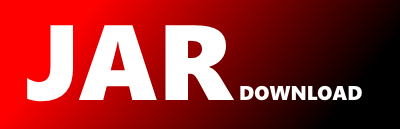
crash.commands.base.jmx Maven / Gradle / Ivy
package crash.commands.base;
import org.crsh.cli.Argument;
import org.crsh.cli.Command;
import org.crsh.cli.Option;
import org.crsh.cli.Usage;
import org.crsh.command.BaseCommand;
import org.crsh.command.InvocationContext;
import org.crsh.command.PipeCommand;
import org.crsh.command.ScriptException;
import javax.management.JMException;
import javax.management.MBeanAttributeInfo;
import javax.management.MBeanInfo;
import javax.management.MBeanServer;
import javax.management.ObjectInstance;
import javax.management.ObjectName;
import java.io.IOException;
import java.lang.management.ManagementFactory;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
/** @author Julien Viet */
@Usage("Java Management Extensions")
public class jmx extends BaseCommand {
@Usage("find mbeans")
@Command
public void find(
InvocationContext context,
@Usage("The object name pattern")
@Option(names = {"p", "pattern"})
String pattern) throws Exception {
//
ObjectName patternName = pattern != null ? ObjectName.getInstance(pattern) : null;
MBeanServer server = ManagementFactory.getPlatformMBeanServer();
Set instances = server.queryMBeans(patternName, null);
for (ObjectInstance instance : instances) {
context.provide(instance.getObjectName());
}
/*
if (context.piped) {
} else {
UIBuilder ui = new UIBuilder()
ui.table(columns: [1,3]) {
row(bold: true, fg: black, bg: white) {
label("CLASS NAME"); label("OBJECT NAME")
}
instances.each { instance ->
row() {
label(foreground: red, instance.getClassName()); label(instance.objectName)
}
}
}
out << ui;
}
*/
}
@Command
@Usage("return the attributes info of an MBean")
public void attributes(InvocationContext
© 2015 - 2025 Weber Informatics LLC | Privacy Policy