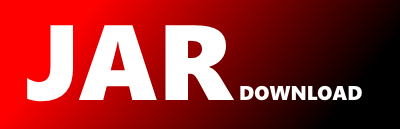
org.craftercms.commons.mongo.UpdateHelper Maven / Gradle / Ivy
/*
* Copyright (C) 2007-2020 Crafter Software Corporation. All Rights Reserved.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License version 3 as published by
* the Free Software Foundation.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.craftercms.commons.mongo;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.commons.collections.MapUtils;
import org.apache.commons.lang3.StringUtils;
/**
* Created by alfonsovasquez on 13/6/16.
*/
public class UpdateHelper {
protected Map setValues;
protected Map unsetValues;
protected Map pushValues;
protected Map pullValues;
public void set(String field, Object value) {
setValues = add(setValues, field, value);
}
public void unset(String field) {
unsetValues = add(unsetValues, field, "");
}
public void push(String field, Object value) {
pushValues = add(pushValues, field, value);
}
public void pushAll(String field, Collection> values) {
pushValues = add(pushValues, field, Collections.singletonMap("$each", values));
}
public void pull(String field, Object value) {
pullValues = add(pullValues, field, value);
}
public void pullAll(String field, Collection> values) {
pullValues = add(pullValues, field, Collections.singletonMap("$in", values));
}
public void pullAllDocuments(String field, String embeddedField, Collection> values) {
pullValues = add(pullValues, field, Collections.singletonMap(embeddedField, Collections.singletonMap("$in",
values)));
}
public void executeUpdate(String id, CrudRepository> repository) throws MongoDataException {
List modifiers = new ArrayList<>();
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy