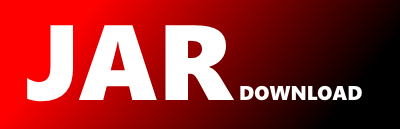
org.cristalise.lookup.ldap.LDAPLookupUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cristalise-ldap Show documentation
Show all versions of cristalise-ldap Show documentation
CRISTAL LDAP Lookup, Authentication and Property Storage Module
/**
* This file is part of the CRISTAL-iSE LDAP lookup plugin.
* Copyright (c) 2001-2016 The CRISTAL Consortium. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation; either version 3 of the License, or (at
* your option) any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; with out even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
* License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this library; if not, write to the Free Software Foundation,
* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA.
*
* http://www.fsf.org/licensing/licenses/lgpl.html
*/
/*
* Lookup helper class.
*/
package org.cristalise.lookup.ldap;
import java.security.KeyManagementException;
//import netscape.ldap.*;
//import netscape.ldap.util.*;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
import java.security.cert.X509Certificate;
import java.util.Random;
import javax.net.ssl.SSLContext;
import javax.net.ssl.SSLSocketFactory;
import javax.net.ssl.TrustManager;
import javax.net.ssl.X509TrustManager;
import org.cristalise.kernel.common.ObjectAlreadyExistsException;
import org.cristalise.kernel.common.ObjectCannotBeUpdated;
import org.cristalise.kernel.common.ObjectNotFoundException;
import org.cristalise.kernel.utils.Logger;
import com.novell.ldap.LDAPAttribute;
import com.novell.ldap.LDAPAttributeSet;
import com.novell.ldap.LDAPConnection;
import com.novell.ldap.LDAPDN;
import com.novell.ldap.LDAPEntry;
import com.novell.ldap.LDAPException;
import com.novell.ldap.LDAPJSSEStartTLSFactory;
import com.novell.ldap.LDAPModification;
import com.novell.ldap.LDAPSearchConstraints;
import com.novell.ldap.LDAPSearchResults;
import com.novell.ldap.LDAPSocketFactory;
import com.novell.ldap.util.Base64;
/**
* @version $Revision: 1.74 $ $Date: 2006/03/03 13:52:21 $
* @author $Author: abranson $
*/
final public class LDAPLookupUtils
{
static final char[] META_CHARS = {'+', '=', '"', ',', '<', '>', ';', '/'};
static final String[] META_ESCAPED = {"2B", "3D", "22", "2C", "3C", "3E", "3B", "2F"};
private static final Random RANDOM = new SecureRandom();
static public LDAPEntry getEntry(LDAPConnection ld, String dn,int dereference)
throws ObjectNotFoundException
{
try {
LDAPSearchConstraints searchCons = new LDAPSearchConstraints();
searchCons.setBatchSize(0);
searchCons.setDereference(dereference);
LDAPEntry thisEntry = ld.read(dn,searchCons);
if (thisEntry != null) return thisEntry;
} catch (LDAPException ex) {
throw new ObjectNotFoundException("LDAP Exception for dn:"+dn+": \n"+ex.getMessage());
}
throw new ObjectNotFoundException(dn+" does not exist");
}
public static String generateUserPassword(String pass) throws NoSuchAlgorithmException {
MessageDigest md = MessageDigest.getInstance("SHA");
md.reset();
String salt = generateSalt(16);
md.update((pass+salt).getBytes());
byte[] hash = md.digest();
byte[] saltBytes = salt.getBytes();
byte[] allBytes = new byte[hash.length+saltBytes.length];
System.arraycopy(hash, 0, allBytes, 0, hash.length);
System.arraycopy(saltBytes, 0, allBytes, hash.length, saltBytes.length);
StringBuffer encPassword = new StringBuffer("{SSHA}");
encPassword.append(Base64.encode(allBytes));
return encPassword.toString();
}
public static String generateSalt(int size) {
byte[] salt = new byte[size];
RANDOM.nextBytes(salt);
return String.valueOf(salt);
}
/**
* Utility method to connect to an LDAP server
* @param lp LDAP properties to connect with
* @return a novell LDAPConnection object
* @throws LDAPException when the connection was unsuccessful
*/
public static LDAPConnection createConnection(LDAPProperties lp) throws LDAPException {
LDAPConnection ld;
if (lp.mUseTLS) try {
LDAPSocketFactory lsf;
if (lp.mIgnoreCertErrors)
lsf = new LDAPJSSEStartTLSFactory(getPermissiveSocketFactory());
else
lsf = new LDAPJSSEStartTLSFactory();
LDAPConnection.setSocketFactory(lsf);
} catch (Exception ex) {
Logger.error(ex);
Logger.die("Could not enable TLS over LDAP");
}
if (lp.mTimeOut == 0) ld = new LDAPConnection();
else ld = new LDAPConnection(lp.mTimeOut);
Logger.msg(3, "LDAPLookup - connecting to " + lp.mHost);
ld.connect(lp.mHost, Integer.valueOf(lp.mPort).intValue());
if (lp.mUseTLS) try {
ld.startTLS();
} catch (Exception ex) {
Logger.error(ex);
Logger.die("Could not enable TLS over LDAP");
}
Logger.msg(3, "LDAPLookup - authenticating user:" + lp.mUser);
ld.bind( LDAPConnection.LDAP_V3, lp.mUser,
String.valueOf(lp.mPassword).getBytes());
Logger.msg(3, "LDAPLookup - authentication successful");
LDAPSearchConstraints searchCons = new LDAPSearchConstraints();
searchCons.setMaxResults(0);
ld.setConstraints(searchCons);
return ld;
}
static public SSLSocketFactory getPermissiveSocketFactory() throws NoSuchAlgorithmException, KeyManagementException {
TrustManager[] trustAllCerts = new TrustManager[]{
new X509TrustManager(){
public X509Certificate[] getAcceptedIssuers(){ return null; }
public void checkClientTrusted(X509Certificate[] certs, String authType) {}
public void checkServerTrusted(X509Certificate[] certs, String authType) {}
}
};
SSLContext sslContext = SSLContext.getInstance("TLS");
sslContext.init(null, trustAllCerts, new SecureRandom());
return sslContext.getSocketFactory();
}
//Given a DN, return an LDAP Entry
static public LDAPEntry getEntry(LDAPConnection ld, String dn)
throws ObjectNotFoundException
{
return getEntry(ld, dn, LDAPSearchConstraints.DEREF_NEVER);
}
static public String getFirstAttributeValue(LDAPEntry anEntry, String attribute) throws ObjectNotFoundException
{
LDAPAttribute attr = anEntry.getAttribute(attribute);
if (attr==null)
throw new ObjectNotFoundException("No attributes named '"+attribute+"'");
return (String)attr.getStringValues().nextElement();
}
static public String[] getAllAttributeValues(LDAPEntry anEntry, String attribute)
{
LDAPAttribute attr = anEntry.getAttribute(attribute);
if (attr!=null)
return attr.getStringValueArray();
return new String[0];
}
static public boolean existsAttributeValue(LDAPEntry anEntry, String attribute, String value)
{
LDAPAttribute attr = anEntry.getAttribute(attribute);
if (attr!=null)
{
String[] attrValues = new String[attr.size()];
attrValues = attr.getStringValueArray();
for (int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy