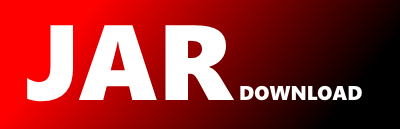
org.crazyyak.dev.common.fine.TraitMap Maven / Gradle / Ivy
/*
* Copyright 2014 Harlan Noonkester
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.crazyyak.dev.common.fine;
import org.crazyyak.dev.common.BeanUtils;
import java.io.Serializable;
import java.util.*;
public class TraitMap implements Serializable {
private static final TraitMap empty = new TraitMap();
public static TraitMap empty() {
return empty;
}
private final Map traitMap;
public TraitMap(Map, ?> givenMap) {
if (givenMap == null) {
this.traitMap = Collections.emptyMap();
return;
}
SortedMap localMap = new TreeMap<>();
for (Map.Entry entry : givenMap.entrySet()) {
if (entry.getKey() == null) {
continue;
}
String key = entry.getKey().toString().toUpperCase();
Object value = entry.getValue();
if (value == null) {
localMap.put(key, null);
} else {
localMap.put(key, value.toString());
}
}
this.traitMap = Collections.unmodifiableSortedMap(localMap);
}
public TraitMap(String... traitStrings) {
this(BeanUtils.toMap(traitStrings));
}
public TraitMap add(TraitMap traitMapArg) {
Map localMap = new HashMap<>(traitMap);
localMap.putAll(traitMapArg.getMap());
return new TraitMap(localMap);
}
public TraitMap add(Map traitMapArg) {
if (traitMapArg == null || traitMapArg.isEmpty()) {
return this;
}
Map localMap = new HashMap<>(traitMap);
localMap.putAll(traitMapArg);
return new TraitMap(localMap);
}
public TraitMap remove(Collection traits) {
if (traits == null || traits.isEmpty()) {
return this;
}
Map localMap = new HashMap<>(traitMap);
for (String trait : traits) {
localMap.remove(trait);
}
return new TraitMap(localMap);
}
public Map getMap() {
return traitMap;
}
public boolean isEmpty() {
return traitMap.isEmpty();
}
public boolean isNotEmpty() {
return !traitMap.isEmpty();
}
public int getSize() {
return traitMap.size();
}
public boolean hasTrait(String key) {
return (key != null) && traitMap.containsKey(key.toUpperCase());
}
public boolean hasValue(String key, String checkValue) {
if (key == null) return false;
String value = traitMap.get(key.toUpperCase());
if (checkValue == null) {
return value == null;
} else {
return checkValue.equals(value);
}
}
public String getValue(String key) {
return (key == null) ? null : traitMap.get(key.toUpperCase());
}
public String getText() {
StringBuilder sb = new StringBuilder();
for (Map.Entry entry : traitMap.entrySet()) {
sb.append(entry.getKey());
sb.append(":");
if (entry.getValue() == null) {
sb.append("null");
} else {
sb.append("\"");
sb.append(entry.getValue());
sb.append("\"");
}
sb.append(", ");
}
if (sb.length() > 2) {
sb.delete(sb.length() - 2, sb.length());
}
return sb.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
} else if (o == null || getClass() != o.getClass()) {
return false;
}
TraitMap that = (TraitMap) o;
return traitMap.equals(that.traitMap);
}
@Override
public int hashCode() {
return traitMap.hashCode();
}
public String toString() {
return getText();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy