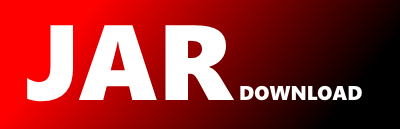
org.coodex.concrete.jaxrs.ConcreteJaxrsApplication Maven / Gradle / Ivy
/*
* Copyright (c) 2018 coodex.org ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.coodex.concrete.jaxrs;
import org.coodex.concrete.common.ConcreteHelper;
import org.coodex.concrete.common.ErrorCodes;
import org.coodex.concrete.common.ErrorMessageFacade;
import org.coodex.concrete.jaxrs.logging.ServerLogger;
import org.coodex.util.LazyServiceLoader;
import org.coodex.util.ServiceLoader;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.ws.rs.core.Application;
import javax.ws.rs.ext.Provider;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.util.Collections;
import java.util.HashSet;
import java.util.Set;
import static org.coodex.concrete.common.ConcreteHelper.foreachClassInPackages;
@SuppressWarnings("unused")
public abstract class ConcreteJaxrsApplication
extends Application
implements
org.coodex.concrete.api.Application {
private static final JaxRSModuleMaker moduleMaker = new JaxRSModuleMaker();
private final static Logger log = LoggerFactory.getLogger(ConcreteJaxrsApplication.class);
private final Set> servicesClasses = new HashSet<>();
private final Set> jaxrsClasses = new HashSet<>();
private final Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy