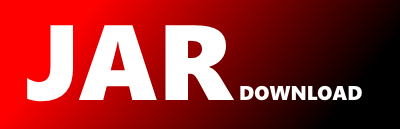
org.crazyyak.dev.common.IoUtils Maven / Gradle / Ivy
/*
* Copyright 2012 Jacob D Parr
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.crazyyak.dev.common;
import java.io.*;
import java.net.*;
import java.nio.file.*;
import java.nio.file.attribute.BasicFileAttributes;
import java.util.*;
public class IoUtils {
public static String toString(URL url) throws IOException {
try {
return toString(url.toURI());
} catch (URISyntaxException e) {
throw new IOException(e);
}
}
public static String toString(URI uri) throws IOException {
File file = new File(uri);
return toString(file);
}
public static String toString(Exception e) {
StringWriter writer = new StringWriter();
e.printStackTrace(new PrintWriter(writer));
return writer.toString();
}
public static String toString(File file) throws IOException {
return toString(new FileReader(file));
}
public static String toString(InputStream is) throws IOException {
return toString(new BufferedReader(new InputStreamReader(is)));
}
public static String toString(Reader reader) throws IOException {
try {
String line = "";
StringBuilder builder = new StringBuilder();
BufferedReader bufferedReader = (reader instanceof BufferedReader) ? (BufferedReader)reader : new BufferedReader(reader);
while ( (line=bufferedReader.readLine()) != null) {
builder.append(line);
builder.append("\n");
}
return builder.toString();
} finally {
reader.close();
}
}
public static void toFile(InputStream inputStream, File file) throws IOException {
if (file.exists() && file.delete() == false) {
throw new IOException("Unable to delete file: " + file.getAbsolutePath());
}
FileOutputStream out = new FileOutputStream(file);
toStream(inputStream, out);
}
public static byte[] toBytes(InputStream in) throws IOException {
return toBytes(in, 1024);
}
public static byte[] toBytes(InputStream in, int initCapacity) throws IOException {
ByteArrayOutputStream out = new ByteArrayOutputStream(initCapacity);
toStream(in, out);
return out.toByteArray();
}
public static void toStream(InputStream in, OutputStream out) throws IOException {
try {
int count;
byte[] buf = new byte[1024];
while( (count = in.read(buf)) != -1) {
if( Thread.interrupted() ) {
throw new IOException("Thread was interrupted.");
}
out.write(buf, 0, count);
}
} finally {
out.close();
in.close();
}
}
public static void write(File file, String result) throws IOException {
FileWriter writer = new FileWriter(file);
try {
writer.write(result);
} finally {
writer.close();
}
}
public static void write(File file, byte[] bytes) throws IOException {
FileOutputStream os = new FileOutputStream(file);
try {
os.write(bytes);
} finally {
os.close();
}
}
public static List toList(File file) throws IOException {
return toList(new FileReader(file));
}
public static List toList(Reader reader) throws IOException {
try {
String line = "";
List list = new ArrayList();
BufferedReader bufferedReader = (reader instanceof BufferedReader) ? (BufferedReader)reader : new BufferedReader(reader);
while ( (line=bufferedReader.readLine()) != null) {
list.add(line);
}
return list;
} finally {
reader.close();
}
}
public static void createPath(Path path) throws IOException {
createPath(path.toFile());
}
public static void createPath(File file) throws IOException {
if (file.exists() == false && file.mkdirs() == false) {
throw new IOException("Unable to create directory: " + file.getAbsolutePath());
}
}
public static void deletePath(File file) throws IOException {
deletePath(file.toPath());
}
public static void deletePath(Path path) throws IOException {
if (path.toFile().exists() == false) {
return; // it doesn't exist.
}
Files.walkFileTree(path, new SimpleFileVisitor() {
@Override
public FileVisitResult visitFile(Path file, BasicFileAttributes attrs) throws IOException {
Files.delete(file);
return FileVisitResult.CONTINUE;
}
@Override
public FileVisitResult postVisitDirectory(Path dir, IOException exc) throws IOException {
Files.delete(dir);
return FileVisitResult.CONTINUE;
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy