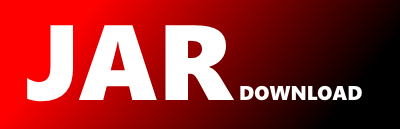
impl.org.controlsfx.skin.AutoCompletePopup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of controlsfx Show documentation
Show all versions of controlsfx Show documentation
High quality UI controls and other tools to complement the core JavaFX distribution
package impl.org.controlsfx.skin;
import javafx.beans.property.ObjectProperty;
import javafx.beans.property.ObjectPropertyBase;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.event.Event;
import javafx.event.EventDispatchChain;
import javafx.event.EventHandler;
import javafx.event.EventType;
import javafx.scene.Node;
import javafx.scene.control.PopupControl;
import javafx.scene.control.Skin;
import javafx.stage.Window;
import javafx.util.StringConverter;
import com.sun.javafx.event.EventHandlerManager;
/**
* The auto-complete-popup provides an list of available suggestions in order
* to complete current user input.
*/
public class AutoCompletePopup extends PopupControl{
/***************************************************************************
* *
* Private fields *
* *
**************************************************************************/
private final static int TITLE_HEIGHT = 28; // HACK: Hard-coded title-bar height
private final ObservableList suggestions = FXCollections.observableArrayList();
private StringConverter converter;
/***************************************************************************
* *
* Inner classes *
* *
**************************************************************************/
/**
* Represents an Event which is fired when the user has selected a suggestion
* for auto-complete
*
* @param
*/
@SuppressWarnings("serial")
public static class SuggestionEvent extends Event {
@SuppressWarnings("rawtypes")
public static final EventType SUGGESTION
= new EventType("SUGGESTION");
private final TE suggestion;
public SuggestionEvent(TE suggestion) {
super(SUGGESTION);
this.suggestion = suggestion;
}
/**
* Returns the suggestion which was chosen by the user
* @return
*/
public TE getSuggestion() {
return suggestion;
}
}
/***************************************************************************
* *
* Constructors *
* *
**************************************************************************/
/**
* Creates a new AutoCompletePopup
*/
public AutoCompletePopup(){
this.setAutoFix(true);
this.setAutoHide(true);
this.setHideOnEscape(true);
getStyleClass().add(DEFAULT_STYLE_CLASS);
}
/***************************************************************************
* *
* Public API *
* *
**************************************************************************/
/**
* Get the suggestions presented by this AutoCompletePopup
* @return
*/
public ObservableList getSuggestions() {
return suggestions;
}
/**
* Show this popup right below the given Node
* @param node
*/
public void show(Node node){
if(node.getScene() == null || node.getScene().getWindow() == null)
throw new IllegalStateException("Can not show popup. The node must be attached to a scene/window.");
Window parent = node.getScene().getWindow();
this.show(
parent,
parent.getX() + node.localToScene(0, 0).getX() +
node.getScene().getX(),
parent.getY() + node.localToScene(0, 0).getY() +
node.getScene().getY() + TITLE_HEIGHT);
}
/**
*/
public void setConverter(StringConverter converter) {
this.converter = converter;
}
/**
*/
public StringConverter getConverter() {
return converter;
}
/***************************************************************************
* *
* Properties *
* *
**************************************************************************/
private final EventHandlerManager eventHandlerManager = new EventHandlerManager(this);
public final ObjectProperty>> onSuggestionProperty() { return onSuggestion; }
public final void setOnSuggestion(EventHandler> value) { onSuggestionProperty().set(value); }
public final EventHandler> getOnSuggestion() { return onSuggestionProperty().get(); }
private ObjectProperty>> onSuggestion = new ObjectPropertyBase>>() {
@SuppressWarnings("rawtypes")
@Override protected void invalidated() {
eventHandlerManager.setEventHandler(SuggestionEvent.SUGGESTION, (EventHandler)(Object)get());
}
@Override
public Object getBean() {
return AutoCompletePopup.this;
}
@Override
public String getName() {
return "onSuggestion";
}
};
/**{@inheritDoc}*/
@Override public EventDispatchChain buildEventDispatchChain(EventDispatchChain tail) {
return super.buildEventDispatchChain(tail).append(eventHandlerManager);
}
/***************************************************************************
* *
* Stylesheet Handling *
* *
**************************************************************************/
public static final String DEFAULT_STYLE_CLASS = "auto-complete-popup";
@Override
protected Skin> createDefaultSkin() {
return new AutoCompletePopupSkin(this);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy