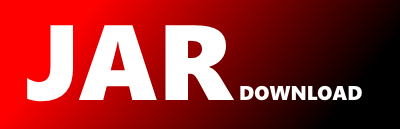
org.cryptomator.cryptolib.common.Scrypt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cryptolib Show documentation
Show all versions of cryptolib Show documentation
This library contains all cryptographic functions that are used by Cryptomator.
package org.cryptomator.cryptolib.common;
/*******************************************************************************
* Copyright (c) 2015, 2016 Sebastian Stenzel and others.
* This file is licensed under the terms of the MIT license.
* See the LICENSE.txt file for more info.
*
* Contributors:
* Sebastian Stenzel - initial API and implementation
*******************************************************************************/
import java.nio.ByteBuffer;
import java.nio.CharBuffer;
import java.nio.charset.Charset;
import java.util.Arrays;
import org.bouncycastle.crypto.generators.SCrypt;
public class Scrypt {
private static final Charset UTF_8 = Charset.forName("UTF-8");
/**
* Derives a key from the given passphrase.
* This implementation makes sure, any copies of the passphrase used during key derivation are overwritten in memory asap (before next GC cycle).
*
* @param passphrase The passphrase
* @param salt Salt, ideally randomly generated
* @param costParam Cost parameter N
, larger than 1, a power of 2 and less than 2^(128 * blockSize / 8)
* @param blockSize Block size r
* @param keyLengthInBytes Key output length dkLen
* @return Derived key
* @see RFC Draft
*/
public static byte[] scrypt(CharSequence passphrase, byte[] salt, int costParam, int blockSize, int keyLengthInBytes) {
// This is an attempt to get the password bytes without copies of the password being created in some dark places inside the JVM:
final ByteBuffer buf = UTF_8.encode(CharBuffer.wrap(passphrase));
final byte[] pw = new byte[buf.remaining()];
buf.get(pw);
try {
return SCrypt.generate(pw, salt, costParam, blockSize, 1, keyLengthInBytes);
} finally {
Arrays.fill(pw, (byte) 0); // overwrite bytes
buf.rewind(); // just resets markers
buf.put(pw); // this is where we overwrite the actual bytes
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy