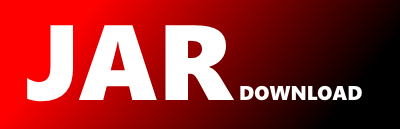
org.contextmapper.dsl.ide.actions.CMLActionService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of context-mapper-lsp Show documentation
Show all versions of context-mapper-lsp Show documentation
Use the ContextMapper language server (LSP) to integrate the ContextMapper DSL within your IDE.
The newest version!
/**
* Copyright 2020 The Context Mapper Project Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.contextmapper.dsl.ide.actions;
import com.google.common.collect.Lists;
import com.google.inject.Inject;
import java.util.List;
import java.util.function.Function;
import org.contextmapper.dsl.cml.CMLResource;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.emf.ecore.resource.Resource;
import org.eclipse.lsp4j.CodeAction;
import org.eclipse.lsp4j.CodeActionParams;
import org.eclipse.lsp4j.Command;
import org.eclipse.lsp4j.Diagnostic;
import org.eclipse.lsp4j.Position;
import org.eclipse.lsp4j.Range;
import org.eclipse.lsp4j.jsonrpc.messages.Either;
import org.eclipse.xtext.ide.server.ILanguageServerAccess;
import org.eclipse.xtext.ide.server.codeActions.ICodeActionService2;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.ListExtensions;
@SuppressWarnings("all")
public class CMLActionService implements ICodeActionService2 {
@Inject
private SelectionContextResolver selectionResolver;
@Inject
private CMLActionRegistry actionRegistry;
@Override
public List> getCodeActions(final ICodeActionService2.Options options) {
final Function>> _function = (ILanguageServerAccess.Context context) -> {
options.setDocument(context.getDocument());
options.setResource(context.getResource());
return this.getActions(options);
};
return options.getLanguageServerAccess().>>doSyncRead(options.getURI(), _function);
}
private List> getActions(final ICodeActionService2.Options options) {
final CodeActionParams params = options.getCodeActionParams();
final Range currentSelectionRange = params.getRange();
final Position startPosition = currentSelectionRange.getStart();
final Position endPosition = currentSelectionRange.getEnd();
Resource _resource = options.getResource();
final CMLResource resource = new CMLResource(_resource);
final List selectedObjects = this.selectionResolver.resolveAllSelectedEObjects(resource,
options.getDocument().getOffSet(startPosition), options.getDocument().getOffSet(endPosition));
final List> allActions = Lists.>newLinkedList();
final Function1> _function = (Command it) -> {
return Either.forLeft(it);
};
allActions.addAll(ListExtensions.map(this.actionRegistry.getApplicableActionCommands(resource, selectedObjects), _function));
boolean _isEmpty = params.getContext().getDiagnostics().isEmpty();
boolean _not = (!_isEmpty);
if (_not) {
List _diagnostics = params.getContext().getDiagnostics();
for (final Diagnostic d : _diagnostics) {
allActions.addAll(this.actionRegistry.getApplicableQuickfixes(d, options));
}
}
return allActions;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy