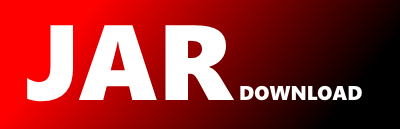
org.cristalise.kernel.entity.ItemPOA Maven / Gradle / Ivy
package org.cristalise.kernel.entity;
/**
* org/cristalise/kernel/entity/ItemPOA.java .
* Generated by the IDL-to-Java compiler (portable), version "3.2"
* from /home/vagrant/workspace/cristal-ise/idl/src/main/idl/Entity.idl
* Friday, April 10, 2020 7:30:08 PM CEST
*/
/**
* ManageableEntity is the CORBA super-interface for Entities. It is uniquely
* identifiable by its system key, and contains XML fragments arranged
* in a tree structure.
**/
public abstract class ItemPOA extends org.omg.PortableServer.Servant
implements org.cristalise.kernel.entity.ItemOperations, org.omg.CORBA.portable.InvokeHandler
{
// Constructors
private static java.util.Hashtable _methods = new java.util.Hashtable ();
static
{
_methods.put ("getSystemKey", new java.lang.Integer (0));
_methods.put ("initialise", new java.lang.Integer (1));
_methods.put ("queryData", new java.lang.Integer (2));
_methods.put ("requestAction", new java.lang.Integer (3));
_methods.put ("delegatedAction", new java.lang.Integer (4));
_methods.put ("queryLifeCycle", new java.lang.Integer (5));
}
public org.omg.CORBA.portable.OutputStream _invoke (String $method,
org.omg.CORBA.portable.InputStream in,
org.omg.CORBA.portable.ResponseHandler $rh)
{
org.omg.CORBA.portable.OutputStream out = null;
java.lang.Integer __method = (java.lang.Integer)_methods.get ($method);
if (__method == null)
throw new org.omg.CORBA.BAD_OPERATION (0, org.omg.CORBA.CompletionStatus.COMPLETED_MAYBE);
switch (__method.intValue ())
{
/**
* System generated unique key of the Entity. It is a 128 bit UUID, expressed as two 64 bit longs in the IDLs, but as a UUID object in the Java kernel. The ItemPath is used as the Item identifier in the kernel and its API,
which can be derived from either a UUID object or a SystemKey structure.
**/
case 0: // entity/Item/getSystemKey
{
org.cristalise.kernel.common.SystemKey $result = null;
$result = this.getSystemKey ();
out = $rh.createReply();
org.cristalise.kernel.common.SystemKeyHelper.write (out, $result);
break;
}
/** Initialises a new Item. Initial properties and the lifecycle are supplied. They should come from the Item's description.
*
* @param agentKey the Agent doing the initialisation
* @param itemProps The XML marshalled {@link org.cristalise.kernel.Property.PropertyArrayList PropertyArrayList} containing the initial
* Property objects of the Item
* @param workflow The XML marshalled new lifecycle of the Item
* @param collection The XML marshalled CollectionArrayList of the initial state of the Item's collections
* @param viewpoint the XML marshalled Viewpoint to be stored to find the Outcome
* @param outcome the XML data to be stored
* @exception ObjectNotFoundException
**/
case 1: // entity/Item/initialise
{
try {
org.cristalise.kernel.common.SystemKey agentKey = org.cristalise.kernel.common.SystemKeyHelper.read (in);
String itemProps = in.read_string ();
String workflow = in.read_string ();
String collections = in.read_string ();
String viewpoint = in.read_string ();
String outcome = in.read_string ();
this.initialise (agentKey, itemProps, workflow, collections, viewpoint, outcome);
out = $rh.createReply();
} catch (org.cristalise.kernel.common.AccessRightsException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.AccessRightsExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.InvalidDataException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.InvalidDataExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.PersistencyException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.PersistencyExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.ObjectNotFoundException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.ObjectNotFoundExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.InvalidCollectionModification $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.InvalidCollectionModificationHelper.write (out, $ex);
}
break;
}
/**
* Returns a chunk of XML which may be a serialized C2KLocalObject, or in the case of Outcomes is merely a fragment of XML.
*
* @param path - All Entity data is arranged in a tree structure which uniquely identifies that object within the Entity it is contained, according to the following scheme:
* LifeCycle/workflow
(Items only): The Workflow object for this Item, containing the graph of activities defining the Item's lifecycle, and the Predefined Step container for data modification
* Collection/{Name}
(Items only): Collection objects defining links between Items
* Property/{Name}
: Name value pairs to idenfity this Entity, define its type, and hold any other oft-changing indicators that would be heavy to extract from Outcomes
* AuditTrail/{ID}
(Items only): Events describing all activity state changes in this Item.
* Outcome/{Schema name}/{Schema version}/{Event ID}
(Items only): XML fragments resulting from the execution of an Activity, validated against the XML Schema defined by that activity.
* ViewPoint/{Schema name}/{Name}
(Items only): A named pointer to the latest version of an Outcome, defined by the Activity.
* Job/{ID}
(Agents only): A persistent Job, reflecting a request for execution of an Activity to this Agent. Not all roles create persistent Jobs like this, only those specifically flagged to do so.
*
* @see org.cristalise.kernel.persistency.ClusterStorage#getPath
*
* @return The XML string of the data. All fragments except Outcomes will deserialize into objects with the kernel CastorXMLUtility available in the Gateway.
*
* @exception ObjectNotFoundException when the path is not present in this Entity
* @exception AccessRightsException Not currently implemented
* @exception PersistencyException when the path could not be loaded because of a problem with the storage subsystem.
**/
case 2: // entity/Item/queryData
{
try {
String path = in.read_string ();
String $result = null;
$result = this.queryData (path);
out = $rh.createReply();
out.write_string ($result);
} catch (org.cristalise.kernel.common.AccessRightsException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.AccessRightsExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.ObjectNotFoundException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.ObjectNotFoundExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.PersistencyException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.PersistencyExceptionHelper.write (out, $ex);
}
break;
}
/**
* Requests a transition of an Activity in this Item's workflow. If possible and permitted, an Event is
* generated and stored, the Activity's state is updated, which may cause the Workflow to proceed. If
* this transition requires Outcome data, this is supplied and stored, and a Viewpoint will be created
* or updated to point to this latest version. In the case of PredefinedSteps, additional data changes
* may be performed in the server data.
*
* This method should not be called directly, as there is a large client side to activity execution
* implemented in the Proxy objects, such as script execution and schema validation.
*
* @param agentKey The SystemKey of the Agent. Some activities may be restricted in which roles may execute them.
* Some transitions cause the activity to be assigned to the executing Agent.
*
* @param stepPath The path in the Workflow to the desired Activity
*
* @param transitionID The transition to be performed
*
* @param requestData The XML Outcome of the work defined by the Activity. Must be valid to the XML Schema,
* though this is not verified on the server, rather in the AgentProxy in the Client API.
*
* @param fileName the name of the file associated with attahment
*
* @param attachment binary data associated with the Outcome (can be empty)
*
* @throws AccessRightsException The Agent is not permitted to perform the operation. Either it does not
* have the correct role, or the Activity is reserved by another Agent. Also thrown when the given Agent ID doesn't exist.
* @throws InvalidTransitionException The Activity is not in the correct state to make the requested transition.
* @throws ObjectNotFoundException The Activity or a container of it does not exist.
* @throws InvalidDataException An activity property for the requested Activity was invalid e.g. SchemaVersion was not a number.
Also thrown when an uncaught Java exception or error occurred.
* @throws PersistencyException There was a problem committing the changes to storage.
* @throws ObjectAlreadyExistsException Not normally thrown, but reserved for PredefinedSteps to throw if they need to.
**/
case 3: // entity/Item/requestAction
{
try {
org.cristalise.kernel.common.SystemKey agentKey = org.cristalise.kernel.common.SystemKeyHelper.read (in);
String stepPath = in.read_string ();
int transitionID = in.read_ulong ();
String requestData = in.read_string ();
String fileName = in.read_string ();
byte attachment[] = org.cristalise.kernel.entity.ItemPackage.OctetSequenceHelper.read (in);
String $result = null;
$result = this.requestAction (agentKey, stepPath, transitionID, requestData, fileName, attachment);
out = $rh.createReply();
out.write_string ($result);
} catch (org.cristalise.kernel.common.AccessRightsException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.AccessRightsExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.InvalidTransitionException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.InvalidTransitionExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.ObjectNotFoundException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.ObjectNotFoundExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.InvalidDataException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.InvalidDataExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.PersistencyException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.PersistencyExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.ObjectAlreadyExistsException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.ObjectAlreadyExistsExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.InvalidCollectionModification $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.InvalidCollectionModificationHelper.write (out, $ex);
}
break;
}
case 4: // entity/Item/delegatedAction
{
try {
org.cristalise.kernel.common.SystemKey agentKey = org.cristalise.kernel.common.SystemKeyHelper.read (in);
org.cristalise.kernel.common.SystemKey delegateAgentKey = org.cristalise.kernel.common.SystemKeyHelper.read (in);
String stepPath = in.read_string ();
int transitionID = in.read_ulong ();
String requestData = in.read_string ();
String fileName = in.read_string ();
byte attachment[] = org.cristalise.kernel.entity.ItemPackage.OctetSequenceHelper.read (in);
String $result = null;
$result = this.delegatedAction (agentKey, delegateAgentKey, stepPath, transitionID, requestData, fileName, attachment);
out = $rh.createReply();
out.write_string ($result);
} catch (org.cristalise.kernel.common.AccessRightsException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.AccessRightsExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.InvalidTransitionException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.InvalidTransitionExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.ObjectNotFoundException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.ObjectNotFoundExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.InvalidDataException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.InvalidDataExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.PersistencyException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.PersistencyExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.ObjectAlreadyExistsException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.ObjectAlreadyExistsExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.InvalidCollectionModification $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.InvalidCollectionModificationHelper.write (out, $ex);
}
break;
}
/**
* Returns a set of Jobs for this Agent on this Item. Each Job represents a possible transition
* of a particular Activity in the Item's lifecycle. The list may be filtered to only refer to
* currently active activities.
*
* @param agentKey The system key of the Agent requesting Jobs.
* @param filter If true, then only Activities which are currently active will be included.
* @return An XML marshalled {@link org.cristalise.kernel.entity.agent.JobArrayList JobArrayList}
* @throws AccessRightsException - when the Agent doesn't exist
* @throws ObjectNotFoundException - when the Item doesn't have a lifecycle
* @throws PersistencyException - when there was a storage or other unknown error
**/
case 5: // entity/Item/queryLifeCycle
{
try {
org.cristalise.kernel.common.SystemKey agentKey = org.cristalise.kernel.common.SystemKeyHelper.read (in);
boolean filter = in.read_boolean ();
String $result = null;
$result = this.queryLifeCycle (agentKey, filter);
out = $rh.createReply();
out.write_string ($result);
} catch (org.cristalise.kernel.common.AccessRightsException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.AccessRightsExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.ObjectNotFoundException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.ObjectNotFoundExceptionHelper.write (out, $ex);
} catch (org.cristalise.kernel.common.PersistencyException $ex) {
out = $rh.createExceptionReply ();
org.cristalise.kernel.common.PersistencyExceptionHelper.write (out, $ex);
}
break;
}
default:
throw new org.omg.CORBA.BAD_OPERATION (0, org.omg.CORBA.CompletionStatus.COMPLETED_MAYBE);
}
return out;
} // _invoke
// Type-specific CORBA::Object operations
private static String[] __ids = {
"IDL:org.cristalise.kernel/entity/Item:1.0"};
public String[] _all_interfaces (org.omg.PortableServer.POA poa, byte[] objectId)
{
return (String[])__ids.clone ();
}
public Item _this()
{
return ItemHelper.narrow(
super._this_object());
}
public Item _this(org.omg.CORBA.ORB orb)
{
return ItemHelper.narrow(
super._this_object(orb));
}
} // class ItemPOA
© 2015 - 2025 Weber Informatics LLC | Privacy Policy