDSL.g:8618:6: (lv_extendsName_8_0= ruleJavaIdentifier )
// InternalContextMappingDSL.g:8619:7: lv_extendsName_8_0= ruleJavaIdentifier
{
newCompositeNode(grammarAccess.getEntityAccess().getExtendsNameJavaIdentifierParserRuleCall_5_1_1_0());
pushFollow(FOLLOW_134);
lv_extendsName_8_0=ruleJavaIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getEntityRule());
}
set(
current,
"extendsName",
lv_extendsName_8_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.JavaIdentifier");
afterParserOrEnumRuleCall();
}
}
}
break;
}
}
break;
}
// InternalContextMappingDSL.g:8638:3: (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )*
loop228:
do {
int alt228=2;
int LA228_0 = input.LA(1);
if ( (LA228_0==123) ) {
alt228=1;
}
switch (alt228) {
case 1 :
// InternalContextMappingDSL.g:8639:4: otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) )
{
otherlv_9=(Token)match(input,123,FOLLOW_105);
newLeafNode(otherlv_9, grammarAccess.getEntityAccess().getWithKeyword_6_0());
// InternalContextMappingDSL.g:8643:4: (otherlv_10= '@' )?
int alt227=2;
int LA227_0 = input.LA(1);
if ( (LA227_0==94) ) {
alt227=1;
}
switch (alt227) {
case 1 :
// InternalContextMappingDSL.g:8644:5: otherlv_10= '@'
{
otherlv_10=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_10, grammarAccess.getEntityAccess().getCommercialAtKeyword_6_1());
}
break;
}
// InternalContextMappingDSL.g:8649:4: ( (otherlv_11= RULE_ID ) )
// InternalContextMappingDSL.g:8650:5: (otherlv_11= RULE_ID )
{
// InternalContextMappingDSL.g:8650:5: (otherlv_11= RULE_ID )
// InternalContextMappingDSL.g:8651:6: otherlv_11= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
otherlv_11=(Token)match(input,RULE_ID,FOLLOW_134);
newLeafNode(otherlv_11, grammarAccess.getEntityAccess().getTraitsTraitCrossReference_6_2_0());
}
}
}
break;
default :
break loop228;
}
} while (true);
// InternalContextMappingDSL.g:8663:3: (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_57_0= ruleAttribute ) ) | ( (lv_references_58_0= ruleReference ) ) | ( (lv_operations_59_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_60_0= ruleRepository ) )? otherlv_61= '}' )?
int alt238=2;
int LA238_0 = input.LA(1);
if ( (LA238_0==RULE_OPEN) ) {
alt238=1;
}
switch (alt238) {
case 1 :
// InternalContextMappingDSL.g:8664:4: otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_57_0= ruleAttribute ) ) | ( (lv_references_58_0= ruleReference ) ) | ( (lv_operations_59_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_60_0= ruleRepository ) )? otherlv_61= '}'
{
otherlv_12=(Token)match(input,RULE_OPEN,FOLLOW_135);
newLeafNode(otherlv_12, grammarAccess.getEntityAccess().getLeftCurlyBracketKeyword_7_0());
// InternalContextMappingDSL.g:8668:4: (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )?
int alt229=2;
int LA229_0 = input.LA(1);
if ( (LA229_0==124) ) {
int LA229_1 = input.LA(2);
if ( (LA229_1==21) ) {
alt229=1;
}
}
switch (alt229) {
case 1 :
// InternalContextMappingDSL.g:8669:5: otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) )
{
otherlv_13=(Token)match(input,124,FOLLOW_89);
newLeafNode(otherlv_13, grammarAccess.getEntityAccess().getPackageKeyword_7_1_0());
otherlv_14=(Token)match(input,21,FOLLOW_10);
newLeafNode(otherlv_14, grammarAccess.getEntityAccess().getEqualsSignKeyword_7_1_1());
// InternalContextMappingDSL.g:8677:5: ( (lv_package_15_0= ruleJavaIdentifier ) )
// InternalContextMappingDSL.g:8678:6: (lv_package_15_0= ruleJavaIdentifier )
{
// InternalContextMappingDSL.g:8678:6: (lv_package_15_0= ruleJavaIdentifier )
// InternalContextMappingDSL.g:8679:7: lv_package_15_0= ruleJavaIdentifier
{
newCompositeNode(grammarAccess.getEntityAccess().getPackageJavaIdentifierParserRuleCall_7_1_2_0());
pushFollow(FOLLOW_135);
lv_package_15_0=ruleJavaIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getEntityRule());
}
set(
current,
"package",
lv_package_15_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.JavaIdentifier");
afterParserOrEnumRuleCall();
}
}
}
break;
}
// InternalContextMappingDSL.g:8697:4: ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:8698:5: ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:8698:5: ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:8699:6: ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getEntityAccess().getUnorderedGroup_7_2());
// InternalContextMappingDSL.g:8702:6: ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:8703:7: ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:8703:7: ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) ) ) )*
loop235:
do {
int alt235=16;
alt235 = dfa235.predict(input);
switch (alt235) {
case 1 :
// InternalContextMappingDSL.g:8704:5: ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) )
{
// InternalContextMappingDSL.g:8704:5: ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) )
// InternalContextMappingDSL.g:8705:6: {...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 0) ) {
throw new FailedPredicateException(input, "ruleEntity", "getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 0)");
}
// InternalContextMappingDSL.g:8705:106: ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) )
// InternalContextMappingDSL.g:8706:7: ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) )
{
getUnorderedGroupHelper().select(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 0);
// InternalContextMappingDSL.g:8709:10: ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) )
// InternalContextMappingDSL.g:8709:11: {...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleEntity", "true");
}
// InternalContextMappingDSL.g:8709:20: ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' )
int alt230=2;
int LA230_0 = input.LA(1);
if ( (LA230_0==RULE_NOT) ) {
alt230=1;
}
else if ( (LA230_0==125) ) {
alt230=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 230, 0, input);
throw nvae;
}
switch (alt230) {
case 1 :
// InternalContextMappingDSL.g:8709:21: ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' )
{
// InternalContextMappingDSL.g:8709:21: ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' )
// InternalContextMappingDSL.g:8710:11: ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking'
{
// InternalContextMappingDSL.g:8710:11: ( (lv_notOptimisticLocking_17_0= RULE_NOT ) )
// InternalContextMappingDSL.g:8711:12: (lv_notOptimisticLocking_17_0= RULE_NOT )
{
// InternalContextMappingDSL.g:8711:12: (lv_notOptimisticLocking_17_0= RULE_NOT )
// InternalContextMappingDSL.g:8712:13: lv_notOptimisticLocking_17_0= RULE_NOT
{
lv_notOptimisticLocking_17_0=(Token)match(input,RULE_NOT,FOLLOW_136);
newLeafNode(lv_notOptimisticLocking_17_0, grammarAccess.getEntityAccess().getNotOptimisticLockingNOTTerminalRuleCall_7_2_0_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
setWithLastConsumed(
current,
"notOptimisticLocking",
true,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.NOT");
}
}
otherlv_18=(Token)match(input,125,FOLLOW_135);
newLeafNode(otherlv_18, grammarAccess.getEntityAccess().getOptimisticLockingKeyword_7_2_0_0_1());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:8734:10: otherlv_19= 'optimisticLocking'
{
otherlv_19=(Token)match(input,125,FOLLOW_135);
newLeafNode(otherlv_19, grammarAccess.getEntityAccess().getOptimisticLockingKeyword_7_2_0_1());
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getEntityAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:8744:5: ({...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) ) )
{
// InternalContextMappingDSL.g:8744:5: ({...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) ) )
// InternalContextMappingDSL.g:8745:6: {...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 1) ) {
throw new FailedPredicateException(input, "ruleEntity", "getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 1)");
}
// InternalContextMappingDSL.g:8745:106: ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) )
// InternalContextMappingDSL.g:8746:7: ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) )
{
getUnorderedGroupHelper().select(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 1);
// InternalContextMappingDSL.g:8749:10: ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) )
// InternalContextMappingDSL.g:8749:11: {...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleEntity", "true");
}
// InternalContextMappingDSL.g:8749:20: ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' )
int alt231=2;
int LA231_0 = input.LA(1);
if ( (LA231_0==RULE_NOT) ) {
alt231=1;
}
else if ( (LA231_0==126) ) {
alt231=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 231, 0, input);
throw nvae;
}
switch (alt231) {
case 1 :
// InternalContextMappingDSL.g:8749:21: ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' )
{
// InternalContextMappingDSL.g:8749:21: ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' )
// InternalContextMappingDSL.g:8750:11: ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable'
{
// InternalContextMappingDSL.g:8750:11: ( (lv_notAuditable_20_0= RULE_NOT ) )
// InternalContextMappingDSL.g:8751:12: (lv_notAuditable_20_0= RULE_NOT )
{
// InternalContextMappingDSL.g:8751:12: (lv_notAuditable_20_0= RULE_NOT )
// InternalContextMappingDSL.g:8752:13: lv_notAuditable_20_0= RULE_NOT
{
lv_notAuditable_20_0=(Token)match(input,RULE_NOT,FOLLOW_137);
newLeafNode(lv_notAuditable_20_0, grammarAccess.getEntityAccess().getNotAuditableNOTTerminalRuleCall_7_2_1_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
setWithLastConsumed(
current,
"notAuditable",
true,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.NOT");
}
}
otherlv_21=(Token)match(input,126,FOLLOW_135);
newLeafNode(otherlv_21, grammarAccess.getEntityAccess().getAuditableKeyword_7_2_1_0_1());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:8774:10: otherlv_22= 'auditable'
{
otherlv_22=(Token)match(input,126,FOLLOW_135);
newLeafNode(otherlv_22, grammarAccess.getEntityAccess().getAuditableKeyword_7_2_1_1());
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getEntityAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:8784:5: ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) )
{
// InternalContextMappingDSL.g:8784:5: ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) )
// InternalContextMappingDSL.g:8785:6: {...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 2) ) {
throw new FailedPredicateException(input, "ruleEntity", "getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 2)");
}
// InternalContextMappingDSL.g:8785:106: ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) )
// InternalContextMappingDSL.g:8786:7: ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 2);
// InternalContextMappingDSL.g:8789:10: ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) )
// InternalContextMappingDSL.g:8789:11: {...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleEntity", "true");
}
// InternalContextMappingDSL.g:8789:20: ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) )
int alt232=2;
int LA232_0 = input.LA(1);
if ( (LA232_0==108) ) {
alt232=1;
}
else if ( (LA232_0==RULE_NOT) ) {
alt232=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 232, 0, input);
throw nvae;
}
switch (alt232) {
case 1 :
// InternalContextMappingDSL.g:8789:21: ( (lv_cache_23_0= 'cache' ) )
{
// InternalContextMappingDSL.g:8789:21: ( (lv_cache_23_0= 'cache' ) )
// InternalContextMappingDSL.g:8790:11: (lv_cache_23_0= 'cache' )
{
// InternalContextMappingDSL.g:8790:11: (lv_cache_23_0= 'cache' )
// InternalContextMappingDSL.g:8791:12: lv_cache_23_0= 'cache'
{
lv_cache_23_0=(Token)match(input,108,FOLLOW_135);
newLeafNode(lv_cache_23_0, grammarAccess.getEntityAccess().getCacheCacheKeyword_7_2_2_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
setWithLastConsumed(current, "cache", true, "cache");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:8804:10: (this_NOT_24= RULE_NOT otherlv_25= 'cache' )
{
// InternalContextMappingDSL.g:8804:10: (this_NOT_24= RULE_NOT otherlv_25= 'cache' )
// InternalContextMappingDSL.g:8805:11: this_NOT_24= RULE_NOT otherlv_25= 'cache'
{
this_NOT_24=(Token)match(input,RULE_NOT,FOLLOW_138);
newLeafNode(this_NOT_24, grammarAccess.getEntityAccess().getNOTTerminalRuleCall_7_2_2_1_0());
otherlv_25=(Token)match(input,108,FOLLOW_135);
newLeafNode(otherlv_25, grammarAccess.getEntityAccess().getCacheKeyword_7_2_2_1_1());
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getEntityAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:8820:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) )
{
// InternalContextMappingDSL.g:8820:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) )
// InternalContextMappingDSL.g:8821:6: {...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 3) ) {
throw new FailedPredicateException(input, "ruleEntity", "getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 3)");
}
// InternalContextMappingDSL.g:8821:106: ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) )
// InternalContextMappingDSL.g:8822:7: ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 3);
// InternalContextMappingDSL.g:8825:10: ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) )
// InternalContextMappingDSL.g:8825:11: {...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleEntity", "true");
}
// InternalContextMappingDSL.g:8825:20: ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) )
int alt233=2;
int LA233_0 = input.LA(1);
if ( (LA233_0==86) ) {
alt233=1;
}
else if ( (LA233_0==87) ) {
alt233=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 233, 0, input);
throw nvae;
}
switch (alt233) {
case 1 :
// InternalContextMappingDSL.g:8825:21: ( (lv_gapClass_26_0= 'gap' ) )
{
// InternalContextMappingDSL.g:8825:21: ( (lv_gapClass_26_0= 'gap' ) )
// InternalContextMappingDSL.g:8826:11: (lv_gapClass_26_0= 'gap' )
{
// InternalContextMappingDSL.g:8826:11: (lv_gapClass_26_0= 'gap' )
// InternalContextMappingDSL.g:8827:12: lv_gapClass_26_0= 'gap'
{
lv_gapClass_26_0=(Token)match(input,86,FOLLOW_135);
newLeafNode(lv_gapClass_26_0, grammarAccess.getEntityAccess().getGapClassGapKeyword_7_2_3_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
setWithLastConsumed(current, "gapClass", true, "gap");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:8840:10: ( (lv_noGapClass_27_0= 'nogap' ) )
{
// InternalContextMappingDSL.g:8840:10: ( (lv_noGapClass_27_0= 'nogap' ) )
// InternalContextMappingDSL.g:8841:11: (lv_noGapClass_27_0= 'nogap' )
{
// InternalContextMappingDSL.g:8841:11: (lv_noGapClass_27_0= 'nogap' )
// InternalContextMappingDSL.g:8842:12: lv_noGapClass_27_0= 'nogap'
{
lv_noGapClass_27_0=(Token)match(input,87,FOLLOW_135);
newLeafNode(lv_noGapClass_27_0, grammarAccess.getEntityAccess().getNoGapClassNogapKeyword_7_2_3_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
setWithLastConsumed(current, "noGapClass", true, "nogap");
}
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getEntityAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 5 :
// InternalContextMappingDSL.g:8860:5: ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) )
{
// InternalContextMappingDSL.g:8860:5: ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) )
// InternalContextMappingDSL.g:8861:6: {...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 4) ) {
throw new FailedPredicateException(input, "ruleEntity", "getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 4)");
}
// InternalContextMappingDSL.g:8861:106: ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) )
// InternalContextMappingDSL.g:8862:7: ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 4);
// InternalContextMappingDSL.g:8865:10: ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) )
// InternalContextMappingDSL.g:8865:11: {...}? => ( (lv_scaffold_28_0= 'scaffold' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleEntity", "true");
}
// InternalContextMappingDSL.g:8865:20: ( (lv_scaffold_28_0= 'scaffold' ) )
// InternalContextMappingDSL.g:8865:21: (lv_scaffold_28_0= 'scaffold' )
{
// InternalContextMappingDSL.g:8865:21: (lv_scaffold_28_0= 'scaffold' )
// InternalContextMappingDSL.g:8866:11: lv_scaffold_28_0= 'scaffold'
{
lv_scaffold_28_0=(Token)match(input,90,FOLLOW_135);
newLeafNode(lv_scaffold_28_0, grammarAccess.getEntityAccess().getScaffoldScaffoldKeyword_7_2_4_0());
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
setWithLastConsumed(current, "scaffold", true, "scaffold");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getEntityAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 6 :
// InternalContextMappingDSL.g:8883:5: ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:8883:5: ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:8884:6: {...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 5) ) {
throw new FailedPredicateException(input, "ruleEntity", "getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 5)");
}
// InternalContextMappingDSL.g:8884:106: ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:8885:7: ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 5);
// InternalContextMappingDSL.g:8888:10: ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:8888:11: {...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleEntity", "true");
}
// InternalContextMappingDSL.g:8888:20: (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:8888:21: otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) )
{
otherlv_29=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_29, grammarAccess.getEntityAccess().getHintKeyword_7_2_5_0());
otherlv_30=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_30, grammarAccess.getEntityAccess().getEqualsSignKeyword_7_2_5_1());
// InternalContextMappingDSL.g:8896:10: ( (lv_hint_31_0= RULE_STRING ) )
// InternalContextMappingDSL.g:8897:11: (lv_hint_31_0= RULE_STRING )
{
// InternalContextMappingDSL.g:8897:11: (lv_hint_31_0= RULE_STRING )
// InternalContextMappingDSL.g:8898:12: lv_hint_31_0= RULE_STRING
{
lv_hint_31_0=(Token)match(input,RULE_STRING,FOLLOW_135);
newLeafNode(lv_hint_31_0, grammarAccess.getEntityAccess().getHintSTRINGTerminalRuleCall_7_2_5_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_31_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getEntityAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 7 :
// InternalContextMappingDSL.g:8920:5: ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:8920:5: ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:8921:6: {...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 6) ) {
throw new FailedPredicateException(input, "ruleEntity", "getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 6)");
}
// InternalContextMappingDSL.g:8921:106: ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:8922:7: ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 6);
// InternalContextMappingDSL.g:8925:10: ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:8925:11: {...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleEntity", "true");
}
// InternalContextMappingDSL.g:8925:20: (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:8925:21: otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) )
{
otherlv_32=(Token)match(input,127,FOLLOW_89);
newLeafNode(otherlv_32, grammarAccess.getEntityAccess().getDatabaseTableKeyword_7_2_6_0());
otherlv_33=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_33, grammarAccess.getEntityAccess().getEqualsSignKeyword_7_2_6_1());
// InternalContextMappingDSL.g:8933:10: ( (lv_databaseTable_34_0= RULE_STRING ) )
// InternalContextMappingDSL.g:8934:11: (lv_databaseTable_34_0= RULE_STRING )
{
// InternalContextMappingDSL.g:8934:11: (lv_databaseTable_34_0= RULE_STRING )
// InternalContextMappingDSL.g:8935:12: lv_databaseTable_34_0= RULE_STRING
{
lv_databaseTable_34_0=(Token)match(input,RULE_STRING,FOLLOW_135);
newLeafNode(lv_databaseTable_34_0, grammarAccess.getEntityAccess().getDatabaseTableSTRINGTerminalRuleCall_7_2_6_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
setWithLastConsumed(
current,
"databaseTable",
lv_databaseTable_34_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getEntityAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 8 :
// InternalContextMappingDSL.g:8957:5: ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:8957:5: ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:8958:6: {...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 7) ) {
throw new FailedPredicateException(input, "ruleEntity", "getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 7)");
}
// InternalContextMappingDSL.g:8958:106: ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:8959:7: ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 7);
// InternalContextMappingDSL.g:8962:10: ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:8962:11: {...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleEntity", "true");
}
// InternalContextMappingDSL.g:8962:20: (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:8962:21: otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) )
{
otherlv_35=(Token)match(input,128,FOLLOW_89);
newLeafNode(otherlv_35, grammarAccess.getEntityAccess().getDiscriminatorValueKeyword_7_2_7_0());
otherlv_36=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_36, grammarAccess.getEntityAccess().getEqualsSignKeyword_7_2_7_1());
// InternalContextMappingDSL.g:8970:10: ( (lv_discriminatorValue_37_0= RULE_STRING ) )
// InternalContextMappingDSL.g:8971:11: (lv_discriminatorValue_37_0= RULE_STRING )
{
// InternalContextMappingDSL.g:8971:11: (lv_discriminatorValue_37_0= RULE_STRING )
// InternalContextMappingDSL.g:8972:12: lv_discriminatorValue_37_0= RULE_STRING
{
lv_discriminatorValue_37_0=(Token)match(input,RULE_STRING,FOLLOW_135);
newLeafNode(lv_discriminatorValue_37_0, grammarAccess.getEntityAccess().getDiscriminatorValueSTRINGTerminalRuleCall_7_2_7_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
setWithLastConsumed(
current,
"discriminatorValue",
lv_discriminatorValue_37_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getEntityAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 9 :
// InternalContextMappingDSL.g:8994:5: ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:8994:5: ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:8995:6: {...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 8) ) {
throw new FailedPredicateException(input, "ruleEntity", "getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 8)");
}
// InternalContextMappingDSL.g:8995:106: ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:8996:7: ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 8);
// InternalContextMappingDSL.g:8999:10: ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:8999:11: {...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleEntity", "true");
}
// InternalContextMappingDSL.g:8999:20: (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:8999:21: otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) )
{
otherlv_38=(Token)match(input,129,FOLLOW_89);
newLeafNode(otherlv_38, grammarAccess.getEntityAccess().getDiscriminatorColumnKeyword_7_2_8_0());
otherlv_39=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_39, grammarAccess.getEntityAccess().getEqualsSignKeyword_7_2_8_1());
// InternalContextMappingDSL.g:9007:10: ( (lv_discriminatorColumn_40_0= RULE_STRING ) )
// InternalContextMappingDSL.g:9008:11: (lv_discriminatorColumn_40_0= RULE_STRING )
{
// InternalContextMappingDSL.g:9008:11: (lv_discriminatorColumn_40_0= RULE_STRING )
// InternalContextMappingDSL.g:9009:12: lv_discriminatorColumn_40_0= RULE_STRING
{
lv_discriminatorColumn_40_0=(Token)match(input,RULE_STRING,FOLLOW_135);
newLeafNode(lv_discriminatorColumn_40_0, grammarAccess.getEntityAccess().getDiscriminatorColumnSTRINGTerminalRuleCall_7_2_8_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
setWithLastConsumed(
current,
"discriminatorColumn",
lv_discriminatorColumn_40_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getEntityAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 10 :
// InternalContextMappingDSL.g:9031:5: ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) )
{
// InternalContextMappingDSL.g:9031:5: ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) )
// InternalContextMappingDSL.g:9032:6: {...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 9) ) {
throw new FailedPredicateException(input, "ruleEntity", "getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 9)");
}
// InternalContextMappingDSL.g:9032:106: ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) )
// InternalContextMappingDSL.g:9033:7: ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 9);
// InternalContextMappingDSL.g:9036:10: ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) )
// InternalContextMappingDSL.g:9036:11: {...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleEntity", "true");
}
// InternalContextMappingDSL.g:9036:20: (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) )
// InternalContextMappingDSL.g:9036:21: otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) )
{
otherlv_41=(Token)match(input,130,FOLLOW_89);
newLeafNode(otherlv_41, grammarAccess.getEntityAccess().getDiscriminatorTypeKeyword_7_2_9_0());
otherlv_42=(Token)match(input,21,FOLLOW_139);
newLeafNode(otherlv_42, grammarAccess.getEntityAccess().getEqualsSignKeyword_7_2_9_1());
// InternalContextMappingDSL.g:9044:10: ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) )
// InternalContextMappingDSL.g:9045:11: (lv_discriminatorType_43_0= ruleDiscriminatorType )
{
// InternalContextMappingDSL.g:9045:11: (lv_discriminatorType_43_0= ruleDiscriminatorType )
// InternalContextMappingDSL.g:9046:12: lv_discriminatorType_43_0= ruleDiscriminatorType
{
newCompositeNode(grammarAccess.getEntityAccess().getDiscriminatorTypeDiscriminatorTypeEnumRuleCall_7_2_9_2_0());
pushFollow(FOLLOW_135);
lv_discriminatorType_43_0=ruleDiscriminatorType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getEntityRule());
}
set(
current,
"discriminatorType",
lv_discriminatorType_43_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.DiscriminatorType");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getEntityAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 11 :
// InternalContextMappingDSL.g:9069:5: ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:9069:5: ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:9070:6: {...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 10) ) {
throw new FailedPredicateException(input, "ruleEntity", "getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 10)");
}
// InternalContextMappingDSL.g:9070:107: ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:9071:7: ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 10);
// InternalContextMappingDSL.g:9074:10: ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:9074:11: {...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleEntity", "true");
}
// InternalContextMappingDSL.g:9074:20: (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:9074:21: otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) )
{
otherlv_44=(Token)match(input,131,FOLLOW_89);
newLeafNode(otherlv_44, grammarAccess.getEntityAccess().getDiscriminatorLengthKeyword_7_2_10_0());
otherlv_45=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_45, grammarAccess.getEntityAccess().getEqualsSignKeyword_7_2_10_1());
// InternalContextMappingDSL.g:9082:10: ( (lv_discriminatorLength_46_0= RULE_STRING ) )
// InternalContextMappingDSL.g:9083:11: (lv_discriminatorLength_46_0= RULE_STRING )
{
// InternalContextMappingDSL.g:9083:11: (lv_discriminatorLength_46_0= RULE_STRING )
// InternalContextMappingDSL.g:9084:12: lv_discriminatorLength_46_0= RULE_STRING
{
lv_discriminatorLength_46_0=(Token)match(input,RULE_STRING,FOLLOW_135);
newLeafNode(lv_discriminatorLength_46_0, grammarAccess.getEntityAccess().getDiscriminatorLengthSTRINGTerminalRuleCall_7_2_10_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
setWithLastConsumed(
current,
"discriminatorLength",
lv_discriminatorLength_46_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getEntityAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 12 :
// InternalContextMappingDSL.g:9106:5: ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) )
{
// InternalContextMappingDSL.g:9106:5: ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) )
// InternalContextMappingDSL.g:9107:6: {...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 11) ) {
throw new FailedPredicateException(input, "ruleEntity", "getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 11)");
}
// InternalContextMappingDSL.g:9107:107: ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) )
// InternalContextMappingDSL.g:9108:7: ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 11);
// InternalContextMappingDSL.g:9111:10: ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) )
// InternalContextMappingDSL.g:9111:11: {...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleEntity", "true");
}
// InternalContextMappingDSL.g:9111:20: (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) )
// InternalContextMappingDSL.g:9111:21: otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) )
{
otherlv_47=(Token)match(input,132,FOLLOW_89);
newLeafNode(otherlv_47, grammarAccess.getEntityAccess().getInheritanceTypeKeyword_7_2_11_0());
otherlv_48=(Token)match(input,21,FOLLOW_140);
newLeafNode(otherlv_48, grammarAccess.getEntityAccess().getEqualsSignKeyword_7_2_11_1());
// InternalContextMappingDSL.g:9119:10: ( (lv_inheritanceType_49_0= ruleInheritanceType ) )
// InternalContextMappingDSL.g:9120:11: (lv_inheritanceType_49_0= ruleInheritanceType )
{
// InternalContextMappingDSL.g:9120:11: (lv_inheritanceType_49_0= ruleInheritanceType )
// InternalContextMappingDSL.g:9121:12: lv_inheritanceType_49_0= ruleInheritanceType
{
newCompositeNode(grammarAccess.getEntityAccess().getInheritanceTypeInheritanceTypeEnumRuleCall_7_2_11_2_0());
pushFollow(FOLLOW_135);
lv_inheritanceType_49_0=ruleInheritanceType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getEntityRule());
}
set(
current,
"inheritanceType",
lv_inheritanceType_49_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.InheritanceType");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getEntityAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 13 :
// InternalContextMappingDSL.g:9144:5: ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:9144:5: ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:9145:6: {...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 12) ) {
throw new FailedPredicateException(input, "ruleEntity", "getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 12)");
}
// InternalContextMappingDSL.g:9145:107: ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:9146:7: ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 12);
// InternalContextMappingDSL.g:9149:10: ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:9149:11: {...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleEntity", "true");
}
// InternalContextMappingDSL.g:9149:20: (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:9149:21: otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) )
{
otherlv_50=(Token)match(input,133,FOLLOW_89);
newLeafNode(otherlv_50, grammarAccess.getEntityAccess().getValidateKeyword_7_2_12_0());
otherlv_51=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_51, grammarAccess.getEntityAccess().getEqualsSignKeyword_7_2_12_1());
// InternalContextMappingDSL.g:9157:10: ( (lv_validate_52_0= RULE_STRING ) )
// InternalContextMappingDSL.g:9158:11: (lv_validate_52_0= RULE_STRING )
{
// InternalContextMappingDSL.g:9158:11: (lv_validate_52_0= RULE_STRING )
// InternalContextMappingDSL.g:9159:12: lv_validate_52_0= RULE_STRING
{
lv_validate_52_0=(Token)match(input,RULE_STRING,FOLLOW_135);
newLeafNode(lv_validate_52_0, grammarAccess.getEntityAccess().getValidateSTRINGTerminalRuleCall_7_2_12_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
setWithLastConsumed(
current,
"validate",
lv_validate_52_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getEntityAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 14 :
// InternalContextMappingDSL.g:9181:5: ({...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) ) )
{
// InternalContextMappingDSL.g:9181:5: ({...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) ) )
// InternalContextMappingDSL.g:9182:6: {...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 13) ) {
throw new FailedPredicateException(input, "ruleEntity", "getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 13)");
}
// InternalContextMappingDSL.g:9182:107: ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) )
// InternalContextMappingDSL.g:9183:7: ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 13);
// InternalContextMappingDSL.g:9186:10: ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) )
// InternalContextMappingDSL.g:9186:11: {...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleEntity", "true");
}
// InternalContextMappingDSL.g:9186:20: ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) )
// InternalContextMappingDSL.g:9186:21: (lv_aggregateRoot_53_0= 'aggregateRoot' )
{
// InternalContextMappingDSL.g:9186:21: (lv_aggregateRoot_53_0= 'aggregateRoot' )
// InternalContextMappingDSL.g:9187:11: lv_aggregateRoot_53_0= 'aggregateRoot'
{
lv_aggregateRoot_53_0=(Token)match(input,134,FOLLOW_135);
newLeafNode(lv_aggregateRoot_53_0, grammarAccess.getEntityAccess().getAggregateRootAggregateRootKeyword_7_2_13_0());
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
setWithLastConsumed(current, "aggregateRoot", true, "aggregateRoot");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getEntityAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 15 :
// InternalContextMappingDSL.g:9204:5: ({...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) ) )
{
// InternalContextMappingDSL.g:9204:5: ({...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) ) )
// InternalContextMappingDSL.g:9205:6: {...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 14) ) {
throw new FailedPredicateException(input, "ruleEntity", "getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 14)");
}
// InternalContextMappingDSL.g:9205:107: ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) )
// InternalContextMappingDSL.g:9206:7: ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 14);
// InternalContextMappingDSL.g:9209:10: ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) )
// InternalContextMappingDSL.g:9209:11: {...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleEntity", "true");
}
// InternalContextMappingDSL.g:9209:20: (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) )
// InternalContextMappingDSL.g:9209:21: otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) )
{
otherlv_54=(Token)match(input,135,FOLLOW_105);
newLeafNode(otherlv_54, grammarAccess.getEntityAccess().getBelongsToKeyword_7_2_14_0());
// InternalContextMappingDSL.g:9213:10: ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) )
// InternalContextMappingDSL.g:9214:11: (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) )
{
// InternalContextMappingDSL.g:9214:11: (otherlv_55= '@' )?
int alt234=2;
int LA234_0 = input.LA(1);
if ( (LA234_0==94) ) {
alt234=1;
}
switch (alt234) {
case 1 :
// InternalContextMappingDSL.g:9215:12: otherlv_55= '@'
{
otherlv_55=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_55, grammarAccess.getEntityAccess().getCommercialAtKeyword_7_2_14_1_0());
}
break;
}
// InternalContextMappingDSL.g:9220:11: ( (otherlv_56= RULE_ID ) )
// InternalContextMappingDSL.g:9221:12: (otherlv_56= RULE_ID )
{
// InternalContextMappingDSL.g:9221:12: (otherlv_56= RULE_ID )
// InternalContextMappingDSL.g:9222:13: otherlv_56= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getEntityRule());
}
otherlv_56=(Token)match(input,RULE_ID,FOLLOW_135);
newLeafNode(otherlv_56, grammarAccess.getEntityAccess().getBelongsToDomainObjectCrossReference_7_2_14_1_1_0());
}
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getEntityAccess().getUnorderedGroup_7_2());
}
}
}
break;
default :
break loop235;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getEntityAccess().getUnorderedGroup_7_2());
}
// InternalContextMappingDSL.g:9247:4: ( ( (lv_attributes_57_0= ruleAttribute ) ) | ( (lv_references_58_0= ruleReference ) ) | ( (lv_operations_59_0= ruleDomainObjectOperation ) ) )*
loop236:
do {
int alt236=4;
switch ( input.LA(1) ) {
case RULE_STRING:
{
switch ( input.LA(2) ) {
case 142:
case 143:
{
alt236=3;
}
break;
case RULE_REF:
{
alt236=2;
}
break;
case RULE_ID:
case 124:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
case 247:
case 248:
case 249:
{
alt236=1;
}
break;
}
}
break;
case RULE_ID:
case 124:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
case 247:
case 248:
case 249:
{
alt236=1;
}
break;
case RULE_REF:
{
alt236=2;
}
break;
case 142:
case 143:
{
alt236=3;
}
break;
}
switch (alt236) {
case 1 :
// InternalContextMappingDSL.g:9248:5: ( (lv_attributes_57_0= ruleAttribute ) )
{
// InternalContextMappingDSL.g:9248:5: ( (lv_attributes_57_0= ruleAttribute ) )
// InternalContextMappingDSL.g:9249:6: (lv_attributes_57_0= ruleAttribute )
{
// InternalContextMappingDSL.g:9249:6: (lv_attributes_57_0= ruleAttribute )
// InternalContextMappingDSL.g:9250:7: lv_attributes_57_0= ruleAttribute
{
newCompositeNode(grammarAccess.getEntityAccess().getAttributesAttributeParserRuleCall_7_3_0_0());
pushFollow(FOLLOW_141);
lv_attributes_57_0=ruleAttribute();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getEntityRule());
}
add(
current,
"attributes",
lv_attributes_57_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Attribute");
afterParserOrEnumRuleCall();
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:9268:5: ( (lv_references_58_0= ruleReference ) )
{
// InternalContextMappingDSL.g:9268:5: ( (lv_references_58_0= ruleReference ) )
// InternalContextMappingDSL.g:9269:6: (lv_references_58_0= ruleReference )
{
// InternalContextMappingDSL.g:9269:6: (lv_references_58_0= ruleReference )
// InternalContextMappingDSL.g:9270:7: lv_references_58_0= ruleReference
{
newCompositeNode(grammarAccess.getEntityAccess().getReferencesReferenceParserRuleCall_7_3_1_0());
pushFollow(FOLLOW_141);
lv_references_58_0=ruleReference();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getEntityRule());
}
add(
current,
"references",
lv_references_58_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Reference");
afterParserOrEnumRuleCall();
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:9288:5: ( (lv_operations_59_0= ruleDomainObjectOperation ) )
{
// InternalContextMappingDSL.g:9288:5: ( (lv_operations_59_0= ruleDomainObjectOperation ) )
// InternalContextMappingDSL.g:9289:6: (lv_operations_59_0= ruleDomainObjectOperation )
{
// InternalContextMappingDSL.g:9289:6: (lv_operations_59_0= ruleDomainObjectOperation )
// InternalContextMappingDSL.g:9290:7: lv_operations_59_0= ruleDomainObjectOperation
{
newCompositeNode(grammarAccess.getEntityAccess().getOperationsDomainObjectOperationParserRuleCall_7_3_2_0());
pushFollow(FOLLOW_141);
lv_operations_59_0=ruleDomainObjectOperation();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getEntityRule());
}
add(
current,
"operations",
lv_operations_59_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.DomainObjectOperation");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop236;
}
} while (true);
// InternalContextMappingDSL.g:9308:4: ( (lv_repository_60_0= ruleRepository ) )?
int alt237=2;
int LA237_0 = input.LA(1);
if ( (LA237_0==RULE_STRING||LA237_0==181) ) {
alt237=1;
}
switch (alt237) {
case 1 :
// InternalContextMappingDSL.g:9309:5: (lv_repository_60_0= ruleRepository )
{
// InternalContextMappingDSL.g:9309:5: (lv_repository_60_0= ruleRepository )
// InternalContextMappingDSL.g:9310:6: lv_repository_60_0= ruleRepository
{
newCompositeNode(grammarAccess.getEntityAccess().getRepositoryRepositoryParserRuleCall_7_4_0());
pushFollow(FOLLOW_36);
lv_repository_60_0=ruleRepository();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getEntityRule());
}
set(
current,
"repository",
lv_repository_60_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Repository");
afterParserOrEnumRuleCall();
}
}
break;
}
otherlv_61=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(otherlv_61, grammarAccess.getEntityAccess().getRightCurlyBracketKeyword_7_5());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleEntity"
// $ANTLR start "entryRuleValueObject"
// InternalContextMappingDSL.g:9336:1: entryRuleValueObject returns [EObject current=null] : iv_ruleValueObject= ruleValueObject EOF ;
public final EObject entryRuleValueObject() throws RecognitionException {
EObject current = null;
EObject iv_ruleValueObject = null;
try {
// InternalContextMappingDSL.g:9336:52: (iv_ruleValueObject= ruleValueObject EOF )
// InternalContextMappingDSL.g:9337:2: iv_ruleValueObject= ruleValueObject EOF
{
newCompositeNode(grammarAccess.getValueObjectRule());
pushFollow(FOLLOW_1);
iv_ruleValueObject=ruleValueObject();
state._fsp--;
current =iv_ruleValueObject;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleValueObject"
// $ANTLR start "ruleValueObject"
// InternalContextMappingDSL.g:9343:1: ruleValueObject returns [EObject current=null] : ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'ValueObject' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_60_0= ruleAttribute ) ) | ( (lv_references_61_0= ruleReference ) ) | ( (lv_operations_62_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_63_0= ruleRepository ) )? otherlv_64= '}' )? ) ;
public final EObject ruleValueObject() throws RecognitionException {
EObject current = null;
Token lv_comment_0_0=null;
Token lv_doc_1_0=null;
Token lv_abstract_2_0=null;
Token otherlv_3=null;
Token lv_name_4_0=null;
Token otherlv_5=null;
Token otherlv_6=null;
Token otherlv_7=null;
Token otherlv_9=null;
Token otherlv_10=null;
Token otherlv_11=null;
Token otherlv_12=null;
Token otherlv_13=null;
Token otherlv_14=null;
Token lv_notOptimisticLocking_17_0=null;
Token otherlv_18=null;
Token otherlv_19=null;
Token lv_notImmutable_20_0=null;
Token otherlv_21=null;
Token otherlv_22=null;
Token lv_cache_23_0=null;
Token this_NOT_24=null;
Token otherlv_25=null;
Token lv_gapClass_26_0=null;
Token lv_noGapClass_27_0=null;
Token lv_scaffold_28_0=null;
Token otherlv_29=null;
Token otherlv_30=null;
Token lv_hint_31_0=null;
Token otherlv_32=null;
Token otherlv_33=null;
Token lv_databaseTable_34_0=null;
Token otherlv_35=null;
Token otherlv_36=null;
Token lv_discriminatorValue_37_0=null;
Token otherlv_38=null;
Token otherlv_39=null;
Token lv_discriminatorColumn_40_0=null;
Token otherlv_41=null;
Token otherlv_42=null;
Token otherlv_44=null;
Token otherlv_45=null;
Token lv_discriminatorLength_46_0=null;
Token otherlv_47=null;
Token otherlv_48=null;
Token otherlv_50=null;
Token otherlv_51=null;
Token lv_validate_52_0=null;
Token lv_persistent_53_0=null;
Token lv_notPersistent_54_0=null;
Token otherlv_55=null;
Token lv_aggregateRoot_56_0=null;
Token otherlv_57=null;
Token otherlv_58=null;
Token otherlv_59=null;
Token otherlv_64=null;
AntlrDatatypeRuleToken lv_extendsName_8_0 = null;
AntlrDatatypeRuleToken lv_package_15_0 = null;
Enumerator lv_discriminatorType_43_0 = null;
Enumerator lv_inheritanceType_49_0 = null;
EObject lv_attributes_60_0 = null;
EObject lv_references_61_0 = null;
EObject lv_operations_62_0 = null;
EObject lv_repository_63_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:9349:2: ( ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'ValueObject' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_60_0= ruleAttribute ) ) | ( (lv_references_61_0= ruleReference ) ) | ( (lv_operations_62_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_63_0= ruleRepository ) )? otherlv_64= '}' )? ) )
// InternalContextMappingDSL.g:9350:2: ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'ValueObject' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_60_0= ruleAttribute ) ) | ( (lv_references_61_0= ruleReference ) ) | ( (lv_operations_62_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_63_0= ruleRepository ) )? otherlv_64= '}' )? )
{
// InternalContextMappingDSL.g:9350:2: ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'ValueObject' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_60_0= ruleAttribute ) ) | ( (lv_references_61_0= ruleReference ) ) | ( (lv_operations_62_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_63_0= ruleRepository ) )? otherlv_64= '}' )? )
// InternalContextMappingDSL.g:9351:3: ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'ValueObject' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_60_0= ruleAttribute ) ) | ( (lv_references_61_0= ruleReference ) ) | ( (lv_operations_62_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_63_0= ruleRepository ) )? otherlv_64= '}' )?
{
// InternalContextMappingDSL.g:9351:3: ( (lv_comment_0_0= RULE_ML_COMMENT ) )?
int alt239=2;
int LA239_0 = input.LA(1);
if ( (LA239_0==RULE_ML_COMMENT) ) {
alt239=1;
}
switch (alt239) {
case 1 :
// InternalContextMappingDSL.g:9352:4: (lv_comment_0_0= RULE_ML_COMMENT )
{
// InternalContextMappingDSL.g:9352:4: (lv_comment_0_0= RULE_ML_COMMENT )
// InternalContextMappingDSL.g:9353:5: lv_comment_0_0= RULE_ML_COMMENT
{
lv_comment_0_0=(Token)match(input,RULE_ML_COMMENT,FOLLOW_142);
newLeafNode(lv_comment_0_0, grammarAccess.getValueObjectAccess().getCommentML_COMMENTTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(
current,
"comment",
lv_comment_0_0,
"org.eclipse.xtext.common.Terminals.ML_COMMENT");
}
}
break;
}
// InternalContextMappingDSL.g:9369:3: ( (lv_doc_1_0= RULE_STRING ) )?
int alt240=2;
int LA240_0 = input.LA(1);
if ( (LA240_0==RULE_STRING) ) {
alt240=1;
}
switch (alt240) {
case 1 :
// InternalContextMappingDSL.g:9370:4: (lv_doc_1_0= RULE_STRING )
{
// InternalContextMappingDSL.g:9370:4: (lv_doc_1_0= RULE_STRING )
// InternalContextMappingDSL.g:9371:5: lv_doc_1_0= RULE_STRING
{
lv_doc_1_0=(Token)match(input,RULE_STRING,FOLLOW_143);
newLeafNode(lv_doc_1_0, grammarAccess.getValueObjectAccess().getDocSTRINGTerminalRuleCall_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_1_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
// InternalContextMappingDSL.g:9387:3: ( (lv_abstract_2_0= 'abstract' ) )?
int alt241=2;
int LA241_0 = input.LA(1);
if ( (LA241_0==120) ) {
alt241=1;
}
switch (alt241) {
case 1 :
// InternalContextMappingDSL.g:9388:4: (lv_abstract_2_0= 'abstract' )
{
// InternalContextMappingDSL.g:9388:4: (lv_abstract_2_0= 'abstract' )
// InternalContextMappingDSL.g:9389:5: lv_abstract_2_0= 'abstract'
{
lv_abstract_2_0=(Token)match(input,120,FOLLOW_144);
newLeafNode(lv_abstract_2_0, grammarAccess.getValueObjectAccess().getAbstractAbstractKeyword_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(current, "abstract", true, "abstract");
}
}
break;
}
otherlv_3=(Token)match(input,136,FOLLOW_10);
newLeafNode(otherlv_3, grammarAccess.getValueObjectAccess().getValueObjectKeyword_3());
// InternalContextMappingDSL.g:9405:3: ( (lv_name_4_0= RULE_ID ) )
// InternalContextMappingDSL.g:9406:4: (lv_name_4_0= RULE_ID )
{
// InternalContextMappingDSL.g:9406:4: (lv_name_4_0= RULE_ID )
// InternalContextMappingDSL.g:9407:5: lv_name_4_0= RULE_ID
{
lv_name_4_0=(Token)match(input,RULE_ID,FOLLOW_133);
newLeafNode(lv_name_4_0, grammarAccess.getValueObjectAccess().getNameIDTerminalRuleCall_4_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(
current,
"name",
lv_name_4_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:9423:3: (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )?
int alt243=2;
int LA243_0 = input.LA(1);
if ( (LA243_0==122) ) {
alt243=1;
}
switch (alt243) {
case 1 :
// InternalContextMappingDSL.g:9424:4: otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) )
{
otherlv_5=(Token)match(input,122,FOLLOW_105);
newLeafNode(otherlv_5, grammarAccess.getValueObjectAccess().getExtendsKeyword_5_0());
// InternalContextMappingDSL.g:9428:4: ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) )
int alt242=2;
int LA242_0 = input.LA(1);
if ( (LA242_0==94) ) {
alt242=1;
}
else if ( (LA242_0==RULE_ID) ) {
alt242=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 242, 0, input);
throw nvae;
}
switch (alt242) {
case 1 :
// InternalContextMappingDSL.g:9429:5: (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:9429:5: (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) )
// InternalContextMappingDSL.g:9430:6: otherlv_6= '@' ( (otherlv_7= RULE_ID ) )
{
otherlv_6=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_6, grammarAccess.getValueObjectAccess().getCommercialAtKeyword_5_1_0_0());
// InternalContextMappingDSL.g:9434:6: ( (otherlv_7= RULE_ID ) )
// InternalContextMappingDSL.g:9435:7: (otherlv_7= RULE_ID )
{
// InternalContextMappingDSL.g:9435:7: (otherlv_7= RULE_ID )
// InternalContextMappingDSL.g:9436:8: otherlv_7= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
otherlv_7=(Token)match(input,RULE_ID,FOLLOW_134);
newLeafNode(otherlv_7, grammarAccess.getValueObjectAccess().getExtendsValueObjectCrossReference_5_1_0_1_0());
}
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:9449:5: ( (lv_extendsName_8_0= ruleJavaIdentifier ) )
{
// InternalContextMappingDSL.g:9449:5: ( (lv_extendsName_8_0= ruleJavaIdentifier ) )
// InternalContextMappingDSL.g:9450:6: (lv_extendsName_8_0= ruleJavaIdentifier )
{
// InternalContextMappingDSL.g:9450:6: (lv_extendsName_8_0= ruleJavaIdentifier )
// InternalContextMappingDSL.g:9451:7: lv_extendsName_8_0= ruleJavaIdentifier
{
newCompositeNode(grammarAccess.getValueObjectAccess().getExtendsNameJavaIdentifierParserRuleCall_5_1_1_0());
pushFollow(FOLLOW_134);
lv_extendsName_8_0=ruleJavaIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getValueObjectRule());
}
set(
current,
"extendsName",
lv_extendsName_8_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.JavaIdentifier");
afterParserOrEnumRuleCall();
}
}
}
break;
}
}
break;
}
// InternalContextMappingDSL.g:9470:3: (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )*
loop245:
do {
int alt245=2;
int LA245_0 = input.LA(1);
if ( (LA245_0==123) ) {
alt245=1;
}
switch (alt245) {
case 1 :
// InternalContextMappingDSL.g:9471:4: otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) )
{
otherlv_9=(Token)match(input,123,FOLLOW_105);
newLeafNode(otherlv_9, grammarAccess.getValueObjectAccess().getWithKeyword_6_0());
// InternalContextMappingDSL.g:9475:4: (otherlv_10= '@' )?
int alt244=2;
int LA244_0 = input.LA(1);
if ( (LA244_0==94) ) {
alt244=1;
}
switch (alt244) {
case 1 :
// InternalContextMappingDSL.g:9476:5: otherlv_10= '@'
{
otherlv_10=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_10, grammarAccess.getValueObjectAccess().getCommercialAtKeyword_6_1());
}
break;
}
// InternalContextMappingDSL.g:9481:4: ( (otherlv_11= RULE_ID ) )
// InternalContextMappingDSL.g:9482:5: (otherlv_11= RULE_ID )
{
// InternalContextMappingDSL.g:9482:5: (otherlv_11= RULE_ID )
// InternalContextMappingDSL.g:9483:6: otherlv_11= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
otherlv_11=(Token)match(input,RULE_ID,FOLLOW_134);
newLeafNode(otherlv_11, grammarAccess.getValueObjectAccess().getTraitsTraitCrossReference_6_2_0());
}
}
}
break;
default :
break loop245;
}
} while (true);
// InternalContextMappingDSL.g:9495:3: (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_60_0= ruleAttribute ) ) | ( (lv_references_61_0= ruleReference ) ) | ( (lv_operations_62_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_63_0= ruleRepository ) )? otherlv_64= '}' )?
int alt256=2;
int LA256_0 = input.LA(1);
if ( (LA256_0==RULE_OPEN) ) {
alt256=1;
}
switch (alt256) {
case 1 :
// InternalContextMappingDSL.g:9496:4: otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_60_0= ruleAttribute ) ) | ( (lv_references_61_0= ruleReference ) ) | ( (lv_operations_62_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_63_0= ruleRepository ) )? otherlv_64= '}'
{
otherlv_12=(Token)match(input,RULE_OPEN,FOLLOW_145);
newLeafNode(otherlv_12, grammarAccess.getValueObjectAccess().getLeftCurlyBracketKeyword_7_0());
// InternalContextMappingDSL.g:9500:4: (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )?
int alt246=2;
int LA246_0 = input.LA(1);
if ( (LA246_0==124) ) {
int LA246_1 = input.LA(2);
if ( (LA246_1==21) ) {
alt246=1;
}
}
switch (alt246) {
case 1 :
// InternalContextMappingDSL.g:9501:5: otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) )
{
otherlv_13=(Token)match(input,124,FOLLOW_89);
newLeafNode(otherlv_13, grammarAccess.getValueObjectAccess().getPackageKeyword_7_1_0());
otherlv_14=(Token)match(input,21,FOLLOW_10);
newLeafNode(otherlv_14, grammarAccess.getValueObjectAccess().getEqualsSignKeyword_7_1_1());
// InternalContextMappingDSL.g:9509:5: ( (lv_package_15_0= ruleJavaIdentifier ) )
// InternalContextMappingDSL.g:9510:6: (lv_package_15_0= ruleJavaIdentifier )
{
// InternalContextMappingDSL.g:9510:6: (lv_package_15_0= ruleJavaIdentifier )
// InternalContextMappingDSL.g:9511:7: lv_package_15_0= ruleJavaIdentifier
{
newCompositeNode(grammarAccess.getValueObjectAccess().getPackageJavaIdentifierParserRuleCall_7_1_2_0());
pushFollow(FOLLOW_145);
lv_package_15_0=ruleJavaIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getValueObjectRule());
}
set(
current,
"package",
lv_package_15_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.JavaIdentifier");
afterParserOrEnumRuleCall();
}
}
}
break;
}
// InternalContextMappingDSL.g:9529:4: ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:9530:5: ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:9530:5: ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:9531:6: ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
// InternalContextMappingDSL.g:9534:6: ( ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:9535:7: ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:9535:7: ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) ) ) )*
loop253:
do {
int alt253=17;
alt253 = dfa253.predict(input);
switch (alt253) {
case 1 :
// InternalContextMappingDSL.g:9536:5: ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) )
{
// InternalContextMappingDSL.g:9536:5: ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) )
// InternalContextMappingDSL.g:9537:6: {...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 0) ) {
throw new FailedPredicateException(input, "ruleValueObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 0)");
}
// InternalContextMappingDSL.g:9537:111: ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) )
// InternalContextMappingDSL.g:9538:7: ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) )
{
getUnorderedGroupHelper().select(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 0);
// InternalContextMappingDSL.g:9541:10: ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) )
// InternalContextMappingDSL.g:9541:11: {...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleValueObject", "true");
}
// InternalContextMappingDSL.g:9541:20: ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' )
int alt247=2;
int LA247_0 = input.LA(1);
if ( (LA247_0==RULE_NOT) ) {
alt247=1;
}
else if ( (LA247_0==125) ) {
alt247=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 247, 0, input);
throw nvae;
}
switch (alt247) {
case 1 :
// InternalContextMappingDSL.g:9541:21: ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' )
{
// InternalContextMappingDSL.g:9541:21: ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' )
// InternalContextMappingDSL.g:9542:11: ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking'
{
// InternalContextMappingDSL.g:9542:11: ( (lv_notOptimisticLocking_17_0= RULE_NOT ) )
// InternalContextMappingDSL.g:9543:12: (lv_notOptimisticLocking_17_0= RULE_NOT )
{
// InternalContextMappingDSL.g:9543:12: (lv_notOptimisticLocking_17_0= RULE_NOT )
// InternalContextMappingDSL.g:9544:13: lv_notOptimisticLocking_17_0= RULE_NOT
{
lv_notOptimisticLocking_17_0=(Token)match(input,RULE_NOT,FOLLOW_136);
newLeafNode(lv_notOptimisticLocking_17_0, grammarAccess.getValueObjectAccess().getNotOptimisticLockingNOTTerminalRuleCall_7_2_0_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(
current,
"notOptimisticLocking",
true,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.NOT");
}
}
otherlv_18=(Token)match(input,125,FOLLOW_145);
newLeafNode(otherlv_18, grammarAccess.getValueObjectAccess().getOptimisticLockingKeyword_7_2_0_0_1());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:9566:10: otherlv_19= 'optimisticLocking'
{
otherlv_19=(Token)match(input,125,FOLLOW_145);
newLeafNode(otherlv_19, grammarAccess.getValueObjectAccess().getOptimisticLockingKeyword_7_2_0_1());
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:9576:5: ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) ) )
{
// InternalContextMappingDSL.g:9576:5: ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) ) )
// InternalContextMappingDSL.g:9577:6: {...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 1) ) {
throw new FailedPredicateException(input, "ruleValueObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 1)");
}
// InternalContextMappingDSL.g:9577:111: ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) )
// InternalContextMappingDSL.g:9578:7: ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) )
{
getUnorderedGroupHelper().select(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 1);
// InternalContextMappingDSL.g:9581:10: ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) )
// InternalContextMappingDSL.g:9581:11: {...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleValueObject", "true");
}
// InternalContextMappingDSL.g:9581:20: ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' )
int alt248=2;
int LA248_0 = input.LA(1);
if ( (LA248_0==RULE_NOT) ) {
alt248=1;
}
else if ( (LA248_0==137) ) {
alt248=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 248, 0, input);
throw nvae;
}
switch (alt248) {
case 1 :
// InternalContextMappingDSL.g:9581:21: ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' )
{
// InternalContextMappingDSL.g:9581:21: ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' )
// InternalContextMappingDSL.g:9582:11: ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable'
{
// InternalContextMappingDSL.g:9582:11: ( (lv_notImmutable_20_0= RULE_NOT ) )
// InternalContextMappingDSL.g:9583:12: (lv_notImmutable_20_0= RULE_NOT )
{
// InternalContextMappingDSL.g:9583:12: (lv_notImmutable_20_0= RULE_NOT )
// InternalContextMappingDSL.g:9584:13: lv_notImmutable_20_0= RULE_NOT
{
lv_notImmutable_20_0=(Token)match(input,RULE_NOT,FOLLOW_146);
newLeafNode(lv_notImmutable_20_0, grammarAccess.getValueObjectAccess().getNotImmutableNOTTerminalRuleCall_7_2_1_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(
current,
"notImmutable",
true,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.NOT");
}
}
otherlv_21=(Token)match(input,137,FOLLOW_145);
newLeafNode(otherlv_21, grammarAccess.getValueObjectAccess().getImmutableKeyword_7_2_1_0_1());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:9606:10: otherlv_22= 'immutable'
{
otherlv_22=(Token)match(input,137,FOLLOW_145);
newLeafNode(otherlv_22, grammarAccess.getValueObjectAccess().getImmutableKeyword_7_2_1_1());
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:9616:5: ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) )
{
// InternalContextMappingDSL.g:9616:5: ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) )
// InternalContextMappingDSL.g:9617:6: {...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 2) ) {
throw new FailedPredicateException(input, "ruleValueObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 2)");
}
// InternalContextMappingDSL.g:9617:111: ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) )
// InternalContextMappingDSL.g:9618:7: ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 2);
// InternalContextMappingDSL.g:9621:10: ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) )
// InternalContextMappingDSL.g:9621:11: {...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleValueObject", "true");
}
// InternalContextMappingDSL.g:9621:20: ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) )
int alt249=2;
int LA249_0 = input.LA(1);
if ( (LA249_0==108) ) {
alt249=1;
}
else if ( (LA249_0==RULE_NOT) ) {
alt249=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 249, 0, input);
throw nvae;
}
switch (alt249) {
case 1 :
// InternalContextMappingDSL.g:9621:21: ( (lv_cache_23_0= 'cache' ) )
{
// InternalContextMappingDSL.g:9621:21: ( (lv_cache_23_0= 'cache' ) )
// InternalContextMappingDSL.g:9622:11: (lv_cache_23_0= 'cache' )
{
// InternalContextMappingDSL.g:9622:11: (lv_cache_23_0= 'cache' )
// InternalContextMappingDSL.g:9623:12: lv_cache_23_0= 'cache'
{
lv_cache_23_0=(Token)match(input,108,FOLLOW_145);
newLeafNode(lv_cache_23_0, grammarAccess.getValueObjectAccess().getCacheCacheKeyword_7_2_2_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(current, "cache", true, "cache");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:9636:10: (this_NOT_24= RULE_NOT otherlv_25= 'cache' )
{
// InternalContextMappingDSL.g:9636:10: (this_NOT_24= RULE_NOT otherlv_25= 'cache' )
// InternalContextMappingDSL.g:9637:11: this_NOT_24= RULE_NOT otherlv_25= 'cache'
{
this_NOT_24=(Token)match(input,RULE_NOT,FOLLOW_138);
newLeafNode(this_NOT_24, grammarAccess.getValueObjectAccess().getNOTTerminalRuleCall_7_2_2_1_0());
otherlv_25=(Token)match(input,108,FOLLOW_145);
newLeafNode(otherlv_25, grammarAccess.getValueObjectAccess().getCacheKeyword_7_2_2_1_1());
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:9652:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) )
{
// InternalContextMappingDSL.g:9652:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) )
// InternalContextMappingDSL.g:9653:6: {...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 3) ) {
throw new FailedPredicateException(input, "ruleValueObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 3)");
}
// InternalContextMappingDSL.g:9653:111: ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) )
// InternalContextMappingDSL.g:9654:7: ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 3);
// InternalContextMappingDSL.g:9657:10: ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) )
// InternalContextMappingDSL.g:9657:11: {...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleValueObject", "true");
}
// InternalContextMappingDSL.g:9657:20: ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) )
int alt250=2;
int LA250_0 = input.LA(1);
if ( (LA250_0==86) ) {
alt250=1;
}
else if ( (LA250_0==87) ) {
alt250=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 250, 0, input);
throw nvae;
}
switch (alt250) {
case 1 :
// InternalContextMappingDSL.g:9657:21: ( (lv_gapClass_26_0= 'gap' ) )
{
// InternalContextMappingDSL.g:9657:21: ( (lv_gapClass_26_0= 'gap' ) )
// InternalContextMappingDSL.g:9658:11: (lv_gapClass_26_0= 'gap' )
{
// InternalContextMappingDSL.g:9658:11: (lv_gapClass_26_0= 'gap' )
// InternalContextMappingDSL.g:9659:12: lv_gapClass_26_0= 'gap'
{
lv_gapClass_26_0=(Token)match(input,86,FOLLOW_145);
newLeafNode(lv_gapClass_26_0, grammarAccess.getValueObjectAccess().getGapClassGapKeyword_7_2_3_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(current, "gapClass", true, "gap");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:9672:10: ( (lv_noGapClass_27_0= 'nogap' ) )
{
// InternalContextMappingDSL.g:9672:10: ( (lv_noGapClass_27_0= 'nogap' ) )
// InternalContextMappingDSL.g:9673:11: (lv_noGapClass_27_0= 'nogap' )
{
// InternalContextMappingDSL.g:9673:11: (lv_noGapClass_27_0= 'nogap' )
// InternalContextMappingDSL.g:9674:12: lv_noGapClass_27_0= 'nogap'
{
lv_noGapClass_27_0=(Token)match(input,87,FOLLOW_145);
newLeafNode(lv_noGapClass_27_0, grammarAccess.getValueObjectAccess().getNoGapClassNogapKeyword_7_2_3_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(current, "noGapClass", true, "nogap");
}
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 5 :
// InternalContextMappingDSL.g:9692:5: ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) )
{
// InternalContextMappingDSL.g:9692:5: ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) )
// InternalContextMappingDSL.g:9693:6: {...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 4) ) {
throw new FailedPredicateException(input, "ruleValueObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 4)");
}
// InternalContextMappingDSL.g:9693:111: ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) )
// InternalContextMappingDSL.g:9694:7: ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 4);
// InternalContextMappingDSL.g:9697:10: ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) )
// InternalContextMappingDSL.g:9697:11: {...}? => ( (lv_scaffold_28_0= 'scaffold' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleValueObject", "true");
}
// InternalContextMappingDSL.g:9697:20: ( (lv_scaffold_28_0= 'scaffold' ) )
// InternalContextMappingDSL.g:9697:21: (lv_scaffold_28_0= 'scaffold' )
{
// InternalContextMappingDSL.g:9697:21: (lv_scaffold_28_0= 'scaffold' )
// InternalContextMappingDSL.g:9698:11: lv_scaffold_28_0= 'scaffold'
{
lv_scaffold_28_0=(Token)match(input,90,FOLLOW_145);
newLeafNode(lv_scaffold_28_0, grammarAccess.getValueObjectAccess().getScaffoldScaffoldKeyword_7_2_4_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(current, "scaffold", true, "scaffold");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 6 :
// InternalContextMappingDSL.g:9715:5: ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:9715:5: ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:9716:6: {...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 5) ) {
throw new FailedPredicateException(input, "ruleValueObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 5)");
}
// InternalContextMappingDSL.g:9716:111: ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:9717:7: ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 5);
// InternalContextMappingDSL.g:9720:10: ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:9720:11: {...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleValueObject", "true");
}
// InternalContextMappingDSL.g:9720:20: (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:9720:21: otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) )
{
otherlv_29=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_29, grammarAccess.getValueObjectAccess().getHintKeyword_7_2_5_0());
otherlv_30=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_30, grammarAccess.getValueObjectAccess().getEqualsSignKeyword_7_2_5_1());
// InternalContextMappingDSL.g:9728:10: ( (lv_hint_31_0= RULE_STRING ) )
// InternalContextMappingDSL.g:9729:11: (lv_hint_31_0= RULE_STRING )
{
// InternalContextMappingDSL.g:9729:11: (lv_hint_31_0= RULE_STRING )
// InternalContextMappingDSL.g:9730:12: lv_hint_31_0= RULE_STRING
{
lv_hint_31_0=(Token)match(input,RULE_STRING,FOLLOW_145);
newLeafNode(lv_hint_31_0, grammarAccess.getValueObjectAccess().getHintSTRINGTerminalRuleCall_7_2_5_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_31_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 7 :
// InternalContextMappingDSL.g:9752:5: ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:9752:5: ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:9753:6: {...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 6) ) {
throw new FailedPredicateException(input, "ruleValueObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 6)");
}
// InternalContextMappingDSL.g:9753:111: ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:9754:7: ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 6);
// InternalContextMappingDSL.g:9757:10: ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:9757:11: {...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleValueObject", "true");
}
// InternalContextMappingDSL.g:9757:20: (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:9757:21: otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) )
{
otherlv_32=(Token)match(input,127,FOLLOW_89);
newLeafNode(otherlv_32, grammarAccess.getValueObjectAccess().getDatabaseTableKeyword_7_2_6_0());
otherlv_33=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_33, grammarAccess.getValueObjectAccess().getEqualsSignKeyword_7_2_6_1());
// InternalContextMappingDSL.g:9765:10: ( (lv_databaseTable_34_0= RULE_STRING ) )
// InternalContextMappingDSL.g:9766:11: (lv_databaseTable_34_0= RULE_STRING )
{
// InternalContextMappingDSL.g:9766:11: (lv_databaseTable_34_0= RULE_STRING )
// InternalContextMappingDSL.g:9767:12: lv_databaseTable_34_0= RULE_STRING
{
lv_databaseTable_34_0=(Token)match(input,RULE_STRING,FOLLOW_145);
newLeafNode(lv_databaseTable_34_0, grammarAccess.getValueObjectAccess().getDatabaseTableSTRINGTerminalRuleCall_7_2_6_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(
current,
"databaseTable",
lv_databaseTable_34_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 8 :
// InternalContextMappingDSL.g:9789:5: ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:9789:5: ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:9790:6: {...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 7) ) {
throw new FailedPredicateException(input, "ruleValueObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 7)");
}
// InternalContextMappingDSL.g:9790:111: ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:9791:7: ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 7);
// InternalContextMappingDSL.g:9794:10: ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:9794:11: {...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleValueObject", "true");
}
// InternalContextMappingDSL.g:9794:20: (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:9794:21: otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) )
{
otherlv_35=(Token)match(input,128,FOLLOW_89);
newLeafNode(otherlv_35, grammarAccess.getValueObjectAccess().getDiscriminatorValueKeyword_7_2_7_0());
otherlv_36=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_36, grammarAccess.getValueObjectAccess().getEqualsSignKeyword_7_2_7_1());
// InternalContextMappingDSL.g:9802:10: ( (lv_discriminatorValue_37_0= RULE_STRING ) )
// InternalContextMappingDSL.g:9803:11: (lv_discriminatorValue_37_0= RULE_STRING )
{
// InternalContextMappingDSL.g:9803:11: (lv_discriminatorValue_37_0= RULE_STRING )
// InternalContextMappingDSL.g:9804:12: lv_discriminatorValue_37_0= RULE_STRING
{
lv_discriminatorValue_37_0=(Token)match(input,RULE_STRING,FOLLOW_145);
newLeafNode(lv_discriminatorValue_37_0, grammarAccess.getValueObjectAccess().getDiscriminatorValueSTRINGTerminalRuleCall_7_2_7_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(
current,
"discriminatorValue",
lv_discriminatorValue_37_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 9 :
// InternalContextMappingDSL.g:9826:5: ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:9826:5: ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:9827:6: {...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 8) ) {
throw new FailedPredicateException(input, "ruleValueObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 8)");
}
// InternalContextMappingDSL.g:9827:111: ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:9828:7: ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 8);
// InternalContextMappingDSL.g:9831:10: ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:9831:11: {...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleValueObject", "true");
}
// InternalContextMappingDSL.g:9831:20: (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:9831:21: otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) )
{
otherlv_38=(Token)match(input,129,FOLLOW_89);
newLeafNode(otherlv_38, grammarAccess.getValueObjectAccess().getDiscriminatorColumnKeyword_7_2_8_0());
otherlv_39=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_39, grammarAccess.getValueObjectAccess().getEqualsSignKeyword_7_2_8_1());
// InternalContextMappingDSL.g:9839:10: ( (lv_discriminatorColumn_40_0= RULE_STRING ) )
// InternalContextMappingDSL.g:9840:11: (lv_discriminatorColumn_40_0= RULE_STRING )
{
// InternalContextMappingDSL.g:9840:11: (lv_discriminatorColumn_40_0= RULE_STRING )
// InternalContextMappingDSL.g:9841:12: lv_discriminatorColumn_40_0= RULE_STRING
{
lv_discriminatorColumn_40_0=(Token)match(input,RULE_STRING,FOLLOW_145);
newLeafNode(lv_discriminatorColumn_40_0, grammarAccess.getValueObjectAccess().getDiscriminatorColumnSTRINGTerminalRuleCall_7_2_8_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(
current,
"discriminatorColumn",
lv_discriminatorColumn_40_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 10 :
// InternalContextMappingDSL.g:9863:5: ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) )
{
// InternalContextMappingDSL.g:9863:5: ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) )
// InternalContextMappingDSL.g:9864:6: {...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 9) ) {
throw new FailedPredicateException(input, "ruleValueObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 9)");
}
// InternalContextMappingDSL.g:9864:111: ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) )
// InternalContextMappingDSL.g:9865:7: ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 9);
// InternalContextMappingDSL.g:9868:10: ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) )
// InternalContextMappingDSL.g:9868:11: {...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleValueObject", "true");
}
// InternalContextMappingDSL.g:9868:20: (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) )
// InternalContextMappingDSL.g:9868:21: otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) )
{
otherlv_41=(Token)match(input,130,FOLLOW_89);
newLeafNode(otherlv_41, grammarAccess.getValueObjectAccess().getDiscriminatorTypeKeyword_7_2_9_0());
otherlv_42=(Token)match(input,21,FOLLOW_139);
newLeafNode(otherlv_42, grammarAccess.getValueObjectAccess().getEqualsSignKeyword_7_2_9_1());
// InternalContextMappingDSL.g:9876:10: ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) )
// InternalContextMappingDSL.g:9877:11: (lv_discriminatorType_43_0= ruleDiscriminatorType )
{
// InternalContextMappingDSL.g:9877:11: (lv_discriminatorType_43_0= ruleDiscriminatorType )
// InternalContextMappingDSL.g:9878:12: lv_discriminatorType_43_0= ruleDiscriminatorType
{
newCompositeNode(grammarAccess.getValueObjectAccess().getDiscriminatorTypeDiscriminatorTypeEnumRuleCall_7_2_9_2_0());
pushFollow(FOLLOW_145);
lv_discriminatorType_43_0=ruleDiscriminatorType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getValueObjectRule());
}
set(
current,
"discriminatorType",
lv_discriminatorType_43_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.DiscriminatorType");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 11 :
// InternalContextMappingDSL.g:9901:5: ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:9901:5: ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:9902:6: {...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 10) ) {
throw new FailedPredicateException(input, "ruleValueObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 10)");
}
// InternalContextMappingDSL.g:9902:112: ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:9903:7: ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 10);
// InternalContextMappingDSL.g:9906:10: ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:9906:11: {...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleValueObject", "true");
}
// InternalContextMappingDSL.g:9906:20: (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:9906:21: otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) )
{
otherlv_44=(Token)match(input,131,FOLLOW_89);
newLeafNode(otherlv_44, grammarAccess.getValueObjectAccess().getDiscriminatorLengthKeyword_7_2_10_0());
otherlv_45=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_45, grammarAccess.getValueObjectAccess().getEqualsSignKeyword_7_2_10_1());
// InternalContextMappingDSL.g:9914:10: ( (lv_discriminatorLength_46_0= RULE_STRING ) )
// InternalContextMappingDSL.g:9915:11: (lv_discriminatorLength_46_0= RULE_STRING )
{
// InternalContextMappingDSL.g:9915:11: (lv_discriminatorLength_46_0= RULE_STRING )
// InternalContextMappingDSL.g:9916:12: lv_discriminatorLength_46_0= RULE_STRING
{
lv_discriminatorLength_46_0=(Token)match(input,RULE_STRING,FOLLOW_145);
newLeafNode(lv_discriminatorLength_46_0, grammarAccess.getValueObjectAccess().getDiscriminatorLengthSTRINGTerminalRuleCall_7_2_10_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(
current,
"discriminatorLength",
lv_discriminatorLength_46_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 12 :
// InternalContextMappingDSL.g:9938:5: ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) )
{
// InternalContextMappingDSL.g:9938:5: ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) )
// InternalContextMappingDSL.g:9939:6: {...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 11) ) {
throw new FailedPredicateException(input, "ruleValueObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 11)");
}
// InternalContextMappingDSL.g:9939:112: ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) )
// InternalContextMappingDSL.g:9940:7: ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 11);
// InternalContextMappingDSL.g:9943:10: ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) )
// InternalContextMappingDSL.g:9943:11: {...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleValueObject", "true");
}
// InternalContextMappingDSL.g:9943:20: (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) )
// InternalContextMappingDSL.g:9943:21: otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) )
{
otherlv_47=(Token)match(input,132,FOLLOW_89);
newLeafNode(otherlv_47, grammarAccess.getValueObjectAccess().getInheritanceTypeKeyword_7_2_11_0());
otherlv_48=(Token)match(input,21,FOLLOW_140);
newLeafNode(otherlv_48, grammarAccess.getValueObjectAccess().getEqualsSignKeyword_7_2_11_1());
// InternalContextMappingDSL.g:9951:10: ( (lv_inheritanceType_49_0= ruleInheritanceType ) )
// InternalContextMappingDSL.g:9952:11: (lv_inheritanceType_49_0= ruleInheritanceType )
{
// InternalContextMappingDSL.g:9952:11: (lv_inheritanceType_49_0= ruleInheritanceType )
// InternalContextMappingDSL.g:9953:12: lv_inheritanceType_49_0= ruleInheritanceType
{
newCompositeNode(grammarAccess.getValueObjectAccess().getInheritanceTypeInheritanceTypeEnumRuleCall_7_2_11_2_0());
pushFollow(FOLLOW_145);
lv_inheritanceType_49_0=ruleInheritanceType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getValueObjectRule());
}
set(
current,
"inheritanceType",
lv_inheritanceType_49_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.InheritanceType");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 13 :
// InternalContextMappingDSL.g:9976:5: ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:9976:5: ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:9977:6: {...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 12) ) {
throw new FailedPredicateException(input, "ruleValueObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 12)");
}
// InternalContextMappingDSL.g:9977:112: ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:9978:7: ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 12);
// InternalContextMappingDSL.g:9981:10: ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:9981:11: {...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleValueObject", "true");
}
// InternalContextMappingDSL.g:9981:20: (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:9981:21: otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) )
{
otherlv_50=(Token)match(input,133,FOLLOW_89);
newLeafNode(otherlv_50, grammarAccess.getValueObjectAccess().getValidateKeyword_7_2_12_0());
otherlv_51=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_51, grammarAccess.getValueObjectAccess().getEqualsSignKeyword_7_2_12_1());
// InternalContextMappingDSL.g:9989:10: ( (lv_validate_52_0= RULE_STRING ) )
// InternalContextMappingDSL.g:9990:11: (lv_validate_52_0= RULE_STRING )
{
// InternalContextMappingDSL.g:9990:11: (lv_validate_52_0= RULE_STRING )
// InternalContextMappingDSL.g:9991:12: lv_validate_52_0= RULE_STRING
{
lv_validate_52_0=(Token)match(input,RULE_STRING,FOLLOW_145);
newLeafNode(lv_validate_52_0, grammarAccess.getValueObjectAccess().getValidateSTRINGTerminalRuleCall_7_2_12_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(
current,
"validate",
lv_validate_52_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 14 :
// InternalContextMappingDSL.g:10013:5: ({...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) ) )
{
// InternalContextMappingDSL.g:10013:5: ({...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) ) )
// InternalContextMappingDSL.g:10014:6: {...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 13) ) {
throw new FailedPredicateException(input, "ruleValueObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 13)");
}
// InternalContextMappingDSL.g:10014:112: ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) )
// InternalContextMappingDSL.g:10015:7: ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 13);
// InternalContextMappingDSL.g:10018:10: ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) )
// InternalContextMappingDSL.g:10018:11: {...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleValueObject", "true");
}
// InternalContextMappingDSL.g:10018:20: ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) )
int alt251=2;
int LA251_0 = input.LA(1);
if ( (LA251_0==138) ) {
alt251=1;
}
else if ( (LA251_0==RULE_NOT) ) {
alt251=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 251, 0, input);
throw nvae;
}
switch (alt251) {
case 1 :
// InternalContextMappingDSL.g:10018:21: ( (lv_persistent_53_0= 'persistent' ) )
{
// InternalContextMappingDSL.g:10018:21: ( (lv_persistent_53_0= 'persistent' ) )
// InternalContextMappingDSL.g:10019:11: (lv_persistent_53_0= 'persistent' )
{
// InternalContextMappingDSL.g:10019:11: (lv_persistent_53_0= 'persistent' )
// InternalContextMappingDSL.g:10020:12: lv_persistent_53_0= 'persistent'
{
lv_persistent_53_0=(Token)match(input,138,FOLLOW_145);
newLeafNode(lv_persistent_53_0, grammarAccess.getValueObjectAccess().getPersistentPersistentKeyword_7_2_13_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(current, "persistent", true, "persistent");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:10033:10: ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' )
{
// InternalContextMappingDSL.g:10033:10: ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' )
// InternalContextMappingDSL.g:10034:11: ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent'
{
// InternalContextMappingDSL.g:10034:11: ( (lv_notPersistent_54_0= RULE_NOT ) )
// InternalContextMappingDSL.g:10035:12: (lv_notPersistent_54_0= RULE_NOT )
{
// InternalContextMappingDSL.g:10035:12: (lv_notPersistent_54_0= RULE_NOT )
// InternalContextMappingDSL.g:10036:13: lv_notPersistent_54_0= RULE_NOT
{
lv_notPersistent_54_0=(Token)match(input,RULE_NOT,FOLLOW_147);
newLeafNode(lv_notPersistent_54_0, grammarAccess.getValueObjectAccess().getNotPersistentNOTTerminalRuleCall_7_2_13_1_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(
current,
"notPersistent",
true,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.NOT");
}
}
otherlv_55=(Token)match(input,138,FOLLOW_145);
newLeafNode(otherlv_55, grammarAccess.getValueObjectAccess().getPersistentKeyword_7_2_13_1_1());
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 15 :
// InternalContextMappingDSL.g:10063:5: ({...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) ) )
{
// InternalContextMappingDSL.g:10063:5: ({...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) ) )
// InternalContextMappingDSL.g:10064:6: {...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 14) ) {
throw new FailedPredicateException(input, "ruleValueObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 14)");
}
// InternalContextMappingDSL.g:10064:112: ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) )
// InternalContextMappingDSL.g:10065:7: ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 14);
// InternalContextMappingDSL.g:10068:10: ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) )
// InternalContextMappingDSL.g:10068:11: {...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleValueObject", "true");
}
// InternalContextMappingDSL.g:10068:20: ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) )
// InternalContextMappingDSL.g:10068:21: (lv_aggregateRoot_56_0= 'aggregateRoot' )
{
// InternalContextMappingDSL.g:10068:21: (lv_aggregateRoot_56_0= 'aggregateRoot' )
// InternalContextMappingDSL.g:10069:11: lv_aggregateRoot_56_0= 'aggregateRoot'
{
lv_aggregateRoot_56_0=(Token)match(input,134,FOLLOW_145);
newLeafNode(lv_aggregateRoot_56_0, grammarAccess.getValueObjectAccess().getAggregateRootAggregateRootKeyword_7_2_14_0());
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
setWithLastConsumed(current, "aggregateRoot", true, "aggregateRoot");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 16 :
// InternalContextMappingDSL.g:10086:5: ({...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) ) )
{
// InternalContextMappingDSL.g:10086:5: ({...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) ) )
// InternalContextMappingDSL.g:10087:6: {...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 15) ) {
throw new FailedPredicateException(input, "ruleValueObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 15)");
}
// InternalContextMappingDSL.g:10087:112: ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) )
// InternalContextMappingDSL.g:10088:7: ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 15);
// InternalContextMappingDSL.g:10091:10: ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) )
// InternalContextMappingDSL.g:10091:11: {...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleValueObject", "true");
}
// InternalContextMappingDSL.g:10091:20: (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) )
// InternalContextMappingDSL.g:10091:21: otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) )
{
otherlv_57=(Token)match(input,135,FOLLOW_105);
newLeafNode(otherlv_57, grammarAccess.getValueObjectAccess().getBelongsToKeyword_7_2_15_0());
// InternalContextMappingDSL.g:10095:10: ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) )
// InternalContextMappingDSL.g:10096:11: (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) )
{
// InternalContextMappingDSL.g:10096:11: (otherlv_58= '@' )?
int alt252=2;
int LA252_0 = input.LA(1);
if ( (LA252_0==94) ) {
alt252=1;
}
switch (alt252) {
case 1 :
// InternalContextMappingDSL.g:10097:12: otherlv_58= '@'
{
otherlv_58=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_58, grammarAccess.getValueObjectAccess().getCommercialAtKeyword_7_2_15_1_0());
}
break;
}
// InternalContextMappingDSL.g:10102:11: ( (otherlv_59= RULE_ID ) )
// InternalContextMappingDSL.g:10103:12: (otherlv_59= RULE_ID )
{
// InternalContextMappingDSL.g:10103:12: (otherlv_59= RULE_ID )
// InternalContextMappingDSL.g:10104:13: otherlv_59= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getValueObjectRule());
}
otherlv_59=(Token)match(input,RULE_ID,FOLLOW_145);
newLeafNode(otherlv_59, grammarAccess.getValueObjectAccess().getBelongsToDomainObjectCrossReference_7_2_15_1_1_0());
}
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
}
}
}
break;
default :
break loop253;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2());
}
// InternalContextMappingDSL.g:10129:4: ( ( (lv_attributes_60_0= ruleAttribute ) ) | ( (lv_references_61_0= ruleReference ) ) | ( (lv_operations_62_0= ruleDomainObjectOperation ) ) )*
loop254:
do {
int alt254=4;
switch ( input.LA(1) ) {
case RULE_STRING:
{
switch ( input.LA(2) ) {
case RULE_ID:
case 124:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
case 247:
case 248:
case 249:
{
alt254=1;
}
break;
case 142:
case 143:
{
alt254=3;
}
break;
case RULE_REF:
{
alt254=2;
}
break;
}
}
break;
case RULE_ID:
case 124:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
case 247:
case 248:
case 249:
{
alt254=1;
}
break;
case RULE_REF:
{
alt254=2;
}
break;
case 142:
case 143:
{
alt254=3;
}
break;
}
switch (alt254) {
case 1 :
// InternalContextMappingDSL.g:10130:5: ( (lv_attributes_60_0= ruleAttribute ) )
{
// InternalContextMappingDSL.g:10130:5: ( (lv_attributes_60_0= ruleAttribute ) )
// InternalContextMappingDSL.g:10131:6: (lv_attributes_60_0= ruleAttribute )
{
// InternalContextMappingDSL.g:10131:6: (lv_attributes_60_0= ruleAttribute )
// InternalContextMappingDSL.g:10132:7: lv_attributes_60_0= ruleAttribute
{
newCompositeNode(grammarAccess.getValueObjectAccess().getAttributesAttributeParserRuleCall_7_3_0_0());
pushFollow(FOLLOW_141);
lv_attributes_60_0=ruleAttribute();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getValueObjectRule());
}
add(
current,
"attributes",
lv_attributes_60_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Attribute");
afterParserOrEnumRuleCall();
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:10150:5: ( (lv_references_61_0= ruleReference ) )
{
// InternalContextMappingDSL.g:10150:5: ( (lv_references_61_0= ruleReference ) )
// InternalContextMappingDSL.g:10151:6: (lv_references_61_0= ruleReference )
{
// InternalContextMappingDSL.g:10151:6: (lv_references_61_0= ruleReference )
// InternalContextMappingDSL.g:10152:7: lv_references_61_0= ruleReference
{
newCompositeNode(grammarAccess.getValueObjectAccess().getReferencesReferenceParserRuleCall_7_3_1_0());
pushFollow(FOLLOW_141);
lv_references_61_0=ruleReference();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getValueObjectRule());
}
add(
current,
"references",
lv_references_61_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Reference");
afterParserOrEnumRuleCall();
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:10170:5: ( (lv_operations_62_0= ruleDomainObjectOperation ) )
{
// InternalContextMappingDSL.g:10170:5: ( (lv_operations_62_0= ruleDomainObjectOperation ) )
// InternalContextMappingDSL.g:10171:6: (lv_operations_62_0= ruleDomainObjectOperation )
{
// InternalContextMappingDSL.g:10171:6: (lv_operations_62_0= ruleDomainObjectOperation )
// InternalContextMappingDSL.g:10172:7: lv_operations_62_0= ruleDomainObjectOperation
{
newCompositeNode(grammarAccess.getValueObjectAccess().getOperationsDomainObjectOperationParserRuleCall_7_3_2_0());
pushFollow(FOLLOW_141);
lv_operations_62_0=ruleDomainObjectOperation();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getValueObjectRule());
}
add(
current,
"operations",
lv_operations_62_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.DomainObjectOperation");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop254;
}
} while (true);
// InternalContextMappingDSL.g:10190:4: ( (lv_repository_63_0= ruleRepository ) )?
int alt255=2;
int LA255_0 = input.LA(1);
if ( (LA255_0==RULE_STRING||LA255_0==181) ) {
alt255=1;
}
switch (alt255) {
case 1 :
// InternalContextMappingDSL.g:10191:5: (lv_repository_63_0= ruleRepository )
{
// InternalContextMappingDSL.g:10191:5: (lv_repository_63_0= ruleRepository )
// InternalContextMappingDSL.g:10192:6: lv_repository_63_0= ruleRepository
{
newCompositeNode(grammarAccess.getValueObjectAccess().getRepositoryRepositoryParserRuleCall_7_4_0());
pushFollow(FOLLOW_36);
lv_repository_63_0=ruleRepository();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getValueObjectRule());
}
set(
current,
"repository",
lv_repository_63_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Repository");
afterParserOrEnumRuleCall();
}
}
break;
}
otherlv_64=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(otherlv_64, grammarAccess.getValueObjectAccess().getRightCurlyBracketKeyword_7_5());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleValueObject"
// $ANTLR start "entryRuleDomainEvent"
// InternalContextMappingDSL.g:10218:1: entryRuleDomainEvent returns [EObject current=null] : iv_ruleDomainEvent= ruleDomainEvent EOF ;
public final EObject entryRuleDomainEvent() throws RecognitionException {
EObject current = null;
EObject iv_ruleDomainEvent = null;
try {
// InternalContextMappingDSL.g:10218:52: (iv_ruleDomainEvent= ruleDomainEvent EOF )
// InternalContextMappingDSL.g:10219:2: iv_ruleDomainEvent= ruleDomainEvent EOF
{
newCompositeNode(grammarAccess.getDomainEventRule());
pushFollow(FOLLOW_1);
iv_ruleDomainEvent=ruleDomainEvent();
state._fsp--;
current =iv_ruleDomainEvent;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleDomainEvent"
// $ANTLR start "ruleDomainEvent"
// InternalContextMappingDSL.g:10225:1: ruleDomainEvent returns [EObject current=null] : ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'DomainEvent' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_52_0= ruleAttribute ) ) | ( (lv_references_53_0= ruleReference ) ) | ( (lv_operations_54_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_55_0= ruleRepository ) )? otherlv_56= '}' )? ) ;
public final EObject ruleDomainEvent() throws RecognitionException {
EObject current = null;
Token lv_comment_0_0=null;
Token lv_doc_1_0=null;
Token lv_abstract_2_0=null;
Token otherlv_3=null;
Token lv_name_4_0=null;
Token otherlv_5=null;
Token otherlv_6=null;
Token otherlv_7=null;
Token otherlv_9=null;
Token otherlv_10=null;
Token otherlv_11=null;
Token otherlv_12=null;
Token otherlv_13=null;
Token otherlv_14=null;
Token lv_cache_17_0=null;
Token this_NOT_18=null;
Token otherlv_19=null;
Token lv_gapClass_20_0=null;
Token lv_noGapClass_21_0=null;
Token lv_scaffold_22_0=null;
Token otherlv_23=null;
Token otherlv_24=null;
Token lv_hint_25_0=null;
Token otherlv_26=null;
Token otherlv_27=null;
Token lv_databaseTable_28_0=null;
Token otherlv_29=null;
Token otherlv_30=null;
Token lv_discriminatorValue_31_0=null;
Token otherlv_32=null;
Token otherlv_33=null;
Token lv_discriminatorColumn_34_0=null;
Token otherlv_35=null;
Token otherlv_36=null;
Token otherlv_38=null;
Token otherlv_39=null;
Token lv_discriminatorLength_40_0=null;
Token otherlv_41=null;
Token otherlv_42=null;
Token otherlv_44=null;
Token otherlv_45=null;
Token lv_validate_46_0=null;
Token lv_persistent_47_0=null;
Token lv_aggregateRoot_48_0=null;
Token otherlv_49=null;
Token otherlv_50=null;
Token otherlv_51=null;
Token otherlv_56=null;
AntlrDatatypeRuleToken lv_extendsName_8_0 = null;
AntlrDatatypeRuleToken lv_package_15_0 = null;
Enumerator lv_discriminatorType_37_0 = null;
Enumerator lv_inheritanceType_43_0 = null;
EObject lv_attributes_52_0 = null;
EObject lv_references_53_0 = null;
EObject lv_operations_54_0 = null;
EObject lv_repository_55_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:10231:2: ( ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'DomainEvent' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_52_0= ruleAttribute ) ) | ( (lv_references_53_0= ruleReference ) ) | ( (lv_operations_54_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_55_0= ruleRepository ) )? otherlv_56= '}' )? ) )
// InternalContextMappingDSL.g:10232:2: ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'DomainEvent' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_52_0= ruleAttribute ) ) | ( (lv_references_53_0= ruleReference ) ) | ( (lv_operations_54_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_55_0= ruleRepository ) )? otherlv_56= '}' )? )
{
// InternalContextMappingDSL.g:10232:2: ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'DomainEvent' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_52_0= ruleAttribute ) ) | ( (lv_references_53_0= ruleReference ) ) | ( (lv_operations_54_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_55_0= ruleRepository ) )? otherlv_56= '}' )? )
// InternalContextMappingDSL.g:10233:3: ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'DomainEvent' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_52_0= ruleAttribute ) ) | ( (lv_references_53_0= ruleReference ) ) | ( (lv_operations_54_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_55_0= ruleRepository ) )? otherlv_56= '}' )?
{
// InternalContextMappingDSL.g:10233:3: ( (lv_comment_0_0= RULE_ML_COMMENT ) )?
int alt257=2;
int LA257_0 = input.LA(1);
if ( (LA257_0==RULE_ML_COMMENT) ) {
alt257=1;
}
switch (alt257) {
case 1 :
// InternalContextMappingDSL.g:10234:4: (lv_comment_0_0= RULE_ML_COMMENT )
{
// InternalContextMappingDSL.g:10234:4: (lv_comment_0_0= RULE_ML_COMMENT )
// InternalContextMappingDSL.g:10235:5: lv_comment_0_0= RULE_ML_COMMENT
{
lv_comment_0_0=(Token)match(input,RULE_ML_COMMENT,FOLLOW_148);
newLeafNode(lv_comment_0_0, grammarAccess.getDomainEventAccess().getCommentML_COMMENTTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
setWithLastConsumed(
current,
"comment",
lv_comment_0_0,
"org.eclipse.xtext.common.Terminals.ML_COMMENT");
}
}
break;
}
// InternalContextMappingDSL.g:10251:3: ( (lv_doc_1_0= RULE_STRING ) )?
int alt258=2;
int LA258_0 = input.LA(1);
if ( (LA258_0==RULE_STRING) ) {
alt258=1;
}
switch (alt258) {
case 1 :
// InternalContextMappingDSL.g:10252:4: (lv_doc_1_0= RULE_STRING )
{
// InternalContextMappingDSL.g:10252:4: (lv_doc_1_0= RULE_STRING )
// InternalContextMappingDSL.g:10253:5: lv_doc_1_0= RULE_STRING
{
lv_doc_1_0=(Token)match(input,RULE_STRING,FOLLOW_149);
newLeafNode(lv_doc_1_0, grammarAccess.getDomainEventAccess().getDocSTRINGTerminalRuleCall_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_1_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
// InternalContextMappingDSL.g:10269:3: ( (lv_abstract_2_0= 'abstract' ) )?
int alt259=2;
int LA259_0 = input.LA(1);
if ( (LA259_0==120) ) {
alt259=1;
}
switch (alt259) {
case 1 :
// InternalContextMappingDSL.g:10270:4: (lv_abstract_2_0= 'abstract' )
{
// InternalContextMappingDSL.g:10270:4: (lv_abstract_2_0= 'abstract' )
// InternalContextMappingDSL.g:10271:5: lv_abstract_2_0= 'abstract'
{
lv_abstract_2_0=(Token)match(input,120,FOLLOW_150);
newLeafNode(lv_abstract_2_0, grammarAccess.getDomainEventAccess().getAbstractAbstractKeyword_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
setWithLastConsumed(current, "abstract", true, "abstract");
}
}
break;
}
otherlv_3=(Token)match(input,139,FOLLOW_10);
newLeafNode(otherlv_3, grammarAccess.getDomainEventAccess().getDomainEventKeyword_3());
// InternalContextMappingDSL.g:10287:3: ( (lv_name_4_0= RULE_ID ) )
// InternalContextMappingDSL.g:10288:4: (lv_name_4_0= RULE_ID )
{
// InternalContextMappingDSL.g:10288:4: (lv_name_4_0= RULE_ID )
// InternalContextMappingDSL.g:10289:5: lv_name_4_0= RULE_ID
{
lv_name_4_0=(Token)match(input,RULE_ID,FOLLOW_133);
newLeafNode(lv_name_4_0, grammarAccess.getDomainEventAccess().getNameIDTerminalRuleCall_4_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
setWithLastConsumed(
current,
"name",
lv_name_4_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:10305:3: (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )?
int alt261=2;
int LA261_0 = input.LA(1);
if ( (LA261_0==122) ) {
alt261=1;
}
switch (alt261) {
case 1 :
// InternalContextMappingDSL.g:10306:4: otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) )
{
otherlv_5=(Token)match(input,122,FOLLOW_105);
newLeafNode(otherlv_5, grammarAccess.getDomainEventAccess().getExtendsKeyword_5_0());
// InternalContextMappingDSL.g:10310:4: ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) )
int alt260=2;
int LA260_0 = input.LA(1);
if ( (LA260_0==94) ) {
alt260=1;
}
else if ( (LA260_0==RULE_ID) ) {
alt260=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 260, 0, input);
throw nvae;
}
switch (alt260) {
case 1 :
// InternalContextMappingDSL.g:10311:5: (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:10311:5: (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) )
// InternalContextMappingDSL.g:10312:6: otherlv_6= '@' ( (otherlv_7= RULE_ID ) )
{
otherlv_6=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_6, grammarAccess.getDomainEventAccess().getCommercialAtKeyword_5_1_0_0());
// InternalContextMappingDSL.g:10316:6: ( (otherlv_7= RULE_ID ) )
// InternalContextMappingDSL.g:10317:7: (otherlv_7= RULE_ID )
{
// InternalContextMappingDSL.g:10317:7: (otherlv_7= RULE_ID )
// InternalContextMappingDSL.g:10318:8: otherlv_7= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
otherlv_7=(Token)match(input,RULE_ID,FOLLOW_134);
newLeafNode(otherlv_7, grammarAccess.getDomainEventAccess().getExtendsDomainEventCrossReference_5_1_0_1_0());
}
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:10331:5: ( (lv_extendsName_8_0= ruleJavaIdentifier ) )
{
// InternalContextMappingDSL.g:10331:5: ( (lv_extendsName_8_0= ruleJavaIdentifier ) )
// InternalContextMappingDSL.g:10332:6: (lv_extendsName_8_0= ruleJavaIdentifier )
{
// InternalContextMappingDSL.g:10332:6: (lv_extendsName_8_0= ruleJavaIdentifier )
// InternalContextMappingDSL.g:10333:7: lv_extendsName_8_0= ruleJavaIdentifier
{
newCompositeNode(grammarAccess.getDomainEventAccess().getExtendsNameJavaIdentifierParserRuleCall_5_1_1_0());
pushFollow(FOLLOW_134);
lv_extendsName_8_0=ruleJavaIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDomainEventRule());
}
set(
current,
"extendsName",
lv_extendsName_8_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.JavaIdentifier");
afterParserOrEnumRuleCall();
}
}
}
break;
}
}
break;
}
// InternalContextMappingDSL.g:10352:3: (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )*
loop263:
do {
int alt263=2;
int LA263_0 = input.LA(1);
if ( (LA263_0==123) ) {
alt263=1;
}
switch (alt263) {
case 1 :
// InternalContextMappingDSL.g:10353:4: otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) )
{
otherlv_9=(Token)match(input,123,FOLLOW_105);
newLeafNode(otherlv_9, grammarAccess.getDomainEventAccess().getWithKeyword_6_0());
// InternalContextMappingDSL.g:10357:4: (otherlv_10= '@' )?
int alt262=2;
int LA262_0 = input.LA(1);
if ( (LA262_0==94) ) {
alt262=1;
}
switch (alt262) {
case 1 :
// InternalContextMappingDSL.g:10358:5: otherlv_10= '@'
{
otherlv_10=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_10, grammarAccess.getDomainEventAccess().getCommercialAtKeyword_6_1());
}
break;
}
// InternalContextMappingDSL.g:10363:4: ( (otherlv_11= RULE_ID ) )
// InternalContextMappingDSL.g:10364:5: (otherlv_11= RULE_ID )
{
// InternalContextMappingDSL.g:10364:5: (otherlv_11= RULE_ID )
// InternalContextMappingDSL.g:10365:6: otherlv_11= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
otherlv_11=(Token)match(input,RULE_ID,FOLLOW_134);
newLeafNode(otherlv_11, grammarAccess.getDomainEventAccess().getTraitsTraitCrossReference_6_2_0());
}
}
}
break;
default :
break loop263;
}
} while (true);
// InternalContextMappingDSL.g:10377:3: (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_52_0= ruleAttribute ) ) | ( (lv_references_53_0= ruleReference ) ) | ( (lv_operations_54_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_55_0= ruleRepository ) )? otherlv_56= '}' )?
int alt271=2;
int LA271_0 = input.LA(1);
if ( (LA271_0==RULE_OPEN) ) {
alt271=1;
}
switch (alt271) {
case 1 :
// InternalContextMappingDSL.g:10378:4: otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_52_0= ruleAttribute ) ) | ( (lv_references_53_0= ruleReference ) ) | ( (lv_operations_54_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_55_0= ruleRepository ) )? otherlv_56= '}'
{
otherlv_12=(Token)match(input,RULE_OPEN,FOLLOW_151);
newLeafNode(otherlv_12, grammarAccess.getDomainEventAccess().getLeftCurlyBracketKeyword_7_0());
// InternalContextMappingDSL.g:10382:4: (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )?
int alt264=2;
int LA264_0 = input.LA(1);
if ( (LA264_0==124) ) {
int LA264_1 = input.LA(2);
if ( (LA264_1==21) ) {
alt264=1;
}
}
switch (alt264) {
case 1 :
// InternalContextMappingDSL.g:10383:5: otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) )
{
otherlv_13=(Token)match(input,124,FOLLOW_89);
newLeafNode(otherlv_13, grammarAccess.getDomainEventAccess().getPackageKeyword_7_1_0());
otherlv_14=(Token)match(input,21,FOLLOW_10);
newLeafNode(otherlv_14, grammarAccess.getDomainEventAccess().getEqualsSignKeyword_7_1_1());
// InternalContextMappingDSL.g:10391:5: ( (lv_package_15_0= ruleJavaIdentifier ) )
// InternalContextMappingDSL.g:10392:6: (lv_package_15_0= ruleJavaIdentifier )
{
// InternalContextMappingDSL.g:10392:6: (lv_package_15_0= ruleJavaIdentifier )
// InternalContextMappingDSL.g:10393:7: lv_package_15_0= ruleJavaIdentifier
{
newCompositeNode(grammarAccess.getDomainEventAccess().getPackageJavaIdentifierParserRuleCall_7_1_2_0());
pushFollow(FOLLOW_151);
lv_package_15_0=ruleJavaIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDomainEventRule());
}
set(
current,
"package",
lv_package_15_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.JavaIdentifier");
afterParserOrEnumRuleCall();
}
}
}
break;
}
// InternalContextMappingDSL.g:10411:4: ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:10412:5: ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:10412:5: ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:10413:6: ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2());
// InternalContextMappingDSL.g:10416:6: ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:10417:7: ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:10417:7: ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )*
loop268:
do {
int alt268=15;
alt268 = dfa268.predict(input);
switch (alt268) {
case 1 :
// InternalContextMappingDSL.g:10418:5: ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) )
{
// InternalContextMappingDSL.g:10418:5: ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) )
// InternalContextMappingDSL.g:10419:6: {...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 0) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 0)");
}
// InternalContextMappingDSL.g:10419:111: ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) )
// InternalContextMappingDSL.g:10420:7: ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 0);
// InternalContextMappingDSL.g:10423:10: ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) )
// InternalContextMappingDSL.g:10423:11: {...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "true");
}
// InternalContextMappingDSL.g:10423:20: ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) )
int alt265=2;
int LA265_0 = input.LA(1);
if ( (LA265_0==108) ) {
alt265=1;
}
else if ( (LA265_0==RULE_NOT) ) {
alt265=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 265, 0, input);
throw nvae;
}
switch (alt265) {
case 1 :
// InternalContextMappingDSL.g:10423:21: ( (lv_cache_17_0= 'cache' ) )
{
// InternalContextMappingDSL.g:10423:21: ( (lv_cache_17_0= 'cache' ) )
// InternalContextMappingDSL.g:10424:11: (lv_cache_17_0= 'cache' )
{
// InternalContextMappingDSL.g:10424:11: (lv_cache_17_0= 'cache' )
// InternalContextMappingDSL.g:10425:12: lv_cache_17_0= 'cache'
{
lv_cache_17_0=(Token)match(input,108,FOLLOW_151);
newLeafNode(lv_cache_17_0, grammarAccess.getDomainEventAccess().getCacheCacheKeyword_7_2_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
setWithLastConsumed(current, "cache", true, "cache");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:10438:10: (this_NOT_18= RULE_NOT otherlv_19= 'cache' )
{
// InternalContextMappingDSL.g:10438:10: (this_NOT_18= RULE_NOT otherlv_19= 'cache' )
// InternalContextMappingDSL.g:10439:11: this_NOT_18= RULE_NOT otherlv_19= 'cache'
{
this_NOT_18=(Token)match(input,RULE_NOT,FOLLOW_138);
newLeafNode(this_NOT_18, grammarAccess.getDomainEventAccess().getNOTTerminalRuleCall_7_2_0_1_0());
otherlv_19=(Token)match(input,108,FOLLOW_151);
newLeafNode(otherlv_19, grammarAccess.getDomainEventAccess().getCacheKeyword_7_2_0_1_1());
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:10454:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) )
{
// InternalContextMappingDSL.g:10454:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) )
// InternalContextMappingDSL.g:10455:6: {...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 1) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 1)");
}
// InternalContextMappingDSL.g:10455:111: ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) )
// InternalContextMappingDSL.g:10456:7: ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 1);
// InternalContextMappingDSL.g:10459:10: ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) )
// InternalContextMappingDSL.g:10459:11: {...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "true");
}
// InternalContextMappingDSL.g:10459:20: ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) )
int alt266=2;
int LA266_0 = input.LA(1);
if ( (LA266_0==86) ) {
alt266=1;
}
else if ( (LA266_0==87) ) {
alt266=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 266, 0, input);
throw nvae;
}
switch (alt266) {
case 1 :
// InternalContextMappingDSL.g:10459:21: ( (lv_gapClass_20_0= 'gap' ) )
{
// InternalContextMappingDSL.g:10459:21: ( (lv_gapClass_20_0= 'gap' ) )
// InternalContextMappingDSL.g:10460:11: (lv_gapClass_20_0= 'gap' )
{
// InternalContextMappingDSL.g:10460:11: (lv_gapClass_20_0= 'gap' )
// InternalContextMappingDSL.g:10461:12: lv_gapClass_20_0= 'gap'
{
lv_gapClass_20_0=(Token)match(input,86,FOLLOW_151);
newLeafNode(lv_gapClass_20_0, grammarAccess.getDomainEventAccess().getGapClassGapKeyword_7_2_1_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
setWithLastConsumed(current, "gapClass", true, "gap");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:10474:10: ( (lv_noGapClass_21_0= 'nogap' ) )
{
// InternalContextMappingDSL.g:10474:10: ( (lv_noGapClass_21_0= 'nogap' ) )
// InternalContextMappingDSL.g:10475:11: (lv_noGapClass_21_0= 'nogap' )
{
// InternalContextMappingDSL.g:10475:11: (lv_noGapClass_21_0= 'nogap' )
// InternalContextMappingDSL.g:10476:12: lv_noGapClass_21_0= 'nogap'
{
lv_noGapClass_21_0=(Token)match(input,87,FOLLOW_151);
newLeafNode(lv_noGapClass_21_0, grammarAccess.getDomainEventAccess().getNoGapClassNogapKeyword_7_2_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
setWithLastConsumed(current, "noGapClass", true, "nogap");
}
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:10494:5: ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) )
{
// InternalContextMappingDSL.g:10494:5: ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) )
// InternalContextMappingDSL.g:10495:6: {...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 2) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 2)");
}
// InternalContextMappingDSL.g:10495:111: ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) )
// InternalContextMappingDSL.g:10496:7: ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 2);
// InternalContextMappingDSL.g:10499:10: ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) )
// InternalContextMappingDSL.g:10499:11: {...}? => ( (lv_scaffold_22_0= 'scaffold' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "true");
}
// InternalContextMappingDSL.g:10499:20: ( (lv_scaffold_22_0= 'scaffold' ) )
// InternalContextMappingDSL.g:10499:21: (lv_scaffold_22_0= 'scaffold' )
{
// InternalContextMappingDSL.g:10499:21: (lv_scaffold_22_0= 'scaffold' )
// InternalContextMappingDSL.g:10500:11: lv_scaffold_22_0= 'scaffold'
{
lv_scaffold_22_0=(Token)match(input,90,FOLLOW_151);
newLeafNode(lv_scaffold_22_0, grammarAccess.getDomainEventAccess().getScaffoldScaffoldKeyword_7_2_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
setWithLastConsumed(current, "scaffold", true, "scaffold");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:10517:5: ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:10517:5: ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:10518:6: {...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 3) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 3)");
}
// InternalContextMappingDSL.g:10518:111: ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:10519:7: ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 3);
// InternalContextMappingDSL.g:10522:10: ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:10522:11: {...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "true");
}
// InternalContextMappingDSL.g:10522:20: (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:10522:21: otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) )
{
otherlv_23=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_23, grammarAccess.getDomainEventAccess().getHintKeyword_7_2_3_0());
otherlv_24=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_24, grammarAccess.getDomainEventAccess().getEqualsSignKeyword_7_2_3_1());
// InternalContextMappingDSL.g:10530:10: ( (lv_hint_25_0= RULE_STRING ) )
// InternalContextMappingDSL.g:10531:11: (lv_hint_25_0= RULE_STRING )
{
// InternalContextMappingDSL.g:10531:11: (lv_hint_25_0= RULE_STRING )
// InternalContextMappingDSL.g:10532:12: lv_hint_25_0= RULE_STRING
{
lv_hint_25_0=(Token)match(input,RULE_STRING,FOLLOW_151);
newLeafNode(lv_hint_25_0, grammarAccess.getDomainEventAccess().getHintSTRINGTerminalRuleCall_7_2_3_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_25_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 5 :
// InternalContextMappingDSL.g:10554:5: ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:10554:5: ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:10555:6: {...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 4) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 4)");
}
// InternalContextMappingDSL.g:10555:111: ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:10556:7: ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 4);
// InternalContextMappingDSL.g:10559:10: ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:10559:11: {...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "true");
}
// InternalContextMappingDSL.g:10559:20: (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:10559:21: otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) )
{
otherlv_26=(Token)match(input,127,FOLLOW_89);
newLeafNode(otherlv_26, grammarAccess.getDomainEventAccess().getDatabaseTableKeyword_7_2_4_0());
otherlv_27=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_27, grammarAccess.getDomainEventAccess().getEqualsSignKeyword_7_2_4_1());
// InternalContextMappingDSL.g:10567:10: ( (lv_databaseTable_28_0= RULE_STRING ) )
// InternalContextMappingDSL.g:10568:11: (lv_databaseTable_28_0= RULE_STRING )
{
// InternalContextMappingDSL.g:10568:11: (lv_databaseTable_28_0= RULE_STRING )
// InternalContextMappingDSL.g:10569:12: lv_databaseTable_28_0= RULE_STRING
{
lv_databaseTable_28_0=(Token)match(input,RULE_STRING,FOLLOW_151);
newLeafNode(lv_databaseTable_28_0, grammarAccess.getDomainEventAccess().getDatabaseTableSTRINGTerminalRuleCall_7_2_4_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
setWithLastConsumed(
current,
"databaseTable",
lv_databaseTable_28_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 6 :
// InternalContextMappingDSL.g:10591:5: ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:10591:5: ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:10592:6: {...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 5) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 5)");
}
// InternalContextMappingDSL.g:10592:111: ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:10593:7: ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 5);
// InternalContextMappingDSL.g:10596:10: ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:10596:11: {...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "true");
}
// InternalContextMappingDSL.g:10596:20: (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:10596:21: otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) )
{
otherlv_29=(Token)match(input,128,FOLLOW_89);
newLeafNode(otherlv_29, grammarAccess.getDomainEventAccess().getDiscriminatorValueKeyword_7_2_5_0());
otherlv_30=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_30, grammarAccess.getDomainEventAccess().getEqualsSignKeyword_7_2_5_1());
// InternalContextMappingDSL.g:10604:10: ( (lv_discriminatorValue_31_0= RULE_STRING ) )
// InternalContextMappingDSL.g:10605:11: (lv_discriminatorValue_31_0= RULE_STRING )
{
// InternalContextMappingDSL.g:10605:11: (lv_discriminatorValue_31_0= RULE_STRING )
// InternalContextMappingDSL.g:10606:12: lv_discriminatorValue_31_0= RULE_STRING
{
lv_discriminatorValue_31_0=(Token)match(input,RULE_STRING,FOLLOW_151);
newLeafNode(lv_discriminatorValue_31_0, grammarAccess.getDomainEventAccess().getDiscriminatorValueSTRINGTerminalRuleCall_7_2_5_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
setWithLastConsumed(
current,
"discriminatorValue",
lv_discriminatorValue_31_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 7 :
// InternalContextMappingDSL.g:10628:5: ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:10628:5: ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:10629:6: {...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 6) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 6)");
}
// InternalContextMappingDSL.g:10629:111: ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:10630:7: ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 6);
// InternalContextMappingDSL.g:10633:10: ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:10633:11: {...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "true");
}
// InternalContextMappingDSL.g:10633:20: (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:10633:21: otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) )
{
otherlv_32=(Token)match(input,129,FOLLOW_89);
newLeafNode(otherlv_32, grammarAccess.getDomainEventAccess().getDiscriminatorColumnKeyword_7_2_6_0());
otherlv_33=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_33, grammarAccess.getDomainEventAccess().getEqualsSignKeyword_7_2_6_1());
// InternalContextMappingDSL.g:10641:10: ( (lv_discriminatorColumn_34_0= RULE_STRING ) )
// InternalContextMappingDSL.g:10642:11: (lv_discriminatorColumn_34_0= RULE_STRING )
{
// InternalContextMappingDSL.g:10642:11: (lv_discriminatorColumn_34_0= RULE_STRING )
// InternalContextMappingDSL.g:10643:12: lv_discriminatorColumn_34_0= RULE_STRING
{
lv_discriminatorColumn_34_0=(Token)match(input,RULE_STRING,FOLLOW_151);
newLeafNode(lv_discriminatorColumn_34_0, grammarAccess.getDomainEventAccess().getDiscriminatorColumnSTRINGTerminalRuleCall_7_2_6_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
setWithLastConsumed(
current,
"discriminatorColumn",
lv_discriminatorColumn_34_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 8 :
// InternalContextMappingDSL.g:10665:5: ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) )
{
// InternalContextMappingDSL.g:10665:5: ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) )
// InternalContextMappingDSL.g:10666:6: {...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 7) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 7)");
}
// InternalContextMappingDSL.g:10666:111: ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) )
// InternalContextMappingDSL.g:10667:7: ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 7);
// InternalContextMappingDSL.g:10670:10: ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) )
// InternalContextMappingDSL.g:10670:11: {...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "true");
}
// InternalContextMappingDSL.g:10670:20: (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) )
// InternalContextMappingDSL.g:10670:21: otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) )
{
otherlv_35=(Token)match(input,130,FOLLOW_89);
newLeafNode(otherlv_35, grammarAccess.getDomainEventAccess().getDiscriminatorTypeKeyword_7_2_7_0());
otherlv_36=(Token)match(input,21,FOLLOW_139);
newLeafNode(otherlv_36, grammarAccess.getDomainEventAccess().getEqualsSignKeyword_7_2_7_1());
// InternalContextMappingDSL.g:10678:10: ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) )
// InternalContextMappingDSL.g:10679:11: (lv_discriminatorType_37_0= ruleDiscriminatorType )
{
// InternalContextMappingDSL.g:10679:11: (lv_discriminatorType_37_0= ruleDiscriminatorType )
// InternalContextMappingDSL.g:10680:12: lv_discriminatorType_37_0= ruleDiscriminatorType
{
newCompositeNode(grammarAccess.getDomainEventAccess().getDiscriminatorTypeDiscriminatorTypeEnumRuleCall_7_2_7_2_0());
pushFollow(FOLLOW_151);
lv_discriminatorType_37_0=ruleDiscriminatorType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDomainEventRule());
}
set(
current,
"discriminatorType",
lv_discriminatorType_37_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.DiscriminatorType");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 9 :
// InternalContextMappingDSL.g:10703:5: ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:10703:5: ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:10704:6: {...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 8) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 8)");
}
// InternalContextMappingDSL.g:10704:111: ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:10705:7: ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 8);
// InternalContextMappingDSL.g:10708:10: ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:10708:11: {...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "true");
}
// InternalContextMappingDSL.g:10708:20: (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:10708:21: otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) )
{
otherlv_38=(Token)match(input,131,FOLLOW_89);
newLeafNode(otherlv_38, grammarAccess.getDomainEventAccess().getDiscriminatorLengthKeyword_7_2_8_0());
otherlv_39=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_39, grammarAccess.getDomainEventAccess().getEqualsSignKeyword_7_2_8_1());
// InternalContextMappingDSL.g:10716:10: ( (lv_discriminatorLength_40_0= RULE_STRING ) )
// InternalContextMappingDSL.g:10717:11: (lv_discriminatorLength_40_0= RULE_STRING )
{
// InternalContextMappingDSL.g:10717:11: (lv_discriminatorLength_40_0= RULE_STRING )
// InternalContextMappingDSL.g:10718:12: lv_discriminatorLength_40_0= RULE_STRING
{
lv_discriminatorLength_40_0=(Token)match(input,RULE_STRING,FOLLOW_151);
newLeafNode(lv_discriminatorLength_40_0, grammarAccess.getDomainEventAccess().getDiscriminatorLengthSTRINGTerminalRuleCall_7_2_8_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
setWithLastConsumed(
current,
"discriminatorLength",
lv_discriminatorLength_40_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 10 :
// InternalContextMappingDSL.g:10740:5: ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) )
{
// InternalContextMappingDSL.g:10740:5: ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) )
// InternalContextMappingDSL.g:10741:6: {...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 9) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 9)");
}
// InternalContextMappingDSL.g:10741:111: ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) )
// InternalContextMappingDSL.g:10742:7: ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 9);
// InternalContextMappingDSL.g:10745:10: ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) )
// InternalContextMappingDSL.g:10745:11: {...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "true");
}
// InternalContextMappingDSL.g:10745:20: (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) )
// InternalContextMappingDSL.g:10745:21: otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) )
{
otherlv_41=(Token)match(input,132,FOLLOW_89);
newLeafNode(otherlv_41, grammarAccess.getDomainEventAccess().getInheritanceTypeKeyword_7_2_9_0());
otherlv_42=(Token)match(input,21,FOLLOW_140);
newLeafNode(otherlv_42, grammarAccess.getDomainEventAccess().getEqualsSignKeyword_7_2_9_1());
// InternalContextMappingDSL.g:10753:10: ( (lv_inheritanceType_43_0= ruleInheritanceType ) )
// InternalContextMappingDSL.g:10754:11: (lv_inheritanceType_43_0= ruleInheritanceType )
{
// InternalContextMappingDSL.g:10754:11: (lv_inheritanceType_43_0= ruleInheritanceType )
// InternalContextMappingDSL.g:10755:12: lv_inheritanceType_43_0= ruleInheritanceType
{
newCompositeNode(grammarAccess.getDomainEventAccess().getInheritanceTypeInheritanceTypeEnumRuleCall_7_2_9_2_0());
pushFollow(FOLLOW_151);
lv_inheritanceType_43_0=ruleInheritanceType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDomainEventRule());
}
set(
current,
"inheritanceType",
lv_inheritanceType_43_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.InheritanceType");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 11 :
// InternalContextMappingDSL.g:10778:5: ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:10778:5: ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:10779:6: {...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 10) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 10)");
}
// InternalContextMappingDSL.g:10779:112: ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:10780:7: ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 10);
// InternalContextMappingDSL.g:10783:10: ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:10783:11: {...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "true");
}
// InternalContextMappingDSL.g:10783:20: (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:10783:21: otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) )
{
otherlv_44=(Token)match(input,133,FOLLOW_89);
newLeafNode(otherlv_44, grammarAccess.getDomainEventAccess().getValidateKeyword_7_2_10_0());
otherlv_45=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_45, grammarAccess.getDomainEventAccess().getEqualsSignKeyword_7_2_10_1());
// InternalContextMappingDSL.g:10791:10: ( (lv_validate_46_0= RULE_STRING ) )
// InternalContextMappingDSL.g:10792:11: (lv_validate_46_0= RULE_STRING )
{
// InternalContextMappingDSL.g:10792:11: (lv_validate_46_0= RULE_STRING )
// InternalContextMappingDSL.g:10793:12: lv_validate_46_0= RULE_STRING
{
lv_validate_46_0=(Token)match(input,RULE_STRING,FOLLOW_151);
newLeafNode(lv_validate_46_0, grammarAccess.getDomainEventAccess().getValidateSTRINGTerminalRuleCall_7_2_10_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
setWithLastConsumed(
current,
"validate",
lv_validate_46_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 12 :
// InternalContextMappingDSL.g:10815:5: ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) )
{
// InternalContextMappingDSL.g:10815:5: ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) )
// InternalContextMappingDSL.g:10816:6: {...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 11) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 11)");
}
// InternalContextMappingDSL.g:10816:112: ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) )
// InternalContextMappingDSL.g:10817:7: ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 11);
// InternalContextMappingDSL.g:10820:10: ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) )
// InternalContextMappingDSL.g:10820:11: {...}? => ( (lv_persistent_47_0= 'persistent' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "true");
}
// InternalContextMappingDSL.g:10820:20: ( (lv_persistent_47_0= 'persistent' ) )
// InternalContextMappingDSL.g:10820:21: (lv_persistent_47_0= 'persistent' )
{
// InternalContextMappingDSL.g:10820:21: (lv_persistent_47_0= 'persistent' )
// InternalContextMappingDSL.g:10821:11: lv_persistent_47_0= 'persistent'
{
lv_persistent_47_0=(Token)match(input,138,FOLLOW_151);
newLeafNode(lv_persistent_47_0, grammarAccess.getDomainEventAccess().getPersistentPersistentKeyword_7_2_11_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
setWithLastConsumed(current, "persistent", true, "persistent");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 13 :
// InternalContextMappingDSL.g:10838:5: ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) )
{
// InternalContextMappingDSL.g:10838:5: ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) )
// InternalContextMappingDSL.g:10839:6: {...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 12) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 12)");
}
// InternalContextMappingDSL.g:10839:112: ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) )
// InternalContextMappingDSL.g:10840:7: ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 12);
// InternalContextMappingDSL.g:10843:10: ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) )
// InternalContextMappingDSL.g:10843:11: {...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "true");
}
// InternalContextMappingDSL.g:10843:20: ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) )
// InternalContextMappingDSL.g:10843:21: (lv_aggregateRoot_48_0= 'aggregateRoot' )
{
// InternalContextMappingDSL.g:10843:21: (lv_aggregateRoot_48_0= 'aggregateRoot' )
// InternalContextMappingDSL.g:10844:11: lv_aggregateRoot_48_0= 'aggregateRoot'
{
lv_aggregateRoot_48_0=(Token)match(input,134,FOLLOW_151);
newLeafNode(lv_aggregateRoot_48_0, grammarAccess.getDomainEventAccess().getAggregateRootAggregateRootKeyword_7_2_12_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
setWithLastConsumed(current, "aggregateRoot", true, "aggregateRoot");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 14 :
// InternalContextMappingDSL.g:10861:5: ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) )
{
// InternalContextMappingDSL.g:10861:5: ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) )
// InternalContextMappingDSL.g:10862:6: {...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 13) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 13)");
}
// InternalContextMappingDSL.g:10862:112: ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) )
// InternalContextMappingDSL.g:10863:7: ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 13);
// InternalContextMappingDSL.g:10866:10: ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) )
// InternalContextMappingDSL.g:10866:11: {...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomainEvent", "true");
}
// InternalContextMappingDSL.g:10866:20: (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) )
// InternalContextMappingDSL.g:10866:21: otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) )
{
otherlv_49=(Token)match(input,135,FOLLOW_105);
newLeafNode(otherlv_49, grammarAccess.getDomainEventAccess().getBelongsToKeyword_7_2_13_0());
// InternalContextMappingDSL.g:10870:10: ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) )
// InternalContextMappingDSL.g:10871:11: (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) )
{
// InternalContextMappingDSL.g:10871:11: (otherlv_50= '@' )?
int alt267=2;
int LA267_0 = input.LA(1);
if ( (LA267_0==94) ) {
alt267=1;
}
switch (alt267) {
case 1 :
// InternalContextMappingDSL.g:10872:12: otherlv_50= '@'
{
otherlv_50=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_50, grammarAccess.getDomainEventAccess().getCommercialAtKeyword_7_2_13_1_0());
}
break;
}
// InternalContextMappingDSL.g:10877:11: ( (otherlv_51= RULE_ID ) )
// InternalContextMappingDSL.g:10878:12: (otherlv_51= RULE_ID )
{
// InternalContextMappingDSL.g:10878:12: (otherlv_51= RULE_ID )
// InternalContextMappingDSL.g:10879:13: otherlv_51= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getDomainEventRule());
}
otherlv_51=(Token)match(input,RULE_ID,FOLLOW_151);
newLeafNode(otherlv_51, grammarAccess.getDomainEventAccess().getBelongsToDomainObjectCrossReference_7_2_13_1_1_0());
}
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
default :
break loop268;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2());
}
// InternalContextMappingDSL.g:10904:4: ( ( (lv_attributes_52_0= ruleAttribute ) ) | ( (lv_references_53_0= ruleReference ) ) | ( (lv_operations_54_0= ruleDomainObjectOperation ) ) )*
loop269:
do {
int alt269=4;
switch ( input.LA(1) ) {
case RULE_STRING:
{
switch ( input.LA(2) ) {
case 142:
case 143:
{
alt269=3;
}
break;
case RULE_REF:
{
alt269=2;
}
break;
case RULE_ID:
case 124:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
case 247:
case 248:
case 249:
{
alt269=1;
}
break;
}
}
break;
case RULE_ID:
case 124:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
case 247:
case 248:
case 249:
{
alt269=1;
}
break;
case RULE_REF:
{
alt269=2;
}
break;
case 142:
case 143:
{
alt269=3;
}
break;
}
switch (alt269) {
case 1 :
// InternalContextMappingDSL.g:10905:5: ( (lv_attributes_52_0= ruleAttribute ) )
{
// InternalContextMappingDSL.g:10905:5: ( (lv_attributes_52_0= ruleAttribute ) )
// InternalContextMappingDSL.g:10906:6: (lv_attributes_52_0= ruleAttribute )
{
// InternalContextMappingDSL.g:10906:6: (lv_attributes_52_0= ruleAttribute )
// InternalContextMappingDSL.g:10907:7: lv_attributes_52_0= ruleAttribute
{
newCompositeNode(grammarAccess.getDomainEventAccess().getAttributesAttributeParserRuleCall_7_3_0_0());
pushFollow(FOLLOW_141);
lv_attributes_52_0=ruleAttribute();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDomainEventRule());
}
add(
current,
"attributes",
lv_attributes_52_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Attribute");
afterParserOrEnumRuleCall();
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:10925:5: ( (lv_references_53_0= ruleReference ) )
{
// InternalContextMappingDSL.g:10925:5: ( (lv_references_53_0= ruleReference ) )
// InternalContextMappingDSL.g:10926:6: (lv_references_53_0= ruleReference )
{
// InternalContextMappingDSL.g:10926:6: (lv_references_53_0= ruleReference )
// InternalContextMappingDSL.g:10927:7: lv_references_53_0= ruleReference
{
newCompositeNode(grammarAccess.getDomainEventAccess().getReferencesReferenceParserRuleCall_7_3_1_0());
pushFollow(FOLLOW_141);
lv_references_53_0=ruleReference();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDomainEventRule());
}
add(
current,
"references",
lv_references_53_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Reference");
afterParserOrEnumRuleCall();
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:10945:5: ( (lv_operations_54_0= ruleDomainObjectOperation ) )
{
// InternalContextMappingDSL.g:10945:5: ( (lv_operations_54_0= ruleDomainObjectOperation ) )
// InternalContextMappingDSL.g:10946:6: (lv_operations_54_0= ruleDomainObjectOperation )
{
// InternalContextMappingDSL.g:10946:6: (lv_operations_54_0= ruleDomainObjectOperation )
// InternalContextMappingDSL.g:10947:7: lv_operations_54_0= ruleDomainObjectOperation
{
newCompositeNode(grammarAccess.getDomainEventAccess().getOperationsDomainObjectOperationParserRuleCall_7_3_2_0());
pushFollow(FOLLOW_141);
lv_operations_54_0=ruleDomainObjectOperation();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDomainEventRule());
}
add(
current,
"operations",
lv_operations_54_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.DomainObjectOperation");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop269;
}
} while (true);
// InternalContextMappingDSL.g:10965:4: ( (lv_repository_55_0= ruleRepository ) )?
int alt270=2;
int LA270_0 = input.LA(1);
if ( (LA270_0==RULE_STRING||LA270_0==181) ) {
alt270=1;
}
switch (alt270) {
case 1 :
// InternalContextMappingDSL.g:10966:5: (lv_repository_55_0= ruleRepository )
{
// InternalContextMappingDSL.g:10966:5: (lv_repository_55_0= ruleRepository )
// InternalContextMappingDSL.g:10967:6: lv_repository_55_0= ruleRepository
{
newCompositeNode(grammarAccess.getDomainEventAccess().getRepositoryRepositoryParserRuleCall_7_4_0());
pushFollow(FOLLOW_36);
lv_repository_55_0=ruleRepository();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDomainEventRule());
}
set(
current,
"repository",
lv_repository_55_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Repository");
afterParserOrEnumRuleCall();
}
}
break;
}
otherlv_56=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(otherlv_56, grammarAccess.getDomainEventAccess().getRightCurlyBracketKeyword_7_5());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleDomainEvent"
// $ANTLR start "entryRuleCommandEvent"
// InternalContextMappingDSL.g:10993:1: entryRuleCommandEvent returns [EObject current=null] : iv_ruleCommandEvent= ruleCommandEvent EOF ;
public final EObject entryRuleCommandEvent() throws RecognitionException {
EObject current = null;
EObject iv_ruleCommandEvent = null;
try {
// InternalContextMappingDSL.g:10993:53: (iv_ruleCommandEvent= ruleCommandEvent EOF )
// InternalContextMappingDSL.g:10994:2: iv_ruleCommandEvent= ruleCommandEvent EOF
{
newCompositeNode(grammarAccess.getCommandEventRule());
pushFollow(FOLLOW_1);
iv_ruleCommandEvent=ruleCommandEvent();
state._fsp--;
current =iv_ruleCommandEvent;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleCommandEvent"
// $ANTLR start "ruleCommandEvent"
// InternalContextMappingDSL.g:11000:1: ruleCommandEvent returns [EObject current=null] : ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'CommandEvent' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_52_0= ruleAttribute ) ) | ( (lv_references_53_0= ruleReference ) ) | ( (lv_operations_54_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_55_0= ruleRepository ) )? otherlv_56= '}' )? ) ;
public final EObject ruleCommandEvent() throws RecognitionException {
EObject current = null;
Token lv_comment_0_0=null;
Token lv_doc_1_0=null;
Token lv_abstract_2_0=null;
Token otherlv_3=null;
Token lv_name_4_0=null;
Token otherlv_5=null;
Token otherlv_6=null;
Token otherlv_7=null;
Token otherlv_9=null;
Token otherlv_10=null;
Token otherlv_11=null;
Token otherlv_12=null;
Token otherlv_13=null;
Token otherlv_14=null;
Token lv_cache_17_0=null;
Token this_NOT_18=null;
Token otherlv_19=null;
Token lv_gapClass_20_0=null;
Token lv_noGapClass_21_0=null;
Token lv_scaffold_22_0=null;
Token otherlv_23=null;
Token otherlv_24=null;
Token lv_hint_25_0=null;
Token otherlv_26=null;
Token otherlv_27=null;
Token lv_databaseTable_28_0=null;
Token otherlv_29=null;
Token otherlv_30=null;
Token lv_discriminatorValue_31_0=null;
Token otherlv_32=null;
Token otherlv_33=null;
Token lv_discriminatorColumn_34_0=null;
Token otherlv_35=null;
Token otherlv_36=null;
Token otherlv_38=null;
Token otherlv_39=null;
Token lv_discriminatorLength_40_0=null;
Token otherlv_41=null;
Token otherlv_42=null;
Token otherlv_44=null;
Token otherlv_45=null;
Token lv_validate_46_0=null;
Token lv_persistent_47_0=null;
Token lv_aggregateRoot_48_0=null;
Token otherlv_49=null;
Token otherlv_50=null;
Token otherlv_51=null;
Token otherlv_56=null;
AntlrDatatypeRuleToken lv_extendsName_8_0 = null;
AntlrDatatypeRuleToken lv_package_15_0 = null;
Enumerator lv_discriminatorType_37_0 = null;
Enumerator lv_inheritanceType_43_0 = null;
EObject lv_attributes_52_0 = null;
EObject lv_references_53_0 = null;
EObject lv_operations_54_0 = null;
EObject lv_repository_55_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:11006:2: ( ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'CommandEvent' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_52_0= ruleAttribute ) ) | ( (lv_references_53_0= ruleReference ) ) | ( (lv_operations_54_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_55_0= ruleRepository ) )? otherlv_56= '}' )? ) )
// InternalContextMappingDSL.g:11007:2: ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'CommandEvent' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_52_0= ruleAttribute ) ) | ( (lv_references_53_0= ruleReference ) ) | ( (lv_operations_54_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_55_0= ruleRepository ) )? otherlv_56= '}' )? )
{
// InternalContextMappingDSL.g:11007:2: ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'CommandEvent' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_52_0= ruleAttribute ) ) | ( (lv_references_53_0= ruleReference ) ) | ( (lv_operations_54_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_55_0= ruleRepository ) )? otherlv_56= '}' )? )
// InternalContextMappingDSL.g:11008:3: ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'CommandEvent' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )* (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_52_0= ruleAttribute ) ) | ( (lv_references_53_0= ruleReference ) ) | ( (lv_operations_54_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_55_0= ruleRepository ) )? otherlv_56= '}' )?
{
// InternalContextMappingDSL.g:11008:3: ( (lv_comment_0_0= RULE_ML_COMMENT ) )?
int alt272=2;
int LA272_0 = input.LA(1);
if ( (LA272_0==RULE_ML_COMMENT) ) {
alt272=1;
}
switch (alt272) {
case 1 :
// InternalContextMappingDSL.g:11009:4: (lv_comment_0_0= RULE_ML_COMMENT )
{
// InternalContextMappingDSL.g:11009:4: (lv_comment_0_0= RULE_ML_COMMENT )
// InternalContextMappingDSL.g:11010:5: lv_comment_0_0= RULE_ML_COMMENT
{
lv_comment_0_0=(Token)match(input,RULE_ML_COMMENT,FOLLOW_152);
newLeafNode(lv_comment_0_0, grammarAccess.getCommandEventAccess().getCommentML_COMMENTTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
setWithLastConsumed(
current,
"comment",
lv_comment_0_0,
"org.eclipse.xtext.common.Terminals.ML_COMMENT");
}
}
break;
}
// InternalContextMappingDSL.g:11026:3: ( (lv_doc_1_0= RULE_STRING ) )?
int alt273=2;
int LA273_0 = input.LA(1);
if ( (LA273_0==RULE_STRING) ) {
alt273=1;
}
switch (alt273) {
case 1 :
// InternalContextMappingDSL.g:11027:4: (lv_doc_1_0= RULE_STRING )
{
// InternalContextMappingDSL.g:11027:4: (lv_doc_1_0= RULE_STRING )
// InternalContextMappingDSL.g:11028:5: lv_doc_1_0= RULE_STRING
{
lv_doc_1_0=(Token)match(input,RULE_STRING,FOLLOW_153);
newLeafNode(lv_doc_1_0, grammarAccess.getCommandEventAccess().getDocSTRINGTerminalRuleCall_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_1_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
// InternalContextMappingDSL.g:11044:3: ( (lv_abstract_2_0= 'abstract' ) )?
int alt274=2;
int LA274_0 = input.LA(1);
if ( (LA274_0==120) ) {
alt274=1;
}
switch (alt274) {
case 1 :
// InternalContextMappingDSL.g:11045:4: (lv_abstract_2_0= 'abstract' )
{
// InternalContextMappingDSL.g:11045:4: (lv_abstract_2_0= 'abstract' )
// InternalContextMappingDSL.g:11046:5: lv_abstract_2_0= 'abstract'
{
lv_abstract_2_0=(Token)match(input,120,FOLLOW_154);
newLeafNode(lv_abstract_2_0, grammarAccess.getCommandEventAccess().getAbstractAbstractKeyword_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
setWithLastConsumed(current, "abstract", true, "abstract");
}
}
break;
}
otherlv_3=(Token)match(input,140,FOLLOW_10);
newLeafNode(otherlv_3, grammarAccess.getCommandEventAccess().getCommandEventKeyword_3());
// InternalContextMappingDSL.g:11062:3: ( (lv_name_4_0= RULE_ID ) )
// InternalContextMappingDSL.g:11063:4: (lv_name_4_0= RULE_ID )
{
// InternalContextMappingDSL.g:11063:4: (lv_name_4_0= RULE_ID )
// InternalContextMappingDSL.g:11064:5: lv_name_4_0= RULE_ID
{
lv_name_4_0=(Token)match(input,RULE_ID,FOLLOW_133);
newLeafNode(lv_name_4_0, grammarAccess.getCommandEventAccess().getNameIDTerminalRuleCall_4_0());
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
setWithLastConsumed(
current,
"name",
lv_name_4_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:11080:3: (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )?
int alt276=2;
int LA276_0 = input.LA(1);
if ( (LA276_0==122) ) {
alt276=1;
}
switch (alt276) {
case 1 :
// InternalContextMappingDSL.g:11081:4: otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) )
{
otherlv_5=(Token)match(input,122,FOLLOW_105);
newLeafNode(otherlv_5, grammarAccess.getCommandEventAccess().getExtendsKeyword_5_0());
// InternalContextMappingDSL.g:11085:4: ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) )
int alt275=2;
int LA275_0 = input.LA(1);
if ( (LA275_0==94) ) {
alt275=1;
}
else if ( (LA275_0==RULE_ID) ) {
alt275=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 275, 0, input);
throw nvae;
}
switch (alt275) {
case 1 :
// InternalContextMappingDSL.g:11086:5: (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:11086:5: (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) )
// InternalContextMappingDSL.g:11087:6: otherlv_6= '@' ( (otherlv_7= RULE_ID ) )
{
otherlv_6=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_6, grammarAccess.getCommandEventAccess().getCommercialAtKeyword_5_1_0_0());
// InternalContextMappingDSL.g:11091:6: ( (otherlv_7= RULE_ID ) )
// InternalContextMappingDSL.g:11092:7: (otherlv_7= RULE_ID )
{
// InternalContextMappingDSL.g:11092:7: (otherlv_7= RULE_ID )
// InternalContextMappingDSL.g:11093:8: otherlv_7= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
otherlv_7=(Token)match(input,RULE_ID,FOLLOW_134);
newLeafNode(otherlv_7, grammarAccess.getCommandEventAccess().getExtendsCommandEventCrossReference_5_1_0_1_0());
}
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:11106:5: ( (lv_extendsName_8_0= ruleJavaIdentifier ) )
{
// InternalContextMappingDSL.g:11106:5: ( (lv_extendsName_8_0= ruleJavaIdentifier ) )
// InternalContextMappingDSL.g:11107:6: (lv_extendsName_8_0= ruleJavaIdentifier )
{
// InternalContextMappingDSL.g:11107:6: (lv_extendsName_8_0= ruleJavaIdentifier )
// InternalContextMappingDSL.g:11108:7: lv_extendsName_8_0= ruleJavaIdentifier
{
newCompositeNode(grammarAccess.getCommandEventAccess().getExtendsNameJavaIdentifierParserRuleCall_5_1_1_0());
pushFollow(FOLLOW_134);
lv_extendsName_8_0=ruleJavaIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCommandEventRule());
}
set(
current,
"extendsName",
lv_extendsName_8_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.JavaIdentifier");
afterParserOrEnumRuleCall();
}
}
}
break;
}
}
break;
}
// InternalContextMappingDSL.g:11127:3: (otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) ) )*
loop278:
do {
int alt278=2;
int LA278_0 = input.LA(1);
if ( (LA278_0==123) ) {
alt278=1;
}
switch (alt278) {
case 1 :
// InternalContextMappingDSL.g:11128:4: otherlv_9= 'with' (otherlv_10= '@' )? ( (otherlv_11= RULE_ID ) )
{
otherlv_9=(Token)match(input,123,FOLLOW_105);
newLeafNode(otherlv_9, grammarAccess.getCommandEventAccess().getWithKeyword_6_0());
// InternalContextMappingDSL.g:11132:4: (otherlv_10= '@' )?
int alt277=2;
int LA277_0 = input.LA(1);
if ( (LA277_0==94) ) {
alt277=1;
}
switch (alt277) {
case 1 :
// InternalContextMappingDSL.g:11133:5: otherlv_10= '@'
{
otherlv_10=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_10, grammarAccess.getCommandEventAccess().getCommercialAtKeyword_6_1());
}
break;
}
// InternalContextMappingDSL.g:11138:4: ( (otherlv_11= RULE_ID ) )
// InternalContextMappingDSL.g:11139:5: (otherlv_11= RULE_ID )
{
// InternalContextMappingDSL.g:11139:5: (otherlv_11= RULE_ID )
// InternalContextMappingDSL.g:11140:6: otherlv_11= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
otherlv_11=(Token)match(input,RULE_ID,FOLLOW_134);
newLeafNode(otherlv_11, grammarAccess.getCommandEventAccess().getTraitsTraitCrossReference_6_2_0());
}
}
}
break;
default :
break loop278;
}
} while (true);
// InternalContextMappingDSL.g:11152:3: (otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_52_0= ruleAttribute ) ) | ( (lv_references_53_0= ruleReference ) ) | ( (lv_operations_54_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_55_0= ruleRepository ) )? otherlv_56= '}' )?
int alt286=2;
int LA286_0 = input.LA(1);
if ( (LA286_0==RULE_OPEN) ) {
alt286=1;
}
switch (alt286) {
case 1 :
// InternalContextMappingDSL.g:11153:4: otherlv_12= '{' (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_52_0= ruleAttribute ) ) | ( (lv_references_53_0= ruleReference ) ) | ( (lv_operations_54_0= ruleDomainObjectOperation ) ) )* ( (lv_repository_55_0= ruleRepository ) )? otherlv_56= '}'
{
otherlv_12=(Token)match(input,RULE_OPEN,FOLLOW_151);
newLeafNode(otherlv_12, grammarAccess.getCommandEventAccess().getLeftCurlyBracketKeyword_7_0());
// InternalContextMappingDSL.g:11157:4: (otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) ) )?
int alt279=2;
int LA279_0 = input.LA(1);
if ( (LA279_0==124) ) {
int LA279_1 = input.LA(2);
if ( (LA279_1==21) ) {
alt279=1;
}
}
switch (alt279) {
case 1 :
// InternalContextMappingDSL.g:11158:5: otherlv_13= 'package' otherlv_14= '=' ( (lv_package_15_0= ruleJavaIdentifier ) )
{
otherlv_13=(Token)match(input,124,FOLLOW_89);
newLeafNode(otherlv_13, grammarAccess.getCommandEventAccess().getPackageKeyword_7_1_0());
otherlv_14=(Token)match(input,21,FOLLOW_10);
newLeafNode(otherlv_14, grammarAccess.getCommandEventAccess().getEqualsSignKeyword_7_1_1());
// InternalContextMappingDSL.g:11166:5: ( (lv_package_15_0= ruleJavaIdentifier ) )
// InternalContextMappingDSL.g:11167:6: (lv_package_15_0= ruleJavaIdentifier )
{
// InternalContextMappingDSL.g:11167:6: (lv_package_15_0= ruleJavaIdentifier )
// InternalContextMappingDSL.g:11168:7: lv_package_15_0= ruleJavaIdentifier
{
newCompositeNode(grammarAccess.getCommandEventAccess().getPackageJavaIdentifierParserRuleCall_7_1_2_0());
pushFollow(FOLLOW_151);
lv_package_15_0=ruleJavaIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCommandEventRule());
}
set(
current,
"package",
lv_package_15_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.JavaIdentifier");
afterParserOrEnumRuleCall();
}
}
}
break;
}
// InternalContextMappingDSL.g:11186:4: ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:11187:5: ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:11187:5: ( ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:11188:6: ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2());
// InternalContextMappingDSL.g:11191:6: ( ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:11192:7: ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:11192:7: ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )*
loop283:
do {
int alt283=15;
alt283 = dfa283.predict(input);
switch (alt283) {
case 1 :
// InternalContextMappingDSL.g:11193:5: ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) )
{
// InternalContextMappingDSL.g:11193:5: ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) )
// InternalContextMappingDSL.g:11194:6: {...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 0) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 0)");
}
// InternalContextMappingDSL.g:11194:112: ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) )
// InternalContextMappingDSL.g:11195:7: ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 0);
// InternalContextMappingDSL.g:11198:10: ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) )
// InternalContextMappingDSL.g:11198:11: {...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "true");
}
// InternalContextMappingDSL.g:11198:20: ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) )
int alt280=2;
int LA280_0 = input.LA(1);
if ( (LA280_0==108) ) {
alt280=1;
}
else if ( (LA280_0==RULE_NOT) ) {
alt280=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 280, 0, input);
throw nvae;
}
switch (alt280) {
case 1 :
// InternalContextMappingDSL.g:11198:21: ( (lv_cache_17_0= 'cache' ) )
{
// InternalContextMappingDSL.g:11198:21: ( (lv_cache_17_0= 'cache' ) )
// InternalContextMappingDSL.g:11199:11: (lv_cache_17_0= 'cache' )
{
// InternalContextMappingDSL.g:11199:11: (lv_cache_17_0= 'cache' )
// InternalContextMappingDSL.g:11200:12: lv_cache_17_0= 'cache'
{
lv_cache_17_0=(Token)match(input,108,FOLLOW_151);
newLeafNode(lv_cache_17_0, grammarAccess.getCommandEventAccess().getCacheCacheKeyword_7_2_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
setWithLastConsumed(current, "cache", true, "cache");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:11213:10: (this_NOT_18= RULE_NOT otherlv_19= 'cache' )
{
// InternalContextMappingDSL.g:11213:10: (this_NOT_18= RULE_NOT otherlv_19= 'cache' )
// InternalContextMappingDSL.g:11214:11: this_NOT_18= RULE_NOT otherlv_19= 'cache'
{
this_NOT_18=(Token)match(input,RULE_NOT,FOLLOW_138);
newLeafNode(this_NOT_18, grammarAccess.getCommandEventAccess().getNOTTerminalRuleCall_7_2_0_1_0());
otherlv_19=(Token)match(input,108,FOLLOW_151);
newLeafNode(otherlv_19, grammarAccess.getCommandEventAccess().getCacheKeyword_7_2_0_1_1());
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:11229:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) )
{
// InternalContextMappingDSL.g:11229:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) )
// InternalContextMappingDSL.g:11230:6: {...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 1) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 1)");
}
// InternalContextMappingDSL.g:11230:112: ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) )
// InternalContextMappingDSL.g:11231:7: ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 1);
// InternalContextMappingDSL.g:11234:10: ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) )
// InternalContextMappingDSL.g:11234:11: {...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "true");
}
// InternalContextMappingDSL.g:11234:20: ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) )
int alt281=2;
int LA281_0 = input.LA(1);
if ( (LA281_0==86) ) {
alt281=1;
}
else if ( (LA281_0==87) ) {
alt281=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 281, 0, input);
throw nvae;
}
switch (alt281) {
case 1 :
// InternalContextMappingDSL.g:11234:21: ( (lv_gapClass_20_0= 'gap' ) )
{
// InternalContextMappingDSL.g:11234:21: ( (lv_gapClass_20_0= 'gap' ) )
// InternalContextMappingDSL.g:11235:11: (lv_gapClass_20_0= 'gap' )
{
// InternalContextMappingDSL.g:11235:11: (lv_gapClass_20_0= 'gap' )
// InternalContextMappingDSL.g:11236:12: lv_gapClass_20_0= 'gap'
{
lv_gapClass_20_0=(Token)match(input,86,FOLLOW_151);
newLeafNode(lv_gapClass_20_0, grammarAccess.getCommandEventAccess().getGapClassGapKeyword_7_2_1_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
setWithLastConsumed(current, "gapClass", true, "gap");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:11249:10: ( (lv_noGapClass_21_0= 'nogap' ) )
{
// InternalContextMappingDSL.g:11249:10: ( (lv_noGapClass_21_0= 'nogap' ) )
// InternalContextMappingDSL.g:11250:11: (lv_noGapClass_21_0= 'nogap' )
{
// InternalContextMappingDSL.g:11250:11: (lv_noGapClass_21_0= 'nogap' )
// InternalContextMappingDSL.g:11251:12: lv_noGapClass_21_0= 'nogap'
{
lv_noGapClass_21_0=(Token)match(input,87,FOLLOW_151);
newLeafNode(lv_noGapClass_21_0, grammarAccess.getCommandEventAccess().getNoGapClassNogapKeyword_7_2_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
setWithLastConsumed(current, "noGapClass", true, "nogap");
}
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:11269:5: ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) )
{
// InternalContextMappingDSL.g:11269:5: ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) )
// InternalContextMappingDSL.g:11270:6: {...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 2) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 2)");
}
// InternalContextMappingDSL.g:11270:112: ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) )
// InternalContextMappingDSL.g:11271:7: ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 2);
// InternalContextMappingDSL.g:11274:10: ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) )
// InternalContextMappingDSL.g:11274:11: {...}? => ( (lv_scaffold_22_0= 'scaffold' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "true");
}
// InternalContextMappingDSL.g:11274:20: ( (lv_scaffold_22_0= 'scaffold' ) )
// InternalContextMappingDSL.g:11274:21: (lv_scaffold_22_0= 'scaffold' )
{
// InternalContextMappingDSL.g:11274:21: (lv_scaffold_22_0= 'scaffold' )
// InternalContextMappingDSL.g:11275:11: lv_scaffold_22_0= 'scaffold'
{
lv_scaffold_22_0=(Token)match(input,90,FOLLOW_151);
newLeafNode(lv_scaffold_22_0, grammarAccess.getCommandEventAccess().getScaffoldScaffoldKeyword_7_2_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
setWithLastConsumed(current, "scaffold", true, "scaffold");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:11292:5: ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:11292:5: ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:11293:6: {...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 3) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 3)");
}
// InternalContextMappingDSL.g:11293:112: ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:11294:7: ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 3);
// InternalContextMappingDSL.g:11297:10: ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:11297:11: {...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "true");
}
// InternalContextMappingDSL.g:11297:20: (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:11297:21: otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) )
{
otherlv_23=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_23, grammarAccess.getCommandEventAccess().getHintKeyword_7_2_3_0());
otherlv_24=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_24, grammarAccess.getCommandEventAccess().getEqualsSignKeyword_7_2_3_1());
// InternalContextMappingDSL.g:11305:10: ( (lv_hint_25_0= RULE_STRING ) )
// InternalContextMappingDSL.g:11306:11: (lv_hint_25_0= RULE_STRING )
{
// InternalContextMappingDSL.g:11306:11: (lv_hint_25_0= RULE_STRING )
// InternalContextMappingDSL.g:11307:12: lv_hint_25_0= RULE_STRING
{
lv_hint_25_0=(Token)match(input,RULE_STRING,FOLLOW_151);
newLeafNode(lv_hint_25_0, grammarAccess.getCommandEventAccess().getHintSTRINGTerminalRuleCall_7_2_3_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_25_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 5 :
// InternalContextMappingDSL.g:11329:5: ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:11329:5: ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:11330:6: {...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 4) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 4)");
}
// InternalContextMappingDSL.g:11330:112: ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:11331:7: ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 4);
// InternalContextMappingDSL.g:11334:10: ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:11334:11: {...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "true");
}
// InternalContextMappingDSL.g:11334:20: (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:11334:21: otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) )
{
otherlv_26=(Token)match(input,127,FOLLOW_89);
newLeafNode(otherlv_26, grammarAccess.getCommandEventAccess().getDatabaseTableKeyword_7_2_4_0());
otherlv_27=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_27, grammarAccess.getCommandEventAccess().getEqualsSignKeyword_7_2_4_1());
// InternalContextMappingDSL.g:11342:10: ( (lv_databaseTable_28_0= RULE_STRING ) )
// InternalContextMappingDSL.g:11343:11: (lv_databaseTable_28_0= RULE_STRING )
{
// InternalContextMappingDSL.g:11343:11: (lv_databaseTable_28_0= RULE_STRING )
// InternalContextMappingDSL.g:11344:12: lv_databaseTable_28_0= RULE_STRING
{
lv_databaseTable_28_0=(Token)match(input,RULE_STRING,FOLLOW_151);
newLeafNode(lv_databaseTable_28_0, grammarAccess.getCommandEventAccess().getDatabaseTableSTRINGTerminalRuleCall_7_2_4_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
setWithLastConsumed(
current,
"databaseTable",
lv_databaseTable_28_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 6 :
// InternalContextMappingDSL.g:11366:5: ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:11366:5: ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:11367:6: {...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 5) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 5)");
}
// InternalContextMappingDSL.g:11367:112: ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:11368:7: ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 5);
// InternalContextMappingDSL.g:11371:10: ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:11371:11: {...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "true");
}
// InternalContextMappingDSL.g:11371:20: (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:11371:21: otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) )
{
otherlv_29=(Token)match(input,128,FOLLOW_89);
newLeafNode(otherlv_29, grammarAccess.getCommandEventAccess().getDiscriminatorValueKeyword_7_2_5_0());
otherlv_30=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_30, grammarAccess.getCommandEventAccess().getEqualsSignKeyword_7_2_5_1());
// InternalContextMappingDSL.g:11379:10: ( (lv_discriminatorValue_31_0= RULE_STRING ) )
// InternalContextMappingDSL.g:11380:11: (lv_discriminatorValue_31_0= RULE_STRING )
{
// InternalContextMappingDSL.g:11380:11: (lv_discriminatorValue_31_0= RULE_STRING )
// InternalContextMappingDSL.g:11381:12: lv_discriminatorValue_31_0= RULE_STRING
{
lv_discriminatorValue_31_0=(Token)match(input,RULE_STRING,FOLLOW_151);
newLeafNode(lv_discriminatorValue_31_0, grammarAccess.getCommandEventAccess().getDiscriminatorValueSTRINGTerminalRuleCall_7_2_5_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
setWithLastConsumed(
current,
"discriminatorValue",
lv_discriminatorValue_31_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 7 :
// InternalContextMappingDSL.g:11403:5: ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:11403:5: ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:11404:6: {...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 6) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 6)");
}
// InternalContextMappingDSL.g:11404:112: ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:11405:7: ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 6);
// InternalContextMappingDSL.g:11408:10: ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:11408:11: {...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "true");
}
// InternalContextMappingDSL.g:11408:20: (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:11408:21: otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) )
{
otherlv_32=(Token)match(input,129,FOLLOW_89);
newLeafNode(otherlv_32, grammarAccess.getCommandEventAccess().getDiscriminatorColumnKeyword_7_2_6_0());
otherlv_33=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_33, grammarAccess.getCommandEventAccess().getEqualsSignKeyword_7_2_6_1());
// InternalContextMappingDSL.g:11416:10: ( (lv_discriminatorColumn_34_0= RULE_STRING ) )
// InternalContextMappingDSL.g:11417:11: (lv_discriminatorColumn_34_0= RULE_STRING )
{
// InternalContextMappingDSL.g:11417:11: (lv_discriminatorColumn_34_0= RULE_STRING )
// InternalContextMappingDSL.g:11418:12: lv_discriminatorColumn_34_0= RULE_STRING
{
lv_discriminatorColumn_34_0=(Token)match(input,RULE_STRING,FOLLOW_151);
newLeafNode(lv_discriminatorColumn_34_0, grammarAccess.getCommandEventAccess().getDiscriminatorColumnSTRINGTerminalRuleCall_7_2_6_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
setWithLastConsumed(
current,
"discriminatorColumn",
lv_discriminatorColumn_34_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 8 :
// InternalContextMappingDSL.g:11440:5: ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) )
{
// InternalContextMappingDSL.g:11440:5: ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) )
// InternalContextMappingDSL.g:11441:6: {...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 7) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 7)");
}
// InternalContextMappingDSL.g:11441:112: ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) )
// InternalContextMappingDSL.g:11442:7: ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 7);
// InternalContextMappingDSL.g:11445:10: ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) )
// InternalContextMappingDSL.g:11445:11: {...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "true");
}
// InternalContextMappingDSL.g:11445:20: (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) )
// InternalContextMappingDSL.g:11445:21: otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) )
{
otherlv_35=(Token)match(input,130,FOLLOW_89);
newLeafNode(otherlv_35, grammarAccess.getCommandEventAccess().getDiscriminatorTypeKeyword_7_2_7_0());
otherlv_36=(Token)match(input,21,FOLLOW_139);
newLeafNode(otherlv_36, grammarAccess.getCommandEventAccess().getEqualsSignKeyword_7_2_7_1());
// InternalContextMappingDSL.g:11453:10: ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) )
// InternalContextMappingDSL.g:11454:11: (lv_discriminatorType_37_0= ruleDiscriminatorType )
{
// InternalContextMappingDSL.g:11454:11: (lv_discriminatorType_37_0= ruleDiscriminatorType )
// InternalContextMappingDSL.g:11455:12: lv_discriminatorType_37_0= ruleDiscriminatorType
{
newCompositeNode(grammarAccess.getCommandEventAccess().getDiscriminatorTypeDiscriminatorTypeEnumRuleCall_7_2_7_2_0());
pushFollow(FOLLOW_151);
lv_discriminatorType_37_0=ruleDiscriminatorType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCommandEventRule());
}
set(
current,
"discriminatorType",
lv_discriminatorType_37_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.DiscriminatorType");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 9 :
// InternalContextMappingDSL.g:11478:5: ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:11478:5: ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:11479:6: {...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 8) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 8)");
}
// InternalContextMappingDSL.g:11479:112: ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:11480:7: ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 8);
// InternalContextMappingDSL.g:11483:10: ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:11483:11: {...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "true");
}
// InternalContextMappingDSL.g:11483:20: (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:11483:21: otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) )
{
otherlv_38=(Token)match(input,131,FOLLOW_89);
newLeafNode(otherlv_38, grammarAccess.getCommandEventAccess().getDiscriminatorLengthKeyword_7_2_8_0());
otherlv_39=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_39, grammarAccess.getCommandEventAccess().getEqualsSignKeyword_7_2_8_1());
// InternalContextMappingDSL.g:11491:10: ( (lv_discriminatorLength_40_0= RULE_STRING ) )
// InternalContextMappingDSL.g:11492:11: (lv_discriminatorLength_40_0= RULE_STRING )
{
// InternalContextMappingDSL.g:11492:11: (lv_discriminatorLength_40_0= RULE_STRING )
// InternalContextMappingDSL.g:11493:12: lv_discriminatorLength_40_0= RULE_STRING
{
lv_discriminatorLength_40_0=(Token)match(input,RULE_STRING,FOLLOW_151);
newLeafNode(lv_discriminatorLength_40_0, grammarAccess.getCommandEventAccess().getDiscriminatorLengthSTRINGTerminalRuleCall_7_2_8_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
setWithLastConsumed(
current,
"discriminatorLength",
lv_discriminatorLength_40_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 10 :
// InternalContextMappingDSL.g:11515:5: ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) )
{
// InternalContextMappingDSL.g:11515:5: ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) )
// InternalContextMappingDSL.g:11516:6: {...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 9) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 9)");
}
// InternalContextMappingDSL.g:11516:112: ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) )
// InternalContextMappingDSL.g:11517:7: ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 9);
// InternalContextMappingDSL.g:11520:10: ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) )
// InternalContextMappingDSL.g:11520:11: {...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "true");
}
// InternalContextMappingDSL.g:11520:20: (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) )
// InternalContextMappingDSL.g:11520:21: otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) )
{
otherlv_41=(Token)match(input,132,FOLLOW_89);
newLeafNode(otherlv_41, grammarAccess.getCommandEventAccess().getInheritanceTypeKeyword_7_2_9_0());
otherlv_42=(Token)match(input,21,FOLLOW_140);
newLeafNode(otherlv_42, grammarAccess.getCommandEventAccess().getEqualsSignKeyword_7_2_9_1());
// InternalContextMappingDSL.g:11528:10: ( (lv_inheritanceType_43_0= ruleInheritanceType ) )
// InternalContextMappingDSL.g:11529:11: (lv_inheritanceType_43_0= ruleInheritanceType )
{
// InternalContextMappingDSL.g:11529:11: (lv_inheritanceType_43_0= ruleInheritanceType )
// InternalContextMappingDSL.g:11530:12: lv_inheritanceType_43_0= ruleInheritanceType
{
newCompositeNode(grammarAccess.getCommandEventAccess().getInheritanceTypeInheritanceTypeEnumRuleCall_7_2_9_2_0());
pushFollow(FOLLOW_151);
lv_inheritanceType_43_0=ruleInheritanceType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCommandEventRule());
}
set(
current,
"inheritanceType",
lv_inheritanceType_43_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.InheritanceType");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 11 :
// InternalContextMappingDSL.g:11553:5: ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:11553:5: ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:11554:6: {...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 10) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 10)");
}
// InternalContextMappingDSL.g:11554:113: ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:11555:7: ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 10);
// InternalContextMappingDSL.g:11558:10: ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:11558:11: {...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "true");
}
// InternalContextMappingDSL.g:11558:20: (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:11558:21: otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) )
{
otherlv_44=(Token)match(input,133,FOLLOW_89);
newLeafNode(otherlv_44, grammarAccess.getCommandEventAccess().getValidateKeyword_7_2_10_0());
otherlv_45=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_45, grammarAccess.getCommandEventAccess().getEqualsSignKeyword_7_2_10_1());
// InternalContextMappingDSL.g:11566:10: ( (lv_validate_46_0= RULE_STRING ) )
// InternalContextMappingDSL.g:11567:11: (lv_validate_46_0= RULE_STRING )
{
// InternalContextMappingDSL.g:11567:11: (lv_validate_46_0= RULE_STRING )
// InternalContextMappingDSL.g:11568:12: lv_validate_46_0= RULE_STRING
{
lv_validate_46_0=(Token)match(input,RULE_STRING,FOLLOW_151);
newLeafNode(lv_validate_46_0, grammarAccess.getCommandEventAccess().getValidateSTRINGTerminalRuleCall_7_2_10_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
setWithLastConsumed(
current,
"validate",
lv_validate_46_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 12 :
// InternalContextMappingDSL.g:11590:5: ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) )
{
// InternalContextMappingDSL.g:11590:5: ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) )
// InternalContextMappingDSL.g:11591:6: {...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 11) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 11)");
}
// InternalContextMappingDSL.g:11591:113: ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) )
// InternalContextMappingDSL.g:11592:7: ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 11);
// InternalContextMappingDSL.g:11595:10: ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) )
// InternalContextMappingDSL.g:11595:11: {...}? => ( (lv_persistent_47_0= 'persistent' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "true");
}
// InternalContextMappingDSL.g:11595:20: ( (lv_persistent_47_0= 'persistent' ) )
// InternalContextMappingDSL.g:11595:21: (lv_persistent_47_0= 'persistent' )
{
// InternalContextMappingDSL.g:11595:21: (lv_persistent_47_0= 'persistent' )
// InternalContextMappingDSL.g:11596:11: lv_persistent_47_0= 'persistent'
{
lv_persistent_47_0=(Token)match(input,138,FOLLOW_151);
newLeafNode(lv_persistent_47_0, grammarAccess.getCommandEventAccess().getPersistentPersistentKeyword_7_2_11_0());
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
setWithLastConsumed(current, "persistent", true, "persistent");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 13 :
// InternalContextMappingDSL.g:11613:5: ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) )
{
// InternalContextMappingDSL.g:11613:5: ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) )
// InternalContextMappingDSL.g:11614:6: {...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 12) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 12)");
}
// InternalContextMappingDSL.g:11614:113: ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) )
// InternalContextMappingDSL.g:11615:7: ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 12);
// InternalContextMappingDSL.g:11618:10: ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) )
// InternalContextMappingDSL.g:11618:11: {...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "true");
}
// InternalContextMappingDSL.g:11618:20: ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) )
// InternalContextMappingDSL.g:11618:21: (lv_aggregateRoot_48_0= 'aggregateRoot' )
{
// InternalContextMappingDSL.g:11618:21: (lv_aggregateRoot_48_0= 'aggregateRoot' )
// InternalContextMappingDSL.g:11619:11: lv_aggregateRoot_48_0= 'aggregateRoot'
{
lv_aggregateRoot_48_0=(Token)match(input,134,FOLLOW_151);
newLeafNode(lv_aggregateRoot_48_0, grammarAccess.getCommandEventAccess().getAggregateRootAggregateRootKeyword_7_2_12_0());
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
setWithLastConsumed(current, "aggregateRoot", true, "aggregateRoot");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
case 14 :
// InternalContextMappingDSL.g:11636:5: ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) )
{
// InternalContextMappingDSL.g:11636:5: ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) )
// InternalContextMappingDSL.g:11637:6: {...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 13) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 13)");
}
// InternalContextMappingDSL.g:11637:113: ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) )
// InternalContextMappingDSL.g:11638:7: ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 13);
// InternalContextMappingDSL.g:11641:10: ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) )
// InternalContextMappingDSL.g:11641:11: {...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleCommandEvent", "true");
}
// InternalContextMappingDSL.g:11641:20: (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) )
// InternalContextMappingDSL.g:11641:21: otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) )
{
otherlv_49=(Token)match(input,135,FOLLOW_105);
newLeafNode(otherlv_49, grammarAccess.getCommandEventAccess().getBelongsToKeyword_7_2_13_0());
// InternalContextMappingDSL.g:11645:10: ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) )
// InternalContextMappingDSL.g:11646:11: (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) )
{
// InternalContextMappingDSL.g:11646:11: (otherlv_50= '@' )?
int alt282=2;
int LA282_0 = input.LA(1);
if ( (LA282_0==94) ) {
alt282=1;
}
switch (alt282) {
case 1 :
// InternalContextMappingDSL.g:11647:12: otherlv_50= '@'
{
otherlv_50=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_50, grammarAccess.getCommandEventAccess().getCommercialAtKeyword_7_2_13_1_0());
}
break;
}
// InternalContextMappingDSL.g:11652:11: ( (otherlv_51= RULE_ID ) )
// InternalContextMappingDSL.g:11653:12: (otherlv_51= RULE_ID )
{
// InternalContextMappingDSL.g:11653:12: (otherlv_51= RULE_ID )
// InternalContextMappingDSL.g:11654:13: otherlv_51= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getCommandEventRule());
}
otherlv_51=(Token)match(input,RULE_ID,FOLLOW_151);
newLeafNode(otherlv_51, grammarAccess.getCommandEventAccess().getBelongsToDomainObjectCrossReference_7_2_13_1_1_0());
}
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2());
}
}
}
break;
default :
break loop283;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2());
}
// InternalContextMappingDSL.g:11679:4: ( ( (lv_attributes_52_0= ruleAttribute ) ) | ( (lv_references_53_0= ruleReference ) ) | ( (lv_operations_54_0= ruleDomainObjectOperation ) ) )*
loop284:
do {
int alt284=4;
switch ( input.LA(1) ) {
case RULE_STRING:
{
switch ( input.LA(2) ) {
case RULE_REF:
{
alt284=2;
}
break;
case RULE_ID:
case 124:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
case 247:
case 248:
case 249:
{
alt284=1;
}
break;
case 142:
case 143:
{
alt284=3;
}
break;
}
}
break;
case RULE_ID:
case 124:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
case 247:
case 248:
case 249:
{
alt284=1;
}
break;
case RULE_REF:
{
alt284=2;
}
break;
case 142:
case 143:
{
alt284=3;
}
break;
}
switch (alt284) {
case 1 :
// InternalContextMappingDSL.g:11680:5: ( (lv_attributes_52_0= ruleAttribute ) )
{
// InternalContextMappingDSL.g:11680:5: ( (lv_attributes_52_0= ruleAttribute ) )
// InternalContextMappingDSL.g:11681:6: (lv_attributes_52_0= ruleAttribute )
{
// InternalContextMappingDSL.g:11681:6: (lv_attributes_52_0= ruleAttribute )
// InternalContextMappingDSL.g:11682:7: lv_attributes_52_0= ruleAttribute
{
newCompositeNode(grammarAccess.getCommandEventAccess().getAttributesAttributeParserRuleCall_7_3_0_0());
pushFollow(FOLLOW_141);
lv_attributes_52_0=ruleAttribute();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCommandEventRule());
}
add(
current,
"attributes",
lv_attributes_52_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Attribute");
afterParserOrEnumRuleCall();
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:11700:5: ( (lv_references_53_0= ruleReference ) )
{
// InternalContextMappingDSL.g:11700:5: ( (lv_references_53_0= ruleReference ) )
// InternalContextMappingDSL.g:11701:6: (lv_references_53_0= ruleReference )
{
// InternalContextMappingDSL.g:11701:6: (lv_references_53_0= ruleReference )
// InternalContextMappingDSL.g:11702:7: lv_references_53_0= ruleReference
{
newCompositeNode(grammarAccess.getCommandEventAccess().getReferencesReferenceParserRuleCall_7_3_1_0());
pushFollow(FOLLOW_141);
lv_references_53_0=ruleReference();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCommandEventRule());
}
add(
current,
"references",
lv_references_53_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Reference");
afterParserOrEnumRuleCall();
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:11720:5: ( (lv_operations_54_0= ruleDomainObjectOperation ) )
{
// InternalContextMappingDSL.g:11720:5: ( (lv_operations_54_0= ruleDomainObjectOperation ) )
// InternalContextMappingDSL.g:11721:6: (lv_operations_54_0= ruleDomainObjectOperation )
{
// InternalContextMappingDSL.g:11721:6: (lv_operations_54_0= ruleDomainObjectOperation )
// InternalContextMappingDSL.g:11722:7: lv_operations_54_0= ruleDomainObjectOperation
{
newCompositeNode(grammarAccess.getCommandEventAccess().getOperationsDomainObjectOperationParserRuleCall_7_3_2_0());
pushFollow(FOLLOW_141);
lv_operations_54_0=ruleDomainObjectOperation();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCommandEventRule());
}
add(
current,
"operations",
lv_operations_54_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.DomainObjectOperation");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop284;
}
} while (true);
// InternalContextMappingDSL.g:11740:4: ( (lv_repository_55_0= ruleRepository ) )?
int alt285=2;
int LA285_0 = input.LA(1);
if ( (LA285_0==RULE_STRING||LA285_0==181) ) {
alt285=1;
}
switch (alt285) {
case 1 :
// InternalContextMappingDSL.g:11741:5: (lv_repository_55_0= ruleRepository )
{
// InternalContextMappingDSL.g:11741:5: (lv_repository_55_0= ruleRepository )
// InternalContextMappingDSL.g:11742:6: lv_repository_55_0= ruleRepository
{
newCompositeNode(grammarAccess.getCommandEventAccess().getRepositoryRepositoryParserRuleCall_7_4_0());
pushFollow(FOLLOW_36);
lv_repository_55_0=ruleRepository();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getCommandEventRule());
}
set(
current,
"repository",
lv_repository_55_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Repository");
afterParserOrEnumRuleCall();
}
}
break;
}
otherlv_56=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(otherlv_56, grammarAccess.getCommandEventAccess().getRightCurlyBracketKeyword_7_5());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleCommandEvent"
// $ANTLR start "entryRuleTrait"
// InternalContextMappingDSL.g:11768:1: entryRuleTrait returns [EObject current=null] : iv_ruleTrait= ruleTrait EOF ;
public final EObject entryRuleTrait() throws RecognitionException {
EObject current = null;
EObject iv_ruleTrait = null;
try {
// InternalContextMappingDSL.g:11768:46: (iv_ruleTrait= ruleTrait EOF )
// InternalContextMappingDSL.g:11769:2: iv_ruleTrait= ruleTrait EOF
{
newCompositeNode(grammarAccess.getTraitRule());
pushFollow(FOLLOW_1);
iv_ruleTrait=ruleTrait();
state._fsp--;
current =iv_ruleTrait;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleTrait"
// $ANTLR start "ruleTrait"
// InternalContextMappingDSL.g:11775:1: ruleTrait returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Trait' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= '{' (otherlv_4= 'package' otherlv_5= '=' ( (lv_package_6_0= ruleJavaIdentifier ) ) )? (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )? ( ( (lv_attributes_10_0= ruleAttribute ) ) | ( (lv_references_11_0= ruleReference ) ) | ( (lv_operations_12_0= ruleDomainObjectOperation ) ) )* otherlv_13= '}' )? ) ;
public final EObject ruleTrait() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token otherlv_1=null;
Token lv_name_2_0=null;
Token otherlv_3=null;
Token otherlv_4=null;
Token otherlv_5=null;
Token otherlv_7=null;
Token otherlv_8=null;
Token lv_hint_9_0=null;
Token otherlv_13=null;
AntlrDatatypeRuleToken lv_package_6_0 = null;
EObject lv_attributes_10_0 = null;
EObject lv_references_11_0 = null;
EObject lv_operations_12_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:11781:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Trait' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= '{' (otherlv_4= 'package' otherlv_5= '=' ( (lv_package_6_0= ruleJavaIdentifier ) ) )? (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )? ( ( (lv_attributes_10_0= ruleAttribute ) ) | ( (lv_references_11_0= ruleReference ) ) | ( (lv_operations_12_0= ruleDomainObjectOperation ) ) )* otherlv_13= '}' )? ) )
// InternalContextMappingDSL.g:11782:2: ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Trait' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= '{' (otherlv_4= 'package' otherlv_5= '=' ( (lv_package_6_0= ruleJavaIdentifier ) ) )? (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )? ( ( (lv_attributes_10_0= ruleAttribute ) ) | ( (lv_references_11_0= ruleReference ) ) | ( (lv_operations_12_0= ruleDomainObjectOperation ) ) )* otherlv_13= '}' )? )
{
// InternalContextMappingDSL.g:11782:2: ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Trait' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= '{' (otherlv_4= 'package' otherlv_5= '=' ( (lv_package_6_0= ruleJavaIdentifier ) ) )? (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )? ( ( (lv_attributes_10_0= ruleAttribute ) ) | ( (lv_references_11_0= ruleReference ) ) | ( (lv_operations_12_0= ruleDomainObjectOperation ) ) )* otherlv_13= '}' )? )
// InternalContextMappingDSL.g:11783:3: ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Trait' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= '{' (otherlv_4= 'package' otherlv_5= '=' ( (lv_package_6_0= ruleJavaIdentifier ) ) )? (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )? ( ( (lv_attributes_10_0= ruleAttribute ) ) | ( (lv_references_11_0= ruleReference ) ) | ( (lv_operations_12_0= ruleDomainObjectOperation ) ) )* otherlv_13= '}' )?
{
// InternalContextMappingDSL.g:11783:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt287=2;
int LA287_0 = input.LA(1);
if ( (LA287_0==RULE_STRING) ) {
alt287=1;
}
switch (alt287) {
case 1 :
// InternalContextMappingDSL.g:11784:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:11784:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:11785:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_155);
newLeafNode(lv_doc_0_0, grammarAccess.getTraitAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getTraitRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
otherlv_1=(Token)match(input,141,FOLLOW_10);
newLeafNode(otherlv_1, grammarAccess.getTraitAccess().getTraitKeyword_1());
// InternalContextMappingDSL.g:11805:3: ( (lv_name_2_0= RULE_ID ) )
// InternalContextMappingDSL.g:11806:4: (lv_name_2_0= RULE_ID )
{
// InternalContextMappingDSL.g:11806:4: (lv_name_2_0= RULE_ID )
// InternalContextMappingDSL.g:11807:5: lv_name_2_0= RULE_ID
{
lv_name_2_0=(Token)match(input,RULE_ID,FOLLOW_23);
newLeafNode(lv_name_2_0, grammarAccess.getTraitAccess().getNameIDTerminalRuleCall_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getTraitRule());
}
setWithLastConsumed(
current,
"name",
lv_name_2_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:11823:3: (otherlv_3= '{' (otherlv_4= 'package' otherlv_5= '=' ( (lv_package_6_0= ruleJavaIdentifier ) ) )? (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )? ( ( (lv_attributes_10_0= ruleAttribute ) ) | ( (lv_references_11_0= ruleReference ) ) | ( (lv_operations_12_0= ruleDomainObjectOperation ) ) )* otherlv_13= '}' )?
int alt291=2;
int LA291_0 = input.LA(1);
if ( (LA291_0==RULE_OPEN) ) {
alt291=1;
}
switch (alt291) {
case 1 :
// InternalContextMappingDSL.g:11824:4: otherlv_3= '{' (otherlv_4= 'package' otherlv_5= '=' ( (lv_package_6_0= ruleJavaIdentifier ) ) )? (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )? ( ( (lv_attributes_10_0= ruleAttribute ) ) | ( (lv_references_11_0= ruleReference ) ) | ( (lv_operations_12_0= ruleDomainObjectOperation ) ) )* otherlv_13= '}'
{
otherlv_3=(Token)match(input,RULE_OPEN,FOLLOW_156);
newLeafNode(otherlv_3, grammarAccess.getTraitAccess().getLeftCurlyBracketKeyword_3_0());
// InternalContextMappingDSL.g:11828:4: (otherlv_4= 'package' otherlv_5= '=' ( (lv_package_6_0= ruleJavaIdentifier ) ) )?
int alt288=2;
int LA288_0 = input.LA(1);
if ( (LA288_0==124) ) {
int LA288_1 = input.LA(2);
if ( (LA288_1==21) ) {
alt288=1;
}
}
switch (alt288) {
case 1 :
// InternalContextMappingDSL.g:11829:5: otherlv_4= 'package' otherlv_5= '=' ( (lv_package_6_0= ruleJavaIdentifier ) )
{
otherlv_4=(Token)match(input,124,FOLLOW_89);
newLeafNode(otherlv_4, grammarAccess.getTraitAccess().getPackageKeyword_3_1_0());
otherlv_5=(Token)match(input,21,FOLLOW_10);
newLeafNode(otherlv_5, grammarAccess.getTraitAccess().getEqualsSignKeyword_3_1_1());
// InternalContextMappingDSL.g:11837:5: ( (lv_package_6_0= ruleJavaIdentifier ) )
// InternalContextMappingDSL.g:11838:6: (lv_package_6_0= ruleJavaIdentifier )
{
// InternalContextMappingDSL.g:11838:6: (lv_package_6_0= ruleJavaIdentifier )
// InternalContextMappingDSL.g:11839:7: lv_package_6_0= ruleJavaIdentifier
{
newCompositeNode(grammarAccess.getTraitAccess().getPackageJavaIdentifierParserRuleCall_3_1_2_0());
pushFollow(FOLLOW_156);
lv_package_6_0=ruleJavaIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getTraitRule());
}
set(
current,
"package",
lv_package_6_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.JavaIdentifier");
afterParserOrEnumRuleCall();
}
}
}
break;
}
// InternalContextMappingDSL.g:11857:4: (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )?
int alt289=2;
int LA289_0 = input.LA(1);
if ( (LA289_0==82) ) {
alt289=1;
}
switch (alt289) {
case 1 :
// InternalContextMappingDSL.g:11858:5: otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) )
{
otherlv_7=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_7, grammarAccess.getTraitAccess().getHintKeyword_3_2_0());
otherlv_8=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_8, grammarAccess.getTraitAccess().getEqualsSignKeyword_3_2_1());
// InternalContextMappingDSL.g:11866:5: ( (lv_hint_9_0= RULE_STRING ) )
// InternalContextMappingDSL.g:11867:6: (lv_hint_9_0= RULE_STRING )
{
// InternalContextMappingDSL.g:11867:6: (lv_hint_9_0= RULE_STRING )
// InternalContextMappingDSL.g:11868:7: lv_hint_9_0= RULE_STRING
{
lv_hint_9_0=(Token)match(input,RULE_STRING,FOLLOW_157);
newLeafNode(lv_hint_9_0, grammarAccess.getTraitAccess().getHintSTRINGTerminalRuleCall_3_2_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getTraitRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_9_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
// InternalContextMappingDSL.g:11885:4: ( ( (lv_attributes_10_0= ruleAttribute ) ) | ( (lv_references_11_0= ruleReference ) ) | ( (lv_operations_12_0= ruleDomainObjectOperation ) ) )*
loop290:
do {
int alt290=4;
switch ( input.LA(1) ) {
case RULE_STRING:
{
switch ( input.LA(2) ) {
case 142:
case 143:
{
alt290=3;
}
break;
case RULE_REF:
{
alt290=2;
}
break;
case RULE_ID:
case 124:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
case 247:
case 248:
case 249:
{
alt290=1;
}
break;
}
}
break;
case RULE_ID:
case 124:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
case 247:
case 248:
case 249:
{
alt290=1;
}
break;
case RULE_REF:
{
alt290=2;
}
break;
case 142:
case 143:
{
alt290=3;
}
break;
}
switch (alt290) {
case 1 :
// InternalContextMappingDSL.g:11886:5: ( (lv_attributes_10_0= ruleAttribute ) )
{
// InternalContextMappingDSL.g:11886:5: ( (lv_attributes_10_0= ruleAttribute ) )
// InternalContextMappingDSL.g:11887:6: (lv_attributes_10_0= ruleAttribute )
{
// InternalContextMappingDSL.g:11887:6: (lv_attributes_10_0= ruleAttribute )
// InternalContextMappingDSL.g:11888:7: lv_attributes_10_0= ruleAttribute
{
newCompositeNode(grammarAccess.getTraitAccess().getAttributesAttributeParserRuleCall_3_3_0_0());
pushFollow(FOLLOW_157);
lv_attributes_10_0=ruleAttribute();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getTraitRule());
}
add(
current,
"attributes",
lv_attributes_10_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Attribute");
afterParserOrEnumRuleCall();
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:11906:5: ( (lv_references_11_0= ruleReference ) )
{
// InternalContextMappingDSL.g:11906:5: ( (lv_references_11_0= ruleReference ) )
// InternalContextMappingDSL.g:11907:6: (lv_references_11_0= ruleReference )
{
// InternalContextMappingDSL.g:11907:6: (lv_references_11_0= ruleReference )
// InternalContextMappingDSL.g:11908:7: lv_references_11_0= ruleReference
{
newCompositeNode(grammarAccess.getTraitAccess().getReferencesReferenceParserRuleCall_3_3_1_0());
pushFollow(FOLLOW_157);
lv_references_11_0=ruleReference();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getTraitRule());
}
add(
current,
"references",
lv_references_11_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Reference");
afterParserOrEnumRuleCall();
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:11926:5: ( (lv_operations_12_0= ruleDomainObjectOperation ) )
{
// InternalContextMappingDSL.g:11926:5: ( (lv_operations_12_0= ruleDomainObjectOperation ) )
// InternalContextMappingDSL.g:11927:6: (lv_operations_12_0= ruleDomainObjectOperation )
{
// InternalContextMappingDSL.g:11927:6: (lv_operations_12_0= ruleDomainObjectOperation )
// InternalContextMappingDSL.g:11928:7: lv_operations_12_0= ruleDomainObjectOperation
{
newCompositeNode(grammarAccess.getTraitAccess().getOperationsDomainObjectOperationParserRuleCall_3_3_2_0());
pushFollow(FOLLOW_157);
lv_operations_12_0=ruleDomainObjectOperation();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getTraitRule());
}
add(
current,
"operations",
lv_operations_12_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.DomainObjectOperation");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop290;
}
} while (true);
otherlv_13=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(otherlv_13, grammarAccess.getTraitAccess().getRightCurlyBracketKeyword_3_4());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleTrait"
// $ANTLR start "entryRuleDomainObjectOperation"
// InternalContextMappingDSL.g:11955:1: entryRuleDomainObjectOperation returns [EObject current=null] : iv_ruleDomainObjectOperation= ruleDomainObjectOperation EOF ;
public final EObject entryRuleDomainObjectOperation() throws RecognitionException {
EObject current = null;
EObject iv_ruleDomainObjectOperation = null;
try {
// InternalContextMappingDSL.g:11955:62: (iv_ruleDomainObjectOperation= ruleDomainObjectOperation EOF )
// InternalContextMappingDSL.g:11956:2: iv_ruleDomainObjectOperation= ruleDomainObjectOperation EOF
{
newCompositeNode(grammarAccess.getDomainObjectOperationRule());
pushFollow(FOLLOW_1);
iv_ruleDomainObjectOperation=ruleDomainObjectOperation();
state._fsp--;
current =iv_ruleDomainObjectOperation;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleDomainObjectOperation"
// $ANTLR start "ruleDomainObjectOperation"
// InternalContextMappingDSL.g:11962:1: ruleDomainObjectOperation returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? (otherlv_1= 'def' | otherlv_2= '*' ) ( (lv_abstract_3_0= 'abstract' ) )? ( (lv_visibility_4_0= ruleVisibility ) )? ( ( (lv_returnType_5_0= ruleComplexType ) ) | otherlv_6= 'void' )? ( (lv_name_7_0= RULE_ID ) ) (otherlv_8= '(' ( (lv_parameters_9_0= ruleParameter ) )? (otherlv_10= ',' ( (lv_parameters_11_0= ruleParameter ) ) )* otherlv_12= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) otherlv_19= ';' ) ;
public final EObject ruleDomainObjectOperation() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token otherlv_1=null;
Token otherlv_2=null;
Token lv_abstract_3_0=null;
Token otherlv_6=null;
Token lv_name_7_0=null;
Token otherlv_8=null;
Token otherlv_10=null;
Token otherlv_12=null;
Token otherlv_14=null;
Token otherlv_16=null;
Token otherlv_17=null;
Token lv_hint_18_0=null;
Token otherlv_19=null;
Enumerator lv_visibility_4_0 = null;
EObject lv_returnType_5_0 = null;
EObject lv_parameters_9_0 = null;
EObject lv_parameters_11_0 = null;
AntlrDatatypeRuleToken lv_throws_15_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:11968:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? (otherlv_1= 'def' | otherlv_2= '*' ) ( (lv_abstract_3_0= 'abstract' ) )? ( (lv_visibility_4_0= ruleVisibility ) )? ( ( (lv_returnType_5_0= ruleComplexType ) ) | otherlv_6= 'void' )? ( (lv_name_7_0= RULE_ID ) ) (otherlv_8= '(' ( (lv_parameters_9_0= ruleParameter ) )? (otherlv_10= ',' ( (lv_parameters_11_0= ruleParameter ) ) )* otherlv_12= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) otherlv_19= ';' ) )
// InternalContextMappingDSL.g:11969:2: ( ( (lv_doc_0_0= RULE_STRING ) )? (otherlv_1= 'def' | otherlv_2= '*' ) ( (lv_abstract_3_0= 'abstract' ) )? ( (lv_visibility_4_0= ruleVisibility ) )? ( ( (lv_returnType_5_0= ruleComplexType ) ) | otherlv_6= 'void' )? ( (lv_name_7_0= RULE_ID ) ) (otherlv_8= '(' ( (lv_parameters_9_0= ruleParameter ) )? (otherlv_10= ',' ( (lv_parameters_11_0= ruleParameter ) ) )* otherlv_12= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) otherlv_19= ';' )
{
// InternalContextMappingDSL.g:11969:2: ( ( (lv_doc_0_0= RULE_STRING ) )? (otherlv_1= 'def' | otherlv_2= '*' ) ( (lv_abstract_3_0= 'abstract' ) )? ( (lv_visibility_4_0= ruleVisibility ) )? ( ( (lv_returnType_5_0= ruleComplexType ) ) | otherlv_6= 'void' )? ( (lv_name_7_0= RULE_ID ) ) (otherlv_8= '(' ( (lv_parameters_9_0= ruleParameter ) )? (otherlv_10= ',' ( (lv_parameters_11_0= ruleParameter ) ) )* otherlv_12= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) otherlv_19= ';' )
// InternalContextMappingDSL.g:11970:3: ( (lv_doc_0_0= RULE_STRING ) )? (otherlv_1= 'def' | otherlv_2= '*' ) ( (lv_abstract_3_0= 'abstract' ) )? ( (lv_visibility_4_0= ruleVisibility ) )? ( ( (lv_returnType_5_0= ruleComplexType ) ) | otherlv_6= 'void' )? ( (lv_name_7_0= RULE_ID ) ) (otherlv_8= '(' ( (lv_parameters_9_0= ruleParameter ) )? (otherlv_10= ',' ( (lv_parameters_11_0= ruleParameter ) ) )* otherlv_12= ')' )? ( ( ( ( ({...}? => ( ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) otherlv_19= ';'
{
// InternalContextMappingDSL.g:11970:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt292=2;
int LA292_0 = input.LA(1);
if ( (LA292_0==RULE_STRING) ) {
alt292=1;
}
switch (alt292) {
case 1 :
// InternalContextMappingDSL.g:11971:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:11971:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:11972:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_158);
newLeafNode(lv_doc_0_0, grammarAccess.getDomainObjectOperationAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainObjectOperationRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
// InternalContextMappingDSL.g:11988:3: (otherlv_1= 'def' | otherlv_2= '*' )
int alt293=2;
int LA293_0 = input.LA(1);
if ( (LA293_0==142) ) {
alt293=1;
}
else if ( (LA293_0==143) ) {
alt293=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 293, 0, input);
throw nvae;
}
switch (alt293) {
case 1 :
// InternalContextMappingDSL.g:11989:4: otherlv_1= 'def'
{
otherlv_1=(Token)match(input,142,FOLLOW_159);
newLeafNode(otherlv_1, grammarAccess.getDomainObjectOperationAccess().getDefKeyword_1_0());
}
break;
case 2 :
// InternalContextMappingDSL.g:11994:4: otherlv_2= '*'
{
otherlv_2=(Token)match(input,143,FOLLOW_159);
newLeafNode(otherlv_2, grammarAccess.getDomainObjectOperationAccess().getAsteriskKeyword_1_1());
}
break;
}
// InternalContextMappingDSL.g:11999:3: ( (lv_abstract_3_0= 'abstract' ) )?
int alt294=2;
int LA294_0 = input.LA(1);
if ( (LA294_0==120) ) {
alt294=1;
}
switch (alt294) {
case 1 :
// InternalContextMappingDSL.g:12000:4: (lv_abstract_3_0= 'abstract' )
{
// InternalContextMappingDSL.g:12000:4: (lv_abstract_3_0= 'abstract' )
// InternalContextMappingDSL.g:12001:5: lv_abstract_3_0= 'abstract'
{
lv_abstract_3_0=(Token)match(input,120,FOLLOW_110);
newLeafNode(lv_abstract_3_0, grammarAccess.getDomainObjectOperationAccess().getAbstractAbstractKeyword_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainObjectOperationRule());
}
setWithLastConsumed(current, "abstract", true, "abstract");
}
}
break;
}
// InternalContextMappingDSL.g:12013:3: ( (lv_visibility_4_0= ruleVisibility ) )?
int alt295=2;
int LA295_0 = input.LA(1);
if ( (LA295_0==124||(LA295_0>=247 && LA295_0<=249)) ) {
alt295=1;
}
switch (alt295) {
case 1 :
// InternalContextMappingDSL.g:12014:4: (lv_visibility_4_0= ruleVisibility )
{
// InternalContextMappingDSL.g:12014:4: (lv_visibility_4_0= ruleVisibility )
// InternalContextMappingDSL.g:12015:5: lv_visibility_4_0= ruleVisibility
{
newCompositeNode(grammarAccess.getDomainObjectOperationAccess().getVisibilityVisibilityEnumRuleCall_3_0());
pushFollow(FOLLOW_111);
lv_visibility_4_0=ruleVisibility();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDomainObjectOperationRule());
}
set(
current,
"visibility",
lv_visibility_4_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Visibility");
afterParserOrEnumRuleCall();
}
}
break;
}
// InternalContextMappingDSL.g:12032:3: ( ( (lv_returnType_5_0= ruleComplexType ) ) | otherlv_6= 'void' )?
int alt296=3;
switch ( input.LA(1) ) {
case RULE_MAP_COLLECTION_TYPE:
case 94:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
{
alt296=1;
}
break;
case RULE_ID:
{
int LA296_2 = input.LA(2);
if ( (LA296_2==RULE_ID||LA296_2==106||LA296_2==118) ) {
alt296=1;
}
}
break;
case 101:
{
alt296=2;
}
break;
}
switch (alt296) {
case 1 :
// InternalContextMappingDSL.g:12033:4: ( (lv_returnType_5_0= ruleComplexType ) )
{
// InternalContextMappingDSL.g:12033:4: ( (lv_returnType_5_0= ruleComplexType ) )
// InternalContextMappingDSL.g:12034:5: (lv_returnType_5_0= ruleComplexType )
{
// InternalContextMappingDSL.g:12034:5: (lv_returnType_5_0= ruleComplexType )
// InternalContextMappingDSL.g:12035:6: lv_returnType_5_0= ruleComplexType
{
newCompositeNode(grammarAccess.getDomainObjectOperationAccess().getReturnTypeComplexTypeParserRuleCall_4_0_0());
pushFollow(FOLLOW_10);
lv_returnType_5_0=ruleComplexType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDomainObjectOperationRule());
}
set(
current,
"returnType",
lv_returnType_5_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.ComplexType");
afterParserOrEnumRuleCall();
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:12053:4: otherlv_6= 'void'
{
otherlv_6=(Token)match(input,101,FOLLOW_10);
newLeafNode(otherlv_6, grammarAccess.getDomainObjectOperationAccess().getVoidKeyword_4_1());
}
break;
}
// InternalContextMappingDSL.g:12058:3: ( (lv_name_7_0= RULE_ID ) )
// InternalContextMappingDSL.g:12059:4: (lv_name_7_0= RULE_ID )
{
// InternalContextMappingDSL.g:12059:4: (lv_name_7_0= RULE_ID )
// InternalContextMappingDSL.g:12060:5: lv_name_7_0= RULE_ID
{
lv_name_7_0=(Token)match(input,RULE_ID,FOLLOW_160);
newLeafNode(lv_name_7_0, grammarAccess.getDomainObjectOperationAccess().getNameIDTerminalRuleCall_5_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainObjectOperationRule());
}
setWithLastConsumed(
current,
"name",
lv_name_7_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:12076:3: (otherlv_8= '(' ( (lv_parameters_9_0= ruleParameter ) )? (otherlv_10= ',' ( (lv_parameters_11_0= ruleParameter ) ) )* otherlv_12= ')' )?
int alt299=2;
int LA299_0 = input.LA(1);
if ( (LA299_0==102) ) {
alt299=1;
}
switch (alt299) {
case 1 :
// InternalContextMappingDSL.g:12077:4: otherlv_8= '(' ( (lv_parameters_9_0= ruleParameter ) )? (otherlv_10= ',' ( (lv_parameters_11_0= ruleParameter ) ) )* otherlv_12= ')'
{
otherlv_8=(Token)match(input,102,FOLLOW_113);
newLeafNode(otherlv_8, grammarAccess.getDomainObjectOperationAccess().getLeftParenthesisKeyword_6_0());
// InternalContextMappingDSL.g:12081:4: ( (lv_parameters_9_0= ruleParameter ) )?
int alt297=2;
int LA297_0 = input.LA(1);
if ( ((LA297_0>=RULE_STRING && LA297_0<=RULE_ID)||LA297_0==RULE_MAP_COLLECTION_TYPE||LA297_0==94||(LA297_0>=185 && LA297_0<=206)||LA297_0==233||(LA297_0>=243 && LA297_0<=246)) ) {
alt297=1;
}
switch (alt297) {
case 1 :
// InternalContextMappingDSL.g:12082:5: (lv_parameters_9_0= ruleParameter )
{
// InternalContextMappingDSL.g:12082:5: (lv_parameters_9_0= ruleParameter )
// InternalContextMappingDSL.g:12083:6: lv_parameters_9_0= ruleParameter
{
newCompositeNode(grammarAccess.getDomainObjectOperationAccess().getParametersParameterParserRuleCall_6_1_0());
pushFollow(FOLLOW_114);
lv_parameters_9_0=ruleParameter();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDomainObjectOperationRule());
}
add(
current,
"parameters",
lv_parameters_9_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Parameter");
afterParserOrEnumRuleCall();
}
}
break;
}
// InternalContextMappingDSL.g:12100:4: (otherlv_10= ',' ( (lv_parameters_11_0= ruleParameter ) ) )*
loop298:
do {
int alt298=2;
int LA298_0 = input.LA(1);
if ( (LA298_0==24) ) {
alt298=1;
}
switch (alt298) {
case 1 :
// InternalContextMappingDSL.g:12101:5: otherlv_10= ',' ( (lv_parameters_11_0= ruleParameter ) )
{
otherlv_10=(Token)match(input,24,FOLLOW_115);
newLeafNode(otherlv_10, grammarAccess.getDomainObjectOperationAccess().getCommaKeyword_6_2_0());
// InternalContextMappingDSL.g:12105:5: ( (lv_parameters_11_0= ruleParameter ) )
// InternalContextMappingDSL.g:12106:6: (lv_parameters_11_0= ruleParameter )
{
// InternalContextMappingDSL.g:12106:6: (lv_parameters_11_0= ruleParameter )
// InternalContextMappingDSL.g:12107:7: lv_parameters_11_0= ruleParameter
{
newCompositeNode(grammarAccess.getDomainObjectOperationAccess().getParametersParameterParserRuleCall_6_2_1_0());
pushFollow(FOLLOW_114);
lv_parameters_11_0=ruleParameter();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDomainObjectOperationRule());
}
add(
current,
"parameters",
lv_parameters_11_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Parameter");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop298;
}
} while (true);
otherlv_12=(Token)match(input,103,FOLLOW_161);
newLeafNode(otherlv_12, grammarAccess.getDomainObjectOperationAccess().getRightParenthesisKeyword_6_3());
}
break;
}
// InternalContextMappingDSL.g:12130:3: ( ( ( ( ({...}? => ( ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:12131:4: ( ( ( ({...}? => ( ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:12131:4: ( ( ( ({...}? => ( ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:12132:5: ( ( ({...}? => ( ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getDomainObjectOperationAccess().getUnorderedGroup_7());
// InternalContextMappingDSL.g:12135:5: ( ( ({...}? => ( ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:12136:6: ( ({...}? => ( ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:12136:6: ( ({...}? => ( ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )*
loop300:
do {
int alt300=3;
int LA300_0 = input.LA(1);
if ( LA300_0 == 104 && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainObjectOperationAccess().getUnorderedGroup_7(), 0) ) {
alt300=1;
}
else if ( LA300_0 == 82 && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainObjectOperationAccess().getUnorderedGroup_7(), 1) ) {
alt300=2;
}
switch (alt300) {
case 1 :
// InternalContextMappingDSL.g:12137:4: ({...}? => ( ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) ) ) )
{
// InternalContextMappingDSL.g:12137:4: ({...}? => ( ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) ) ) )
// InternalContextMappingDSL.g:12138:5: {...}? => ( ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainObjectOperationAccess().getUnorderedGroup_7(), 0) ) {
throw new FailedPredicateException(input, "ruleDomainObjectOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainObjectOperationAccess().getUnorderedGroup_7(), 0)");
}
// InternalContextMappingDSL.g:12138:118: ( ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) ) )
// InternalContextMappingDSL.g:12139:6: ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDomainObjectOperationAccess().getUnorderedGroup_7(), 0);
// InternalContextMappingDSL.g:12142:9: ({...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) ) )
// InternalContextMappingDSL.g:12142:10: {...}? => (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomainObjectOperation", "true");
}
// InternalContextMappingDSL.g:12142:19: (otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) ) )
// InternalContextMappingDSL.g:12142:20: otherlv_14= 'throws' ( (lv_throws_15_0= ruleThrowsIdentifier ) )
{
otherlv_14=(Token)match(input,104,FOLLOW_10);
newLeafNode(otherlv_14, grammarAccess.getDomainObjectOperationAccess().getThrowsKeyword_7_0_0());
// InternalContextMappingDSL.g:12146:9: ( (lv_throws_15_0= ruleThrowsIdentifier ) )
// InternalContextMappingDSL.g:12147:10: (lv_throws_15_0= ruleThrowsIdentifier )
{
// InternalContextMappingDSL.g:12147:10: (lv_throws_15_0= ruleThrowsIdentifier )
// InternalContextMappingDSL.g:12148:11: lv_throws_15_0= ruleThrowsIdentifier
{
newCompositeNode(grammarAccess.getDomainObjectOperationAccess().getThrowsThrowsIdentifierParserRuleCall_7_0_1_0());
pushFollow(FOLLOW_161);
lv_throws_15_0=ruleThrowsIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDomainObjectOperationRule());
}
set(
current,
"throws",
lv_throws_15_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.ThrowsIdentifier");
afterParserOrEnumRuleCall();
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainObjectOperationAccess().getUnorderedGroup_7());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:12171:4: ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:12171:4: ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:12172:5: {...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDomainObjectOperationAccess().getUnorderedGroup_7(), 1) ) {
throw new FailedPredicateException(input, "ruleDomainObjectOperation", "getUnorderedGroupHelper().canSelect(grammarAccess.getDomainObjectOperationAccess().getUnorderedGroup_7(), 1)");
}
// InternalContextMappingDSL.g:12172:118: ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:12173:6: ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDomainObjectOperationAccess().getUnorderedGroup_7(), 1);
// InternalContextMappingDSL.g:12176:9: ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:12176:10: {...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDomainObjectOperation", "true");
}
// InternalContextMappingDSL.g:12176:19: (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:12176:20: otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) )
{
otherlv_16=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_16, grammarAccess.getDomainObjectOperationAccess().getHintKeyword_7_1_0());
otherlv_17=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_17, grammarAccess.getDomainObjectOperationAccess().getEqualsSignKeyword_7_1_1());
// InternalContextMappingDSL.g:12184:9: ( (lv_hint_18_0= RULE_STRING ) )
// InternalContextMappingDSL.g:12185:10: (lv_hint_18_0= RULE_STRING )
{
// InternalContextMappingDSL.g:12185:10: (lv_hint_18_0= RULE_STRING )
// InternalContextMappingDSL.g:12186:11: lv_hint_18_0= RULE_STRING
{
lv_hint_18_0=(Token)match(input,RULE_STRING,FOLLOW_161);
newLeafNode(lv_hint_18_0, grammarAccess.getDomainObjectOperationAccess().getHintSTRINGTerminalRuleCall_7_1_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDomainObjectOperationRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_18_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDomainObjectOperationAccess().getUnorderedGroup_7());
}
}
}
break;
default :
break loop300;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getDomainObjectOperationAccess().getUnorderedGroup_7());
}
otherlv_19=(Token)match(input,105,FOLLOW_2);
newLeafNode(otherlv_19, grammarAccess.getDomainObjectOperationAccess().getSemicolonKeyword_8());
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleDomainObjectOperation"
// $ANTLR start "entryRuleDataTransferObject"
// InternalContextMappingDSL.g:12223:1: entryRuleDataTransferObject returns [EObject current=null] : iv_ruleDataTransferObject= ruleDataTransferObject EOF ;
public final EObject entryRuleDataTransferObject() throws RecognitionException {
EObject current = null;
EObject iv_ruleDataTransferObject = null;
try {
// InternalContextMappingDSL.g:12223:59: (iv_ruleDataTransferObject= ruleDataTransferObject EOF )
// InternalContextMappingDSL.g:12224:2: iv_ruleDataTransferObject= ruleDataTransferObject EOF
{
newCompositeNode(grammarAccess.getDataTransferObjectRule());
pushFollow(FOLLOW_1);
iv_ruleDataTransferObject=ruleDataTransferObject();
state._fsp--;
current =iv_ruleDataTransferObject;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleDataTransferObject"
// $ANTLR start "ruleDataTransferObject"
// InternalContextMappingDSL.g:12230:1: ruleDataTransferObject returns [EObject current=null] : ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'DataTransferObject' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= '{' (otherlv_10= 'package' otherlv_11= '=' ( (lv_package_12_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_22_0= ruleDtoAttribute ) ) | ( (lv_references_23_0= ruleDtoReference ) ) )* otherlv_24= '}' )? ) ;
public final EObject ruleDataTransferObject() throws RecognitionException {
EObject current = null;
Token lv_comment_0_0=null;
Token lv_doc_1_0=null;
Token lv_abstract_2_0=null;
Token otherlv_3=null;
Token lv_name_4_0=null;
Token otherlv_5=null;
Token otherlv_6=null;
Token otherlv_7=null;
Token otherlv_9=null;
Token otherlv_10=null;
Token otherlv_11=null;
Token lv_gapClass_14_0=null;
Token lv_noGapClass_15_0=null;
Token otherlv_16=null;
Token otherlv_17=null;
Token lv_hint_18_0=null;
Token otherlv_19=null;
Token otherlv_20=null;
Token lv_validate_21_0=null;
Token otherlv_24=null;
AntlrDatatypeRuleToken lv_extendsName_8_0 = null;
AntlrDatatypeRuleToken lv_package_12_0 = null;
EObject lv_attributes_22_0 = null;
EObject lv_references_23_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:12236:2: ( ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'DataTransferObject' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= '{' (otherlv_10= 'package' otherlv_11= '=' ( (lv_package_12_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_22_0= ruleDtoAttribute ) ) | ( (lv_references_23_0= ruleDtoReference ) ) )* otherlv_24= '}' )? ) )
// InternalContextMappingDSL.g:12237:2: ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'DataTransferObject' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= '{' (otherlv_10= 'package' otherlv_11= '=' ( (lv_package_12_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_22_0= ruleDtoAttribute ) ) | ( (lv_references_23_0= ruleDtoReference ) ) )* otherlv_24= '}' )? )
{
// InternalContextMappingDSL.g:12237:2: ( ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'DataTransferObject' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= '{' (otherlv_10= 'package' otherlv_11= '=' ( (lv_package_12_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_22_0= ruleDtoAttribute ) ) | ( (lv_references_23_0= ruleDtoReference ) ) )* otherlv_24= '}' )? )
// InternalContextMappingDSL.g:12238:3: ( (lv_comment_0_0= RULE_ML_COMMENT ) )? ( (lv_doc_1_0= RULE_STRING ) )? ( (lv_abstract_2_0= 'abstract' ) )? otherlv_3= 'DataTransferObject' ( (lv_name_4_0= RULE_ID ) ) (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )? (otherlv_9= '{' (otherlv_10= 'package' otherlv_11= '=' ( (lv_package_12_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_22_0= ruleDtoAttribute ) ) | ( (lv_references_23_0= ruleDtoReference ) ) )* otherlv_24= '}' )?
{
// InternalContextMappingDSL.g:12238:3: ( (lv_comment_0_0= RULE_ML_COMMENT ) )?
int alt301=2;
int LA301_0 = input.LA(1);
if ( (LA301_0==RULE_ML_COMMENT) ) {
alt301=1;
}
switch (alt301) {
case 1 :
// InternalContextMappingDSL.g:12239:4: (lv_comment_0_0= RULE_ML_COMMENT )
{
// InternalContextMappingDSL.g:12239:4: (lv_comment_0_0= RULE_ML_COMMENT )
// InternalContextMappingDSL.g:12240:5: lv_comment_0_0= RULE_ML_COMMENT
{
lv_comment_0_0=(Token)match(input,RULE_ML_COMMENT,FOLLOW_162);
newLeafNode(lv_comment_0_0, grammarAccess.getDataTransferObjectAccess().getCommentML_COMMENTTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDataTransferObjectRule());
}
setWithLastConsumed(
current,
"comment",
lv_comment_0_0,
"org.eclipse.xtext.common.Terminals.ML_COMMENT");
}
}
break;
}
// InternalContextMappingDSL.g:12256:3: ( (lv_doc_1_0= RULE_STRING ) )?
int alt302=2;
int LA302_0 = input.LA(1);
if ( (LA302_0==RULE_STRING) ) {
alt302=1;
}
switch (alt302) {
case 1 :
// InternalContextMappingDSL.g:12257:4: (lv_doc_1_0= RULE_STRING )
{
// InternalContextMappingDSL.g:12257:4: (lv_doc_1_0= RULE_STRING )
// InternalContextMappingDSL.g:12258:5: lv_doc_1_0= RULE_STRING
{
lv_doc_1_0=(Token)match(input,RULE_STRING,FOLLOW_163);
newLeafNode(lv_doc_1_0, grammarAccess.getDataTransferObjectAccess().getDocSTRINGTerminalRuleCall_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDataTransferObjectRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_1_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
// InternalContextMappingDSL.g:12274:3: ( (lv_abstract_2_0= 'abstract' ) )?
int alt303=2;
int LA303_0 = input.LA(1);
if ( (LA303_0==120) ) {
alt303=1;
}
switch (alt303) {
case 1 :
// InternalContextMappingDSL.g:12275:4: (lv_abstract_2_0= 'abstract' )
{
// InternalContextMappingDSL.g:12275:4: (lv_abstract_2_0= 'abstract' )
// InternalContextMappingDSL.g:12276:5: lv_abstract_2_0= 'abstract'
{
lv_abstract_2_0=(Token)match(input,120,FOLLOW_164);
newLeafNode(lv_abstract_2_0, grammarAccess.getDataTransferObjectAccess().getAbstractAbstractKeyword_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDataTransferObjectRule());
}
setWithLastConsumed(current, "abstract", true, "abstract");
}
}
break;
}
otherlv_3=(Token)match(input,144,FOLLOW_10);
newLeafNode(otherlv_3, grammarAccess.getDataTransferObjectAccess().getDataTransferObjectKeyword_3());
// InternalContextMappingDSL.g:12292:3: ( (lv_name_4_0= RULE_ID ) )
// InternalContextMappingDSL.g:12293:4: (lv_name_4_0= RULE_ID )
{
// InternalContextMappingDSL.g:12293:4: (lv_name_4_0= RULE_ID )
// InternalContextMappingDSL.g:12294:5: lv_name_4_0= RULE_ID
{
lv_name_4_0=(Token)match(input,RULE_ID,FOLLOW_165);
newLeafNode(lv_name_4_0, grammarAccess.getDataTransferObjectAccess().getNameIDTerminalRuleCall_4_0());
if (current==null) {
current = createModelElement(grammarAccess.getDataTransferObjectRule());
}
setWithLastConsumed(
current,
"name",
lv_name_4_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:12310:3: (otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) ) )?
int alt305=2;
int LA305_0 = input.LA(1);
if ( (LA305_0==122) ) {
alt305=1;
}
switch (alt305) {
case 1 :
// InternalContextMappingDSL.g:12311:4: otherlv_5= 'extends' ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) )
{
otherlv_5=(Token)match(input,122,FOLLOW_105);
newLeafNode(otherlv_5, grammarAccess.getDataTransferObjectAccess().getExtendsKeyword_5_0());
// InternalContextMappingDSL.g:12315:4: ( (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( (lv_extendsName_8_0= ruleJavaIdentifier ) ) )
int alt304=2;
int LA304_0 = input.LA(1);
if ( (LA304_0==94) ) {
alt304=1;
}
else if ( (LA304_0==RULE_ID) ) {
alt304=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 304, 0, input);
throw nvae;
}
switch (alt304) {
case 1 :
// InternalContextMappingDSL.g:12316:5: (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:12316:5: (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) )
// InternalContextMappingDSL.g:12317:6: otherlv_6= '@' ( (otherlv_7= RULE_ID ) )
{
otherlv_6=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_6, grammarAccess.getDataTransferObjectAccess().getCommercialAtKeyword_5_1_0_0());
// InternalContextMappingDSL.g:12321:6: ( (otherlv_7= RULE_ID ) )
// InternalContextMappingDSL.g:12322:7: (otherlv_7= RULE_ID )
{
// InternalContextMappingDSL.g:12322:7: (otherlv_7= RULE_ID )
// InternalContextMappingDSL.g:12323:8: otherlv_7= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getDataTransferObjectRule());
}
otherlv_7=(Token)match(input,RULE_ID,FOLLOW_23);
newLeafNode(otherlv_7, grammarAccess.getDataTransferObjectAccess().getExtendsDataTransferObjectCrossReference_5_1_0_1_0());
}
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:12336:5: ( (lv_extendsName_8_0= ruleJavaIdentifier ) )
{
// InternalContextMappingDSL.g:12336:5: ( (lv_extendsName_8_0= ruleJavaIdentifier ) )
// InternalContextMappingDSL.g:12337:6: (lv_extendsName_8_0= ruleJavaIdentifier )
{
// InternalContextMappingDSL.g:12337:6: (lv_extendsName_8_0= ruleJavaIdentifier )
// InternalContextMappingDSL.g:12338:7: lv_extendsName_8_0= ruleJavaIdentifier
{
newCompositeNode(grammarAccess.getDataTransferObjectAccess().getExtendsNameJavaIdentifierParserRuleCall_5_1_1_0());
pushFollow(FOLLOW_23);
lv_extendsName_8_0=ruleJavaIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDataTransferObjectRule());
}
set(
current,
"extendsName",
lv_extendsName_8_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.JavaIdentifier");
afterParserOrEnumRuleCall();
}
}
}
break;
}
}
break;
}
// InternalContextMappingDSL.g:12357:3: (otherlv_9= '{' (otherlv_10= 'package' otherlv_11= '=' ( (lv_package_12_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_22_0= ruleDtoAttribute ) ) | ( (lv_references_23_0= ruleDtoReference ) ) )* otherlv_24= '}' )?
int alt310=2;
int LA310_0 = input.LA(1);
if ( (LA310_0==RULE_OPEN) ) {
alt310=1;
}
switch (alt310) {
case 1 :
// InternalContextMappingDSL.g:12358:4: otherlv_9= '{' (otherlv_10= 'package' otherlv_11= '=' ( (lv_package_12_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_22_0= ruleDtoAttribute ) ) | ( (lv_references_23_0= ruleDtoReference ) ) )* otherlv_24= '}'
{
otherlv_9=(Token)match(input,RULE_OPEN,FOLLOW_166);
newLeafNode(otherlv_9, grammarAccess.getDataTransferObjectAccess().getLeftCurlyBracketKeyword_6_0());
// InternalContextMappingDSL.g:12362:4: (otherlv_10= 'package' otherlv_11= '=' ( (lv_package_12_0= ruleJavaIdentifier ) ) )?
int alt306=2;
int LA306_0 = input.LA(1);
if ( (LA306_0==124) ) {
int LA306_1 = input.LA(2);
if ( (LA306_1==21) ) {
alt306=1;
}
}
switch (alt306) {
case 1 :
// InternalContextMappingDSL.g:12363:5: otherlv_10= 'package' otherlv_11= '=' ( (lv_package_12_0= ruleJavaIdentifier ) )
{
otherlv_10=(Token)match(input,124,FOLLOW_89);
newLeafNode(otherlv_10, grammarAccess.getDataTransferObjectAccess().getPackageKeyword_6_1_0());
otherlv_11=(Token)match(input,21,FOLLOW_10);
newLeafNode(otherlv_11, grammarAccess.getDataTransferObjectAccess().getEqualsSignKeyword_6_1_1());
// InternalContextMappingDSL.g:12371:5: ( (lv_package_12_0= ruleJavaIdentifier ) )
// InternalContextMappingDSL.g:12372:6: (lv_package_12_0= ruleJavaIdentifier )
{
// InternalContextMappingDSL.g:12372:6: (lv_package_12_0= ruleJavaIdentifier )
// InternalContextMappingDSL.g:12373:7: lv_package_12_0= ruleJavaIdentifier
{
newCompositeNode(grammarAccess.getDataTransferObjectAccess().getPackageJavaIdentifierParserRuleCall_6_1_2_0());
pushFollow(FOLLOW_166);
lv_package_12_0=ruleJavaIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDataTransferObjectRule());
}
set(
current,
"package",
lv_package_12_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.JavaIdentifier");
afterParserOrEnumRuleCall();
}
}
}
break;
}
// InternalContextMappingDSL.g:12391:4: ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:12392:5: ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:12392:5: ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:12393:6: ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getDataTransferObjectAccess().getUnorderedGroup_6_2());
// InternalContextMappingDSL.g:12396:6: ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:12397:7: ( ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:12397:7: ( ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) ) ) )*
loop308:
do {
int alt308=4;
int LA308_0 = input.LA(1);
if ( LA308_0 >= 86 && LA308_0 <= 87 && getUnorderedGroupHelper().canSelect(grammarAccess.getDataTransferObjectAccess().getUnorderedGroup_6_2(), 0) ) {
alt308=1;
}
else if ( LA308_0 == 82 && getUnorderedGroupHelper().canSelect(grammarAccess.getDataTransferObjectAccess().getUnorderedGroup_6_2(), 1) ) {
alt308=2;
}
else if ( LA308_0 == 133 && getUnorderedGroupHelper().canSelect(grammarAccess.getDataTransferObjectAccess().getUnorderedGroup_6_2(), 2) ) {
alt308=3;
}
switch (alt308) {
case 1 :
// InternalContextMappingDSL.g:12398:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) )
{
// InternalContextMappingDSL.g:12398:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) )
// InternalContextMappingDSL.g:12399:6: {...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDataTransferObjectAccess().getUnorderedGroup_6_2(), 0) ) {
throw new FailedPredicateException(input, "ruleDataTransferObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getDataTransferObjectAccess().getUnorderedGroup_6_2(), 0)");
}
// InternalContextMappingDSL.g:12399:118: ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) )
// InternalContextMappingDSL.g:12400:7: ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDataTransferObjectAccess().getUnorderedGroup_6_2(), 0);
// InternalContextMappingDSL.g:12403:10: ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) )
// InternalContextMappingDSL.g:12403:11: {...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDataTransferObject", "true");
}
// InternalContextMappingDSL.g:12403:20: ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) )
int alt307=2;
int LA307_0 = input.LA(1);
if ( (LA307_0==86) ) {
alt307=1;
}
else if ( (LA307_0==87) ) {
alt307=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 307, 0, input);
throw nvae;
}
switch (alt307) {
case 1 :
// InternalContextMappingDSL.g:12403:21: ( (lv_gapClass_14_0= 'gap' ) )
{
// InternalContextMappingDSL.g:12403:21: ( (lv_gapClass_14_0= 'gap' ) )
// InternalContextMappingDSL.g:12404:11: (lv_gapClass_14_0= 'gap' )
{
// InternalContextMappingDSL.g:12404:11: (lv_gapClass_14_0= 'gap' )
// InternalContextMappingDSL.g:12405:12: lv_gapClass_14_0= 'gap'
{
lv_gapClass_14_0=(Token)match(input,86,FOLLOW_166);
newLeafNode(lv_gapClass_14_0, grammarAccess.getDataTransferObjectAccess().getGapClassGapKeyword_6_2_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDataTransferObjectRule());
}
setWithLastConsumed(current, "gapClass", true, "gap");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:12418:10: ( (lv_noGapClass_15_0= 'nogap' ) )
{
// InternalContextMappingDSL.g:12418:10: ( (lv_noGapClass_15_0= 'nogap' ) )
// InternalContextMappingDSL.g:12419:11: (lv_noGapClass_15_0= 'nogap' )
{
// InternalContextMappingDSL.g:12419:11: (lv_noGapClass_15_0= 'nogap' )
// InternalContextMappingDSL.g:12420:12: lv_noGapClass_15_0= 'nogap'
{
lv_noGapClass_15_0=(Token)match(input,87,FOLLOW_166);
newLeafNode(lv_noGapClass_15_0, grammarAccess.getDataTransferObjectAccess().getNoGapClassNogapKeyword_6_2_0_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDataTransferObjectRule());
}
setWithLastConsumed(current, "noGapClass", true, "nogap");
}
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDataTransferObjectAccess().getUnorderedGroup_6_2());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:12438:5: ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:12438:5: ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:12439:6: {...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDataTransferObjectAccess().getUnorderedGroup_6_2(), 1) ) {
throw new FailedPredicateException(input, "ruleDataTransferObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getDataTransferObjectAccess().getUnorderedGroup_6_2(), 1)");
}
// InternalContextMappingDSL.g:12439:118: ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:12440:7: ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDataTransferObjectAccess().getUnorderedGroup_6_2(), 1);
// InternalContextMappingDSL.g:12443:10: ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:12443:11: {...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDataTransferObject", "true");
}
// InternalContextMappingDSL.g:12443:20: (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:12443:21: otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) )
{
otherlv_16=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_16, grammarAccess.getDataTransferObjectAccess().getHintKeyword_6_2_1_0());
otherlv_17=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_17, grammarAccess.getDataTransferObjectAccess().getEqualsSignKeyword_6_2_1_1());
// InternalContextMappingDSL.g:12451:10: ( (lv_hint_18_0= RULE_STRING ) )
// InternalContextMappingDSL.g:12452:11: (lv_hint_18_0= RULE_STRING )
{
// InternalContextMappingDSL.g:12452:11: (lv_hint_18_0= RULE_STRING )
// InternalContextMappingDSL.g:12453:12: lv_hint_18_0= RULE_STRING
{
lv_hint_18_0=(Token)match(input,RULE_STRING,FOLLOW_166);
newLeafNode(lv_hint_18_0, grammarAccess.getDataTransferObjectAccess().getHintSTRINGTerminalRuleCall_6_2_1_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDataTransferObjectRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_18_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDataTransferObjectAccess().getUnorderedGroup_6_2());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:12475:5: ({...}? => ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:12475:5: ({...}? => ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:12476:6: {...}? => ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDataTransferObjectAccess().getUnorderedGroup_6_2(), 2) ) {
throw new FailedPredicateException(input, "ruleDataTransferObject", "getUnorderedGroupHelper().canSelect(grammarAccess.getDataTransferObjectAccess().getUnorderedGroup_6_2(), 2)");
}
// InternalContextMappingDSL.g:12476:118: ( ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:12477:7: ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDataTransferObjectAccess().getUnorderedGroup_6_2(), 2);
// InternalContextMappingDSL.g:12480:10: ({...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:12480:11: {...}? => (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDataTransferObject", "true");
}
// InternalContextMappingDSL.g:12480:20: (otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:12480:21: otherlv_19= 'validate' otherlv_20= '=' ( (lv_validate_21_0= RULE_STRING ) )
{
otherlv_19=(Token)match(input,133,FOLLOW_89);
newLeafNode(otherlv_19, grammarAccess.getDataTransferObjectAccess().getValidateKeyword_6_2_2_0());
otherlv_20=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_20, grammarAccess.getDataTransferObjectAccess().getEqualsSignKeyword_6_2_2_1());
// InternalContextMappingDSL.g:12488:10: ( (lv_validate_21_0= RULE_STRING ) )
// InternalContextMappingDSL.g:12489:11: (lv_validate_21_0= RULE_STRING )
{
// InternalContextMappingDSL.g:12489:11: (lv_validate_21_0= RULE_STRING )
// InternalContextMappingDSL.g:12490:12: lv_validate_21_0= RULE_STRING
{
lv_validate_21_0=(Token)match(input,RULE_STRING,FOLLOW_166);
newLeafNode(lv_validate_21_0, grammarAccess.getDataTransferObjectAccess().getValidateSTRINGTerminalRuleCall_6_2_2_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDataTransferObjectRule());
}
setWithLastConsumed(
current,
"validate",
lv_validate_21_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDataTransferObjectAccess().getUnorderedGroup_6_2());
}
}
}
break;
default :
break loop308;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getDataTransferObjectAccess().getUnorderedGroup_6_2());
}
// InternalContextMappingDSL.g:12519:4: ( ( (lv_attributes_22_0= ruleDtoAttribute ) ) | ( (lv_references_23_0= ruleDtoReference ) ) )*
loop309:
do {
int alt309=3;
switch ( input.LA(1) ) {
case RULE_STRING:
{
int LA309_2 = input.LA(2);
if ( (LA309_2==RULE_ID||LA309_2==124||(LA309_2>=185 && LA309_2<=206)||LA309_2==233||(LA309_2>=243 && LA309_2<=249)) ) {
alt309=1;
}
else if ( (LA309_2==RULE_REF) ) {
alt309=2;
}
}
break;
case RULE_ID:
case 124:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
case 247:
case 248:
case 249:
{
alt309=1;
}
break;
case RULE_REF:
{
alt309=2;
}
break;
}
switch (alt309) {
case 1 :
// InternalContextMappingDSL.g:12520:5: ( (lv_attributes_22_0= ruleDtoAttribute ) )
{
// InternalContextMappingDSL.g:12520:5: ( (lv_attributes_22_0= ruleDtoAttribute ) )
// InternalContextMappingDSL.g:12521:6: (lv_attributes_22_0= ruleDtoAttribute )
{
// InternalContextMappingDSL.g:12521:6: (lv_attributes_22_0= ruleDtoAttribute )
// InternalContextMappingDSL.g:12522:7: lv_attributes_22_0= ruleDtoAttribute
{
newCompositeNode(grammarAccess.getDataTransferObjectAccess().getAttributesDtoAttributeParserRuleCall_6_3_0_0());
pushFollow(FOLLOW_167);
lv_attributes_22_0=ruleDtoAttribute();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDataTransferObjectRule());
}
add(
current,
"attributes",
lv_attributes_22_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.DtoAttribute");
afterParserOrEnumRuleCall();
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:12540:5: ( (lv_references_23_0= ruleDtoReference ) )
{
// InternalContextMappingDSL.g:12540:5: ( (lv_references_23_0= ruleDtoReference ) )
// InternalContextMappingDSL.g:12541:6: (lv_references_23_0= ruleDtoReference )
{
// InternalContextMappingDSL.g:12541:6: (lv_references_23_0= ruleDtoReference )
// InternalContextMappingDSL.g:12542:7: lv_references_23_0= ruleDtoReference
{
newCompositeNode(grammarAccess.getDataTransferObjectAccess().getReferencesDtoReferenceParserRuleCall_6_3_1_0());
pushFollow(FOLLOW_167);
lv_references_23_0=ruleDtoReference();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDataTransferObjectRule());
}
add(
current,
"references",
lv_references_23_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.DtoReference");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop309;
}
} while (true);
otherlv_24=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(otherlv_24, grammarAccess.getDataTransferObjectAccess().getRightCurlyBracketKeyword_6_4());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleDataTransferObject"
// $ANTLR start "entryRuleBasicType"
// InternalContextMappingDSL.g:12569:1: entryRuleBasicType returns [EObject current=null] : iv_ruleBasicType= ruleBasicType EOF ;
public final EObject entryRuleBasicType() throws RecognitionException {
EObject current = null;
EObject iv_ruleBasicType = null;
try {
// InternalContextMappingDSL.g:12569:50: (iv_ruleBasicType= ruleBasicType EOF )
// InternalContextMappingDSL.g:12570:2: iv_ruleBasicType= ruleBasicType EOF
{
newCompositeNode(grammarAccess.getBasicTypeRule());
pushFollow(FOLLOW_1);
iv_ruleBasicType=ruleBasicType();
state._fsp--;
current =iv_ruleBasicType;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleBasicType"
// $ANTLR start "ruleBasicType"
// InternalContextMappingDSL.g:12576:1: ruleBasicType returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'BasicType' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= 'with' (otherlv_4= '@' )? ( (otherlv_5= RULE_ID ) ) )* (otherlv_6= '{' (otherlv_7= 'package' otherlv_8= '=' ( (lv_package_9_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_19_0= ruleAttribute ) ) | ( (lv_references_20_0= ruleReference ) ) | ( (lv_operations_21_0= ruleDomainObjectOperation ) ) )* otherlv_22= '}' )? ) ;
public final EObject ruleBasicType() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token otherlv_1=null;
Token lv_name_2_0=null;
Token otherlv_3=null;
Token otherlv_4=null;
Token otherlv_5=null;
Token otherlv_6=null;
Token otherlv_7=null;
Token otherlv_8=null;
Token lv_notImmutable_11_0=null;
Token otherlv_12=null;
Token otherlv_13=null;
Token lv_gapClass_14_0=null;
Token lv_noGapClass_15_0=null;
Token otherlv_16=null;
Token otherlv_17=null;
Token lv_hint_18_0=null;
Token otherlv_22=null;
AntlrDatatypeRuleToken lv_package_9_0 = null;
EObject lv_attributes_19_0 = null;
EObject lv_references_20_0 = null;
EObject lv_operations_21_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:12582:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'BasicType' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= 'with' (otherlv_4= '@' )? ( (otherlv_5= RULE_ID ) ) )* (otherlv_6= '{' (otherlv_7= 'package' otherlv_8= '=' ( (lv_package_9_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_19_0= ruleAttribute ) ) | ( (lv_references_20_0= ruleReference ) ) | ( (lv_operations_21_0= ruleDomainObjectOperation ) ) )* otherlv_22= '}' )? ) )
// InternalContextMappingDSL.g:12583:2: ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'BasicType' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= 'with' (otherlv_4= '@' )? ( (otherlv_5= RULE_ID ) ) )* (otherlv_6= '{' (otherlv_7= 'package' otherlv_8= '=' ( (lv_package_9_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_19_0= ruleAttribute ) ) | ( (lv_references_20_0= ruleReference ) ) | ( (lv_operations_21_0= ruleDomainObjectOperation ) ) )* otherlv_22= '}' )? )
{
// InternalContextMappingDSL.g:12583:2: ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'BasicType' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= 'with' (otherlv_4= '@' )? ( (otherlv_5= RULE_ID ) ) )* (otherlv_6= '{' (otherlv_7= 'package' otherlv_8= '=' ( (lv_package_9_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_19_0= ruleAttribute ) ) | ( (lv_references_20_0= ruleReference ) ) | ( (lv_operations_21_0= ruleDomainObjectOperation ) ) )* otherlv_22= '}' )? )
// InternalContextMappingDSL.g:12584:3: ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'BasicType' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= 'with' (otherlv_4= '@' )? ( (otherlv_5= RULE_ID ) ) )* (otherlv_6= '{' (otherlv_7= 'package' otherlv_8= '=' ( (lv_package_9_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_19_0= ruleAttribute ) ) | ( (lv_references_20_0= ruleReference ) ) | ( (lv_operations_21_0= ruleDomainObjectOperation ) ) )* otherlv_22= '}' )?
{
// InternalContextMappingDSL.g:12584:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt311=2;
int LA311_0 = input.LA(1);
if ( (LA311_0==RULE_STRING) ) {
alt311=1;
}
switch (alt311) {
case 1 :
// InternalContextMappingDSL.g:12585:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:12585:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:12586:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_168);
newLeafNode(lv_doc_0_0, grammarAccess.getBasicTypeAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getBasicTypeRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
otherlv_1=(Token)match(input,145,FOLLOW_10);
newLeafNode(otherlv_1, grammarAccess.getBasicTypeAccess().getBasicTypeKeyword_1());
// InternalContextMappingDSL.g:12606:3: ( (lv_name_2_0= RULE_ID ) )
// InternalContextMappingDSL.g:12607:4: (lv_name_2_0= RULE_ID )
{
// InternalContextMappingDSL.g:12607:4: (lv_name_2_0= RULE_ID )
// InternalContextMappingDSL.g:12608:5: lv_name_2_0= RULE_ID
{
lv_name_2_0=(Token)match(input,RULE_ID,FOLLOW_134);
newLeafNode(lv_name_2_0, grammarAccess.getBasicTypeAccess().getNameIDTerminalRuleCall_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getBasicTypeRule());
}
setWithLastConsumed(
current,
"name",
lv_name_2_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:12624:3: (otherlv_3= 'with' (otherlv_4= '@' )? ( (otherlv_5= RULE_ID ) ) )*
loop313:
do {
int alt313=2;
int LA313_0 = input.LA(1);
if ( (LA313_0==123) ) {
alt313=1;
}
switch (alt313) {
case 1 :
// InternalContextMappingDSL.g:12625:4: otherlv_3= 'with' (otherlv_4= '@' )? ( (otherlv_5= RULE_ID ) )
{
otherlv_3=(Token)match(input,123,FOLLOW_105);
newLeafNode(otherlv_3, grammarAccess.getBasicTypeAccess().getWithKeyword_3_0());
// InternalContextMappingDSL.g:12629:4: (otherlv_4= '@' )?
int alt312=2;
int LA312_0 = input.LA(1);
if ( (LA312_0==94) ) {
alt312=1;
}
switch (alt312) {
case 1 :
// InternalContextMappingDSL.g:12630:5: otherlv_4= '@'
{
otherlv_4=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_4, grammarAccess.getBasicTypeAccess().getCommercialAtKeyword_3_1());
}
break;
}
// InternalContextMappingDSL.g:12635:4: ( (otherlv_5= RULE_ID ) )
// InternalContextMappingDSL.g:12636:5: (otherlv_5= RULE_ID )
{
// InternalContextMappingDSL.g:12636:5: (otherlv_5= RULE_ID )
// InternalContextMappingDSL.g:12637:6: otherlv_5= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getBasicTypeRule());
}
otherlv_5=(Token)match(input,RULE_ID,FOLLOW_134);
newLeafNode(otherlv_5, grammarAccess.getBasicTypeAccess().getTraitsTraitCrossReference_3_2_0());
}
}
}
break;
default :
break loop313;
}
} while (true);
// InternalContextMappingDSL.g:12649:3: (otherlv_6= '{' (otherlv_7= 'package' otherlv_8= '=' ( (lv_package_9_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_19_0= ruleAttribute ) ) | ( (lv_references_20_0= ruleReference ) ) | ( (lv_operations_21_0= ruleDomainObjectOperation ) ) )* otherlv_22= '}' )?
int alt319=2;
int LA319_0 = input.LA(1);
if ( (LA319_0==RULE_OPEN) ) {
alt319=1;
}
switch (alt319) {
case 1 :
// InternalContextMappingDSL.g:12650:4: otherlv_6= '{' (otherlv_7= 'package' otherlv_8= '=' ( (lv_package_9_0= ruleJavaIdentifier ) ) )? ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) ( ( (lv_attributes_19_0= ruleAttribute ) ) | ( (lv_references_20_0= ruleReference ) ) | ( (lv_operations_21_0= ruleDomainObjectOperation ) ) )* otherlv_22= '}'
{
otherlv_6=(Token)match(input,RULE_OPEN,FOLLOW_169);
newLeafNode(otherlv_6, grammarAccess.getBasicTypeAccess().getLeftCurlyBracketKeyword_4_0());
// InternalContextMappingDSL.g:12654:4: (otherlv_7= 'package' otherlv_8= '=' ( (lv_package_9_0= ruleJavaIdentifier ) ) )?
int alt314=2;
int LA314_0 = input.LA(1);
if ( (LA314_0==124) ) {
int LA314_1 = input.LA(2);
if ( (LA314_1==21) ) {
alt314=1;
}
}
switch (alt314) {
case 1 :
// InternalContextMappingDSL.g:12655:5: otherlv_7= 'package' otherlv_8= '=' ( (lv_package_9_0= ruleJavaIdentifier ) )
{
otherlv_7=(Token)match(input,124,FOLLOW_89);
newLeafNode(otherlv_7, grammarAccess.getBasicTypeAccess().getPackageKeyword_4_1_0());
otherlv_8=(Token)match(input,21,FOLLOW_10);
newLeafNode(otherlv_8, grammarAccess.getBasicTypeAccess().getEqualsSignKeyword_4_1_1());
// InternalContextMappingDSL.g:12663:5: ( (lv_package_9_0= ruleJavaIdentifier ) )
// InternalContextMappingDSL.g:12664:6: (lv_package_9_0= ruleJavaIdentifier )
{
// InternalContextMappingDSL.g:12664:6: (lv_package_9_0= ruleJavaIdentifier )
// InternalContextMappingDSL.g:12665:7: lv_package_9_0= ruleJavaIdentifier
{
newCompositeNode(grammarAccess.getBasicTypeAccess().getPackageJavaIdentifierParserRuleCall_4_1_2_0());
pushFollow(FOLLOW_169);
lv_package_9_0=ruleJavaIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getBasicTypeRule());
}
set(
current,
"package",
lv_package_9_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.JavaIdentifier");
afterParserOrEnumRuleCall();
}
}
}
break;
}
// InternalContextMappingDSL.g:12683:4: ( ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:12684:5: ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:12684:5: ( ( ( ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:12685:6: ( ( ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getBasicTypeAccess().getUnorderedGroup_4_2());
// InternalContextMappingDSL.g:12688:6: ( ( ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:12689:7: ( ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:12689:7: ( ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) ) )*
loop317:
do {
int alt317=4;
int LA317_0 = input.LA(1);
if ( ( LA317_0 == RULE_NOT || LA317_0 == 137 ) && getUnorderedGroupHelper().canSelect(grammarAccess.getBasicTypeAccess().getUnorderedGroup_4_2(), 0) ) {
alt317=1;
}
else if ( LA317_0 >= 86 && LA317_0 <= 87 && getUnorderedGroupHelper().canSelect(grammarAccess.getBasicTypeAccess().getUnorderedGroup_4_2(), 1) ) {
alt317=2;
}
else if ( LA317_0 == 82 && getUnorderedGroupHelper().canSelect(grammarAccess.getBasicTypeAccess().getUnorderedGroup_4_2(), 2) ) {
alt317=3;
}
switch (alt317) {
case 1 :
// InternalContextMappingDSL.g:12690:5: ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) ) )
{
// InternalContextMappingDSL.g:12690:5: ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) ) )
// InternalContextMappingDSL.g:12691:6: {...}? => ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getBasicTypeAccess().getUnorderedGroup_4_2(), 0) ) {
throw new FailedPredicateException(input, "ruleBasicType", "getUnorderedGroupHelper().canSelect(grammarAccess.getBasicTypeAccess().getUnorderedGroup_4_2(), 0)");
}
// InternalContextMappingDSL.g:12691:109: ( ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) ) )
// InternalContextMappingDSL.g:12692:7: ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) )
{
getUnorderedGroupHelper().select(grammarAccess.getBasicTypeAccess().getUnorderedGroup_4_2(), 0);
// InternalContextMappingDSL.g:12695:10: ({...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' ) )
// InternalContextMappingDSL.g:12695:11: {...}? => ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleBasicType", "true");
}
// InternalContextMappingDSL.g:12695:20: ( ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' ) | otherlv_13= 'immutable' )
int alt315=2;
int LA315_0 = input.LA(1);
if ( (LA315_0==RULE_NOT) ) {
alt315=1;
}
else if ( (LA315_0==137) ) {
alt315=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 315, 0, input);
throw nvae;
}
switch (alt315) {
case 1 :
// InternalContextMappingDSL.g:12695:21: ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' )
{
// InternalContextMappingDSL.g:12695:21: ( ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable' )
// InternalContextMappingDSL.g:12696:11: ( (lv_notImmutable_11_0= RULE_NOT ) ) otherlv_12= 'immutable'
{
// InternalContextMappingDSL.g:12696:11: ( (lv_notImmutable_11_0= RULE_NOT ) )
// InternalContextMappingDSL.g:12697:12: (lv_notImmutable_11_0= RULE_NOT )
{
// InternalContextMappingDSL.g:12697:12: (lv_notImmutable_11_0= RULE_NOT )
// InternalContextMappingDSL.g:12698:13: lv_notImmutable_11_0= RULE_NOT
{
lv_notImmutable_11_0=(Token)match(input,RULE_NOT,FOLLOW_146);
newLeafNode(lv_notImmutable_11_0, grammarAccess.getBasicTypeAccess().getNotImmutableNOTTerminalRuleCall_4_2_0_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getBasicTypeRule());
}
setWithLastConsumed(
current,
"notImmutable",
true,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.NOT");
}
}
otherlv_12=(Token)match(input,137,FOLLOW_169);
newLeafNode(otherlv_12, grammarAccess.getBasicTypeAccess().getImmutableKeyword_4_2_0_0_1());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:12720:10: otherlv_13= 'immutable'
{
otherlv_13=(Token)match(input,137,FOLLOW_169);
newLeafNode(otherlv_13, grammarAccess.getBasicTypeAccess().getImmutableKeyword_4_2_0_1());
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getBasicTypeAccess().getUnorderedGroup_4_2());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:12730:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) )
{
// InternalContextMappingDSL.g:12730:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) ) )
// InternalContextMappingDSL.g:12731:6: {...}? => ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getBasicTypeAccess().getUnorderedGroup_4_2(), 1) ) {
throw new FailedPredicateException(input, "ruleBasicType", "getUnorderedGroupHelper().canSelect(grammarAccess.getBasicTypeAccess().getUnorderedGroup_4_2(), 1)");
}
// InternalContextMappingDSL.g:12731:109: ( ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) ) )
// InternalContextMappingDSL.g:12732:7: ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getBasicTypeAccess().getUnorderedGroup_4_2(), 1);
// InternalContextMappingDSL.g:12735:10: ({...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) ) )
// InternalContextMappingDSL.g:12735:11: {...}? => ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleBasicType", "true");
}
// InternalContextMappingDSL.g:12735:20: ( ( (lv_gapClass_14_0= 'gap' ) ) | ( (lv_noGapClass_15_0= 'nogap' ) ) )
int alt316=2;
int LA316_0 = input.LA(1);
if ( (LA316_0==86) ) {
alt316=1;
}
else if ( (LA316_0==87) ) {
alt316=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 316, 0, input);
throw nvae;
}
switch (alt316) {
case 1 :
// InternalContextMappingDSL.g:12735:21: ( (lv_gapClass_14_0= 'gap' ) )
{
// InternalContextMappingDSL.g:12735:21: ( (lv_gapClass_14_0= 'gap' ) )
// InternalContextMappingDSL.g:12736:11: (lv_gapClass_14_0= 'gap' )
{
// InternalContextMappingDSL.g:12736:11: (lv_gapClass_14_0= 'gap' )
// InternalContextMappingDSL.g:12737:12: lv_gapClass_14_0= 'gap'
{
lv_gapClass_14_0=(Token)match(input,86,FOLLOW_169);
newLeafNode(lv_gapClass_14_0, grammarAccess.getBasicTypeAccess().getGapClassGapKeyword_4_2_1_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getBasicTypeRule());
}
setWithLastConsumed(current, "gapClass", true, "gap");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:12750:10: ( (lv_noGapClass_15_0= 'nogap' ) )
{
// InternalContextMappingDSL.g:12750:10: ( (lv_noGapClass_15_0= 'nogap' ) )
// InternalContextMappingDSL.g:12751:11: (lv_noGapClass_15_0= 'nogap' )
{
// InternalContextMappingDSL.g:12751:11: (lv_noGapClass_15_0= 'nogap' )
// InternalContextMappingDSL.g:12752:12: lv_noGapClass_15_0= 'nogap'
{
lv_noGapClass_15_0=(Token)match(input,87,FOLLOW_169);
newLeafNode(lv_noGapClass_15_0, grammarAccess.getBasicTypeAccess().getNoGapClassNogapKeyword_4_2_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getBasicTypeRule());
}
setWithLastConsumed(current, "noGapClass", true, "nogap");
}
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getBasicTypeAccess().getUnorderedGroup_4_2());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:12770:5: ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:12770:5: ({...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:12771:6: {...}? => ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getBasicTypeAccess().getUnorderedGroup_4_2(), 2) ) {
throw new FailedPredicateException(input, "ruleBasicType", "getUnorderedGroupHelper().canSelect(grammarAccess.getBasicTypeAccess().getUnorderedGroup_4_2(), 2)");
}
// InternalContextMappingDSL.g:12771:109: ( ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:12772:7: ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getBasicTypeAccess().getUnorderedGroup_4_2(), 2);
// InternalContextMappingDSL.g:12775:10: ({...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:12775:11: {...}? => (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleBasicType", "true");
}
// InternalContextMappingDSL.g:12775:20: (otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:12775:21: otherlv_16= 'hint' otherlv_17= '=' ( (lv_hint_18_0= RULE_STRING ) )
{
otherlv_16=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_16, grammarAccess.getBasicTypeAccess().getHintKeyword_4_2_2_0());
otherlv_17=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_17, grammarAccess.getBasicTypeAccess().getEqualsSignKeyword_4_2_2_1());
// InternalContextMappingDSL.g:12783:10: ( (lv_hint_18_0= RULE_STRING ) )
// InternalContextMappingDSL.g:12784:11: (lv_hint_18_0= RULE_STRING )
{
// InternalContextMappingDSL.g:12784:11: (lv_hint_18_0= RULE_STRING )
// InternalContextMappingDSL.g:12785:12: lv_hint_18_0= RULE_STRING
{
lv_hint_18_0=(Token)match(input,RULE_STRING,FOLLOW_169);
newLeafNode(lv_hint_18_0, grammarAccess.getBasicTypeAccess().getHintSTRINGTerminalRuleCall_4_2_2_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getBasicTypeRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_18_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getBasicTypeAccess().getUnorderedGroup_4_2());
}
}
}
break;
default :
break loop317;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getBasicTypeAccess().getUnorderedGroup_4_2());
}
// InternalContextMappingDSL.g:12814:4: ( ( (lv_attributes_19_0= ruleAttribute ) ) | ( (lv_references_20_0= ruleReference ) ) | ( (lv_operations_21_0= ruleDomainObjectOperation ) ) )*
loop318:
do {
int alt318=4;
switch ( input.LA(1) ) {
case RULE_STRING:
{
switch ( input.LA(2) ) {
case RULE_ID:
case 124:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
case 247:
case 248:
case 249:
{
alt318=1;
}
break;
case 142:
case 143:
{
alt318=3;
}
break;
case RULE_REF:
{
alt318=2;
}
break;
}
}
break;
case RULE_ID:
case 124:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
case 247:
case 248:
case 249:
{
alt318=1;
}
break;
case RULE_REF:
{
alt318=2;
}
break;
case 142:
case 143:
{
alt318=3;
}
break;
}
switch (alt318) {
case 1 :
// InternalContextMappingDSL.g:12815:5: ( (lv_attributes_19_0= ruleAttribute ) )
{
// InternalContextMappingDSL.g:12815:5: ( (lv_attributes_19_0= ruleAttribute ) )
// InternalContextMappingDSL.g:12816:6: (lv_attributes_19_0= ruleAttribute )
{
// InternalContextMappingDSL.g:12816:6: (lv_attributes_19_0= ruleAttribute )
// InternalContextMappingDSL.g:12817:7: lv_attributes_19_0= ruleAttribute
{
newCompositeNode(grammarAccess.getBasicTypeAccess().getAttributesAttributeParserRuleCall_4_3_0_0());
pushFollow(FOLLOW_157);
lv_attributes_19_0=ruleAttribute();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getBasicTypeRule());
}
add(
current,
"attributes",
lv_attributes_19_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Attribute");
afterParserOrEnumRuleCall();
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:12835:5: ( (lv_references_20_0= ruleReference ) )
{
// InternalContextMappingDSL.g:12835:5: ( (lv_references_20_0= ruleReference ) )
// InternalContextMappingDSL.g:12836:6: (lv_references_20_0= ruleReference )
{
// InternalContextMappingDSL.g:12836:6: (lv_references_20_0= ruleReference )
// InternalContextMappingDSL.g:12837:7: lv_references_20_0= ruleReference
{
newCompositeNode(grammarAccess.getBasicTypeAccess().getReferencesReferenceParserRuleCall_4_3_1_0());
pushFollow(FOLLOW_157);
lv_references_20_0=ruleReference();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getBasicTypeRule());
}
add(
current,
"references",
lv_references_20_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Reference");
afterParserOrEnumRuleCall();
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:12855:5: ( (lv_operations_21_0= ruleDomainObjectOperation ) )
{
// InternalContextMappingDSL.g:12855:5: ( (lv_operations_21_0= ruleDomainObjectOperation ) )
// InternalContextMappingDSL.g:12856:6: (lv_operations_21_0= ruleDomainObjectOperation )
{
// InternalContextMappingDSL.g:12856:6: (lv_operations_21_0= ruleDomainObjectOperation )
// InternalContextMappingDSL.g:12857:7: lv_operations_21_0= ruleDomainObjectOperation
{
newCompositeNode(grammarAccess.getBasicTypeAccess().getOperationsDomainObjectOperationParserRuleCall_4_3_2_0());
pushFollow(FOLLOW_157);
lv_operations_21_0=ruleDomainObjectOperation();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getBasicTypeRule());
}
add(
current,
"operations",
lv_operations_21_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.DomainObjectOperation");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop318;
}
} while (true);
otherlv_22=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(otherlv_22, grammarAccess.getBasicTypeAccess().getRightCurlyBracketKeyword_4_4());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleBasicType"
// $ANTLR start "entryRuleAttribute"
// InternalContextMappingDSL.g:12884:1: entryRuleAttribute returns [EObject current=null] : iv_ruleAttribute= ruleAttribute EOF ;
public final EObject entryRuleAttribute() throws RecognitionException {
EObject current = null;
EObject iv_ruleAttribute = null;
try {
// InternalContextMappingDSL.g:12884:50: (iv_ruleAttribute= ruleAttribute EOF )
// InternalContextMappingDSL.g:12885:2: iv_ruleAttribute= ruleAttribute EOF
{
newCompositeNode(grammarAccess.getAttributeRule());
pushFollow(FOLLOW_1);
iv_ruleAttribute=ruleAttribute();
state._fsp--;
current =iv_ruleAttribute;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleAttribute"
// $ANTLR start "ruleAttribute"
// InternalContextMappingDSL.g:12891:1: ruleAttribute returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( ( (lv_collectionType_2_0= ruleCollectionType ) ) otherlv_3= '<' ( (lv_type_4_0= ruleType ) ) otherlv_5= '>' ) | ( (lv_type_6_0= ruleType ) ) ) ( (lv_name_7_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_9_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_index_21_0= 'index' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_85_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) (otherlv_92= ';' )? ) ;
public final EObject ruleAttribute() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token otherlv_3=null;
Token otherlv_5=null;
Token lv_name_7_0=null;
Token lv_key_9_0=null;
Token lv_notChangeable_10_0=null;
Token otherlv_11=null;
Token otherlv_12=null;
Token lv_required_13_0=null;
Token this_NOT_14=null;
Token otherlv_15=null;
Token lv_nullable_16_0=null;
Token this_NOT_17=null;
Token otherlv_18=null;
Token otherlv_19=null;
Token lv_nullableMessage_20_0=null;
Token lv_index_21_0=null;
Token lv_assertFalse_22_0=null;
Token otherlv_23=null;
Token lv_assertFalseMessage_24_0=null;
Token lv_assertTrue_25_0=null;
Token otherlv_26=null;
Token lv_assertTrueMessage_27_0=null;
Token otherlv_28=null;
Token otherlv_29=null;
Token lv_hint_30_0=null;
Token lv_creditCardNumber_31_0=null;
Token otherlv_32=null;
Token lv_creditCardNumberMessage_33_0=null;
Token otherlv_34=null;
Token otherlv_35=null;
Token lv_digits_36_0=null;
Token lv_email_37_0=null;
Token otherlv_38=null;
Token lv_emailMessage_39_0=null;
Token lv_future_40_0=null;
Token otherlv_41=null;
Token lv_futureMessage_42_0=null;
Token lv_past_43_0=null;
Token otherlv_44=null;
Token lv_pastMessage_45_0=null;
Token otherlv_46=null;
Token otherlv_47=null;
Token lv_max_48_0=null;
Token otherlv_49=null;
Token otherlv_50=null;
Token lv_min_51_0=null;
Token otherlv_52=null;
Token otherlv_53=null;
Token lv_decimalMax_54_0=null;
Token otherlv_55=null;
Token otherlv_56=null;
Token lv_decimalMin_57_0=null;
Token lv_notEmpty_58_0=null;
Token otherlv_59=null;
Token lv_notEmptyMessage_60_0=null;
Token lv_notBlank_61_0=null;
Token otherlv_62=null;
Token lv_notBlankMessage_63_0=null;
Token otherlv_64=null;
Token otherlv_65=null;
Token lv_pattern_66_0=null;
Token otherlv_67=null;
Token otherlv_68=null;
Token lv_range_69_0=null;
Token otherlv_70=null;
Token otherlv_71=null;
Token lv_size_72_0=null;
Token otherlv_73=null;
Token otherlv_74=null;
Token lv_length_75_0=null;
Token otherlv_76=null;
Token otherlv_77=null;
Token lv_scriptAssert_78_0=null;
Token otherlv_79=null;
Token otherlv_80=null;
Token lv_url_81_0=null;
Token otherlv_82=null;
Token otherlv_83=null;
Token lv_validate_84_0=null;
Token lv_transient_85_0=null;
Token otherlv_86=null;
Token otherlv_87=null;
Token lv_databaseColumn_88_0=null;
Token otherlv_89=null;
Token otherlv_90=null;
Token lv_databaseType_91_0=null;
Token otherlv_92=null;
Enumerator lv_visibility_1_0 = null;
Enumerator lv_collectionType_2_0 = null;
AntlrDatatypeRuleToken lv_type_4_0 = null;
AntlrDatatypeRuleToken lv_type_6_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:12897:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( ( (lv_collectionType_2_0= ruleCollectionType ) ) otherlv_3= '<' ( (lv_type_4_0= ruleType ) ) otherlv_5= '>' ) | ( (lv_type_6_0= ruleType ) ) ) ( (lv_name_7_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_9_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_index_21_0= 'index' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_85_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) (otherlv_92= ';' )? ) )
// InternalContextMappingDSL.g:12898:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( ( (lv_collectionType_2_0= ruleCollectionType ) ) otherlv_3= '<' ( (lv_type_4_0= ruleType ) ) otherlv_5= '>' ) | ( (lv_type_6_0= ruleType ) ) ) ( (lv_name_7_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_9_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_index_21_0= 'index' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_85_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) (otherlv_92= ';' )? )
{
// InternalContextMappingDSL.g:12898:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( ( (lv_collectionType_2_0= ruleCollectionType ) ) otherlv_3= '<' ( (lv_type_4_0= ruleType ) ) otherlv_5= '>' ) | ( (lv_type_6_0= ruleType ) ) ) ( (lv_name_7_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_9_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_index_21_0= 'index' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_85_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) (otherlv_92= ';' )? )
// InternalContextMappingDSL.g:12899:3: ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( ( (lv_collectionType_2_0= ruleCollectionType ) ) otherlv_3= '<' ( (lv_type_4_0= ruleType ) ) otherlv_5= '>' ) | ( (lv_type_6_0= ruleType ) ) ) ( (lv_name_7_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_9_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_index_21_0= 'index' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_85_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) (otherlv_92= ';' )?
{
// InternalContextMappingDSL.g:12899:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt320=2;
int LA320_0 = input.LA(1);
if ( (LA320_0==RULE_STRING) ) {
alt320=1;
}
switch (alt320) {
case 1 :
// InternalContextMappingDSL.g:12900:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:12900:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:12901:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_170);
newLeafNode(lv_doc_0_0, grammarAccess.getAttributeAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
// InternalContextMappingDSL.g:12917:3: ( (lv_visibility_1_0= ruleVisibility ) )?
int alt321=2;
int LA321_0 = input.LA(1);
if ( (LA321_0==124||(LA321_0>=247 && LA321_0<=249)) ) {
alt321=1;
}
switch (alt321) {
case 1 :
// InternalContextMappingDSL.g:12918:4: (lv_visibility_1_0= ruleVisibility )
{
// InternalContextMappingDSL.g:12918:4: (lv_visibility_1_0= ruleVisibility )
// InternalContextMappingDSL.g:12919:5: lv_visibility_1_0= ruleVisibility
{
newCompositeNode(grammarAccess.getAttributeAccess().getVisibilityVisibilityEnumRuleCall_1_0());
pushFollow(FOLLOW_171);
lv_visibility_1_0=ruleVisibility();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getAttributeRule());
}
set(
current,
"visibility",
lv_visibility_1_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Visibility");
afterParserOrEnumRuleCall();
}
}
break;
}
// InternalContextMappingDSL.g:12936:3: ( ( ( (lv_collectionType_2_0= ruleCollectionType ) ) otherlv_3= '<' ( (lv_type_4_0= ruleType ) ) otherlv_5= '>' ) | ( (lv_type_6_0= ruleType ) ) )
int alt322=2;
int LA322_0 = input.LA(1);
if ( (LA322_0==233||(LA322_0>=243 && LA322_0<=246)) ) {
alt322=1;
}
else if ( (LA322_0==RULE_ID||(LA322_0>=185 && LA322_0<=206)) ) {
alt322=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 322, 0, input);
throw nvae;
}
switch (alt322) {
case 1 :
// InternalContextMappingDSL.g:12937:4: ( ( (lv_collectionType_2_0= ruleCollectionType ) ) otherlv_3= '<' ( (lv_type_4_0= ruleType ) ) otherlv_5= '>' )
{
// InternalContextMappingDSL.g:12937:4: ( ( (lv_collectionType_2_0= ruleCollectionType ) ) otherlv_3= '<' ( (lv_type_4_0= ruleType ) ) otherlv_5= '>' )
// InternalContextMappingDSL.g:12938:5: ( (lv_collectionType_2_0= ruleCollectionType ) ) otherlv_3= '<' ( (lv_type_4_0= ruleType ) ) otherlv_5= '>'
{
// InternalContextMappingDSL.g:12938:5: ( (lv_collectionType_2_0= ruleCollectionType ) )
// InternalContextMappingDSL.g:12939:6: (lv_collectionType_2_0= ruleCollectionType )
{
// InternalContextMappingDSL.g:12939:6: (lv_collectionType_2_0= ruleCollectionType )
// InternalContextMappingDSL.g:12940:7: lv_collectionType_2_0= ruleCollectionType
{
newCompositeNode(grammarAccess.getAttributeAccess().getCollectionTypeCollectionTypeEnumRuleCall_2_0_0_0());
pushFollow(FOLLOW_126);
lv_collectionType_2_0=ruleCollectionType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getAttributeRule());
}
set(
current,
"collectionType",
lv_collectionType_2_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.CollectionType");
afterParserOrEnumRuleCall();
}
}
otherlv_3=(Token)match(input,118,FOLLOW_172);
newLeafNode(otherlv_3, grammarAccess.getAttributeAccess().getLessThanSignKeyword_2_0_1());
// InternalContextMappingDSL.g:12961:5: ( (lv_type_4_0= ruleType ) )
// InternalContextMappingDSL.g:12962:6: (lv_type_4_0= ruleType )
{
// InternalContextMappingDSL.g:12962:6: (lv_type_4_0= ruleType )
// InternalContextMappingDSL.g:12963:7: lv_type_4_0= ruleType
{
newCompositeNode(grammarAccess.getAttributeAccess().getTypeTypeParserRuleCall_2_0_2_0());
pushFollow(FOLLOW_128);
lv_type_4_0=ruleType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getAttributeRule());
}
set(
current,
"type",
lv_type_4_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Type");
afterParserOrEnumRuleCall();
}
}
otherlv_5=(Token)match(input,119,FOLLOW_10);
newLeafNode(otherlv_5, grammarAccess.getAttributeAccess().getGreaterThanSignKeyword_2_0_3());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:12986:4: ( (lv_type_6_0= ruleType ) )
{
// InternalContextMappingDSL.g:12986:4: ( (lv_type_6_0= ruleType ) )
// InternalContextMappingDSL.g:12987:5: (lv_type_6_0= ruleType )
{
// InternalContextMappingDSL.g:12987:5: (lv_type_6_0= ruleType )
// InternalContextMappingDSL.g:12988:6: lv_type_6_0= ruleType
{
newCompositeNode(grammarAccess.getAttributeAccess().getTypeTypeParserRuleCall_2_1_0());
pushFollow(FOLLOW_10);
lv_type_6_0=ruleType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getAttributeRule());
}
set(
current,
"type",
lv_type_6_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Type");
afterParserOrEnumRuleCall();
}
}
}
break;
}
// InternalContextMappingDSL.g:13006:3: ( (lv_name_7_0= RULE_ID ) )
// InternalContextMappingDSL.g:13007:4: (lv_name_7_0= RULE_ID )
{
// InternalContextMappingDSL.g:13007:4: (lv_name_7_0= RULE_ID )
// InternalContextMappingDSL.g:13008:5: lv_name_7_0= RULE_ID
{
lv_name_7_0=(Token)match(input,RULE_ID,FOLLOW_173);
newLeafNode(lv_name_7_0, grammarAccess.getAttributeAccess().getNameIDTerminalRuleCall_3_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"name",
lv_name_7_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:13024:3: ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_9_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_index_21_0= 'index' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_85_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:13025:4: ( ( ( ({...}? => ( ({...}? => ( (lv_key_9_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_index_21_0= 'index' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_85_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:13025:4: ( ( ( ({...}? => ( ({...}? => ( (lv_key_9_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_index_21_0= 'index' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_85_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:13026:5: ( ( ({...}? => ( ({...}? => ( (lv_key_9_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_index_21_0= 'index' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_85_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
// InternalContextMappingDSL.g:13029:5: ( ( ({...}? => ( ({...}? => ( (lv_key_9_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_index_21_0= 'index' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_85_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:13030:6: ( ({...}? => ( ({...}? => ( (lv_key_9_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_index_21_0= 'index' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_85_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:13030:6: ( ({...}? => ( ({...}? => ( (lv_key_9_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_index_21_0= 'index' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_85_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) ) ) ) )*
loop335:
do {
int alt335=30;
alt335 = dfa335.predict(input);
switch (alt335) {
case 1 :
// InternalContextMappingDSL.g:13031:4: ({...}? => ( ({...}? => ( (lv_key_9_0= 'key' ) ) ) ) )
{
// InternalContextMappingDSL.g:13031:4: ({...}? => ( ({...}? => ( (lv_key_9_0= 'key' ) ) ) ) )
// InternalContextMappingDSL.g:13032:5: {...}? => ( ({...}? => ( (lv_key_9_0= 'key' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 0) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 0)");
}
// InternalContextMappingDSL.g:13032:106: ( ({...}? => ( (lv_key_9_0= 'key' ) ) ) )
// InternalContextMappingDSL.g:13033:6: ({...}? => ( (lv_key_9_0= 'key' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 0);
// InternalContextMappingDSL.g:13036:9: ({...}? => ( (lv_key_9_0= 'key' ) ) )
// InternalContextMappingDSL.g:13036:10: {...}? => ( (lv_key_9_0= 'key' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13036:19: ( (lv_key_9_0= 'key' ) )
// InternalContextMappingDSL.g:13036:20: (lv_key_9_0= 'key' )
{
// InternalContextMappingDSL.g:13036:20: (lv_key_9_0= 'key' )
// InternalContextMappingDSL.g:13037:10: lv_key_9_0= 'key'
{
lv_key_9_0=(Token)match(input,146,FOLLOW_173);
newLeafNode(lv_key_9_0, grammarAccess.getAttributeAccess().getKeyKeyKeyword_4_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(current, "key", true, "key");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:13054:4: ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) ) ) )
{
// InternalContextMappingDSL.g:13054:4: ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) ) ) )
// InternalContextMappingDSL.g:13055:5: {...}? => ( ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 1) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 1)");
}
// InternalContextMappingDSL.g:13055:106: ( ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) ) )
// InternalContextMappingDSL.g:13056:6: ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 1);
// InternalContextMappingDSL.g:13059:9: ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) )
// InternalContextMappingDSL.g:13059:10: {...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13059:19: ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' )
int alt323=2;
int LA323_0 = input.LA(1);
if ( (LA323_0==RULE_NOT) ) {
alt323=1;
}
else if ( (LA323_0==147) ) {
alt323=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 323, 0, input);
throw nvae;
}
switch (alt323) {
case 1 :
// InternalContextMappingDSL.g:13059:20: ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' )
{
// InternalContextMappingDSL.g:13059:20: ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' )
// InternalContextMappingDSL.g:13060:10: ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable'
{
// InternalContextMappingDSL.g:13060:10: ( (lv_notChangeable_10_0= RULE_NOT ) )
// InternalContextMappingDSL.g:13061:11: (lv_notChangeable_10_0= RULE_NOT )
{
// InternalContextMappingDSL.g:13061:11: (lv_notChangeable_10_0= RULE_NOT )
// InternalContextMappingDSL.g:13062:12: lv_notChangeable_10_0= RULE_NOT
{
lv_notChangeable_10_0=(Token)match(input,RULE_NOT,FOLLOW_174);
newLeafNode(lv_notChangeable_10_0, grammarAccess.getAttributeAccess().getNotChangeableNOTTerminalRuleCall_4_1_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"notChangeable",
true,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.NOT");
}
}
otherlv_11=(Token)match(input,147,FOLLOW_173);
newLeafNode(otherlv_11, grammarAccess.getAttributeAccess().getChangeableKeyword_4_1_0_1());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:13084:9: otherlv_12= 'changeable'
{
otherlv_12=(Token)match(input,147,FOLLOW_173);
newLeafNode(otherlv_12, grammarAccess.getAttributeAccess().getChangeableKeyword_4_1_1());
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:13094:4: ({...}? => ( ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) ) ) )
{
// InternalContextMappingDSL.g:13094:4: ({...}? => ( ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) ) ) )
// InternalContextMappingDSL.g:13095:5: {...}? => ( ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 2) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 2)");
}
// InternalContextMappingDSL.g:13095:106: ( ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) ) )
// InternalContextMappingDSL.g:13096:6: ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 2);
// InternalContextMappingDSL.g:13099:9: ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) )
// InternalContextMappingDSL.g:13099:10: {...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13099:19: ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) )
int alt324=2;
int LA324_0 = input.LA(1);
if ( (LA324_0==148) ) {
alt324=1;
}
else if ( (LA324_0==RULE_NOT) ) {
alt324=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 324, 0, input);
throw nvae;
}
switch (alt324) {
case 1 :
// InternalContextMappingDSL.g:13099:20: ( (lv_required_13_0= 'required' ) )
{
// InternalContextMappingDSL.g:13099:20: ( (lv_required_13_0= 'required' ) )
// InternalContextMappingDSL.g:13100:10: (lv_required_13_0= 'required' )
{
// InternalContextMappingDSL.g:13100:10: (lv_required_13_0= 'required' )
// InternalContextMappingDSL.g:13101:11: lv_required_13_0= 'required'
{
lv_required_13_0=(Token)match(input,148,FOLLOW_173);
newLeafNode(lv_required_13_0, grammarAccess.getAttributeAccess().getRequiredRequiredKeyword_4_2_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(current, "required", true, "required");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:13114:9: (this_NOT_14= RULE_NOT otherlv_15= 'required' )
{
// InternalContextMappingDSL.g:13114:9: (this_NOT_14= RULE_NOT otherlv_15= 'required' )
// InternalContextMappingDSL.g:13115:10: this_NOT_14= RULE_NOT otherlv_15= 'required'
{
this_NOT_14=(Token)match(input,RULE_NOT,FOLLOW_175);
newLeafNode(this_NOT_14, grammarAccess.getAttributeAccess().getNOTTerminalRuleCall_4_2_1_0());
otherlv_15=(Token)match(input,148,FOLLOW_173);
newLeafNode(otherlv_15, grammarAccess.getAttributeAccess().getRequiredKeyword_4_2_1_1());
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:13130:4: ({...}? => ( ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:13130:4: ({...}? => ( ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:13131:5: {...}? => ( ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 3) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 3)");
}
// InternalContextMappingDSL.g:13131:106: ( ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:13132:6: ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 3);
// InternalContextMappingDSL.g:13135:9: ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:13135:10: {...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13135:19: ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:13135:20: ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:13135:20: ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) )
int alt325=2;
int LA325_0 = input.LA(1);
if ( (LA325_0==149) ) {
alt325=1;
}
else if ( (LA325_0==RULE_NOT) ) {
alt325=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 325, 0, input);
throw nvae;
}
switch (alt325) {
case 1 :
// InternalContextMappingDSL.g:13136:10: ( (lv_nullable_16_0= 'nullable' ) )
{
// InternalContextMappingDSL.g:13136:10: ( (lv_nullable_16_0= 'nullable' ) )
// InternalContextMappingDSL.g:13137:11: (lv_nullable_16_0= 'nullable' )
{
// InternalContextMappingDSL.g:13137:11: (lv_nullable_16_0= 'nullable' )
// InternalContextMappingDSL.g:13138:12: lv_nullable_16_0= 'nullable'
{
lv_nullable_16_0=(Token)match(input,149,FOLLOW_176);
newLeafNode(lv_nullable_16_0, grammarAccess.getAttributeAccess().getNullableNullableKeyword_4_3_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(current, "nullable", true, "nullable");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:13151:10: (this_NOT_17= RULE_NOT otherlv_18= 'nullable' )
{
// InternalContextMappingDSL.g:13151:10: (this_NOT_17= RULE_NOT otherlv_18= 'nullable' )
// InternalContextMappingDSL.g:13152:11: this_NOT_17= RULE_NOT otherlv_18= 'nullable'
{
this_NOT_17=(Token)match(input,RULE_NOT,FOLLOW_177);
newLeafNode(this_NOT_17, grammarAccess.getAttributeAccess().getNOTTerminalRuleCall_4_3_0_1_0());
otherlv_18=(Token)match(input,149,FOLLOW_176);
newLeafNode(otherlv_18, grammarAccess.getAttributeAccess().getNullableKeyword_4_3_0_1_1());
}
}
break;
}
// InternalContextMappingDSL.g:13162:9: (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )?
int alt326=2;
int LA326_0 = input.LA(1);
if ( (LA326_0==21) ) {
alt326=1;
}
switch (alt326) {
case 1 :
// InternalContextMappingDSL.g:13163:10: otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) )
{
otherlv_19=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_19, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_3_1_0());
// InternalContextMappingDSL.g:13167:10: ( (lv_nullableMessage_20_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13168:11: (lv_nullableMessage_20_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13168:11: (lv_nullableMessage_20_0= RULE_STRING )
// InternalContextMappingDSL.g:13169:12: lv_nullableMessage_20_0= RULE_STRING
{
lv_nullableMessage_20_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_nullableMessage_20_0, grammarAccess.getAttributeAccess().getNullableMessageSTRINGTerminalRuleCall_4_3_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"nullableMessage",
lv_nullableMessage_20_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 5 :
// InternalContextMappingDSL.g:13192:4: ({...}? => ( ({...}? => ( (lv_index_21_0= 'index' ) ) ) ) )
{
// InternalContextMappingDSL.g:13192:4: ({...}? => ( ({...}? => ( (lv_index_21_0= 'index' ) ) ) ) )
// InternalContextMappingDSL.g:13193:5: {...}? => ( ({...}? => ( (lv_index_21_0= 'index' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 4) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 4)");
}
// InternalContextMappingDSL.g:13193:106: ( ({...}? => ( (lv_index_21_0= 'index' ) ) ) )
// InternalContextMappingDSL.g:13194:6: ({...}? => ( (lv_index_21_0= 'index' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 4);
// InternalContextMappingDSL.g:13197:9: ({...}? => ( (lv_index_21_0= 'index' ) ) )
// InternalContextMappingDSL.g:13197:10: {...}? => ( (lv_index_21_0= 'index' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13197:19: ( (lv_index_21_0= 'index' ) )
// InternalContextMappingDSL.g:13197:20: (lv_index_21_0= 'index' )
{
// InternalContextMappingDSL.g:13197:20: (lv_index_21_0= 'index' )
// InternalContextMappingDSL.g:13198:10: lv_index_21_0= 'index'
{
lv_index_21_0=(Token)match(input,150,FOLLOW_173);
newLeafNode(lv_index_21_0, grammarAccess.getAttributeAccess().getIndexIndexKeyword_4_4_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(current, "index", true, "index");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 6 :
// InternalContextMappingDSL.g:13215:4: ({...}? => ( ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:13215:4: ({...}? => ( ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:13216:5: {...}? => ( ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 5) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 5)");
}
// InternalContextMappingDSL.g:13216:106: ( ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:13217:6: ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 5);
// InternalContextMappingDSL.g:13220:9: ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:13220:10: {...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13220:19: ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:13220:20: ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:13220:20: ( (lv_assertFalse_22_0= 'assertFalse' ) )
// InternalContextMappingDSL.g:13221:10: (lv_assertFalse_22_0= 'assertFalse' )
{
// InternalContextMappingDSL.g:13221:10: (lv_assertFalse_22_0= 'assertFalse' )
// InternalContextMappingDSL.g:13222:11: lv_assertFalse_22_0= 'assertFalse'
{
lv_assertFalse_22_0=(Token)match(input,151,FOLLOW_176);
newLeafNode(lv_assertFalse_22_0, grammarAccess.getAttributeAccess().getAssertFalseAssertFalseKeyword_4_5_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(current, "assertFalse", true, "assertFalse");
}
}
// InternalContextMappingDSL.g:13234:9: (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )?
int alt327=2;
int LA327_0 = input.LA(1);
if ( (LA327_0==21) ) {
alt327=1;
}
switch (alt327) {
case 1 :
// InternalContextMappingDSL.g:13235:10: otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) )
{
otherlv_23=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_23, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_5_1_0());
// InternalContextMappingDSL.g:13239:10: ( (lv_assertFalseMessage_24_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13240:11: (lv_assertFalseMessage_24_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13240:11: (lv_assertFalseMessage_24_0= RULE_STRING )
// InternalContextMappingDSL.g:13241:12: lv_assertFalseMessage_24_0= RULE_STRING
{
lv_assertFalseMessage_24_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_assertFalseMessage_24_0, grammarAccess.getAttributeAccess().getAssertFalseMessageSTRINGTerminalRuleCall_4_5_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"assertFalseMessage",
lv_assertFalseMessage_24_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 7 :
// InternalContextMappingDSL.g:13264:4: ({...}? => ( ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:13264:4: ({...}? => ( ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:13265:5: {...}? => ( ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 6) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 6)");
}
// InternalContextMappingDSL.g:13265:106: ( ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:13266:6: ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 6);
// InternalContextMappingDSL.g:13269:9: ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:13269:10: {...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13269:19: ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:13269:20: ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:13269:20: ( (lv_assertTrue_25_0= 'assertTrue' ) )
// InternalContextMappingDSL.g:13270:10: (lv_assertTrue_25_0= 'assertTrue' )
{
// InternalContextMappingDSL.g:13270:10: (lv_assertTrue_25_0= 'assertTrue' )
// InternalContextMappingDSL.g:13271:11: lv_assertTrue_25_0= 'assertTrue'
{
lv_assertTrue_25_0=(Token)match(input,152,FOLLOW_176);
newLeafNode(lv_assertTrue_25_0, grammarAccess.getAttributeAccess().getAssertTrueAssertTrueKeyword_4_6_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(current, "assertTrue", true, "assertTrue");
}
}
// InternalContextMappingDSL.g:13283:9: (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )?
int alt328=2;
int LA328_0 = input.LA(1);
if ( (LA328_0==21) ) {
alt328=1;
}
switch (alt328) {
case 1 :
// InternalContextMappingDSL.g:13284:10: otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) )
{
otherlv_26=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_26, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_6_1_0());
// InternalContextMappingDSL.g:13288:10: ( (lv_assertTrueMessage_27_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13289:11: (lv_assertTrueMessage_27_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13289:11: (lv_assertTrueMessage_27_0= RULE_STRING )
// InternalContextMappingDSL.g:13290:12: lv_assertTrueMessage_27_0= RULE_STRING
{
lv_assertTrueMessage_27_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_assertTrueMessage_27_0, grammarAccess.getAttributeAccess().getAssertTrueMessageSTRINGTerminalRuleCall_4_6_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"assertTrueMessage",
lv_assertTrueMessage_27_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 8 :
// InternalContextMappingDSL.g:13313:4: ({...}? => ( ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:13313:4: ({...}? => ( ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:13314:5: {...}? => ( ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 7) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 7)");
}
// InternalContextMappingDSL.g:13314:106: ( ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:13315:6: ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 7);
// InternalContextMappingDSL.g:13318:9: ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:13318:10: {...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13318:19: (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:13318:20: otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) )
{
otherlv_28=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_28, grammarAccess.getAttributeAccess().getHintKeyword_4_7_0());
otherlv_29=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_29, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_7_1());
// InternalContextMappingDSL.g:13326:9: ( (lv_hint_30_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13327:10: (lv_hint_30_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13327:10: (lv_hint_30_0= RULE_STRING )
// InternalContextMappingDSL.g:13328:11: lv_hint_30_0= RULE_STRING
{
lv_hint_30_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_hint_30_0, grammarAccess.getAttributeAccess().getHintSTRINGTerminalRuleCall_4_7_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_30_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 9 :
// InternalContextMappingDSL.g:13350:4: ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:13350:4: ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:13351:5: {...}? => ( ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 8) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 8)");
}
// InternalContextMappingDSL.g:13351:106: ( ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:13352:6: ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 8);
// InternalContextMappingDSL.g:13355:9: ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:13355:10: {...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13355:19: ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:13355:20: ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:13355:20: ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) )
// InternalContextMappingDSL.g:13356:10: (lv_creditCardNumber_31_0= 'creditCardNumber' )
{
// InternalContextMappingDSL.g:13356:10: (lv_creditCardNumber_31_0= 'creditCardNumber' )
// InternalContextMappingDSL.g:13357:11: lv_creditCardNumber_31_0= 'creditCardNumber'
{
lv_creditCardNumber_31_0=(Token)match(input,153,FOLLOW_176);
newLeafNode(lv_creditCardNumber_31_0, grammarAccess.getAttributeAccess().getCreditCardNumberCreditCardNumberKeyword_4_8_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(current, "creditCardNumber", true, "creditCardNumber");
}
}
// InternalContextMappingDSL.g:13369:9: (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )?
int alt329=2;
int LA329_0 = input.LA(1);
if ( (LA329_0==21) ) {
alt329=1;
}
switch (alt329) {
case 1 :
// InternalContextMappingDSL.g:13370:10: otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) )
{
otherlv_32=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_32, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_8_1_0());
// InternalContextMappingDSL.g:13374:10: ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13375:11: (lv_creditCardNumberMessage_33_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13375:11: (lv_creditCardNumberMessage_33_0= RULE_STRING )
// InternalContextMappingDSL.g:13376:12: lv_creditCardNumberMessage_33_0= RULE_STRING
{
lv_creditCardNumberMessage_33_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_creditCardNumberMessage_33_0, grammarAccess.getAttributeAccess().getCreditCardNumberMessageSTRINGTerminalRuleCall_4_8_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"creditCardNumberMessage",
lv_creditCardNumberMessage_33_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 10 :
// InternalContextMappingDSL.g:13399:4: ({...}? => ( ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:13399:4: ({...}? => ( ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:13400:5: {...}? => ( ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 9) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 9)");
}
// InternalContextMappingDSL.g:13400:106: ( ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:13401:6: ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 9);
// InternalContextMappingDSL.g:13404:9: ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:13404:10: {...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13404:19: (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:13404:20: otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) )
{
otherlv_34=(Token)match(input,154,FOLLOW_89);
newLeafNode(otherlv_34, grammarAccess.getAttributeAccess().getDigitsKeyword_4_9_0());
otherlv_35=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_35, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_9_1());
// InternalContextMappingDSL.g:13412:9: ( (lv_digits_36_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13413:10: (lv_digits_36_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13413:10: (lv_digits_36_0= RULE_STRING )
// InternalContextMappingDSL.g:13414:11: lv_digits_36_0= RULE_STRING
{
lv_digits_36_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_digits_36_0, grammarAccess.getAttributeAccess().getDigitsSTRINGTerminalRuleCall_4_9_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"digits",
lv_digits_36_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 11 :
// InternalContextMappingDSL.g:13436:4: ({...}? => ( ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:13436:4: ({...}? => ( ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:13437:5: {...}? => ( ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 10) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 10)");
}
// InternalContextMappingDSL.g:13437:107: ( ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:13438:6: ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 10);
// InternalContextMappingDSL.g:13441:9: ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:13441:10: {...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13441:19: ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:13441:20: ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:13441:20: ( (lv_email_37_0= 'email' ) )
// InternalContextMappingDSL.g:13442:10: (lv_email_37_0= 'email' )
{
// InternalContextMappingDSL.g:13442:10: (lv_email_37_0= 'email' )
// InternalContextMappingDSL.g:13443:11: lv_email_37_0= 'email'
{
lv_email_37_0=(Token)match(input,155,FOLLOW_176);
newLeafNode(lv_email_37_0, grammarAccess.getAttributeAccess().getEmailEmailKeyword_4_10_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(current, "email", true, "email");
}
}
// InternalContextMappingDSL.g:13455:9: (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )?
int alt330=2;
int LA330_0 = input.LA(1);
if ( (LA330_0==21) ) {
alt330=1;
}
switch (alt330) {
case 1 :
// InternalContextMappingDSL.g:13456:10: otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) )
{
otherlv_38=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_38, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_10_1_0());
// InternalContextMappingDSL.g:13460:10: ( (lv_emailMessage_39_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13461:11: (lv_emailMessage_39_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13461:11: (lv_emailMessage_39_0= RULE_STRING )
// InternalContextMappingDSL.g:13462:12: lv_emailMessage_39_0= RULE_STRING
{
lv_emailMessage_39_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_emailMessage_39_0, grammarAccess.getAttributeAccess().getEmailMessageSTRINGTerminalRuleCall_4_10_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"emailMessage",
lv_emailMessage_39_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 12 :
// InternalContextMappingDSL.g:13485:4: ({...}? => ( ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:13485:4: ({...}? => ( ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:13486:5: {...}? => ( ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 11) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 11)");
}
// InternalContextMappingDSL.g:13486:107: ( ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:13487:6: ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 11);
// InternalContextMappingDSL.g:13490:9: ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:13490:10: {...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13490:19: ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:13490:20: ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:13490:20: ( (lv_future_40_0= 'future' ) )
// InternalContextMappingDSL.g:13491:10: (lv_future_40_0= 'future' )
{
// InternalContextMappingDSL.g:13491:10: (lv_future_40_0= 'future' )
// InternalContextMappingDSL.g:13492:11: lv_future_40_0= 'future'
{
lv_future_40_0=(Token)match(input,156,FOLLOW_176);
newLeafNode(lv_future_40_0, grammarAccess.getAttributeAccess().getFutureFutureKeyword_4_11_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(current, "future", true, "future");
}
}
// InternalContextMappingDSL.g:13504:9: (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )?
int alt331=2;
int LA331_0 = input.LA(1);
if ( (LA331_0==21) ) {
alt331=1;
}
switch (alt331) {
case 1 :
// InternalContextMappingDSL.g:13505:10: otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) )
{
otherlv_41=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_41, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_11_1_0());
// InternalContextMappingDSL.g:13509:10: ( (lv_futureMessage_42_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13510:11: (lv_futureMessage_42_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13510:11: (lv_futureMessage_42_0= RULE_STRING )
// InternalContextMappingDSL.g:13511:12: lv_futureMessage_42_0= RULE_STRING
{
lv_futureMessage_42_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_futureMessage_42_0, grammarAccess.getAttributeAccess().getFutureMessageSTRINGTerminalRuleCall_4_11_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"futureMessage",
lv_futureMessage_42_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 13 :
// InternalContextMappingDSL.g:13534:4: ({...}? => ( ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:13534:4: ({...}? => ( ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:13535:5: {...}? => ( ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 12) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 12)");
}
// InternalContextMappingDSL.g:13535:107: ( ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:13536:6: ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 12);
// InternalContextMappingDSL.g:13539:9: ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:13539:10: {...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13539:19: ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:13539:20: ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:13539:20: ( (lv_past_43_0= 'past' ) )
// InternalContextMappingDSL.g:13540:10: (lv_past_43_0= 'past' )
{
// InternalContextMappingDSL.g:13540:10: (lv_past_43_0= 'past' )
// InternalContextMappingDSL.g:13541:11: lv_past_43_0= 'past'
{
lv_past_43_0=(Token)match(input,157,FOLLOW_176);
newLeafNode(lv_past_43_0, grammarAccess.getAttributeAccess().getPastPastKeyword_4_12_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(current, "past", true, "past");
}
}
// InternalContextMappingDSL.g:13553:9: (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )?
int alt332=2;
int LA332_0 = input.LA(1);
if ( (LA332_0==21) ) {
alt332=1;
}
switch (alt332) {
case 1 :
// InternalContextMappingDSL.g:13554:10: otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) )
{
otherlv_44=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_44, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_12_1_0());
// InternalContextMappingDSL.g:13558:10: ( (lv_pastMessage_45_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13559:11: (lv_pastMessage_45_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13559:11: (lv_pastMessage_45_0= RULE_STRING )
// InternalContextMappingDSL.g:13560:12: lv_pastMessage_45_0= RULE_STRING
{
lv_pastMessage_45_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_pastMessage_45_0, grammarAccess.getAttributeAccess().getPastMessageSTRINGTerminalRuleCall_4_12_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"pastMessage",
lv_pastMessage_45_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 14 :
// InternalContextMappingDSL.g:13583:4: ({...}? => ( ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:13583:4: ({...}? => ( ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:13584:5: {...}? => ( ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 13) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 13)");
}
// InternalContextMappingDSL.g:13584:107: ( ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:13585:6: ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 13);
// InternalContextMappingDSL.g:13588:9: ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:13588:10: {...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13588:19: (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:13588:20: otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) )
{
otherlv_46=(Token)match(input,158,FOLLOW_89);
newLeafNode(otherlv_46, grammarAccess.getAttributeAccess().getMaxKeyword_4_13_0());
otherlv_47=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_47, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_13_1());
// InternalContextMappingDSL.g:13596:9: ( (lv_max_48_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13597:10: (lv_max_48_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13597:10: (lv_max_48_0= RULE_STRING )
// InternalContextMappingDSL.g:13598:11: lv_max_48_0= RULE_STRING
{
lv_max_48_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_max_48_0, grammarAccess.getAttributeAccess().getMaxSTRINGTerminalRuleCall_4_13_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"max",
lv_max_48_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 15 :
// InternalContextMappingDSL.g:13620:4: ({...}? => ( ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:13620:4: ({...}? => ( ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:13621:5: {...}? => ( ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 14) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 14)");
}
// InternalContextMappingDSL.g:13621:107: ( ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:13622:6: ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 14);
// InternalContextMappingDSL.g:13625:9: ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:13625:10: {...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13625:19: (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:13625:20: otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) )
{
otherlv_49=(Token)match(input,159,FOLLOW_89);
newLeafNode(otherlv_49, grammarAccess.getAttributeAccess().getMinKeyword_4_14_0());
otherlv_50=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_50, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_14_1());
// InternalContextMappingDSL.g:13633:9: ( (lv_min_51_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13634:10: (lv_min_51_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13634:10: (lv_min_51_0= RULE_STRING )
// InternalContextMappingDSL.g:13635:11: lv_min_51_0= RULE_STRING
{
lv_min_51_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_min_51_0, grammarAccess.getAttributeAccess().getMinSTRINGTerminalRuleCall_4_14_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"min",
lv_min_51_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 16 :
// InternalContextMappingDSL.g:13657:4: ({...}? => ( ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:13657:4: ({...}? => ( ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:13658:5: {...}? => ( ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 15) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 15)");
}
// InternalContextMappingDSL.g:13658:107: ( ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:13659:6: ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 15);
// InternalContextMappingDSL.g:13662:9: ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:13662:10: {...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13662:19: (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:13662:20: otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) )
{
otherlv_52=(Token)match(input,160,FOLLOW_89);
newLeafNode(otherlv_52, grammarAccess.getAttributeAccess().getDecimalMaxKeyword_4_15_0());
otherlv_53=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_53, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_15_1());
// InternalContextMappingDSL.g:13670:9: ( (lv_decimalMax_54_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13671:10: (lv_decimalMax_54_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13671:10: (lv_decimalMax_54_0= RULE_STRING )
// InternalContextMappingDSL.g:13672:11: lv_decimalMax_54_0= RULE_STRING
{
lv_decimalMax_54_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_decimalMax_54_0, grammarAccess.getAttributeAccess().getDecimalMaxSTRINGTerminalRuleCall_4_15_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"decimalMax",
lv_decimalMax_54_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 17 :
// InternalContextMappingDSL.g:13694:4: ({...}? => ( ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:13694:4: ({...}? => ( ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:13695:5: {...}? => ( ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 16) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 16)");
}
// InternalContextMappingDSL.g:13695:107: ( ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:13696:6: ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 16);
// InternalContextMappingDSL.g:13699:9: ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:13699:10: {...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13699:19: (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:13699:20: otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) )
{
otherlv_55=(Token)match(input,161,FOLLOW_89);
newLeafNode(otherlv_55, grammarAccess.getAttributeAccess().getDecimalMinKeyword_4_16_0());
otherlv_56=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_56, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_16_1());
// InternalContextMappingDSL.g:13707:9: ( (lv_decimalMin_57_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13708:10: (lv_decimalMin_57_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13708:10: (lv_decimalMin_57_0= RULE_STRING )
// InternalContextMappingDSL.g:13709:11: lv_decimalMin_57_0= RULE_STRING
{
lv_decimalMin_57_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_decimalMin_57_0, grammarAccess.getAttributeAccess().getDecimalMinSTRINGTerminalRuleCall_4_16_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"decimalMin",
lv_decimalMin_57_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 18 :
// InternalContextMappingDSL.g:13731:4: ({...}? => ( ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:13731:4: ({...}? => ( ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:13732:5: {...}? => ( ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 17) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 17)");
}
// InternalContextMappingDSL.g:13732:107: ( ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:13733:6: ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 17);
// InternalContextMappingDSL.g:13736:9: ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:13736:10: {...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13736:19: ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:13736:20: ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:13736:20: ( (lv_notEmpty_58_0= 'notEmpty' ) )
// InternalContextMappingDSL.g:13737:10: (lv_notEmpty_58_0= 'notEmpty' )
{
// InternalContextMappingDSL.g:13737:10: (lv_notEmpty_58_0= 'notEmpty' )
// InternalContextMappingDSL.g:13738:11: lv_notEmpty_58_0= 'notEmpty'
{
lv_notEmpty_58_0=(Token)match(input,162,FOLLOW_176);
newLeafNode(lv_notEmpty_58_0, grammarAccess.getAttributeAccess().getNotEmptyNotEmptyKeyword_4_17_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(current, "notEmpty", true, "notEmpty");
}
}
// InternalContextMappingDSL.g:13750:9: (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )?
int alt333=2;
int LA333_0 = input.LA(1);
if ( (LA333_0==21) ) {
alt333=1;
}
switch (alt333) {
case 1 :
// InternalContextMappingDSL.g:13751:10: otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) )
{
otherlv_59=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_59, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_17_1_0());
// InternalContextMappingDSL.g:13755:10: ( (lv_notEmptyMessage_60_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13756:11: (lv_notEmptyMessage_60_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13756:11: (lv_notEmptyMessage_60_0= RULE_STRING )
// InternalContextMappingDSL.g:13757:12: lv_notEmptyMessage_60_0= RULE_STRING
{
lv_notEmptyMessage_60_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_notEmptyMessage_60_0, grammarAccess.getAttributeAccess().getNotEmptyMessageSTRINGTerminalRuleCall_4_17_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"notEmptyMessage",
lv_notEmptyMessage_60_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 19 :
// InternalContextMappingDSL.g:13780:4: ({...}? => ( ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:13780:4: ({...}? => ( ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:13781:5: {...}? => ( ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 18) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 18)");
}
// InternalContextMappingDSL.g:13781:107: ( ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:13782:6: ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 18);
// InternalContextMappingDSL.g:13785:9: ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:13785:10: {...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13785:19: ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:13785:20: ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:13785:20: ( (lv_notBlank_61_0= 'notBlank' ) )
// InternalContextMappingDSL.g:13786:10: (lv_notBlank_61_0= 'notBlank' )
{
// InternalContextMappingDSL.g:13786:10: (lv_notBlank_61_0= 'notBlank' )
// InternalContextMappingDSL.g:13787:11: lv_notBlank_61_0= 'notBlank'
{
lv_notBlank_61_0=(Token)match(input,163,FOLLOW_176);
newLeafNode(lv_notBlank_61_0, grammarAccess.getAttributeAccess().getNotBlankNotBlankKeyword_4_18_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(current, "notBlank", true, "notBlank");
}
}
// InternalContextMappingDSL.g:13799:9: (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )?
int alt334=2;
int LA334_0 = input.LA(1);
if ( (LA334_0==21) ) {
alt334=1;
}
switch (alt334) {
case 1 :
// InternalContextMappingDSL.g:13800:10: otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) )
{
otherlv_62=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_62, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_18_1_0());
// InternalContextMappingDSL.g:13804:10: ( (lv_notBlankMessage_63_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13805:11: (lv_notBlankMessage_63_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13805:11: (lv_notBlankMessage_63_0= RULE_STRING )
// InternalContextMappingDSL.g:13806:12: lv_notBlankMessage_63_0= RULE_STRING
{
lv_notBlankMessage_63_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_notBlankMessage_63_0, grammarAccess.getAttributeAccess().getNotBlankMessageSTRINGTerminalRuleCall_4_18_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"notBlankMessage",
lv_notBlankMessage_63_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 20 :
// InternalContextMappingDSL.g:13829:4: ({...}? => ( ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:13829:4: ({...}? => ( ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:13830:5: {...}? => ( ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 19) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 19)");
}
// InternalContextMappingDSL.g:13830:107: ( ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:13831:6: ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 19);
// InternalContextMappingDSL.g:13834:9: ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:13834:10: {...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13834:19: (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:13834:20: otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) )
{
otherlv_64=(Token)match(input,164,FOLLOW_89);
newLeafNode(otherlv_64, grammarAccess.getAttributeAccess().getPatternKeyword_4_19_0());
otherlv_65=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_65, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_19_1());
// InternalContextMappingDSL.g:13842:9: ( (lv_pattern_66_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13843:10: (lv_pattern_66_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13843:10: (lv_pattern_66_0= RULE_STRING )
// InternalContextMappingDSL.g:13844:11: lv_pattern_66_0= RULE_STRING
{
lv_pattern_66_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_pattern_66_0, grammarAccess.getAttributeAccess().getPatternSTRINGTerminalRuleCall_4_19_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"pattern",
lv_pattern_66_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 21 :
// InternalContextMappingDSL.g:13866:4: ({...}? => ( ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:13866:4: ({...}? => ( ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:13867:5: {...}? => ( ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 20) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 20)");
}
// InternalContextMappingDSL.g:13867:107: ( ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:13868:6: ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 20);
// InternalContextMappingDSL.g:13871:9: ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:13871:10: {...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13871:19: (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:13871:20: otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) )
{
otherlv_67=(Token)match(input,165,FOLLOW_89);
newLeafNode(otherlv_67, grammarAccess.getAttributeAccess().getRangeKeyword_4_20_0());
otherlv_68=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_68, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_20_1());
// InternalContextMappingDSL.g:13879:9: ( (lv_range_69_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13880:10: (lv_range_69_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13880:10: (lv_range_69_0= RULE_STRING )
// InternalContextMappingDSL.g:13881:11: lv_range_69_0= RULE_STRING
{
lv_range_69_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_range_69_0, grammarAccess.getAttributeAccess().getRangeSTRINGTerminalRuleCall_4_20_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"range",
lv_range_69_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 22 :
// InternalContextMappingDSL.g:13903:4: ({...}? => ( ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:13903:4: ({...}? => ( ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:13904:5: {...}? => ( ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 21) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 21)");
}
// InternalContextMappingDSL.g:13904:107: ( ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:13905:6: ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 21);
// InternalContextMappingDSL.g:13908:9: ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:13908:10: {...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13908:19: (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:13908:20: otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) )
{
otherlv_70=(Token)match(input,166,FOLLOW_89);
newLeafNode(otherlv_70, grammarAccess.getAttributeAccess().getSizeKeyword_4_21_0());
otherlv_71=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_71, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_21_1());
// InternalContextMappingDSL.g:13916:9: ( (lv_size_72_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13917:10: (lv_size_72_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13917:10: (lv_size_72_0= RULE_STRING )
// InternalContextMappingDSL.g:13918:11: lv_size_72_0= RULE_STRING
{
lv_size_72_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_size_72_0, grammarAccess.getAttributeAccess().getSizeSTRINGTerminalRuleCall_4_21_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"size",
lv_size_72_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 23 :
// InternalContextMappingDSL.g:13940:4: ({...}? => ( ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:13940:4: ({...}? => ( ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:13941:5: {...}? => ( ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 22) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 22)");
}
// InternalContextMappingDSL.g:13941:107: ( ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:13942:6: ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 22);
// InternalContextMappingDSL.g:13945:9: ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:13945:10: {...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13945:19: (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:13945:20: otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) )
{
otherlv_73=(Token)match(input,167,FOLLOW_89);
newLeafNode(otherlv_73, grammarAccess.getAttributeAccess().getLengthKeyword_4_22_0());
otherlv_74=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_74, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_22_1());
// InternalContextMappingDSL.g:13953:9: ( (lv_length_75_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13954:10: (lv_length_75_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13954:10: (lv_length_75_0= RULE_STRING )
// InternalContextMappingDSL.g:13955:11: lv_length_75_0= RULE_STRING
{
lv_length_75_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_length_75_0, grammarAccess.getAttributeAccess().getLengthSTRINGTerminalRuleCall_4_22_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"length",
lv_length_75_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 24 :
// InternalContextMappingDSL.g:13977:4: ({...}? => ( ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:13977:4: ({...}? => ( ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:13978:5: {...}? => ( ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 23) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 23)");
}
// InternalContextMappingDSL.g:13978:107: ( ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:13979:6: ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 23);
// InternalContextMappingDSL.g:13982:9: ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:13982:10: {...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:13982:19: (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:13982:20: otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) )
{
otherlv_76=(Token)match(input,168,FOLLOW_89);
newLeafNode(otherlv_76, grammarAccess.getAttributeAccess().getScriptAssertKeyword_4_23_0());
otherlv_77=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_77, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_23_1());
// InternalContextMappingDSL.g:13990:9: ( (lv_scriptAssert_78_0= RULE_STRING ) )
// InternalContextMappingDSL.g:13991:10: (lv_scriptAssert_78_0= RULE_STRING )
{
// InternalContextMappingDSL.g:13991:10: (lv_scriptAssert_78_0= RULE_STRING )
// InternalContextMappingDSL.g:13992:11: lv_scriptAssert_78_0= RULE_STRING
{
lv_scriptAssert_78_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_scriptAssert_78_0, grammarAccess.getAttributeAccess().getScriptAssertSTRINGTerminalRuleCall_4_23_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"scriptAssert",
lv_scriptAssert_78_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 25 :
// InternalContextMappingDSL.g:14014:4: ({...}? => ( ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:14014:4: ({...}? => ( ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:14015:5: {...}? => ( ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 24) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 24)");
}
// InternalContextMappingDSL.g:14015:107: ( ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:14016:6: ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 24);
// InternalContextMappingDSL.g:14019:9: ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:14019:10: {...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:14019:19: (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:14019:20: otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) )
{
otherlv_79=(Token)match(input,169,FOLLOW_89);
newLeafNode(otherlv_79, grammarAccess.getAttributeAccess().getUrlKeyword_4_24_0());
otherlv_80=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_80, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_24_1());
// InternalContextMappingDSL.g:14027:9: ( (lv_url_81_0= RULE_STRING ) )
// InternalContextMappingDSL.g:14028:10: (lv_url_81_0= RULE_STRING )
{
// InternalContextMappingDSL.g:14028:10: (lv_url_81_0= RULE_STRING )
// InternalContextMappingDSL.g:14029:11: lv_url_81_0= RULE_STRING
{
lv_url_81_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_url_81_0, grammarAccess.getAttributeAccess().getUrlSTRINGTerminalRuleCall_4_24_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"url",
lv_url_81_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 26 :
// InternalContextMappingDSL.g:14051:4: ({...}? => ( ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:14051:4: ({...}? => ( ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:14052:5: {...}? => ( ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 25) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 25)");
}
// InternalContextMappingDSL.g:14052:107: ( ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:14053:6: ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 25);
// InternalContextMappingDSL.g:14056:9: ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:14056:10: {...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:14056:19: (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:14056:20: otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) )
{
otherlv_82=(Token)match(input,133,FOLLOW_89);
newLeafNode(otherlv_82, grammarAccess.getAttributeAccess().getValidateKeyword_4_25_0());
otherlv_83=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_83, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_25_1());
// InternalContextMappingDSL.g:14064:9: ( (lv_validate_84_0= RULE_STRING ) )
// InternalContextMappingDSL.g:14065:10: (lv_validate_84_0= RULE_STRING )
{
// InternalContextMappingDSL.g:14065:10: (lv_validate_84_0= RULE_STRING )
// InternalContextMappingDSL.g:14066:11: lv_validate_84_0= RULE_STRING
{
lv_validate_84_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_validate_84_0, grammarAccess.getAttributeAccess().getValidateSTRINGTerminalRuleCall_4_25_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"validate",
lv_validate_84_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 27 :
// InternalContextMappingDSL.g:14088:4: ({...}? => ( ({...}? => ( (lv_transient_85_0= 'transient' ) ) ) ) )
{
// InternalContextMappingDSL.g:14088:4: ({...}? => ( ({...}? => ( (lv_transient_85_0= 'transient' ) ) ) ) )
// InternalContextMappingDSL.g:14089:5: {...}? => ( ({...}? => ( (lv_transient_85_0= 'transient' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 26) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 26)");
}
// InternalContextMappingDSL.g:14089:107: ( ({...}? => ( (lv_transient_85_0= 'transient' ) ) ) )
// InternalContextMappingDSL.g:14090:6: ({...}? => ( (lv_transient_85_0= 'transient' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 26);
// InternalContextMappingDSL.g:14093:9: ({...}? => ( (lv_transient_85_0= 'transient' ) ) )
// InternalContextMappingDSL.g:14093:10: {...}? => ( (lv_transient_85_0= 'transient' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:14093:19: ( (lv_transient_85_0= 'transient' ) )
// InternalContextMappingDSL.g:14093:20: (lv_transient_85_0= 'transient' )
{
// InternalContextMappingDSL.g:14093:20: (lv_transient_85_0= 'transient' )
// InternalContextMappingDSL.g:14094:10: lv_transient_85_0= 'transient'
{
lv_transient_85_0=(Token)match(input,170,FOLLOW_173);
newLeafNode(lv_transient_85_0, grammarAccess.getAttributeAccess().getTransientTransientKeyword_4_26_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(current, "transient", true, "transient");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 28 :
// InternalContextMappingDSL.g:14111:4: ({...}? => ( ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:14111:4: ({...}? => ( ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:14112:5: {...}? => ( ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 27) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 27)");
}
// InternalContextMappingDSL.g:14112:107: ( ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:14113:6: ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 27);
// InternalContextMappingDSL.g:14116:9: ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:14116:10: {...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:14116:19: (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:14116:20: otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) )
{
otherlv_86=(Token)match(input,171,FOLLOW_89);
newLeafNode(otherlv_86, grammarAccess.getAttributeAccess().getDatabaseColumnKeyword_4_27_0());
otherlv_87=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_87, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_27_1());
// InternalContextMappingDSL.g:14124:9: ( (lv_databaseColumn_88_0= RULE_STRING ) )
// InternalContextMappingDSL.g:14125:10: (lv_databaseColumn_88_0= RULE_STRING )
{
// InternalContextMappingDSL.g:14125:10: (lv_databaseColumn_88_0= RULE_STRING )
// InternalContextMappingDSL.g:14126:11: lv_databaseColumn_88_0= RULE_STRING
{
lv_databaseColumn_88_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_databaseColumn_88_0, grammarAccess.getAttributeAccess().getDatabaseColumnSTRINGTerminalRuleCall_4_27_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"databaseColumn",
lv_databaseColumn_88_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
case 29 :
// InternalContextMappingDSL.g:14148:4: ({...}? => ( ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:14148:4: ({...}? => ( ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:14149:5: {...}? => ( ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 28) ) {
throw new FailedPredicateException(input, "ruleAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 28)");
}
// InternalContextMappingDSL.g:14149:107: ( ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:14150:6: ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 28);
// InternalContextMappingDSL.g:14153:9: ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:14153:10: {...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleAttribute", "true");
}
// InternalContextMappingDSL.g:14153:19: (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:14153:20: otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) )
{
otherlv_89=(Token)match(input,172,FOLLOW_89);
newLeafNode(otherlv_89, grammarAccess.getAttributeAccess().getDatabaseTypeKeyword_4_28_0());
otherlv_90=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_90, grammarAccess.getAttributeAccess().getEqualsSignKeyword_4_28_1());
// InternalContextMappingDSL.g:14161:9: ( (lv_databaseType_91_0= RULE_STRING ) )
// InternalContextMappingDSL.g:14162:10: (lv_databaseType_91_0= RULE_STRING )
{
// InternalContextMappingDSL.g:14162:10: (lv_databaseType_91_0= RULE_STRING )
// InternalContextMappingDSL.g:14163:11: lv_databaseType_91_0= RULE_STRING
{
lv_databaseType_91_0=(Token)match(input,RULE_STRING,FOLLOW_173);
newLeafNode(lv_databaseType_91_0, grammarAccess.getAttributeAccess().getDatabaseTypeSTRINGTerminalRuleCall_4_28_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getAttributeRule());
}
setWithLastConsumed(
current,
"databaseType",
lv_databaseType_91_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
}
}
break;
default :
break loop335;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getAttributeAccess().getUnorderedGroup_4());
}
// InternalContextMappingDSL.g:14192:3: (otherlv_92= ';' )?
int alt336=2;
int LA336_0 = input.LA(1);
if ( (LA336_0==105) ) {
alt336=1;
}
switch (alt336) {
case 1 :
// InternalContextMappingDSL.g:14193:4: otherlv_92= ';'
{
otherlv_92=(Token)match(input,105,FOLLOW_2);
newLeafNode(otherlv_92, grammarAccess.getAttributeAccess().getSemicolonKeyword_5());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleAttribute"
// $ANTLR start "entryRuleReference"
// InternalContextMappingDSL.g:14202:1: entryRuleReference returns [EObject current=null] : iv_ruleReference= ruleReference EOF ;
public final EObject entryRuleReference() throws RecognitionException {
EObject current = null;
EObject iv_ruleReference = null;
try {
// InternalContextMappingDSL.g:14202:50: (iv_ruleReference= ruleReference EOF )
// InternalContextMappingDSL.g:14203:2: iv_ruleReference= ruleReference EOF
{
newCompositeNode(grammarAccess.getReferenceRule());
pushFollow(FOLLOW_1);
iv_ruleReference=ruleReference();
state._fsp--;
current =iv_ruleReference;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleReference"
// $ANTLR start "ruleReference"
// InternalContextMappingDSL.g:14209:1: ruleReference returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? this_REF_1= RULE_REF ( (lv_visibility_2_0= ruleVisibility ) )? ( ( ( (lv_collectionType_3_0= ruleCollectionType ) ) otherlv_4= '<' ( (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) ) ) otherlv_7= '>' ) | ( (otherlv_8= '@' )? ( (otherlv_9= RULE_ID ) ) ) ) ( (lv_name_10_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_12_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_60_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) ) ) ) )* ) ) ) (otherlv_68= ';' )? ) ;
public final EObject ruleReference() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token this_REF_1=null;
Token otherlv_4=null;
Token otherlv_5=null;
Token otherlv_6=null;
Token otherlv_7=null;
Token otherlv_8=null;
Token otherlv_9=null;
Token lv_name_10_0=null;
Token lv_key_12_0=null;
Token lv_notChangeable_13_0=null;
Token otherlv_14=null;
Token otherlv_15=null;
Token lv_required_16_0=null;
Token this_NOT_17=null;
Token otherlv_18=null;
Token lv_nullable_19_0=null;
Token this_NOT_20=null;
Token otherlv_21=null;
Token otherlv_22=null;
Token lv_nullableMessage_23_0=null;
Token otherlv_24=null;
Token otherlv_25=null;
Token lv_hint_26_0=null;
Token otherlv_27=null;
Token otherlv_28=null;
Token lv_cascade_29_0=null;
Token otherlv_30=null;
Token otherlv_31=null;
Token lv_fetch_32_0=null;
Token lv_cache_33_0=null;
Token this_NOT_34=null;
Token otherlv_35=null;
Token lv_inverse_36_0=null;
Token this_NOT_37=null;
Token otherlv_38=null;
Token otherlv_39=null;
Token otherlv_40=null;
Token lv_databaseColumn_41_0=null;
Token otherlv_42=null;
Token otherlv_43=null;
Token lv_databaseJoinTable_44_0=null;
Token otherlv_45=null;
Token otherlv_46=null;
Token lv_databaseJoinColumn_47_0=null;
Token lv_notEmpty_48_0=null;
Token otherlv_49=null;
Token lv_notEmptyMessage_50_0=null;
Token otherlv_51=null;
Token otherlv_52=null;
Token lv_size_53_0=null;
Token lv_valid_54_0=null;
Token otherlv_55=null;
Token lv_validMessage_56_0=null;
Token otherlv_57=null;
Token otherlv_58=null;
Token lv_validate_59_0=null;
Token lv_transient_60_0=null;
Token otherlv_61=null;
Token otherlv_62=null;
Token lv_orderBy_63_0=null;
Token lv_orderColumn_64_0=null;
Token otherlv_65=null;
Token lv_orderColumnName_66_0=null;
Token otherlv_68=null;
Enumerator lv_visibility_2_0 = null;
Enumerator lv_collectionType_3_0 = null;
EObject lv_oppositeHolder_67_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:14215:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? this_REF_1= RULE_REF ( (lv_visibility_2_0= ruleVisibility ) )? ( ( ( (lv_collectionType_3_0= ruleCollectionType ) ) otherlv_4= '<' ( (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) ) ) otherlv_7= '>' ) | ( (otherlv_8= '@' )? ( (otherlv_9= RULE_ID ) ) ) ) ( (lv_name_10_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_12_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_60_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) ) ) ) )* ) ) ) (otherlv_68= ';' )? ) )
// InternalContextMappingDSL.g:14216:2: ( ( (lv_doc_0_0= RULE_STRING ) )? this_REF_1= RULE_REF ( (lv_visibility_2_0= ruleVisibility ) )? ( ( ( (lv_collectionType_3_0= ruleCollectionType ) ) otherlv_4= '<' ( (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) ) ) otherlv_7= '>' ) | ( (otherlv_8= '@' )? ( (otherlv_9= RULE_ID ) ) ) ) ( (lv_name_10_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_12_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_60_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) ) ) ) )* ) ) ) (otherlv_68= ';' )? )
{
// InternalContextMappingDSL.g:14216:2: ( ( (lv_doc_0_0= RULE_STRING ) )? this_REF_1= RULE_REF ( (lv_visibility_2_0= ruleVisibility ) )? ( ( ( (lv_collectionType_3_0= ruleCollectionType ) ) otherlv_4= '<' ( (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) ) ) otherlv_7= '>' ) | ( (otherlv_8= '@' )? ( (otherlv_9= RULE_ID ) ) ) ) ( (lv_name_10_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_12_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_60_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) ) ) ) )* ) ) ) (otherlv_68= ';' )? )
// InternalContextMappingDSL.g:14217:3: ( (lv_doc_0_0= RULE_STRING ) )? this_REF_1= RULE_REF ( (lv_visibility_2_0= ruleVisibility ) )? ( ( ( (lv_collectionType_3_0= ruleCollectionType ) ) otherlv_4= '<' ( (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) ) ) otherlv_7= '>' ) | ( (otherlv_8= '@' )? ( (otherlv_9= RULE_ID ) ) ) ) ( (lv_name_10_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_12_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_60_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) ) ) ) )* ) ) ) (otherlv_68= ';' )?
{
// InternalContextMappingDSL.g:14217:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt337=2;
int LA337_0 = input.LA(1);
if ( (LA337_0==RULE_STRING) ) {
alt337=1;
}
switch (alt337) {
case 1 :
// InternalContextMappingDSL.g:14218:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:14218:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:14219:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_178);
newLeafNode(lv_doc_0_0, grammarAccess.getReferenceAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
this_REF_1=(Token)match(input,RULE_REF,FOLLOW_179);
newLeafNode(this_REF_1, grammarAccess.getReferenceAccess().getREFTerminalRuleCall_1());
// InternalContextMappingDSL.g:14239:3: ( (lv_visibility_2_0= ruleVisibility ) )?
int alt338=2;
int LA338_0 = input.LA(1);
if ( (LA338_0==124||(LA338_0>=247 && LA338_0<=249)) ) {
alt338=1;
}
switch (alt338) {
case 1 :
// InternalContextMappingDSL.g:14240:4: (lv_visibility_2_0= ruleVisibility )
{
// InternalContextMappingDSL.g:14240:4: (lv_visibility_2_0= ruleVisibility )
// InternalContextMappingDSL.g:14241:5: lv_visibility_2_0= ruleVisibility
{
newCompositeNode(grammarAccess.getReferenceAccess().getVisibilityVisibilityEnumRuleCall_2_0());
pushFollow(FOLLOW_180);
lv_visibility_2_0=ruleVisibility();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getReferenceRule());
}
set(
current,
"visibility",
lv_visibility_2_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Visibility");
afterParserOrEnumRuleCall();
}
}
break;
}
// InternalContextMappingDSL.g:14258:3: ( ( ( (lv_collectionType_3_0= ruleCollectionType ) ) otherlv_4= '<' ( (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) ) ) otherlv_7= '>' ) | ( (otherlv_8= '@' )? ( (otherlv_9= RULE_ID ) ) ) )
int alt341=2;
int LA341_0 = input.LA(1);
if ( (LA341_0==233||(LA341_0>=243 && LA341_0<=246)) ) {
alt341=1;
}
else if ( (LA341_0==RULE_ID||LA341_0==94) ) {
alt341=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 341, 0, input);
throw nvae;
}
switch (alt341) {
case 1 :
// InternalContextMappingDSL.g:14259:4: ( ( (lv_collectionType_3_0= ruleCollectionType ) ) otherlv_4= '<' ( (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) ) ) otherlv_7= '>' )
{
// InternalContextMappingDSL.g:14259:4: ( ( (lv_collectionType_3_0= ruleCollectionType ) ) otherlv_4= '<' ( (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) ) ) otherlv_7= '>' )
// InternalContextMappingDSL.g:14260:5: ( (lv_collectionType_3_0= ruleCollectionType ) ) otherlv_4= '<' ( (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) ) ) otherlv_7= '>'
{
// InternalContextMappingDSL.g:14260:5: ( (lv_collectionType_3_0= ruleCollectionType ) )
// InternalContextMappingDSL.g:14261:6: (lv_collectionType_3_0= ruleCollectionType )
{
// InternalContextMappingDSL.g:14261:6: (lv_collectionType_3_0= ruleCollectionType )
// InternalContextMappingDSL.g:14262:7: lv_collectionType_3_0= ruleCollectionType
{
newCompositeNode(grammarAccess.getReferenceAccess().getCollectionTypeCollectionTypeEnumRuleCall_3_0_0_0());
pushFollow(FOLLOW_126);
lv_collectionType_3_0=ruleCollectionType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getReferenceRule());
}
set(
current,
"collectionType",
lv_collectionType_3_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.CollectionType");
afterParserOrEnumRuleCall();
}
}
otherlv_4=(Token)match(input,118,FOLLOW_105);
newLeafNode(otherlv_4, grammarAccess.getReferenceAccess().getLessThanSignKeyword_3_0_1());
// InternalContextMappingDSL.g:14283:5: ( (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) ) )
// InternalContextMappingDSL.g:14284:6: (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) )
{
// InternalContextMappingDSL.g:14284:6: (otherlv_5= '@' )?
int alt339=2;
int LA339_0 = input.LA(1);
if ( (LA339_0==94) ) {
alt339=1;
}
switch (alt339) {
case 1 :
// InternalContextMappingDSL.g:14285:7: otherlv_5= '@'
{
otherlv_5=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_5, grammarAccess.getReferenceAccess().getCommercialAtKeyword_3_0_2_0());
}
break;
}
// InternalContextMappingDSL.g:14290:6: ( (otherlv_6= RULE_ID ) )
// InternalContextMappingDSL.g:14291:7: (otherlv_6= RULE_ID )
{
// InternalContextMappingDSL.g:14291:7: (otherlv_6= RULE_ID )
// InternalContextMappingDSL.g:14292:8: otherlv_6= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
otherlv_6=(Token)match(input,RULE_ID,FOLLOW_128);
newLeafNode(otherlv_6, grammarAccess.getReferenceAccess().getDomainObjectTypeSimpleDomainObjectCrossReference_3_0_2_1_0());
}
}
}
otherlv_7=(Token)match(input,119,FOLLOW_10);
newLeafNode(otherlv_7, grammarAccess.getReferenceAccess().getGreaterThanSignKeyword_3_0_3());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:14310:4: ( (otherlv_8= '@' )? ( (otherlv_9= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:14310:4: ( (otherlv_8= '@' )? ( (otherlv_9= RULE_ID ) ) )
// InternalContextMappingDSL.g:14311:5: (otherlv_8= '@' )? ( (otherlv_9= RULE_ID ) )
{
// InternalContextMappingDSL.g:14311:5: (otherlv_8= '@' )?
int alt340=2;
int LA340_0 = input.LA(1);
if ( (LA340_0==94) ) {
alt340=1;
}
switch (alt340) {
case 1 :
// InternalContextMappingDSL.g:14312:6: otherlv_8= '@'
{
otherlv_8=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_8, grammarAccess.getReferenceAccess().getCommercialAtKeyword_3_1_0());
}
break;
}
// InternalContextMappingDSL.g:14317:5: ( (otherlv_9= RULE_ID ) )
// InternalContextMappingDSL.g:14318:6: (otherlv_9= RULE_ID )
{
// InternalContextMappingDSL.g:14318:6: (otherlv_9= RULE_ID )
// InternalContextMappingDSL.g:14319:7: otherlv_9= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
otherlv_9=(Token)match(input,RULE_ID,FOLLOW_10);
newLeafNode(otherlv_9, grammarAccess.getReferenceAccess().getDomainObjectTypeSimpleDomainObjectCrossReference_3_1_1_0());
}
}
}
}
break;
}
// InternalContextMappingDSL.g:14332:3: ( (lv_name_10_0= RULE_ID ) )
// InternalContextMappingDSL.g:14333:4: (lv_name_10_0= RULE_ID )
{
// InternalContextMappingDSL.g:14333:4: (lv_name_10_0= RULE_ID )
// InternalContextMappingDSL.g:14334:5: lv_name_10_0= RULE_ID
{
lv_name_10_0=(Token)match(input,RULE_ID,FOLLOW_181);
newLeafNode(lv_name_10_0, grammarAccess.getReferenceAccess().getNameIDTerminalRuleCall_4_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(
current,
"name",
lv_name_10_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:14350:3: ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_12_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_60_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:14351:4: ( ( ( ({...}? => ( ({...}? => ( (lv_key_12_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_60_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:14351:4: ( ( ( ({...}? => ( ({...}? => ( (lv_key_12_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_60_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:14352:5: ( ( ({...}? => ( ({...}? => ( (lv_key_12_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_60_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
// InternalContextMappingDSL.g:14355:5: ( ( ({...}? => ( ({...}? => ( (lv_key_12_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_60_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) ) ) ) )* )
// InternalContextMappingDSL.g:14356:6: ( ({...}? => ( ({...}? => ( (lv_key_12_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_60_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:14356:6: ( ({...}? => ( ({...}? => ( (lv_key_12_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_60_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) ) ) ) )*
loop351:
do {
int alt351=21;
alt351 = dfa351.predict(input);
switch (alt351) {
case 1 :
// InternalContextMappingDSL.g:14357:4: ({...}? => ( ({...}? => ( (lv_key_12_0= 'key' ) ) ) ) )
{
// InternalContextMappingDSL.g:14357:4: ({...}? => ( ({...}? => ( (lv_key_12_0= 'key' ) ) ) ) )
// InternalContextMappingDSL.g:14358:5: {...}? => ( ({...}? => ( (lv_key_12_0= 'key' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 0) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 0)");
}
// InternalContextMappingDSL.g:14358:106: ( ({...}? => ( (lv_key_12_0= 'key' ) ) ) )
// InternalContextMappingDSL.g:14359:6: ({...}? => ( (lv_key_12_0= 'key' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 0);
// InternalContextMappingDSL.g:14362:9: ({...}? => ( (lv_key_12_0= 'key' ) ) )
// InternalContextMappingDSL.g:14362:10: {...}? => ( (lv_key_12_0= 'key' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:14362:19: ( (lv_key_12_0= 'key' ) )
// InternalContextMappingDSL.g:14362:20: (lv_key_12_0= 'key' )
{
// InternalContextMappingDSL.g:14362:20: (lv_key_12_0= 'key' )
// InternalContextMappingDSL.g:14363:10: lv_key_12_0= 'key'
{
lv_key_12_0=(Token)match(input,146,FOLLOW_181);
newLeafNode(lv_key_12_0, grammarAccess.getReferenceAccess().getKeyKeyKeyword_5_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(current, "key", true, "key");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:14380:4: ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) ) ) )
{
// InternalContextMappingDSL.g:14380:4: ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) ) ) )
// InternalContextMappingDSL.g:14381:5: {...}? => ( ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 1) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 1)");
}
// InternalContextMappingDSL.g:14381:106: ( ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) ) )
// InternalContextMappingDSL.g:14382:6: ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 1);
// InternalContextMappingDSL.g:14385:9: ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) )
// InternalContextMappingDSL.g:14385:10: {...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:14385:19: ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' )
int alt342=2;
int LA342_0 = input.LA(1);
if ( (LA342_0==RULE_NOT) ) {
alt342=1;
}
else if ( (LA342_0==147) ) {
alt342=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 342, 0, input);
throw nvae;
}
switch (alt342) {
case 1 :
// InternalContextMappingDSL.g:14385:20: ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' )
{
// InternalContextMappingDSL.g:14385:20: ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' )
// InternalContextMappingDSL.g:14386:10: ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable'
{
// InternalContextMappingDSL.g:14386:10: ( (lv_notChangeable_13_0= RULE_NOT ) )
// InternalContextMappingDSL.g:14387:11: (lv_notChangeable_13_0= RULE_NOT )
{
// InternalContextMappingDSL.g:14387:11: (lv_notChangeable_13_0= RULE_NOT )
// InternalContextMappingDSL.g:14388:12: lv_notChangeable_13_0= RULE_NOT
{
lv_notChangeable_13_0=(Token)match(input,RULE_NOT,FOLLOW_174);
newLeafNode(lv_notChangeable_13_0, grammarAccess.getReferenceAccess().getNotChangeableNOTTerminalRuleCall_5_1_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(
current,
"notChangeable",
true,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.NOT");
}
}
otherlv_14=(Token)match(input,147,FOLLOW_181);
newLeafNode(otherlv_14, grammarAccess.getReferenceAccess().getChangeableKeyword_5_1_0_1());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:14410:9: otherlv_15= 'changeable'
{
otherlv_15=(Token)match(input,147,FOLLOW_181);
newLeafNode(otherlv_15, grammarAccess.getReferenceAccess().getChangeableKeyword_5_1_1());
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:14420:4: ({...}? => ( ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) ) ) )
{
// InternalContextMappingDSL.g:14420:4: ({...}? => ( ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) ) ) )
// InternalContextMappingDSL.g:14421:5: {...}? => ( ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 2) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 2)");
}
// InternalContextMappingDSL.g:14421:106: ( ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) ) )
// InternalContextMappingDSL.g:14422:6: ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 2);
// InternalContextMappingDSL.g:14425:9: ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) )
// InternalContextMappingDSL.g:14425:10: {...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:14425:19: ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) )
int alt343=2;
int LA343_0 = input.LA(1);
if ( (LA343_0==148) ) {
alt343=1;
}
else if ( (LA343_0==RULE_NOT) ) {
alt343=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 343, 0, input);
throw nvae;
}
switch (alt343) {
case 1 :
// InternalContextMappingDSL.g:14425:20: ( (lv_required_16_0= 'required' ) )
{
// InternalContextMappingDSL.g:14425:20: ( (lv_required_16_0= 'required' ) )
// InternalContextMappingDSL.g:14426:10: (lv_required_16_0= 'required' )
{
// InternalContextMappingDSL.g:14426:10: (lv_required_16_0= 'required' )
// InternalContextMappingDSL.g:14427:11: lv_required_16_0= 'required'
{
lv_required_16_0=(Token)match(input,148,FOLLOW_181);
newLeafNode(lv_required_16_0, grammarAccess.getReferenceAccess().getRequiredRequiredKeyword_5_2_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(current, "required", true, "required");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:14440:9: (this_NOT_17= RULE_NOT otherlv_18= 'required' )
{
// InternalContextMappingDSL.g:14440:9: (this_NOT_17= RULE_NOT otherlv_18= 'required' )
// InternalContextMappingDSL.g:14441:10: this_NOT_17= RULE_NOT otherlv_18= 'required'
{
this_NOT_17=(Token)match(input,RULE_NOT,FOLLOW_175);
newLeafNode(this_NOT_17, grammarAccess.getReferenceAccess().getNOTTerminalRuleCall_5_2_1_0());
otherlv_18=(Token)match(input,148,FOLLOW_181);
newLeafNode(otherlv_18, grammarAccess.getReferenceAccess().getRequiredKeyword_5_2_1_1());
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:14456:4: ({...}? => ( ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:14456:4: ({...}? => ( ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:14457:5: {...}? => ( ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 3) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 3)");
}
// InternalContextMappingDSL.g:14457:106: ( ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:14458:6: ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 3);
// InternalContextMappingDSL.g:14461:9: ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:14461:10: {...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:14461:19: ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:14461:20: ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:14461:20: ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) )
int alt344=2;
int LA344_0 = input.LA(1);
if ( (LA344_0==149) ) {
alt344=1;
}
else if ( (LA344_0==RULE_NOT) ) {
alt344=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 344, 0, input);
throw nvae;
}
switch (alt344) {
case 1 :
// InternalContextMappingDSL.g:14462:10: ( (lv_nullable_19_0= 'nullable' ) )
{
// InternalContextMappingDSL.g:14462:10: ( (lv_nullable_19_0= 'nullable' ) )
// InternalContextMappingDSL.g:14463:11: (lv_nullable_19_0= 'nullable' )
{
// InternalContextMappingDSL.g:14463:11: (lv_nullable_19_0= 'nullable' )
// InternalContextMappingDSL.g:14464:12: lv_nullable_19_0= 'nullable'
{
lv_nullable_19_0=(Token)match(input,149,FOLLOW_182);
newLeafNode(lv_nullable_19_0, grammarAccess.getReferenceAccess().getNullableNullableKeyword_5_3_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(current, "nullable", true, "nullable");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:14477:10: (this_NOT_20= RULE_NOT otherlv_21= 'nullable' )
{
// InternalContextMappingDSL.g:14477:10: (this_NOT_20= RULE_NOT otherlv_21= 'nullable' )
// InternalContextMappingDSL.g:14478:11: this_NOT_20= RULE_NOT otherlv_21= 'nullable'
{
this_NOT_20=(Token)match(input,RULE_NOT,FOLLOW_177);
newLeafNode(this_NOT_20, grammarAccess.getReferenceAccess().getNOTTerminalRuleCall_5_3_0_1_0());
otherlv_21=(Token)match(input,149,FOLLOW_182);
newLeafNode(otherlv_21, grammarAccess.getReferenceAccess().getNullableKeyword_5_3_0_1_1());
}
}
break;
}
// InternalContextMappingDSL.g:14488:9: (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )?
int alt345=2;
int LA345_0 = input.LA(1);
if ( (LA345_0==21) ) {
alt345=1;
}
switch (alt345) {
case 1 :
// InternalContextMappingDSL.g:14489:10: otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) )
{
otherlv_22=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_22, grammarAccess.getReferenceAccess().getEqualsSignKeyword_5_3_1_0());
// InternalContextMappingDSL.g:14493:10: ( (lv_nullableMessage_23_0= RULE_STRING ) )
// InternalContextMappingDSL.g:14494:11: (lv_nullableMessage_23_0= RULE_STRING )
{
// InternalContextMappingDSL.g:14494:11: (lv_nullableMessage_23_0= RULE_STRING )
// InternalContextMappingDSL.g:14495:12: lv_nullableMessage_23_0= RULE_STRING
{
lv_nullableMessage_23_0=(Token)match(input,RULE_STRING,FOLLOW_181);
newLeafNode(lv_nullableMessage_23_0, grammarAccess.getReferenceAccess().getNullableMessageSTRINGTerminalRuleCall_5_3_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(
current,
"nullableMessage",
lv_nullableMessage_23_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 5 :
// InternalContextMappingDSL.g:14518:4: ({...}? => ( ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:14518:4: ({...}? => ( ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:14519:5: {...}? => ( ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 4) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 4)");
}
// InternalContextMappingDSL.g:14519:106: ( ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:14520:6: ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 4);
// InternalContextMappingDSL.g:14523:9: ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:14523:10: {...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:14523:19: (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:14523:20: otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) )
{
otherlv_24=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_24, grammarAccess.getReferenceAccess().getHintKeyword_5_4_0());
otherlv_25=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_25, grammarAccess.getReferenceAccess().getEqualsSignKeyword_5_4_1());
// InternalContextMappingDSL.g:14531:9: ( (lv_hint_26_0= RULE_STRING ) )
// InternalContextMappingDSL.g:14532:10: (lv_hint_26_0= RULE_STRING )
{
// InternalContextMappingDSL.g:14532:10: (lv_hint_26_0= RULE_STRING )
// InternalContextMappingDSL.g:14533:11: lv_hint_26_0= RULE_STRING
{
lv_hint_26_0=(Token)match(input,RULE_STRING,FOLLOW_181);
newLeafNode(lv_hint_26_0, grammarAccess.getReferenceAccess().getHintSTRINGTerminalRuleCall_5_4_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_26_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 6 :
// InternalContextMappingDSL.g:14555:4: ({...}? => ( ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:14555:4: ({...}? => ( ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:14556:5: {...}? => ( ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 5) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 5)");
}
// InternalContextMappingDSL.g:14556:106: ( ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:14557:6: ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 5);
// InternalContextMappingDSL.g:14560:9: ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:14560:10: {...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:14560:19: (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:14560:20: otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) )
{
otherlv_27=(Token)match(input,173,FOLLOW_89);
newLeafNode(otherlv_27, grammarAccess.getReferenceAccess().getCascadeKeyword_5_5_0());
otherlv_28=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_28, grammarAccess.getReferenceAccess().getEqualsSignKeyword_5_5_1());
// InternalContextMappingDSL.g:14568:9: ( (lv_cascade_29_0= RULE_STRING ) )
// InternalContextMappingDSL.g:14569:10: (lv_cascade_29_0= RULE_STRING )
{
// InternalContextMappingDSL.g:14569:10: (lv_cascade_29_0= RULE_STRING )
// InternalContextMappingDSL.g:14570:11: lv_cascade_29_0= RULE_STRING
{
lv_cascade_29_0=(Token)match(input,RULE_STRING,FOLLOW_181);
newLeafNode(lv_cascade_29_0, grammarAccess.getReferenceAccess().getCascadeSTRINGTerminalRuleCall_5_5_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(
current,
"cascade",
lv_cascade_29_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 7 :
// InternalContextMappingDSL.g:14592:4: ({...}? => ( ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:14592:4: ({...}? => ( ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:14593:5: {...}? => ( ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 6) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 6)");
}
// InternalContextMappingDSL.g:14593:106: ( ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:14594:6: ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 6);
// InternalContextMappingDSL.g:14597:9: ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:14597:10: {...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:14597:19: (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:14597:20: otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) )
{
otherlv_30=(Token)match(input,174,FOLLOW_89);
newLeafNode(otherlv_30, grammarAccess.getReferenceAccess().getFetchKeyword_5_6_0());
otherlv_31=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_31, grammarAccess.getReferenceAccess().getEqualsSignKeyword_5_6_1());
// InternalContextMappingDSL.g:14605:9: ( (lv_fetch_32_0= RULE_STRING ) )
// InternalContextMappingDSL.g:14606:10: (lv_fetch_32_0= RULE_STRING )
{
// InternalContextMappingDSL.g:14606:10: (lv_fetch_32_0= RULE_STRING )
// InternalContextMappingDSL.g:14607:11: lv_fetch_32_0= RULE_STRING
{
lv_fetch_32_0=(Token)match(input,RULE_STRING,FOLLOW_181);
newLeafNode(lv_fetch_32_0, grammarAccess.getReferenceAccess().getFetchSTRINGTerminalRuleCall_5_6_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(
current,
"fetch",
lv_fetch_32_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 8 :
// InternalContextMappingDSL.g:14629:4: ({...}? => ( ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) ) ) )
{
// InternalContextMappingDSL.g:14629:4: ({...}? => ( ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) ) ) )
// InternalContextMappingDSL.g:14630:5: {...}? => ( ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 7) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 7)");
}
// InternalContextMappingDSL.g:14630:106: ( ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) ) )
// InternalContextMappingDSL.g:14631:6: ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 7);
// InternalContextMappingDSL.g:14634:9: ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) )
// InternalContextMappingDSL.g:14634:10: {...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:14634:19: ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) )
int alt346=2;
int LA346_0 = input.LA(1);
if ( (LA346_0==108) ) {
alt346=1;
}
else if ( (LA346_0==RULE_NOT) ) {
alt346=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 346, 0, input);
throw nvae;
}
switch (alt346) {
case 1 :
// InternalContextMappingDSL.g:14634:20: ( (lv_cache_33_0= 'cache' ) )
{
// InternalContextMappingDSL.g:14634:20: ( (lv_cache_33_0= 'cache' ) )
// InternalContextMappingDSL.g:14635:10: (lv_cache_33_0= 'cache' )
{
// InternalContextMappingDSL.g:14635:10: (lv_cache_33_0= 'cache' )
// InternalContextMappingDSL.g:14636:11: lv_cache_33_0= 'cache'
{
lv_cache_33_0=(Token)match(input,108,FOLLOW_181);
newLeafNode(lv_cache_33_0, grammarAccess.getReferenceAccess().getCacheCacheKeyword_5_7_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(current, "cache", true, "cache");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:14649:9: (this_NOT_34= RULE_NOT otherlv_35= 'cache' )
{
// InternalContextMappingDSL.g:14649:9: (this_NOT_34= RULE_NOT otherlv_35= 'cache' )
// InternalContextMappingDSL.g:14650:10: this_NOT_34= RULE_NOT otherlv_35= 'cache'
{
this_NOT_34=(Token)match(input,RULE_NOT,FOLLOW_138);
newLeafNode(this_NOT_34, grammarAccess.getReferenceAccess().getNOTTerminalRuleCall_5_7_1_0());
otherlv_35=(Token)match(input,108,FOLLOW_181);
newLeafNode(otherlv_35, grammarAccess.getReferenceAccess().getCacheKeyword_5_7_1_1());
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 9 :
// InternalContextMappingDSL.g:14665:4: ({...}? => ( ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) ) ) )
{
// InternalContextMappingDSL.g:14665:4: ({...}? => ( ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) ) ) )
// InternalContextMappingDSL.g:14666:5: {...}? => ( ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 8) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 8)");
}
// InternalContextMappingDSL.g:14666:106: ( ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) ) )
// InternalContextMappingDSL.g:14667:6: ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 8);
// InternalContextMappingDSL.g:14670:9: ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) )
// InternalContextMappingDSL.g:14670:10: {...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:14670:19: ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) )
int alt347=2;
int LA347_0 = input.LA(1);
if ( (LA347_0==175) ) {
alt347=1;
}
else if ( (LA347_0==RULE_NOT) ) {
alt347=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 347, 0, input);
throw nvae;
}
switch (alt347) {
case 1 :
// InternalContextMappingDSL.g:14670:20: ( (lv_inverse_36_0= 'inverse' ) )
{
// InternalContextMappingDSL.g:14670:20: ( (lv_inverse_36_0= 'inverse' ) )
// InternalContextMappingDSL.g:14671:10: (lv_inverse_36_0= 'inverse' )
{
// InternalContextMappingDSL.g:14671:10: (lv_inverse_36_0= 'inverse' )
// InternalContextMappingDSL.g:14672:11: lv_inverse_36_0= 'inverse'
{
lv_inverse_36_0=(Token)match(input,175,FOLLOW_181);
newLeafNode(lv_inverse_36_0, grammarAccess.getReferenceAccess().getInverseInverseKeyword_5_8_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(current, "inverse", true, "inverse");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:14685:9: (this_NOT_37= RULE_NOT otherlv_38= 'inverse' )
{
// InternalContextMappingDSL.g:14685:9: (this_NOT_37= RULE_NOT otherlv_38= 'inverse' )
// InternalContextMappingDSL.g:14686:10: this_NOT_37= RULE_NOT otherlv_38= 'inverse'
{
this_NOT_37=(Token)match(input,RULE_NOT,FOLLOW_183);
newLeafNode(this_NOT_37, grammarAccess.getReferenceAccess().getNOTTerminalRuleCall_5_8_1_0());
otherlv_38=(Token)match(input,175,FOLLOW_181);
newLeafNode(otherlv_38, grammarAccess.getReferenceAccess().getInverseKeyword_5_8_1_1());
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 10 :
// InternalContextMappingDSL.g:14701:4: ({...}? => ( ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:14701:4: ({...}? => ( ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:14702:5: {...}? => ( ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 9) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 9)");
}
// InternalContextMappingDSL.g:14702:106: ( ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:14703:6: ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 9);
// InternalContextMappingDSL.g:14706:9: ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:14706:10: {...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:14706:19: (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:14706:20: otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) )
{
otherlv_39=(Token)match(input,171,FOLLOW_89);
newLeafNode(otherlv_39, grammarAccess.getReferenceAccess().getDatabaseColumnKeyword_5_9_0());
otherlv_40=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_40, grammarAccess.getReferenceAccess().getEqualsSignKeyword_5_9_1());
// InternalContextMappingDSL.g:14714:9: ( (lv_databaseColumn_41_0= RULE_STRING ) )
// InternalContextMappingDSL.g:14715:10: (lv_databaseColumn_41_0= RULE_STRING )
{
// InternalContextMappingDSL.g:14715:10: (lv_databaseColumn_41_0= RULE_STRING )
// InternalContextMappingDSL.g:14716:11: lv_databaseColumn_41_0= RULE_STRING
{
lv_databaseColumn_41_0=(Token)match(input,RULE_STRING,FOLLOW_181);
newLeafNode(lv_databaseColumn_41_0, grammarAccess.getReferenceAccess().getDatabaseColumnSTRINGTerminalRuleCall_5_9_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(
current,
"databaseColumn",
lv_databaseColumn_41_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 11 :
// InternalContextMappingDSL.g:14738:4: ({...}? => ( ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:14738:4: ({...}? => ( ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:14739:5: {...}? => ( ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 10) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 10)");
}
// InternalContextMappingDSL.g:14739:107: ( ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:14740:6: ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 10);
// InternalContextMappingDSL.g:14743:9: ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:14743:10: {...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:14743:19: (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:14743:20: otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) )
{
otherlv_42=(Token)match(input,176,FOLLOW_89);
newLeafNode(otherlv_42, grammarAccess.getReferenceAccess().getDatabaseJoinTableKeyword_5_10_0());
otherlv_43=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_43, grammarAccess.getReferenceAccess().getEqualsSignKeyword_5_10_1());
// InternalContextMappingDSL.g:14751:9: ( (lv_databaseJoinTable_44_0= RULE_STRING ) )
// InternalContextMappingDSL.g:14752:10: (lv_databaseJoinTable_44_0= RULE_STRING )
{
// InternalContextMappingDSL.g:14752:10: (lv_databaseJoinTable_44_0= RULE_STRING )
// InternalContextMappingDSL.g:14753:11: lv_databaseJoinTable_44_0= RULE_STRING
{
lv_databaseJoinTable_44_0=(Token)match(input,RULE_STRING,FOLLOW_181);
newLeafNode(lv_databaseJoinTable_44_0, grammarAccess.getReferenceAccess().getDatabaseJoinTableSTRINGTerminalRuleCall_5_10_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(
current,
"databaseJoinTable",
lv_databaseJoinTable_44_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 12 :
// InternalContextMappingDSL.g:14775:4: ({...}? => ( ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:14775:4: ({...}? => ( ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:14776:5: {...}? => ( ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 11) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 11)");
}
// InternalContextMappingDSL.g:14776:107: ( ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:14777:6: ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 11);
// InternalContextMappingDSL.g:14780:9: ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:14780:10: {...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:14780:19: (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:14780:20: otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) )
{
otherlv_45=(Token)match(input,177,FOLLOW_89);
newLeafNode(otherlv_45, grammarAccess.getReferenceAccess().getDatabaseJoinColumnKeyword_5_11_0());
otherlv_46=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_46, grammarAccess.getReferenceAccess().getEqualsSignKeyword_5_11_1());
// InternalContextMappingDSL.g:14788:9: ( (lv_databaseJoinColumn_47_0= RULE_STRING ) )
// InternalContextMappingDSL.g:14789:10: (lv_databaseJoinColumn_47_0= RULE_STRING )
{
// InternalContextMappingDSL.g:14789:10: (lv_databaseJoinColumn_47_0= RULE_STRING )
// InternalContextMappingDSL.g:14790:11: lv_databaseJoinColumn_47_0= RULE_STRING
{
lv_databaseJoinColumn_47_0=(Token)match(input,RULE_STRING,FOLLOW_181);
newLeafNode(lv_databaseJoinColumn_47_0, grammarAccess.getReferenceAccess().getDatabaseJoinColumnSTRINGTerminalRuleCall_5_11_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(
current,
"databaseJoinColumn",
lv_databaseJoinColumn_47_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 13 :
// InternalContextMappingDSL.g:14812:4: ({...}? => ( ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:14812:4: ({...}? => ( ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:14813:5: {...}? => ( ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 12) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 12)");
}
// InternalContextMappingDSL.g:14813:107: ( ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:14814:6: ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 12);
// InternalContextMappingDSL.g:14817:9: ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:14817:10: {...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:14817:19: ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:14817:20: ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:14817:20: ( (lv_notEmpty_48_0= 'notEmpty' ) )
// InternalContextMappingDSL.g:14818:10: (lv_notEmpty_48_0= 'notEmpty' )
{
// InternalContextMappingDSL.g:14818:10: (lv_notEmpty_48_0= 'notEmpty' )
// InternalContextMappingDSL.g:14819:11: lv_notEmpty_48_0= 'notEmpty'
{
lv_notEmpty_48_0=(Token)match(input,162,FOLLOW_182);
newLeafNode(lv_notEmpty_48_0, grammarAccess.getReferenceAccess().getNotEmptyNotEmptyKeyword_5_12_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(current, "notEmpty", true, "notEmpty");
}
}
// InternalContextMappingDSL.g:14831:9: (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )?
int alt348=2;
int LA348_0 = input.LA(1);
if ( (LA348_0==21) ) {
alt348=1;
}
switch (alt348) {
case 1 :
// InternalContextMappingDSL.g:14832:10: otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) )
{
otherlv_49=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_49, grammarAccess.getReferenceAccess().getEqualsSignKeyword_5_12_1_0());
// InternalContextMappingDSL.g:14836:10: ( (lv_notEmptyMessage_50_0= RULE_STRING ) )
// InternalContextMappingDSL.g:14837:11: (lv_notEmptyMessage_50_0= RULE_STRING )
{
// InternalContextMappingDSL.g:14837:11: (lv_notEmptyMessage_50_0= RULE_STRING )
// InternalContextMappingDSL.g:14838:12: lv_notEmptyMessage_50_0= RULE_STRING
{
lv_notEmptyMessage_50_0=(Token)match(input,RULE_STRING,FOLLOW_181);
newLeafNode(lv_notEmptyMessage_50_0, grammarAccess.getReferenceAccess().getNotEmptyMessageSTRINGTerminalRuleCall_5_12_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(
current,
"notEmptyMessage",
lv_notEmptyMessage_50_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 14 :
// InternalContextMappingDSL.g:14861:4: ({...}? => ( ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:14861:4: ({...}? => ( ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:14862:5: {...}? => ( ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 13) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 13)");
}
// InternalContextMappingDSL.g:14862:107: ( ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:14863:6: ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 13);
// InternalContextMappingDSL.g:14866:9: ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:14866:10: {...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:14866:19: (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:14866:20: otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) )
{
otherlv_51=(Token)match(input,166,FOLLOW_89);
newLeafNode(otherlv_51, grammarAccess.getReferenceAccess().getSizeKeyword_5_13_0());
otherlv_52=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_52, grammarAccess.getReferenceAccess().getEqualsSignKeyword_5_13_1());
// InternalContextMappingDSL.g:14874:9: ( (lv_size_53_0= RULE_STRING ) )
// InternalContextMappingDSL.g:14875:10: (lv_size_53_0= RULE_STRING )
{
// InternalContextMappingDSL.g:14875:10: (lv_size_53_0= RULE_STRING )
// InternalContextMappingDSL.g:14876:11: lv_size_53_0= RULE_STRING
{
lv_size_53_0=(Token)match(input,RULE_STRING,FOLLOW_181);
newLeafNode(lv_size_53_0, grammarAccess.getReferenceAccess().getSizeSTRINGTerminalRuleCall_5_13_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(
current,
"size",
lv_size_53_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 15 :
// InternalContextMappingDSL.g:14898:4: ({...}? => ( ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:14898:4: ({...}? => ( ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:14899:5: {...}? => ( ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 14) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 14)");
}
// InternalContextMappingDSL.g:14899:107: ( ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:14900:6: ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 14);
// InternalContextMappingDSL.g:14903:9: ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:14903:10: {...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:14903:19: ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:14903:20: ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:14903:20: ( (lv_valid_54_0= 'valid' ) )
// InternalContextMappingDSL.g:14904:10: (lv_valid_54_0= 'valid' )
{
// InternalContextMappingDSL.g:14904:10: (lv_valid_54_0= 'valid' )
// InternalContextMappingDSL.g:14905:11: lv_valid_54_0= 'valid'
{
lv_valid_54_0=(Token)match(input,178,FOLLOW_182);
newLeafNode(lv_valid_54_0, grammarAccess.getReferenceAccess().getValidValidKeyword_5_14_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(current, "valid", true, "valid");
}
}
// InternalContextMappingDSL.g:14917:9: (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )?
int alt349=2;
int LA349_0 = input.LA(1);
if ( (LA349_0==21) ) {
alt349=1;
}
switch (alt349) {
case 1 :
// InternalContextMappingDSL.g:14918:10: otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) )
{
otherlv_55=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_55, grammarAccess.getReferenceAccess().getEqualsSignKeyword_5_14_1_0());
// InternalContextMappingDSL.g:14922:10: ( (lv_validMessage_56_0= RULE_STRING ) )
// InternalContextMappingDSL.g:14923:11: (lv_validMessage_56_0= RULE_STRING )
{
// InternalContextMappingDSL.g:14923:11: (lv_validMessage_56_0= RULE_STRING )
// InternalContextMappingDSL.g:14924:12: lv_validMessage_56_0= RULE_STRING
{
lv_validMessage_56_0=(Token)match(input,RULE_STRING,FOLLOW_181);
newLeafNode(lv_validMessage_56_0, grammarAccess.getReferenceAccess().getValidMessageSTRINGTerminalRuleCall_5_14_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(
current,
"validMessage",
lv_validMessage_56_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 16 :
// InternalContextMappingDSL.g:14947:4: ({...}? => ( ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:14947:4: ({...}? => ( ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:14948:5: {...}? => ( ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 15) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 15)");
}
// InternalContextMappingDSL.g:14948:107: ( ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:14949:6: ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 15);
// InternalContextMappingDSL.g:14952:9: ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:14952:10: {...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:14952:19: (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:14952:20: otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) )
{
otherlv_57=(Token)match(input,133,FOLLOW_89);
newLeafNode(otherlv_57, grammarAccess.getReferenceAccess().getValidateKeyword_5_15_0());
otherlv_58=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_58, grammarAccess.getReferenceAccess().getEqualsSignKeyword_5_15_1());
// InternalContextMappingDSL.g:14960:9: ( (lv_validate_59_0= RULE_STRING ) )
// InternalContextMappingDSL.g:14961:10: (lv_validate_59_0= RULE_STRING )
{
// InternalContextMappingDSL.g:14961:10: (lv_validate_59_0= RULE_STRING )
// InternalContextMappingDSL.g:14962:11: lv_validate_59_0= RULE_STRING
{
lv_validate_59_0=(Token)match(input,RULE_STRING,FOLLOW_181);
newLeafNode(lv_validate_59_0, grammarAccess.getReferenceAccess().getValidateSTRINGTerminalRuleCall_5_15_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(
current,
"validate",
lv_validate_59_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 17 :
// InternalContextMappingDSL.g:14984:4: ({...}? => ( ({...}? => ( (lv_transient_60_0= 'transient' ) ) ) ) )
{
// InternalContextMappingDSL.g:14984:4: ({...}? => ( ({...}? => ( (lv_transient_60_0= 'transient' ) ) ) ) )
// InternalContextMappingDSL.g:14985:5: {...}? => ( ({...}? => ( (lv_transient_60_0= 'transient' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 16) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 16)");
}
// InternalContextMappingDSL.g:14985:107: ( ({...}? => ( (lv_transient_60_0= 'transient' ) ) ) )
// InternalContextMappingDSL.g:14986:6: ({...}? => ( (lv_transient_60_0= 'transient' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 16);
// InternalContextMappingDSL.g:14989:9: ({...}? => ( (lv_transient_60_0= 'transient' ) ) )
// InternalContextMappingDSL.g:14989:10: {...}? => ( (lv_transient_60_0= 'transient' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:14989:19: ( (lv_transient_60_0= 'transient' ) )
// InternalContextMappingDSL.g:14989:20: (lv_transient_60_0= 'transient' )
{
// InternalContextMappingDSL.g:14989:20: (lv_transient_60_0= 'transient' )
// InternalContextMappingDSL.g:14990:10: lv_transient_60_0= 'transient'
{
lv_transient_60_0=(Token)match(input,170,FOLLOW_181);
newLeafNode(lv_transient_60_0, grammarAccess.getReferenceAccess().getTransientTransientKeyword_5_16_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(current, "transient", true, "transient");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 18 :
// InternalContextMappingDSL.g:15007:4: ({...}? => ( ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:15007:4: ({...}? => ( ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:15008:5: {...}? => ( ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 17) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 17)");
}
// InternalContextMappingDSL.g:15008:107: ( ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:15009:6: ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 17);
// InternalContextMappingDSL.g:15012:9: ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:15012:10: {...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:15012:19: (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:15012:20: otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) )
{
otherlv_61=(Token)match(input,179,FOLLOW_89);
newLeafNode(otherlv_61, grammarAccess.getReferenceAccess().getOrderbyKeyword_5_17_0());
otherlv_62=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_62, grammarAccess.getReferenceAccess().getEqualsSignKeyword_5_17_1());
// InternalContextMappingDSL.g:15020:9: ( (lv_orderBy_63_0= RULE_STRING ) )
// InternalContextMappingDSL.g:15021:10: (lv_orderBy_63_0= RULE_STRING )
{
// InternalContextMappingDSL.g:15021:10: (lv_orderBy_63_0= RULE_STRING )
// InternalContextMappingDSL.g:15022:11: lv_orderBy_63_0= RULE_STRING
{
lv_orderBy_63_0=(Token)match(input,RULE_STRING,FOLLOW_181);
newLeafNode(lv_orderBy_63_0, grammarAccess.getReferenceAccess().getOrderBySTRINGTerminalRuleCall_5_17_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(
current,
"orderBy",
lv_orderBy_63_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 19 :
// InternalContextMappingDSL.g:15044:4: ({...}? => ( ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:15044:4: ({...}? => ( ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:15045:5: {...}? => ( ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 18) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 18)");
}
// InternalContextMappingDSL.g:15045:107: ( ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:15046:6: ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 18);
// InternalContextMappingDSL.g:15049:9: ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:15049:10: {...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:15049:19: ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:15049:20: ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:15049:20: ( (lv_orderColumn_64_0= 'orderColumn' ) )
// InternalContextMappingDSL.g:15050:10: (lv_orderColumn_64_0= 'orderColumn' )
{
// InternalContextMappingDSL.g:15050:10: (lv_orderColumn_64_0= 'orderColumn' )
// InternalContextMappingDSL.g:15051:11: lv_orderColumn_64_0= 'orderColumn'
{
lv_orderColumn_64_0=(Token)match(input,180,FOLLOW_182);
newLeafNode(lv_orderColumn_64_0, grammarAccess.getReferenceAccess().getOrderColumnOrderColumnKeyword_5_18_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(current, "orderColumn", true, "orderColumn");
}
}
// InternalContextMappingDSL.g:15063:9: (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )?
int alt350=2;
int LA350_0 = input.LA(1);
if ( (LA350_0==21) ) {
alt350=1;
}
switch (alt350) {
case 1 :
// InternalContextMappingDSL.g:15064:10: otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) )
{
otherlv_65=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_65, grammarAccess.getReferenceAccess().getEqualsSignKeyword_5_18_1_0());
// InternalContextMappingDSL.g:15068:10: ( (lv_orderColumnName_66_0= RULE_STRING ) )
// InternalContextMappingDSL.g:15069:11: (lv_orderColumnName_66_0= RULE_STRING )
{
// InternalContextMappingDSL.g:15069:11: (lv_orderColumnName_66_0= RULE_STRING )
// InternalContextMappingDSL.g:15070:12: lv_orderColumnName_66_0= RULE_STRING
{
lv_orderColumnName_66_0=(Token)match(input,RULE_STRING,FOLLOW_181);
newLeafNode(lv_orderColumnName_66_0, grammarAccess.getReferenceAccess().getOrderColumnNameSTRINGTerminalRuleCall_5_18_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getReferenceRule());
}
setWithLastConsumed(
current,
"orderColumnName",
lv_orderColumnName_66_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
case 20 :
// InternalContextMappingDSL.g:15093:4: ({...}? => ( ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) ) ) )
{
// InternalContextMappingDSL.g:15093:4: ({...}? => ( ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) ) ) )
// InternalContextMappingDSL.g:15094:5: {...}? => ( ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 19) ) {
throw new FailedPredicateException(input, "ruleReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 19)");
}
// InternalContextMappingDSL.g:15094:107: ( ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) ) )
// InternalContextMappingDSL.g:15095:6: ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 19);
// InternalContextMappingDSL.g:15098:9: ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) )
// InternalContextMappingDSL.g:15098:10: {...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleReference", "true");
}
// InternalContextMappingDSL.g:15098:19: ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) )
// InternalContextMappingDSL.g:15098:20: (lv_oppositeHolder_67_0= ruleOppositeHolder )
{
// InternalContextMappingDSL.g:15098:20: (lv_oppositeHolder_67_0= ruleOppositeHolder )
// InternalContextMappingDSL.g:15099:10: lv_oppositeHolder_67_0= ruleOppositeHolder
{
newCompositeNode(grammarAccess.getReferenceAccess().getOppositeHolderOppositeHolderParserRuleCall_5_19_0());
pushFollow(FOLLOW_181);
lv_oppositeHolder_67_0=ruleOppositeHolder();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getReferenceRule());
}
set(
current,
"oppositeHolder",
lv_oppositeHolder_67_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.OppositeHolder");
afterParserOrEnumRuleCall();
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
}
}
break;
default :
break loop351;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getReferenceAccess().getUnorderedGroup_5());
}
// InternalContextMappingDSL.g:15128:3: (otherlv_68= ';' )?
int alt352=2;
int LA352_0 = input.LA(1);
if ( (LA352_0==105) ) {
alt352=1;
}
switch (alt352) {
case 1 :
// InternalContextMappingDSL.g:15129:4: otherlv_68= ';'
{
otherlv_68=(Token)match(input,105,FOLLOW_2);
newLeafNode(otherlv_68, grammarAccess.getReferenceAccess().getSemicolonKeyword_6());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleReference"
// $ANTLR start "entryRuleDtoAttribute"
// InternalContextMappingDSL.g:15138:1: entryRuleDtoAttribute returns [EObject current=null] : iv_ruleDtoAttribute= ruleDtoAttribute EOF ;
public final EObject entryRuleDtoAttribute() throws RecognitionException {
EObject current = null;
EObject iv_ruleDtoAttribute = null;
try {
// InternalContextMappingDSL.g:15138:53: (iv_ruleDtoAttribute= ruleDtoAttribute EOF )
// InternalContextMappingDSL.g:15139:2: iv_ruleDtoAttribute= ruleDtoAttribute EOF
{
newCompositeNode(grammarAccess.getDtoAttributeRule());
pushFollow(FOLLOW_1);
iv_ruleDtoAttribute=ruleDtoAttribute();
state._fsp--;
current =iv_ruleDtoAttribute;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleDtoAttribute"
// $ANTLR start "ruleDtoAttribute"
// InternalContextMappingDSL.g:15145:1: ruleDtoAttribute returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( (lv_collectionType_2_0= ruleCollectionType ) ) otherlv_3= '<' )? ( (lv_type_4_0= ruleType ) ) (otherlv_5= '>' )? ( (lv_name_6_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_8_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_20_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) (otherlv_84= ';' )? ) ;
public final EObject ruleDtoAttribute() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token otherlv_3=null;
Token otherlv_5=null;
Token lv_name_6_0=null;
Token lv_key_8_0=null;
Token lv_notChangeable_9_0=null;
Token otherlv_10=null;
Token otherlv_11=null;
Token lv_required_12_0=null;
Token this_NOT_13=null;
Token otherlv_14=null;
Token lv_nullable_15_0=null;
Token this_NOT_16=null;
Token otherlv_17=null;
Token otherlv_18=null;
Token lv_nullableMessage_19_0=null;
Token lv_transient_20_0=null;
Token lv_assertFalse_21_0=null;
Token otherlv_22=null;
Token lv_assertFalseMessage_23_0=null;
Token lv_assertTrue_24_0=null;
Token otherlv_25=null;
Token lv_assertTrueMessage_26_0=null;
Token lv_creditCardNumber_27_0=null;
Token otherlv_28=null;
Token lv_creditCardNumberMessage_29_0=null;
Token otherlv_30=null;
Token otherlv_31=null;
Token lv_digits_32_0=null;
Token lv_email_33_0=null;
Token otherlv_34=null;
Token lv_emailMessage_35_0=null;
Token lv_future_36_0=null;
Token otherlv_37=null;
Token lv_futureMessage_38_0=null;
Token lv_past_39_0=null;
Token otherlv_40=null;
Token lv_pastMessage_41_0=null;
Token otherlv_42=null;
Token otherlv_43=null;
Token lv_max_44_0=null;
Token otherlv_45=null;
Token otherlv_46=null;
Token lv_min_47_0=null;
Token otherlv_48=null;
Token otherlv_49=null;
Token lv_decimalMax_50_0=null;
Token otherlv_51=null;
Token otherlv_52=null;
Token lv_decimalMin_53_0=null;
Token lv_notEmpty_54_0=null;
Token otherlv_55=null;
Token lv_notEmptyMessage_56_0=null;
Token lv_notBlank_57_0=null;
Token otherlv_58=null;
Token lv_notBlankMessage_59_0=null;
Token otherlv_60=null;
Token otherlv_61=null;
Token lv_pattern_62_0=null;
Token otherlv_63=null;
Token otherlv_64=null;
Token lv_range_65_0=null;
Token otherlv_66=null;
Token otherlv_67=null;
Token lv_size_68_0=null;
Token otherlv_69=null;
Token otherlv_70=null;
Token lv_length_71_0=null;
Token otherlv_72=null;
Token otherlv_73=null;
Token lv_scriptAssert_74_0=null;
Token otherlv_75=null;
Token otherlv_76=null;
Token lv_url_77_0=null;
Token otherlv_78=null;
Token otherlv_79=null;
Token lv_validate_80_0=null;
Token otherlv_81=null;
Token otherlv_82=null;
Token lv_hint_83_0=null;
Token otherlv_84=null;
Enumerator lv_visibility_1_0 = null;
Enumerator lv_collectionType_2_0 = null;
AntlrDatatypeRuleToken lv_type_4_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:15151:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( (lv_collectionType_2_0= ruleCollectionType ) ) otherlv_3= '<' )? ( (lv_type_4_0= ruleType ) ) (otherlv_5= '>' )? ( (lv_name_6_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_8_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_20_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) (otherlv_84= ';' )? ) )
// InternalContextMappingDSL.g:15152:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( (lv_collectionType_2_0= ruleCollectionType ) ) otherlv_3= '<' )? ( (lv_type_4_0= ruleType ) ) (otherlv_5= '>' )? ( (lv_name_6_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_8_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_20_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) (otherlv_84= ';' )? )
{
// InternalContextMappingDSL.g:15152:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( (lv_collectionType_2_0= ruleCollectionType ) ) otherlv_3= '<' )? ( (lv_type_4_0= ruleType ) ) (otherlv_5= '>' )? ( (lv_name_6_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_8_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_20_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) (otherlv_84= ';' )? )
// InternalContextMappingDSL.g:15153:3: ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_visibility_1_0= ruleVisibility ) )? ( ( (lv_collectionType_2_0= ruleCollectionType ) ) otherlv_3= '<' )? ( (lv_type_4_0= ruleType ) ) (otherlv_5= '>' )? ( (lv_name_6_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_8_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_20_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) (otherlv_84= ';' )?
{
// InternalContextMappingDSL.g:15153:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt353=2;
int LA353_0 = input.LA(1);
if ( (LA353_0==RULE_STRING) ) {
alt353=1;
}
switch (alt353) {
case 1 :
// InternalContextMappingDSL.g:15154:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:15154:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:15155:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_170);
newLeafNode(lv_doc_0_0, grammarAccess.getDtoAttributeAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
// InternalContextMappingDSL.g:15171:3: ( (lv_visibility_1_0= ruleVisibility ) )?
int alt354=2;
int LA354_0 = input.LA(1);
if ( (LA354_0==124||(LA354_0>=247 && LA354_0<=249)) ) {
alt354=1;
}
switch (alt354) {
case 1 :
// InternalContextMappingDSL.g:15172:4: (lv_visibility_1_0= ruleVisibility )
{
// InternalContextMappingDSL.g:15172:4: (lv_visibility_1_0= ruleVisibility )
// InternalContextMappingDSL.g:15173:5: lv_visibility_1_0= ruleVisibility
{
newCompositeNode(grammarAccess.getDtoAttributeAccess().getVisibilityVisibilityEnumRuleCall_1_0());
pushFollow(FOLLOW_171);
lv_visibility_1_0=ruleVisibility();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDtoAttributeRule());
}
set(
current,
"visibility",
lv_visibility_1_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Visibility");
afterParserOrEnumRuleCall();
}
}
break;
}
// InternalContextMappingDSL.g:15190:3: ( ( (lv_collectionType_2_0= ruleCollectionType ) ) otherlv_3= '<' )?
int alt355=2;
int LA355_0 = input.LA(1);
if ( (LA355_0==233||(LA355_0>=243 && LA355_0<=246)) ) {
alt355=1;
}
switch (alt355) {
case 1 :
// InternalContextMappingDSL.g:15191:4: ( (lv_collectionType_2_0= ruleCollectionType ) ) otherlv_3= '<'
{
// InternalContextMappingDSL.g:15191:4: ( (lv_collectionType_2_0= ruleCollectionType ) )
// InternalContextMappingDSL.g:15192:5: (lv_collectionType_2_0= ruleCollectionType )
{
// InternalContextMappingDSL.g:15192:5: (lv_collectionType_2_0= ruleCollectionType )
// InternalContextMappingDSL.g:15193:6: lv_collectionType_2_0= ruleCollectionType
{
newCompositeNode(grammarAccess.getDtoAttributeAccess().getCollectionTypeCollectionTypeEnumRuleCall_2_0_0());
pushFollow(FOLLOW_126);
lv_collectionType_2_0=ruleCollectionType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDtoAttributeRule());
}
set(
current,
"collectionType",
lv_collectionType_2_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.CollectionType");
afterParserOrEnumRuleCall();
}
}
otherlv_3=(Token)match(input,118,FOLLOW_172);
newLeafNode(otherlv_3, grammarAccess.getDtoAttributeAccess().getLessThanSignKeyword_2_1());
}
break;
}
// InternalContextMappingDSL.g:15215:3: ( (lv_type_4_0= ruleType ) )
// InternalContextMappingDSL.g:15216:4: (lv_type_4_0= ruleType )
{
// InternalContextMappingDSL.g:15216:4: (lv_type_4_0= ruleType )
// InternalContextMappingDSL.g:15217:5: lv_type_4_0= ruleType
{
newCompositeNode(grammarAccess.getDtoAttributeAccess().getTypeTypeParserRuleCall_3_0());
pushFollow(FOLLOW_184);
lv_type_4_0=ruleType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDtoAttributeRule());
}
set(
current,
"type",
lv_type_4_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Type");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:15234:3: (otherlv_5= '>' )?
int alt356=2;
int LA356_0 = input.LA(1);
if ( (LA356_0==119) ) {
alt356=1;
}
switch (alt356) {
case 1 :
// InternalContextMappingDSL.g:15235:4: otherlv_5= '>'
{
otherlv_5=(Token)match(input,119,FOLLOW_10);
newLeafNode(otherlv_5, grammarAccess.getDtoAttributeAccess().getGreaterThanSignKeyword_4());
}
break;
}
// InternalContextMappingDSL.g:15240:3: ( (lv_name_6_0= RULE_ID ) )
// InternalContextMappingDSL.g:15241:4: (lv_name_6_0= RULE_ID )
{
// InternalContextMappingDSL.g:15241:4: (lv_name_6_0= RULE_ID )
// InternalContextMappingDSL.g:15242:5: lv_name_6_0= RULE_ID
{
lv_name_6_0=(Token)match(input,RULE_ID,FOLLOW_185);
newLeafNode(lv_name_6_0, grammarAccess.getDtoAttributeAccess().getNameIDTerminalRuleCall_5_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"name",
lv_name_6_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:15258:3: ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_8_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_20_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:15259:4: ( ( ( ({...}? => ( ({...}? => ( (lv_key_8_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_20_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:15259:4: ( ( ( ({...}? => ( ({...}? => ( (lv_key_8_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_20_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:15260:5: ( ( ({...}? => ( ({...}? => ( (lv_key_8_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_20_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
// InternalContextMappingDSL.g:15263:5: ( ( ({...}? => ( ({...}? => ( (lv_key_8_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_20_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:15264:6: ( ({...}? => ( ({...}? => ( (lv_key_8_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_20_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:15264:6: ( ({...}? => ( ({...}? => ( (lv_key_8_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_20_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) ) ) ) )*
loop369:
do {
int alt369=27;
alt369 = dfa369.predict(input);
switch (alt369) {
case 1 :
// InternalContextMappingDSL.g:15265:4: ({...}? => ( ({...}? => ( (lv_key_8_0= 'key' ) ) ) ) )
{
// InternalContextMappingDSL.g:15265:4: ({...}? => ( ({...}? => ( (lv_key_8_0= 'key' ) ) ) ) )
// InternalContextMappingDSL.g:15266:5: {...}? => ( ({...}? => ( (lv_key_8_0= 'key' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 0) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 0)");
}
// InternalContextMappingDSL.g:15266:109: ( ({...}? => ( (lv_key_8_0= 'key' ) ) ) )
// InternalContextMappingDSL.g:15267:6: ({...}? => ( (lv_key_8_0= 'key' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 0);
// InternalContextMappingDSL.g:15270:9: ({...}? => ( (lv_key_8_0= 'key' ) ) )
// InternalContextMappingDSL.g:15270:10: {...}? => ( (lv_key_8_0= 'key' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15270:19: ( (lv_key_8_0= 'key' ) )
// InternalContextMappingDSL.g:15270:20: (lv_key_8_0= 'key' )
{
// InternalContextMappingDSL.g:15270:20: (lv_key_8_0= 'key' )
// InternalContextMappingDSL.g:15271:10: lv_key_8_0= 'key'
{
lv_key_8_0=(Token)match(input,146,FOLLOW_185);
newLeafNode(lv_key_8_0, grammarAccess.getDtoAttributeAccess().getKeyKeyKeyword_6_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(current, "key", true, "key");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:15288:4: ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) ) ) )
{
// InternalContextMappingDSL.g:15288:4: ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) ) ) )
// InternalContextMappingDSL.g:15289:5: {...}? => ( ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 1) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 1)");
}
// InternalContextMappingDSL.g:15289:109: ( ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) ) )
// InternalContextMappingDSL.g:15290:6: ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 1);
// InternalContextMappingDSL.g:15293:9: ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) )
// InternalContextMappingDSL.g:15293:10: {...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15293:19: ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' )
int alt357=2;
int LA357_0 = input.LA(1);
if ( (LA357_0==RULE_NOT) ) {
alt357=1;
}
else if ( (LA357_0==147) ) {
alt357=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 357, 0, input);
throw nvae;
}
switch (alt357) {
case 1 :
// InternalContextMappingDSL.g:15293:20: ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' )
{
// InternalContextMappingDSL.g:15293:20: ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' )
// InternalContextMappingDSL.g:15294:10: ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable'
{
// InternalContextMappingDSL.g:15294:10: ( (lv_notChangeable_9_0= RULE_NOT ) )
// InternalContextMappingDSL.g:15295:11: (lv_notChangeable_9_0= RULE_NOT )
{
// InternalContextMappingDSL.g:15295:11: (lv_notChangeable_9_0= RULE_NOT )
// InternalContextMappingDSL.g:15296:12: lv_notChangeable_9_0= RULE_NOT
{
lv_notChangeable_9_0=(Token)match(input,RULE_NOT,FOLLOW_174);
newLeafNode(lv_notChangeable_9_0, grammarAccess.getDtoAttributeAccess().getNotChangeableNOTTerminalRuleCall_6_1_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"notChangeable",
true,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.NOT");
}
}
otherlv_10=(Token)match(input,147,FOLLOW_185);
newLeafNode(otherlv_10, grammarAccess.getDtoAttributeAccess().getChangeableKeyword_6_1_0_1());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:15318:9: otherlv_11= 'changeable'
{
otherlv_11=(Token)match(input,147,FOLLOW_185);
newLeafNode(otherlv_11, grammarAccess.getDtoAttributeAccess().getChangeableKeyword_6_1_1());
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:15328:4: ({...}? => ( ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) ) ) )
{
// InternalContextMappingDSL.g:15328:4: ({...}? => ( ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) ) ) )
// InternalContextMappingDSL.g:15329:5: {...}? => ( ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 2) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 2)");
}
// InternalContextMappingDSL.g:15329:109: ( ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) ) )
// InternalContextMappingDSL.g:15330:6: ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 2);
// InternalContextMappingDSL.g:15333:9: ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) )
// InternalContextMappingDSL.g:15333:10: {...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15333:19: ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) )
int alt358=2;
int LA358_0 = input.LA(1);
if ( (LA358_0==148) ) {
alt358=1;
}
else if ( (LA358_0==RULE_NOT) ) {
alt358=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 358, 0, input);
throw nvae;
}
switch (alt358) {
case 1 :
// InternalContextMappingDSL.g:15333:20: ( (lv_required_12_0= 'required' ) )
{
// InternalContextMappingDSL.g:15333:20: ( (lv_required_12_0= 'required' ) )
// InternalContextMappingDSL.g:15334:10: (lv_required_12_0= 'required' )
{
// InternalContextMappingDSL.g:15334:10: (lv_required_12_0= 'required' )
// InternalContextMappingDSL.g:15335:11: lv_required_12_0= 'required'
{
lv_required_12_0=(Token)match(input,148,FOLLOW_185);
newLeafNode(lv_required_12_0, grammarAccess.getDtoAttributeAccess().getRequiredRequiredKeyword_6_2_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(current, "required", true, "required");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:15348:9: (this_NOT_13= RULE_NOT otherlv_14= 'required' )
{
// InternalContextMappingDSL.g:15348:9: (this_NOT_13= RULE_NOT otherlv_14= 'required' )
// InternalContextMappingDSL.g:15349:10: this_NOT_13= RULE_NOT otherlv_14= 'required'
{
this_NOT_13=(Token)match(input,RULE_NOT,FOLLOW_175);
newLeafNode(this_NOT_13, grammarAccess.getDtoAttributeAccess().getNOTTerminalRuleCall_6_2_1_0());
otherlv_14=(Token)match(input,148,FOLLOW_185);
newLeafNode(otherlv_14, grammarAccess.getDtoAttributeAccess().getRequiredKeyword_6_2_1_1());
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:15364:4: ({...}? => ( ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:15364:4: ({...}? => ( ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:15365:5: {...}? => ( ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 3) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 3)");
}
// InternalContextMappingDSL.g:15365:109: ( ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:15366:6: ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 3);
// InternalContextMappingDSL.g:15369:9: ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:15369:10: {...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15369:19: ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:15369:20: ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:15369:20: ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) )
int alt359=2;
int LA359_0 = input.LA(1);
if ( (LA359_0==149) ) {
alt359=1;
}
else if ( (LA359_0==RULE_NOT) ) {
alt359=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 359, 0, input);
throw nvae;
}
switch (alt359) {
case 1 :
// InternalContextMappingDSL.g:15370:10: ( (lv_nullable_15_0= 'nullable' ) )
{
// InternalContextMappingDSL.g:15370:10: ( (lv_nullable_15_0= 'nullable' ) )
// InternalContextMappingDSL.g:15371:11: (lv_nullable_15_0= 'nullable' )
{
// InternalContextMappingDSL.g:15371:11: (lv_nullable_15_0= 'nullable' )
// InternalContextMappingDSL.g:15372:12: lv_nullable_15_0= 'nullable'
{
lv_nullable_15_0=(Token)match(input,149,FOLLOW_186);
newLeafNode(lv_nullable_15_0, grammarAccess.getDtoAttributeAccess().getNullableNullableKeyword_6_3_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(current, "nullable", true, "nullable");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:15385:10: (this_NOT_16= RULE_NOT otherlv_17= 'nullable' )
{
// InternalContextMappingDSL.g:15385:10: (this_NOT_16= RULE_NOT otherlv_17= 'nullable' )
// InternalContextMappingDSL.g:15386:11: this_NOT_16= RULE_NOT otherlv_17= 'nullable'
{
this_NOT_16=(Token)match(input,RULE_NOT,FOLLOW_177);
newLeafNode(this_NOT_16, grammarAccess.getDtoAttributeAccess().getNOTTerminalRuleCall_6_3_0_1_0());
otherlv_17=(Token)match(input,149,FOLLOW_186);
newLeafNode(otherlv_17, grammarAccess.getDtoAttributeAccess().getNullableKeyword_6_3_0_1_1());
}
}
break;
}
// InternalContextMappingDSL.g:15396:9: (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )?
int alt360=2;
int LA360_0 = input.LA(1);
if ( (LA360_0==21) ) {
alt360=1;
}
switch (alt360) {
case 1 :
// InternalContextMappingDSL.g:15397:10: otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) )
{
otherlv_18=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_18, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_3_1_0());
// InternalContextMappingDSL.g:15401:10: ( (lv_nullableMessage_19_0= RULE_STRING ) )
// InternalContextMappingDSL.g:15402:11: (lv_nullableMessage_19_0= RULE_STRING )
{
// InternalContextMappingDSL.g:15402:11: (lv_nullableMessage_19_0= RULE_STRING )
// InternalContextMappingDSL.g:15403:12: lv_nullableMessage_19_0= RULE_STRING
{
lv_nullableMessage_19_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_nullableMessage_19_0, grammarAccess.getDtoAttributeAccess().getNullableMessageSTRINGTerminalRuleCall_6_3_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"nullableMessage",
lv_nullableMessage_19_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 5 :
// InternalContextMappingDSL.g:15426:4: ({...}? => ( ({...}? => ( (lv_transient_20_0= 'transient' ) ) ) ) )
{
// InternalContextMappingDSL.g:15426:4: ({...}? => ( ({...}? => ( (lv_transient_20_0= 'transient' ) ) ) ) )
// InternalContextMappingDSL.g:15427:5: {...}? => ( ({...}? => ( (lv_transient_20_0= 'transient' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 4) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 4)");
}
// InternalContextMappingDSL.g:15427:109: ( ({...}? => ( (lv_transient_20_0= 'transient' ) ) ) )
// InternalContextMappingDSL.g:15428:6: ({...}? => ( (lv_transient_20_0= 'transient' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 4);
// InternalContextMappingDSL.g:15431:9: ({...}? => ( (lv_transient_20_0= 'transient' ) ) )
// InternalContextMappingDSL.g:15431:10: {...}? => ( (lv_transient_20_0= 'transient' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15431:19: ( (lv_transient_20_0= 'transient' ) )
// InternalContextMappingDSL.g:15431:20: (lv_transient_20_0= 'transient' )
{
// InternalContextMappingDSL.g:15431:20: (lv_transient_20_0= 'transient' )
// InternalContextMappingDSL.g:15432:10: lv_transient_20_0= 'transient'
{
lv_transient_20_0=(Token)match(input,170,FOLLOW_185);
newLeafNode(lv_transient_20_0, grammarAccess.getDtoAttributeAccess().getTransientTransientKeyword_6_4_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(current, "transient", true, "transient");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 6 :
// InternalContextMappingDSL.g:15449:4: ({...}? => ( ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:15449:4: ({...}? => ( ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:15450:5: {...}? => ( ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 5) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 5)");
}
// InternalContextMappingDSL.g:15450:109: ( ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:15451:6: ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 5);
// InternalContextMappingDSL.g:15454:9: ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:15454:10: {...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15454:19: ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:15454:20: ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:15454:20: ( (lv_assertFalse_21_0= 'assertFalse' ) )
// InternalContextMappingDSL.g:15455:10: (lv_assertFalse_21_0= 'assertFalse' )
{
// InternalContextMappingDSL.g:15455:10: (lv_assertFalse_21_0= 'assertFalse' )
// InternalContextMappingDSL.g:15456:11: lv_assertFalse_21_0= 'assertFalse'
{
lv_assertFalse_21_0=(Token)match(input,151,FOLLOW_186);
newLeafNode(lv_assertFalse_21_0, grammarAccess.getDtoAttributeAccess().getAssertFalseAssertFalseKeyword_6_5_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(current, "assertFalse", true, "assertFalse");
}
}
// InternalContextMappingDSL.g:15468:9: (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )?
int alt361=2;
int LA361_0 = input.LA(1);
if ( (LA361_0==21) ) {
alt361=1;
}
switch (alt361) {
case 1 :
// InternalContextMappingDSL.g:15469:10: otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) )
{
otherlv_22=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_22, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_5_1_0());
// InternalContextMappingDSL.g:15473:10: ( (lv_assertFalseMessage_23_0= RULE_STRING ) )
// InternalContextMappingDSL.g:15474:11: (lv_assertFalseMessage_23_0= RULE_STRING )
{
// InternalContextMappingDSL.g:15474:11: (lv_assertFalseMessage_23_0= RULE_STRING )
// InternalContextMappingDSL.g:15475:12: lv_assertFalseMessage_23_0= RULE_STRING
{
lv_assertFalseMessage_23_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_assertFalseMessage_23_0, grammarAccess.getDtoAttributeAccess().getAssertFalseMessageSTRINGTerminalRuleCall_6_5_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"assertFalseMessage",
lv_assertFalseMessage_23_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 7 :
// InternalContextMappingDSL.g:15498:4: ({...}? => ( ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:15498:4: ({...}? => ( ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:15499:5: {...}? => ( ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 6) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 6)");
}
// InternalContextMappingDSL.g:15499:109: ( ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:15500:6: ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 6);
// InternalContextMappingDSL.g:15503:9: ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:15503:10: {...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15503:19: ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:15503:20: ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:15503:20: ( (lv_assertTrue_24_0= 'assertTrue' ) )
// InternalContextMappingDSL.g:15504:10: (lv_assertTrue_24_0= 'assertTrue' )
{
// InternalContextMappingDSL.g:15504:10: (lv_assertTrue_24_0= 'assertTrue' )
// InternalContextMappingDSL.g:15505:11: lv_assertTrue_24_0= 'assertTrue'
{
lv_assertTrue_24_0=(Token)match(input,152,FOLLOW_186);
newLeafNode(lv_assertTrue_24_0, grammarAccess.getDtoAttributeAccess().getAssertTrueAssertTrueKeyword_6_6_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(current, "assertTrue", true, "assertTrue");
}
}
// InternalContextMappingDSL.g:15517:9: (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )?
int alt362=2;
int LA362_0 = input.LA(1);
if ( (LA362_0==21) ) {
alt362=1;
}
switch (alt362) {
case 1 :
// InternalContextMappingDSL.g:15518:10: otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) )
{
otherlv_25=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_25, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_6_1_0());
// InternalContextMappingDSL.g:15522:10: ( (lv_assertTrueMessage_26_0= RULE_STRING ) )
// InternalContextMappingDSL.g:15523:11: (lv_assertTrueMessage_26_0= RULE_STRING )
{
// InternalContextMappingDSL.g:15523:11: (lv_assertTrueMessage_26_0= RULE_STRING )
// InternalContextMappingDSL.g:15524:12: lv_assertTrueMessage_26_0= RULE_STRING
{
lv_assertTrueMessage_26_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_assertTrueMessage_26_0, grammarAccess.getDtoAttributeAccess().getAssertTrueMessageSTRINGTerminalRuleCall_6_6_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"assertTrueMessage",
lv_assertTrueMessage_26_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 8 :
// InternalContextMappingDSL.g:15547:4: ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:15547:4: ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:15548:5: {...}? => ( ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 7) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 7)");
}
// InternalContextMappingDSL.g:15548:109: ( ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:15549:6: ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 7);
// InternalContextMappingDSL.g:15552:9: ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:15552:10: {...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15552:19: ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:15552:20: ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:15552:20: ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) )
// InternalContextMappingDSL.g:15553:10: (lv_creditCardNumber_27_0= 'creditCardNumber' )
{
// InternalContextMappingDSL.g:15553:10: (lv_creditCardNumber_27_0= 'creditCardNumber' )
// InternalContextMappingDSL.g:15554:11: lv_creditCardNumber_27_0= 'creditCardNumber'
{
lv_creditCardNumber_27_0=(Token)match(input,153,FOLLOW_186);
newLeafNode(lv_creditCardNumber_27_0, grammarAccess.getDtoAttributeAccess().getCreditCardNumberCreditCardNumberKeyword_6_7_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(current, "creditCardNumber", true, "creditCardNumber");
}
}
// InternalContextMappingDSL.g:15566:9: (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )?
int alt363=2;
int LA363_0 = input.LA(1);
if ( (LA363_0==21) ) {
alt363=1;
}
switch (alt363) {
case 1 :
// InternalContextMappingDSL.g:15567:10: otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) )
{
otherlv_28=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_28, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_7_1_0());
// InternalContextMappingDSL.g:15571:10: ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) )
// InternalContextMappingDSL.g:15572:11: (lv_creditCardNumberMessage_29_0= RULE_STRING )
{
// InternalContextMappingDSL.g:15572:11: (lv_creditCardNumberMessage_29_0= RULE_STRING )
// InternalContextMappingDSL.g:15573:12: lv_creditCardNumberMessage_29_0= RULE_STRING
{
lv_creditCardNumberMessage_29_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_creditCardNumberMessage_29_0, grammarAccess.getDtoAttributeAccess().getCreditCardNumberMessageSTRINGTerminalRuleCall_6_7_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"creditCardNumberMessage",
lv_creditCardNumberMessage_29_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 9 :
// InternalContextMappingDSL.g:15596:4: ({...}? => ( ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:15596:4: ({...}? => ( ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:15597:5: {...}? => ( ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 8) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 8)");
}
// InternalContextMappingDSL.g:15597:109: ( ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:15598:6: ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 8);
// InternalContextMappingDSL.g:15601:9: ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:15601:10: {...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15601:19: (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:15601:20: otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) )
{
otherlv_30=(Token)match(input,154,FOLLOW_89);
newLeafNode(otherlv_30, grammarAccess.getDtoAttributeAccess().getDigitsKeyword_6_8_0());
otherlv_31=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_31, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_8_1());
// InternalContextMappingDSL.g:15609:9: ( (lv_digits_32_0= RULE_STRING ) )
// InternalContextMappingDSL.g:15610:10: (lv_digits_32_0= RULE_STRING )
{
// InternalContextMappingDSL.g:15610:10: (lv_digits_32_0= RULE_STRING )
// InternalContextMappingDSL.g:15611:11: lv_digits_32_0= RULE_STRING
{
lv_digits_32_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_digits_32_0, grammarAccess.getDtoAttributeAccess().getDigitsSTRINGTerminalRuleCall_6_8_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"digits",
lv_digits_32_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 10 :
// InternalContextMappingDSL.g:15633:4: ({...}? => ( ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:15633:4: ({...}? => ( ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:15634:5: {...}? => ( ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 9) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 9)");
}
// InternalContextMappingDSL.g:15634:109: ( ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:15635:6: ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 9);
// InternalContextMappingDSL.g:15638:9: ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:15638:10: {...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15638:19: ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:15638:20: ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:15638:20: ( (lv_email_33_0= 'email' ) )
// InternalContextMappingDSL.g:15639:10: (lv_email_33_0= 'email' )
{
// InternalContextMappingDSL.g:15639:10: (lv_email_33_0= 'email' )
// InternalContextMappingDSL.g:15640:11: lv_email_33_0= 'email'
{
lv_email_33_0=(Token)match(input,155,FOLLOW_186);
newLeafNode(lv_email_33_0, grammarAccess.getDtoAttributeAccess().getEmailEmailKeyword_6_9_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(current, "email", true, "email");
}
}
// InternalContextMappingDSL.g:15652:9: (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )?
int alt364=2;
int LA364_0 = input.LA(1);
if ( (LA364_0==21) ) {
alt364=1;
}
switch (alt364) {
case 1 :
// InternalContextMappingDSL.g:15653:10: otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) )
{
otherlv_34=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_34, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_9_1_0());
// InternalContextMappingDSL.g:15657:10: ( (lv_emailMessage_35_0= RULE_STRING ) )
// InternalContextMappingDSL.g:15658:11: (lv_emailMessage_35_0= RULE_STRING )
{
// InternalContextMappingDSL.g:15658:11: (lv_emailMessage_35_0= RULE_STRING )
// InternalContextMappingDSL.g:15659:12: lv_emailMessage_35_0= RULE_STRING
{
lv_emailMessage_35_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_emailMessage_35_0, grammarAccess.getDtoAttributeAccess().getEmailMessageSTRINGTerminalRuleCall_6_9_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"emailMessage",
lv_emailMessage_35_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 11 :
// InternalContextMappingDSL.g:15682:4: ({...}? => ( ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:15682:4: ({...}? => ( ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:15683:5: {...}? => ( ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 10) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 10)");
}
// InternalContextMappingDSL.g:15683:110: ( ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:15684:6: ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 10);
// InternalContextMappingDSL.g:15687:9: ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:15687:10: {...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15687:19: ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:15687:20: ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:15687:20: ( (lv_future_36_0= 'future' ) )
// InternalContextMappingDSL.g:15688:10: (lv_future_36_0= 'future' )
{
// InternalContextMappingDSL.g:15688:10: (lv_future_36_0= 'future' )
// InternalContextMappingDSL.g:15689:11: lv_future_36_0= 'future'
{
lv_future_36_0=(Token)match(input,156,FOLLOW_186);
newLeafNode(lv_future_36_0, grammarAccess.getDtoAttributeAccess().getFutureFutureKeyword_6_10_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(current, "future", true, "future");
}
}
// InternalContextMappingDSL.g:15701:9: (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )?
int alt365=2;
int LA365_0 = input.LA(1);
if ( (LA365_0==21) ) {
alt365=1;
}
switch (alt365) {
case 1 :
// InternalContextMappingDSL.g:15702:10: otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) )
{
otherlv_37=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_37, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_10_1_0());
// InternalContextMappingDSL.g:15706:10: ( (lv_futureMessage_38_0= RULE_STRING ) )
// InternalContextMappingDSL.g:15707:11: (lv_futureMessage_38_0= RULE_STRING )
{
// InternalContextMappingDSL.g:15707:11: (lv_futureMessage_38_0= RULE_STRING )
// InternalContextMappingDSL.g:15708:12: lv_futureMessage_38_0= RULE_STRING
{
lv_futureMessage_38_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_futureMessage_38_0, grammarAccess.getDtoAttributeAccess().getFutureMessageSTRINGTerminalRuleCall_6_10_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"futureMessage",
lv_futureMessage_38_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 12 :
// InternalContextMappingDSL.g:15731:4: ({...}? => ( ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:15731:4: ({...}? => ( ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:15732:5: {...}? => ( ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 11) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 11)");
}
// InternalContextMappingDSL.g:15732:110: ( ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:15733:6: ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 11);
// InternalContextMappingDSL.g:15736:9: ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:15736:10: {...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15736:19: ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:15736:20: ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:15736:20: ( (lv_past_39_0= 'past' ) )
// InternalContextMappingDSL.g:15737:10: (lv_past_39_0= 'past' )
{
// InternalContextMappingDSL.g:15737:10: (lv_past_39_0= 'past' )
// InternalContextMappingDSL.g:15738:11: lv_past_39_0= 'past'
{
lv_past_39_0=(Token)match(input,157,FOLLOW_186);
newLeafNode(lv_past_39_0, grammarAccess.getDtoAttributeAccess().getPastPastKeyword_6_11_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(current, "past", true, "past");
}
}
// InternalContextMappingDSL.g:15750:9: (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )?
int alt366=2;
int LA366_0 = input.LA(1);
if ( (LA366_0==21) ) {
alt366=1;
}
switch (alt366) {
case 1 :
// InternalContextMappingDSL.g:15751:10: otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) )
{
otherlv_40=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_40, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_11_1_0());
// InternalContextMappingDSL.g:15755:10: ( (lv_pastMessage_41_0= RULE_STRING ) )
// InternalContextMappingDSL.g:15756:11: (lv_pastMessage_41_0= RULE_STRING )
{
// InternalContextMappingDSL.g:15756:11: (lv_pastMessage_41_0= RULE_STRING )
// InternalContextMappingDSL.g:15757:12: lv_pastMessage_41_0= RULE_STRING
{
lv_pastMessage_41_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_pastMessage_41_0, grammarAccess.getDtoAttributeAccess().getPastMessageSTRINGTerminalRuleCall_6_11_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"pastMessage",
lv_pastMessage_41_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 13 :
// InternalContextMappingDSL.g:15780:4: ({...}? => ( ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:15780:4: ({...}? => ( ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:15781:5: {...}? => ( ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 12) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 12)");
}
// InternalContextMappingDSL.g:15781:110: ( ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:15782:6: ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 12);
// InternalContextMappingDSL.g:15785:9: ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:15785:10: {...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15785:19: (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:15785:20: otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) )
{
otherlv_42=(Token)match(input,158,FOLLOW_89);
newLeafNode(otherlv_42, grammarAccess.getDtoAttributeAccess().getMaxKeyword_6_12_0());
otherlv_43=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_43, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_12_1());
// InternalContextMappingDSL.g:15793:9: ( (lv_max_44_0= RULE_STRING ) )
// InternalContextMappingDSL.g:15794:10: (lv_max_44_0= RULE_STRING )
{
// InternalContextMappingDSL.g:15794:10: (lv_max_44_0= RULE_STRING )
// InternalContextMappingDSL.g:15795:11: lv_max_44_0= RULE_STRING
{
lv_max_44_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_max_44_0, grammarAccess.getDtoAttributeAccess().getMaxSTRINGTerminalRuleCall_6_12_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"max",
lv_max_44_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 14 :
// InternalContextMappingDSL.g:15817:4: ({...}? => ( ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:15817:4: ({...}? => ( ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:15818:5: {...}? => ( ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 13) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 13)");
}
// InternalContextMappingDSL.g:15818:110: ( ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:15819:6: ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 13);
// InternalContextMappingDSL.g:15822:9: ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:15822:10: {...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15822:19: (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:15822:20: otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) )
{
otherlv_45=(Token)match(input,159,FOLLOW_89);
newLeafNode(otherlv_45, grammarAccess.getDtoAttributeAccess().getMinKeyword_6_13_0());
otherlv_46=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_46, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_13_1());
// InternalContextMappingDSL.g:15830:9: ( (lv_min_47_0= RULE_STRING ) )
// InternalContextMappingDSL.g:15831:10: (lv_min_47_0= RULE_STRING )
{
// InternalContextMappingDSL.g:15831:10: (lv_min_47_0= RULE_STRING )
// InternalContextMappingDSL.g:15832:11: lv_min_47_0= RULE_STRING
{
lv_min_47_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_min_47_0, grammarAccess.getDtoAttributeAccess().getMinSTRINGTerminalRuleCall_6_13_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"min",
lv_min_47_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 15 :
// InternalContextMappingDSL.g:15854:4: ({...}? => ( ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:15854:4: ({...}? => ( ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:15855:5: {...}? => ( ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 14) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 14)");
}
// InternalContextMappingDSL.g:15855:110: ( ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:15856:6: ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 14);
// InternalContextMappingDSL.g:15859:9: ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:15859:10: {...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15859:19: (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:15859:20: otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) )
{
otherlv_48=(Token)match(input,160,FOLLOW_89);
newLeafNode(otherlv_48, grammarAccess.getDtoAttributeAccess().getDecimalMaxKeyword_6_14_0());
otherlv_49=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_49, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_14_1());
// InternalContextMappingDSL.g:15867:9: ( (lv_decimalMax_50_0= RULE_STRING ) )
// InternalContextMappingDSL.g:15868:10: (lv_decimalMax_50_0= RULE_STRING )
{
// InternalContextMappingDSL.g:15868:10: (lv_decimalMax_50_0= RULE_STRING )
// InternalContextMappingDSL.g:15869:11: lv_decimalMax_50_0= RULE_STRING
{
lv_decimalMax_50_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_decimalMax_50_0, grammarAccess.getDtoAttributeAccess().getDecimalMaxSTRINGTerminalRuleCall_6_14_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"decimalMax",
lv_decimalMax_50_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 16 :
// InternalContextMappingDSL.g:15891:4: ({...}? => ( ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:15891:4: ({...}? => ( ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:15892:5: {...}? => ( ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 15) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 15)");
}
// InternalContextMappingDSL.g:15892:110: ( ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:15893:6: ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 15);
// InternalContextMappingDSL.g:15896:9: ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:15896:10: {...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15896:19: (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:15896:20: otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) )
{
otherlv_51=(Token)match(input,161,FOLLOW_89);
newLeafNode(otherlv_51, grammarAccess.getDtoAttributeAccess().getDecimalMinKeyword_6_15_0());
otherlv_52=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_52, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_15_1());
// InternalContextMappingDSL.g:15904:9: ( (lv_decimalMin_53_0= RULE_STRING ) )
// InternalContextMappingDSL.g:15905:10: (lv_decimalMin_53_0= RULE_STRING )
{
// InternalContextMappingDSL.g:15905:10: (lv_decimalMin_53_0= RULE_STRING )
// InternalContextMappingDSL.g:15906:11: lv_decimalMin_53_0= RULE_STRING
{
lv_decimalMin_53_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_decimalMin_53_0, grammarAccess.getDtoAttributeAccess().getDecimalMinSTRINGTerminalRuleCall_6_15_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"decimalMin",
lv_decimalMin_53_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 17 :
// InternalContextMappingDSL.g:15928:4: ({...}? => ( ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:15928:4: ({...}? => ( ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:15929:5: {...}? => ( ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 16) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 16)");
}
// InternalContextMappingDSL.g:15929:110: ( ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:15930:6: ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 16);
// InternalContextMappingDSL.g:15933:9: ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:15933:10: {...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15933:19: ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:15933:20: ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:15933:20: ( (lv_notEmpty_54_0= 'notEmpty' ) )
// InternalContextMappingDSL.g:15934:10: (lv_notEmpty_54_0= 'notEmpty' )
{
// InternalContextMappingDSL.g:15934:10: (lv_notEmpty_54_0= 'notEmpty' )
// InternalContextMappingDSL.g:15935:11: lv_notEmpty_54_0= 'notEmpty'
{
lv_notEmpty_54_0=(Token)match(input,162,FOLLOW_186);
newLeafNode(lv_notEmpty_54_0, grammarAccess.getDtoAttributeAccess().getNotEmptyNotEmptyKeyword_6_16_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(current, "notEmpty", true, "notEmpty");
}
}
// InternalContextMappingDSL.g:15947:9: (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )?
int alt367=2;
int LA367_0 = input.LA(1);
if ( (LA367_0==21) ) {
alt367=1;
}
switch (alt367) {
case 1 :
// InternalContextMappingDSL.g:15948:10: otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) )
{
otherlv_55=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_55, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_16_1_0());
// InternalContextMappingDSL.g:15952:10: ( (lv_notEmptyMessage_56_0= RULE_STRING ) )
// InternalContextMappingDSL.g:15953:11: (lv_notEmptyMessage_56_0= RULE_STRING )
{
// InternalContextMappingDSL.g:15953:11: (lv_notEmptyMessage_56_0= RULE_STRING )
// InternalContextMappingDSL.g:15954:12: lv_notEmptyMessage_56_0= RULE_STRING
{
lv_notEmptyMessage_56_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_notEmptyMessage_56_0, grammarAccess.getDtoAttributeAccess().getNotEmptyMessageSTRINGTerminalRuleCall_6_16_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"notEmptyMessage",
lv_notEmptyMessage_56_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 18 :
// InternalContextMappingDSL.g:15977:4: ({...}? => ( ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:15977:4: ({...}? => ( ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:15978:5: {...}? => ( ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 17) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 17)");
}
// InternalContextMappingDSL.g:15978:110: ( ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:15979:6: ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 17);
// InternalContextMappingDSL.g:15982:9: ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:15982:10: {...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:15982:19: ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:15982:20: ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:15982:20: ( (lv_notBlank_57_0= 'notBlank' ) )
// InternalContextMappingDSL.g:15983:10: (lv_notBlank_57_0= 'notBlank' )
{
// InternalContextMappingDSL.g:15983:10: (lv_notBlank_57_0= 'notBlank' )
// InternalContextMappingDSL.g:15984:11: lv_notBlank_57_0= 'notBlank'
{
lv_notBlank_57_0=(Token)match(input,163,FOLLOW_186);
newLeafNode(lv_notBlank_57_0, grammarAccess.getDtoAttributeAccess().getNotBlankNotBlankKeyword_6_17_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(current, "notBlank", true, "notBlank");
}
}
// InternalContextMappingDSL.g:15996:9: (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )?
int alt368=2;
int LA368_0 = input.LA(1);
if ( (LA368_0==21) ) {
alt368=1;
}
switch (alt368) {
case 1 :
// InternalContextMappingDSL.g:15997:10: otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) )
{
otherlv_58=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_58, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_17_1_0());
// InternalContextMappingDSL.g:16001:10: ( (lv_notBlankMessage_59_0= RULE_STRING ) )
// InternalContextMappingDSL.g:16002:11: (lv_notBlankMessage_59_0= RULE_STRING )
{
// InternalContextMappingDSL.g:16002:11: (lv_notBlankMessage_59_0= RULE_STRING )
// InternalContextMappingDSL.g:16003:12: lv_notBlankMessage_59_0= RULE_STRING
{
lv_notBlankMessage_59_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_notBlankMessage_59_0, grammarAccess.getDtoAttributeAccess().getNotBlankMessageSTRINGTerminalRuleCall_6_17_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"notBlankMessage",
lv_notBlankMessage_59_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 19 :
// InternalContextMappingDSL.g:16026:4: ({...}? => ( ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:16026:4: ({...}? => ( ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:16027:5: {...}? => ( ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 18) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 18)");
}
// InternalContextMappingDSL.g:16027:110: ( ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:16028:6: ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 18);
// InternalContextMappingDSL.g:16031:9: ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:16031:10: {...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:16031:19: (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:16031:20: otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) )
{
otherlv_60=(Token)match(input,164,FOLLOW_89);
newLeafNode(otherlv_60, grammarAccess.getDtoAttributeAccess().getPatternKeyword_6_18_0());
otherlv_61=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_61, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_18_1());
// InternalContextMappingDSL.g:16039:9: ( (lv_pattern_62_0= RULE_STRING ) )
// InternalContextMappingDSL.g:16040:10: (lv_pattern_62_0= RULE_STRING )
{
// InternalContextMappingDSL.g:16040:10: (lv_pattern_62_0= RULE_STRING )
// InternalContextMappingDSL.g:16041:11: lv_pattern_62_0= RULE_STRING
{
lv_pattern_62_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_pattern_62_0, grammarAccess.getDtoAttributeAccess().getPatternSTRINGTerminalRuleCall_6_18_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"pattern",
lv_pattern_62_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 20 :
// InternalContextMappingDSL.g:16063:4: ({...}? => ( ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:16063:4: ({...}? => ( ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:16064:5: {...}? => ( ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 19) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 19)");
}
// InternalContextMappingDSL.g:16064:110: ( ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:16065:6: ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 19);
// InternalContextMappingDSL.g:16068:9: ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:16068:10: {...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:16068:19: (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:16068:20: otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) )
{
otherlv_63=(Token)match(input,165,FOLLOW_89);
newLeafNode(otherlv_63, grammarAccess.getDtoAttributeAccess().getRangeKeyword_6_19_0());
otherlv_64=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_64, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_19_1());
// InternalContextMappingDSL.g:16076:9: ( (lv_range_65_0= RULE_STRING ) )
// InternalContextMappingDSL.g:16077:10: (lv_range_65_0= RULE_STRING )
{
// InternalContextMappingDSL.g:16077:10: (lv_range_65_0= RULE_STRING )
// InternalContextMappingDSL.g:16078:11: lv_range_65_0= RULE_STRING
{
lv_range_65_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_range_65_0, grammarAccess.getDtoAttributeAccess().getRangeSTRINGTerminalRuleCall_6_19_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"range",
lv_range_65_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 21 :
// InternalContextMappingDSL.g:16100:4: ({...}? => ( ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:16100:4: ({...}? => ( ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:16101:5: {...}? => ( ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 20) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 20)");
}
// InternalContextMappingDSL.g:16101:110: ( ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:16102:6: ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 20);
// InternalContextMappingDSL.g:16105:9: ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:16105:10: {...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:16105:19: (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:16105:20: otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) )
{
otherlv_66=(Token)match(input,166,FOLLOW_89);
newLeafNode(otherlv_66, grammarAccess.getDtoAttributeAccess().getSizeKeyword_6_20_0());
otherlv_67=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_67, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_20_1());
// InternalContextMappingDSL.g:16113:9: ( (lv_size_68_0= RULE_STRING ) )
// InternalContextMappingDSL.g:16114:10: (lv_size_68_0= RULE_STRING )
{
// InternalContextMappingDSL.g:16114:10: (lv_size_68_0= RULE_STRING )
// InternalContextMappingDSL.g:16115:11: lv_size_68_0= RULE_STRING
{
lv_size_68_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_size_68_0, grammarAccess.getDtoAttributeAccess().getSizeSTRINGTerminalRuleCall_6_20_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"size",
lv_size_68_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 22 :
// InternalContextMappingDSL.g:16137:4: ({...}? => ( ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:16137:4: ({...}? => ( ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:16138:5: {...}? => ( ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 21) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 21)");
}
// InternalContextMappingDSL.g:16138:110: ( ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:16139:6: ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 21);
// InternalContextMappingDSL.g:16142:9: ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:16142:10: {...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:16142:19: (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:16142:20: otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) )
{
otherlv_69=(Token)match(input,167,FOLLOW_89);
newLeafNode(otherlv_69, grammarAccess.getDtoAttributeAccess().getLengthKeyword_6_21_0());
otherlv_70=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_70, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_21_1());
// InternalContextMappingDSL.g:16150:9: ( (lv_length_71_0= RULE_STRING ) )
// InternalContextMappingDSL.g:16151:10: (lv_length_71_0= RULE_STRING )
{
// InternalContextMappingDSL.g:16151:10: (lv_length_71_0= RULE_STRING )
// InternalContextMappingDSL.g:16152:11: lv_length_71_0= RULE_STRING
{
lv_length_71_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_length_71_0, grammarAccess.getDtoAttributeAccess().getLengthSTRINGTerminalRuleCall_6_21_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"length",
lv_length_71_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 23 :
// InternalContextMappingDSL.g:16174:4: ({...}? => ( ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:16174:4: ({...}? => ( ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:16175:5: {...}? => ( ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 22) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 22)");
}
// InternalContextMappingDSL.g:16175:110: ( ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:16176:6: ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 22);
// InternalContextMappingDSL.g:16179:9: ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:16179:10: {...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:16179:19: (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:16179:20: otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) )
{
otherlv_72=(Token)match(input,168,FOLLOW_89);
newLeafNode(otherlv_72, grammarAccess.getDtoAttributeAccess().getScriptAssertKeyword_6_22_0());
otherlv_73=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_73, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_22_1());
// InternalContextMappingDSL.g:16187:9: ( (lv_scriptAssert_74_0= RULE_STRING ) )
// InternalContextMappingDSL.g:16188:10: (lv_scriptAssert_74_0= RULE_STRING )
{
// InternalContextMappingDSL.g:16188:10: (lv_scriptAssert_74_0= RULE_STRING )
// InternalContextMappingDSL.g:16189:11: lv_scriptAssert_74_0= RULE_STRING
{
lv_scriptAssert_74_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_scriptAssert_74_0, grammarAccess.getDtoAttributeAccess().getScriptAssertSTRINGTerminalRuleCall_6_22_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"scriptAssert",
lv_scriptAssert_74_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 24 :
// InternalContextMappingDSL.g:16211:4: ({...}? => ( ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:16211:4: ({...}? => ( ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:16212:5: {...}? => ( ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 23) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 23)");
}
// InternalContextMappingDSL.g:16212:110: ( ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:16213:6: ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 23);
// InternalContextMappingDSL.g:16216:9: ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:16216:10: {...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:16216:19: (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:16216:20: otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) )
{
otherlv_75=(Token)match(input,169,FOLLOW_89);
newLeafNode(otherlv_75, grammarAccess.getDtoAttributeAccess().getUrlKeyword_6_23_0());
otherlv_76=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_76, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_23_1());
// InternalContextMappingDSL.g:16224:9: ( (lv_url_77_0= RULE_STRING ) )
// InternalContextMappingDSL.g:16225:10: (lv_url_77_0= RULE_STRING )
{
// InternalContextMappingDSL.g:16225:10: (lv_url_77_0= RULE_STRING )
// InternalContextMappingDSL.g:16226:11: lv_url_77_0= RULE_STRING
{
lv_url_77_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_url_77_0, grammarAccess.getDtoAttributeAccess().getUrlSTRINGTerminalRuleCall_6_23_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"url",
lv_url_77_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 25 :
// InternalContextMappingDSL.g:16248:4: ({...}? => ( ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:16248:4: ({...}? => ( ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:16249:5: {...}? => ( ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 24) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 24)");
}
// InternalContextMappingDSL.g:16249:110: ( ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:16250:6: ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 24);
// InternalContextMappingDSL.g:16253:9: ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:16253:10: {...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:16253:19: (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:16253:20: otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) )
{
otherlv_78=(Token)match(input,133,FOLLOW_89);
newLeafNode(otherlv_78, grammarAccess.getDtoAttributeAccess().getValidateKeyword_6_24_0());
otherlv_79=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_79, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_24_1());
// InternalContextMappingDSL.g:16261:9: ( (lv_validate_80_0= RULE_STRING ) )
// InternalContextMappingDSL.g:16262:10: (lv_validate_80_0= RULE_STRING )
{
// InternalContextMappingDSL.g:16262:10: (lv_validate_80_0= RULE_STRING )
// InternalContextMappingDSL.g:16263:11: lv_validate_80_0= RULE_STRING
{
lv_validate_80_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_validate_80_0, grammarAccess.getDtoAttributeAccess().getValidateSTRINGTerminalRuleCall_6_24_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"validate",
lv_validate_80_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
case 26 :
// InternalContextMappingDSL.g:16285:4: ({...}? => ( ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:16285:4: ({...}? => ( ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:16286:5: {...}? => ( ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 25) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 25)");
}
// InternalContextMappingDSL.g:16286:110: ( ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:16287:6: ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 25);
// InternalContextMappingDSL.g:16290:9: ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:16290:10: {...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoAttribute", "true");
}
// InternalContextMappingDSL.g:16290:19: (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:16290:20: otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) )
{
otherlv_81=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_81, grammarAccess.getDtoAttributeAccess().getHintKeyword_6_25_0());
otherlv_82=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_82, grammarAccess.getDtoAttributeAccess().getEqualsSignKeyword_6_25_1());
// InternalContextMappingDSL.g:16298:9: ( (lv_hint_83_0= RULE_STRING ) )
// InternalContextMappingDSL.g:16299:10: (lv_hint_83_0= RULE_STRING )
{
// InternalContextMappingDSL.g:16299:10: (lv_hint_83_0= RULE_STRING )
// InternalContextMappingDSL.g:16300:11: lv_hint_83_0= RULE_STRING
{
lv_hint_83_0=(Token)match(input,RULE_STRING,FOLLOW_185);
newLeafNode(lv_hint_83_0, grammarAccess.getDtoAttributeAccess().getHintSTRINGTerminalRuleCall_6_25_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoAttributeRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_83_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
}
}
break;
default :
break loop369;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6());
}
// InternalContextMappingDSL.g:16329:3: (otherlv_84= ';' )?
int alt370=2;
int LA370_0 = input.LA(1);
if ( (LA370_0==105) ) {
alt370=1;
}
switch (alt370) {
case 1 :
// InternalContextMappingDSL.g:16330:4: otherlv_84= ';'
{
otherlv_84=(Token)match(input,105,FOLLOW_2);
newLeafNode(otherlv_84, grammarAccess.getDtoAttributeAccess().getSemicolonKeyword_7());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleDtoAttribute"
// $ANTLR start "entryRuleDtoReference"
// InternalContextMappingDSL.g:16339:1: entryRuleDtoReference returns [EObject current=null] : iv_ruleDtoReference= ruleDtoReference EOF ;
public final EObject entryRuleDtoReference() throws RecognitionException {
EObject current = null;
EObject iv_ruleDtoReference = null;
try {
// InternalContextMappingDSL.g:16339:53: (iv_ruleDtoReference= ruleDtoReference EOF )
// InternalContextMappingDSL.g:16340:2: iv_ruleDtoReference= ruleDtoReference EOF
{
newCompositeNode(grammarAccess.getDtoReferenceRule());
pushFollow(FOLLOW_1);
iv_ruleDtoReference=ruleDtoReference();
state._fsp--;
current =iv_ruleDtoReference;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleDtoReference"
// $ANTLR start "ruleDtoReference"
// InternalContextMappingDSL.g:16346:1: ruleDtoReference returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? this_REF_1= RULE_REF ( (lv_visibility_2_0= ruleVisibility ) )? ( ( (lv_collectionType_3_0= ruleCollectionType ) ) otherlv_4= '<' )? ( (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) ) ) (otherlv_7= '>' )? ( (lv_name_8_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_10_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_22_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) (otherlv_38= ';' )? ) ;
public final EObject ruleDtoReference() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token this_REF_1=null;
Token otherlv_4=null;
Token otherlv_5=null;
Token otherlv_6=null;
Token otherlv_7=null;
Token lv_name_8_0=null;
Token lv_key_10_0=null;
Token lv_notChangeable_11_0=null;
Token otherlv_12=null;
Token otherlv_13=null;
Token lv_required_14_0=null;
Token this_NOT_15=null;
Token otherlv_16=null;
Token lv_nullable_17_0=null;
Token this_NOT_18=null;
Token otherlv_19=null;
Token otherlv_20=null;
Token lv_nullableMessage_21_0=null;
Token lv_transient_22_0=null;
Token lv_notEmpty_23_0=null;
Token otherlv_24=null;
Token lv_notEmptyMessage_25_0=null;
Token otherlv_26=null;
Token otherlv_27=null;
Token lv_size_28_0=null;
Token lv_valid_29_0=null;
Token otherlv_30=null;
Token lv_validMessage_31_0=null;
Token otherlv_32=null;
Token otherlv_33=null;
Token lv_validate_34_0=null;
Token otherlv_35=null;
Token otherlv_36=null;
Token lv_hint_37_0=null;
Token otherlv_38=null;
Enumerator lv_visibility_2_0 = null;
Enumerator lv_collectionType_3_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:16352:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? this_REF_1= RULE_REF ( (lv_visibility_2_0= ruleVisibility ) )? ( ( (lv_collectionType_3_0= ruleCollectionType ) ) otherlv_4= '<' )? ( (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) ) ) (otherlv_7= '>' )? ( (lv_name_8_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_10_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_22_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) (otherlv_38= ';' )? ) )
// InternalContextMappingDSL.g:16353:2: ( ( (lv_doc_0_0= RULE_STRING ) )? this_REF_1= RULE_REF ( (lv_visibility_2_0= ruleVisibility ) )? ( ( (lv_collectionType_3_0= ruleCollectionType ) ) otherlv_4= '<' )? ( (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) ) ) (otherlv_7= '>' )? ( (lv_name_8_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_10_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_22_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) (otherlv_38= ';' )? )
{
// InternalContextMappingDSL.g:16353:2: ( ( (lv_doc_0_0= RULE_STRING ) )? this_REF_1= RULE_REF ( (lv_visibility_2_0= ruleVisibility ) )? ( ( (lv_collectionType_3_0= ruleCollectionType ) ) otherlv_4= '<' )? ( (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) ) ) (otherlv_7= '>' )? ( (lv_name_8_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_10_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_22_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) (otherlv_38= ';' )? )
// InternalContextMappingDSL.g:16354:3: ( (lv_doc_0_0= RULE_STRING ) )? this_REF_1= RULE_REF ( (lv_visibility_2_0= ruleVisibility ) )? ( ( (lv_collectionType_3_0= ruleCollectionType ) ) otherlv_4= '<' )? ( (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) ) ) (otherlv_7= '>' )? ( (lv_name_8_0= RULE_ID ) ) ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_10_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_22_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) ) ) ) )* ) ) ) (otherlv_38= ';' )?
{
// InternalContextMappingDSL.g:16354:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt371=2;
int LA371_0 = input.LA(1);
if ( (LA371_0==RULE_STRING) ) {
alt371=1;
}
switch (alt371) {
case 1 :
// InternalContextMappingDSL.g:16355:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:16355:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:16356:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_178);
newLeafNode(lv_doc_0_0, grammarAccess.getDtoReferenceAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoReferenceRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
this_REF_1=(Token)match(input,RULE_REF,FOLLOW_179);
newLeafNode(this_REF_1, grammarAccess.getDtoReferenceAccess().getREFTerminalRuleCall_1());
// InternalContextMappingDSL.g:16376:3: ( (lv_visibility_2_0= ruleVisibility ) )?
int alt372=2;
int LA372_0 = input.LA(1);
if ( (LA372_0==124||(LA372_0>=247 && LA372_0<=249)) ) {
alt372=1;
}
switch (alt372) {
case 1 :
// InternalContextMappingDSL.g:16377:4: (lv_visibility_2_0= ruleVisibility )
{
// InternalContextMappingDSL.g:16377:4: (lv_visibility_2_0= ruleVisibility )
// InternalContextMappingDSL.g:16378:5: lv_visibility_2_0= ruleVisibility
{
newCompositeNode(grammarAccess.getDtoReferenceAccess().getVisibilityVisibilityEnumRuleCall_2_0());
pushFollow(FOLLOW_180);
lv_visibility_2_0=ruleVisibility();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDtoReferenceRule());
}
set(
current,
"visibility",
lv_visibility_2_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Visibility");
afterParserOrEnumRuleCall();
}
}
break;
}
// InternalContextMappingDSL.g:16395:3: ( ( (lv_collectionType_3_0= ruleCollectionType ) ) otherlv_4= '<' )?
int alt373=2;
int LA373_0 = input.LA(1);
if ( (LA373_0==233||(LA373_0>=243 && LA373_0<=246)) ) {
alt373=1;
}
switch (alt373) {
case 1 :
// InternalContextMappingDSL.g:16396:4: ( (lv_collectionType_3_0= ruleCollectionType ) ) otherlv_4= '<'
{
// InternalContextMappingDSL.g:16396:4: ( (lv_collectionType_3_0= ruleCollectionType ) )
// InternalContextMappingDSL.g:16397:5: (lv_collectionType_3_0= ruleCollectionType )
{
// InternalContextMappingDSL.g:16397:5: (lv_collectionType_3_0= ruleCollectionType )
// InternalContextMappingDSL.g:16398:6: lv_collectionType_3_0= ruleCollectionType
{
newCompositeNode(grammarAccess.getDtoReferenceAccess().getCollectionTypeCollectionTypeEnumRuleCall_3_0_0());
pushFollow(FOLLOW_126);
lv_collectionType_3_0=ruleCollectionType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getDtoReferenceRule());
}
set(
current,
"collectionType",
lv_collectionType_3_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.CollectionType");
afterParserOrEnumRuleCall();
}
}
otherlv_4=(Token)match(input,118,FOLLOW_105);
newLeafNode(otherlv_4, grammarAccess.getDtoReferenceAccess().getLessThanSignKeyword_3_1());
}
break;
}
// InternalContextMappingDSL.g:16420:3: ( (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) ) )
// InternalContextMappingDSL.g:16421:4: (otherlv_5= '@' )? ( (otherlv_6= RULE_ID ) )
{
// InternalContextMappingDSL.g:16421:4: (otherlv_5= '@' )?
int alt374=2;
int LA374_0 = input.LA(1);
if ( (LA374_0==94) ) {
alt374=1;
}
switch (alt374) {
case 1 :
// InternalContextMappingDSL.g:16422:5: otherlv_5= '@'
{
otherlv_5=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_5, grammarAccess.getDtoReferenceAccess().getCommercialAtKeyword_4_0());
}
break;
}
// InternalContextMappingDSL.g:16427:4: ( (otherlv_6= RULE_ID ) )
// InternalContextMappingDSL.g:16428:5: (otherlv_6= RULE_ID )
{
// InternalContextMappingDSL.g:16428:5: (otherlv_6= RULE_ID )
// InternalContextMappingDSL.g:16429:6: otherlv_6= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getDtoReferenceRule());
}
otherlv_6=(Token)match(input,RULE_ID,FOLLOW_184);
newLeafNode(otherlv_6, grammarAccess.getDtoReferenceAccess().getDomainObjectTypeSimpleDomainObjectCrossReference_4_1_0());
}
}
}
// InternalContextMappingDSL.g:16441:3: (otherlv_7= '>' )?
int alt375=2;
int LA375_0 = input.LA(1);
if ( (LA375_0==119) ) {
alt375=1;
}
switch (alt375) {
case 1 :
// InternalContextMappingDSL.g:16442:4: otherlv_7= '>'
{
otherlv_7=(Token)match(input,119,FOLLOW_10);
newLeafNode(otherlv_7, grammarAccess.getDtoReferenceAccess().getGreaterThanSignKeyword_5());
}
break;
}
// InternalContextMappingDSL.g:16447:3: ( (lv_name_8_0= RULE_ID ) )
// InternalContextMappingDSL.g:16448:4: (lv_name_8_0= RULE_ID )
{
// InternalContextMappingDSL.g:16448:4: (lv_name_8_0= RULE_ID )
// InternalContextMappingDSL.g:16449:5: lv_name_8_0= RULE_ID
{
lv_name_8_0=(Token)match(input,RULE_ID,FOLLOW_187);
newLeafNode(lv_name_8_0, grammarAccess.getDtoReferenceAccess().getNameIDTerminalRuleCall_6_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoReferenceRule());
}
setWithLastConsumed(
current,
"name",
lv_name_8_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:16465:3: ( ( ( ( ({...}? => ( ({...}? => ( (lv_key_10_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_22_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:16466:4: ( ( ( ({...}? => ( ({...}? => ( (lv_key_10_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_22_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:16466:4: ( ( ( ({...}? => ( ({...}? => ( (lv_key_10_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_22_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:16467:5: ( ( ({...}? => ( ({...}? => ( (lv_key_10_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_22_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7());
// InternalContextMappingDSL.g:16470:5: ( ( ({...}? => ( ({...}? => ( (lv_key_10_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_22_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) ) ) ) )* )
// InternalContextMappingDSL.g:16471:6: ( ({...}? => ( ({...}? => ( (lv_key_10_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_22_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:16471:6: ( ({...}? => ( ({...}? => ( (lv_key_10_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_22_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) ) ) ) )*
loop382:
do {
int alt382=11;
alt382 = dfa382.predict(input);
switch (alt382) {
case 1 :
// InternalContextMappingDSL.g:16472:4: ({...}? => ( ({...}? => ( (lv_key_10_0= 'key' ) ) ) ) )
{
// InternalContextMappingDSL.g:16472:4: ({...}? => ( ({...}? => ( (lv_key_10_0= 'key' ) ) ) ) )
// InternalContextMappingDSL.g:16473:5: {...}? => ( ({...}? => ( (lv_key_10_0= 'key' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 0) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 0)");
}
// InternalContextMappingDSL.g:16473:109: ( ({...}? => ( (lv_key_10_0= 'key' ) ) ) )
// InternalContextMappingDSL.g:16474:6: ({...}? => ( (lv_key_10_0= 'key' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 0);
// InternalContextMappingDSL.g:16477:9: ({...}? => ( (lv_key_10_0= 'key' ) ) )
// InternalContextMappingDSL.g:16477:10: {...}? => ( (lv_key_10_0= 'key' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "true");
}
// InternalContextMappingDSL.g:16477:19: ( (lv_key_10_0= 'key' ) )
// InternalContextMappingDSL.g:16477:20: (lv_key_10_0= 'key' )
{
// InternalContextMappingDSL.g:16477:20: (lv_key_10_0= 'key' )
// InternalContextMappingDSL.g:16478:10: lv_key_10_0= 'key'
{
lv_key_10_0=(Token)match(input,146,FOLLOW_187);
newLeafNode(lv_key_10_0, grammarAccess.getDtoReferenceAccess().getKeyKeyKeyword_7_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoReferenceRule());
}
setWithLastConsumed(current, "key", true, "key");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:16495:4: ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) ) ) )
{
// InternalContextMappingDSL.g:16495:4: ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) ) ) )
// InternalContextMappingDSL.g:16496:5: {...}? => ( ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 1) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 1)");
}
// InternalContextMappingDSL.g:16496:109: ( ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) ) )
// InternalContextMappingDSL.g:16497:6: ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 1);
// InternalContextMappingDSL.g:16500:9: ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) )
// InternalContextMappingDSL.g:16500:10: {...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "true");
}
// InternalContextMappingDSL.g:16500:19: ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' )
int alt376=2;
int LA376_0 = input.LA(1);
if ( (LA376_0==RULE_NOT) ) {
alt376=1;
}
else if ( (LA376_0==147) ) {
alt376=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 376, 0, input);
throw nvae;
}
switch (alt376) {
case 1 :
// InternalContextMappingDSL.g:16500:20: ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' )
{
// InternalContextMappingDSL.g:16500:20: ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' )
// InternalContextMappingDSL.g:16501:10: ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable'
{
// InternalContextMappingDSL.g:16501:10: ( (lv_notChangeable_11_0= RULE_NOT ) )
// InternalContextMappingDSL.g:16502:11: (lv_notChangeable_11_0= RULE_NOT )
{
// InternalContextMappingDSL.g:16502:11: (lv_notChangeable_11_0= RULE_NOT )
// InternalContextMappingDSL.g:16503:12: lv_notChangeable_11_0= RULE_NOT
{
lv_notChangeable_11_0=(Token)match(input,RULE_NOT,FOLLOW_174);
newLeafNode(lv_notChangeable_11_0, grammarAccess.getDtoReferenceAccess().getNotChangeableNOTTerminalRuleCall_7_1_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoReferenceRule());
}
setWithLastConsumed(
current,
"notChangeable",
true,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.NOT");
}
}
otherlv_12=(Token)match(input,147,FOLLOW_187);
newLeafNode(otherlv_12, grammarAccess.getDtoReferenceAccess().getChangeableKeyword_7_1_0_1());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:16525:9: otherlv_13= 'changeable'
{
otherlv_13=(Token)match(input,147,FOLLOW_187);
newLeafNode(otherlv_13, grammarAccess.getDtoReferenceAccess().getChangeableKeyword_7_1_1());
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:16535:4: ({...}? => ( ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) ) ) )
{
// InternalContextMappingDSL.g:16535:4: ({...}? => ( ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) ) ) )
// InternalContextMappingDSL.g:16536:5: {...}? => ( ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 2) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 2)");
}
// InternalContextMappingDSL.g:16536:109: ( ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) ) )
// InternalContextMappingDSL.g:16537:6: ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 2);
// InternalContextMappingDSL.g:16540:9: ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) )
// InternalContextMappingDSL.g:16540:10: {...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "true");
}
// InternalContextMappingDSL.g:16540:19: ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) )
int alt377=2;
int LA377_0 = input.LA(1);
if ( (LA377_0==148) ) {
alt377=1;
}
else if ( (LA377_0==RULE_NOT) ) {
alt377=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 377, 0, input);
throw nvae;
}
switch (alt377) {
case 1 :
// InternalContextMappingDSL.g:16540:20: ( (lv_required_14_0= 'required' ) )
{
// InternalContextMappingDSL.g:16540:20: ( (lv_required_14_0= 'required' ) )
// InternalContextMappingDSL.g:16541:10: (lv_required_14_0= 'required' )
{
// InternalContextMappingDSL.g:16541:10: (lv_required_14_0= 'required' )
// InternalContextMappingDSL.g:16542:11: lv_required_14_0= 'required'
{
lv_required_14_0=(Token)match(input,148,FOLLOW_187);
newLeafNode(lv_required_14_0, grammarAccess.getDtoReferenceAccess().getRequiredRequiredKeyword_7_2_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoReferenceRule());
}
setWithLastConsumed(current, "required", true, "required");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:16555:9: (this_NOT_15= RULE_NOT otherlv_16= 'required' )
{
// InternalContextMappingDSL.g:16555:9: (this_NOT_15= RULE_NOT otherlv_16= 'required' )
// InternalContextMappingDSL.g:16556:10: this_NOT_15= RULE_NOT otherlv_16= 'required'
{
this_NOT_15=(Token)match(input,RULE_NOT,FOLLOW_175);
newLeafNode(this_NOT_15, grammarAccess.getDtoReferenceAccess().getNOTTerminalRuleCall_7_2_1_0());
otherlv_16=(Token)match(input,148,FOLLOW_187);
newLeafNode(otherlv_16, grammarAccess.getDtoReferenceAccess().getRequiredKeyword_7_2_1_1());
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7());
}
}
}
break;
case 4 :
// InternalContextMappingDSL.g:16571:4: ({...}? => ( ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:16571:4: ({...}? => ( ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:16572:5: {...}? => ( ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 3) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 3)");
}
// InternalContextMappingDSL.g:16572:109: ( ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:16573:6: ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 3);
// InternalContextMappingDSL.g:16576:9: ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:16576:10: {...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "true");
}
// InternalContextMappingDSL.g:16576:19: ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:16576:20: ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:16576:20: ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) )
int alt378=2;
int LA378_0 = input.LA(1);
if ( (LA378_0==149) ) {
alt378=1;
}
else if ( (LA378_0==RULE_NOT) ) {
alt378=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 378, 0, input);
throw nvae;
}
switch (alt378) {
case 1 :
// InternalContextMappingDSL.g:16577:10: ( (lv_nullable_17_0= 'nullable' ) )
{
// InternalContextMappingDSL.g:16577:10: ( (lv_nullable_17_0= 'nullable' ) )
// InternalContextMappingDSL.g:16578:11: (lv_nullable_17_0= 'nullable' )
{
// InternalContextMappingDSL.g:16578:11: (lv_nullable_17_0= 'nullable' )
// InternalContextMappingDSL.g:16579:12: lv_nullable_17_0= 'nullable'
{
lv_nullable_17_0=(Token)match(input,149,FOLLOW_188);
newLeafNode(lv_nullable_17_0, grammarAccess.getDtoReferenceAccess().getNullableNullableKeyword_7_3_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoReferenceRule());
}
setWithLastConsumed(current, "nullable", true, "nullable");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:16592:10: (this_NOT_18= RULE_NOT otherlv_19= 'nullable' )
{
// InternalContextMappingDSL.g:16592:10: (this_NOT_18= RULE_NOT otherlv_19= 'nullable' )
// InternalContextMappingDSL.g:16593:11: this_NOT_18= RULE_NOT otherlv_19= 'nullable'
{
this_NOT_18=(Token)match(input,RULE_NOT,FOLLOW_177);
newLeafNode(this_NOT_18, grammarAccess.getDtoReferenceAccess().getNOTTerminalRuleCall_7_3_0_1_0());
otherlv_19=(Token)match(input,149,FOLLOW_188);
newLeafNode(otherlv_19, grammarAccess.getDtoReferenceAccess().getNullableKeyword_7_3_0_1_1());
}
}
break;
}
// InternalContextMappingDSL.g:16603:9: (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )?
int alt379=2;
int LA379_0 = input.LA(1);
if ( (LA379_0==21) ) {
alt379=1;
}
switch (alt379) {
case 1 :
// InternalContextMappingDSL.g:16604:10: otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) )
{
otherlv_20=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_20, grammarAccess.getDtoReferenceAccess().getEqualsSignKeyword_7_3_1_0());
// InternalContextMappingDSL.g:16608:10: ( (lv_nullableMessage_21_0= RULE_STRING ) )
// InternalContextMappingDSL.g:16609:11: (lv_nullableMessage_21_0= RULE_STRING )
{
// InternalContextMappingDSL.g:16609:11: (lv_nullableMessage_21_0= RULE_STRING )
// InternalContextMappingDSL.g:16610:12: lv_nullableMessage_21_0= RULE_STRING
{
lv_nullableMessage_21_0=(Token)match(input,RULE_STRING,FOLLOW_187);
newLeafNode(lv_nullableMessage_21_0, grammarAccess.getDtoReferenceAccess().getNullableMessageSTRINGTerminalRuleCall_7_3_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoReferenceRule());
}
setWithLastConsumed(
current,
"nullableMessage",
lv_nullableMessage_21_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7());
}
}
}
break;
case 5 :
// InternalContextMappingDSL.g:16633:4: ({...}? => ( ({...}? => ( (lv_transient_22_0= 'transient' ) ) ) ) )
{
// InternalContextMappingDSL.g:16633:4: ({...}? => ( ({...}? => ( (lv_transient_22_0= 'transient' ) ) ) ) )
// InternalContextMappingDSL.g:16634:5: {...}? => ( ({...}? => ( (lv_transient_22_0= 'transient' ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 4) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 4)");
}
// InternalContextMappingDSL.g:16634:109: ( ({...}? => ( (lv_transient_22_0= 'transient' ) ) ) )
// InternalContextMappingDSL.g:16635:6: ({...}? => ( (lv_transient_22_0= 'transient' ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 4);
// InternalContextMappingDSL.g:16638:9: ({...}? => ( (lv_transient_22_0= 'transient' ) ) )
// InternalContextMappingDSL.g:16638:10: {...}? => ( (lv_transient_22_0= 'transient' ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "true");
}
// InternalContextMappingDSL.g:16638:19: ( (lv_transient_22_0= 'transient' ) )
// InternalContextMappingDSL.g:16638:20: (lv_transient_22_0= 'transient' )
{
// InternalContextMappingDSL.g:16638:20: (lv_transient_22_0= 'transient' )
// InternalContextMappingDSL.g:16639:10: lv_transient_22_0= 'transient'
{
lv_transient_22_0=(Token)match(input,170,FOLLOW_187);
newLeafNode(lv_transient_22_0, grammarAccess.getDtoReferenceAccess().getTransientTransientKeyword_7_4_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoReferenceRule());
}
setWithLastConsumed(current, "transient", true, "transient");
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7());
}
}
}
break;
case 6 :
// InternalContextMappingDSL.g:16656:4: ({...}? => ( ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:16656:4: ({...}? => ( ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:16657:5: {...}? => ( ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 5) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 5)");
}
// InternalContextMappingDSL.g:16657:109: ( ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:16658:6: ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 5);
// InternalContextMappingDSL.g:16661:9: ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:16661:10: {...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "true");
}
// InternalContextMappingDSL.g:16661:19: ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:16661:20: ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:16661:20: ( (lv_notEmpty_23_0= 'notEmpty' ) )
// InternalContextMappingDSL.g:16662:10: (lv_notEmpty_23_0= 'notEmpty' )
{
// InternalContextMappingDSL.g:16662:10: (lv_notEmpty_23_0= 'notEmpty' )
// InternalContextMappingDSL.g:16663:11: lv_notEmpty_23_0= 'notEmpty'
{
lv_notEmpty_23_0=(Token)match(input,162,FOLLOW_188);
newLeafNode(lv_notEmpty_23_0, grammarAccess.getDtoReferenceAccess().getNotEmptyNotEmptyKeyword_7_5_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoReferenceRule());
}
setWithLastConsumed(current, "notEmpty", true, "notEmpty");
}
}
// InternalContextMappingDSL.g:16675:9: (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )?
int alt380=2;
int LA380_0 = input.LA(1);
if ( (LA380_0==21) ) {
alt380=1;
}
switch (alt380) {
case 1 :
// InternalContextMappingDSL.g:16676:10: otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) )
{
otherlv_24=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_24, grammarAccess.getDtoReferenceAccess().getEqualsSignKeyword_7_5_1_0());
// InternalContextMappingDSL.g:16680:10: ( (lv_notEmptyMessage_25_0= RULE_STRING ) )
// InternalContextMappingDSL.g:16681:11: (lv_notEmptyMessage_25_0= RULE_STRING )
{
// InternalContextMappingDSL.g:16681:11: (lv_notEmptyMessage_25_0= RULE_STRING )
// InternalContextMappingDSL.g:16682:12: lv_notEmptyMessage_25_0= RULE_STRING
{
lv_notEmptyMessage_25_0=(Token)match(input,RULE_STRING,FOLLOW_187);
newLeafNode(lv_notEmptyMessage_25_0, grammarAccess.getDtoReferenceAccess().getNotEmptyMessageSTRINGTerminalRuleCall_7_5_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoReferenceRule());
}
setWithLastConsumed(
current,
"notEmptyMessage",
lv_notEmptyMessage_25_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7());
}
}
}
break;
case 7 :
// InternalContextMappingDSL.g:16705:4: ({...}? => ( ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:16705:4: ({...}? => ( ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:16706:5: {...}? => ( ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 6) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 6)");
}
// InternalContextMappingDSL.g:16706:109: ( ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:16707:6: ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 6);
// InternalContextMappingDSL.g:16710:9: ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:16710:10: {...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "true");
}
// InternalContextMappingDSL.g:16710:19: (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:16710:20: otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) )
{
otherlv_26=(Token)match(input,166,FOLLOW_89);
newLeafNode(otherlv_26, grammarAccess.getDtoReferenceAccess().getSizeKeyword_7_6_0());
otherlv_27=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_27, grammarAccess.getDtoReferenceAccess().getEqualsSignKeyword_7_6_1());
// InternalContextMappingDSL.g:16718:9: ( (lv_size_28_0= RULE_STRING ) )
// InternalContextMappingDSL.g:16719:10: (lv_size_28_0= RULE_STRING )
{
// InternalContextMappingDSL.g:16719:10: (lv_size_28_0= RULE_STRING )
// InternalContextMappingDSL.g:16720:11: lv_size_28_0= RULE_STRING
{
lv_size_28_0=(Token)match(input,RULE_STRING,FOLLOW_187);
newLeafNode(lv_size_28_0, grammarAccess.getDtoReferenceAccess().getSizeSTRINGTerminalRuleCall_7_6_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoReferenceRule());
}
setWithLastConsumed(
current,
"size",
lv_size_28_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7());
}
}
}
break;
case 8 :
// InternalContextMappingDSL.g:16742:4: ({...}? => ( ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) ) ) )
{
// InternalContextMappingDSL.g:16742:4: ({...}? => ( ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) ) ) )
// InternalContextMappingDSL.g:16743:5: {...}? => ( ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 7) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 7)");
}
// InternalContextMappingDSL.g:16743:109: ( ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) ) )
// InternalContextMappingDSL.g:16744:6: ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 7);
// InternalContextMappingDSL.g:16747:9: ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) )
// InternalContextMappingDSL.g:16747:10: {...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "true");
}
// InternalContextMappingDSL.g:16747:19: ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? )
// InternalContextMappingDSL.g:16747:20: ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )?
{
// InternalContextMappingDSL.g:16747:20: ( (lv_valid_29_0= 'valid' ) )
// InternalContextMappingDSL.g:16748:10: (lv_valid_29_0= 'valid' )
{
// InternalContextMappingDSL.g:16748:10: (lv_valid_29_0= 'valid' )
// InternalContextMappingDSL.g:16749:11: lv_valid_29_0= 'valid'
{
lv_valid_29_0=(Token)match(input,178,FOLLOW_188);
newLeafNode(lv_valid_29_0, grammarAccess.getDtoReferenceAccess().getValidValidKeyword_7_7_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoReferenceRule());
}
setWithLastConsumed(current, "valid", true, "valid");
}
}
// InternalContextMappingDSL.g:16761:9: (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )?
int alt381=2;
int LA381_0 = input.LA(1);
if ( (LA381_0==21) ) {
alt381=1;
}
switch (alt381) {
case 1 :
// InternalContextMappingDSL.g:16762:10: otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) )
{
otherlv_30=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_30, grammarAccess.getDtoReferenceAccess().getEqualsSignKeyword_7_7_1_0());
// InternalContextMappingDSL.g:16766:10: ( (lv_validMessage_31_0= RULE_STRING ) )
// InternalContextMappingDSL.g:16767:11: (lv_validMessage_31_0= RULE_STRING )
{
// InternalContextMappingDSL.g:16767:11: (lv_validMessage_31_0= RULE_STRING )
// InternalContextMappingDSL.g:16768:12: lv_validMessage_31_0= RULE_STRING
{
lv_validMessage_31_0=(Token)match(input,RULE_STRING,FOLLOW_187);
newLeafNode(lv_validMessage_31_0, grammarAccess.getDtoReferenceAccess().getValidMessageSTRINGTerminalRuleCall_7_7_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoReferenceRule());
}
setWithLastConsumed(
current,
"validMessage",
lv_validMessage_31_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7());
}
}
}
break;
case 9 :
// InternalContextMappingDSL.g:16791:4: ({...}? => ( ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:16791:4: ({...}? => ( ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:16792:5: {...}? => ( ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 8) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 8)");
}
// InternalContextMappingDSL.g:16792:109: ( ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:16793:6: ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 8);
// InternalContextMappingDSL.g:16796:9: ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:16796:10: {...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "true");
}
// InternalContextMappingDSL.g:16796:19: (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:16796:20: otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) )
{
otherlv_32=(Token)match(input,133,FOLLOW_89);
newLeafNode(otherlv_32, grammarAccess.getDtoReferenceAccess().getValidateKeyword_7_8_0());
otherlv_33=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_33, grammarAccess.getDtoReferenceAccess().getEqualsSignKeyword_7_8_1());
// InternalContextMappingDSL.g:16804:9: ( (lv_validate_34_0= RULE_STRING ) )
// InternalContextMappingDSL.g:16805:10: (lv_validate_34_0= RULE_STRING )
{
// InternalContextMappingDSL.g:16805:10: (lv_validate_34_0= RULE_STRING )
// InternalContextMappingDSL.g:16806:11: lv_validate_34_0= RULE_STRING
{
lv_validate_34_0=(Token)match(input,RULE_STRING,FOLLOW_187);
newLeafNode(lv_validate_34_0, grammarAccess.getDtoReferenceAccess().getValidateSTRINGTerminalRuleCall_7_8_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoReferenceRule());
}
setWithLastConsumed(
current,
"validate",
lv_validate_34_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7());
}
}
}
break;
case 10 :
// InternalContextMappingDSL.g:16828:4: ({...}? => ( ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:16828:4: ({...}? => ( ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:16829:5: {...}? => ( ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 9) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 9)");
}
// InternalContextMappingDSL.g:16829:109: ( ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:16830:6: ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 9);
// InternalContextMappingDSL.g:16833:9: ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:16833:10: {...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleDtoReference", "true");
}
// InternalContextMappingDSL.g:16833:19: (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:16833:20: otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) )
{
otherlv_35=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_35, grammarAccess.getDtoReferenceAccess().getHintKeyword_7_9_0());
otherlv_36=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_36, grammarAccess.getDtoReferenceAccess().getEqualsSignKeyword_7_9_1());
// InternalContextMappingDSL.g:16841:9: ( (lv_hint_37_0= RULE_STRING ) )
// InternalContextMappingDSL.g:16842:10: (lv_hint_37_0= RULE_STRING )
{
// InternalContextMappingDSL.g:16842:10: (lv_hint_37_0= RULE_STRING )
// InternalContextMappingDSL.g:16843:11: lv_hint_37_0= RULE_STRING
{
lv_hint_37_0=(Token)match(input,RULE_STRING,FOLLOW_187);
newLeafNode(lv_hint_37_0, grammarAccess.getDtoReferenceAccess().getHintSTRINGTerminalRuleCall_7_9_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getDtoReferenceRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_37_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7());
}
}
}
break;
default :
break loop382;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7());
}
// InternalContextMappingDSL.g:16872:3: (otherlv_38= ';' )?
int alt383=2;
int LA383_0 = input.LA(1);
if ( (LA383_0==105) ) {
alt383=1;
}
switch (alt383) {
case 1 :
// InternalContextMappingDSL.g:16873:4: otherlv_38= ';'
{
otherlv_38=(Token)match(input,105,FOLLOW_2);
newLeafNode(otherlv_38, grammarAccess.getDtoReferenceAccess().getSemicolonKeyword_8());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleDtoReference"
// $ANTLR start "entryRuleOppositeHolder"
// InternalContextMappingDSL.g:16882:1: entryRuleOppositeHolder returns [EObject current=null] : iv_ruleOppositeHolder= ruleOppositeHolder EOF ;
public final EObject entryRuleOppositeHolder() throws RecognitionException {
EObject current = null;
EObject iv_ruleOppositeHolder = null;
try {
// InternalContextMappingDSL.g:16882:55: (iv_ruleOppositeHolder= ruleOppositeHolder EOF )
// InternalContextMappingDSL.g:16883:2: iv_ruleOppositeHolder= ruleOppositeHolder EOF
{
newCompositeNode(grammarAccess.getOppositeHolderRule());
pushFollow(FOLLOW_1);
iv_ruleOppositeHolder=ruleOppositeHolder();
state._fsp--;
current =iv_ruleOppositeHolder;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleOppositeHolder"
// $ANTLR start "ruleOppositeHolder"
// InternalContextMappingDSL.g:16889:1: ruleOppositeHolder returns [EObject current=null] : (this_OPPOSITE_0= RULE_OPPOSITE ( (otherlv_1= RULE_ID ) ) ) ;
public final EObject ruleOppositeHolder() throws RecognitionException {
EObject current = null;
Token this_OPPOSITE_0=null;
Token otherlv_1=null;
enterRule();
try {
// InternalContextMappingDSL.g:16895:2: ( (this_OPPOSITE_0= RULE_OPPOSITE ( (otherlv_1= RULE_ID ) ) ) )
// InternalContextMappingDSL.g:16896:2: (this_OPPOSITE_0= RULE_OPPOSITE ( (otherlv_1= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:16896:2: (this_OPPOSITE_0= RULE_OPPOSITE ( (otherlv_1= RULE_ID ) ) )
// InternalContextMappingDSL.g:16897:3: this_OPPOSITE_0= RULE_OPPOSITE ( (otherlv_1= RULE_ID ) )
{
this_OPPOSITE_0=(Token)match(input,RULE_OPPOSITE,FOLLOW_10);
newLeafNode(this_OPPOSITE_0, grammarAccess.getOppositeHolderAccess().getOPPOSITETerminalRuleCall_0());
// InternalContextMappingDSL.g:16901:3: ( (otherlv_1= RULE_ID ) )
// InternalContextMappingDSL.g:16902:4: (otherlv_1= RULE_ID )
{
// InternalContextMappingDSL.g:16902:4: (otherlv_1= RULE_ID )
// InternalContextMappingDSL.g:16903:5: otherlv_1= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getOppositeHolderRule());
}
otherlv_1=(Token)match(input,RULE_ID,FOLLOW_2);
newLeafNode(otherlv_1, grammarAccess.getOppositeHolderAccess().getOppositeReferenceCrossReference_1_0());
}
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleOppositeHolder"
// $ANTLR start "entryRuleRepository"
// InternalContextMappingDSL.g:16918:1: entryRuleRepository returns [EObject current=null] : iv_ruleRepository= ruleRepository EOF ;
public final EObject entryRuleRepository() throws RecognitionException {
EObject current = null;
EObject iv_ruleRepository = null;
try {
// InternalContextMappingDSL.g:16918:51: (iv_ruleRepository= ruleRepository EOF )
// InternalContextMappingDSL.g:16919:2: iv_ruleRepository= ruleRepository EOF
{
newCompositeNode(grammarAccess.getRepositoryRule());
pushFollow(FOLLOW_1);
iv_ruleRepository=ruleRepository();
state._fsp--;
current =iv_ruleRepository;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleRepository"
// $ANTLR start "ruleRepository"
// InternalContextMappingDSL.g:16925:1: ruleRepository returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Repository' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) ) ) )* ) ) ) ( (lv_dependencies_11_0= ruleDependency ) )* ( (lv_operations_12_0= ruleRepositoryOperation ) )* otherlv_13= '}' )? ) ;
public final EObject ruleRepository() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token otherlv_1=null;
Token lv_name_2_0=null;
Token otherlv_3=null;
Token lv_gapClass_5_0=null;
Token lv_noGapClass_6_0=null;
Token otherlv_7=null;
Token otherlv_8=null;
Token lv_hint_9_0=null;
Token otherlv_13=null;
EObject lv_subscribe_10_0 = null;
EObject lv_dependencies_11_0 = null;
EObject lv_operations_12_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:16931:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Repository' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) ) ) )* ) ) ) ( (lv_dependencies_11_0= ruleDependency ) )* ( (lv_operations_12_0= ruleRepositoryOperation ) )* otherlv_13= '}' )? ) )
// InternalContextMappingDSL.g:16932:2: ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Repository' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) ) ) )* ) ) ) ( (lv_dependencies_11_0= ruleDependency ) )* ( (lv_operations_12_0= ruleRepositoryOperation ) )* otherlv_13= '}' )? )
{
// InternalContextMappingDSL.g:16932:2: ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Repository' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) ) ) )* ) ) ) ( (lv_dependencies_11_0= ruleDependency ) )* ( (lv_operations_12_0= ruleRepositoryOperation ) )* otherlv_13= '}' )? )
// InternalContextMappingDSL.g:16933:3: ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'Repository' ( (lv_name_2_0= RULE_ID ) ) (otherlv_3= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) ) ) )* ) ) ) ( (lv_dependencies_11_0= ruleDependency ) )* ( (lv_operations_12_0= ruleRepositoryOperation ) )* otherlv_13= '}' )?
{
// InternalContextMappingDSL.g:16933:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt384=2;
int LA384_0 = input.LA(1);
if ( (LA384_0==RULE_STRING) ) {
alt384=1;
}
switch (alt384) {
case 1 :
// InternalContextMappingDSL.g:16934:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:16934:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:16935:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_189);
newLeafNode(lv_doc_0_0, grammarAccess.getRepositoryAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
otherlv_1=(Token)match(input,181,FOLLOW_10);
newLeafNode(otherlv_1, grammarAccess.getRepositoryAccess().getRepositoryKeyword_1());
// InternalContextMappingDSL.g:16955:3: ( (lv_name_2_0= RULE_ID ) )
// InternalContextMappingDSL.g:16956:4: (lv_name_2_0= RULE_ID )
{
// InternalContextMappingDSL.g:16956:4: (lv_name_2_0= RULE_ID )
// InternalContextMappingDSL.g:16957:5: lv_name_2_0= RULE_ID
{
lv_name_2_0=(Token)match(input,RULE_ID,FOLLOW_23);
newLeafNode(lv_name_2_0, grammarAccess.getRepositoryAccess().getNameIDTerminalRuleCall_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryRule());
}
setWithLastConsumed(
current,
"name",
lv_name_2_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:16973:3: (otherlv_3= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) ) ) )* ) ) ) ( (lv_dependencies_11_0= ruleDependency ) )* ( (lv_operations_12_0= ruleRepositoryOperation ) )* otherlv_13= '}' )?
int alt389=2;
int LA389_0 = input.LA(1);
if ( (LA389_0==RULE_OPEN) ) {
alt389=1;
}
switch (alt389) {
case 1 :
// InternalContextMappingDSL.g:16974:4: otherlv_3= '{' ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) ) ) )* ) ) ) ( (lv_dependencies_11_0= ruleDependency ) )* ( (lv_operations_12_0= ruleRepositoryOperation ) )* otherlv_13= '}'
{
otherlv_3=(Token)match(input,RULE_OPEN,FOLLOW_96);
newLeafNode(otherlv_3, grammarAccess.getRepositoryAccess().getLeftCurlyBracketKeyword_3_0());
// InternalContextMappingDSL.g:16978:4: ( ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) ) ) )* ) ) )
// InternalContextMappingDSL.g:16979:5: ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) ) ) )* ) )
{
// InternalContextMappingDSL.g:16979:5: ( ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) ) ) )* ) )
// InternalContextMappingDSL.g:16980:6: ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) ) ) )* )
{
getUnorderedGroupHelper().enter(grammarAccess.getRepositoryAccess().getUnorderedGroup_3_1());
// InternalContextMappingDSL.g:16983:6: ( ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) ) ) )* )
// InternalContextMappingDSL.g:16984:7: ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) ) ) )*
{
// InternalContextMappingDSL.g:16984:7: ( ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) ) ) )*
loop386:
do {
int alt386=4;
int LA386_0 = input.LA(1);
if ( LA386_0 >= 86 && LA386_0 <= 87 && getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryAccess().getUnorderedGroup_3_1(), 0) ) {
alt386=1;
}
else if ( LA386_0 == 82 && getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryAccess().getUnorderedGroup_3_1(), 1) ) {
alt386=2;
}
else if ( LA386_0 == 97 && getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryAccess().getUnorderedGroup_3_1(), 2) ) {
alt386=3;
}
switch (alt386) {
case 1 :
// InternalContextMappingDSL.g:16985:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) )
{
// InternalContextMappingDSL.g:16985:5: ({...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) ) )
// InternalContextMappingDSL.g:16986:6: {...}? => ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryAccess().getUnorderedGroup_3_1(), 0) ) {
throw new FailedPredicateException(input, "ruleRepository", "getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryAccess().getUnorderedGroup_3_1(), 0)");
}
// InternalContextMappingDSL.g:16986:110: ( ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) ) )
// InternalContextMappingDSL.g:16987:7: ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getRepositoryAccess().getUnorderedGroup_3_1(), 0);
// InternalContextMappingDSL.g:16990:10: ({...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) ) )
// InternalContextMappingDSL.g:16990:11: {...}? => ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleRepository", "true");
}
// InternalContextMappingDSL.g:16990:20: ( ( (lv_gapClass_5_0= 'gap' ) ) | ( (lv_noGapClass_6_0= 'nogap' ) ) )
int alt385=2;
int LA385_0 = input.LA(1);
if ( (LA385_0==86) ) {
alt385=1;
}
else if ( (LA385_0==87) ) {
alt385=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 385, 0, input);
throw nvae;
}
switch (alt385) {
case 1 :
// InternalContextMappingDSL.g:16990:21: ( (lv_gapClass_5_0= 'gap' ) )
{
// InternalContextMappingDSL.g:16990:21: ( (lv_gapClass_5_0= 'gap' ) )
// InternalContextMappingDSL.g:16991:11: (lv_gapClass_5_0= 'gap' )
{
// InternalContextMappingDSL.g:16991:11: (lv_gapClass_5_0= 'gap' )
// InternalContextMappingDSL.g:16992:12: lv_gapClass_5_0= 'gap'
{
lv_gapClass_5_0=(Token)match(input,86,FOLLOW_96);
newLeafNode(lv_gapClass_5_0, grammarAccess.getRepositoryAccess().getGapClassGapKeyword_3_1_0_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryRule());
}
setWithLastConsumed(current, "gapClass", true, "gap");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:17005:10: ( (lv_noGapClass_6_0= 'nogap' ) )
{
// InternalContextMappingDSL.g:17005:10: ( (lv_noGapClass_6_0= 'nogap' ) )
// InternalContextMappingDSL.g:17006:11: (lv_noGapClass_6_0= 'nogap' )
{
// InternalContextMappingDSL.g:17006:11: (lv_noGapClass_6_0= 'nogap' )
// InternalContextMappingDSL.g:17007:12: lv_noGapClass_6_0= 'nogap'
{
lv_noGapClass_6_0=(Token)match(input,87,FOLLOW_96);
newLeafNode(lv_noGapClass_6_0, grammarAccess.getRepositoryAccess().getNoGapClassNogapKeyword_3_1_0_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryRule());
}
setWithLastConsumed(current, "noGapClass", true, "nogap");
}
}
}
break;
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getRepositoryAccess().getUnorderedGroup_3_1());
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:17025:5: ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) )
{
// InternalContextMappingDSL.g:17025:5: ({...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) ) )
// InternalContextMappingDSL.g:17026:6: {...}? => ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryAccess().getUnorderedGroup_3_1(), 1) ) {
throw new FailedPredicateException(input, "ruleRepository", "getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryAccess().getUnorderedGroup_3_1(), 1)");
}
// InternalContextMappingDSL.g:17026:110: ( ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) ) )
// InternalContextMappingDSL.g:17027:7: ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getRepositoryAccess().getUnorderedGroup_3_1(), 1);
// InternalContextMappingDSL.g:17030:10: ({...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) ) )
// InternalContextMappingDSL.g:17030:11: {...}? => (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleRepository", "true");
}
// InternalContextMappingDSL.g:17030:20: (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )
// InternalContextMappingDSL.g:17030:21: otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) )
{
otherlv_7=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_7, grammarAccess.getRepositoryAccess().getHintKeyword_3_1_1_0());
otherlv_8=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_8, grammarAccess.getRepositoryAccess().getEqualsSignKeyword_3_1_1_1());
// InternalContextMappingDSL.g:17038:10: ( (lv_hint_9_0= RULE_STRING ) )
// InternalContextMappingDSL.g:17039:11: (lv_hint_9_0= RULE_STRING )
{
// InternalContextMappingDSL.g:17039:11: (lv_hint_9_0= RULE_STRING )
// InternalContextMappingDSL.g:17040:12: lv_hint_9_0= RULE_STRING
{
lv_hint_9_0=(Token)match(input,RULE_STRING,FOLLOW_96);
newLeafNode(lv_hint_9_0, grammarAccess.getRepositoryAccess().getHintSTRINGTerminalRuleCall_3_1_1_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getRepositoryRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_9_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getRepositoryAccess().getUnorderedGroup_3_1());
}
}
}
break;
case 3 :
// InternalContextMappingDSL.g:17062:5: ({...}? => ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) ) )
{
// InternalContextMappingDSL.g:17062:5: ({...}? => ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) ) )
// InternalContextMappingDSL.g:17063:6: {...}? => ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) )
{
if ( ! getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryAccess().getUnorderedGroup_3_1(), 2) ) {
throw new FailedPredicateException(input, "ruleRepository", "getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryAccess().getUnorderedGroup_3_1(), 2)");
}
// InternalContextMappingDSL.g:17063:110: ( ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) ) )
// InternalContextMappingDSL.g:17064:7: ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) )
{
getUnorderedGroupHelper().select(grammarAccess.getRepositoryAccess().getUnorderedGroup_3_1(), 2);
// InternalContextMappingDSL.g:17067:10: ({...}? => ( (lv_subscribe_10_0= ruleSubscribe ) ) )
// InternalContextMappingDSL.g:17067:11: {...}? => ( (lv_subscribe_10_0= ruleSubscribe ) )
{
if ( !((true)) ) {
throw new FailedPredicateException(input, "ruleRepository", "true");
}
// InternalContextMappingDSL.g:17067:20: ( (lv_subscribe_10_0= ruleSubscribe ) )
// InternalContextMappingDSL.g:17067:21: (lv_subscribe_10_0= ruleSubscribe )
{
// InternalContextMappingDSL.g:17067:21: (lv_subscribe_10_0= ruleSubscribe )
// InternalContextMappingDSL.g:17068:11: lv_subscribe_10_0= ruleSubscribe
{
newCompositeNode(grammarAccess.getRepositoryAccess().getSubscribeSubscribeParserRuleCall_3_1_2_0());
pushFollow(FOLLOW_96);
lv_subscribe_10_0=ruleSubscribe();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getRepositoryRule());
}
set(
current,
"subscribe",
lv_subscribe_10_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Subscribe");
afterParserOrEnumRuleCall();
}
}
}
getUnorderedGroupHelper().returnFromSelection(grammarAccess.getRepositoryAccess().getUnorderedGroup_3_1());
}
}
}
break;
default :
break loop386;
}
} while (true);
}
}
getUnorderedGroupHelper().leave(grammarAccess.getRepositoryAccess().getUnorderedGroup_3_1());
}
// InternalContextMappingDSL.g:17097:4: ( (lv_dependencies_11_0= ruleDependency ) )*
loop387:
do {
int alt387=2;
int LA387_0 = input.LA(1);
if ( (LA387_0==119||LA387_0==182) ) {
alt387=1;
}
switch (alt387) {
case 1 :
// InternalContextMappingDSL.g:17098:5: (lv_dependencies_11_0= ruleDependency )
{
// InternalContextMappingDSL.g:17098:5: (lv_dependencies_11_0= ruleDependency )
// InternalContextMappingDSL.g:17099:6: lv_dependencies_11_0= ruleDependency
{
newCompositeNode(grammarAccess.getRepositoryAccess().getDependenciesDependencyParserRuleCall_3_2_0());
pushFollow(FOLLOW_97);
lv_dependencies_11_0=ruleDependency();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getRepositoryRule());
}
add(
current,
"dependencies",
lv_dependencies_11_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Dependency");
afterParserOrEnumRuleCall();
}
}
break;
default :
break loop387;
}
} while (true);
// InternalContextMappingDSL.g:17116:4: ( (lv_operations_12_0= ruleRepositoryOperation ) )*
loop388:
do {
int alt388=2;
int LA388_0 = input.LA(1);
if ( ((LA388_0>=RULE_STRING && LA388_0<=RULE_ID)||LA388_0==RULE_MAP_COLLECTION_TYPE||LA388_0==94||LA388_0==101||LA388_0==124||(LA388_0>=185 && LA388_0<=206)||LA388_0==233||(LA388_0>=243 && LA388_0<=249)) ) {
alt388=1;
}
switch (alt388) {
case 1 :
// InternalContextMappingDSL.g:17117:5: (lv_operations_12_0= ruleRepositoryOperation )
{
// InternalContextMappingDSL.g:17117:5: (lv_operations_12_0= ruleRepositoryOperation )
// InternalContextMappingDSL.g:17118:6: lv_operations_12_0= ruleRepositoryOperation
{
newCompositeNode(grammarAccess.getRepositoryAccess().getOperationsRepositoryOperationParserRuleCall_3_3_0());
pushFollow(FOLLOW_98);
lv_operations_12_0=ruleRepositoryOperation();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getRepositoryRule());
}
add(
current,
"operations",
lv_operations_12_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.RepositoryOperation");
afterParserOrEnumRuleCall();
}
}
break;
default :
break loop388;
}
} while (true);
otherlv_13=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(otherlv_13, grammarAccess.getRepositoryAccess().getRightCurlyBracketKeyword_3_4());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleRepository"
// $ANTLR start "entryRuleServiceDependency"
// InternalContextMappingDSL.g:17144:1: entryRuleServiceDependency returns [EObject current=null] : iv_ruleServiceDependency= ruleServiceDependency EOF ;
public final EObject entryRuleServiceDependency() throws RecognitionException {
EObject current = null;
EObject iv_ruleServiceDependency = null;
try {
// InternalContextMappingDSL.g:17144:58: (iv_ruleServiceDependency= ruleServiceDependency EOF )
// InternalContextMappingDSL.g:17145:2: iv_ruleServiceDependency= ruleServiceDependency EOF
{
newCompositeNode(grammarAccess.getServiceDependencyRule());
pushFollow(FOLLOW_1);
iv_ruleServiceDependency=ruleServiceDependency();
state._fsp--;
current =iv_ruleServiceDependency;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleServiceDependency"
// $ANTLR start "ruleServiceDependency"
// InternalContextMappingDSL.g:17151:1: ruleServiceDependency returns [EObject current=null] : ( (otherlv_0= '>' | otherlv_1= 'inject' ) (otherlv_2= '@' ( (otherlv_3= RULE_ID ) ) ) ) ;
public final EObject ruleServiceDependency() throws RecognitionException {
EObject current = null;
Token otherlv_0=null;
Token otherlv_1=null;
Token otherlv_2=null;
Token otherlv_3=null;
enterRule();
try {
// InternalContextMappingDSL.g:17157:2: ( ( (otherlv_0= '>' | otherlv_1= 'inject' ) (otherlv_2= '@' ( (otherlv_3= RULE_ID ) ) ) ) )
// InternalContextMappingDSL.g:17158:2: ( (otherlv_0= '>' | otherlv_1= 'inject' ) (otherlv_2= '@' ( (otherlv_3= RULE_ID ) ) ) )
{
// InternalContextMappingDSL.g:17158:2: ( (otherlv_0= '>' | otherlv_1= 'inject' ) (otherlv_2= '@' ( (otherlv_3= RULE_ID ) ) ) )
// InternalContextMappingDSL.g:17159:3: (otherlv_0= '>' | otherlv_1= 'inject' ) (otherlv_2= '@' ( (otherlv_3= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:17159:3: (otherlv_0= '>' | otherlv_1= 'inject' )
int alt390=2;
int LA390_0 = input.LA(1);
if ( (LA390_0==119) ) {
alt390=1;
}
else if ( (LA390_0==182) ) {
alt390=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 390, 0, input);
throw nvae;
}
switch (alt390) {
case 1 :
// InternalContextMappingDSL.g:17160:4: otherlv_0= '>'
{
otherlv_0=(Token)match(input,119,FOLLOW_127);
newLeafNode(otherlv_0, grammarAccess.getServiceDependencyAccess().getGreaterThanSignKeyword_0_0());
}
break;
case 2 :
// InternalContextMappingDSL.g:17165:4: otherlv_1= 'inject'
{
otherlv_1=(Token)match(input,182,FOLLOW_127);
newLeafNode(otherlv_1, grammarAccess.getServiceDependencyAccess().getInjectKeyword_0_1());
}
break;
}
// InternalContextMappingDSL.g:17170:3: (otherlv_2= '@' ( (otherlv_3= RULE_ID ) ) )
// InternalContextMappingDSL.g:17171:4: otherlv_2= '@' ( (otherlv_3= RULE_ID ) )
{
otherlv_2=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_2, grammarAccess.getServiceDependencyAccess().getCommercialAtKeyword_1_0());
// InternalContextMappingDSL.g:17175:4: ( (otherlv_3= RULE_ID ) )
// InternalContextMappingDSL.g:17176:5: (otherlv_3= RULE_ID )
{
// InternalContextMappingDSL.g:17176:5: (otherlv_3= RULE_ID )
// InternalContextMappingDSL.g:17177:6: otherlv_3= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getServiceDependencyRule());
}
otherlv_3=(Token)match(input,RULE_ID,FOLLOW_2);
newLeafNode(otherlv_3, grammarAccess.getServiceDependencyAccess().getDependencyServiceCrossReference_1_1_0());
}
}
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleServiceDependency"
// $ANTLR start "entryRuleDependency"
// InternalContextMappingDSL.g:17193:1: entryRuleDependency returns [EObject current=null] : iv_ruleDependency= ruleDependency EOF ;
public final EObject entryRuleDependency() throws RecognitionException {
EObject current = null;
EObject iv_ruleDependency = null;
try {
// InternalContextMappingDSL.g:17193:51: (iv_ruleDependency= ruleDependency EOF )
// InternalContextMappingDSL.g:17194:2: iv_ruleDependency= ruleDependency EOF
{
newCompositeNode(grammarAccess.getDependencyRule());
pushFollow(FOLLOW_1);
iv_ruleDependency=ruleDependency();
state._fsp--;
current =iv_ruleDependency;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleDependency"
// $ANTLR start "ruleDependency"
// InternalContextMappingDSL.g:17200:1: ruleDependency returns [EObject current=null] : ( (otherlv_0= '>' | otherlv_1= 'inject' ) ( (otherlv_2= '@' ( (otherlv_3= RULE_ID ) ) ) | ( (lv_name_4_0= RULE_ID ) ) ) ) ;
public final EObject ruleDependency() throws RecognitionException {
EObject current = null;
Token otherlv_0=null;
Token otherlv_1=null;
Token otherlv_2=null;
Token otherlv_3=null;
Token lv_name_4_0=null;
enterRule();
try {
// InternalContextMappingDSL.g:17206:2: ( ( (otherlv_0= '>' | otherlv_1= 'inject' ) ( (otherlv_2= '@' ( (otherlv_3= RULE_ID ) ) ) | ( (lv_name_4_0= RULE_ID ) ) ) ) )
// InternalContextMappingDSL.g:17207:2: ( (otherlv_0= '>' | otherlv_1= 'inject' ) ( (otherlv_2= '@' ( (otherlv_3= RULE_ID ) ) ) | ( (lv_name_4_0= RULE_ID ) ) ) )
{
// InternalContextMappingDSL.g:17207:2: ( (otherlv_0= '>' | otherlv_1= 'inject' ) ( (otherlv_2= '@' ( (otherlv_3= RULE_ID ) ) ) | ( (lv_name_4_0= RULE_ID ) ) ) )
// InternalContextMappingDSL.g:17208:3: (otherlv_0= '>' | otherlv_1= 'inject' ) ( (otherlv_2= '@' ( (otherlv_3= RULE_ID ) ) ) | ( (lv_name_4_0= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:17208:3: (otherlv_0= '>' | otherlv_1= 'inject' )
int alt391=2;
int LA391_0 = input.LA(1);
if ( (LA391_0==119) ) {
alt391=1;
}
else if ( (LA391_0==182) ) {
alt391=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 391, 0, input);
throw nvae;
}
switch (alt391) {
case 1 :
// InternalContextMappingDSL.g:17209:4: otherlv_0= '>'
{
otherlv_0=(Token)match(input,119,FOLLOW_105);
newLeafNode(otherlv_0, grammarAccess.getDependencyAccess().getGreaterThanSignKeyword_0_0());
}
break;
case 2 :
// InternalContextMappingDSL.g:17214:4: otherlv_1= 'inject'
{
otherlv_1=(Token)match(input,182,FOLLOW_105);
newLeafNode(otherlv_1, grammarAccess.getDependencyAccess().getInjectKeyword_0_1());
}
break;
}
// InternalContextMappingDSL.g:17219:3: ( (otherlv_2= '@' ( (otherlv_3= RULE_ID ) ) ) | ( (lv_name_4_0= RULE_ID ) ) )
int alt392=2;
int LA392_0 = input.LA(1);
if ( (LA392_0==94) ) {
alt392=1;
}
else if ( (LA392_0==RULE_ID) ) {
alt392=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 392, 0, input);
throw nvae;
}
switch (alt392) {
case 1 :
// InternalContextMappingDSL.g:17220:4: (otherlv_2= '@' ( (otherlv_3= RULE_ID ) ) )
{
// InternalContextMappingDSL.g:17220:4: (otherlv_2= '@' ( (otherlv_3= RULE_ID ) ) )
// InternalContextMappingDSL.g:17221:5: otherlv_2= '@' ( (otherlv_3= RULE_ID ) )
{
otherlv_2=(Token)match(input,94,FOLLOW_10);
newLeafNode(otherlv_2, grammarAccess.getDependencyAccess().getCommercialAtKeyword_1_0_0());
// InternalContextMappingDSL.g:17225:5: ( (otherlv_3= RULE_ID ) )
// InternalContextMappingDSL.g:17226:6: (otherlv_3= RULE_ID )
{
// InternalContextMappingDSL.g:17226:6: (otherlv_3= RULE_ID )
// InternalContextMappingDSL.g:17227:7: otherlv_3= RULE_ID
{
if (current==null) {
current = createModelElement(grammarAccess.getDependencyRule());
}
otherlv_3=(Token)match(input,RULE_ID,FOLLOW_2);
newLeafNode(otherlv_3, grammarAccess.getDependencyAccess().getDependencyServiceRepositoryOptionCrossReference_1_0_1_0());
}
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:17240:4: ( (lv_name_4_0= RULE_ID ) )
{
// InternalContextMappingDSL.g:17240:4: ( (lv_name_4_0= RULE_ID ) )
// InternalContextMappingDSL.g:17241:5: (lv_name_4_0= RULE_ID )
{
// InternalContextMappingDSL.g:17241:5: (lv_name_4_0= RULE_ID )
// InternalContextMappingDSL.g:17242:6: lv_name_4_0= RULE_ID
{
lv_name_4_0=(Token)match(input,RULE_ID,FOLLOW_2);
newLeafNode(lv_name_4_0, grammarAccess.getDependencyAccess().getNameIDTerminalRuleCall_1_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getDependencyRule());
}
setWithLastConsumed(
current,
"name",
lv_name_4_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleDependency"
// $ANTLR start "entryRuleEnum"
// InternalContextMappingDSL.g:17263:1: entryRuleEnum returns [EObject current=null] : iv_ruleEnum= ruleEnum EOF ;
public final EObject entryRuleEnum() throws RecognitionException {
EObject current = null;
EObject iv_ruleEnum = null;
try {
// InternalContextMappingDSL.g:17263:45: (iv_ruleEnum= ruleEnum EOF )
// InternalContextMappingDSL.g:17264:2: iv_ruleEnum= ruleEnum EOF
{
newCompositeNode(grammarAccess.getEnumRule());
pushFollow(FOLLOW_1);
iv_ruleEnum=ruleEnum();
state._fsp--;
current =iv_ruleEnum;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleEnum"
// $ANTLR start "ruleEnum"
// InternalContextMappingDSL.g:17270:1: ruleEnum returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'enum' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' (otherlv_4= 'package' otherlv_5= '=' ( (lv_package_6_0= ruleJavaIdentifier ) ) )? (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )? ( (lv_ordinal_10_0= 'ordinal' ) )? ( (lv_attributes_11_0= ruleEnumAttribute ) )* ( (lv_values_12_0= ruleEnumValue ) ) (otherlv_13= ',' ( (lv_values_14_0= ruleEnumValue ) ) )* (otherlv_15= ';' )? otherlv_16= '}' ) ;
public final EObject ruleEnum() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token otherlv_1=null;
Token lv_name_2_0=null;
Token otherlv_3=null;
Token otherlv_4=null;
Token otherlv_5=null;
Token otherlv_7=null;
Token otherlv_8=null;
Token lv_hint_9_0=null;
Token lv_ordinal_10_0=null;
Token otherlv_13=null;
Token otherlv_15=null;
Token otherlv_16=null;
AntlrDatatypeRuleToken lv_package_6_0 = null;
EObject lv_attributes_11_0 = null;
EObject lv_values_12_0 = null;
EObject lv_values_14_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:17276:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'enum' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' (otherlv_4= 'package' otherlv_5= '=' ( (lv_package_6_0= ruleJavaIdentifier ) ) )? (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )? ( (lv_ordinal_10_0= 'ordinal' ) )? ( (lv_attributes_11_0= ruleEnumAttribute ) )* ( (lv_values_12_0= ruleEnumValue ) ) (otherlv_13= ',' ( (lv_values_14_0= ruleEnumValue ) ) )* (otherlv_15= ';' )? otherlv_16= '}' ) )
// InternalContextMappingDSL.g:17277:2: ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'enum' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' (otherlv_4= 'package' otherlv_5= '=' ( (lv_package_6_0= ruleJavaIdentifier ) ) )? (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )? ( (lv_ordinal_10_0= 'ordinal' ) )? ( (lv_attributes_11_0= ruleEnumAttribute ) )* ( (lv_values_12_0= ruleEnumValue ) ) (otherlv_13= ',' ( (lv_values_14_0= ruleEnumValue ) ) )* (otherlv_15= ';' )? otherlv_16= '}' )
{
// InternalContextMappingDSL.g:17277:2: ( ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'enum' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' (otherlv_4= 'package' otherlv_5= '=' ( (lv_package_6_0= ruleJavaIdentifier ) ) )? (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )? ( (lv_ordinal_10_0= 'ordinal' ) )? ( (lv_attributes_11_0= ruleEnumAttribute ) )* ( (lv_values_12_0= ruleEnumValue ) ) (otherlv_13= ',' ( (lv_values_14_0= ruleEnumValue ) ) )* (otherlv_15= ';' )? otherlv_16= '}' )
// InternalContextMappingDSL.g:17278:3: ( (lv_doc_0_0= RULE_STRING ) )? otherlv_1= 'enum' ( (lv_name_2_0= RULE_ID ) ) otherlv_3= '{' (otherlv_4= 'package' otherlv_5= '=' ( (lv_package_6_0= ruleJavaIdentifier ) ) )? (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )? ( (lv_ordinal_10_0= 'ordinal' ) )? ( (lv_attributes_11_0= ruleEnumAttribute ) )* ( (lv_values_12_0= ruleEnumValue ) ) (otherlv_13= ',' ( (lv_values_14_0= ruleEnumValue ) ) )* (otherlv_15= ';' )? otherlv_16= '}'
{
// InternalContextMappingDSL.g:17278:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt393=2;
int LA393_0 = input.LA(1);
if ( (LA393_0==RULE_STRING) ) {
alt393=1;
}
switch (alt393) {
case 1 :
// InternalContextMappingDSL.g:17279:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:17279:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:17280:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_190);
newLeafNode(lv_doc_0_0, grammarAccess.getEnumAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getEnumRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
otherlv_1=(Token)match(input,183,FOLLOW_10);
newLeafNode(otherlv_1, grammarAccess.getEnumAccess().getEnumKeyword_1());
// InternalContextMappingDSL.g:17300:3: ( (lv_name_2_0= RULE_ID ) )
// InternalContextMappingDSL.g:17301:4: (lv_name_2_0= RULE_ID )
{
// InternalContextMappingDSL.g:17301:4: (lv_name_2_0= RULE_ID )
// InternalContextMappingDSL.g:17302:5: lv_name_2_0= RULE_ID
{
lv_name_2_0=(Token)match(input,RULE_ID,FOLLOW_6);
newLeafNode(lv_name_2_0, grammarAccess.getEnumAccess().getNameIDTerminalRuleCall_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getEnumRule());
}
setWithLastConsumed(
current,
"name",
lv_name_2_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
otherlv_3=(Token)match(input,RULE_OPEN,FOLLOW_191);
newLeafNode(otherlv_3, grammarAccess.getEnumAccess().getLeftCurlyBracketKeyword_3());
// InternalContextMappingDSL.g:17322:3: (otherlv_4= 'package' otherlv_5= '=' ( (lv_package_6_0= ruleJavaIdentifier ) ) )?
int alt394=2;
int LA394_0 = input.LA(1);
if ( (LA394_0==124) ) {
alt394=1;
}
switch (alt394) {
case 1 :
// InternalContextMappingDSL.g:17323:4: otherlv_4= 'package' otherlv_5= '=' ( (lv_package_6_0= ruleJavaIdentifier ) )
{
otherlv_4=(Token)match(input,124,FOLLOW_89);
newLeafNode(otherlv_4, grammarAccess.getEnumAccess().getPackageKeyword_4_0());
otherlv_5=(Token)match(input,21,FOLLOW_10);
newLeafNode(otherlv_5, grammarAccess.getEnumAccess().getEqualsSignKeyword_4_1());
// InternalContextMappingDSL.g:17331:4: ( (lv_package_6_0= ruleJavaIdentifier ) )
// InternalContextMappingDSL.g:17332:5: (lv_package_6_0= ruleJavaIdentifier )
{
// InternalContextMappingDSL.g:17332:5: (lv_package_6_0= ruleJavaIdentifier )
// InternalContextMappingDSL.g:17333:6: lv_package_6_0= ruleJavaIdentifier
{
newCompositeNode(grammarAccess.getEnumAccess().getPackageJavaIdentifierParserRuleCall_4_2_0());
pushFollow(FOLLOW_191);
lv_package_6_0=ruleJavaIdentifier();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getEnumRule());
}
set(
current,
"package",
lv_package_6_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.JavaIdentifier");
afterParserOrEnumRuleCall();
}
}
}
break;
}
// InternalContextMappingDSL.g:17351:3: (otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) ) )?
int alt395=2;
int LA395_0 = input.LA(1);
if ( (LA395_0==82) ) {
alt395=1;
}
switch (alt395) {
case 1 :
// InternalContextMappingDSL.g:17352:4: otherlv_7= 'hint' otherlv_8= '=' ( (lv_hint_9_0= RULE_STRING ) )
{
otherlv_7=(Token)match(input,82,FOLLOW_89);
newLeafNode(otherlv_7, grammarAccess.getEnumAccess().getHintKeyword_5_0());
otherlv_8=(Token)match(input,21,FOLLOW_4);
newLeafNode(otherlv_8, grammarAccess.getEnumAccess().getEqualsSignKeyword_5_1());
// InternalContextMappingDSL.g:17360:4: ( (lv_hint_9_0= RULE_STRING ) )
// InternalContextMappingDSL.g:17361:5: (lv_hint_9_0= RULE_STRING )
{
// InternalContextMappingDSL.g:17361:5: (lv_hint_9_0= RULE_STRING )
// InternalContextMappingDSL.g:17362:6: lv_hint_9_0= RULE_STRING
{
lv_hint_9_0=(Token)match(input,RULE_STRING,FOLLOW_191);
newLeafNode(lv_hint_9_0, grammarAccess.getEnumAccess().getHintSTRINGTerminalRuleCall_5_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getEnumRule());
}
setWithLastConsumed(
current,
"hint",
lv_hint_9_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
}
// InternalContextMappingDSL.g:17379:3: ( (lv_ordinal_10_0= 'ordinal' ) )?
int alt396=2;
int LA396_0 = input.LA(1);
if ( (LA396_0==184) ) {
alt396=1;
}
switch (alt396) {
case 1 :
// InternalContextMappingDSL.g:17380:4: (lv_ordinal_10_0= 'ordinal' )
{
// InternalContextMappingDSL.g:17380:4: (lv_ordinal_10_0= 'ordinal' )
// InternalContextMappingDSL.g:17381:5: lv_ordinal_10_0= 'ordinal'
{
lv_ordinal_10_0=(Token)match(input,184,FOLLOW_191);
newLeafNode(lv_ordinal_10_0, grammarAccess.getEnumAccess().getOrdinalOrdinalKeyword_6_0());
if (current==null) {
current = createModelElement(grammarAccess.getEnumRule());
}
setWithLastConsumed(current, "ordinal", true, "ordinal");
}
}
break;
}
// InternalContextMappingDSL.g:17393:3: ( (lv_attributes_11_0= ruleEnumAttribute ) )*
loop397:
do {
int alt397=2;
switch ( input.LA(1) ) {
case RULE_STRING:
{
int LA397_1 = input.LA(2);
if ( ((LA397_1>=185 && LA397_1<=206)) ) {
alt397=1;
}
else if ( (LA397_1==RULE_ID) ) {
int LA397_2 = input.LA(3);
if ( (LA397_2==RULE_ID||LA397_2==106) ) {
alt397=1;
}
}
}
break;
case RULE_ID:
{
int LA397_2 = input.LA(2);
if ( (LA397_2==RULE_ID||LA397_2==106) ) {
alt397=1;
}
}
break;
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
{
alt397=1;
}
break;
}
switch (alt397) {
case 1 :
// InternalContextMappingDSL.g:17394:4: (lv_attributes_11_0= ruleEnumAttribute )
{
// InternalContextMappingDSL.g:17394:4: (lv_attributes_11_0= ruleEnumAttribute )
// InternalContextMappingDSL.g:17395:5: lv_attributes_11_0= ruleEnumAttribute
{
newCompositeNode(grammarAccess.getEnumAccess().getAttributesEnumAttributeParserRuleCall_7_0());
pushFollow(FOLLOW_191);
lv_attributes_11_0=ruleEnumAttribute();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getEnumRule());
}
add(
current,
"attributes",
lv_attributes_11_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.EnumAttribute");
afterParserOrEnumRuleCall();
}
}
break;
default :
break loop397;
}
} while (true);
// InternalContextMappingDSL.g:17412:3: ( (lv_values_12_0= ruleEnumValue ) )
// InternalContextMappingDSL.g:17413:4: (lv_values_12_0= ruleEnumValue )
{
// InternalContextMappingDSL.g:17413:4: (lv_values_12_0= ruleEnumValue )
// InternalContextMappingDSL.g:17414:5: lv_values_12_0= ruleEnumValue
{
newCompositeNode(grammarAccess.getEnumAccess().getValuesEnumValueParserRuleCall_8_0());
pushFollow(FOLLOW_192);
lv_values_12_0=ruleEnumValue();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getEnumRule());
}
add(
current,
"values",
lv_values_12_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.EnumValue");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:17431:3: (otherlv_13= ',' ( (lv_values_14_0= ruleEnumValue ) ) )*
loop398:
do {
int alt398=2;
int LA398_0 = input.LA(1);
if ( (LA398_0==24) ) {
alt398=1;
}
switch (alt398) {
case 1 :
// InternalContextMappingDSL.g:17432:4: otherlv_13= ',' ( (lv_values_14_0= ruleEnumValue ) )
{
otherlv_13=(Token)match(input,24,FOLLOW_191);
newLeafNode(otherlv_13, grammarAccess.getEnumAccess().getCommaKeyword_9_0());
// InternalContextMappingDSL.g:17436:4: ( (lv_values_14_0= ruleEnumValue ) )
// InternalContextMappingDSL.g:17437:5: (lv_values_14_0= ruleEnumValue )
{
// InternalContextMappingDSL.g:17437:5: (lv_values_14_0= ruleEnumValue )
// InternalContextMappingDSL.g:17438:6: lv_values_14_0= ruleEnumValue
{
newCompositeNode(grammarAccess.getEnumAccess().getValuesEnumValueParserRuleCall_9_1_0());
pushFollow(FOLLOW_192);
lv_values_14_0=ruleEnumValue();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getEnumRule());
}
add(
current,
"values",
lv_values_14_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.EnumValue");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop398;
}
} while (true);
// InternalContextMappingDSL.g:17456:3: (otherlv_15= ';' )?
int alt399=2;
int LA399_0 = input.LA(1);
if ( (LA399_0==105) ) {
alt399=1;
}
switch (alt399) {
case 1 :
// InternalContextMappingDSL.g:17457:4: otherlv_15= ';'
{
otherlv_15=(Token)match(input,105,FOLLOW_36);
newLeafNode(otherlv_15, grammarAccess.getEnumAccess().getSemicolonKeyword_10());
}
break;
}
otherlv_16=(Token)match(input,RULE_CLOSE,FOLLOW_2);
newLeafNode(otherlv_16, grammarAccess.getEnumAccess().getRightCurlyBracketKeyword_11());
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleEnum"
// $ANTLR start "entryRuleEnumAttribute"
// InternalContextMappingDSL.g:17470:1: entryRuleEnumAttribute returns [EObject current=null] : iv_ruleEnumAttribute= ruleEnumAttribute EOF ;
public final EObject entryRuleEnumAttribute() throws RecognitionException {
EObject current = null;
EObject iv_ruleEnumAttribute = null;
try {
// InternalContextMappingDSL.g:17470:54: (iv_ruleEnumAttribute= ruleEnumAttribute EOF )
// InternalContextMappingDSL.g:17471:2: iv_ruleEnumAttribute= ruleEnumAttribute EOF
{
newCompositeNode(grammarAccess.getEnumAttributeRule());
pushFollow(FOLLOW_1);
iv_ruleEnumAttribute=ruleEnumAttribute();
state._fsp--;
current =iv_ruleEnumAttribute;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleEnumAttribute"
// $ANTLR start "ruleEnumAttribute"
// InternalContextMappingDSL.g:17477:1: ruleEnumAttribute returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_type_1_0= ruleType ) ) ( (lv_name_2_0= RULE_ID ) ) ( (lv_key_3_0= 'key' ) )? (otherlv_4= ';' )? ) ;
public final EObject ruleEnumAttribute() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token lv_name_2_0=null;
Token lv_key_3_0=null;
Token otherlv_4=null;
AntlrDatatypeRuleToken lv_type_1_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:17483:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_type_1_0= ruleType ) ) ( (lv_name_2_0= RULE_ID ) ) ( (lv_key_3_0= 'key' ) )? (otherlv_4= ';' )? ) )
// InternalContextMappingDSL.g:17484:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_type_1_0= ruleType ) ) ( (lv_name_2_0= RULE_ID ) ) ( (lv_key_3_0= 'key' ) )? (otherlv_4= ';' )? )
{
// InternalContextMappingDSL.g:17484:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_type_1_0= ruleType ) ) ( (lv_name_2_0= RULE_ID ) ) ( (lv_key_3_0= 'key' ) )? (otherlv_4= ';' )? )
// InternalContextMappingDSL.g:17485:3: ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_type_1_0= ruleType ) ) ( (lv_name_2_0= RULE_ID ) ) ( (lv_key_3_0= 'key' ) )? (otherlv_4= ';' )?
{
// InternalContextMappingDSL.g:17485:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt400=2;
int LA400_0 = input.LA(1);
if ( (LA400_0==RULE_STRING) ) {
alt400=1;
}
switch (alt400) {
case 1 :
// InternalContextMappingDSL.g:17486:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:17486:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:17487:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_172);
newLeafNode(lv_doc_0_0, grammarAccess.getEnumAttributeAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getEnumAttributeRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
// InternalContextMappingDSL.g:17503:3: ( (lv_type_1_0= ruleType ) )
// InternalContextMappingDSL.g:17504:4: (lv_type_1_0= ruleType )
{
// InternalContextMappingDSL.g:17504:4: (lv_type_1_0= ruleType )
// InternalContextMappingDSL.g:17505:5: lv_type_1_0= ruleType
{
newCompositeNode(grammarAccess.getEnumAttributeAccess().getTypeTypeParserRuleCall_1_0());
pushFollow(FOLLOW_10);
lv_type_1_0=ruleType();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getEnumAttributeRule());
}
set(
current,
"type",
lv_type_1_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.Type");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:17522:3: ( (lv_name_2_0= RULE_ID ) )
// InternalContextMappingDSL.g:17523:4: (lv_name_2_0= RULE_ID )
{
// InternalContextMappingDSL.g:17523:4: (lv_name_2_0= RULE_ID )
// InternalContextMappingDSL.g:17524:5: lv_name_2_0= RULE_ID
{
lv_name_2_0=(Token)match(input,RULE_ID,FOLLOW_193);
newLeafNode(lv_name_2_0, grammarAccess.getEnumAttributeAccess().getNameIDTerminalRuleCall_2_0());
if (current==null) {
current = createModelElement(grammarAccess.getEnumAttributeRule());
}
setWithLastConsumed(
current,
"name",
lv_name_2_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:17540:3: ( (lv_key_3_0= 'key' ) )?
int alt401=2;
int LA401_0 = input.LA(1);
if ( (LA401_0==146) ) {
alt401=1;
}
switch (alt401) {
case 1 :
// InternalContextMappingDSL.g:17541:4: (lv_key_3_0= 'key' )
{
// InternalContextMappingDSL.g:17541:4: (lv_key_3_0= 'key' )
// InternalContextMappingDSL.g:17542:5: lv_key_3_0= 'key'
{
lv_key_3_0=(Token)match(input,146,FOLLOW_194);
newLeafNode(lv_key_3_0, grammarAccess.getEnumAttributeAccess().getKeyKeyKeyword_3_0());
if (current==null) {
current = createModelElement(grammarAccess.getEnumAttributeRule());
}
setWithLastConsumed(current, "key", true, "key");
}
}
break;
}
// InternalContextMappingDSL.g:17554:3: (otherlv_4= ';' )?
int alt402=2;
int LA402_0 = input.LA(1);
if ( (LA402_0==105) ) {
alt402=1;
}
switch (alt402) {
case 1 :
// InternalContextMappingDSL.g:17555:4: otherlv_4= ';'
{
otherlv_4=(Token)match(input,105,FOLLOW_2);
newLeafNode(otherlv_4, grammarAccess.getEnumAttributeAccess().getSemicolonKeyword_4());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleEnumAttribute"
// $ANTLR start "entryRuleEnumValue"
// InternalContextMappingDSL.g:17564:1: entryRuleEnumValue returns [EObject current=null] : iv_ruleEnumValue= ruleEnumValue EOF ;
public final EObject entryRuleEnumValue() throws RecognitionException {
EObject current = null;
EObject iv_ruleEnumValue = null;
try {
// InternalContextMappingDSL.g:17564:50: (iv_ruleEnumValue= ruleEnumValue EOF )
// InternalContextMappingDSL.g:17565:2: iv_ruleEnumValue= ruleEnumValue EOF
{
newCompositeNode(grammarAccess.getEnumValueRule());
pushFollow(FOLLOW_1);
iv_ruleEnumValue=ruleEnumValue();
state._fsp--;
current =iv_ruleEnumValue;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleEnumValue"
// $ANTLR start "ruleEnumValue"
// InternalContextMappingDSL.g:17571:1: ruleEnumValue returns [EObject current=null] : ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_name_1_0= RULE_ID ) ) (otherlv_2= '(' ( (lv_parameters_3_0= ruleEnumParameter ) ) (otherlv_4= ',' ( (lv_parameters_5_0= ruleEnumParameter ) ) )* otherlv_6= ')' )? ) ;
public final EObject ruleEnumValue() throws RecognitionException {
EObject current = null;
Token lv_doc_0_0=null;
Token lv_name_1_0=null;
Token otherlv_2=null;
Token otherlv_4=null;
Token otherlv_6=null;
EObject lv_parameters_3_0 = null;
EObject lv_parameters_5_0 = null;
enterRule();
try {
// InternalContextMappingDSL.g:17577:2: ( ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_name_1_0= RULE_ID ) ) (otherlv_2= '(' ( (lv_parameters_3_0= ruleEnumParameter ) ) (otherlv_4= ',' ( (lv_parameters_5_0= ruleEnumParameter ) ) )* otherlv_6= ')' )? ) )
// InternalContextMappingDSL.g:17578:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_name_1_0= RULE_ID ) ) (otherlv_2= '(' ( (lv_parameters_3_0= ruleEnumParameter ) ) (otherlv_4= ',' ( (lv_parameters_5_0= ruleEnumParameter ) ) )* otherlv_6= ')' )? )
{
// InternalContextMappingDSL.g:17578:2: ( ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_name_1_0= RULE_ID ) ) (otherlv_2= '(' ( (lv_parameters_3_0= ruleEnumParameter ) ) (otherlv_4= ',' ( (lv_parameters_5_0= ruleEnumParameter ) ) )* otherlv_6= ')' )? )
// InternalContextMappingDSL.g:17579:3: ( (lv_doc_0_0= RULE_STRING ) )? ( (lv_name_1_0= RULE_ID ) ) (otherlv_2= '(' ( (lv_parameters_3_0= ruleEnumParameter ) ) (otherlv_4= ',' ( (lv_parameters_5_0= ruleEnumParameter ) ) )* otherlv_6= ')' )?
{
// InternalContextMappingDSL.g:17579:3: ( (lv_doc_0_0= RULE_STRING ) )?
int alt403=2;
int LA403_0 = input.LA(1);
if ( (LA403_0==RULE_STRING) ) {
alt403=1;
}
switch (alt403) {
case 1 :
// InternalContextMappingDSL.g:17580:4: (lv_doc_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:17580:4: (lv_doc_0_0= RULE_STRING )
// InternalContextMappingDSL.g:17581:5: lv_doc_0_0= RULE_STRING
{
lv_doc_0_0=(Token)match(input,RULE_STRING,FOLLOW_10);
newLeafNode(lv_doc_0_0, grammarAccess.getEnumValueAccess().getDocSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getEnumValueRule());
}
setWithLastConsumed(
current,
"doc",
lv_doc_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
break;
}
// InternalContextMappingDSL.g:17597:3: ( (lv_name_1_0= RULE_ID ) )
// InternalContextMappingDSL.g:17598:4: (lv_name_1_0= RULE_ID )
{
// InternalContextMappingDSL.g:17598:4: (lv_name_1_0= RULE_ID )
// InternalContextMappingDSL.g:17599:5: lv_name_1_0= RULE_ID
{
lv_name_1_0=(Token)match(input,RULE_ID,FOLLOW_195);
newLeafNode(lv_name_1_0, grammarAccess.getEnumValueAccess().getNameIDTerminalRuleCall_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getEnumValueRule());
}
setWithLastConsumed(
current,
"name",
lv_name_1_0,
"org.eclipse.xtext.common.Terminals.ID");
}
}
// InternalContextMappingDSL.g:17615:3: (otherlv_2= '(' ( (lv_parameters_3_0= ruleEnumParameter ) ) (otherlv_4= ',' ( (lv_parameters_5_0= ruleEnumParameter ) ) )* otherlv_6= ')' )?
int alt405=2;
int LA405_0 = input.LA(1);
if ( (LA405_0==102) ) {
alt405=1;
}
switch (alt405) {
case 1 :
// InternalContextMappingDSL.g:17616:4: otherlv_2= '(' ( (lv_parameters_3_0= ruleEnumParameter ) ) (otherlv_4= ',' ( (lv_parameters_5_0= ruleEnumParameter ) ) )* otherlv_6= ')'
{
otherlv_2=(Token)match(input,102,FOLLOW_196);
newLeafNode(otherlv_2, grammarAccess.getEnumValueAccess().getLeftParenthesisKeyword_2_0());
// InternalContextMappingDSL.g:17620:4: ( (lv_parameters_3_0= ruleEnumParameter ) )
// InternalContextMappingDSL.g:17621:5: (lv_parameters_3_0= ruleEnumParameter )
{
// InternalContextMappingDSL.g:17621:5: (lv_parameters_3_0= ruleEnumParameter )
// InternalContextMappingDSL.g:17622:6: lv_parameters_3_0= ruleEnumParameter
{
newCompositeNode(grammarAccess.getEnumValueAccess().getParametersEnumParameterParserRuleCall_2_1_0());
pushFollow(FOLLOW_114);
lv_parameters_3_0=ruleEnumParameter();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getEnumValueRule());
}
add(
current,
"parameters",
lv_parameters_3_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.EnumParameter");
afterParserOrEnumRuleCall();
}
}
// InternalContextMappingDSL.g:17639:4: (otherlv_4= ',' ( (lv_parameters_5_0= ruleEnumParameter ) ) )*
loop404:
do {
int alt404=2;
int LA404_0 = input.LA(1);
if ( (LA404_0==24) ) {
alt404=1;
}
switch (alt404) {
case 1 :
// InternalContextMappingDSL.g:17640:5: otherlv_4= ',' ( (lv_parameters_5_0= ruleEnumParameter ) )
{
otherlv_4=(Token)match(input,24,FOLLOW_196);
newLeafNode(otherlv_4, grammarAccess.getEnumValueAccess().getCommaKeyword_2_2_0());
// InternalContextMappingDSL.g:17644:5: ( (lv_parameters_5_0= ruleEnumParameter ) )
// InternalContextMappingDSL.g:17645:6: (lv_parameters_5_0= ruleEnumParameter )
{
// InternalContextMappingDSL.g:17645:6: (lv_parameters_5_0= ruleEnumParameter )
// InternalContextMappingDSL.g:17646:7: lv_parameters_5_0= ruleEnumParameter
{
newCompositeNode(grammarAccess.getEnumValueAccess().getParametersEnumParameterParserRuleCall_2_2_1_0());
pushFollow(FOLLOW_114);
lv_parameters_5_0=ruleEnumParameter();
state._fsp--;
if (current==null) {
current = createModelElementForParent(grammarAccess.getEnumValueRule());
}
add(
current,
"parameters",
lv_parameters_5_0,
"org.contextmapper.tactic.dsl.TacticDDDLanguage.EnumParameter");
afterParserOrEnumRuleCall();
}
}
}
break;
default :
break loop404;
}
} while (true);
otherlv_6=(Token)match(input,103,FOLLOW_2);
newLeafNode(otherlv_6, grammarAccess.getEnumValueAccess().getRightParenthesisKeyword_2_3());
}
break;
}
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleEnumValue"
// $ANTLR start "entryRuleEnumParameter"
// InternalContextMappingDSL.g:17673:1: entryRuleEnumParameter returns [EObject current=null] : iv_ruleEnumParameter= ruleEnumParameter EOF ;
public final EObject entryRuleEnumParameter() throws RecognitionException {
EObject current = null;
EObject iv_ruleEnumParameter = null;
try {
// InternalContextMappingDSL.g:17673:54: (iv_ruleEnumParameter= ruleEnumParameter EOF )
// InternalContextMappingDSL.g:17674:2: iv_ruleEnumParameter= ruleEnumParameter EOF
{
newCompositeNode(grammarAccess.getEnumParameterRule());
pushFollow(FOLLOW_1);
iv_ruleEnumParameter=ruleEnumParameter();
state._fsp--;
current =iv_ruleEnumParameter;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleEnumParameter"
// $ANTLR start "ruleEnumParameter"
// InternalContextMappingDSL.g:17680:1: ruleEnumParameter returns [EObject current=null] : ( ( (lv_value_0_0= RULE_STRING ) ) | ( (lv_integerValue_1_0= RULE_INT ) ) ) ;
public final EObject ruleEnumParameter() throws RecognitionException {
EObject current = null;
Token lv_value_0_0=null;
Token lv_integerValue_1_0=null;
enterRule();
try {
// InternalContextMappingDSL.g:17686:2: ( ( ( (lv_value_0_0= RULE_STRING ) ) | ( (lv_integerValue_1_0= RULE_INT ) ) ) )
// InternalContextMappingDSL.g:17687:2: ( ( (lv_value_0_0= RULE_STRING ) ) | ( (lv_integerValue_1_0= RULE_INT ) ) )
{
// InternalContextMappingDSL.g:17687:2: ( ( (lv_value_0_0= RULE_STRING ) ) | ( (lv_integerValue_1_0= RULE_INT ) ) )
int alt406=2;
int LA406_0 = input.LA(1);
if ( (LA406_0==RULE_STRING) ) {
alt406=1;
}
else if ( (LA406_0==RULE_INT) ) {
alt406=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 406, 0, input);
throw nvae;
}
switch (alt406) {
case 1 :
// InternalContextMappingDSL.g:17688:3: ( (lv_value_0_0= RULE_STRING ) )
{
// InternalContextMappingDSL.g:17688:3: ( (lv_value_0_0= RULE_STRING ) )
// InternalContextMappingDSL.g:17689:4: (lv_value_0_0= RULE_STRING )
{
// InternalContextMappingDSL.g:17689:4: (lv_value_0_0= RULE_STRING )
// InternalContextMappingDSL.g:17690:5: lv_value_0_0= RULE_STRING
{
lv_value_0_0=(Token)match(input,RULE_STRING,FOLLOW_2);
newLeafNode(lv_value_0_0, grammarAccess.getEnumParameterAccess().getValueSTRINGTerminalRuleCall_0_0());
if (current==null) {
current = createModelElement(grammarAccess.getEnumParameterRule());
}
setWithLastConsumed(
current,
"value",
lv_value_0_0,
"org.eclipse.xtext.common.Terminals.STRING");
}
}
}
break;
case 2 :
// InternalContextMappingDSL.g:17707:3: ( (lv_integerValue_1_0= RULE_INT ) )
{
// InternalContextMappingDSL.g:17707:3: ( (lv_integerValue_1_0= RULE_INT ) )
// InternalContextMappingDSL.g:17708:4: (lv_integerValue_1_0= RULE_INT )
{
// InternalContextMappingDSL.g:17708:4: (lv_integerValue_1_0= RULE_INT )
// InternalContextMappingDSL.g:17709:5: lv_integerValue_1_0= RULE_INT
{
lv_integerValue_1_0=(Token)match(input,RULE_INT,FOLLOW_2);
newLeafNode(lv_integerValue_1_0, grammarAccess.getEnumParameterAccess().getIntegerValueINTTerminalRuleCall_1_0());
if (current==null) {
current = createModelElement(grammarAccess.getEnumParameterRule());
}
setWithLastConsumed(
current,
"integerValue",
lv_integerValue_1_0,
"org.eclipse.xtext.common.Terminals.INT");
}
}
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleEnumParameter"
// $ANTLR start "entryRuleProperty"
// InternalContextMappingDSL.g:17729:1: entryRuleProperty returns [EObject current=null] : iv_ruleProperty= ruleProperty EOF ;
public final EObject entryRuleProperty() throws RecognitionException {
EObject current = null;
EObject iv_ruleProperty = null;
try {
// InternalContextMappingDSL.g:17729:49: (iv_ruleProperty= ruleProperty EOF )
// InternalContextMappingDSL.g:17730:2: iv_ruleProperty= ruleProperty EOF
{
newCompositeNode(grammarAccess.getPropertyRule());
pushFollow(FOLLOW_1);
iv_ruleProperty=ruleProperty();
state._fsp--;
current =iv_ruleProperty;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleProperty"
// $ANTLR start "ruleProperty"
// InternalContextMappingDSL.g:17736:1: ruleProperty returns [EObject current=null] : (this_Attribute_0= ruleAttribute | this_Reference_1= ruleReference ) ;
public final EObject ruleProperty() throws RecognitionException {
EObject current = null;
EObject this_Attribute_0 = null;
EObject this_Reference_1 = null;
enterRule();
try {
// InternalContextMappingDSL.g:17742:2: ( (this_Attribute_0= ruleAttribute | this_Reference_1= ruleReference ) )
// InternalContextMappingDSL.g:17743:2: (this_Attribute_0= ruleAttribute | this_Reference_1= ruleReference )
{
// InternalContextMappingDSL.g:17743:2: (this_Attribute_0= ruleAttribute | this_Reference_1= ruleReference )
int alt407=2;
switch ( input.LA(1) ) {
case RULE_STRING:
{
int LA407_1 = input.LA(2);
if ( (LA407_1==RULE_REF) ) {
alt407=2;
}
else if ( (LA407_1==RULE_ID||LA407_1==124||(LA407_1>=185 && LA407_1<=206)||LA407_1==233||(LA407_1>=243 && LA407_1<=249)) ) {
alt407=1;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 407, 1, input);
throw nvae;
}
}
break;
case RULE_ID:
case 124:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
case 247:
case 248:
case 249:
{
alt407=1;
}
break;
case RULE_REF:
{
alt407=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 407, 0, input);
throw nvae;
}
switch (alt407) {
case 1 :
// InternalContextMappingDSL.g:17744:3: this_Attribute_0= ruleAttribute
{
newCompositeNode(grammarAccess.getPropertyAccess().getAttributeParserRuleCall_0());
pushFollow(FOLLOW_2);
this_Attribute_0=ruleAttribute();
state._fsp--;
current = this_Attribute_0;
afterParserOrEnumRuleCall();
}
break;
case 2 :
// InternalContextMappingDSL.g:17753:3: this_Reference_1= ruleReference
{
newCompositeNode(grammarAccess.getPropertyAccess().getReferenceParserRuleCall_1());
pushFollow(FOLLOW_2);
this_Reference_1=ruleReference();
state._fsp--;
current = this_Reference_1;
afterParserOrEnumRuleCall();
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleProperty"
// $ANTLR start "entryRuleDtoProperty"
// InternalContextMappingDSL.g:17765:1: entryRuleDtoProperty returns [EObject current=null] : iv_ruleDtoProperty= ruleDtoProperty EOF ;
public final EObject entryRuleDtoProperty() throws RecognitionException {
EObject current = null;
EObject iv_ruleDtoProperty = null;
try {
// InternalContextMappingDSL.g:17765:52: (iv_ruleDtoProperty= ruleDtoProperty EOF )
// InternalContextMappingDSL.g:17766:2: iv_ruleDtoProperty= ruleDtoProperty EOF
{
newCompositeNode(grammarAccess.getDtoPropertyRule());
pushFollow(FOLLOW_1);
iv_ruleDtoProperty=ruleDtoProperty();
state._fsp--;
current =iv_ruleDtoProperty;
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleDtoProperty"
// $ANTLR start "ruleDtoProperty"
// InternalContextMappingDSL.g:17772:1: ruleDtoProperty returns [EObject current=null] : (this_DtoAttribute_0= ruleDtoAttribute | this_DtoReference_1= ruleDtoReference ) ;
public final EObject ruleDtoProperty() throws RecognitionException {
EObject current = null;
EObject this_DtoAttribute_0 = null;
EObject this_DtoReference_1 = null;
enterRule();
try {
// InternalContextMappingDSL.g:17778:2: ( (this_DtoAttribute_0= ruleDtoAttribute | this_DtoReference_1= ruleDtoReference ) )
// InternalContextMappingDSL.g:17779:2: (this_DtoAttribute_0= ruleDtoAttribute | this_DtoReference_1= ruleDtoReference )
{
// InternalContextMappingDSL.g:17779:2: (this_DtoAttribute_0= ruleDtoAttribute | this_DtoReference_1= ruleDtoReference )
int alt408=2;
switch ( input.LA(1) ) {
case RULE_STRING:
{
int LA408_1 = input.LA(2);
if ( (LA408_1==RULE_ID||LA408_1==124||(LA408_1>=185 && LA408_1<=206)||LA408_1==233||(LA408_1>=243 && LA408_1<=249)) ) {
alt408=1;
}
else if ( (LA408_1==RULE_REF) ) {
alt408=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 408, 1, input);
throw nvae;
}
}
break;
case RULE_ID:
case 124:
case 185:
case 186:
case 187:
case 188:
case 189:
case 190:
case 191:
case 192:
case 193:
case 194:
case 195:
case 196:
case 197:
case 198:
case 199:
case 200:
case 201:
case 202:
case 203:
case 204:
case 205:
case 206:
case 233:
case 243:
case 244:
case 245:
case 246:
case 247:
case 248:
case 249:
{
alt408=1;
}
break;
case RULE_REF:
{
alt408=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 408, 0, input);
throw nvae;
}
switch (alt408) {
case 1 :
// InternalContextMappingDSL.g:17780:3: this_DtoAttribute_0= ruleDtoAttribute
{
newCompositeNode(grammarAccess.getDtoPropertyAccess().getDtoAttributeParserRuleCall_0());
pushFollow(FOLLOW_2);
this_DtoAttribute_0=ruleDtoAttribute();
state._fsp--;
current = this_DtoAttribute_0;
afterParserOrEnumRuleCall();
}
break;
case 2 :
// InternalContextMappingDSL.g:17789:3: this_DtoReference_1= ruleDtoReference
{
newCompositeNode(grammarAccess.getDtoPropertyAccess().getDtoReferenceParserRuleCall_1());
pushFollow(FOLLOW_2);
this_DtoReference_1=ruleDtoReference();
state._fsp--;
current = this_DtoReference_1;
afterParserOrEnumRuleCall();
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleDtoProperty"
// $ANTLR start "entryRuleType"
// InternalContextMappingDSL.g:17801:1: entryRuleType returns [String current=null] : iv_ruleType= ruleType EOF ;
public final String entryRuleType() throws RecognitionException {
String current = null;
AntlrDatatypeRuleToken iv_ruleType = null;
try {
// InternalContextMappingDSL.g:17801:44: (iv_ruleType= ruleType EOF )
// InternalContextMappingDSL.g:17802:2: iv_ruleType= ruleType EOF
{
newCompositeNode(grammarAccess.getTypeRule());
pushFollow(FOLLOW_1);
iv_ruleType=ruleType();
state._fsp--;
current =iv_ruleType.getText();
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleType"
// $ANTLR start "ruleType"
// InternalContextMappingDSL.g:17808:1: ruleType returns [AntlrDatatypeRuleToken current=new AntlrDatatypeRuleToken()] : (kw= 'String' | kw= 'int' | kw= 'Integer' | kw= 'long' | kw= 'Long' | kw= 'boolean' | kw= 'Boolean' | kw= 'Date' | kw= 'DateTime' | kw= 'Timestamp' | kw= 'BigDecimal' | kw= 'BigInteger' | kw= 'double' | kw= 'Double' | kw= 'float' | kw= 'Float' | kw= 'Key' | kw= 'PagingParameter' | kw= 'PagedResult' | kw= 'Blob' | kw= 'Clob' | kw= 'Object[]' | this_JavaIdentifier_22= ruleJavaIdentifier ) ;
public final AntlrDatatypeRuleToken ruleType() throws RecognitionException {
AntlrDatatypeRuleToken current = new AntlrDatatypeRuleToken();
Token kw=null;
AntlrDatatypeRuleToken this_JavaIdentifier_22 = null;
enterRule();
try {
// InternalContextMappingDSL.g:17814:2: ( (kw= 'String' | kw= 'int' | kw= 'Integer' | kw= 'long' | kw= 'Long' | kw= 'boolean' | kw= 'Boolean' | kw= 'Date' | kw= 'DateTime' | kw= 'Timestamp' | kw= 'BigDecimal' | kw= 'BigInteger' | kw= 'double' | kw= 'Double' | kw= 'float' | kw= 'Float' | kw= 'Key' | kw= 'PagingParameter' | kw= 'PagedResult' | kw= 'Blob' | kw= 'Clob' | kw= 'Object[]' | this_JavaIdentifier_22= ruleJavaIdentifier ) )
// InternalContextMappingDSL.g:17815:2: (kw= 'String' | kw= 'int' | kw= 'Integer' | kw= 'long' | kw= 'Long' | kw= 'boolean' | kw= 'Boolean' | kw= 'Date' | kw= 'DateTime' | kw= 'Timestamp' | kw= 'BigDecimal' | kw= 'BigInteger' | kw= 'double' | kw= 'Double' | kw= 'float' | kw= 'Float' | kw= 'Key' | kw= 'PagingParameter' | kw= 'PagedResult' | kw= 'Blob' | kw= 'Clob' | kw= 'Object[]' | this_JavaIdentifier_22= ruleJavaIdentifier )
{
// InternalContextMappingDSL.g:17815:2: (kw= 'String' | kw= 'int' | kw= 'Integer' | kw= 'long' | kw= 'Long' | kw= 'boolean' | kw= 'Boolean' | kw= 'Date' | kw= 'DateTime' | kw= 'Timestamp' | kw= 'BigDecimal' | kw= 'BigInteger' | kw= 'double' | kw= 'Double' | kw= 'float' | kw= 'Float' | kw= 'Key' | kw= 'PagingParameter' | kw= 'PagedResult' | kw= 'Blob' | kw= 'Clob' | kw= 'Object[]' | this_JavaIdentifier_22= ruleJavaIdentifier )
int alt409=23;
switch ( input.LA(1) ) {
case 185:
{
alt409=1;
}
break;
case 186:
{
alt409=2;
}
break;
case 187:
{
alt409=3;
}
break;
case 188:
{
alt409=4;
}
break;
case 189:
{
alt409=5;
}
break;
case 190:
{
alt409=6;
}
break;
case 191:
{
alt409=7;
}
break;
case 192:
{
alt409=8;
}
break;
case 193:
{
alt409=9;
}
break;
case 194:
{
alt409=10;
}
break;
case 195:
{
alt409=11;
}
break;
case 196:
{
alt409=12;
}
break;
case 197:
{
alt409=13;
}
break;
case 198:
{
alt409=14;
}
break;
case 199:
{
alt409=15;
}
break;
case 200:
{
alt409=16;
}
break;
case 201:
{
alt409=17;
}
break;
case 202:
{
alt409=18;
}
break;
case 203:
{
alt409=19;
}
break;
case 204:
{
alt409=20;
}
break;
case 205:
{
alt409=21;
}
break;
case 206:
{
alt409=22;
}
break;
case RULE_ID:
{
alt409=23;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 409, 0, input);
throw nvae;
}
switch (alt409) {
case 1 :
// InternalContextMappingDSL.g:17816:3: kw= 'String'
{
kw=(Token)match(input,185,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getStringKeyword_0());
}
break;
case 2 :
// InternalContextMappingDSL.g:17822:3: kw= 'int'
{
kw=(Token)match(input,186,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getIntKeyword_1());
}
break;
case 3 :
// InternalContextMappingDSL.g:17828:3: kw= 'Integer'
{
kw=(Token)match(input,187,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getIntegerKeyword_2());
}
break;
case 4 :
// InternalContextMappingDSL.g:17834:3: kw= 'long'
{
kw=(Token)match(input,188,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getLongKeyword_3());
}
break;
case 5 :
// InternalContextMappingDSL.g:17840:3: kw= 'Long'
{
kw=(Token)match(input,189,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getLongKeyword_4());
}
break;
case 6 :
// InternalContextMappingDSL.g:17846:3: kw= 'boolean'
{
kw=(Token)match(input,190,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getBooleanKeyword_5());
}
break;
case 7 :
// InternalContextMappingDSL.g:17852:3: kw= 'Boolean'
{
kw=(Token)match(input,191,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getBooleanKeyword_6());
}
break;
case 8 :
// InternalContextMappingDSL.g:17858:3: kw= 'Date'
{
kw=(Token)match(input,192,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getDateKeyword_7());
}
break;
case 9 :
// InternalContextMappingDSL.g:17864:3: kw= 'DateTime'
{
kw=(Token)match(input,193,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getDateTimeKeyword_8());
}
break;
case 10 :
// InternalContextMappingDSL.g:17870:3: kw= 'Timestamp'
{
kw=(Token)match(input,194,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getTimestampKeyword_9());
}
break;
case 11 :
// InternalContextMappingDSL.g:17876:3: kw= 'BigDecimal'
{
kw=(Token)match(input,195,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getBigDecimalKeyword_10());
}
break;
case 12 :
// InternalContextMappingDSL.g:17882:3: kw= 'BigInteger'
{
kw=(Token)match(input,196,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getBigIntegerKeyword_11());
}
break;
case 13 :
// InternalContextMappingDSL.g:17888:3: kw= 'double'
{
kw=(Token)match(input,197,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getDoubleKeyword_12());
}
break;
case 14 :
// InternalContextMappingDSL.g:17894:3: kw= 'Double'
{
kw=(Token)match(input,198,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getDoubleKeyword_13());
}
break;
case 15 :
// InternalContextMappingDSL.g:17900:3: kw= 'float'
{
kw=(Token)match(input,199,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getFloatKeyword_14());
}
break;
case 16 :
// InternalContextMappingDSL.g:17906:3: kw= 'Float'
{
kw=(Token)match(input,200,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getFloatKeyword_15());
}
break;
case 17 :
// InternalContextMappingDSL.g:17912:3: kw= 'Key'
{
kw=(Token)match(input,201,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getKeyKeyword_16());
}
break;
case 18 :
// InternalContextMappingDSL.g:17918:3: kw= 'PagingParameter'
{
kw=(Token)match(input,202,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getPagingParameterKeyword_17());
}
break;
case 19 :
// InternalContextMappingDSL.g:17924:3: kw= 'PagedResult'
{
kw=(Token)match(input,203,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getPagedResultKeyword_18());
}
break;
case 20 :
// InternalContextMappingDSL.g:17930:3: kw= 'Blob'
{
kw=(Token)match(input,204,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getBlobKeyword_19());
}
break;
case 21 :
// InternalContextMappingDSL.g:17936:3: kw= 'Clob'
{
kw=(Token)match(input,205,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getClobKeyword_20());
}
break;
case 22 :
// InternalContextMappingDSL.g:17942:3: kw= 'Object[]'
{
kw=(Token)match(input,206,FOLLOW_2);
current.merge(kw);
newLeafNode(kw, grammarAccess.getTypeAccess().getObjectKeyword_21());
}
break;
case 23 :
// InternalContextMappingDSL.g:17948:3: this_JavaIdentifier_22= ruleJavaIdentifier
{
newCompositeNode(grammarAccess.getTypeAccess().getJavaIdentifierParserRuleCall_22());
pushFollow(FOLLOW_2);
this_JavaIdentifier_22=ruleJavaIdentifier();
state._fsp--;
current.merge(this_JavaIdentifier_22);
afterParserOrEnumRuleCall();
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleType"
// $ANTLR start "entryRuleJavaIdentifier"
// InternalContextMappingDSL.g:17962:1: entryRuleJavaIdentifier returns [String current=null] : iv_ruleJavaIdentifier= ruleJavaIdentifier EOF ;
public final String entryRuleJavaIdentifier() throws RecognitionException {
String current = null;
AntlrDatatypeRuleToken iv_ruleJavaIdentifier = null;
try {
// InternalContextMappingDSL.g:17962:54: (iv_ruleJavaIdentifier= ruleJavaIdentifier EOF )
// InternalContextMappingDSL.g:17963:2: iv_ruleJavaIdentifier= ruleJavaIdentifier EOF
{
newCompositeNode(grammarAccess.getJavaIdentifierRule());
pushFollow(FOLLOW_1);
iv_ruleJavaIdentifier=ruleJavaIdentifier();
state._fsp--;
current =iv_ruleJavaIdentifier.getText();
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleJavaIdentifier"
// $ANTLR start "ruleJavaIdentifier"
// InternalContextMappingDSL.g:17969:1: ruleJavaIdentifier returns [AntlrDatatypeRuleToken current=new AntlrDatatypeRuleToken()] : (this_ID_0= RULE_ID (kw= '.' this_ID_2= RULE_ID )* ) ;
public final AntlrDatatypeRuleToken ruleJavaIdentifier() throws RecognitionException {
AntlrDatatypeRuleToken current = new AntlrDatatypeRuleToken();
Token this_ID_0=null;
Token kw=null;
Token this_ID_2=null;
enterRule();
try {
// InternalContextMappingDSL.g:17975:2: ( (this_ID_0= RULE_ID (kw= '.' this_ID_2= RULE_ID )* ) )
// InternalContextMappingDSL.g:17976:2: (this_ID_0= RULE_ID (kw= '.' this_ID_2= RULE_ID )* )
{
// InternalContextMappingDSL.g:17976:2: (this_ID_0= RULE_ID (kw= '.' this_ID_2= RULE_ID )* )
// InternalContextMappingDSL.g:17977:3: this_ID_0= RULE_ID (kw= '.' this_ID_2= RULE_ID )*
{
this_ID_0=(Token)match(input,RULE_ID,FOLLOW_197);
current.merge(this_ID_0);
newLeafNode(this_ID_0, grammarAccess.getJavaIdentifierAccess().getIDTerminalRuleCall_0());
// InternalContextMappingDSL.g:17984:3: (kw= '.' this_ID_2= RULE_ID )*
loop410:
do {
int alt410=2;
int LA410_0 = input.LA(1);
if ( (LA410_0==106) ) {
alt410=1;
}
switch (alt410) {
case 1 :
// InternalContextMappingDSL.g:17985:4: kw= '.' this_ID_2= RULE_ID
{
kw=(Token)match(input,106,FOLLOW_10);
current.merge(kw);
newLeafNode(kw, grammarAccess.getJavaIdentifierAccess().getFullStopKeyword_1_0());
this_ID_2=(Token)match(input,RULE_ID,FOLLOW_197);
current.merge(this_ID_2);
newLeafNode(this_ID_2, grammarAccess.getJavaIdentifierAccess().getIDTerminalRuleCall_1_1());
}
break;
default :
break loop410;
}
} while (true);
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleJavaIdentifier"
// $ANTLR start "entryRuleChannelIdentifier"
// InternalContextMappingDSL.g:18002:1: entryRuleChannelIdentifier returns [String current=null] : iv_ruleChannelIdentifier= ruleChannelIdentifier EOF ;
public final String entryRuleChannelIdentifier() throws RecognitionException {
String current = null;
AntlrDatatypeRuleToken iv_ruleChannelIdentifier = null;
try {
// InternalContextMappingDSL.g:18002:57: (iv_ruleChannelIdentifier= ruleChannelIdentifier EOF )
// InternalContextMappingDSL.g:18003:2: iv_ruleChannelIdentifier= ruleChannelIdentifier EOF
{
newCompositeNode(grammarAccess.getChannelIdentifierRule());
pushFollow(FOLLOW_1);
iv_ruleChannelIdentifier=ruleChannelIdentifier();
state._fsp--;
current =iv_ruleChannelIdentifier.getText();
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleChannelIdentifier"
// $ANTLR start "ruleChannelIdentifier"
// InternalContextMappingDSL.g:18009:1: ruleChannelIdentifier returns [AntlrDatatypeRuleToken current=new AntlrDatatypeRuleToken()] : (this_ID_0= RULE_ID ( (kw= '.' | kw= '/' | kw= ':' ) this_ID_4= RULE_ID )* ) ;
public final AntlrDatatypeRuleToken ruleChannelIdentifier() throws RecognitionException {
AntlrDatatypeRuleToken current = new AntlrDatatypeRuleToken();
Token this_ID_0=null;
Token kw=null;
Token this_ID_4=null;
enterRule();
try {
// InternalContextMappingDSL.g:18015:2: ( (this_ID_0= RULE_ID ( (kw= '.' | kw= '/' | kw= ':' ) this_ID_4= RULE_ID )* ) )
// InternalContextMappingDSL.g:18016:2: (this_ID_0= RULE_ID ( (kw= '.' | kw= '/' | kw= ':' ) this_ID_4= RULE_ID )* )
{
// InternalContextMappingDSL.g:18016:2: (this_ID_0= RULE_ID ( (kw= '.' | kw= '/' | kw= ':' ) this_ID_4= RULE_ID )* )
// InternalContextMappingDSL.g:18017:3: this_ID_0= RULE_ID ( (kw= '.' | kw= '/' | kw= ':' ) this_ID_4= RULE_ID )*
{
this_ID_0=(Token)match(input,RULE_ID,FOLLOW_198);
current.merge(this_ID_0);
newLeafNode(this_ID_0, grammarAccess.getChannelIdentifierAccess().getIDTerminalRuleCall_0());
// InternalContextMappingDSL.g:18024:3: ( (kw= '.' | kw= '/' | kw= ':' ) this_ID_4= RULE_ID )*
loop412:
do {
int alt412=2;
int LA412_0 = input.LA(1);
if ( (LA412_0==40||LA412_0==106||LA412_0==207) ) {
alt412=1;
}
switch (alt412) {
case 1 :
// InternalContextMappingDSL.g:18025:4: (kw= '.' | kw= '/' | kw= ':' ) this_ID_4= RULE_ID
{
// InternalContextMappingDSL.g:18025:4: (kw= '.' | kw= '/' | kw= ':' )
int alt411=3;
switch ( input.LA(1) ) {
case 106:
{
alt411=1;
}
break;
case 207:
{
alt411=2;
}
break;
case 40:
{
alt411=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 411, 0, input);
throw nvae;
}
switch (alt411) {
case 1 :
// InternalContextMappingDSL.g:18026:5: kw= '.'
{
kw=(Token)match(input,106,FOLLOW_10);
current.merge(kw);
newLeafNode(kw, grammarAccess.getChannelIdentifierAccess().getFullStopKeyword_1_0_0());
}
break;
case 2 :
// InternalContextMappingDSL.g:18032:5: kw= '/'
{
kw=(Token)match(input,207,FOLLOW_10);
current.merge(kw);
newLeafNode(kw, grammarAccess.getChannelIdentifierAccess().getSolidusKeyword_1_0_1());
}
break;
case 3 :
// InternalContextMappingDSL.g:18038:5: kw= ':'
{
kw=(Token)match(input,40,FOLLOW_10);
current.merge(kw);
newLeafNode(kw, grammarAccess.getChannelIdentifierAccess().getColonKeyword_1_0_2());
}
break;
}
this_ID_4=(Token)match(input,RULE_ID,FOLLOW_198);
current.merge(this_ID_4);
newLeafNode(this_ID_4, grammarAccess.getChannelIdentifierAccess().getIDTerminalRuleCall_1_1());
}
break;
default :
break loop412;
}
} while (true);
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleChannelIdentifier"
// $ANTLR start "entryRuleThrowsIdentifier"
// InternalContextMappingDSL.g:18056:1: entryRuleThrowsIdentifier returns [String current=null] : iv_ruleThrowsIdentifier= ruleThrowsIdentifier EOF ;
public final String entryRuleThrowsIdentifier() throws RecognitionException {
String current = null;
AntlrDatatypeRuleToken iv_ruleThrowsIdentifier = null;
try {
// InternalContextMappingDSL.g:18056:56: (iv_ruleThrowsIdentifier= ruleThrowsIdentifier EOF )
// InternalContextMappingDSL.g:18057:2: iv_ruleThrowsIdentifier= ruleThrowsIdentifier EOF
{
newCompositeNode(grammarAccess.getThrowsIdentifierRule());
pushFollow(FOLLOW_1);
iv_ruleThrowsIdentifier=ruleThrowsIdentifier();
state._fsp--;
current =iv_ruleThrowsIdentifier.getText();
match(input,EOF,FOLLOW_2);
}
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "entryRuleThrowsIdentifier"
// $ANTLR start "ruleThrowsIdentifier"
// InternalContextMappingDSL.g:18063:1: ruleThrowsIdentifier returns [AntlrDatatypeRuleToken current=new AntlrDatatypeRuleToken()] : (this_JavaIdentifier_0= ruleJavaIdentifier (kw= ',' this_JavaIdentifier_2= ruleJavaIdentifier )* ) ;
public final AntlrDatatypeRuleToken ruleThrowsIdentifier() throws RecognitionException {
AntlrDatatypeRuleToken current = new AntlrDatatypeRuleToken();
Token kw=null;
AntlrDatatypeRuleToken this_JavaIdentifier_0 = null;
AntlrDatatypeRuleToken this_JavaIdentifier_2 = null;
enterRule();
try {
// InternalContextMappingDSL.g:18069:2: ( (this_JavaIdentifier_0= ruleJavaIdentifier (kw= ',' this_JavaIdentifier_2= ruleJavaIdentifier )* ) )
// InternalContextMappingDSL.g:18070:2: (this_JavaIdentifier_0= ruleJavaIdentifier (kw= ',' this_JavaIdentifier_2= ruleJavaIdentifier )* )
{
// InternalContextMappingDSL.g:18070:2: (this_JavaIdentifier_0= ruleJavaIdentifier (kw= ',' this_JavaIdentifier_2= ruleJavaIdentifier )* )
// InternalContextMappingDSL.g:18071:3: this_JavaIdentifier_0= ruleJavaIdentifier (kw= ',' this_JavaIdentifier_2= ruleJavaIdentifier )*
{
newCompositeNode(grammarAccess.getThrowsIdentifierAccess().getJavaIdentifierParserRuleCall_0());
pushFollow(FOLLOW_199);
this_JavaIdentifier_0=ruleJavaIdentifier();
state._fsp--;
current.merge(this_JavaIdentifier_0);
afterParserOrEnumRuleCall();
// InternalContextMappingDSL.g:18081:3: (kw= ',' this_JavaIdentifier_2= ruleJavaIdentifier )*
loop413:
do {
int alt413=2;
int LA413_0 = input.LA(1);
if ( (LA413_0==24) ) {
alt413=1;
}
switch (alt413) {
case 1 :
// InternalContextMappingDSL.g:18082:4: kw= ',' this_JavaIdentifier_2= ruleJavaIdentifier
{
kw=(Token)match(input,24,FOLLOW_10);
current.merge(kw);
newLeafNode(kw, grammarAccess.getThrowsIdentifierAccess().getCommaKeyword_1_0());
newCompositeNode(grammarAccess.getThrowsIdentifierAccess().getJavaIdentifierParserRuleCall_1_1());
pushFollow(FOLLOW_199);
this_JavaIdentifier_2=ruleJavaIdentifier();
state._fsp--;
current.merge(this_JavaIdentifier_2);
afterParserOrEnumRuleCall();
}
break;
default :
break loop413;
}
} while (true);
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleThrowsIdentifier"
// $ANTLR start "ruleUpstreamRole"
// InternalContextMappingDSL.g:18102:1: ruleUpstreamRole returns [Enumerator current=null] : ( (enumLiteral_0= 'PL' ) | (enumLiteral_1= 'OHS' ) ) ;
public final Enumerator ruleUpstreamRole() throws RecognitionException {
Enumerator current = null;
Token enumLiteral_0=null;
Token enumLiteral_1=null;
enterRule();
try {
// InternalContextMappingDSL.g:18108:2: ( ( (enumLiteral_0= 'PL' ) | (enumLiteral_1= 'OHS' ) ) )
// InternalContextMappingDSL.g:18109:2: ( (enumLiteral_0= 'PL' ) | (enumLiteral_1= 'OHS' ) )
{
// InternalContextMappingDSL.g:18109:2: ( (enumLiteral_0= 'PL' ) | (enumLiteral_1= 'OHS' ) )
int alt414=2;
int LA414_0 = input.LA(1);
if ( (LA414_0==208) ) {
alt414=1;
}
else if ( (LA414_0==209) ) {
alt414=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 414, 0, input);
throw nvae;
}
switch (alt414) {
case 1 :
// InternalContextMappingDSL.g:18110:3: (enumLiteral_0= 'PL' )
{
// InternalContextMappingDSL.g:18110:3: (enumLiteral_0= 'PL' )
// InternalContextMappingDSL.g:18111:4: enumLiteral_0= 'PL'
{
enumLiteral_0=(Token)match(input,208,FOLLOW_2);
current = grammarAccess.getUpstreamRoleAccess().getPUBLISHED_LANGUAGEEnumLiteralDeclaration_0().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_0, grammarAccess.getUpstreamRoleAccess().getPUBLISHED_LANGUAGEEnumLiteralDeclaration_0());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:18118:3: (enumLiteral_1= 'OHS' )
{
// InternalContextMappingDSL.g:18118:3: (enumLiteral_1= 'OHS' )
// InternalContextMappingDSL.g:18119:4: enumLiteral_1= 'OHS'
{
enumLiteral_1=(Token)match(input,209,FOLLOW_2);
current = grammarAccess.getUpstreamRoleAccess().getOPEN_HOST_SERVICEEnumLiteralDeclaration_1().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_1, grammarAccess.getUpstreamRoleAccess().getOPEN_HOST_SERVICEEnumLiteralDeclaration_1());
}
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleUpstreamRole"
// $ANTLR start "ruleDownstreamRole"
// InternalContextMappingDSL.g:18129:1: ruleDownstreamRole returns [Enumerator current=null] : ( (enumLiteral_0= 'ACL' ) | (enumLiteral_1= 'CF' ) ) ;
public final Enumerator ruleDownstreamRole() throws RecognitionException {
Enumerator current = null;
Token enumLiteral_0=null;
Token enumLiteral_1=null;
enterRule();
try {
// InternalContextMappingDSL.g:18135:2: ( ( (enumLiteral_0= 'ACL' ) | (enumLiteral_1= 'CF' ) ) )
// InternalContextMappingDSL.g:18136:2: ( (enumLiteral_0= 'ACL' ) | (enumLiteral_1= 'CF' ) )
{
// InternalContextMappingDSL.g:18136:2: ( (enumLiteral_0= 'ACL' ) | (enumLiteral_1= 'CF' ) )
int alt415=2;
int LA415_0 = input.LA(1);
if ( (LA415_0==210) ) {
alt415=1;
}
else if ( (LA415_0==211) ) {
alt415=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 415, 0, input);
throw nvae;
}
switch (alt415) {
case 1 :
// InternalContextMappingDSL.g:18137:3: (enumLiteral_0= 'ACL' )
{
// InternalContextMappingDSL.g:18137:3: (enumLiteral_0= 'ACL' )
// InternalContextMappingDSL.g:18138:4: enumLiteral_0= 'ACL'
{
enumLiteral_0=(Token)match(input,210,FOLLOW_2);
current = grammarAccess.getDownstreamRoleAccess().getANTICORRUPTION_LAYEREnumLiteralDeclaration_0().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_0, grammarAccess.getDownstreamRoleAccess().getANTICORRUPTION_LAYEREnumLiteralDeclaration_0());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:18145:3: (enumLiteral_1= 'CF' )
{
// InternalContextMappingDSL.g:18145:3: (enumLiteral_1= 'CF' )
// InternalContextMappingDSL.g:18146:4: enumLiteral_1= 'CF'
{
enumLiteral_1=(Token)match(input,211,FOLLOW_2);
current = grammarAccess.getDownstreamRoleAccess().getCONFORMISTEnumLiteralDeclaration_1().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_1, grammarAccess.getDownstreamRoleAccess().getCONFORMISTEnumLiteralDeclaration_1());
}
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleDownstreamRole"
// $ANTLR start "ruleContextMapState"
// InternalContextMappingDSL.g:18156:1: ruleContextMapState returns [Enumerator current=null] : ( (enumLiteral_0= 'AS_IS' ) | (enumLiteral_1= 'TO_BE' ) ) ;
public final Enumerator ruleContextMapState() throws RecognitionException {
Enumerator current = null;
Token enumLiteral_0=null;
Token enumLiteral_1=null;
enterRule();
try {
// InternalContextMappingDSL.g:18162:2: ( ( (enumLiteral_0= 'AS_IS' ) | (enumLiteral_1= 'TO_BE' ) ) )
// InternalContextMappingDSL.g:18163:2: ( (enumLiteral_0= 'AS_IS' ) | (enumLiteral_1= 'TO_BE' ) )
{
// InternalContextMappingDSL.g:18163:2: ( (enumLiteral_0= 'AS_IS' ) | (enumLiteral_1= 'TO_BE' ) )
int alt416=2;
int LA416_0 = input.LA(1);
if ( (LA416_0==212) ) {
alt416=1;
}
else if ( (LA416_0==213) ) {
alt416=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 416, 0, input);
throw nvae;
}
switch (alt416) {
case 1 :
// InternalContextMappingDSL.g:18164:3: (enumLiteral_0= 'AS_IS' )
{
// InternalContextMappingDSL.g:18164:3: (enumLiteral_0= 'AS_IS' )
// InternalContextMappingDSL.g:18165:4: enumLiteral_0= 'AS_IS'
{
enumLiteral_0=(Token)match(input,212,FOLLOW_2);
current = grammarAccess.getContextMapStateAccess().getAS_ISEnumLiteralDeclaration_0().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_0, grammarAccess.getContextMapStateAccess().getAS_ISEnumLiteralDeclaration_0());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:18172:3: (enumLiteral_1= 'TO_BE' )
{
// InternalContextMappingDSL.g:18172:3: (enumLiteral_1= 'TO_BE' )
// InternalContextMappingDSL.g:18173:4: enumLiteral_1= 'TO_BE'
{
enumLiteral_1=(Token)match(input,213,FOLLOW_2);
current = grammarAccess.getContextMapStateAccess().getTO_BEEnumLiteralDeclaration_1().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_1, grammarAccess.getContextMapStateAccess().getTO_BEEnumLiteralDeclaration_1());
}
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleContextMapState"
// $ANTLR start "ruleContextMapType"
// InternalContextMappingDSL.g:18183:1: ruleContextMapType returns [Enumerator current=null] : ( (enumLiteral_0= 'SYSTEM_LANDSCAPE' ) | (enumLiteral_1= 'ORGANIZATIONAL' ) ) ;
public final Enumerator ruleContextMapType() throws RecognitionException {
Enumerator current = null;
Token enumLiteral_0=null;
Token enumLiteral_1=null;
enterRule();
try {
// InternalContextMappingDSL.g:18189:2: ( ( (enumLiteral_0= 'SYSTEM_LANDSCAPE' ) | (enumLiteral_1= 'ORGANIZATIONAL' ) ) )
// InternalContextMappingDSL.g:18190:2: ( (enumLiteral_0= 'SYSTEM_LANDSCAPE' ) | (enumLiteral_1= 'ORGANIZATIONAL' ) )
{
// InternalContextMappingDSL.g:18190:2: ( (enumLiteral_0= 'SYSTEM_LANDSCAPE' ) | (enumLiteral_1= 'ORGANIZATIONAL' ) )
int alt417=2;
int LA417_0 = input.LA(1);
if ( (LA417_0==214) ) {
alt417=1;
}
else if ( (LA417_0==215) ) {
alt417=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 417, 0, input);
throw nvae;
}
switch (alt417) {
case 1 :
// InternalContextMappingDSL.g:18191:3: (enumLiteral_0= 'SYSTEM_LANDSCAPE' )
{
// InternalContextMappingDSL.g:18191:3: (enumLiteral_0= 'SYSTEM_LANDSCAPE' )
// InternalContextMappingDSL.g:18192:4: enumLiteral_0= 'SYSTEM_LANDSCAPE'
{
enumLiteral_0=(Token)match(input,214,FOLLOW_2);
current = grammarAccess.getContextMapTypeAccess().getSYSTEM_LANDSCAPEEnumLiteralDeclaration_0().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_0, grammarAccess.getContextMapTypeAccess().getSYSTEM_LANDSCAPEEnumLiteralDeclaration_0());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:18199:3: (enumLiteral_1= 'ORGANIZATIONAL' )
{
// InternalContextMappingDSL.g:18199:3: (enumLiteral_1= 'ORGANIZATIONAL' )
// InternalContextMappingDSL.g:18200:4: enumLiteral_1= 'ORGANIZATIONAL'
{
enumLiteral_1=(Token)match(input,215,FOLLOW_2);
current = grammarAccess.getContextMapTypeAccess().getORGANIZATIONALEnumLiteralDeclaration_1().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_1, grammarAccess.getContextMapTypeAccess().getORGANIZATIONALEnumLiteralDeclaration_1());
}
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleContextMapType"
// $ANTLR start "ruleBoundedContextType"
// InternalContextMappingDSL.g:18210:1: ruleBoundedContextType returns [Enumerator current=null] : ( (enumLiteral_0= 'FEATURE' ) | (enumLiteral_1= 'APPLICATION' ) | (enumLiteral_2= 'SYSTEM' ) | (enumLiteral_3= 'TEAM' ) ) ;
public final Enumerator ruleBoundedContextType() throws RecognitionException {
Enumerator current = null;
Token enumLiteral_0=null;
Token enumLiteral_1=null;
Token enumLiteral_2=null;
Token enumLiteral_3=null;
enterRule();
try {
// InternalContextMappingDSL.g:18216:2: ( ( (enumLiteral_0= 'FEATURE' ) | (enumLiteral_1= 'APPLICATION' ) | (enumLiteral_2= 'SYSTEM' ) | (enumLiteral_3= 'TEAM' ) ) )
// InternalContextMappingDSL.g:18217:2: ( (enumLiteral_0= 'FEATURE' ) | (enumLiteral_1= 'APPLICATION' ) | (enumLiteral_2= 'SYSTEM' ) | (enumLiteral_3= 'TEAM' ) )
{
// InternalContextMappingDSL.g:18217:2: ( (enumLiteral_0= 'FEATURE' ) | (enumLiteral_1= 'APPLICATION' ) | (enumLiteral_2= 'SYSTEM' ) | (enumLiteral_3= 'TEAM' ) )
int alt418=4;
switch ( input.LA(1) ) {
case 216:
{
alt418=1;
}
break;
case 217:
{
alt418=2;
}
break;
case 218:
{
alt418=3;
}
break;
case 219:
{
alt418=4;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 418, 0, input);
throw nvae;
}
switch (alt418) {
case 1 :
// InternalContextMappingDSL.g:18218:3: (enumLiteral_0= 'FEATURE' )
{
// InternalContextMappingDSL.g:18218:3: (enumLiteral_0= 'FEATURE' )
// InternalContextMappingDSL.g:18219:4: enumLiteral_0= 'FEATURE'
{
enumLiteral_0=(Token)match(input,216,FOLLOW_2);
current = grammarAccess.getBoundedContextTypeAccess().getFEATUREEnumLiteralDeclaration_0().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_0, grammarAccess.getBoundedContextTypeAccess().getFEATUREEnumLiteralDeclaration_0());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:18226:3: (enumLiteral_1= 'APPLICATION' )
{
// InternalContextMappingDSL.g:18226:3: (enumLiteral_1= 'APPLICATION' )
// InternalContextMappingDSL.g:18227:4: enumLiteral_1= 'APPLICATION'
{
enumLiteral_1=(Token)match(input,217,FOLLOW_2);
current = grammarAccess.getBoundedContextTypeAccess().getAPPLICATIONEnumLiteralDeclaration_1().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_1, grammarAccess.getBoundedContextTypeAccess().getAPPLICATIONEnumLiteralDeclaration_1());
}
}
break;
case 3 :
// InternalContextMappingDSL.g:18234:3: (enumLiteral_2= 'SYSTEM' )
{
// InternalContextMappingDSL.g:18234:3: (enumLiteral_2= 'SYSTEM' )
// InternalContextMappingDSL.g:18235:4: enumLiteral_2= 'SYSTEM'
{
enumLiteral_2=(Token)match(input,218,FOLLOW_2);
current = grammarAccess.getBoundedContextTypeAccess().getSYSTEMEnumLiteralDeclaration_2().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_2, grammarAccess.getBoundedContextTypeAccess().getSYSTEMEnumLiteralDeclaration_2());
}
}
break;
case 4 :
// InternalContextMappingDSL.g:18242:3: (enumLiteral_3= 'TEAM' )
{
// InternalContextMappingDSL.g:18242:3: (enumLiteral_3= 'TEAM' )
// InternalContextMappingDSL.g:18243:4: enumLiteral_3= 'TEAM'
{
enumLiteral_3=(Token)match(input,219,FOLLOW_2);
current = grammarAccess.getBoundedContextTypeAccess().getTEAMEnumLiteralDeclaration_3().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_3, grammarAccess.getBoundedContextTypeAccess().getTEAMEnumLiteralDeclaration_3());
}
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleBoundedContextType"
// $ANTLR start "ruleSubDomainType"
// InternalContextMappingDSL.g:18253:1: ruleSubDomainType returns [Enumerator current=null] : ( (enumLiteral_0= 'CORE_DOMAIN' ) | (enumLiteral_1= 'SUPPORTING_DOMAIN' ) | (enumLiteral_2= 'GENERIC_SUBDOMAIN' ) ) ;
public final Enumerator ruleSubDomainType() throws RecognitionException {
Enumerator current = null;
Token enumLiteral_0=null;
Token enumLiteral_1=null;
Token enumLiteral_2=null;
enterRule();
try {
// InternalContextMappingDSL.g:18259:2: ( ( (enumLiteral_0= 'CORE_DOMAIN' ) | (enumLiteral_1= 'SUPPORTING_DOMAIN' ) | (enumLiteral_2= 'GENERIC_SUBDOMAIN' ) ) )
// InternalContextMappingDSL.g:18260:2: ( (enumLiteral_0= 'CORE_DOMAIN' ) | (enumLiteral_1= 'SUPPORTING_DOMAIN' ) | (enumLiteral_2= 'GENERIC_SUBDOMAIN' ) )
{
// InternalContextMappingDSL.g:18260:2: ( (enumLiteral_0= 'CORE_DOMAIN' ) | (enumLiteral_1= 'SUPPORTING_DOMAIN' ) | (enumLiteral_2= 'GENERIC_SUBDOMAIN' ) )
int alt419=3;
switch ( input.LA(1) ) {
case 220:
{
alt419=1;
}
break;
case 221:
{
alt419=2;
}
break;
case 222:
{
alt419=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 419, 0, input);
throw nvae;
}
switch (alt419) {
case 1 :
// InternalContextMappingDSL.g:18261:3: (enumLiteral_0= 'CORE_DOMAIN' )
{
// InternalContextMappingDSL.g:18261:3: (enumLiteral_0= 'CORE_DOMAIN' )
// InternalContextMappingDSL.g:18262:4: enumLiteral_0= 'CORE_DOMAIN'
{
enumLiteral_0=(Token)match(input,220,FOLLOW_2);
current = grammarAccess.getSubDomainTypeAccess().getCORE_DOMAINEnumLiteralDeclaration_0().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_0, grammarAccess.getSubDomainTypeAccess().getCORE_DOMAINEnumLiteralDeclaration_0());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:18269:3: (enumLiteral_1= 'SUPPORTING_DOMAIN' )
{
// InternalContextMappingDSL.g:18269:3: (enumLiteral_1= 'SUPPORTING_DOMAIN' )
// InternalContextMappingDSL.g:18270:4: enumLiteral_1= 'SUPPORTING_DOMAIN'
{
enumLiteral_1=(Token)match(input,221,FOLLOW_2);
current = grammarAccess.getSubDomainTypeAccess().getSUPPORTING_DOMAINEnumLiteralDeclaration_1().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_1, grammarAccess.getSubDomainTypeAccess().getSUPPORTING_DOMAINEnumLiteralDeclaration_1());
}
}
break;
case 3 :
// InternalContextMappingDSL.g:18277:3: (enumLiteral_2= 'GENERIC_SUBDOMAIN' )
{
// InternalContextMappingDSL.g:18277:3: (enumLiteral_2= 'GENERIC_SUBDOMAIN' )
// InternalContextMappingDSL.g:18278:4: enumLiteral_2= 'GENERIC_SUBDOMAIN'
{
enumLiteral_2=(Token)match(input,222,FOLLOW_2);
current = grammarAccess.getSubDomainTypeAccess().getGENERIC_SUBDOMAINEnumLiteralDeclaration_2().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_2, grammarAccess.getSubDomainTypeAccess().getGENERIC_SUBDOMAINEnumLiteralDeclaration_2());
}
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleSubDomainType"
// $ANTLR start "ruleDownstreamGovernanceRights"
// InternalContextMappingDSL.g:18288:1: ruleDownstreamGovernanceRights returns [Enumerator current=null] : ( (enumLiteral_0= 'INFLUENCER' ) | (enumLiteral_1= 'OPINION_LEADER' ) | (enumLiteral_2= 'VETO_RIGHT' ) | (enumLiteral_3= 'DECISION_MAKER' ) | (enumLiteral_4= 'MONOPOLIST' ) ) ;
public final Enumerator ruleDownstreamGovernanceRights() throws RecognitionException {
Enumerator current = null;
Token enumLiteral_0=null;
Token enumLiteral_1=null;
Token enumLiteral_2=null;
Token enumLiteral_3=null;
Token enumLiteral_4=null;
enterRule();
try {
// InternalContextMappingDSL.g:18294:2: ( ( (enumLiteral_0= 'INFLUENCER' ) | (enumLiteral_1= 'OPINION_LEADER' ) | (enumLiteral_2= 'VETO_RIGHT' ) | (enumLiteral_3= 'DECISION_MAKER' ) | (enumLiteral_4= 'MONOPOLIST' ) ) )
// InternalContextMappingDSL.g:18295:2: ( (enumLiteral_0= 'INFLUENCER' ) | (enumLiteral_1= 'OPINION_LEADER' ) | (enumLiteral_2= 'VETO_RIGHT' ) | (enumLiteral_3= 'DECISION_MAKER' ) | (enumLiteral_4= 'MONOPOLIST' ) )
{
// InternalContextMappingDSL.g:18295:2: ( (enumLiteral_0= 'INFLUENCER' ) | (enumLiteral_1= 'OPINION_LEADER' ) | (enumLiteral_2= 'VETO_RIGHT' ) | (enumLiteral_3= 'DECISION_MAKER' ) | (enumLiteral_4= 'MONOPOLIST' ) )
int alt420=5;
switch ( input.LA(1) ) {
case 223:
{
alt420=1;
}
break;
case 224:
{
alt420=2;
}
break;
case 225:
{
alt420=3;
}
break;
case 226:
{
alt420=4;
}
break;
case 227:
{
alt420=5;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 420, 0, input);
throw nvae;
}
switch (alt420) {
case 1 :
// InternalContextMappingDSL.g:18296:3: (enumLiteral_0= 'INFLUENCER' )
{
// InternalContextMappingDSL.g:18296:3: (enumLiteral_0= 'INFLUENCER' )
// InternalContextMappingDSL.g:18297:4: enumLiteral_0= 'INFLUENCER'
{
enumLiteral_0=(Token)match(input,223,FOLLOW_2);
current = grammarAccess.getDownstreamGovernanceRightsAccess().getINFLUENCEREnumLiteralDeclaration_0().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_0, grammarAccess.getDownstreamGovernanceRightsAccess().getINFLUENCEREnumLiteralDeclaration_0());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:18304:3: (enumLiteral_1= 'OPINION_LEADER' )
{
// InternalContextMappingDSL.g:18304:3: (enumLiteral_1= 'OPINION_LEADER' )
// InternalContextMappingDSL.g:18305:4: enumLiteral_1= 'OPINION_LEADER'
{
enumLiteral_1=(Token)match(input,224,FOLLOW_2);
current = grammarAccess.getDownstreamGovernanceRightsAccess().getOPINION_LEADEREnumLiteralDeclaration_1().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_1, grammarAccess.getDownstreamGovernanceRightsAccess().getOPINION_LEADEREnumLiteralDeclaration_1());
}
}
break;
case 3 :
// InternalContextMappingDSL.g:18312:3: (enumLiteral_2= 'VETO_RIGHT' )
{
// InternalContextMappingDSL.g:18312:3: (enumLiteral_2= 'VETO_RIGHT' )
// InternalContextMappingDSL.g:18313:4: enumLiteral_2= 'VETO_RIGHT'
{
enumLiteral_2=(Token)match(input,225,FOLLOW_2);
current = grammarAccess.getDownstreamGovernanceRightsAccess().getVETO_RIGHTEnumLiteralDeclaration_2().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_2, grammarAccess.getDownstreamGovernanceRightsAccess().getVETO_RIGHTEnumLiteralDeclaration_2());
}
}
break;
case 4 :
// InternalContextMappingDSL.g:18320:3: (enumLiteral_3= 'DECISION_MAKER' )
{
// InternalContextMappingDSL.g:18320:3: (enumLiteral_3= 'DECISION_MAKER' )
// InternalContextMappingDSL.g:18321:4: enumLiteral_3= 'DECISION_MAKER'
{
enumLiteral_3=(Token)match(input,226,FOLLOW_2);
current = grammarAccess.getDownstreamGovernanceRightsAccess().getDECISION_MAKEREnumLiteralDeclaration_3().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_3, grammarAccess.getDownstreamGovernanceRightsAccess().getDECISION_MAKEREnumLiteralDeclaration_3());
}
}
break;
case 5 :
// InternalContextMappingDSL.g:18328:3: (enumLiteral_4= 'MONOPOLIST' )
{
// InternalContextMappingDSL.g:18328:3: (enumLiteral_4= 'MONOPOLIST' )
// InternalContextMappingDSL.g:18329:4: enumLiteral_4= 'MONOPOLIST'
{
enumLiteral_4=(Token)match(input,227,FOLLOW_2);
current = grammarAccess.getDownstreamGovernanceRightsAccess().getMONOPOLISTEnumLiteralDeclaration_4().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_4, grammarAccess.getDownstreamGovernanceRightsAccess().getMONOPOLISTEnumLiteralDeclaration_4());
}
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleDownstreamGovernanceRights"
// $ANTLR start "ruleKnowledgeLevel"
// InternalContextMappingDSL.g:18339:1: ruleKnowledgeLevel returns [Enumerator current=null] : ( (enumLiteral_0= 'META' ) | (enumLiteral_1= 'CONCRETE' ) ) ;
public final Enumerator ruleKnowledgeLevel() throws RecognitionException {
Enumerator current = null;
Token enumLiteral_0=null;
Token enumLiteral_1=null;
enterRule();
try {
// InternalContextMappingDSL.g:18345:2: ( ( (enumLiteral_0= 'META' ) | (enumLiteral_1= 'CONCRETE' ) ) )
// InternalContextMappingDSL.g:18346:2: ( (enumLiteral_0= 'META' ) | (enumLiteral_1= 'CONCRETE' ) )
{
// InternalContextMappingDSL.g:18346:2: ( (enumLiteral_0= 'META' ) | (enumLiteral_1= 'CONCRETE' ) )
int alt421=2;
int LA421_0 = input.LA(1);
if ( (LA421_0==228) ) {
alt421=1;
}
else if ( (LA421_0==229) ) {
alt421=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 421, 0, input);
throw nvae;
}
switch (alt421) {
case 1 :
// InternalContextMappingDSL.g:18347:3: (enumLiteral_0= 'META' )
{
// InternalContextMappingDSL.g:18347:3: (enumLiteral_0= 'META' )
// InternalContextMappingDSL.g:18348:4: enumLiteral_0= 'META'
{
enumLiteral_0=(Token)match(input,228,FOLLOW_2);
current = grammarAccess.getKnowledgeLevelAccess().getMETAEnumLiteralDeclaration_0().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_0, grammarAccess.getKnowledgeLevelAccess().getMETAEnumLiteralDeclaration_0());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:18355:3: (enumLiteral_1= 'CONCRETE' )
{
// InternalContextMappingDSL.g:18355:3: (enumLiteral_1= 'CONCRETE' )
// InternalContextMappingDSL.g:18356:4: enumLiteral_1= 'CONCRETE'
{
enumLiteral_1=(Token)match(input,229,FOLLOW_2);
current = grammarAccess.getKnowledgeLevelAccess().getCONCRETEEnumLiteralDeclaration_1().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_1, grammarAccess.getKnowledgeLevelAccess().getCONCRETEEnumLiteralDeclaration_1());
}
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleKnowledgeLevel"
// $ANTLR start "ruleLikelihoodForChange"
// InternalContextMappingDSL.g:18366:1: ruleLikelihoodForChange returns [Enumerator current=null] : ( (enumLiteral_0= 'NORMAL' ) | (enumLiteral_1= 'RARELY' ) | (enumLiteral_2= 'OFTEN' ) ) ;
public final Enumerator ruleLikelihoodForChange() throws RecognitionException {
Enumerator current = null;
Token enumLiteral_0=null;
Token enumLiteral_1=null;
Token enumLiteral_2=null;
enterRule();
try {
// InternalContextMappingDSL.g:18372:2: ( ( (enumLiteral_0= 'NORMAL' ) | (enumLiteral_1= 'RARELY' ) | (enumLiteral_2= 'OFTEN' ) ) )
// InternalContextMappingDSL.g:18373:2: ( (enumLiteral_0= 'NORMAL' ) | (enumLiteral_1= 'RARELY' ) | (enumLiteral_2= 'OFTEN' ) )
{
// InternalContextMappingDSL.g:18373:2: ( (enumLiteral_0= 'NORMAL' ) | (enumLiteral_1= 'RARELY' ) | (enumLiteral_2= 'OFTEN' ) )
int alt422=3;
switch ( input.LA(1) ) {
case 230:
{
alt422=1;
}
break;
case 231:
{
alt422=2;
}
break;
case 232:
{
alt422=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 422, 0, input);
throw nvae;
}
switch (alt422) {
case 1 :
// InternalContextMappingDSL.g:18374:3: (enumLiteral_0= 'NORMAL' )
{
// InternalContextMappingDSL.g:18374:3: (enumLiteral_0= 'NORMAL' )
// InternalContextMappingDSL.g:18375:4: enumLiteral_0= 'NORMAL'
{
enumLiteral_0=(Token)match(input,230,FOLLOW_2);
current = grammarAccess.getLikelihoodForChangeAccess().getNORMALEnumLiteralDeclaration_0().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_0, grammarAccess.getLikelihoodForChangeAccess().getNORMALEnumLiteralDeclaration_0());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:18382:3: (enumLiteral_1= 'RARELY' )
{
// InternalContextMappingDSL.g:18382:3: (enumLiteral_1= 'RARELY' )
// InternalContextMappingDSL.g:18383:4: enumLiteral_1= 'RARELY'
{
enumLiteral_1=(Token)match(input,231,FOLLOW_2);
current = grammarAccess.getLikelihoodForChangeAccess().getRARELYEnumLiteralDeclaration_1().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_1, grammarAccess.getLikelihoodForChangeAccess().getRARELYEnumLiteralDeclaration_1());
}
}
break;
case 3 :
// InternalContextMappingDSL.g:18390:3: (enumLiteral_2= 'OFTEN' )
{
// InternalContextMappingDSL.g:18390:3: (enumLiteral_2= 'OFTEN' )
// InternalContextMappingDSL.g:18391:4: enumLiteral_2= 'OFTEN'
{
enumLiteral_2=(Token)match(input,232,FOLLOW_2);
current = grammarAccess.getLikelihoodForChangeAccess().getOFTENEnumLiteralDeclaration_2().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_2, grammarAccess.getLikelihoodForChangeAccess().getOFTENEnumLiteralDeclaration_2());
}
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleLikelihoodForChange"
// $ANTLR start "ruleHttpMethod"
// InternalContextMappingDSL.g:18401:1: ruleHttpMethod returns [Enumerator current=null] : ( (enumLiteral_0= 'None' ) | (enumLiteral_1= 'GET' ) | (enumLiteral_2= 'POST' ) | (enumLiteral_3= 'PUT' ) | (enumLiteral_4= 'DELETE' ) ) ;
public final Enumerator ruleHttpMethod() throws RecognitionException {
Enumerator current = null;
Token enumLiteral_0=null;
Token enumLiteral_1=null;
Token enumLiteral_2=null;
Token enumLiteral_3=null;
Token enumLiteral_4=null;
enterRule();
try {
// InternalContextMappingDSL.g:18407:2: ( ( (enumLiteral_0= 'None' ) | (enumLiteral_1= 'GET' ) | (enumLiteral_2= 'POST' ) | (enumLiteral_3= 'PUT' ) | (enumLiteral_4= 'DELETE' ) ) )
// InternalContextMappingDSL.g:18408:2: ( (enumLiteral_0= 'None' ) | (enumLiteral_1= 'GET' ) | (enumLiteral_2= 'POST' ) | (enumLiteral_3= 'PUT' ) | (enumLiteral_4= 'DELETE' ) )
{
// InternalContextMappingDSL.g:18408:2: ( (enumLiteral_0= 'None' ) | (enumLiteral_1= 'GET' ) | (enumLiteral_2= 'POST' ) | (enumLiteral_3= 'PUT' ) | (enumLiteral_4= 'DELETE' ) )
int alt423=5;
switch ( input.LA(1) ) {
case 233:
{
alt423=1;
}
break;
case 234:
{
alt423=2;
}
break;
case 235:
{
alt423=3;
}
break;
case 236:
{
alt423=4;
}
break;
case 237:
{
alt423=5;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 423, 0, input);
throw nvae;
}
switch (alt423) {
case 1 :
// InternalContextMappingDSL.g:18409:3: (enumLiteral_0= 'None' )
{
// InternalContextMappingDSL.g:18409:3: (enumLiteral_0= 'None' )
// InternalContextMappingDSL.g:18410:4: enumLiteral_0= 'None'
{
enumLiteral_0=(Token)match(input,233,FOLLOW_2);
current = grammarAccess.getHttpMethodAccess().getNoneEnumLiteralDeclaration_0().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_0, grammarAccess.getHttpMethodAccess().getNoneEnumLiteralDeclaration_0());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:18417:3: (enumLiteral_1= 'GET' )
{
// InternalContextMappingDSL.g:18417:3: (enumLiteral_1= 'GET' )
// InternalContextMappingDSL.g:18418:4: enumLiteral_1= 'GET'
{
enumLiteral_1=(Token)match(input,234,FOLLOW_2);
current = grammarAccess.getHttpMethodAccess().getGETEnumLiteralDeclaration_1().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_1, grammarAccess.getHttpMethodAccess().getGETEnumLiteralDeclaration_1());
}
}
break;
case 3 :
// InternalContextMappingDSL.g:18425:3: (enumLiteral_2= 'POST' )
{
// InternalContextMappingDSL.g:18425:3: (enumLiteral_2= 'POST' )
// InternalContextMappingDSL.g:18426:4: enumLiteral_2= 'POST'
{
enumLiteral_2=(Token)match(input,235,FOLLOW_2);
current = grammarAccess.getHttpMethodAccess().getPOSTEnumLiteralDeclaration_2().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_2, grammarAccess.getHttpMethodAccess().getPOSTEnumLiteralDeclaration_2());
}
}
break;
case 4 :
// InternalContextMappingDSL.g:18433:3: (enumLiteral_3= 'PUT' )
{
// InternalContextMappingDSL.g:18433:3: (enumLiteral_3= 'PUT' )
// InternalContextMappingDSL.g:18434:4: enumLiteral_3= 'PUT'
{
enumLiteral_3=(Token)match(input,236,FOLLOW_2);
current = grammarAccess.getHttpMethodAccess().getPUTEnumLiteralDeclaration_3().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_3, grammarAccess.getHttpMethodAccess().getPUTEnumLiteralDeclaration_3());
}
}
break;
case 5 :
// InternalContextMappingDSL.g:18441:3: (enumLiteral_4= 'DELETE' )
{
// InternalContextMappingDSL.g:18441:3: (enumLiteral_4= 'DELETE' )
// InternalContextMappingDSL.g:18442:4: enumLiteral_4= 'DELETE'
{
enumLiteral_4=(Token)match(input,237,FOLLOW_2);
current = grammarAccess.getHttpMethodAccess().getDELETEEnumLiteralDeclaration_4().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_4, grammarAccess.getHttpMethodAccess().getDELETEEnumLiteralDeclaration_4());
}
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleHttpMethod"
// $ANTLR start "ruleInheritanceType"
// InternalContextMappingDSL.g:18452:1: ruleInheritanceType returns [Enumerator current=null] : ( (enumLiteral_0= 'JOINED' ) | (enumLiteral_1= 'SINGLE_TABLE' ) ) ;
public final Enumerator ruleInheritanceType() throws RecognitionException {
Enumerator current = null;
Token enumLiteral_0=null;
Token enumLiteral_1=null;
enterRule();
try {
// InternalContextMappingDSL.g:18458:2: ( ( (enumLiteral_0= 'JOINED' ) | (enumLiteral_1= 'SINGLE_TABLE' ) ) )
// InternalContextMappingDSL.g:18459:2: ( (enumLiteral_0= 'JOINED' ) | (enumLiteral_1= 'SINGLE_TABLE' ) )
{
// InternalContextMappingDSL.g:18459:2: ( (enumLiteral_0= 'JOINED' ) | (enumLiteral_1= 'SINGLE_TABLE' ) )
int alt424=2;
int LA424_0 = input.LA(1);
if ( (LA424_0==238) ) {
alt424=1;
}
else if ( (LA424_0==239) ) {
alt424=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 424, 0, input);
throw nvae;
}
switch (alt424) {
case 1 :
// InternalContextMappingDSL.g:18460:3: (enumLiteral_0= 'JOINED' )
{
// InternalContextMappingDSL.g:18460:3: (enumLiteral_0= 'JOINED' )
// InternalContextMappingDSL.g:18461:4: enumLiteral_0= 'JOINED'
{
enumLiteral_0=(Token)match(input,238,FOLLOW_2);
current = grammarAccess.getInheritanceTypeAccess().getJOINEDEnumLiteralDeclaration_0().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_0, grammarAccess.getInheritanceTypeAccess().getJOINEDEnumLiteralDeclaration_0());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:18468:3: (enumLiteral_1= 'SINGLE_TABLE' )
{
// InternalContextMappingDSL.g:18468:3: (enumLiteral_1= 'SINGLE_TABLE' )
// InternalContextMappingDSL.g:18469:4: enumLiteral_1= 'SINGLE_TABLE'
{
enumLiteral_1=(Token)match(input,239,FOLLOW_2);
current = grammarAccess.getInheritanceTypeAccess().getSINGLE_TABLEEnumLiteralDeclaration_1().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_1, grammarAccess.getInheritanceTypeAccess().getSINGLE_TABLEEnumLiteralDeclaration_1());
}
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleInheritanceType"
// $ANTLR start "ruleDiscriminatorType"
// InternalContextMappingDSL.g:18479:1: ruleDiscriminatorType returns [Enumerator current=null] : ( (enumLiteral_0= 'STRING' ) | (enumLiteral_1= 'CHAR' ) | (enumLiteral_2= 'INTEGER' ) ) ;
public final Enumerator ruleDiscriminatorType() throws RecognitionException {
Enumerator current = null;
Token enumLiteral_0=null;
Token enumLiteral_1=null;
Token enumLiteral_2=null;
enterRule();
try {
// InternalContextMappingDSL.g:18485:2: ( ( (enumLiteral_0= 'STRING' ) | (enumLiteral_1= 'CHAR' ) | (enumLiteral_2= 'INTEGER' ) ) )
// InternalContextMappingDSL.g:18486:2: ( (enumLiteral_0= 'STRING' ) | (enumLiteral_1= 'CHAR' ) | (enumLiteral_2= 'INTEGER' ) )
{
// InternalContextMappingDSL.g:18486:2: ( (enumLiteral_0= 'STRING' ) | (enumLiteral_1= 'CHAR' ) | (enumLiteral_2= 'INTEGER' ) )
int alt425=3;
switch ( input.LA(1) ) {
case 240:
{
alt425=1;
}
break;
case 241:
{
alt425=2;
}
break;
case 242:
{
alt425=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 425, 0, input);
throw nvae;
}
switch (alt425) {
case 1 :
// InternalContextMappingDSL.g:18487:3: (enumLiteral_0= 'STRING' )
{
// InternalContextMappingDSL.g:18487:3: (enumLiteral_0= 'STRING' )
// InternalContextMappingDSL.g:18488:4: enumLiteral_0= 'STRING'
{
enumLiteral_0=(Token)match(input,240,FOLLOW_2);
current = grammarAccess.getDiscriminatorTypeAccess().getSTRINGEnumLiteralDeclaration_0().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_0, grammarAccess.getDiscriminatorTypeAccess().getSTRINGEnumLiteralDeclaration_0());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:18495:3: (enumLiteral_1= 'CHAR' )
{
// InternalContextMappingDSL.g:18495:3: (enumLiteral_1= 'CHAR' )
// InternalContextMappingDSL.g:18496:4: enumLiteral_1= 'CHAR'
{
enumLiteral_1=(Token)match(input,241,FOLLOW_2);
current = grammarAccess.getDiscriminatorTypeAccess().getCHAREnumLiteralDeclaration_1().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_1, grammarAccess.getDiscriminatorTypeAccess().getCHAREnumLiteralDeclaration_1());
}
}
break;
case 3 :
// InternalContextMappingDSL.g:18503:3: (enumLiteral_2= 'INTEGER' )
{
// InternalContextMappingDSL.g:18503:3: (enumLiteral_2= 'INTEGER' )
// InternalContextMappingDSL.g:18504:4: enumLiteral_2= 'INTEGER'
{
enumLiteral_2=(Token)match(input,242,FOLLOW_2);
current = grammarAccess.getDiscriminatorTypeAccess().getINTEGEREnumLiteralDeclaration_2().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_2, grammarAccess.getDiscriminatorTypeAccess().getINTEGEREnumLiteralDeclaration_2());
}
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleDiscriminatorType"
// $ANTLR start "ruleCollectionType"
// InternalContextMappingDSL.g:18514:1: ruleCollectionType returns [Enumerator current=null] : ( (enumLiteral_0= 'None' ) | (enumLiteral_1= 'Set' ) | (enumLiteral_2= 'List' ) | (enumLiteral_3= 'Bag' ) | (enumLiteral_4= 'Collection' ) ) ;
public final Enumerator ruleCollectionType() throws RecognitionException {
Enumerator current = null;
Token enumLiteral_0=null;
Token enumLiteral_1=null;
Token enumLiteral_2=null;
Token enumLiteral_3=null;
Token enumLiteral_4=null;
enterRule();
try {
// InternalContextMappingDSL.g:18520:2: ( ( (enumLiteral_0= 'None' ) | (enumLiteral_1= 'Set' ) | (enumLiteral_2= 'List' ) | (enumLiteral_3= 'Bag' ) | (enumLiteral_4= 'Collection' ) ) )
// InternalContextMappingDSL.g:18521:2: ( (enumLiteral_0= 'None' ) | (enumLiteral_1= 'Set' ) | (enumLiteral_2= 'List' ) | (enumLiteral_3= 'Bag' ) | (enumLiteral_4= 'Collection' ) )
{
// InternalContextMappingDSL.g:18521:2: ( (enumLiteral_0= 'None' ) | (enumLiteral_1= 'Set' ) | (enumLiteral_2= 'List' ) | (enumLiteral_3= 'Bag' ) | (enumLiteral_4= 'Collection' ) )
int alt426=5;
switch ( input.LA(1) ) {
case 233:
{
alt426=1;
}
break;
case 243:
{
alt426=2;
}
break;
case 244:
{
alt426=3;
}
break;
case 245:
{
alt426=4;
}
break;
case 246:
{
alt426=5;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 426, 0, input);
throw nvae;
}
switch (alt426) {
case 1 :
// InternalContextMappingDSL.g:18522:3: (enumLiteral_0= 'None' )
{
// InternalContextMappingDSL.g:18522:3: (enumLiteral_0= 'None' )
// InternalContextMappingDSL.g:18523:4: enumLiteral_0= 'None'
{
enumLiteral_0=(Token)match(input,233,FOLLOW_2);
current = grammarAccess.getCollectionTypeAccess().getNoneEnumLiteralDeclaration_0().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_0, grammarAccess.getCollectionTypeAccess().getNoneEnumLiteralDeclaration_0());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:18530:3: (enumLiteral_1= 'Set' )
{
// InternalContextMappingDSL.g:18530:3: (enumLiteral_1= 'Set' )
// InternalContextMappingDSL.g:18531:4: enumLiteral_1= 'Set'
{
enumLiteral_1=(Token)match(input,243,FOLLOW_2);
current = grammarAccess.getCollectionTypeAccess().getSetEnumLiteralDeclaration_1().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_1, grammarAccess.getCollectionTypeAccess().getSetEnumLiteralDeclaration_1());
}
}
break;
case 3 :
// InternalContextMappingDSL.g:18538:3: (enumLiteral_2= 'List' )
{
// InternalContextMappingDSL.g:18538:3: (enumLiteral_2= 'List' )
// InternalContextMappingDSL.g:18539:4: enumLiteral_2= 'List'
{
enumLiteral_2=(Token)match(input,244,FOLLOW_2);
current = grammarAccess.getCollectionTypeAccess().getListEnumLiteralDeclaration_2().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_2, grammarAccess.getCollectionTypeAccess().getListEnumLiteralDeclaration_2());
}
}
break;
case 4 :
// InternalContextMappingDSL.g:18546:3: (enumLiteral_3= 'Bag' )
{
// InternalContextMappingDSL.g:18546:3: (enumLiteral_3= 'Bag' )
// InternalContextMappingDSL.g:18547:4: enumLiteral_3= 'Bag'
{
enumLiteral_3=(Token)match(input,245,FOLLOW_2);
current = grammarAccess.getCollectionTypeAccess().getBagEnumLiteralDeclaration_3().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_3, grammarAccess.getCollectionTypeAccess().getBagEnumLiteralDeclaration_3());
}
}
break;
case 5 :
// InternalContextMappingDSL.g:18554:3: (enumLiteral_4= 'Collection' )
{
// InternalContextMappingDSL.g:18554:3: (enumLiteral_4= 'Collection' )
// InternalContextMappingDSL.g:18555:4: enumLiteral_4= 'Collection'
{
enumLiteral_4=(Token)match(input,246,FOLLOW_2);
current = grammarAccess.getCollectionTypeAccess().getCollectionEnumLiteralDeclaration_4().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_4, grammarAccess.getCollectionTypeAccess().getCollectionEnumLiteralDeclaration_4());
}
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleCollectionType"
// $ANTLR start "ruleVisibility"
// InternalContextMappingDSL.g:18565:1: ruleVisibility returns [Enumerator current=null] : ( (enumLiteral_0= 'public' ) | (enumLiteral_1= 'protected' ) | (enumLiteral_2= 'private' ) | (enumLiteral_3= 'package' ) ) ;
public final Enumerator ruleVisibility() throws RecognitionException {
Enumerator current = null;
Token enumLiteral_0=null;
Token enumLiteral_1=null;
Token enumLiteral_2=null;
Token enumLiteral_3=null;
enterRule();
try {
// InternalContextMappingDSL.g:18571:2: ( ( (enumLiteral_0= 'public' ) | (enumLiteral_1= 'protected' ) | (enumLiteral_2= 'private' ) | (enumLiteral_3= 'package' ) ) )
// InternalContextMappingDSL.g:18572:2: ( (enumLiteral_0= 'public' ) | (enumLiteral_1= 'protected' ) | (enumLiteral_2= 'private' ) | (enumLiteral_3= 'package' ) )
{
// InternalContextMappingDSL.g:18572:2: ( (enumLiteral_0= 'public' ) | (enumLiteral_1= 'protected' ) | (enumLiteral_2= 'private' ) | (enumLiteral_3= 'package' ) )
int alt427=4;
switch ( input.LA(1) ) {
case 247:
{
alt427=1;
}
break;
case 248:
{
alt427=2;
}
break;
case 249:
{
alt427=3;
}
break;
case 124:
{
alt427=4;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 427, 0, input);
throw nvae;
}
switch (alt427) {
case 1 :
// InternalContextMappingDSL.g:18573:3: (enumLiteral_0= 'public' )
{
// InternalContextMappingDSL.g:18573:3: (enumLiteral_0= 'public' )
// InternalContextMappingDSL.g:18574:4: enumLiteral_0= 'public'
{
enumLiteral_0=(Token)match(input,247,FOLLOW_2);
current = grammarAccess.getVisibilityAccess().getPublicEnumLiteralDeclaration_0().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_0, grammarAccess.getVisibilityAccess().getPublicEnumLiteralDeclaration_0());
}
}
break;
case 2 :
// InternalContextMappingDSL.g:18581:3: (enumLiteral_1= 'protected' )
{
// InternalContextMappingDSL.g:18581:3: (enumLiteral_1= 'protected' )
// InternalContextMappingDSL.g:18582:4: enumLiteral_1= 'protected'
{
enumLiteral_1=(Token)match(input,248,FOLLOW_2);
current = grammarAccess.getVisibilityAccess().getProtectedEnumLiteralDeclaration_1().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_1, grammarAccess.getVisibilityAccess().getProtectedEnumLiteralDeclaration_1());
}
}
break;
case 3 :
// InternalContextMappingDSL.g:18589:3: (enumLiteral_2= 'private' )
{
// InternalContextMappingDSL.g:18589:3: (enumLiteral_2= 'private' )
// InternalContextMappingDSL.g:18590:4: enumLiteral_2= 'private'
{
enumLiteral_2=(Token)match(input,249,FOLLOW_2);
current = grammarAccess.getVisibilityAccess().getPrivateEnumLiteralDeclaration_2().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_2, grammarAccess.getVisibilityAccess().getPrivateEnumLiteralDeclaration_2());
}
}
break;
case 4 :
// InternalContextMappingDSL.g:18597:3: (enumLiteral_3= 'package' )
{
// InternalContextMappingDSL.g:18597:3: (enumLiteral_3= 'package' )
// InternalContextMappingDSL.g:18598:4: enumLiteral_3= 'package'
{
enumLiteral_3=(Token)match(input,124,FOLLOW_2);
current = grammarAccess.getVisibilityAccess().getPackageEnumLiteralDeclaration_3().getEnumLiteral().getInstance();
newLeafNode(enumLiteral_3, grammarAccess.getVisibilityAccess().getPackageEnumLiteralDeclaration_3());
}
}
break;
}
}
leaveRule();
}
catch (RecognitionException re) {
recover(input,re);
appendSkippedTokens();
}
finally {
}
return current;
}
// $ANTLR end "ruleVisibility"
// Delegated rules
protected DFA39 dfa39 = new DFA39(this);
protected DFA44 dfa44 = new DFA44(this);
protected DFA82 dfa82 = new DFA82(this);
protected DFA73 dfa73 = new DFA73(this);
protected DFA157 dfa157 = new DFA157(this);
protected DFA212 dfa212 = new DFA212(this);
protected DFA219 dfa219 = new DFA219(this);
protected DFA216 dfa216 = new DFA216(this);
protected DFA218 dfa218 = new DFA218(this);
protected DFA220 dfa220 = new DFA220(this);
protected DFA235 dfa235 = new DFA235(this);
protected DFA253 dfa253 = new DFA253(this);
protected DFA268 dfa268 = new DFA268(this);
protected DFA283 dfa283 = new DFA283(this);
protected DFA335 dfa335 = new DFA335(this);
protected DFA351 dfa351 = new DFA351(this);
protected DFA369 dfa369 = new DFA369(this);
protected DFA382 dfa382 = new DFA382(this);
static final String dfa_1s = "\20\uffff";
static final String dfa_2s = "\1\6\1\43\1\44\1\uffff\1\44\2\45\1\6\2\46\2\6\4\uffff";
static final String dfa_3s = "\1\43\1\47\1\44\1\uffff\1\44\2\45\1\6\2\46\2\43\4\uffff";
static final String dfa_4s = "\3\uffff\1\5\10\uffff\1\3\1\1\1\4\1\2";
static final String dfa_5s = "\20\uffff}>";
static final String[] dfa_6s = {
"\1\1\34\uffff\1\2",
"\1\4\3\uffff\1\3",
"\1\5",
"",
"\1\6",
"\1\7",
"\1\10",
"\1\11",
"\1\12",
"\1\13",
"\1\14\34\uffff\1\15",
"\1\16\34\uffff\1\17",
"",
"",
"",
""
};
static final short[] dfa_1 = DFA.unpackEncodedString(dfa_1s);
static final char[] dfa_2 = DFA.unpackEncodedStringToUnsignedChars(dfa_2s);
static final char[] dfa_3 = DFA.unpackEncodedStringToUnsignedChars(dfa_3s);
static final short[] dfa_4 = DFA.unpackEncodedString(dfa_4s);
static final short[] dfa_5 = DFA.unpackEncodedString(dfa_5s);
static final short[][] dfa_6 = unpackEncodedStringArray(dfa_6s);
class DFA39 extends DFA {
public DFA39(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 39;
this.eot = dfa_1;
this.eof = dfa_1;
this.min = dfa_2;
this.max = dfa_3;
this.accept = dfa_4;
this.special = dfa_5;
this.transition = dfa_6;
}
public String getDescription() {
return "1392:3: ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'P' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'P' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) ) | (otherlv_9= '[' otherlv_10= 'P' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'P' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) ) | ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'P' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'P' otherlv_26= ']' ) | (otherlv_27= '[' otherlv_28= 'P' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'P' otherlv_35= ']' ) | ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Partnership' ( (otherlv_38= RULE_ID ) ) ) )";
}
}
static final String dfa_7s = "\21\uffff";
static final String dfa_8s = "\1\6\1\43\1\51\1\uffff\1\51\1\uffff\2\45\1\6\2\46\2\6\4\uffff";
static final String dfa_9s = "\1\43\1\52\1\51\1\uffff\1\51\1\uffff\2\45\1\6\2\46\2\43\4\uffff";
static final String dfa_10s = "\3\uffff\1\6\1\uffff\1\5\7\uffff\1\3\1\1\1\2\1\4";
static final String dfa_11s = "\21\uffff}>";
static final String[] dfa_12s = {
"\1\1\34\uffff\1\2",
"\1\4\2\uffff\1\3\3\uffff\1\5",
"\1\6",
"",
"\1\7",
"",
"\1\10",
"\1\11",
"\1\12",
"\1\13",
"\1\14",
"\1\15\34\uffff\1\16",
"\1\20\34\uffff\1\17",
"",
"",
"",
""
};
static final short[] dfa_7 = DFA.unpackEncodedString(dfa_7s);
static final char[] dfa_8 = DFA.unpackEncodedStringToUnsignedChars(dfa_8s);
static final char[] dfa_9 = DFA.unpackEncodedStringToUnsignedChars(dfa_9s);
static final short[] dfa_10 = DFA.unpackEncodedString(dfa_10s);
static final short[] dfa_11 = DFA.unpackEncodedString(dfa_11s);
static final short[][] dfa_12 = unpackEncodedStringArray(dfa_12s);
class DFA44 extends DFA {
public DFA44(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 44;
this.eot = dfa_7;
this.eof = dfa_7;
this.min = dfa_8;
this.max = dfa_9;
this.accept = dfa_10;
this.special = dfa_11;
this.transition = dfa_12;
}
public String getDescription() {
return "1737:3: ( ( ( (otherlv_0= RULE_ID ) ) otherlv_1= '[' otherlv_2= 'SK' otherlv_3= ']' otherlv_4= '<->' otherlv_5= '[' otherlv_6= 'SK' otherlv_7= ']' ( (otherlv_8= RULE_ID ) ) ) | (otherlv_9= '[' otherlv_10= 'SK' otherlv_11= ']' ( (otherlv_12= RULE_ID ) ) otherlv_13= '<->' otherlv_14= '[' otherlv_15= 'SK' otherlv_16= ']' ( (otherlv_17= RULE_ID ) ) ) | ( ( (otherlv_18= RULE_ID ) ) otherlv_19= '[' otherlv_20= 'SK' otherlv_21= ']' otherlv_22= '<->' ( (otherlv_23= RULE_ID ) ) otherlv_24= '[' otherlv_25= 'SK' otherlv_26= ']' ) | (otherlv_27= '[' otherlv_28= 'SK' otherlv_29= ']' ( (otherlv_30= RULE_ID ) ) otherlv_31= '<->' ( (otherlv_32= RULE_ID ) ) otherlv_33= '[' otherlv_34= 'SK' otherlv_35= ']' ) | ( ( (otherlv_36= RULE_ID ) ) otherlv_37= 'Shared-Kernel' ( (otherlv_38= RULE_ID ) ) ) | ( ( (otherlv_39= RULE_ID ) ) otherlv_40= '<->' ( (otherlv_41= RULE_ID ) ) ) )";
}
}
static final String dfa_13s = "\26\uffff";
static final String dfa_14s = "\1\6\1\43\1\45\2\uffff\2\30\1\57\4\30\1\u00d2\1\56\1\63\1\64\1\u00d0\1\54\4\30";
static final String dfa_15s = "\1\6\1\66\1\u00d3\2\uffff\2\45\1\66\4\45\1\u00d3\1\65\1\u00d1\1\u00d3\1\u00d1\1\66\4\45";
static final String dfa_16s = "\3\uffff\1\1\1\2\21\uffff";
static final String dfa_17s = "\26\uffff}>";
static final String[] dfa_18s = {
"\1\1",
"\1\2\10\uffff\1\4\1\uffff\3\4\4\uffff\2\3",
"\1\7\5\uffff\1\10\1\uffff\1\11\5\uffff\2\3\u009b\uffff\1\12\1\13\1\5\1\6",
"",
"",
"\1\14\14\uffff\1\15",
"\1\14\14\uffff\1\15",
"\2\4\4\uffff\2\3",
"\1\16\14\uffff\1\4",
"\1\17\14\uffff\1\4",
"\1\20\14\uffff\1\21",
"\1\20\14\uffff\1\21",
"\1\22\1\23",
"\1\4\1\uffff\1\4\4\uffff\1\3",
"\1\3\u009c\uffff\2\4",
"\1\3\u009d\uffff\2\4",
"\1\24\1\25",
"\1\4\2\uffff\1\4\6\uffff\1\3",
"\1\14\14\uffff\1\15",
"\1\14\14\uffff\1\15",
"\1\20\14\uffff\1\21",
"\1\20\14\uffff\1\21"
};
static final short[] dfa_13 = DFA.unpackEncodedString(dfa_13s);
static final char[] dfa_14 = DFA.unpackEncodedStringToUnsignedChars(dfa_14s);
static final char[] dfa_15 = DFA.unpackEncodedStringToUnsignedChars(dfa_15s);
static final short[] dfa_16 = DFA.unpackEncodedString(dfa_16s);
static final short[] dfa_17 = DFA.unpackEncodedString(dfa_17s);
static final short[][] dfa_18 = unpackEncodedStringArray(dfa_18s);
class DFA82 extends DFA {
public DFA82(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 82;
this.eot = dfa_13;
this.eof = dfa_13;
this.min = dfa_14;
this.max = dfa_15;
this.accept = dfa_16;
this.special = dfa_17;
this.transition = dfa_18;
}
public String getDescription() {
return "2114:2: (this_CustomerSupplierRelationship_0= ruleCustomerSupplierRelationship | ( ( ( ( (otherlv_1= RULE_ID ) ) ( (otherlv_2= '[' otherlv_3= 'U' otherlv_4= ']' ) | ( (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* ) otherlv_11= ']' ) )? otherlv_12= '->' ( (otherlv_13= '[' otherlv_14= 'D' otherlv_15= ']' ) | ( (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* ) otherlv_22= ']' ) )? ( (otherlv_23= RULE_ID ) ) ) | ( ( (otherlv_24= RULE_ID ) ) ( (otherlv_25= '[' otherlv_26= 'D' otherlv_27= ']' ) | ( (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* ) otherlv_34= ']' ) )? otherlv_35= '<-' ( (otherlv_36= '[' otherlv_37= 'U' otherlv_38= ']' ) | ( (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* ) otherlv_45= ']' ) )? ( (otherlv_46= RULE_ID ) ) ) | ( ( (otherlv_47= RULE_ID ) ) (otherlv_48= '[' ( ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) ) (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )* )? otherlv_52= ']' )? otherlv_53= 'Upstream-Downstream' (otherlv_54= '[' ( ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) ) (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )* )? otherlv_58= ']' )? ( (otherlv_59= RULE_ID ) ) ) | ( ( (otherlv_60= RULE_ID ) ) (otherlv_61= '[' ( ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) ) (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )* )? otherlv_65= ']' )? otherlv_66= 'Downstream-Upstream' (otherlv_67= '[' ( ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) ) (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )* )? otherlv_71= ']' )? ( (otherlv_72= RULE_ID ) ) ) ) (otherlv_73= ':' ( (lv_name_74_0= RULE_ID ) ) )? (this_OPEN_75= RULE_OPEN ( ( ( ( ({...}? => ( ({...}? => (otherlv_77= 'implementationTechnology' (otherlv_78= '=' )? ( (lv_implementationTechnology_79_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (otherlv_80= 'exposedAggregates' (otherlv_81= '=' )? ( (otherlv_82= RULE_ID ) ) ) (otherlv_83= ',' ( (otherlv_84= RULE_ID ) ) )* ( (lv_exposedAggregatesComment_85_0= RULE_SL_COMMENT ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'downstreamRights' (otherlv_87= '=' )? ( (lv_downstreamGovernanceRights_88_0= ruleDownstreamGovernanceRights ) ) ) ) ) ) )* ) ) ) this_CLOSE_89= RULE_CLOSE )? ) )";
}
}
static final String dfa_19s = "\24\uffff";
static final String dfa_20s = "\1\6\1\43\1\45\4\uffff\2\30\1\57\2\30\1\u00d2\1\56\1\u00d0\1\54\4\30";
static final String dfa_21s = "\1\6\1\60\1\u00d3\4\uffff\2\45\1\60\2\45\1\u00d3\1\60\1\u00d1\1\57\4\45";
static final String dfa_22s = "\3\uffff\1\3\1\1\1\4\1\2\15\uffff";
static final String dfa_23s = "\24\uffff}>";
static final String[] dfa_24s = {
"\1\1",
"\1\2\10\uffff\1\4\1\uffff\1\6\1\3\1\5",
"\1\11\5\uffff\1\4\1\uffff\1\6\u00a2\uffff\1\12\1\13\1\7\1\10",
"",
"",
"",
"",
"\1\14\14\uffff\1\15",
"\1\14\14\uffff\1\15",
"\1\3\1\5",
"\1\16\14\uffff\1\17",
"\1\16\14\uffff\1\17",
"\1\20\1\21",
"\1\6\1\uffff\1\5",
"\1\22\1\23",
"\1\4\2\uffff\1\3",
"\1\14\14\uffff\1\15",
"\1\14\14\uffff\1\15",
"\1\16\14\uffff\1\17",
"\1\16\14\uffff\1\17"
};
static final short[] dfa_19 = DFA.unpackEncodedString(dfa_19s);
static final char[] dfa_20 = DFA.unpackEncodedStringToUnsignedChars(dfa_20s);
static final char[] dfa_21 = DFA.unpackEncodedStringToUnsignedChars(dfa_21s);
static final short[] dfa_22 = DFA.unpackEncodedString(dfa_22s);
static final short[] dfa_23 = DFA.unpackEncodedString(dfa_23s);
static final short[][] dfa_24 = unpackEncodedStringArray(dfa_24s);
class DFA73 extends DFA {
public DFA73(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 73;
this.eot = dfa_19;
this.eof = dfa_19;
this.min = dfa_20;
this.max = dfa_21;
this.accept = dfa_22;
this.special = dfa_23;
this.transition = dfa_24;
}
public String getDescription() {
return "2125:4: ( ( ( (otherlv_1= RULE_ID ) ) ( (otherlv_2= '[' otherlv_3= 'U' otherlv_4= ']' ) | ( (otherlv_5= '[' (otherlv_6= 'U' otherlv_7= ',' )? ( (lv_upstreamRoles_8_0= ruleUpstreamRole ) ) (otherlv_9= ',' ( (lv_upstreamRoles_10_0= ruleUpstreamRole ) ) )* ) otherlv_11= ']' ) )? otherlv_12= '->' ( (otherlv_13= '[' otherlv_14= 'D' otherlv_15= ']' ) | ( (otherlv_16= '[' (otherlv_17= 'D' otherlv_18= ',' )? ( (lv_downstreamRoles_19_0= ruleDownstreamRole ) ) (otherlv_20= ',' ( (lv_downstreamRoles_21_0= ruleDownstreamRole ) ) )* ) otherlv_22= ']' ) )? ( (otherlv_23= RULE_ID ) ) ) | ( ( (otherlv_24= RULE_ID ) ) ( (otherlv_25= '[' otherlv_26= 'D' otherlv_27= ']' ) | ( (otherlv_28= '[' (otherlv_29= 'D' otherlv_30= ',' )? ( (lv_downstreamRoles_31_0= ruleDownstreamRole ) ) (otherlv_32= ',' ( (lv_downstreamRoles_33_0= ruleDownstreamRole ) ) )* ) otherlv_34= ']' ) )? otherlv_35= '<-' ( (otherlv_36= '[' otherlv_37= 'U' otherlv_38= ']' ) | ( (otherlv_39= '[' (otherlv_40= 'U' otherlv_41= ',' )? ( (lv_upstreamRoles_42_0= ruleUpstreamRole ) ) (otherlv_43= ',' ( (lv_upstreamRoles_44_0= ruleUpstreamRole ) ) )* ) otherlv_45= ']' ) )? ( (otherlv_46= RULE_ID ) ) ) | ( ( (otherlv_47= RULE_ID ) ) (otherlv_48= '[' ( ( (lv_upstreamRoles_49_0= ruleUpstreamRole ) ) (otherlv_50= ',' ( (lv_upstreamRoles_51_0= ruleUpstreamRole ) ) )* )? otherlv_52= ']' )? otherlv_53= 'Upstream-Downstream' (otherlv_54= '[' ( ( (lv_downstreamRoles_55_0= ruleDownstreamRole ) ) (otherlv_56= ',' ( (lv_downstreamRoles_57_0= ruleDownstreamRole ) ) )* )? otherlv_58= ']' )? ( (otherlv_59= RULE_ID ) ) ) | ( ( (otherlv_60= RULE_ID ) ) (otherlv_61= '[' ( ( (lv_downstreamRoles_62_0= ruleDownstreamRole ) ) (otherlv_63= ',' ( (lv_downstreamRoles_64_0= ruleDownstreamRole ) ) )* )? otherlv_65= ']' )? otherlv_66= 'Downstream-Upstream' (otherlv_67= '[' ( ( (lv_upstreamRoles_68_0= ruleUpstreamRole ) ) (otherlv_69= ',' ( (lv_upstreamRoles_70_0= ruleUpstreamRole ) ) )* )? otherlv_71= ']' )? ( (otherlv_72= RULE_ID ) ) ) )";
}
}
static final String dfa_25s = "\12\uffff";
static final String dfa_26s = "\1\5\1\uffff\1\5\1\67\5\uffff\1\67";
static final String dfa_27s = "\1\u00b7\1\uffff\1\u0090\1\u00b7\5\uffff\1\u0090";
static final String dfa_28s = "\1\uffff\1\6\2\uffff\1\1\1\2\1\3\1\4\1\5\1\uffff";
static final String dfa_29s = "\12\uffff}>";
static final String[] dfa_30s = {
"\1\3\2\uffff\1\1\1\2\55\uffff\1\10\35\uffff\1\4\3\uffff\1\5\2\uffff\1\6\33\uffff\2\7\16\uffff\1\7\2\uffff\3\7\2\uffff\2\7\45\uffff\1\7",
"",
"\1\11\61\uffff\1\10\35\uffff\1\4\42\uffff\2\7\16\uffff\1\7\2\uffff\2\7\3\uffff\1\7",
"\1\10\35\uffff\1\4\3\uffff\1\5\2\uffff\1\6\33\uffff\2\7\16\uffff\1\7\2\uffff\3\7\2\uffff\2\7\45\uffff\1\7",
"",
"",
"",
"",
"",
"\1\10\35\uffff\1\4\42\uffff\2\7\16\uffff\1\7\2\uffff\2\7\3\uffff\1\7"
};
static final short[] dfa_25 = DFA.unpackEncodedString(dfa_25s);
static final char[] dfa_26 = DFA.unpackEncodedStringToUnsignedChars(dfa_26s);
static final char[] dfa_27 = DFA.unpackEncodedStringToUnsignedChars(dfa_27s);
static final short[] dfa_28 = DFA.unpackEncodedString(dfa_28s);
static final short[] dfa_29 = DFA.unpackEncodedString(dfa_29s);
static final short[][] dfa_30 = unpackEncodedStringArray(dfa_30s);
class DFA157 extends DFA {
public DFA157(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 157;
this.eot = dfa_25;
this.eof = dfa_25;
this.min = dfa_26;
this.max = dfa_27;
this.accept = dfa_28;
this.special = dfa_29;
this.transition = dfa_30;
}
public String getDescription() {
return "()* loopback of 5265:4: ( ( (lv_services_11_0= ruleService ) ) | ( (lv_resources_12_0= ruleResource ) ) | ( (lv_consumers_13_0= ruleConsumer ) ) | ( (lv_domainObjects_14_0= ruleSimpleDomainObject ) ) | ( (lv_aggregates_15_0= ruleAggregate ) ) )*";
}
}
static final String dfa_31s = "\17\uffff";
static final String dfa_32s = "\1\12\16\uffff";
static final String dfa_33s = "\1\164\16\uffff";
static final String dfa_34s = "\1\uffff\1\16\1\1\1\2\1\3\1\4\1\5\1\6\1\7\1\10\1\11\1\12\1\13\1\14\1\15";
static final String dfa_35s = "\1\0\16\uffff}>";
static final String[] dfa_36s = {
"\1\1\107\uffff\1\3\3\uffff\2\5\14\uffff\1\16\3\uffff\1\2\1\1\2\uffff\1\4\1\6\1\7\1\10\1\11\1\12\1\13\1\14\1\15",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] dfa_31 = DFA.unpackEncodedString(dfa_31s);
static final char[] dfa_32 = DFA.unpackEncodedStringToUnsignedChars(dfa_32s);
static final char[] dfa_33 = DFA.unpackEncodedStringToUnsignedChars(dfa_33s);
static final short[] dfa_34 = DFA.unpackEncodedString(dfa_34s);
static final short[] dfa_35 = DFA.unpackEncodedString(dfa_35s);
static final short[][] dfa_36 = unpackEncodedStringArray(dfa_36s);
class DFA212 extends DFA {
public DFA212(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 212;
this.eot = dfa_31;
this.eof = dfa_31;
this.min = dfa_32;
this.max = dfa_33;
this.accept = dfa_34;
this.special = dfa_35;
this.transition = dfa_36;
}
public String getDescription() {
return "()* loopback of 7460:6: ( ({...}? => ( ({...}? => (otherlv_11= 'throws' ( (lv_throws_12_0= ruleThrowsIdentifier ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_13= 'hint' otherlv_14= '=' ( (lv_hint_15_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_cache_16_0= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapOperation_17_0= 'gap' ) ) | ( (lv_noGapOperation_18_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_19= 'query' otherlv_20= '=' ( (lv_query_21_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_22= 'condition' otherlv_23= '=' ( (lv_condition_24_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_25= 'select' otherlv_26= '=' ( (lv_select_27_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'groupBy' otherlv_29= '=' ( (lv_groupBy_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_31= 'orderBy' otherlv_32= '=' ( (lv_orderBy_33_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_construct_34_0= 'construct' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_build_35_0= 'build' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_map_36_0= 'map' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_publish_37_0= rulePublish ) ) ) ) ) )*";
}
public int specialStateTransition(int s, IntStream _input) throws NoViableAltException {
TokenStream input = (TokenStream)_input;
int _s = s;
switch ( s ) {
case 0 :
int LA212_0 = input.LA(1);
int index212_0 = input.index();
input.rewind();
s = -1;
if ( (LA212_0==RULE_DELEGATE||LA212_0==105) ) {s = 1;}
else if ( LA212_0 == 104 && getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 0) ) {s = 2;}
else if ( LA212_0 == 82 && getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 1) ) {s = 3;}
else if ( LA212_0 == 108 && getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 2) ) {s = 4;}
else if ( LA212_0 >= 86 && LA212_0 <= 87 && getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 3) ) {s = 5;}
else if ( LA212_0 == 109 && getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 4) ) {s = 6;}
else if ( LA212_0 == 110 && getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 5) ) {s = 7;}
else if ( LA212_0 == 111 && getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 6) ) {s = 8;}
else if ( LA212_0 == 112 && getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 7) ) {s = 9;}
else if ( LA212_0 == 113 && getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 8) ) {s = 10;}
else if ( LA212_0 == 114 && getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 9) ) {s = 11;}
else if ( LA212_0 == 115 && getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 10) ) {s = 12;}
else if ( LA212_0 == 116 && getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 11) ) {s = 13;}
else if ( LA212_0 == 100 && getUnorderedGroupHelper().canSelect(grammarAccess.getRepositoryOperationAccess().getUnorderedGroup_5(), 12) ) {s = 14;}
input.seek(index212_0);
if ( s>=0 ) return s;
break;
}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 212, _s, input);
error(nvae);
throw nvae;
}
}
static final String dfa_37s = "\37\uffff";
static final String dfa_38s = "\1\uffff\27\34\6\uffff\1\34";
static final String dfa_39s = "\30\6\5\uffff\2\6";
static final String dfa_40s = "\1\u00f6\27\166\5\uffff\1\6\1\166";
static final String dfa_41s = "\30\uffff\1\3\1\4\1\5\1\2\1\1\2\uffff";
static final String dfa_42s = "\37\uffff}>";
static final String[] dfa_43s = {
"\1\27\4\uffff\1\32\122\uffff\1\30\132\uffff\1\1\1\2\1\3\1\4\1\5\1\6\1\7\1\10\1\11\1\12\1\13\1\14\1\15\1\16\1\17\1\20\1\21\1\22\1\23\1\24\1\25\1\26\32\uffff\1\31\11\uffff\4\31",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\157\uffff\1\33",
"\1\34\143\uffff\1\35\13\uffff\1\33",
"",
"",
"",
"",
"",
"\1\36",
"\1\34\143\uffff\1\35\13\uffff\1\33"
};
static final short[] dfa_37 = DFA.unpackEncodedString(dfa_37s);
static final short[] dfa_38 = DFA.unpackEncodedString(dfa_38s);
static final char[] dfa_39 = DFA.unpackEncodedStringToUnsignedChars(dfa_39s);
static final char[] dfa_40 = DFA.unpackEncodedStringToUnsignedChars(dfa_40s);
static final short[] dfa_41 = DFA.unpackEncodedString(dfa_41s);
static final short[] dfa_42 = DFA.unpackEncodedString(dfa_42s);
static final short[][] dfa_43 = unpackEncodedStringArray(dfa_43s);
class DFA219 extends DFA {
public DFA219(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 219;
this.eot = dfa_37;
this.eof = dfa_38;
this.min = dfa_39;
this.max = dfa_40;
this.accept = dfa_41;
this.special = dfa_42;
this.transition = dfa_43;
}
public String getDescription() {
return "8025:2: ( ( (lv_type_0_0= ruleType ) ) | ( ( (lv_type_1_0= ruleType ) ) otherlv_2= '<' otherlv_3= '@' ( (otherlv_4= RULE_ID ) ) otherlv_5= '>' ) | (otherlv_6= '@' ( (otherlv_7= RULE_ID ) ) ) | ( ( (lv_collectionType_8_0= ruleCollectionType ) ) otherlv_9= '<' ( (otherlv_10= '@' ( (otherlv_11= RULE_ID ) ) ) | ( (lv_type_12_0= ruleType ) ) | ( ( (lv_type_13_0= ruleType ) ) otherlv_14= '<' otherlv_15= '@' ( (otherlv_16= RULE_ID ) ) otherlv_17= '>' ) ) otherlv_18= '>' ) | ( ( (lv_mapCollectionType_19_0= RULE_MAP_COLLECTION_TYPE ) ) otherlv_20= '<' ( ( (lv_mapKeyType_21_0= ruleType ) ) | (otherlv_22= '@' ( (otherlv_23= RULE_ID ) ) ) ) otherlv_24= ',' ( (otherlv_25= '@' ( (otherlv_26= RULE_ID ) ) ) | ( (lv_type_27_0= ruleType ) ) | ( ( (lv_type_28_0= ruleType ) ) otherlv_29= '<' otherlv_30= '@' ( (otherlv_31= RULE_ID ) ) otherlv_32= '>' ) ) otherlv_33= '>' ) )";
}
}
static final String dfa_44s = "\35\uffff";
static final String dfa_45s = "\1\6\1\uffff\26\166\1\152\2\uffff\1\6\1\152";
static final String dfa_46s = "\1\u00ce\1\uffff\27\167\2\uffff\1\6\1\167";
static final String dfa_47s = "\1\uffff\1\1\27\uffff\1\3\1\2\2\uffff";
static final String dfa_48s = "\35\uffff}>";
static final String[] dfa_49s = {
"\1\30\127\uffff\1\1\132\uffff\1\2\1\3\1\4\1\5\1\6\1\7\1\10\1\11\1\12\1\13\1\14\1\15\1\16\1\17\1\20\1\21\1\22\1\23\1\24\1\25\1\26\1\27",
"",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\31\1\32",
"\1\33\13\uffff\1\31\1\32",
"",
"",
"\1\34",
"\1\33\13\uffff\1\31\1\32"
};
static final short[] dfa_44 = DFA.unpackEncodedString(dfa_44s);
static final char[] dfa_45 = DFA.unpackEncodedStringToUnsignedChars(dfa_45s);
static final char[] dfa_46 = DFA.unpackEncodedStringToUnsignedChars(dfa_46s);
static final short[] dfa_47 = DFA.unpackEncodedString(dfa_47s);
static final short[] dfa_48 = DFA.unpackEncodedString(dfa_48s);
static final short[][] dfa_49 = unpackEncodedStringArray(dfa_49s);
class DFA216 extends DFA {
public DFA216(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 216;
this.eot = dfa_44;
this.eof = dfa_44;
this.min = dfa_45;
this.max = dfa_46;
this.accept = dfa_47;
this.special = dfa_48;
this.transition = dfa_49;
}
public String getDescription() {
return "8137:4: ( (otherlv_10= '@' ( (otherlv_11= RULE_ID ) ) ) | ( (lv_type_12_0= ruleType ) ) | ( ( (lv_type_13_0= ruleType ) ) otherlv_14= '<' otherlv_15= '@' ( (otherlv_16= RULE_ID ) ) otherlv_17= '>' ) )";
}
}
static final String dfa_50s = "\1\uffff\1\1\27\uffff\1\2\1\3\2\uffff";
static final String[] dfa_51s = {
"\1\30\127\uffff\1\1\132\uffff\1\2\1\3\1\4\1\5\1\6\1\7\1\10\1\11\1\12\1\13\1\14\1\15\1\16\1\17\1\20\1\21\1\22\1\23\1\24\1\25\1\26\1\27",
"",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\32\1\31",
"\1\33\13\uffff\1\32\1\31",
"",
"",
"\1\34",
"\1\33\13\uffff\1\32\1\31"
};
static final short[] dfa_50 = DFA.unpackEncodedString(dfa_50s);
static final short[][] dfa_51 = unpackEncodedStringArray(dfa_51s);
class DFA218 extends DFA {
public DFA218(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 218;
this.eot = dfa_44;
this.eof = dfa_44;
this.min = dfa_45;
this.max = dfa_46;
this.accept = dfa_50;
this.special = dfa_48;
this.transition = dfa_51;
}
public String getDescription() {
return "8299:4: ( (otherlv_25= '@' ( (otherlv_26= RULE_ID ) ) ) | ( (lv_type_27_0= ruleType ) ) | ( ( (lv_type_28_0= ruleType ) ) otherlv_29= '<' otherlv_30= '@' ( (otherlv_31= RULE_ID ) ) otherlv_32= '>' ) )";
}
}
static final String dfa_52s = "\1\5\1\170\2\uffff\1\5\1\171\3\uffff\1\170";
static final String dfa_53s = "\2\u00b7\2\uffff\2\u0090\3\uffff\1\u0090";
static final String dfa_54s = "\2\uffff\1\1\1\2\2\uffff\1\3\1\4\1\5\1\uffff";
static final String[] dfa_55s = {
"\1\1\3\uffff\1\4\156\uffff\1\5\1\6\16\uffff\1\6\2\uffff\2\6\1\10\2\uffff\1\7\1\2\45\uffff\1\3",
"\1\5\1\6\16\uffff\1\6\2\uffff\2\6\1\10\2\uffff\1\7\1\2\45\uffff\1\3",
"",
"",
"\1\11\162\uffff\1\5\1\6\16\uffff\1\6\2\uffff\2\6\3\uffff\1\7",
"\1\6\16\uffff\1\6\2\uffff\2\6\3\uffff\1\7",
"",
"",
"",
"\1\5\1\6\16\uffff\1\6\2\uffff\2\6\3\uffff\1\7"
};
static final char[] dfa_52 = DFA.unpackEncodedStringToUnsignedChars(dfa_52s);
static final char[] dfa_53 = DFA.unpackEncodedStringToUnsignedChars(dfa_53s);
static final short[] dfa_54 = DFA.unpackEncodedString(dfa_54s);
static final short[][] dfa_55 = unpackEncodedStringArray(dfa_55s);
class DFA220 extends DFA {
public DFA220(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 220;
this.eot = dfa_25;
this.eof = dfa_25;
this.min = dfa_52;
this.max = dfa_53;
this.accept = dfa_54;
this.special = dfa_29;
this.transition = dfa_55;
}
public String getDescription() {
return "8410:2: (this_BasicType_0= ruleBasicType | this_Enum_1= ruleEnum | this_DomainObject_2= ruleDomainObject | this_DataTransferObject_3= ruleDataTransferObject | this_Trait_4= ruleTrait )";
}
}
static final String dfa_56s = "\22\uffff";
static final String dfa_57s = "\1\5\1\uffff\1\154\17\uffff";
static final String dfa_58s = "\1\u00f9\1\uffff\1\176\17\uffff";
static final String dfa_59s = "\1\uffff\1\20\1\uffff\1\1\1\2\1\3\1\4\1\5\1\6\1\7\1\10\1\11\1\12\1\13\1\14\1\15\1\16\1\17";
static final String dfa_60s = "\1\0\1\uffff\1\1\17\uffff}>";
static final String[] dfa_61s = {
"\2\1\1\uffff\1\1\3\uffff\1\2\1\1\104\uffff\1\10\3\uffff\2\6\2\uffff\1\7\21\uffff\1\5\17\uffff\1\1\1\3\1\4\1\11\1\12\1\13\1\14\1\15\1\16\1\17\1\20\1\21\6\uffff\2\1\45\uffff\1\1\3\uffff\26\1\32\uffff\1\1\11\uffff\7\1",
"",
"\1\5\20\uffff\1\3\1\4",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] dfa_56 = DFA.unpackEncodedString(dfa_56s);
static final char[] dfa_57 = DFA.unpackEncodedStringToUnsignedChars(dfa_57s);
static final char[] dfa_58 = DFA.unpackEncodedStringToUnsignedChars(dfa_58s);
static final short[] dfa_59 = DFA.unpackEncodedString(dfa_59s);
static final short[] dfa_60 = DFA.unpackEncodedString(dfa_60s);
static final short[][] dfa_61 = unpackEncodedStringArray(dfa_61s);
class DFA235 extends DFA {
public DFA235(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 235;
this.eot = dfa_56;
this.eof = dfa_56;
this.min = dfa_57;
this.max = dfa_58;
this.accept = dfa_59;
this.special = dfa_60;
this.transition = dfa_61;
}
public String getDescription() {
return "()* loopback of 8703:7: ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notAuditable_20_0= RULE_NOT ) ) otherlv_21= 'auditable' ) | otherlv_22= 'auditable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_53_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_54= 'belongsTo' ( (otherlv_55= '@' )? ( (otherlv_56= RULE_ID ) ) ) ) ) ) ) )*";
}
public int specialStateTransition(int s, IntStream _input) throws NoViableAltException {
TokenStream input = (TokenStream)_input;
int _s = s;
switch ( s ) {
case 0 :
int LA235_0 = input.LA(1);
int index235_0 = input.index();
input.rewind();
s = -1;
if ( ((LA235_0>=RULE_STRING && LA235_0<=RULE_ID)||LA235_0==RULE_CLOSE||LA235_0==RULE_REF||LA235_0==124||(LA235_0>=142 && LA235_0<=143)||LA235_0==181||(LA235_0>=185 && LA235_0<=206)||LA235_0==233||(LA235_0>=243 && LA235_0<=249)) ) {s = 1;}
else if ( LA235_0 == RULE_NOT && ( getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 2) || getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 1) || getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 0) ) ) {s = 2;}
else if ( LA235_0 == 125 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 0) ) {s = 3;}
else if ( LA235_0 == 126 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 1) ) {s = 4;}
else if ( LA235_0 == 108 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 2) ) {s = 5;}
else if ( LA235_0 >= 86 && LA235_0 <= 87 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 3) ) {s = 6;}
else if ( LA235_0 == 90 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 4) ) {s = 7;}
else if ( LA235_0 == 82 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 5) ) {s = 8;}
else if ( LA235_0 == 127 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 6) ) {s = 9;}
else if ( LA235_0 == 128 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 7) ) {s = 10;}
else if ( LA235_0 == 129 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 8) ) {s = 11;}
else if ( LA235_0 == 130 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 9) ) {s = 12;}
else if ( LA235_0 == 131 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 10) ) {s = 13;}
else if ( LA235_0 == 132 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 11) ) {s = 14;}
else if ( LA235_0 == 133 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 12) ) {s = 15;}
else if ( LA235_0 == 134 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 13) ) {s = 16;}
else if ( LA235_0 == 135 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 14) ) {s = 17;}
input.seek(index235_0);
if ( s>=0 ) return s;
break;
case 1 :
int LA235_2 = input.LA(1);
int index235_2 = input.index();
input.rewind();
s = -1;
if ( LA235_2 == 125 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 0) ) {s = 3;}
else if ( LA235_2 == 126 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 1) ) {s = 4;}
else if ( LA235_2 == 108 && getUnorderedGroupHelper().canSelect(grammarAccess.getEntityAccess().getUnorderedGroup_7_2(), 2) ) {s = 5;}
input.seek(index235_2);
if ( s>=0 ) return s;
break;
}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 235, _s, input);
error(nvae);
throw nvae;
}
}
static final String dfa_62s = "\23\uffff";
static final String dfa_63s = "\1\5\1\uffff\1\154\20\uffff";
static final String dfa_64s = "\1\u00f9\1\uffff\1\u008a\20\uffff";
static final String dfa_65s = "\1\uffff\1\21\1\uffff\1\1\1\2\1\3\1\4\1\5\1\6\1\7\1\10\1\11\1\12\1\13\1\14\1\15\1\16\1\17\1\20";
static final String dfa_66s = "\1\1\1\uffff\1\0\20\uffff}>";
static final String[] dfa_67s = {
"\2\1\1\uffff\1\1\3\uffff\1\2\1\1\104\uffff\1\10\3\uffff\2\6\2\uffff\1\7\21\uffff\1\5\17\uffff\1\1\1\3\1\uffff\1\11\1\12\1\13\1\14\1\15\1\16\1\17\1\21\1\22\1\uffff\1\4\1\20\3\uffff\2\1\45\uffff\1\1\3\uffff\26\1\32\uffff\1\1\11\uffff\7\1",
"",
"\1\5\20\uffff\1\3\13\uffff\1\4\1\20",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] dfa_62 = DFA.unpackEncodedString(dfa_62s);
static final char[] dfa_63 = DFA.unpackEncodedStringToUnsignedChars(dfa_63s);
static final char[] dfa_64 = DFA.unpackEncodedStringToUnsignedChars(dfa_64s);
static final short[] dfa_65 = DFA.unpackEncodedString(dfa_65s);
static final short[] dfa_66 = DFA.unpackEncodedString(dfa_66s);
static final short[][] dfa_67 = unpackEncodedStringArray(dfa_67s);
class DFA253 extends DFA {
public DFA253(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 253;
this.eot = dfa_62;
this.eof = dfa_62;
this.min = dfa_63;
this.max = dfa_64;
this.accept = dfa_65;
this.special = dfa_66;
this.transition = dfa_67;
}
public String getDescription() {
return "()* loopback of 9535:7: ( ({...}? => ( ({...}? => ( ( ( (lv_notOptimisticLocking_17_0= RULE_NOT ) ) otherlv_18= 'optimisticLocking' ) | otherlv_19= 'optimisticLocking' ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notImmutable_20_0= RULE_NOT ) ) otherlv_21= 'immutable' ) | otherlv_22= 'immutable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_23_0= 'cache' ) ) | (this_NOT_24= RULE_NOT otherlv_25= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_26_0= 'gap' ) ) | ( (lv_noGapClass_27_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_28_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'hint' otherlv_30= '=' ( (lv_hint_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'databaseTable' otherlv_33= '=' ( (lv_databaseTable_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorValue' otherlv_36= '=' ( (lv_discriminatorValue_37_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorColumn' otherlv_39= '=' ( (lv_discriminatorColumn_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'discriminatorType' otherlv_42= '=' ( (lv_discriminatorType_43_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'discriminatorLength' otherlv_45= '=' ( (lv_discriminatorLength_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_47= 'inheritanceType' otherlv_48= '=' ( (lv_inheritanceType_49_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_50= 'validate' otherlv_51= '=' ( (lv_validate_52_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_persistent_53_0= 'persistent' ) ) | ( ( (lv_notPersistent_54_0= RULE_NOT ) ) otherlv_55= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_56_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'belongsTo' ( (otherlv_58= '@' )? ( (otherlv_59= RULE_ID ) ) ) ) ) ) ) )*";
}
public int specialStateTransition(int s, IntStream _input) throws NoViableAltException {
TokenStream input = (TokenStream)_input;
int _s = s;
switch ( s ) {
case 0 :
int LA253_2 = input.LA(1);
int index253_2 = input.index();
input.rewind();
s = -1;
if ( LA253_2 == 125 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 0) ) {s = 3;}
else if ( LA253_2 == 137 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 1) ) {s = 4;}
else if ( LA253_2 == 138 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 13) ) {s = 16;}
else if ( LA253_2 == 108 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 2) ) {s = 5;}
input.seek(index253_2);
if ( s>=0 ) return s;
break;
case 1 :
int LA253_0 = input.LA(1);
int index253_0 = input.index();
input.rewind();
s = -1;
if ( ((LA253_0>=RULE_STRING && LA253_0<=RULE_ID)||LA253_0==RULE_CLOSE||LA253_0==RULE_REF||LA253_0==124||(LA253_0>=142 && LA253_0<=143)||LA253_0==181||(LA253_0>=185 && LA253_0<=206)||LA253_0==233||(LA253_0>=243 && LA253_0<=249)) ) {s = 1;}
else if ( LA253_0 == RULE_NOT && ( getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 0) || getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 13) || getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 2) || getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 1) ) ) {s = 2;}
else if ( LA253_0 == 125 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 0) ) {s = 3;}
else if ( LA253_0 == 137 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 1) ) {s = 4;}
else if ( LA253_0 == 108 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 2) ) {s = 5;}
else if ( LA253_0 >= 86 && LA253_0 <= 87 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 3) ) {s = 6;}
else if ( LA253_0 == 90 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 4) ) {s = 7;}
else if ( LA253_0 == 82 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 5) ) {s = 8;}
else if ( LA253_0 == 127 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 6) ) {s = 9;}
else if ( LA253_0 == 128 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 7) ) {s = 10;}
else if ( LA253_0 == 129 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 8) ) {s = 11;}
else if ( LA253_0 == 130 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 9) ) {s = 12;}
else if ( LA253_0 == 131 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 10) ) {s = 13;}
else if ( LA253_0 == 132 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 11) ) {s = 14;}
else if ( LA253_0 == 133 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 12) ) {s = 15;}
else if ( LA253_0 == 138 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 13) ) {s = 16;}
else if ( LA253_0 == 134 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 14) ) {s = 17;}
else if ( LA253_0 == 135 && getUnorderedGroupHelper().canSelect(grammarAccess.getValueObjectAccess().getUnorderedGroup_7_2(), 15) ) {s = 18;}
input.seek(index253_0);
if ( s>=0 ) return s;
break;
}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 253, _s, input);
error(nvae);
throw nvae;
}
}
static final String dfa_68s = "\1\5\17\uffff";
static final String dfa_69s = "\1\u00f9\17\uffff";
static final String dfa_70s = "\1\uffff\1\17\1\1\1\2\1\3\1\4\1\5\1\6\1\7\1\10\1\11\1\12\1\13\1\14\1\15\1\16";
static final String dfa_71s = "\1\0\17\uffff}>";
static final String[] dfa_72s = {
"\2\1\1\uffff\1\1\3\uffff\1\2\1\1\104\uffff\1\5\3\uffff\2\3\2\uffff\1\4\21\uffff\1\2\17\uffff\1\1\2\uffff\1\6\1\7\1\10\1\11\1\12\1\13\1\14\1\16\1\17\2\uffff\1\15\3\uffff\2\1\45\uffff\1\1\3\uffff\26\1\32\uffff\1\1\11\uffff\7\1",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final char[] dfa_68 = DFA.unpackEncodedStringToUnsignedChars(dfa_68s);
static final char[] dfa_69 = DFA.unpackEncodedStringToUnsignedChars(dfa_69s);
static final short[] dfa_70 = DFA.unpackEncodedString(dfa_70s);
static final short[] dfa_71 = DFA.unpackEncodedString(dfa_71s);
static final short[][] dfa_72 = unpackEncodedStringArray(dfa_72s);
class DFA268 extends DFA {
public DFA268(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 268;
this.eot = dfa_1;
this.eof = dfa_1;
this.min = dfa_68;
this.max = dfa_69;
this.accept = dfa_70;
this.special = dfa_71;
this.transition = dfa_72;
}
public String getDescription() {
return "()* loopback of 10417:7: ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )*";
}
public int specialStateTransition(int s, IntStream _input) throws NoViableAltException {
TokenStream input = (TokenStream)_input;
int _s = s;
switch ( s ) {
case 0 :
int LA268_0 = input.LA(1);
int index268_0 = input.index();
input.rewind();
s = -1;
if ( ((LA268_0>=RULE_STRING && LA268_0<=RULE_ID)||LA268_0==RULE_CLOSE||LA268_0==RULE_REF||LA268_0==124||(LA268_0>=142 && LA268_0<=143)||LA268_0==181||(LA268_0>=185 && LA268_0<=206)||LA268_0==233||(LA268_0>=243 && LA268_0<=249)) ) {s = 1;}
else if ( ( LA268_0 == RULE_NOT || LA268_0 == 108 ) && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 0) ) {s = 2;}
else if ( LA268_0 >= 86 && LA268_0 <= 87 && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 1) ) {s = 3;}
else if ( LA268_0 == 90 && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 2) ) {s = 4;}
else if ( LA268_0 == 82 && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 3) ) {s = 5;}
else if ( LA268_0 == 127 && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 4) ) {s = 6;}
else if ( LA268_0 == 128 && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 5) ) {s = 7;}
else if ( LA268_0 == 129 && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 6) ) {s = 8;}
else if ( LA268_0 == 130 && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 7) ) {s = 9;}
else if ( LA268_0 == 131 && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 8) ) {s = 10;}
else if ( LA268_0 == 132 && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 9) ) {s = 11;}
else if ( LA268_0 == 133 && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 10) ) {s = 12;}
else if ( LA268_0 == 138 && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 11) ) {s = 13;}
else if ( LA268_0 == 134 && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 12) ) {s = 14;}
else if ( LA268_0 == 135 && getUnorderedGroupHelper().canSelect(grammarAccess.getDomainEventAccess().getUnorderedGroup_7_2(), 13) ) {s = 15;}
input.seek(index268_0);
if ( s>=0 ) return s;
break;
}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 268, _s, input);
error(nvae);
throw nvae;
}
}
class DFA283 extends DFA {
public DFA283(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 283;
this.eot = dfa_1;
this.eof = dfa_1;
this.min = dfa_68;
this.max = dfa_69;
this.accept = dfa_70;
this.special = dfa_71;
this.transition = dfa_72;
}
public String getDescription() {
return "()* loopback of 11192:7: ( ({...}? => ( ({...}? => ( ( (lv_cache_17_0= 'cache' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_gapClass_20_0= 'gap' ) ) | ( (lv_noGapClass_21_0= 'nogap' ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_scaffold_22_0= 'scaffold' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_23= 'hint' otherlv_24= '=' ( (lv_hint_25_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'databaseTable' otherlv_27= '=' ( (lv_databaseTable_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_29= 'discriminatorValue' otherlv_30= '=' ( (lv_discriminatorValue_31_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'discriminatorColumn' otherlv_33= '=' ( (lv_discriminatorColumn_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'discriminatorType' otherlv_36= '=' ( (lv_discriminatorType_37_0= ruleDiscriminatorType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_38= 'discriminatorLength' otherlv_39= '=' ( (lv_discriminatorLength_40_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_41= 'inheritanceType' otherlv_42= '=' ( (lv_inheritanceType_43_0= ruleInheritanceType ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_44= 'validate' otherlv_45= '=' ( (lv_validate_46_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_persistent_47_0= 'persistent' ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_aggregateRoot_48_0= 'aggregateRoot' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'belongsTo' ( (otherlv_50= '@' )? ( (otherlv_51= RULE_ID ) ) ) ) ) ) ) )*";
}
public int specialStateTransition(int s, IntStream _input) throws NoViableAltException {
TokenStream input = (TokenStream)_input;
int _s = s;
switch ( s ) {
case 0 :
int LA283_0 = input.LA(1);
int index283_0 = input.index();
input.rewind();
s = -1;
if ( ((LA283_0>=RULE_STRING && LA283_0<=RULE_ID)||LA283_0==RULE_CLOSE||LA283_0==RULE_REF||LA283_0==124||(LA283_0>=142 && LA283_0<=143)||LA283_0==181||(LA283_0>=185 && LA283_0<=206)||LA283_0==233||(LA283_0>=243 && LA283_0<=249)) ) {s = 1;}
else if ( ( LA283_0 == RULE_NOT || LA283_0 == 108 ) && getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 0) ) {s = 2;}
else if ( LA283_0 >= 86 && LA283_0 <= 87 && getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 1) ) {s = 3;}
else if ( LA283_0 == 90 && getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 2) ) {s = 4;}
else if ( LA283_0 == 82 && getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 3) ) {s = 5;}
else if ( LA283_0 == 127 && getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 4) ) {s = 6;}
else if ( LA283_0 == 128 && getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 5) ) {s = 7;}
else if ( LA283_0 == 129 && getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 6) ) {s = 8;}
else if ( LA283_0 == 130 && getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 7) ) {s = 9;}
else if ( LA283_0 == 131 && getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 8) ) {s = 10;}
else if ( LA283_0 == 132 && getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 9) ) {s = 11;}
else if ( LA283_0 == 133 && getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 10) ) {s = 12;}
else if ( LA283_0 == 138 && getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 11) ) {s = 13;}
else if ( LA283_0 == 134 && getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 12) ) {s = 14;}
else if ( LA283_0 == 135 && getUnorderedGroupHelper().canSelect(grammarAccess.getCommandEventAccess().getUnorderedGroup_7_2(), 13) ) {s = 15;}
input.seek(index283_0);
if ( s>=0 ) return s;
break;
}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 283, _s, input);
error(nvae);
throw nvae;
}
}
static final String dfa_73s = "\40\uffff";
static final String dfa_74s = "\1\1\37\uffff";
static final String dfa_75s = "\1\5\2\uffff\1\u0093\34\uffff";
static final String dfa_76s = "\1\u00f9\2\uffff\1\u0095\34\uffff";
static final String dfa_77s = "\1\uffff\1\36\1\1\1\uffff\1\2\1\3\1\4\1\5\1\6\1\7\1\10\1\11\1\12\1\13\1\14\1\15\1\16\1\17\1\20\1\21\1\22\1\23\1\24\1\25\1\26\1\27\1\30\1\31\1\32\1\33\1\34\1\35";
static final String dfa_78s = "\1\0\2\uffff\1\1\34\uffff}>";
static final String[] dfa_79s = {
"\2\1\1\uffff\1\1\3\uffff\1\3\1\1\104\uffff\1\12\26\uffff\1\1\22\uffff\1\1\10\uffff\1\34\10\uffff\2\1\2\uffff\1\2\1\4\1\5\1\6\1\7\1\10\1\11\1\13\1\14\1\15\1\16\1\17\1\20\1\21\1\22\1\23\1\24\1\25\1\26\1\27\1\30\1\31\1\32\1\33\1\35\1\36\1\37\10\uffff\1\1\3\uffff\26\1\32\uffff\1\1\11\uffff\7\1",
"",
"",
"\1\4\1\5\1\6",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] dfa_73 = DFA.unpackEncodedString(dfa_73s);
static final short[] dfa_74 = DFA.unpackEncodedString(dfa_74s);
static final char[] dfa_75 = DFA.unpackEncodedStringToUnsignedChars(dfa_75s);
static final char[] dfa_76 = DFA.unpackEncodedStringToUnsignedChars(dfa_76s);
static final short[] dfa_77 = DFA.unpackEncodedString(dfa_77s);
static final short[] dfa_78 = DFA.unpackEncodedString(dfa_78s);
static final short[][] dfa_79 = unpackEncodedStringArray(dfa_79s);
class DFA335 extends DFA {
public DFA335(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 335;
this.eot = dfa_73;
this.eof = dfa_74;
this.min = dfa_75;
this.max = dfa_76;
this.accept = dfa_77;
this.special = dfa_78;
this.transition = dfa_79;
}
public String getDescription() {
return "()* loopback of 13030:6: ( ({...}? => ( ({...}? => ( (lv_key_9_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_10_0= RULE_NOT ) ) otherlv_11= 'changeable' ) | otherlv_12= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_13_0= 'required' ) ) | (this_NOT_14= RULE_NOT otherlv_15= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_16_0= 'nullable' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'nullable' ) ) (otherlv_19= '=' ( (lv_nullableMessage_20_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_index_21_0= 'index' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_22_0= 'assertFalse' ) ) (otherlv_23= '=' ( (lv_assertFalseMessage_24_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_25_0= 'assertTrue' ) ) (otherlv_26= '=' ( (lv_assertTrueMessage_27_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_28= 'hint' otherlv_29= '=' ( (lv_hint_30_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_31_0= 'creditCardNumber' ) ) (otherlv_32= '=' ( (lv_creditCardNumberMessage_33_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_34= 'digits' otherlv_35= '=' ( (lv_digits_36_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_37_0= 'email' ) ) (otherlv_38= '=' ( (lv_emailMessage_39_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_40_0= 'future' ) ) (otherlv_41= '=' ( (lv_futureMessage_42_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_43_0= 'past' ) ) (otherlv_44= '=' ( (lv_pastMessage_45_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_46= 'max' otherlv_47= '=' ( (lv_max_48_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_49= 'min' otherlv_50= '=' ( (lv_min_51_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_52= 'decimalMax' otherlv_53= '=' ( (lv_decimalMax_54_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_55= 'decimalMin' otherlv_56= '=' ( (lv_decimalMin_57_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_58_0= 'notEmpty' ) ) (otherlv_59= '=' ( (lv_notEmptyMessage_60_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_61_0= 'notBlank' ) ) (otherlv_62= '=' ( (lv_notBlankMessage_63_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_64= 'pattern' otherlv_65= '=' ( (lv_pattern_66_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_67= 'range' otherlv_68= '=' ( (lv_range_69_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_70= 'size' otherlv_71= '=' ( (lv_size_72_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_73= 'length' otherlv_74= '=' ( (lv_length_75_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_76= 'scriptAssert' otherlv_77= '=' ( (lv_scriptAssert_78_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_79= 'url' otherlv_80= '=' ( (lv_url_81_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_82= 'validate' otherlv_83= '=' ( (lv_validate_84_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_85_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_86= 'databaseColumn' otherlv_87= '=' ( (lv_databaseColumn_88_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_89= 'databaseType' otherlv_90= '=' ( (lv_databaseType_91_0= RULE_STRING ) ) ) ) ) ) )*";
}
public int specialStateTransition(int s, IntStream _input) throws NoViableAltException {
TokenStream input = (TokenStream)_input;
int _s = s;
switch ( s ) {
case 0 :
int LA335_0 = input.LA(1);
int index335_0 = input.index();
input.rewind();
s = -1;
if ( (LA335_0==EOF||(LA335_0>=RULE_STRING && LA335_0<=RULE_ID)||LA335_0==RULE_CLOSE||LA335_0==RULE_REF||LA335_0==105||LA335_0==124||(LA335_0>=142 && LA335_0<=143)||LA335_0==181||(LA335_0>=185 && LA335_0<=206)||LA335_0==233||(LA335_0>=243 && LA335_0<=249)) ) {s = 1;}
else if ( LA335_0 == 146 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 0) ) {s = 2;}
else if ( LA335_0 == RULE_NOT && ( getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 2) || getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 1) || getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 3) ) ) {s = 3;}
else if ( LA335_0 == 147 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 1) ) {s = 4;}
else if ( LA335_0 == 148 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 2) ) {s = 5;}
else if ( LA335_0 == 149 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 3) ) {s = 6;}
else if ( LA335_0 == 150 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 4) ) {s = 7;}
else if ( LA335_0 == 151 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 5) ) {s = 8;}
else if ( LA335_0 == 152 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 6) ) {s = 9;}
else if ( LA335_0 == 82 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 7) ) {s = 10;}
else if ( LA335_0 == 153 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 8) ) {s = 11;}
else if ( LA335_0 == 154 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 9) ) {s = 12;}
else if ( LA335_0 == 155 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 10) ) {s = 13;}
else if ( LA335_0 == 156 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 11) ) {s = 14;}
else if ( LA335_0 == 157 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 12) ) {s = 15;}
else if ( LA335_0 == 158 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 13) ) {s = 16;}
else if ( LA335_0 == 159 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 14) ) {s = 17;}
else if ( LA335_0 == 160 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 15) ) {s = 18;}
else if ( LA335_0 == 161 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 16) ) {s = 19;}
else if ( LA335_0 == 162 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 17) ) {s = 20;}
else if ( LA335_0 == 163 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 18) ) {s = 21;}
else if ( LA335_0 == 164 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 19) ) {s = 22;}
else if ( LA335_0 == 165 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 20) ) {s = 23;}
else if ( LA335_0 == 166 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 21) ) {s = 24;}
else if ( LA335_0 == 167 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 22) ) {s = 25;}
else if ( LA335_0 == 168 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 23) ) {s = 26;}
else if ( LA335_0 == 169 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 24) ) {s = 27;}
else if ( LA335_0 == 133 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 25) ) {s = 28;}
else if ( LA335_0 == 170 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 26) ) {s = 29;}
else if ( LA335_0 == 171 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 27) ) {s = 30;}
else if ( LA335_0 == 172 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 28) ) {s = 31;}
input.seek(index335_0);
if ( s>=0 ) return s;
break;
case 1 :
int LA335_3 = input.LA(1);
int index335_3 = input.index();
input.rewind();
s = -1;
if ( LA335_3 == 147 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 1) ) {s = 4;}
else if ( LA335_3 == 148 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 2) ) {s = 5;}
else if ( LA335_3 == 149 && getUnorderedGroupHelper().canSelect(grammarAccess.getAttributeAccess().getUnorderedGroup_4(), 3) ) {s = 6;}
input.seek(index335_3);
if ( s>=0 ) return s;
break;
}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 335, _s, input);
error(nvae);
throw nvae;
}
}
static final String dfa_80s = "\27\uffff";
static final String dfa_81s = "\1\1\26\uffff";
static final String dfa_82s = "\1\5\2\uffff\1\154\23\uffff";
static final String dfa_83s = "\1\u00f9\2\uffff\1\u00af\23\uffff";
static final String dfa_84s = "\1\uffff\1\25\1\1\1\uffff\1\2\1\3\1\4\1\5\1\6\1\7\1\10\1\11\1\12\1\13\1\14\1\15\1\16\1\17\1\20\1\21\1\22\1\23\1\24";
static final String dfa_85s = "\1\1\2\uffff\1\0\23\uffff}>";
static final String[] dfa_86s = {
"\2\1\1\uffff\1\1\3\uffff\1\3\1\1\1\26\103\uffff\1\7\26\uffff\1\1\2\uffff\1\12\17\uffff\1\1\10\uffff\1\22\10\uffff\2\1\2\uffff\1\2\1\4\1\5\1\6\14\uffff\1\17\3\uffff\1\20\3\uffff\1\23\1\14\1\uffff\1\10\1\11\1\13\1\15\1\16\1\21\1\24\1\25\1\1\3\uffff\26\1\32\uffff\1\1\11\uffff\7\1",
"",
"",
"\1\12\46\uffff\1\4\1\5\1\6\31\uffff\1\13",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] dfa_80 = DFA.unpackEncodedString(dfa_80s);
static final short[] dfa_81 = DFA.unpackEncodedString(dfa_81s);
static final char[] dfa_82 = DFA.unpackEncodedStringToUnsignedChars(dfa_82s);
static final char[] dfa_83 = DFA.unpackEncodedStringToUnsignedChars(dfa_83s);
static final short[] dfa_84 = DFA.unpackEncodedString(dfa_84s);
static final short[] dfa_85 = DFA.unpackEncodedString(dfa_85s);
static final short[][] dfa_86 = unpackEncodedStringArray(dfa_86s);
class DFA351 extends DFA {
public DFA351(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 351;
this.eot = dfa_80;
this.eof = dfa_81;
this.min = dfa_82;
this.max = dfa_83;
this.accept = dfa_84;
this.special = dfa_85;
this.transition = dfa_86;
}
public String getDescription() {
return "()* loopback of 14356:6: ( ({...}? => ( ({...}? => ( (lv_key_12_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_13_0= RULE_NOT ) ) otherlv_14= 'changeable' ) | otherlv_15= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_16_0= 'required' ) ) | (this_NOT_17= RULE_NOT otherlv_18= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_19_0= 'nullable' ) ) | (this_NOT_20= RULE_NOT otherlv_21= 'nullable' ) ) (otherlv_22= '=' ( (lv_nullableMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_24= 'hint' otherlv_25= '=' ( (lv_hint_26_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_27= 'cascade' otherlv_28= '=' ( (lv_cascade_29_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'fetch' otherlv_31= '=' ( (lv_fetch_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_cache_33_0= 'cache' ) ) | (this_NOT_34= RULE_NOT otherlv_35= 'cache' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_inverse_36_0= 'inverse' ) ) | (this_NOT_37= RULE_NOT otherlv_38= 'inverse' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_39= 'databaseColumn' otherlv_40= '=' ( (lv_databaseColumn_41_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'databaseJoinTable' otherlv_43= '=' ( (lv_databaseJoinTable_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'databaseJoinColumn' otherlv_46= '=' ( (lv_databaseJoinColumn_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_48_0= 'notEmpty' ) ) (otherlv_49= '=' ( (lv_notEmptyMessage_50_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'size' otherlv_52= '=' ( (lv_size_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_54_0= 'valid' ) ) (otherlv_55= '=' ( (lv_validMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_57= 'validate' otherlv_58= '=' ( (lv_validate_59_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_60_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_61= 'orderby' otherlv_62= '=' ( (lv_orderBy_63_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_orderColumn_64_0= 'orderColumn' ) ) (otherlv_65= '=' ( (lv_orderColumnName_66_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_oppositeHolder_67_0= ruleOppositeHolder ) ) ) ) ) )*";
}
public int specialStateTransition(int s, IntStream _input) throws NoViableAltException {
TokenStream input = (TokenStream)_input;
int _s = s;
switch ( s ) {
case 0 :
int LA351_3 = input.LA(1);
int index351_3 = input.index();
input.rewind();
s = -1;
if ( LA351_3 == 148 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 2) ) {s = 5;}
else if ( LA351_3 == 149 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 3) ) {s = 6;}
else if ( LA351_3 == 108 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 7) ) {s = 10;}
else if ( LA351_3 == 175 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 8) ) {s = 11;}
else if ( LA351_3 == 147 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 1) ) {s = 4;}
input.seek(index351_3);
if ( s>=0 ) return s;
break;
case 1 :
int LA351_0 = input.LA(1);
int index351_0 = input.index();
input.rewind();
s = -1;
if ( (LA351_0==EOF||(LA351_0>=RULE_STRING && LA351_0<=RULE_ID)||LA351_0==RULE_CLOSE||LA351_0==RULE_REF||LA351_0==105||LA351_0==124||(LA351_0>=142 && LA351_0<=143)||LA351_0==181||(LA351_0>=185 && LA351_0<=206)||LA351_0==233||(LA351_0>=243 && LA351_0<=249)) ) {s = 1;}
else if ( LA351_0 == 146 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 0) ) {s = 2;}
else if ( LA351_0 == RULE_NOT && ( getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 2) || getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 1) || getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 3) || getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 8) || getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 7) ) ) {s = 3;}
else if ( LA351_0 == 147 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 1) ) {s = 4;}
else if ( LA351_0 == 148 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 2) ) {s = 5;}
else if ( LA351_0 == 149 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 3) ) {s = 6;}
else if ( LA351_0 == 82 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 4) ) {s = 7;}
else if ( LA351_0 == 173 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 5) ) {s = 8;}
else if ( LA351_0 == 174 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 6) ) {s = 9;}
else if ( LA351_0 == 108 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 7) ) {s = 10;}
else if ( LA351_0 == 175 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 8) ) {s = 11;}
else if ( LA351_0 == 171 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 9) ) {s = 12;}
else if ( LA351_0 == 176 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 10) ) {s = 13;}
else if ( LA351_0 == 177 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 11) ) {s = 14;}
else if ( LA351_0 == 162 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 12) ) {s = 15;}
else if ( LA351_0 == 166 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 13) ) {s = 16;}
else if ( LA351_0 == 178 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 14) ) {s = 17;}
else if ( LA351_0 == 133 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 15) ) {s = 18;}
else if ( LA351_0 == 170 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 16) ) {s = 19;}
else if ( LA351_0 == 179 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 17) ) {s = 20;}
else if ( LA351_0 == 180 && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 18) ) {s = 21;}
else if ( LA351_0 == RULE_OPPOSITE && getUnorderedGroupHelper().canSelect(grammarAccess.getReferenceAccess().getUnorderedGroup_5(), 19) ) {s = 22;}
input.seek(index351_0);
if ( s>=0 ) return s;
break;
}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 351, _s, input);
error(nvae);
throw nvae;
}
}
static final String dfa_87s = "\1\1\34\uffff";
static final String dfa_88s = "\1\5\2\uffff\1\u0093\31\uffff";
static final String dfa_89s = "\1\u00f9\2\uffff\1\u0095\31\uffff";
static final String dfa_90s = "\1\uffff\1\33\1\1\1\uffff\1\2\1\3\1\4\1\5\1\6\1\7\1\10\1\11\1\12\1\13\1\14\1\15\1\16\1\17\1\20\1\21\1\22\1\23\1\24\1\25\1\26\1\27\1\30\1\31\1\32";
static final String dfa_91s = "\1\1\2\uffff\1\0\31\uffff}>";
static final String[] dfa_92s = {
"\2\1\1\uffff\1\1\3\uffff\1\3\1\1\104\uffff\1\34\26\uffff\1\1\22\uffff\1\1\10\uffff\1\33\14\uffff\1\2\1\4\1\5\1\6\1\uffff\1\10\1\11\1\12\1\13\1\14\1\15\1\16\1\17\1\20\1\21\1\22\1\23\1\24\1\25\1\26\1\27\1\30\1\31\1\32\1\7\16\uffff\26\1\32\uffff\1\1\11\uffff\7\1",
"",
"",
"\1\4\1\5\1\6",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] dfa_87 = DFA.unpackEncodedString(dfa_87s);
static final char[] dfa_88 = DFA.unpackEncodedStringToUnsignedChars(dfa_88s);
static final char[] dfa_89 = DFA.unpackEncodedStringToUnsignedChars(dfa_89s);
static final short[] dfa_90 = DFA.unpackEncodedString(dfa_90s);
static final short[] dfa_91 = DFA.unpackEncodedString(dfa_91s);
static final short[][] dfa_92 = unpackEncodedStringArray(dfa_92s);
class DFA369 extends DFA {
public DFA369(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 369;
this.eot = dfa_44;
this.eof = dfa_87;
this.min = dfa_88;
this.max = dfa_89;
this.accept = dfa_90;
this.special = dfa_91;
this.transition = dfa_92;
}
public String getDescription() {
return "()* loopback of 15264:6: ( ({...}? => ( ({...}? => ( (lv_key_8_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_9_0= RULE_NOT ) ) otherlv_10= 'changeable' ) | otherlv_11= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_12_0= 'required' ) ) | (this_NOT_13= RULE_NOT otherlv_14= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_15_0= 'nullable' ) ) | (this_NOT_16= RULE_NOT otherlv_17= 'nullable' ) ) (otherlv_18= '=' ( (lv_nullableMessage_19_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_20_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertFalse_21_0= 'assertFalse' ) ) (otherlv_22= '=' ( (lv_assertFalseMessage_23_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_assertTrue_24_0= 'assertTrue' ) ) (otherlv_25= '=' ( (lv_assertTrueMessage_26_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_creditCardNumber_27_0= 'creditCardNumber' ) ) (otherlv_28= '=' ( (lv_creditCardNumberMessage_29_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_30= 'digits' otherlv_31= '=' ( (lv_digits_32_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_email_33_0= 'email' ) ) (otherlv_34= '=' ( (lv_emailMessage_35_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_future_36_0= 'future' ) ) (otherlv_37= '=' ( (lv_futureMessage_38_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_past_39_0= 'past' ) ) (otherlv_40= '=' ( (lv_pastMessage_41_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_42= 'max' otherlv_43= '=' ( (lv_max_44_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_45= 'min' otherlv_46= '=' ( (lv_min_47_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_48= 'decimalMax' otherlv_49= '=' ( (lv_decimalMax_50_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_51= 'decimalMin' otherlv_52= '=' ( (lv_decimalMin_53_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_54_0= 'notEmpty' ) ) (otherlv_55= '=' ( (lv_notEmptyMessage_56_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notBlank_57_0= 'notBlank' ) ) (otherlv_58= '=' ( (lv_notBlankMessage_59_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_60= 'pattern' otherlv_61= '=' ( (lv_pattern_62_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_63= 'range' otherlv_64= '=' ( (lv_range_65_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_66= 'size' otherlv_67= '=' ( (lv_size_68_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_69= 'length' otherlv_70= '=' ( (lv_length_71_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_72= 'scriptAssert' otherlv_73= '=' ( (lv_scriptAssert_74_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_75= 'url' otherlv_76= '=' ( (lv_url_77_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_78= 'validate' otherlv_79= '=' ( (lv_validate_80_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_81= 'hint' otherlv_82= '=' ( (lv_hint_83_0= RULE_STRING ) ) ) ) ) ) )*";
}
public int specialStateTransition(int s, IntStream _input) throws NoViableAltException {
TokenStream input = (TokenStream)_input;
int _s = s;
switch ( s ) {
case 0 :
int LA369_3 = input.LA(1);
int index369_3 = input.index();
input.rewind();
s = -1;
if ( LA369_3 == 147 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 1) ) {s = 4;}
else if ( LA369_3 == 148 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 2) ) {s = 5;}
else if ( LA369_3 == 149 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 3) ) {s = 6;}
input.seek(index369_3);
if ( s>=0 ) return s;
break;
case 1 :
int LA369_0 = input.LA(1);
int index369_0 = input.index();
input.rewind();
s = -1;
if ( (LA369_0==EOF||(LA369_0>=RULE_STRING && LA369_0<=RULE_ID)||LA369_0==RULE_CLOSE||LA369_0==RULE_REF||LA369_0==105||LA369_0==124||(LA369_0>=185 && LA369_0<=206)||LA369_0==233||(LA369_0>=243 && LA369_0<=249)) ) {s = 1;}
else if ( LA369_0 == 146 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 0) ) {s = 2;}
else if ( LA369_0 == RULE_NOT && ( getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 2) || getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 3) || getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 1) ) ) {s = 3;}
else if ( LA369_0 == 147 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 1) ) {s = 4;}
else if ( LA369_0 == 148 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 2) ) {s = 5;}
else if ( LA369_0 == 149 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 3) ) {s = 6;}
else if ( LA369_0 == 170 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 4) ) {s = 7;}
else if ( LA369_0 == 151 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 5) ) {s = 8;}
else if ( LA369_0 == 152 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 6) ) {s = 9;}
else if ( LA369_0 == 153 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 7) ) {s = 10;}
else if ( LA369_0 == 154 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 8) ) {s = 11;}
else if ( LA369_0 == 155 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 9) ) {s = 12;}
else if ( LA369_0 == 156 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 10) ) {s = 13;}
else if ( LA369_0 == 157 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 11) ) {s = 14;}
else if ( LA369_0 == 158 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 12) ) {s = 15;}
else if ( LA369_0 == 159 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 13) ) {s = 16;}
else if ( LA369_0 == 160 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 14) ) {s = 17;}
else if ( LA369_0 == 161 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 15) ) {s = 18;}
else if ( LA369_0 == 162 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 16) ) {s = 19;}
else if ( LA369_0 == 163 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 17) ) {s = 20;}
else if ( LA369_0 == 164 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 18) ) {s = 21;}
else if ( LA369_0 == 165 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 19) ) {s = 22;}
else if ( LA369_0 == 166 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 20) ) {s = 23;}
else if ( LA369_0 == 167 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 21) ) {s = 24;}
else if ( LA369_0 == 168 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 22) ) {s = 25;}
else if ( LA369_0 == 169 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 23) ) {s = 26;}
else if ( LA369_0 == 133 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 24) ) {s = 27;}
else if ( LA369_0 == 82 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoAttributeAccess().getUnorderedGroup_6(), 25) ) {s = 28;}
input.seek(index369_0);
if ( s>=0 ) return s;
break;
}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 369, _s, input);
error(nvae);
throw nvae;
}
}
static final String dfa_93s = "\15\uffff";
static final String dfa_94s = "\1\1\14\uffff";
static final String dfa_95s = "\1\5\2\uffff\1\u0093\11\uffff";
static final String dfa_96s = "\1\u00f9\2\uffff\1\u0095\11\uffff";
static final String dfa_97s = "\1\uffff\1\13\1\1\1\uffff\1\2\1\3\1\4\1\5\1\6\1\7\1\10\1\11\1\12";
static final String dfa_98s = "\1\1\2\uffff\1\0\11\uffff}>";
static final String[] dfa_99s = {
"\2\1\1\uffff\1\1\3\uffff\1\3\1\1\104\uffff\1\14\26\uffff\1\1\22\uffff\1\1\10\uffff\1\13\14\uffff\1\2\1\4\1\5\1\6\14\uffff\1\10\3\uffff\1\11\3\uffff\1\7\7\uffff\1\12\6\uffff\26\1\32\uffff\1\1\11\uffff\7\1",
"",
"",
"\1\4\1\5\1\6",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] dfa_93 = DFA.unpackEncodedString(dfa_93s);
static final short[] dfa_94 = DFA.unpackEncodedString(dfa_94s);
static final char[] dfa_95 = DFA.unpackEncodedStringToUnsignedChars(dfa_95s);
static final char[] dfa_96 = DFA.unpackEncodedStringToUnsignedChars(dfa_96s);
static final short[] dfa_97 = DFA.unpackEncodedString(dfa_97s);
static final short[] dfa_98 = DFA.unpackEncodedString(dfa_98s);
static final short[][] dfa_99 = unpackEncodedStringArray(dfa_99s);
class DFA382 extends DFA {
public DFA382(BaseRecognizer recognizer) {
this.recognizer = recognizer;
this.decisionNumber = 382;
this.eot = dfa_93;
this.eof = dfa_94;
this.min = dfa_95;
this.max = dfa_96;
this.accept = dfa_97;
this.special = dfa_98;
this.transition = dfa_99;
}
public String getDescription() {
return "()* loopback of 16471:6: ( ({...}? => ( ({...}? => ( (lv_key_10_0= 'key' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_notChangeable_11_0= RULE_NOT ) ) otherlv_12= 'changeable' ) | otherlv_13= 'changeable' ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_required_14_0= 'required' ) ) | (this_NOT_15= RULE_NOT otherlv_16= 'required' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( ( (lv_nullable_17_0= 'nullable' ) ) | (this_NOT_18= RULE_NOT otherlv_19= 'nullable' ) ) (otherlv_20= '=' ( (lv_nullableMessage_21_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => ( (lv_transient_22_0= 'transient' ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_notEmpty_23_0= 'notEmpty' ) ) (otherlv_24= '=' ( (lv_notEmptyMessage_25_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_26= 'size' otherlv_27= '=' ( (lv_size_28_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => ( ( (lv_valid_29_0= 'valid' ) ) (otherlv_30= '=' ( (lv_validMessage_31_0= RULE_STRING ) ) )? ) ) ) ) | ({...}? => ( ({...}? => (otherlv_32= 'validate' otherlv_33= '=' ( (lv_validate_34_0= RULE_STRING ) ) ) ) ) ) | ({...}? => ( ({...}? => (otherlv_35= 'hint' otherlv_36= '=' ( (lv_hint_37_0= RULE_STRING ) ) ) ) ) ) )*";
}
public int specialStateTransition(int s, IntStream _input) throws NoViableAltException {
TokenStream input = (TokenStream)_input;
int _s = s;
switch ( s ) {
case 0 :
int LA382_3 = input.LA(1);
int index382_3 = input.index();
input.rewind();
s = -1;
if ( LA382_3 == 147 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 1) ) {s = 4;}
else if ( LA382_3 == 148 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 2) ) {s = 5;}
else if ( LA382_3 == 149 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 3) ) {s = 6;}
input.seek(index382_3);
if ( s>=0 ) return s;
break;
case 1 :
int LA382_0 = input.LA(1);
int index382_0 = input.index();
input.rewind();
s = -1;
if ( (LA382_0==EOF||(LA382_0>=RULE_STRING && LA382_0<=RULE_ID)||LA382_0==RULE_CLOSE||LA382_0==RULE_REF||LA382_0==105||LA382_0==124||(LA382_0>=185 && LA382_0<=206)||LA382_0==233||(LA382_0>=243 && LA382_0<=249)) ) {s = 1;}
else if ( LA382_0 == 146 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 0) ) {s = 2;}
else if ( LA382_0 == RULE_NOT && ( getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 2) || getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 3) || getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 1) ) ) {s = 3;}
else if ( LA382_0 == 147 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 1) ) {s = 4;}
else if ( LA382_0 == 148 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 2) ) {s = 5;}
else if ( LA382_0 == 149 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 3) ) {s = 6;}
else if ( LA382_0 == 170 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 4) ) {s = 7;}
else if ( LA382_0 == 162 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 5) ) {s = 8;}
else if ( LA382_0 == 166 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 6) ) {s = 9;}
else if ( LA382_0 == 178 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 7) ) {s = 10;}
else if ( LA382_0 == 133 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 8) ) {s = 11;}
else if ( LA382_0 == 82 && getUnorderedGroupHelper().canSelect(grammarAccess.getDtoReferenceAccess().getUnorderedGroup_7(), 9) ) {s = 12;}
input.seek(index382_0);
if ( s>=0 ) return s;
break;
}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 382, _s, input);
error(nvae);
throw nvae;
}
}
public static final BitSet FOLLOW_1 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_2 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_3 = new BitSet(new long[]{0x10000002020C0012L,0x0000000000000010L});
public static final BitSet FOLLOW_4 = new BitSet(new long[]{0x0000000000000020L});
public static final BitSet FOLLOW_5 = new BitSet(new long[]{0x00000000000000C0L});
public static final BitSet FOLLOW_6 = new BitSet(new long[]{0x0000000000000080L});
public static final BitSet FOLLOW_7 = new BitSet(new long[]{0x0000000800D00140L});
public static final BitSet FOLLOW_8 = new BitSet(new long[]{0x0000000000200000L,0x0000000000000000L,0x0000000000000000L,0x0000000000C00000L});
public static final BitSet FOLLOW_9 = new BitSet(new long[]{0x0000000000200000L,0x0000000000000000L,0x0000000000000000L,0x0000000000300000L});
public static final BitSet FOLLOW_10 = new BitSet(new long[]{0x0000000000000040L});
public static final BitSet FOLLOW_11 = new BitSet(new long[]{0x0000000801800140L});
public static final BitSet FOLLOW_12 = new BitSet(new long[]{0x0000000800000140L});
public static final BitSet FOLLOW_13 = new BitSet(new long[]{0x0000000002000000L});
public static final BitSet FOLLOW_14 = new BitSet(new long[]{0x000000001C000082L});
public static final BitSet FOLLOW_15 = new BitSet(new long[]{0x000000001D000082L});
public static final BitSet FOLLOW_16 = new BitSet(new long[]{0x00800001E0100320L,0x0000000000008000L});
public static final BitSet FOLLOW_17 = new BitSet(new long[]{0x0000000000200020L});
public static final BitSet FOLLOW_18 = new BitSet(new long[]{0x0000000000200000L,0x0000000000000000L,0x0000000000000000L,0x000000000F000000L});
public static final BitSet FOLLOW_19 = new BitSet(new long[]{0x00800001E1100320L,0x0000000000008000L});
public static final BitSet FOLLOW_20 = new BitSet(new long[]{0x0000000000200000L,0x0000000000000000L,0x0000000000000000L,0x0000003000000000L});
public static final BitSet FOLLOW_21 = new BitSet(new long[]{0x0080000000000320L,0x0000000000008000L});
public static final BitSet FOLLOW_22 = new BitSet(new long[]{0x0080000000000320L});
public static final BitSet FOLLOW_23 = new BitSet(new long[]{0x0000000000000082L});
public static final BitSet FOLLOW_24 = new BitSet(new long[]{0x0000000420000100L});
public static final BitSet FOLLOW_25 = new BitSet(new long[]{0x0000000020100320L,0x0300000000200000L});
public static final BitSet FOLLOW_26 = new BitSet(new long[]{0x0000000000200000L,0x0000000000000000L,0x0000000000000000L,0x0000000070000000L});
public static final BitSet FOLLOW_27 = new BitSet(new long[]{0x0000000000000320L,0x0300000000200000L});
public static final BitSet FOLLOW_28 = new BitSet(new long[]{0x0000000000000320L,0x0000000000200000L});
public static final BitSet FOLLOW_29 = new BitSet(new long[]{0x0000000800000000L});
public static final BitSet FOLLOW_30 = new BitSet(new long[]{0x0000001000000000L});
public static final BitSet FOLLOW_31 = new BitSet(new long[]{0x0000002000000000L});
public static final BitSet FOLLOW_32 = new BitSet(new long[]{0x0000004000000000L});
public static final BitSet FOLLOW_33 = new BitSet(new long[]{0x0000010000000082L});
public static final BitSet FOLLOW_34 = new BitSet(new long[]{0x0000008000000000L});
public static final BitSet FOLLOW_35 = new BitSet(new long[]{0x0000000080000100L});
public static final BitSet FOLLOW_36 = new BitSet(new long[]{0x0000000000000100L});
public static final BitSet FOLLOW_37 = new BitSet(new long[]{0x0000020000000000L});
public static final BitSet FOLLOW_38 = new BitSet(new long[]{0x0000040000000000L});
public static final BitSet FOLLOW_39 = new BitSet(new long[]{0x0000100800000000L});
public static final BitSet FOLLOW_40 = new BitSet(new long[]{0x0000080000000000L});
public static final BitSet FOLLOW_41 = new BitSet(new long[]{0x0000100000000000L});
public static final BitSet FOLLOW_42 = new BitSet(new long[]{0x0000080000000000L,0x0000000000000000L,0x0000000000000000L,0x0000000000030000L});
public static final BitSet FOLLOW_43 = new BitSet(new long[]{0x0000000001000000L});
public static final BitSet FOLLOW_44 = new BitSet(new long[]{0x0000002001000000L});
public static final BitSet FOLLOW_45 = new BitSet(new long[]{0x0000000800000040L});
public static final BitSet FOLLOW_46 = new BitSet(new long[]{0x0000200000000000L});
public static final BitSet FOLLOW_47 = new BitSet(new long[]{0x0000200000000000L,0x0000000000000000L,0x0000000000000000L,0x00000000000C0000L});
public static final BitSet FOLLOW_48 = new BitSet(new long[]{0x0000400800000000L});
public static final BitSet FOLLOW_49 = new BitSet(new long[]{0x0000400000000000L});
public static final BitSet FOLLOW_50 = new BitSet(new long[]{0x0000800800000000L});
public static final BitSet FOLLOW_51 = new BitSet(new long[]{0x0000082000000000L,0x0000000000000000L,0x0000000000000000L,0x0000000000030000L});
public static final BitSet FOLLOW_52 = new BitSet(new long[]{0x0000800000000000L});
public static final BitSet FOLLOW_53 = new BitSet(new long[]{0x0000202000000000L,0x0000000000000000L,0x0000000000000000L,0x00000000000C0000L});
public static final BitSet FOLLOW_54 = new BitSet(new long[]{0x0001000800000000L});
public static final BitSet FOLLOW_55 = new BitSet(new long[]{0x0001000000000000L});
public static final BitSet FOLLOW_56 = new BitSet(new long[]{0x0006000080000100L});
public static final BitSet FOLLOW_57 = new BitSet(new long[]{0x0000000000200040L});
public static final BitSet FOLLOW_58 = new BitSet(new long[]{0x0006000081000110L});
public static final BitSet FOLLOW_59 = new BitSet(new long[]{0x0000000000200000L,0x0000000000000000L,0x0000000000000000L,0x0000000F80000000L});
public static final BitSet FOLLOW_60 = new BitSet(new long[]{0x0008080000000000L});
public static final BitSet FOLLOW_61 = new BitSet(new long[]{0x0008000000000000L});
public static final BitSet FOLLOW_62 = new BitSet(new long[]{0x0010200000000000L});
public static final BitSet FOLLOW_63 = new BitSet(new long[]{0x0010000000000000L});
public static final BitSet FOLLOW_64 = new BitSet(new long[]{0x0020000800000000L});
public static final BitSet FOLLOW_65 = new BitSet(new long[]{0x0020000000000000L});
public static final BitSet FOLLOW_66 = new BitSet(new long[]{0x0040000800000000L});
public static final BitSet FOLLOW_67 = new BitSet(new long[]{0x0040000000000000L});
public static final BitSet FOLLOW_68 = new BitSet(new long[]{0x0080000000000020L});
public static final BitSet FOLLOW_69 = new BitSet(new long[]{0x0080000000000000L});
public static final BitSet FOLLOW_70 = new BitSet(new long[]{0x0F00000140000320L,0x0300000012200000L,0x0080000000033900L});
public static final BitSet FOLLOW_71 = new BitSet(new long[]{0x0F00000141000320L,0x0300000012200000L,0x0080000000033900L});
public static final BitSet FOLLOW_72 = new BitSet(new long[]{0x0000000000200000L,0x0000000000000000L,0x0000000000000000L,0x000001C000000000L});
public static final BitSet FOLLOW_73 = new BitSet(new long[]{0x0000000000000320L,0x0300000012200000L,0x0080000000033900L});
public static final BitSet FOLLOW_74 = new BitSet(new long[]{0xE000000000000100L,0x000000000000000DL});
public static final BitSet FOLLOW_75 = new BitSet(new long[]{0x0000000000200020L,0x0000000000007800L});
public static final BitSet FOLLOW_76 = new BitSet(new long[]{0xE000000001000100L,0x000000000000000DL});
public static final BitSet FOLLOW_77 = new BitSet(new long[]{0x0000000000200000L,0x0000000000000002L});
public static final BitSet FOLLOW_78 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000002L});
public static final BitSet FOLLOW_79 = new BitSet(new long[]{0xE000000001000120L,0x000000000000000DL});
public static final BitSet FOLLOW_80 = new BitSet(new long[]{0x0000000000000100L,0x000000000000006DL});
public static final BitSet FOLLOW_81 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000080L});
public static final BitSet FOLLOW_82 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000180L});
public static final BitSet FOLLOW_83 = new BitSet(new long[]{0x0000000001000120L,0x000000000000006DL});
public static final BitSet FOLLOW_84 = new BitSet(new long[]{0x0000000001000100L,0x000000000000006DL});
public static final BitSet FOLLOW_85 = new BitSet(new long[]{0x0000000000000020L,0x0000000000000600L});
public static final BitSet FOLLOW_86 = new BitSet(new long[]{0x0000000000000000L,0x0000000000008000L});
public static final BitSet FOLLOW_87 = new BitSet(new long[]{0x0080000000000320L,0x0300000012270000L,0x0080000000033900L});
public static final BitSet FOLLOW_88 = new BitSet(new long[]{0x0080000000000320L,0x0300000012260000L,0x0080000000033900L});
public static final BitSet FOLLOW_89 = new BitSet(new long[]{0x0000000000200000L});
public static final BitSet FOLLOW_90 = new BitSet(new long[]{0x0080000000000320L,0x0300000012240000L,0x0080000000033900L});
public static final BitSet FOLLOW_91 = new BitSet(new long[]{0x0080000000000320L,0x0300000012200000L,0x0080000000033900L});
public static final BitSet FOLLOW_92 = new BitSet(new long[]{0x0000000000000000L,0x0000000000180000L});
public static final BitSet FOLLOW_93 = new BitSet(new long[]{0x0000000000000000L,0x0000000000020000L});
public static final BitSet FOLLOW_94 = new BitSet(new long[]{0x0000000000000020L,0x0000000000200000L});
public static final BitSet FOLLOW_95 = new BitSet(new long[]{0x0000000000000000L,0x0000000000200000L});
public static final BitSet FOLLOW_96 = new BitSet(new long[]{0x0000000000000960L,0x1080002241C40000L,0xFE40000000000000L,0x03F8020000007FFFL});
public static final BitSet FOLLOW_97 = new BitSet(new long[]{0x0000000000000960L,0x1080002040000000L,0xFE40000000000000L,0x03F8020000007FFFL});
public static final BitSet FOLLOW_98 = new BitSet(new long[]{0x0000000000000960L,0x1000002040000000L,0xFE00000000000000L,0x03F8020000007FFFL});
public static final BitSet FOLLOW_99 = new BitSet(new long[]{0x0000000000000000L,0x0000000002000000L});
public static final BitSet FOLLOW_100 = new BitSet(new long[]{0x0000000000000960L,0x108000004CC40000L,0xFE40000000000000L,0x03F8020000007FFFL});
public static final BitSet FOLLOW_101 = new BitSet(new long[]{0x0000000000000960L,0x1080000040000000L,0xFE40000000000000L,0x03F8020000007FFFL});
public static final BitSet FOLLOW_102 = new BitSet(new long[]{0x0000000000000960L,0x1000000040000000L,0xFE00000000000000L,0x03F8020000007FFFL});
public static final BitSet FOLLOW_103 = new BitSet(new long[]{0x0000000000000000L,0x0000000010000000L});
public static final BitSet FOLLOW_104 = new BitSet(new long[]{0x0000000000000100L,0x00800003A1C40000L,0x0040000000000000L});
public static final BitSet FOLLOW_105 = new BitSet(new long[]{0x0000000000000040L,0x0000000040000000L});
public static final BitSet FOLLOW_106 = new BitSet(new long[]{0x0000000000000100L,0x0000000381C40000L});
public static final BitSet FOLLOW_107 = new BitSet(new long[]{0x0000000000000000L,0x0000000400000000L});
public static final BitSet FOLLOW_108 = new BitSet(new long[]{0x0000000000000002L,0x0000000800000000L});
public static final BitSet FOLLOW_109 = new BitSet(new long[]{0x0000000000000040L,0x0000000440000000L});
public static final BitSet FOLLOW_110 = new BitSet(new long[]{0x0000000000000840L,0x1000002040000000L,0xFE00000000000000L,0x03F8020000007FFFL});
public static final BitSet FOLLOW_111 = new BitSet(new long[]{0x0000000000000840L,0x0000002040000000L,0xFE00000000000000L,0x0078020000007FFFL});
public static final BitSet FOLLOW_112 = new BitSet(new long[]{0x0000000000000400L,0x0000035000040000L});
public static final BitSet FOLLOW_113 = new BitSet(new long[]{0x0000000001000860L,0x0000008040000000L,0xFE00000000000000L,0x0078020000007FFFL});
public static final BitSet FOLLOW_114 = new BitSet(new long[]{0x0000000001000000L,0x0000008000000000L});
public static final BitSet FOLLOW_115 = new BitSet(new long[]{0x0000000000000860L,0x0000000040000000L,0xFE00000000000000L,0x0078020000007FFFL});
public static final BitSet FOLLOW_116 = new BitSet(new long[]{0x0000000000000400L,0x0000031000040000L});
public static final BitSet FOLLOW_117 = new BitSet(new long[]{0x0000000000000000L,0x0000020000000000L});
public static final BitSet FOLLOW_118 = new BitSet(new long[]{0x0000000000000000L,0x0000040000000000L});
public static final BitSet FOLLOW_119 = new BitSet(new long[]{0x0000000000000840L,0x1000000040000000L,0xFE00000000000000L,0x03F8020000007FFFL});
public static final BitSet FOLLOW_120 = new BitSet(new long[]{0x0000000000000840L,0x0000000040000000L,0xFE00000000000000L,0x0078020000007FFFL});
public static final BitSet FOLLOW_121 = new BitSet(new long[]{0x0000000000000400L,0x00000B4008040000L,0x0000000000000000L,0x00003E0000000000L});
public static final BitSet FOLLOW_122 = new BitSet(new long[]{0x0000000000000400L,0x00000B0008040000L,0x0000000000000000L,0x00003E0000000000L});
public static final BitSet FOLLOW_123 = new BitSet(new long[]{0x0000000000000400L,0x001FF35000C40000L});
public static final BitSet FOLLOW_124 = new BitSet(new long[]{0x0000000000000400L,0x001FF31000C40000L});
public static final BitSet FOLLOW_125 = new BitSet(new long[]{0x0000000000000040L,0x0020000000000000L});
public static final BitSet FOLLOW_126 = new BitSet(new long[]{0x0000000000000000L,0x0040000000000000L});
public static final BitSet FOLLOW_127 = new BitSet(new long[]{0x0000000000000000L,0x0000000040000000L});
public static final BitSet FOLLOW_128 = new BitSet(new long[]{0x0000000000000000L,0x0080000000000000L});
public static final BitSet FOLLOW_129 = new BitSet(new long[]{0x0000000000000040L,0x0000000040000000L,0xFE00000000000000L,0x0000000000007FFFL});
public static final BitSet FOLLOW_130 = new BitSet(new long[]{0x0000000000000020L,0x0300000000000000L});
public static final BitSet FOLLOW_131 = new BitSet(new long[]{0x0000000000000000L,0x0300000000000000L});
public static final BitSet FOLLOW_132 = new BitSet(new long[]{0x0000000000000000L,0x0200000000000000L});
public static final BitSet FOLLOW_133 = new BitSet(new long[]{0x0000000000000082L,0x0C00000000000000L});
public static final BitSet FOLLOW_134 = new BitSet(new long[]{0x0000000000000082L,0x0800000000000000L});
public static final BitSet FOLLOW_135 = new BitSet(new long[]{0x0000000000003160L,0xF000100004C40000L,0xFE2000000000C0FFL,0x03F8020000007FFFL});
public static final BitSet FOLLOW_136 = new BitSet(new long[]{0x0000000000000000L,0x2000000000000000L});
public static final BitSet FOLLOW_137 = new BitSet(new long[]{0x0000000000000000L,0x4000000000000000L});
public static final BitSet FOLLOW_138 = new BitSet(new long[]{0x0000000000000000L,0x0000100000000000L});
public static final BitSet FOLLOW_139 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000000L,0x0007000000000000L});
public static final BitSet FOLLOW_140 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000000L,0x0000C00000000000L});
public static final BitSet FOLLOW_141 = new BitSet(new long[]{0x0000000000002160L,0x1000000000000000L,0xFE2000000000C000L,0x03F8020000007FFFL});
public static final BitSet FOLLOW_142 = new BitSet(new long[]{0x0000000000000020L,0x0100000000000000L,0x0000000000000100L});
public static final BitSet FOLLOW_143 = new BitSet(new long[]{0x0000000000000000L,0x0100000000000000L,0x0000000000000100L});
public static final BitSet FOLLOW_144 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000100L});
public static final BitSet FOLLOW_145 = new BitSet(new long[]{0x0000000000003160L,0xB000100004C40000L,0xFE2000000000C6FFL,0x03F8020000007FFFL});
public static final BitSet FOLLOW_146 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000200L});
public static final BitSet FOLLOW_147 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000400L});
public static final BitSet FOLLOW_148 = new BitSet(new long[]{0x0000000000000020L,0x0100000000000000L,0x0000000000000800L});
public static final BitSet FOLLOW_149 = new BitSet(new long[]{0x0000000000000000L,0x0100000000000000L,0x0000000000000800L});
public static final BitSet FOLLOW_150 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000000800L});
public static final BitSet FOLLOW_151 = new BitSet(new long[]{0x0000000000003160L,0x9000100004C40000L,0xFE2000000000C4FFL,0x03F8020000007FFFL});
public static final BitSet FOLLOW_152 = new BitSet(new long[]{0x0000000000000020L,0x0100000000000000L,0x0000000000001000L});
public static final BitSet FOLLOW_153 = new BitSet(new long[]{0x0000000000000000L,0x0100000000000000L,0x0000000000001000L});
public static final BitSet FOLLOW_154 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000001000L});
public static final BitSet FOLLOW_155 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000002000L});
public static final BitSet FOLLOW_156 = new BitSet(new long[]{0x0000000000002160L,0x1000000000040000L,0xFE0000000000C000L,0x03F8020000007FFFL});
public static final BitSet FOLLOW_157 = new BitSet(new long[]{0x0000000000002160L,0x1000000000000000L,0xFE0000000000C000L,0x03F8020000007FFFL});
public static final BitSet FOLLOW_158 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x000000000000C000L});
public static final BitSet FOLLOW_159 = new BitSet(new long[]{0x0000000000000840L,0x1100002040000000L,0xFE00000000000000L,0x03F8020000007FFFL});
public static final BitSet FOLLOW_160 = new BitSet(new long[]{0x0000000000000000L,0x0000034000040000L});
public static final BitSet FOLLOW_161 = new BitSet(new long[]{0x0000000000000000L,0x0000030000040000L});
public static final BitSet FOLLOW_162 = new BitSet(new long[]{0x0000000000000020L,0x0100000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_163 = new BitSet(new long[]{0x0000000000000000L,0x0100000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_164 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_165 = new BitSet(new long[]{0x0000000000000082L,0x0400000000000000L});
public static final BitSet FOLLOW_166 = new BitSet(new long[]{0x0000000000002160L,0x1000000000C40000L,0xFE00000000000020L,0x03F8020000007FFFL});
public static final BitSet FOLLOW_167 = new BitSet(new long[]{0x0000000000002160L,0x1000000000000000L,0xFE00000000000000L,0x03F8020000007FFFL});
public static final BitSet FOLLOW_168 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000020000L});
public static final BitSet FOLLOW_169 = new BitSet(new long[]{0x0000000000003160L,0x1000000000C40000L,0xFE0000000000C200L,0x03F8020000007FFFL});
public static final BitSet FOLLOW_170 = new BitSet(new long[]{0x0000000000000040L,0x1000000000000000L,0xFE00000000000000L,0x03F8020000007FFFL});
public static final BitSet FOLLOW_171 = new BitSet(new long[]{0x0000000000000040L,0x0000000000000000L,0xFE00000000000000L,0x0078020000007FFFL});
public static final BitSet FOLLOW_172 = new BitSet(new long[]{0x0000000000000040L,0x0000000000000000L,0xFE00000000000000L,0x0000000000007FFFL});
public static final BitSet FOLLOW_173 = new BitSet(new long[]{0x0000000000001002L,0x0000020000040000L,0x00001FFFFFFC0020L});
public static final BitSet FOLLOW_174 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000080000L});
public static final BitSet FOLLOW_175 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000100000L});
public static final BitSet FOLLOW_176 = new BitSet(new long[]{0x0000000000201002L,0x0000020000040000L,0x00001FFFFFFC0020L});
public static final BitSet FOLLOW_177 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000000000200000L});
public static final BitSet FOLLOW_178 = new BitSet(new long[]{0x0000000000002000L});
public static final BitSet FOLLOW_179 = new BitSet(new long[]{0x0000000000000040L,0x1000000040000000L,0x0000000000000000L,0x03F8020000000000L});
public static final BitSet FOLLOW_180 = new BitSet(new long[]{0x0000000000000040L,0x0000000040000000L,0x0000000000000000L,0x0078020000000000L});
public static final BitSet FOLLOW_181 = new BitSet(new long[]{0x0000000000005002L,0x0000120000040000L,0x001FEC44003C0020L});
public static final BitSet FOLLOW_182 = new BitSet(new long[]{0x0000000000205002L,0x0000120000040000L,0x001FEC44003C0020L});
public static final BitSet FOLLOW_183 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0000800000000000L});
public static final BitSet FOLLOW_184 = new BitSet(new long[]{0x0000000000000040L,0x0080000000000000L});
public static final BitSet FOLLOW_185 = new BitSet(new long[]{0x0000000000001002L,0x0000020000040000L,0x000007FFFFBC0020L});
public static final BitSet FOLLOW_186 = new BitSet(new long[]{0x0000000000201002L,0x0000020000040000L,0x000007FFFFBC0020L});
public static final BitSet FOLLOW_187 = new BitSet(new long[]{0x0000000000001002L,0x0000020000040000L,0x00040444003C0020L});
public static final BitSet FOLLOW_188 = new BitSet(new long[]{0x0000000000201002L,0x0000020000040000L,0x00040444003C0020L});
public static final BitSet FOLLOW_189 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0020000000000000L});
public static final BitSet FOLLOW_190 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000000L,0x0080000000000000L});
public static final BitSet FOLLOW_191 = new BitSet(new long[]{0x0000000000000060L,0x1000000000040000L,0xFF00000000000000L,0x0000000000007FFFL});
public static final BitSet FOLLOW_192 = new BitSet(new long[]{0x0000000001000100L,0x0000020000000000L});
public static final BitSet FOLLOW_193 = new BitSet(new long[]{0x0000000000000002L,0x0000020000000000L,0x0000000000040000L});
public static final BitSet FOLLOW_194 = new BitSet(new long[]{0x0000000000000002L,0x0000020000000000L});
public static final BitSet FOLLOW_195 = new BitSet(new long[]{0x0000000000000002L,0x0000004000000000L});
public static final BitSet FOLLOW_196 = new BitSet(new long[]{0x0000000000008020L});
public static final BitSet FOLLOW_197 = new BitSet(new long[]{0x0000000000000002L,0x0000040000000000L});
public static final BitSet FOLLOW_198 = new BitSet(new long[]{0x0000010000000002L,0x0000040000000000L,0x0000000000000000L,0x0000000000008000L});
public static final BitSet FOLLOW_199 = new BitSet(new long[]{0x0000000001000002L});
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy