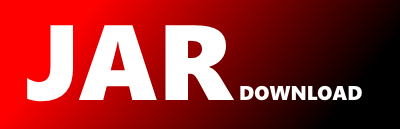
org.contextmapper.dsl.scoping.ContextMappingDSLScopeProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of context-mapper-dsl Show documentation
Show all versions of context-mapper-dsl Show documentation
Use the ContextMapper DSL in your standalone application.
/**
* Copyright 2018 The Context Mapper Project Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.contextmapper.dsl.scoping;
import java.util.ArrayList;
import java.util.function.Consumer;
import org.contextmapper.tactic.dsl.scoping.Scope;
import org.contextmapper.tactic.dsl.tacticdsl.DomainObject;
import org.contextmapper.tactic.dsl.tacticdsl.OppositeHolder;
import org.contextmapper.tactic.dsl.tacticdsl.Reference;
import org.contextmapper.tactic.dsl.tacticdsl.Repository;
import org.contextmapper.tactic.dsl.tacticdsl.RepositoryOperation;
import org.contextmapper.tactic.dsl.tacticdsl.ResourceOperationDelegate;
import org.contextmapper.tactic.dsl.tacticdsl.Service;
import org.contextmapper.tactic.dsl.tacticdsl.ServiceOperation;
import org.contextmapper.tactic.dsl.tacticdsl.ServiceOperationDelegate;
import org.contextmapper.tactic.dsl.tacticdsl.ServiceRepositoryOption;
import org.contextmapper.tactic.dsl.tacticdsl.SimpleDomainObject;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.emf.ecore.EReference;
import org.eclipse.xtext.naming.QualifiedName;
import org.eclipse.xtext.resource.EObjectDescription;
import org.eclipse.xtext.resource.IEObjectDescription;
import org.eclipse.xtext.scoping.IScope;
import org.eclipse.xtext.scoping.impl.AbstractDeclarativeScopeProvider;
/**
* This class contains custom scoping description.
*
* See https://www.eclipse.org/Xtext/documentation/303_runtime_concepts.html#scoping
* on how and when to use it.
*/
@SuppressWarnings("all")
public class ContextMappingDSLScopeProvider extends AbstractDeclarativeScopeProvider {
public IScope scope_DslOppositeHolder_opposite(final OppositeHolder ctx, final EReference ref) {
final Scope scope = new Scope();
final ArrayList elements = new ArrayList();
EObject _eContainer = ctx.eContainer();
SimpleDomainObject _domainObjectType = ((Reference) _eContainer).getDomainObjectType();
final DomainObject domainObject = ((DomainObject) _domainObjectType);
final Consumer _function = (Reference it) -> {
EObject _eContainer_1 = it.eContainer();
boolean _tripleNotEquals = (_eContainer_1 != null);
if (_tripleNotEquals) {
QualifiedName _create = QualifiedName.create(it.getName());
EObjectDescription _eObjectDescription = new EObjectDescription(_create, it, null);
elements.add(_eObjectDescription);
}
};
domainObject.getReferences().forEach(_function);
scope.setElements(elements);
return scope;
}
public IScope scope_DslServiceOperationDelegate_delegateOperation(final ServiceOperationDelegate ctx, final EReference ref) {
final Scope scope = new Scope();
final ArrayList elements = new ArrayList();
final ServiceRepositoryOption option = ctx.getDelegate();
if ((option != null)) {
if ((option instanceof Repository)) {
final Consumer _function = (RepositoryOperation it) -> {
QualifiedName _create = QualifiedName.create(it.getName());
EObjectDescription _eObjectDescription = new EObjectDescription(_create, it, null);
elements.add(_eObjectDescription);
};
((Repository)option).getOperations().forEach(_function);
} else {
final Consumer _function_1 = (ServiceOperation it) -> {
QualifiedName _create = QualifiedName.create(it.getName());
EObjectDescription _eObjectDescription = new EObjectDescription(_create, it, null);
elements.add(_eObjectDescription);
};
((Service) option).getOperations().forEach(_function_1);
}
}
scope.setElements(elements);
return scope;
}
public IScope scope_DslResourceOperationDelegate_delegateOperation(final ResourceOperationDelegate ctx, final EReference ref) {
final Scope scope = new Scope();
final ArrayList elements = new ArrayList();
final Service option = ctx.getDelegate();
if ((option != null)) {
final Consumer _function = (ServiceOperation it) -> {
QualifiedName _create = QualifiedName.create(it.getName());
EObjectDescription _eObjectDescription = new EObjectDescription(_create, it, null);
elements.add(_eObjectDescription);
};
option.getOperations().forEach(_function);
}
scope.setElements(elements);
return scope;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy