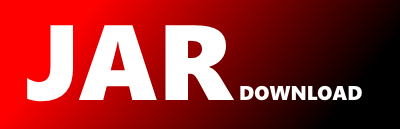
com.builder.uk.watchme.WatchMeFrame Maven / Gradle / Ivy
/**
* Pure Java JFC (Swing 1.1) application.
* This application realizes a windowing application.
*
* This file was automatically generated by
* Omnicore CodeGuide.
*/
package com.builder.uk.watchme;
/*
* #%L
* phynixx-jmx
* %%
* Copyright (C) 2014 csc
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
/**
* Frame class.
*/
public class WatchMeFrame extends JFrame implements PropertyChangeListener {
WatchMeBean watchMeBean;
JLabel count;
JLabel msg;
public WatchMeFrame() {
super("WatchMe");
this.setLayout(new GridLayout(3, 2));
count = new JLabel("");
msg = new JLabel("");
this.add(new JLabel("Count:"));
this.add(count);
this.add(new JLabel("Message:"));
this.add(msg);
JButton inc = new JButton("Increment");
this.add(inc);
// Add window listener.
this.addWindowListener
(
new WindowAdapter() {
/**
* Called when window close button was pressed.
*/
public void windowClosing(WindowEvent e) {
WatchMeFrame.this.windowClosed();
}
}
);
// Add button listener.
inc.addActionListener
(
new ActionListener() {
public void actionPerformed(ActionEvent e) {
WatchMeFrame.this.buttonPressed();
}
}
);
}
public void setWatchMeBean(WatchMeBean watchMeBean) {
this.watchMeBean = watchMeBean;
count.setText(Integer.toString(watchMeBean.getCount()));
msg.setText(watchMeBean.getMsg());
watchMeBean.addPropertyChangeListener(this);
}
protected void buttonPressed() {
if (watchMeBean != null) {
watchMeBean.incCount();
}
}
protected void windowClosed() {
System.exit(0);
}
public void propertyChange(PropertyChangeEvent evt) {
String propname = evt.getPropertyName();
if (propname.equals("msg")) {
msg.setText((String) evt.getNewValue());
} else if (propname.equals("count")) {
count.setText(((Integer) evt.getNewValue()).toString());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy