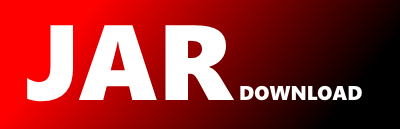
org.coos.messaging.android.PluginFactory Maven / Gradle / Ivy
The newest version!
package org.coos.messaging.android;
/**
* COOS - Connected Objects Operating System (www.connectedobjects.org).
*
* Copyright (C) 2009 Telenor ASA and Tellu AS. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
*
* This library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*
* You may also contact one of the following for additional information:
* Telenor ASA, Snaroyveien 30, N-1331 Fornebu, Norway (www.telenor.no)
* Tellu AS, Hagalokkveien 13, N-1383 Asker, Norway (www.tellu.no)
*/
import org.coos.messaging.*;
import org.coos.messaging.impl.DefaultChannel;
import org.coos.messaging.serializer.ObjectJavaSerializer;
import org.coos.messaging.util.URIHelper;
import org.coos.messaging.util.UuidHelper;
import org.coos.util.macro.MacroSubstituteReader;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.HashMap;
import java.util.Hashtable;
import java.util.Map;
/**
* Factory for Plugin instances
*
* @author Knut Eilif Husa, Tellu AS
*/
public class PluginFactory extends COFactory {
public static final String JVM_TRANSPORT_CLASS = "org.coos.messaging.transport.JvmTransport";
public static final String TCP_TRANSPORT_CLASS = "org.coos.messaging.transport.TCPTransport";
public static final String PLUGIN_CHANNEL_CLASS = "org.coos.messaging.plugin.PluginChannel";
private static Map sharedProcessors = new HashMap();
private PluginFactory() {
SerializerFactory.registerSerializer(Message.SERIALIZATION_METHOD_JAVA, new ObjectJavaSerializer());
}
/**
* This factory method creates a defined plugin with a tcp transport
* @param name name of endpoint
* @param className the endpoint implementation class
* @param properties a set of properties provided for the endpoint
* @param segment the segment, default is "."
* @param hostName Ip host name
* @param port Ip port
* @param cl the coos container. If not specified a default container is user
* @return the Plugin
* @throws Exception
*/
public static Plugin createPluginWithTCPTransport(String name, String className, Hashtable properties, String segment, String hostName, String port, COContainer cl) throws Exception {
if (cl != null) {
cl = new COContainerImpl();
}
Plugin plugin = createPluginEndpoint(name, className, properties, segment, cl);
Channel channel = createPluginChannel(segment, cl);
plugin.addChannel(channel);
properties = new Hashtable();
if (hostName != null)
properties.put("host", hostName);
if (port != null)
properties.put("port", port);
Transport transport = createTransport(TCP_TRANSPORT_CLASS, properties, cl);
channel.setTransport(transport);
return plugin;
}
/**
* This factory method creates a defined plugin with a jvm transport
* @param name name name of endpoint
* @param className the endpoint implementation class
* @param segment the segment, default is "."
* @param coosInstanceName the coos instance name
* @param channelServerName the coos channel server name
* @return the Plugin
* @throws Exception
*/
public static Plugin createPlugin(String name, String className, String segment, String coosInstanceName, String channelServerName)
throws Exception {
COContainer cl = new COContainerImpl();
Plugin plugin = createPluginEndpoint(name, className, null, segment, cl);
Channel channel = createPluginChannel(segment, cl);
plugin.addChannel(channel);
Hashtable properties = new Hashtable();
if (coosInstanceName != null)
properties.put("COOSInstanceName", coosInstanceName);
if (channelServerName != null)
properties.put("ChannelServerName", channelServerName);
Transport transport = createTransport(JVM_TRANSPORT_CLASS, properties, cl);
channel.setTransport(transport);
return plugin;
}
private static Plugin createPluginEndpoint(String name, String className, Hashtable properties, String segment, COContainer cl) throws Exception {
Plugin plugin = new Plugin();
Class> pluginClass = PluginFactory.tryClass(cl, className);
Endpoint endpoint = (Endpoint) pluginClass.newInstance();
endpoint.setCoContainer(cl);
endpoint.setEndpointUri("coos://" + name);
if(properties == null){
properties = new Hashtable();
}
endpoint.setProperties(properties);
plugin.setEndpoint(endpoint);
URIHelper helper = new URIHelper(endpoint.getEndpointUri());
if (helper.isEndpointUuid()) {
endpoint.setEndpointUuid(name);
}
endpoint.setName(name);
if (segment == null) {
segment = ".";
}
return plugin;
}
private static Channel createPluginChannel(String segment, COContainer cl) throws Exception {
Class> channelClass = PluginFactory.tryClass(cl, PLUGIN_CHANNEL_CLASS);
DefaultChannel channel = (DefaultChannel) channelClass.newInstance();
channel.addProtocol("coos");
channel.setCoContainer(cl);
if (segment == null) {
segment = ".";
}
channel.setSegment(segment);
return channel;
}
private static Transport createTransport(String className, Hashtable properties, COContainer cl) throws Exception {
Class> transportClass = PluginFactory.tryClass(cl, className);
Transport transport = (Transport) transportClass.newInstance();
transport.setProperties(properties);
return transport;
}
private static InputStream substitute(InputStream is) throws IOException {
InputStreamReader isr = new InputStreamReader(is);
MacroSubstituteReader msr = new MacroSubstituteReader(isr);
String substituted = msr.substituteMacros();
is = new ByteArrayInputStream(substituted.getBytes());
return is;
}
static class COContainerImpl implements COContainer {
public Class> loadClass(String className) throws ClassNotFoundException {
return Class.forName(className);
}
public InputStream getResource(String resourceName) throws IOException {
InputStream is = COContainer.class.getResourceAsStream(resourceName);
return substitute(is);
}
public Object getObject(String name) {
return null;
}
public void start() {
}
public void stop() {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy