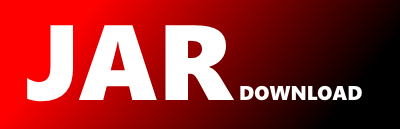
org.crsh.plugins.crowd.CrowdAuthenticationPlugin Maven / Gradle / Ivy
Go to download
This plugin allows to delegate CRaSH authentication to an Atlassian Crowd directory and to add various commands to manage the server
The newest version!
package org.crsh.plugins.crowd;
import com.atlassian.crowd.exception.ApplicationPermissionException;
import com.atlassian.crowd.exception.InvalidAuthenticationException;
import com.atlassian.crowd.integration.rest.service.factory.RestCrowdClientFactory;
import com.atlassian.crowd.service.client.ClientProperties;
import com.atlassian.crowd.service.client.ClientPropertiesImpl;
import com.atlassian.crowd.service.client.ClientResourceLocator;
import com.atlassian.crowd.service.client.CrowdClient;
import org.crsh.auth.AuthenticationPlugin;
import org.crsh.plugin.CRaSHPlugin;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* Allows to use an Atlassian Crowd serer to authenticate on CRaSH
* To use it you need to :
*
* - Define the application on the crowd server side,
* - Use
crash.auth=crowd
in your crash.properties configuration file,
* - Create the
crowd.properties
configuration file
* and add it in your application classpath or by defining its path with the system property crowd.properties (-Dcrowd.properties={FILE-PATH}/crowd.properties
).
*
*/
public class CrowdAuthenticationPlugin extends
CRaSHPlugin implements
AuthenticationPlugin {
/**
* Logger
*/
protected final Logger log = Logger.getLogger(getClass().getName());
/**
* Crowd client instance
*/
private static volatile CrowdClient crowdClient;
/**
* Lock to create the crowd client
*/
private static final Object lock = new Object();
/**
* Get a ready to use CrowdClient.
*
* @return a CrowdClient already initialized
*/
private static CrowdClient getCrowdClient() {
if (crowdClient == null) {
synchronized (lock) {
if (crowdClient == null) {
ClientResourceLocator
crl = new ClientResourceLocator("crowd.properties");
if (crl.getProperties() == null) {
throw new NullPointerException("crowd.properties can not be found in classpath");
}
ClientProperties clientProperties = ClientPropertiesImpl.newInstanceFromResourceLocator(crl);
RestCrowdClientFactory restCrowdClientFactory = new RestCrowdClientFactory();
crowdClient = restCrowdClientFactory.newInstance(clientProperties);
}
}
}
return crowdClient;
}
public Class getCredentialType() {
return String.class;
}
@Override
public String getName() {
return "crowd";
}
@Override
public boolean authenticate(String username, String password) throws Exception {
// Username and passwords are required
if (username == null || username.isEmpty() || password == null || password.isEmpty()) {
log.log(Level.WARNING, "Unable to logon without username and password.");
return false;
}
try {
// Authenticate the user
if (log.isLoggable(Level.FINE)) {
log.log(Level.FINE, "Authenticating '" + username + "' on crowd directory");
}
getCrowdClient().authenticateUser(username, password);
return true;
} catch (InvalidAuthenticationException e) {
log.log(Level.WARNING, "Authentication failed for user '" + username + "'");
return false;
} catch (ApplicationPermissionException e) {
log.log(Level.SEVERE, "Application not authorized to authenticate user '" + username + "'", e);
return false;
}
}
@Override
public AuthenticationPlugin getImplementation() {
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy