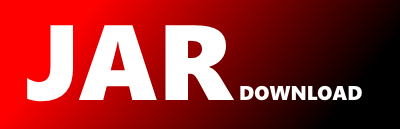
org.coodex.commons.jpa.springdata.SpecCommon Maven / Gradle / Ivy
/*
* Copyright (c) 2017 coodex.org ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.coodex.commons.jpa.springdata;
import org.coodex.commons.jpa.criteria.Operators;
import org.springframework.data.jpa.domain.Specification;
import org.springframework.data.jpa.domain.Specifications;
import javax.persistence.criteria.*;
import java.util.Arrays;
import java.util.Collection;
/**
* 少量调整,移除SpecificationGroup, 增加不定参方法
*
* @author [email protected]
*/
@SuppressWarnings("unchecked")
public class SpecCommon {
public static Path getPath(Root root, String attrName) {
From f = null;
String[] nodes = attrName.split("\\.");
String attr = null;
if (nodes.length == 1) {
f = root;
attr = attrName;
} else {
for (int i = 0; i < nodes.length; i++) {
attr = nodes[i];
if (i < nodes.length - 1) {
f = root.join(attr);
}
}
}
return f.get(attr);
}
public static Specification not(final Specification spec) {
return new Specification() {
@Override
public Predicate toPredicate(Root root, CriteriaQuery> query, CriteriaBuilder cb) {
return cb.not(spec.toPredicate(root, query, cb));
}
};
}
public static Specifications and(Specification... specifications) {
return and(Arrays.asList(specifications));
}
public static Specifications or(Specification... specifications) {
return or(Arrays.asList(specifications));
}
public static Specifications and(Collection> specList) {
Specifications specs = null;
for (Specification s : specList) {
if (specs != null) {
specs = specs.and(s);
} else {
specs = Specifications.where(s);
}
}
return specs;
}
public static Specifications or(Collection> specList) {
Specifications specs = null;
for (Specification s : specList) {
if (specs != null) {
specs = specs.or(s);
} else {
specs = Specifications.where(s);
}
}
return specs;
}
@Deprecated
public static Specification spec(
Class entityClass,
Operators.Logical logical,
String attributeName,
ATTR... attributes) {
return spec(logical, attributeName, attributes);
}
@Deprecated
public static Specification spec(
Operators.Logical logical,
String attributeName,
ATTR... attributes) {
return $spec(logical, attributeName, attributes);
}
protected static Specification $spec(Operators.Logical logical,
String attributeName,
A... attributes) {
return new Spec(logical, attributeName, attributes);
}
@Deprecated
public static Specification memberOf(
Class entityClass, String attributeName, ATTR attr) {
return memberOf(attributeName, attr);
}
/**
* where attr in E.attribute
* @param attributeName
* @param attr
* @param
* @param
* @return
*/
public static Specification memberOf(String attributeName, ATTR attr) {
return new MemberOfSpec(attributeName, attr);
}
/**
* where E.attribute < value
*
* @param attributeName
* @param value
* @param
* @param
* @return
*/
public static Specification lessThen(String attributeName, A value) {
return $spec(Operators.Logical.LESS, attributeName, value);
}
/**
* where E.attribute <= value
*
* @param attributeName
* @param value
* @param
* @param
* @return
*/
public static Specification lessThenEquals(String attributeName, A value) {
return $spec(Operators.Logical.LESS_EQUAL, attributeName, value);
}
/**
* where E.attribute = value
*
* @param attributeName
* @param value
* @param
* @param
* @return
*/
public static Specification equals(String attributeName, A value) {
return $spec(Operators.Logical.EQUAL, attributeName, value);
}
/**
* where E.attribute <> value
*
* @param attributeName
* @param value
* @param
* @param
* @return
*/
public static Specification notEquals(String attributeName, A value) {
return $spec(Operators.Logical.NOT_EQUAL, attributeName, value);
}
/**
* where E.attribute > value
*
* @param attributeName
* @param value
* @param
* @param
* @return
*/
public static Specification greaterThen(String attributeName, A value) {
return $spec(Operators.Logical.GREATER, attributeName, value);
}
/**
* where E.attribute >= value
*
* @param attributeName
* @param value
* @param
* @param
* @return
*/
public static Specification greaterThenEquals(String attributeName, A value) {
return $spec(Operators.Logical.GREATER_EQUAL, attributeName, value);
}
/**
* where E.attribute like %value%
*
* @param attributeName
* @param value
* @param
* @return
*/
public static Specification like(String attributeName, String value) {
return $spec(Operators.Logical.LIKE, attributeName, value);
}
/**
* where E.attribute like value%
*
* @param attributeName
* @param value
* @param
* @return
*/
public static Specification startWith(String attributeName, String value) {
return $spec(Operators.Logical.START_WITH, attributeName, value);
}
/**
* where E.attribute like %value
*
* @param attributeName
* @param value
* @param
* @return
*/
public static Specification endWith(String attributeName, String value) {
return $spec(Operators.Logical.END_WITH, attributeName, value);
}
/**
* where E.attribute like value
*
* @param attributeName
* @param value
* @param
* @return
*/
public static Specification customLike(String attributeName, String value) {
return $spec(Operators.Logical.CUSTOM_LIKE, attributeName, value);
}
/**
* where E.attribute is NULL
*
* @param attributeName
* @param value
* @param
* @param
* @return
*/
public static Specification isNull(String attributeName, A value) {
return $spec(Operators.Logical.IS_NULL, attributeName, value);
}
/**
* where E.attribute is not NULL
*
* @param attributeName
* @param value
* @param
* @param
* @return
*/
public static Specification notNull(String attributeName, A value) {
return $spec(Operators.Logical.NOT_NULL, attributeName, value);
}
/**
* where E.attribute in (values)
*
* @param attributeName
* @param values
* @param
* @param
* @return
*/
public static Specification in(String attributeName, A ... values) {
return $spec(Operators.Logical.IN, attributeName, values);
}
/**
* where E.attribute not in (values)
*
* @param attributeName
* @param values
* @param
* @param
* @return
*/
public static Specification notIn(String attributeName, A ... values) {
return $spec(Operators.Logical.NOT_IN, attributeName, values);
}
/**
* where E.attribute between min and max
* @param attributeName
* @param min
* @param max
* @param
* @param
* @return
*/
public static Specification between(String attributeName, A min, A max) {
return $spec(Operators.Logical.BETWEEN, attributeName, min, max);
}
public static Specifications wrapper(Specifications specifications) {
return specifications == null ? Specifications.where(specifications) : specifications;
}
static class MemberOfSpec implements Specification {
private final ATTR attr;
private final String attributeName;
MemberOfSpec(String attributeName, ATTR attr) {
this.attr = attr;
this.attributeName = attributeName;
}
@Override
public Predicate toPredicate(Root root, CriteriaQuery> query, CriteriaBuilder cb) {
Path> path = SpecCommon.getPath(root, attributeName);
return cb.isMember(attr, path);
}
}
/**
* 根据 [email protected] 思路重设计编码
*
* Created by davidoff shen on 2017-03-17.
*/
static class Spec implements Specification {
private final Operators.Logical logical;
private final ATTR[] attributes;
private final String attributeName;
Spec(Operators.Logical logical, String attributeName, ATTR... attributes) {
this.logical = logical;
this.attributeName = attributeName;
this.attributes = attributes;
}
@Override
public Predicate toPredicate(Root root, CriteriaQuery> query, CriteriaBuilder cb) {
Path path = getPath(root, attributeName);
return logical.getOperator().toPredicate(path, cb, attributes);
}
protected ATTR[] getAttributes() {
return attributes;
}
protected String getAttributeName() {
return attributeName;
}
}
}