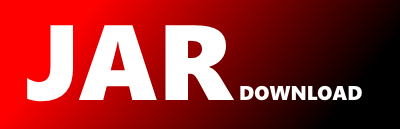
org.cthul.matchers.CthulMatchers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cthul-matchers Show documentation
Show all versions of cthul-matchers Show documentation
Provides hamcrest.org matchers for strings and exceptions,
allows matching code blocks, and provides several utilities for
combining matchers.
The newest version!
// Generated source.
package org.cthul.matchers;
public class CthulMatchers {
public static org.cthul.matchers.object.CIs is(org.hamcrest.Matcher super T> matcher) {
return org.cthul.matchers.object.CIs.is(matcher);
}
public static org.cthul.matchers.object.CIs _is(org.hamcrest.Matcher super T> matcher) {
return org.cthul.matchers.object.CIs._is(matcher);
}
public static org.cthul.matchers.object.CIs has(org.hamcrest.Matcher super T> matcher) {
return org.cthul.matchers.object.CIs.has(matcher);
}
public static org.cthul.matchers.object.CIs not(org.hamcrest.Matcher super T> matcher) {
return org.cthul.matchers.object.CIs.not(matcher);
}
public static org.cthul.matchers.object.CIs _has(org.hamcrest.Matcher super T> matcher) {
return org.cthul.matchers.object.CIs._has(matcher);
}
public static org.cthul.matchers.object.CIs _isNot(org.hamcrest.Matcher super T> matcher) {
return org.cthul.matchers.object.CIs._isNot(matcher);
}
public static org.cthul.matchers.object.CIs _not(org.hamcrest.Matcher super T> matcher) {
return org.cthul.matchers.object.CIs._not(matcher);
}
public static org.cthul.matchers.object.CIs isNot(org.hamcrest.Matcher super T> matcher) {
return org.cthul.matchers.object.CIs.isNot(matcher);
}
/**
* Can the given pattern be found in the string?
*
* @param p
* @return String-Matcher
*/
public static org.hamcrest.Matcher containsPattern(java.util.regex.Pattern p) {
return org.cthul.matchers.object.ContainsPattern.containsPattern(p);
}
/**
* Can the given pattern be found in the string?
*
* @param regex
* @return String-Matcher
*/
public static org.hamcrest.Matcher containsPattern(java.lang.String regex) {
return org.cthul.matchers.object.ContainsPattern.containsPattern(regex);
}
/**
* Does the pattern match the entire string?
*
* @param p
* @return String-Matcher
*/
public static org.hamcrest.Matcher matchesPattern(java.util.regex.Pattern p) {
return org.cthul.matchers.object.ContainsPattern.matchesPattern(p);
}
/**
* Does the pattern match the entire string?
*
* @param regex
* @return String-Matcher
*/
public static org.hamcrest.Matcher matchesPattern(java.lang.String regex) {
return org.cthul.matchers.object.ContainsPattern.matchesPattern(regex);
}
public static org.cthul.matchers.chain.AndChainMatcher.Builder instanceThat(java.lang.Class clazz, org.hamcrest.Matcher super T2>... m) {
return org.cthul.matchers.object.InstanceThat.instanceThat(clazz, m);
}
public static org.cthul.matchers.chain.AndChainMatcher.Builder instanceThat(java.lang.Class clazz, org.hamcrest.Matcher super T2> m) {
return org.cthul.matchers.object.InstanceThat.instanceThat(clazz, m);
}
public static org.cthul.matchers.chain.AndChainMatcher.Builder isInstanceThat(java.lang.Class clazz, org.hamcrest.Matcher super T2> m) {
return org.cthul.matchers.object.InstanceThat.isInstanceThat(clazz, m);
}
public static org.hamcrest.Matcher isInstanceThat(java.lang.Class clazz, org.hamcrest.Matcher super T2>... m) {
return org.cthul.matchers.object.InstanceThat.isInstanceThat(clazz, m);
}
public static org.cthul.matchers.object.InstanceOf a(java.lang.Class clazz) {
return org.cthul.matchers.object.InstanceOf.a(clazz);
}
public static org.cthul.matchers.object.InstanceOf instanceOf(java.lang.Class clazz) {
return org.cthul.matchers.object.InstanceOf.instanceOf(clazz);
}
public static org.cthul.matchers.object.InstanceOf isA(java.lang.Class clazz) {
return org.cthul.matchers.object.InstanceOf.isA(clazz);
}
public static org.cthul.matchers.object.InstanceOf _isA(java.lang.Class clazz) {
return org.cthul.matchers.object.InstanceOf._isA(clazz);
}
public static org.cthul.matchers.object.InstanceOf isInstanceOf(java.lang.Class clazz) {
return org.cthul.matchers.object.InstanceOf.isInstanceOf(clazz);
}
public static org.cthul.matchers.object.InstanceOf _instanceOf(java.lang.Class clazz) {
return org.cthul.matchers.object.InstanceOf._instanceOf(clazz);
}
public static org.hamcrest.Matcher and(org.hamcrest.Matcher super T>... matchers) {
return org.cthul.matchers.chain.AndChainMatcher.and(matchers);
}
public static org.hamcrest.Matcher and(java.util.Collection extends org.hamcrest.Matcher super T>> matchers) {
return org.cthul.matchers.chain.AndChainMatcher.and(matchers);
}
public static org.cthul.matchers.chain.AndChainMatcher.Builder all(org.hamcrest.Matcher super T> m) {
return org.cthul.matchers.chain.AndChainMatcher.all(m);
}
public static org.cthul.matchers.chain.AndChainMatcher.Builder all(org.hamcrest.Matcher super T>... m) {
return org.cthul.matchers.chain.AndChainMatcher.all(m);
}
public static org.cthul.matchers.chain.ChainFactory all() {
return org.cthul.matchers.chain.AndChainMatcher.all();
}
public static org.cthul.matchers.chain.AndChainMatcher.Builder both(org.hamcrest.Matcher super T> m) {
return org.cthul.matchers.chain.AndChainMatcher.both(m);
}
public static org.cthul.matchers.chain.AndChainMatcher.Builder both(org.hamcrest.Matcher super T>... m) {
return org.cthul.matchers.chain.AndChainMatcher.both(m);
}
public static org.cthul.matchers.chain.ChainFactory none() {
return org.cthul.matchers.chain.NOrChainMatcher.none();
}
public static org.hamcrest.Matcher none(org.hamcrest.Matcher super T>... matchers) {
return org.cthul.matchers.chain.NOrChainMatcher.none(matchers);
}
public static org.hamcrest.Matcher none(java.util.Collection extends org.hamcrest.Matcher super T>> matchers) {
return org.cthul.matchers.chain.NOrChainMatcher.none(matchers);
}
public static org.hamcrest.Matcher nor(org.hamcrest.Matcher super T>... matchers) {
return org.cthul.matchers.chain.NOrChainMatcher.nor(matchers);
}
public static org.hamcrest.Matcher nor(java.util.Collection extends org.hamcrest.Matcher super T>> matchers) {
return org.cthul.matchers.chain.NOrChainMatcher.nor(matchers);
}
public static org.cthul.matchers.chain.NOrChainMatcher.Builder neither(org.hamcrest.Matcher super T>... m) {
return org.cthul.matchers.chain.NOrChainMatcher.neither(m);
}
public static org.cthul.matchers.chain.NOrChainMatcher.Builder neither(org.hamcrest.Matcher super T> m) {
return org.cthul.matchers.chain.NOrChainMatcher.neither(m);
}
public static org.hamcrest.Matcher or(java.util.Collection extends org.hamcrest.Matcher super T>> matchers) {
return org.cthul.matchers.chain.OrChainMatcher.or(matchers);
}
public static org.hamcrest.Matcher or(org.hamcrest.Matcher super T>... matchers) {
return org.cthul.matchers.chain.OrChainMatcher.or(matchers);
}
public static org.hamcrest.Matcher any(java.util.Collection extends org.hamcrest.Matcher super T>> matchers) {
return org.cthul.matchers.chain.OrChainMatcher.any(matchers);
}
public static org.hamcrest.Matcher any(org.hamcrest.Matcher super T>... matchers) {
return org.cthul.matchers.chain.OrChainMatcher.any(matchers);
}
public static org.cthul.matchers.chain.ChainFactory any() {
return org.cthul.matchers.chain.OrChainMatcher.any();
}
public static org.cthul.matchers.chain.OrChainMatcher.Builder either(org.hamcrest.Matcher super T>... m) {
return org.cthul.matchers.chain.OrChainMatcher.either(m);
}
public static org.cthul.matchers.chain.OrChainMatcher.Builder either(org.hamcrest.Matcher super T> m) {
return org.cthul.matchers.chain.OrChainMatcher.either(m);
}
public static org.cthul.matchers.chain.SomeOfChainMatcher.SomeOfChainFactory matches(org.hamcrest.Matcher super java.lang.Integer> countMatcher) {
return org.cthul.matchers.chain.SomeOfChainMatcher.matches(countMatcher);
}
public static org.cthul.matchers.chain.SomeOfChainMatcher.SomeOfChainFactory matches(int count) {
return org.cthul.matchers.chain.SomeOfChainMatcher.matches(count);
}
public static org.cthul.matchers.chain.SomeOfChainMatcher.Builder matches(int count, org.hamcrest.Matcher super T>... matchers) {
return org.cthul.matchers.chain.SomeOfChainMatcher.matches(count, matchers);
}
public static org.cthul.matchers.chain.SomeOfChainMatcher.Builder matches(org.hamcrest.Matcher super java.lang.Integer> countMatcher, org.hamcrest.Matcher super T>... matchers) {
return org.cthul.matchers.chain.SomeOfChainMatcher.matches(countMatcher, matchers);
}
public static org.hamcrest.Matcher xor(java.util.Collection extends org.hamcrest.Matcher super T>> matchers) {
return org.cthul.matchers.chain.XOrChainMatcher.xor(matchers);
}
public static org.hamcrest.Matcher xor(org.hamcrest.Matcher super T>... matchers) {
return org.cthul.matchers.chain.XOrChainMatcher.xor(matchers);
}
public static org.cthul.matchers.exceptions.CausedBy directlyCausedBy(org.hamcrest.Matcher super java.lang.Throwable> matcher) {
return org.cthul.matchers.exceptions.CausedBy.directlyCausedBy(matcher);
}
public static org.cthul.matchers.exceptions.CausedBy directlyCausedBy(java.lang.Class extends java.lang.Throwable> clazz) {
return org.cthul.matchers.exceptions.CausedBy.directlyCausedBy(clazz);
}
public static org.cthul.matchers.exceptions.CausedBy directlyCausedBy(java.lang.Class extends java.lang.Throwable> clazz, java.lang.String message) {
return org.cthul.matchers.exceptions.CausedBy.directlyCausedBy(clazz, message);
}
public static org.cthul.matchers.exceptions.CausedBy directlyCausedBy(java.lang.Class extends java.lang.Throwable> clazz, org.hamcrest.Matcher super java.lang.Throwable> matcher) {
return org.cthul.matchers.exceptions.CausedBy.directlyCausedBy(clazz, matcher);
}
public static org.cthul.matchers.exceptions.CausedBy directlyCausedBy(java.lang.Class extends java.lang.Throwable> clazz, java.lang.String message, org.hamcrest.Matcher super java.lang.Throwable> matcher) {
return org.cthul.matchers.exceptions.CausedBy.directlyCausedBy(clazz, message, matcher);
}
public static org.cthul.matchers.exceptions.CausedBy causedBy(java.lang.Class extends java.lang.Throwable> clazz, org.hamcrest.Matcher super java.lang.Throwable> matcher) {
return org.cthul.matchers.exceptions.CausedBy.causedBy(clazz, matcher);
}
public static org.cthul.matchers.exceptions.CausedBy causedBy(java.lang.Class extends java.lang.Throwable> clazz, java.lang.String message) {
return org.cthul.matchers.exceptions.CausedBy.causedBy(clazz, message);
}
public static org.cthul.matchers.exceptions.CausedBy causedBy(java.lang.Class extends java.lang.Throwable> clazz, java.lang.String message, org.hamcrest.Matcher super java.lang.Throwable> matcher) {
return org.cthul.matchers.exceptions.CausedBy.causedBy(clazz, message, matcher);
}
public static org.cthul.matchers.exceptions.CausedBy causedBy(java.lang.Class extends java.lang.Throwable> clazz) {
return org.cthul.matchers.exceptions.CausedBy.causedBy(clazz);
}
public static org.cthul.matchers.exceptions.CausedBy causedBy(java.lang.String message) {
return org.cthul.matchers.exceptions.CausedBy.causedBy(message);
}
public static org.cthul.matchers.exceptions.CausedBy causedBy(org.hamcrest.Matcher super java.lang.Throwable> matcher) {
return org.cthul.matchers.exceptions.CausedBy.causedBy(matcher);
}
public static org.cthul.matchers.exceptions.ExceptionMessage message(org.hamcrest.Matcher super java.lang.String> messageMatcher) {
return org.cthul.matchers.exceptions.ExceptionMessage.message(messageMatcher);
}
public static org.cthul.matchers.exceptions.ExceptionMessage messageIs(java.lang.String message) {
return org.cthul.matchers.exceptions.ExceptionMessage.messageIs(message);
}
public static org.cthul.matchers.exceptions.ExceptionMessage messageContains(java.lang.String regex) {
return org.cthul.matchers.exceptions.ExceptionMessage.messageContains(regex);
}
public static org.cthul.matchers.exceptions.ExceptionMessage messageContains(java.util.regex.Pattern pattern) {
return org.cthul.matchers.exceptions.ExceptionMessage.messageContains(pattern);
}
public static org.cthul.matchers.exceptions.ExceptionMessage messageMatches(java.util.regex.Pattern pattern) {
return org.cthul.matchers.exceptions.ExceptionMessage.messageMatches(pattern);
}
public static org.cthul.matchers.exceptions.ExceptionMessage messageMatches(java.lang.String regex) {
return org.cthul.matchers.exceptions.ExceptionMessage.messageMatches(regex);
}
public static org.hamcrest.Matcher exception(java.lang.String message) {
return org.cthul.matchers.exceptions.IsThrowable.exception(message);
}
public static org.cthul.matchers.object.InstanceOf exception(java.lang.Class clazz) {
return org.cthul.matchers.exceptions.IsThrowable.exception(clazz);
}
public static org.cthul.matchers.object.InstanceOf exception() {
return org.cthul.matchers.exceptions.IsThrowable.exception();
}
public static org.hamcrest.Matcher exception(java.lang.Class clazz, java.lang.String message, org.hamcrest.Matcher super T> matcher) {
return org.cthul.matchers.exceptions.IsThrowable.exception(clazz, message, matcher);
}
public static org.hamcrest.Matcher exception(org.hamcrest.Matcher super java.lang.Exception> matcher) {
return org.cthul.matchers.exceptions.IsThrowable.exception(matcher);
}
public static org.hamcrest.Matcher exception(java.lang.Class extends java.lang.Exception> clazz, java.lang.String message) {
return org.cthul.matchers.exceptions.IsThrowable.exception(clazz, message);
}
public static org.hamcrest.Matcher exception(java.lang.Class clazz, org.hamcrest.Matcher super T> matcher) {
return org.cthul.matchers.exceptions.IsThrowable.exception(clazz, matcher);
}
public static org.hamcrest.Matcher throwable(java.lang.String message) {
return org.cthul.matchers.exceptions.IsThrowable.throwable(message);
}
public static org.cthul.matchers.object.InstanceOf throwable(java.lang.Class clazz) {
return org.cthul.matchers.exceptions.IsThrowable.throwable(clazz);
}
public static org.cthul.matchers.object.InstanceOf throwable() {
return org.cthul.matchers.exceptions.IsThrowable.throwable();
}
public static org.hamcrest.Matcher throwable(java.lang.Class clazz, java.lang.String message, org.hamcrest.Matcher super T> matcher) {
return org.cthul.matchers.exceptions.IsThrowable.throwable(clazz, message, matcher);
}
public static org.hamcrest.Matcher throwable(java.lang.Class extends java.lang.Throwable> clazz, java.lang.String message) {
return org.cthul.matchers.exceptions.IsThrowable.throwable(clazz, message);
}
public static org.hamcrest.Matcher throwable(org.hamcrest.Matcher super java.lang.Throwable> matcher) {
return org.cthul.matchers.exceptions.IsThrowable.throwable(matcher);
}
public static org.hamcrest.Matcher throwable(java.lang.Class clazz, org.hamcrest.Matcher super T> matcher) {
return org.cthul.matchers.exceptions.IsThrowable.throwable(clazz, matcher);
}
/**
* Does the proc raise a throwable that satisfies the condition?
*
* @param clazz
* @param matcher
* @return Proc-Matcher
*/
public static org.hamcrest.Matcher raises(java.lang.Class extends java.lang.Throwable> clazz, org.hamcrest.Matcher super java.lang.Throwable> matcher) {
return org.cthul.matchers.proc.Raises.raises(clazz, matcher);
}
/**
* Does the proc raise a throwable that satisfies the condition?
*
* @param regex
* @return Proc-Matcher
*/
public static org.hamcrest.Matcher raises(java.lang.String regex) {
return org.cthul.matchers.proc.Raises.raises(regex);
}
/**
* Does the proc raise a throwable that satisfies the condition?
*
* @param clazz
* @return Proc-Matcher
*/
public static org.hamcrest.Matcher raises(java.lang.Class extends java.lang.Throwable> clazz) {
return org.cthul.matchers.proc.Raises.raises(clazz);
}
/**
* Does the proc raise a throwable that satisfies the condition?
*
* @param clazz
* @param regex
* @return Proc-Matcher
*/
public static org.hamcrest.Matcher raises(java.lang.Class extends java.lang.Throwable> clazz, java.lang.String regex) {
return org.cthul.matchers.proc.Raises.raises(clazz, regex);
}
/**
* Does the proc raise a throwable that satisfies the condition?
*
* @param throwableMatcher
* @return Proc-Matcher
*/
public static org.hamcrest.Matcher raises(org.hamcrest.Matcher super java.lang.Throwable> throwableMatcher) {
return org.cthul.matchers.proc.Raises.raises(throwableMatcher);
}
/**
* Does the proc raise an exception that satisfies the condition?
*
* @param clazz
* @return Proc-Matcher
*/
public static org.hamcrest.Matcher raisesException(java.lang.Class extends java.lang.Exception> clazz) {
return org.cthul.matchers.proc.Raises.raisesException(clazz);
}
/**
* Does the proc raise an exception that satisfies the condition?
*
* @param regex
* @return Proc-Matcher
*/
public static org.hamcrest.Matcher raisesException(java.lang.String regex) {
return org.cthul.matchers.proc.Raises.raisesException(regex);
}
/**
* Does the proc raise an exception that satisfies the condition?
*
* @param clazz
* @param regex
* @return Proc-Matcher
*/
public static org.hamcrest.Matcher raisesException(java.lang.Class extends java.lang.Exception> clazz, java.lang.String regex) {
return org.cthul.matchers.proc.Raises.raisesException(clazz, regex);
}
/**
* Does the proc raise an exception that satisfies the condition?
*
* @param matcher
* @return Proc-Matcher
*/
public static org.hamcrest.Matcher raisesException(org.hamcrest.Matcher super java.lang.Exception> matcher) {
return org.cthul.matchers.proc.Raises.raisesException(matcher);
}
/**
* Does the proc throw an exception?
*
* @return Proc-Matcher
*/
public static org.hamcrest.Matcher raisesException() {
return org.cthul.matchers.proc.Raises.raisesException();
}
/**
* Does the proc return a value equal to {@code value}?
*
* @param value
* @return Proc-Matcher
*/
public static org.hamcrest.Matcher result(java.lang.Object value) {
return org.cthul.matchers.proc.Returns.result(value);
}
/**
* Does the proc return a value that satisfies the condition?
*
* @param resultMatcher
* @return Proc-Matcher
*/
public static org.hamcrest.Matcher result(org.hamcrest.Matcher> resultMatcher) {
return org.cthul.matchers.proc.Returns.result(resultMatcher);
}
/**
* Does the proc return a value that satisfies the condition?
*
* @param resultMatcher
* @return Proc-Matcher
*/
public static org.hamcrest.Matcher returns(org.hamcrest.Matcher> resultMatcher) {
return org.cthul.matchers.proc.Returns.returns(resultMatcher);
}
/**
* Does the proc complete without throwing an exception?
*
* @return Proc-Matcher
*/
public static org.hamcrest.Matcher returns() {
return org.cthul.matchers.proc.Returns.returns();
}
/**
* Does the proc return a value equal to {@code value}?
*
* @param value
* @return Proc-Matcher
*/
public static org.hamcrest.Matcher returns(java.lang.Object value) {
return org.cthul.matchers.proc.Returns.returns(value);
}
/**
* Does the proc complete without throwing an exception?
*
* @return Proc-Matcher
*/
public static org.hamcrest.Matcher hasResult() {
return org.cthul.matchers.proc.Returns.hasResult();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy